sql示例_操作员之间SQL概述和示例
sql示例
We extract data from SQL Server tables along with various conditions. Usually, we have data in large amounts and SQL Between operator helps to extract a specific range of data from this huge data. For example, suppose we want to know the product sales between Jan 2019 to May 2019. In this case, we can use this operator in a SQL query.
我们从SQL Server表中提取数据以及各种条件。 通常,我们有大量数据, SQL Between运算符可帮助从大量数据中提取特定范围的数据。 例如,假设我们想知道2019年1月至2019年5月之间的产品销售。在这种情况下,我们可以在SQL查询中使用此运算符。
In this article, we will explore the SQL Between operator and its usage scenarios.
在本文中,我们将探讨SQL Between运算符及其使用方案。
SQL Between运算符的语法 (The Syntax of SQL Between operator)
We use SQL Between operator in the Where clause for selecting a range of values. The syntax for SQL Between is as follows
我们在Where子句中使用SQL Between运算符来选择值的范围。 SQL Between的语法如下
SELECT Column_name
FROMtable
WHERE
test_expression
BETWEEN min_value(expression) AND max_value;
- Test_Expression: It is the expression or column on which we need to define a range Test_Expression:这是我们需要在其上定义范围的表达式或列
- Min_value(expression): We define a minimum range for the between operator. Its data type should be the same as of test_expression Min_value(expression):我们为between运算符定义了一个最小范围。 其数据类型应与test_expression相同
- Max_value)expression): It is the maximum range for the between operator. It should also be the same data type as of min_value(expression) and test_expression Max_value)表达式):这是between运算符的最大范围。 它也应该与min_value(expression)和test_expression的数据类型相同
In my example, product sales between Jan 2019 to May 2019, we have min_value as Jan 2019 and max_value as May 2019.
在我的示例中,产品销售在2019年1月到2019年5月之间,我们将min_value作为2019年1月,并将max_value作为2019年5月。
The SQL Between operator returns TRUE if the Test_expression value is greater than or equal to the value of min_value(expression) and less than or equal to the value of max_value ( expression).
如果Test_expression值大于或等于min_value(expression)的值且小于或等于max_value(expression)的值,则SQL Between运算符将返回TRUE。
If the condition is not satisfied, it returns FALSE.
如果不满足条件,则返回FALSE。
Usually, developers confuse on whether the result will be inclusive or exclusive of the specified range. For example, does the result set include (in product sales between Jan 2019 to May 2019) both Jan 2019 and May 2019 sales data and exclude Jan 2019 and May 2019. We will look at this with the next section of examples.
通常,开发人员会混淆结果是包含指定范围还是包含指定范围。 例如,结果集是否同时包含了2019年1月和2019年5月的销售数据(在2019年1月至2019年5月之间的产品销售中),而排除了2019年1月和2019年5月的数据。
Let’s prepare a sample table and insert data into it.
让我们准备一个示例表并将数据插入其中。
I am using ApexSQL Generate as shown in the following screenshot. You can specify a custom date range to select appropriate data.
我正在使用ApexSQL Generate ,如以下屏幕截图所示。 您可以指定自定义日期范围以选择适当的数据。

Once we generate the date, you can check the sample data in the table.
生成日期后,您可以检查表格中的样本数据。

示例1:带有数值SQL Between运算符 (Example 1: SQL Between operator with Numeric values)
We can specify numeric values in the SQL Between operator. Suppose we want to get data from Productdata table for ProductID between 101 and 105.
我们可以在“ SQL Between”运算符中指定数值。 假设我们要从Productdata表中获取产品ID在101和105之间的数据。
Execute the following query to get the data. You can specify numeric value directly in between operator without any single quote.
执行以下查询以获取数据。 您可以直接在运算符之间指定数值,而无需任何单引号。
SELECT *
FROM productdata
WHERE ProductID BETWEEN 101 AND 105;
Previously, we thought of a question – whether the between operator contains inclusive or exclusive values. In the output, we can see that both ProductID 101 and 105 are included in the output of SQL Between.
以前,我们想到了一个问题–中间运算符包含的是包含值还是排他性值。 在输出中,我们可以看到在SQL Between之间的输出中同时包含了ProductID 101和105。
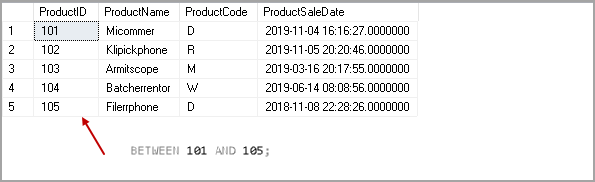
示例2:带有日期范围SQL之间的运算符 (Example 2: SQL Between operator with Date Range)
We can use SQL Between operator to get data for a specific date range. For example, in the following query, we want to get data from ProductSaleDate table in the range of 1st Jan 2019 and 1st April 2019. You need to specify the dates in a single quote.
我们可以使用SQL Between操作符来获取特定日期范围内的数据。 例如,在以下查询中,我们希望从ProductSaleDate表中获取2019年1月1日至2019年4月1日范围内的数据。您需要在单引号中指定日期。
SELECT *
FROM productdata
WHERE ProductSaleDate BETWEEN '2019-01-01' AND '2019-04-01'
ORDER BY ProductSaleDate;

We can use the CAST function to convert the value in the desired date format explicitly. For example, in the following query, we use the CAST function to convert a string into a date data type.
我们可以使用CAST函数将值明确转换为所需的日期格式。 例如,在以下查询中,我们使用CAST函数将字符串转换为日期数据类型。
SELECT *
FROM productdata
WHERE ProductSaleDate BETWEEN CAST('20190101' as date) AND CAST('20190401' as date)
ORDER BY ProductSaleDate;
Similarly, we can use the CAST function to convert a string into a datetime2 data type.
同样,我们可以使用CAST函数将字符串转换为datetime2数据类型。
SELECT *
FROM productdata
WHERE ProductSaleDate BETWEEN CAST('20190101' as datetime2) AND CAST('20190401' as datetime2)
ORDER BY ProductSaleDate;
In our sample data, productsaledate contains date along with the timestamp. In the SQL between the operator, we didn’t specify timestamp to get the required data. If you do not specify any timestamp, SQL automatically specifies timestamp as midnight. We need to b careful in using SQL Between with date columns. Let’s explore these using a further example.
在我们的样本数据中,productsaledate包含日期以及时间戳。 在运算符之间SQL中,我们没有指定时间戳来获取所需的数据。 如果未指定任何时间戳,SQL会自动将时间戳指定为午夜。 我们需要谨慎使用带有日期列SQL Between。 让我们使用另一个示例来探索这些。
Let’s create a table variable and insert few records in it. In the insert statement, you can see we are using timestamp for a few users while few users have the only date.
让我们创建一个表变量,并在其中插入一些记录。 在insert语句中,您可以看到我们为几个用户使用时间戳,而只有几个用户具有唯一的日期。
DECLARE @Users TABLE(Name varchar(40),ModifyDate DateTime)
INSERT INTO @Users (Name, ModifyDate) VALUES ('Raj', '2019-02-01');
INSERT INTO @Users (Name, ModifyDate) VALUES ('Raju', '2019-02-01 09:32:42');
INSERT INTO @Users (Name, ModifyDate) VALUES ('Rajendra', '2019-02-01 15:04:09');
INSERT INTO @Users (Name, ModifyDate) VALUES ('Akshita', '2019-02-03');
INSERT INTO @Users (Name, ModifyDate) VALUES ('Kashish', '2019-02-03 21:42:43');
In the records, you can see SQL Server added timestamp automatically in case we do not specify timestamp explicitly.
在记录中,您可以看到SQL Server自动添加了时间戳,以防万一我们没有明确指定时间戳。
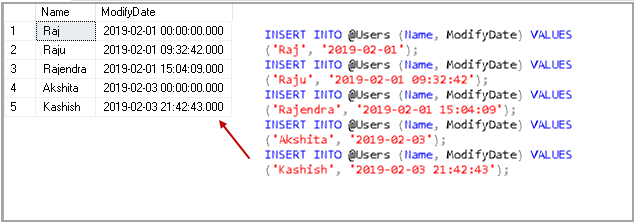
This scenario is also applicable once we retrieve the records using SQL Between operator.
一旦我们使用SQL Between运算符检索记录,此方案也适用。
Let’s select the data from the table variable using the following query.
让我们使用以下查询从表变量中选择数据。
select * from @Users
WHERE ModifyDate BETWEEN '2019-02-01' AND '2019-02-03'
ORDER BY ModifyDate
We should get all the records in the table but let’s view the output.
我们应该获取表中的所有记录,但是让我们查看输出。

In the output, we do not have a record for user Kashish. As stated earlier, if we do not specify timestamp in the SQL Between operator, SQL Server automatically uses timestamp until midnight. Due to this reason, we get the output until 2019-02-03 00:00:00.000.
在输出中,我们没有用户Kashish的记录。 如前所述,如果我们未在“ SQL Between”运算符中指定时间戳,则SQL Server会自动使用时间戳,直到午夜。 由于这个原因,我们得到的输出直到2019-02-03 00:00:00.000。
For User Kashish modifieddate is 2019-02-03 21:42:43 and it does not fulfill condition in SQL Between operator. Due to this, we do not get this user in the output.
对于用户Kashish,修改日期为2019-02-03 21:42:43,它不满足SQL Between运算符中的条件。 因此,我们不会在输出中获得该用户。
Let’s execute the command again with a timestamp in SQL Between operator.
让我们使用SQL Between运算符中的时间戳再次执行命令。
select * from @Users
WHERE ModifyDate BETWEEN '2019-02-01' AND '2019-02-03 22:00:00'
ORDER BY ModifyDate
We get all records this time because it checks for data until 2019-02-03 22:00:000.
我们这次获得所有记录,因为它会检查直到2019-02-03 22:00:000的数据。

示例3:带字符串SQL Between运算符 (Example 3: SQL Between operator with a string)
In this example, we want to get data from Productdata table having ProductCode between A and D. We also need to use a single quote for a string to get inclusive data.
在此示例中,我们要从ProductData表中获取ProductCode在A和D之间的数据。我们还需要对字符串使用单引号来获取包含数据。
SELECT *
FROM productdata
WHERE ProductCode BETWEEN 'A' AND 'C'
ORDER BY ProductCode;
In the output, you can see data for productcode between A and C.
在输出中,您可以看到A和C之间的产品代码数据。

示例4:带字符串SQL NOT Between操作符之间 (Example 4: SQL NOT Between operator with a string)
We might also want to exclude a particular range of data in the output. In this case, we can use SQL Between operator with Not statement.
我们可能还想在输出中排除特定范围的数据。 在这种情况下,我们可以将SQL Between运算符与Not语句一起使用。
Suppose, we want to get the top 10 records from the ProductData table but do not want to include ProductID 104 and 108. We can specify this condition using Not Between operator.
假设我们想从ProductData表中获取前10条记录,但不想包含ProductID 104和108。我们可以使用Not Between运算符指定此条件。
SELECT top 10 *
FROM productdata
WHERE ProductID Not BETWEEN 104 and 108
ORDER BY ProductID;
In the output, we do not have data from ProductID 104 and 108.
在输出中,我们没有来自ProductID 104和108的数据。

结论 (Conclusion)
In this article, we explored SQL Between operator along with its use cases. We should be familiar with the function to get a specific range of data. If you have any comments or questions, feel free to leave them in the comments below.
在本文中,我们探讨了SQL Between运算符及其用例。 我们应该熟悉获取特定范围数据的功能。 如果您有任何意见或疑问,请随时将其留在下面的评论中。
翻译自: https://www.sqlshack.com/sql-between-operator/
sql示例
sql示例_操作员之间SQL概述和示例相关推荐
- sql limit 子句_SQL按子句概述和示例
sql limit 子句 This article will cover the SQL ORDER BY clause including syntax, usage scenarios to so ...
- sql示例_SQL Server Lead功能概述和示例
sql示例 This article explores the SQL Server Lead function and its usage with various examples. 本文通过各种 ...
- sql as关键字_SQL AS关键字概述和示例
sql as关键字 SQL AS keyword is used to give an alias to table or column names in the queries. In this w ...
- java驱动pl sql优点_用PL/SQL和Java开发Oracle8i应用程序
用PL/SQL和Java开发Oracle8 i应用程序 随着Oracle8i的发布,Oracle 在数据库里支持了二种主要的编程语言??PL/SQL和Java.今天,Oracle的许多客户既使用PL/ ...
- @sql 单元测试_如何在SQL单元测试中使用假表?
@sql 单元测试 In this article on SQL unit testing, we will talk about how to isolate SQL unit tests from ...
- sql面试题sql语句_第二轮SQL面试问题
sql面试题sql语句 In this article, we will talk about SQL interview questions and answers that can be aske ...
- pl/sql 测试函数_如何在SQL单元测试中使用伪函数?
pl/sql 测试函数 In this article series, we are exploring SQL unit testing, in general, and also we are r ...
- python sql脚本_使用Python SQL脚本进行数据采样
python sql脚本 介绍 (Introduction) The Python programming language is object oriented, easy to use and, ...
- sql简介香气和sql简介_香气和SQL简介
sql简介香气和sql简介 在你开始前 关于本系列 本教程系列讲授从基本到高级SQL和基本的XQuery主题,并展示如何通过使用SQL查询或XQueries将常见的业务问题表达为数据库查询. 开发人员 ...
最新文章
- Trie树详解及其应用
- 12月21日云栖精选夜读:阿里云总裁胡晓明:AI泡沫过后,下一站是“产业AI”...
- python用pywin32库来隐藏windows文件
- android 6.0 sd卡读写权限,Android 6.0 读写SD卡权限问题
- 一份关于jvm内存调优及原理的学习笔记
- 你还不知“dubbo”是个什么东西吗???
- hibernate分页中跳转到第几页的功能
- android中的饱和机制,Android事件分发机制收藏这一篇就够了,通用流行框架大全...
- 算法的基本控制结构之选择结构
- SPLUNK 安装配置及常用语法
- FGSM对抗样本算法实现
- Java Web应用程序开发-深入体验Java Web开发内幕之初步
- opencv图像处理学习(五十七)——峰值信噪比和结构相似性
- 如何用html和css制作网页,html和css如何实现制作一个网页
- 计算机无法显示大容量,Win7系统电脑提示“usb大容量存储设备 代码10”的解决方法...
- 彻底卸载secureCRT,并重装,包括绿色版SecureCRT删除干净
- 《Spring实战3》第七章 使用Spring MVC构建Web应用程序
- 如何科学评估疫情对业务的影响?
- 菠萝V1一经问世将会掀起怎样的惊涛骇浪?
- 2020数学建模国赛(B)穿越沙漠
热门文章
- 读取无线手柄数据_全透外形,优秀手感,双平台通吃:倍思Switch无线手柄
- HTML网页设计综合题,网页设计(Html5)试题C卷
- lombok构造方法_最佳实践Lombok
- java recoed replay_easymock教程-record-replay-verify模型
- 删除ubuntu旧内核
- Go语言学习笔记——Go语言数据类型
- PostgresException: 42883: function ifnull(integer, integer) does not exist
- Java回顾之JDBC
- 分享开发HTML5手机游戏的5个注意要点
- 计算机网络学习笔记(28. Email消息格式与POP协议)