sql as关键字_SQL AS关键字概述和示例
sql as关键字
SQL AS keyword is used to give an alias to table or column names in the queries. In this way, we can increase the readability and understandability of the query and column headings in the result set.
SQL AS关键字用于为查询中的表名或列名赋予别名。 这样,我们可以提高结果集中查询和列标题的可读性和可理解性。
介绍 (Introduction)
If we aim to write more readable queries, using the short and concise aliases for the tables and columns will be very helpful in this objective. Some queries can be very complex and can contain a lot of joins, besides that incomprehensible table and column names make this issue more complicated and inconvenient. At this point, for such queries, code readability and understandability provide a noticeable benefit to make changes quickly by different developers. Otherwise, working with SQL queries that contain long and complex columns and table names can lead to consuming more energy.
如果我们打算编写更具可读性的查询,则在表和列中使用简短的别名将对实现此目标非常有帮助。 一些查询可能非常复杂,并且可能包含许多联接,此外,令人费解的表和列名称使此问题更加复杂和不便。 在这一点上,对于此类查询,代码的可读性和可理解性为不同开发人员快速进行更改提供了明显的好处。 否则,使用包含长而复杂的列和表名SQL查询可能会消耗更多的精力。
Shortly, SQL AS keyword, in other words, using aliases for the column names generates a temporary name for the column headings and are shown in the result sets of the queries. This concept helps to generate more meaningful and clear column headings.
简短地说,SQL AS关键字,换句话说,为列名使用别名会为列标题生成一个临时名称,并显示在查询的结果集中。 此概念有助于生成更有意义和更清晰的列标题。
Syntax:
句法:
SELECT column_name_1 AS alias_name_1, column_name_2 AS alias_name_2, column_name_N AS alias_name_n
FROM table_name;
Using aliases for the tables can be very useful when a query involved a table more than once.
当查询多次涉及一个表时,为表使用别名可能非常有用。
Syntax:
句法:
SELECT column_name_1,column_name_2,column_name_N
FROM table_name AS table_alias;
or
SELECT column_name_1 AS alias_name_1, column_name_2 AS alias_name_2, column_name_N AS alias_name_n
FROM table_name AS table_alias;
Especially, this usage type is the best practice for the queries that involve join clauses. Now we will make some example in light of this information.
特别是,这种使用类型是涉及连接子句的查询的最佳实践。 现在,我们将根据此信息做出一些示例。
准备数据 (Preparing the data)
Through the following query, we will generate two tables and we will populate some data. Also, you can practice this article’s examples in the SQL Fiddle easily. SQL Fiddle is a free tool that allows us to practice and test the SQL queries online without requiring any database and installation.
通过以下查询,我们将生成两个表,并将填充一些数据。 另外,您可以在SQL Fiddle中轻松地练习本文的示例。 SQL Fiddle是一个免费工具,可让我们在线进行实践和测试SQL查询,而无需任何数据库和安装。
CREATE TABLE Customer_InformationListForSale
(ID INTPRIMARY KEY, CustomerInfList_FirstName VARCHAR(100), CustomerInf_LastName VARCHAR(100), CustomerInf_Age INT, CustomerInf_Country VARCHAR(100)
);
CREATE TABLE OrderTransaction_InformationListForSale
(ID INTPRIMARY KEY, OrderTransaction_Date DATETIME, Customer_InformationListForSale_CustID INT, Amout FLOAT, FOREIGN KEY(Customer_InformationListForSale_CustID) REFERENCES Customer_InformationListForSale(ID)
);
INSERT INTO [Customer_InformationListForSale]
([ID], [CustomerInfList_FirstName], [CustomerInf_LastName], [CustomerInf_Age], [CustomerInf_Country]
)
VALUES
(1, N'Lawrence', N'Williams', 23, N'Indonesia'
);
INSERT INTO [Customer_InformationListForSale]
([ID], [CustomerInfList_FirstName], [CustomerInf_LastName], [CustomerInf_Age], [CustomerInf_Country]
)
VALUES
(2, N'Salvador', N'Smith', 56, N'U.S.A.'
);
INSERT INTO [Customer_InformationListForSale]
([ID], [CustomerInfList_FirstName], [CustomerInf_LastName], [CustomerInf_Age], [CustomerInf_Country]
)
VALUES
(3, N'Ernest', N'Jones', 43, N'China '
);
INSERT INTO [Customer_InformationListForSale]
([ID], [CustomerInfList_FirstName], [CustomerInf_LastName], [CustomerInf_Age], [CustomerInf_Country]
)
VALUES
(4, N'Gilbert', N'Johnson', 56, N'Brazil '
);
INSERT INTO [Customer_InformationListForSale]
([ID], [CustomerInfList_FirstName], [CustomerInf_LastName], [CustomerInf_Age], [CustomerInf_Country]
)
VALUES
(5, N'Jorge', N'Brown', 56, N'India'
);INSERT INTO [OrderTransaction_InformationListForSale]
([ID], [OrderTransaction_Date], [Customer_InformationListForSale_CustID], [Amout]
)
VALUES
(1, CAST('13-Oct-1784' AS DATETIME), 1,
1903.12
);
INSERT INTO [OrderTransaction_InformationListForSale]
([ID], [OrderTransaction_Date], [Customer_InformationListForSale_CustID], [Amout]
)
VALUES
(2, CAST('15-May-1799' AS DATETIME), 2,
345690.12
);
INSERT INTO [OrderTransaction_InformationListForSale]
([ID], [OrderTransaction_Date], [Customer_InformationListForSale_CustID], [Amout]
)
VALUES
(3, CAST('13-Jun-1842' AS DATETIME), 3,
123.90
);
INSERT INTO [OrderTransaction_InformationListForSale]
([ID], [OrderTransaction_Date], [Customer_InformationListForSale_CustID], [Amout]
)
VALUES
(4, CAST('11-Dec-1880' AS DATETIME), 4,
8765
);
INSERT INTO [OrderTransaction_InformationListForSale]
([ID], [OrderTransaction_Date], [Customer_InformationListForSale_CustID], [Amout]
)
VALUES
(5, CAST('11-Nov-1828' AS DATETIME), 5,
17893.123
);
使用SQL AS关键字为列赋予别名 (Giving aliases to columns using the SQL AS keyword)
As mentioned, we can give an alias to the column names to make them more understandable and readable, also this alias does not affect the original column name and it is only valid until the execution of the query. In the following query, we will give FirstName alias to CustomerInfList_FirstName and LastName alias to CustomerInf_LastName.
如前所述,我们可以为列名提供别名,以使其更易于理解和阅读,并且该别名不会影响原始列名,并且仅在执行查询之前有效。 在以下查询中,我们将FirstName别名命名为CustomerInfList_FirstName ,将LastName别名命名为CustomerInf_LastName 。
SELECT CustomerInfList_FirstName
AS FirstName, CustomerInf_LastName
AS LastName
FROM Customer_InformationListForSale


Tip:
小费:
In this tip, we will demonstrate the previous example on SQL Fiddle. At first, we will open the link and the data preparing query will appear in the Schema Panel. We will click the Build Schema in order to create the table and populate the data.
在本技巧中,我们将在SQL Fiddle上演示前面的示例。 首先,我们将打开链接 ,数据准备查询将出现在“模式面板”中。 我们将单击“构建模式”以创建表并填充数据。

After building the tables, we will see “Schema Ready” notification under the Schema Panel. As the last step, we will paste the example query and click the Run SQL button. So, we can execute the query and the result set will appear under the schema and the query panel.
建立表格后,我们将在“架构面板”下看到“架构就绪”通知。 作为最后一步,我们将粘贴示例查询,然后单击“运行SQL”按钮。 因此,我们可以执行查询,结果集将显示在架构和查询面板下。
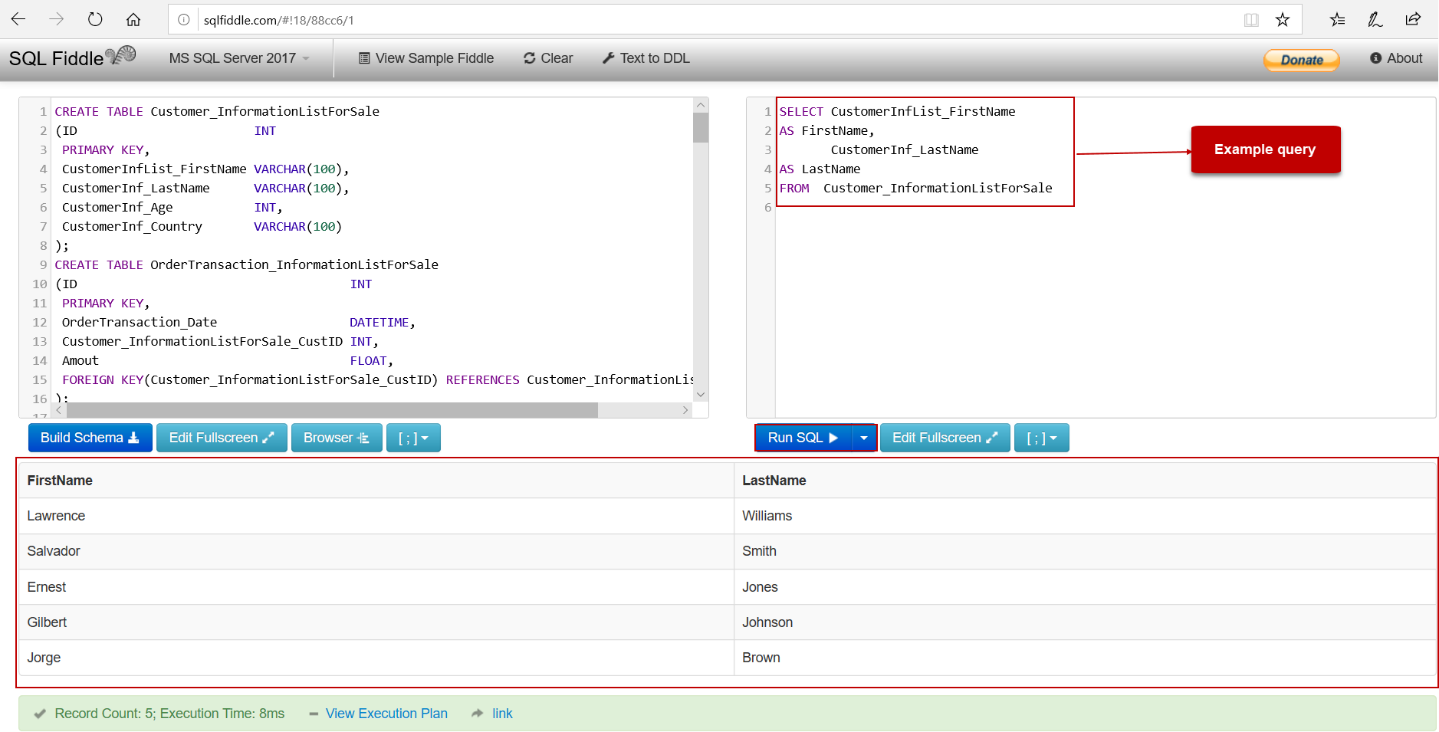
After creating the test tables, we can paste the queries and execute them with the help of the Run SQL button. The result set will appear under page.
创建测试表后,我们可以粘贴查询并在“运行SQL”按钮的帮助下执行查询。 结果集将显示在页面下方。
As you can see we used the AS keyword after the original column name and then we specified the alias. Therefore, the column headings have been changed with the alias and became more understandable.
如您所见,我们在原始列名之后使用了AS关键字,然后指定了别名。 因此,列标题已更改为别名,并且变得更易于理解。
For different cases, we may need to concatenate two different columns. However, if we don’t use an alias, the result set of the column heading will be “(No column name)”
对于不同的情况,我们可能需要连接两个不同的列。 但是,如果我们不使用别名,则列标题的结果集将为“((无列名)”)
SELECT CONCAT_WS(' ', CustomerInfList_FirstName, CustomerInf_LastName)
FROM Customer_InformationListForSale

For this circumstance, we can use SQL AS keyword to specify an alias to this combined column.
在这种情况下,我们可以使用SQL AS关键字为该组合列指定别名。
SELECT CONCAT_WS(' ', CustomerInfList_FirstName, CustomerInf_LastName) AS First_LastName
FROM Customer_InformationListForSale


The result set above shows that we can give an alias to the combined columns.
上面的结果集表明,我们可以为合并的列提供别名。
Most of the SQL built-in functions result does not return any column headings. For example, MIN, MAX, AVG, GETDATE, ABS, SQRT, DATEADD etc. functions act like this.
大多数SQL内置函数的结果均不返回任何列标题。 例如,MIN,MAX,AVG,GETDATE,ABS,SQRT,DATEADD等函数的作用如下。
SELECT MAX(CustomerInf_Age), AVG(CustomerInf_Age), GETDATE()
FROM Customer_InformationListForSale;

Now, we will give aliases to the column headings in the following query.
现在,我们将在以下查询中为列标题赋予别名。
SELECT MAX(CustomerInf_Age) AS CustomerMaximumAge, AVG(CustomerInf_Age) AS CustomerAverageAge, GETDATE() AS DateandTime
FROM Customer_InformationListForSale

If we wish to use space in the aliases, we must enclose the specified alias with quotes. In the following example, we will demonstrate this type of example.
如果我们希望在别名中使用空格,则必须使用引号将指定的别名括起来。 在下面的示例中,我们将演示这种类型的示例。
SELECT MAX(CustomerInf_Age) AS "Customer Maximum Age", AVG(CustomerInf_Age) AS "Customer Average Age", GETDATE() AS "Date and Time"
FROM Customer_InformationListForSale

使用SQL AS关键字为表赋予别名 (Giving aliases to tables using the SQL AS keyword)
When we intend to use a table more than once in a query, we can shorten the table name through the AS syntax. In the following query, we will give Customer alias to Customer_InformationListForSale and CustomerOrders alias to OrderTransaction_InformationListForSale.
当我们打算在一个查询中多次使用一个表时,可以通过AS语法缩短表名。 在以下查询中,我们将Customer别名命名为Customer_InformationListForSale,并将CustomerOrders别名命名为OrderTransaction_InformationListForSale。
SELECT Customer.CustomerInfList_FirstName AS "First Name", Customer.CustomerInf_LastName AS "Last Name", CustomerOrders.Amout AS "Order Amount"
FROM dbo.Customer_InformationListForSale
AS Customer
INNER JOIN
dbo.OrderTransaction_InformationListForSale
AS CustomerOrders
ON Customer.ID = CustomerOrders.Customer_InformationListForSale_CustID


As we can see that the table aliases have been placed after the FROM clause so we did not have to retype these long table names another time anywhere in the query. After the ON keyword, we used the alias of the tables.
如我们所见,表别名已放置在FROM子句之后,因此我们不必在查询中的其他任何地方再次键入这些长表名。 在ON关键字之后,我们使用了表的别名。
If we don’t use the aliases the query text will be like as follows.
如果我们不使用别名,则查询文本将如下所示。
SELECT Customer_InformationListForSale.CustomerInfList_FirstName, Customer_InformationListForSale.CustomerInf_LastName, OrderTransaction_InformationListForSale.Amout
FROM dbo.Customer_InformationListForSale
INNER JOIN
dbo.OrderTransaction_InformationListForSale
ON Customer_InformationListForSale.ID = OrderTransaction_InformationListForSale.Customer_InformationListForSale_CustID

结论 (Conclusion)
In this article, we learned the basic usage of the SQL AS keyword and as evident from our demonstration above, it provides the following advantages:
在本文中,我们学习了SQL AS关键字的基本用法,从上面的演示中可以明显看出,它具有以下优点:
- Improve query readability 提高查询可读性
- Degrade the complexity of the query 降低查询的复杂度
- Avoid striving to retype the long table names 避免尝试重新输入长表名
- It allows us to give more meaningful column headings 它使我们能够给出更有意义的列标题
翻译自: https://www.sqlshack.com/sql-as-keyword-overview-and-examples/
sql as关键字
sql as关键字_SQL AS关键字概述和示例相关推荐
- sql limit 子句_SQL按子句概述和示例
sql limit 子句 This article will cover the SQL ORDER BY clause including syntax, usage scenarios to so ...
- sql字符串函数_SQL字符串函数概述
sql字符串函数 In this article, we will try to give a brief overview of the SQL string functions used in S ...
- sql 自定义函数 示例_SQL滞后函数概述和示例
sql 自定义函数 示例 In the article SQL Server Lead function overview and examples, we explored Lead functio ...
- sql replace函数_SQL REPLACE函数概述
sql replace函数 In this article, I'll show you how to find and replace data within strings. I will dem ...
- sql server端口_SQL Server端口概述
sql server端口 This article is useful for a beginner in SQL Server administration and gives insights a ...
- sql delete语句_SQL Delete语句概述
sql delete语句 This article on the SQL Delete is a part of the SQL essential series on key statements, ...
- sql update 语句_SQL Update语句概述
sql update 语句 In this article, we'll walk-through the SQL update statement to modify one or more exi ...
- sql stuff 函数_SQL STUFF函数概述
sql stuff 函数 This article gives an overview of the SQL STUFF function with various examples. 本文通过各种示 ...
- sql server 别名_SQL Server别名概述
sql server 别名 This article gives an overview of SQL Server Alias and its usage for connecting with S ...
最新文章
- 使用VC内嵌Python实现的一个代码检测工具
- 服务器 | 种类及区别
- 宅在家限制智力输出?这场论文复现赛让思维发光
- Oracle 10中修改字符集(character set)
- python支持向量机回归_Python中支持向量机SVM的使用方法详解
- Eclipse导入他人的Maven工程报错
- curl php 百度,php curl 模拟登录百度主页
- spring总结(01)
- 算法岗面试整理 | 腾讯、字节、美团、阿里
- 【smart-transform】取自Atom的babeljs/cs/ts智能转es5 库
- win8下hosts保存文档失败,提示:请检查文件是否被另一个应用程序打开
- Android 开发神器系列(工具篇)之 Android 屏幕共享工具
- H264格式说明及解析
- 免费的Bootstrap管理后台模板集合
- I帧,P帧,B帧 压缩率对比
- 无线通信中 RSRP RSRQ RSSI SINR的定义和区别
- 如何Troubleshooting当Java Application发生死锁或Hangs
- 一般各类模具开模周期
- hdu 5148 Cities(树形dp)
- 炒股流程|开户流程|如何炒股