最短公共子序列_最短公共超序列
最短公共子序列
Problem statement:
问题陈述:
Given two strings, you have to find the shortest common super sequence between them and print the length of the super sequence.
给定两个字符串,您必须找到它们之间最短的公共超级序列,并打印超级序列的长度。
Input:
T Test case
T no of input string will be given to you.
E.g.
3
abcd abxy
sghk rfgh
svd vjhfd
Constrain
1≤ length (string1) ≤100
1≤ length (string2) ≤100
Output:
Print the length of the shortest super sequence formed from these two strings.
Example
例
T=3
Input:
abcd abxy
Output:
6 (abcdxy)
Input:
sghk rfgh
Output:
6 (srfghk)
Input:
svd vjhfd
Output:
6 (svjhfd)
Explanation with example:
举例说明:
Let there are two strings str1 and str2.
假设有两个字符串str1和str2 。
str1 = "abcd"
str2 = "abxy"
To find out the length of the super sequence from these two string is a bit difficult. To solve this problem we have to follow these steps:
从这两个字符串中找出超级序列的长度有些困难。 要解决此问题,我们必须遵循以下步骤:
We have to find out the longest common subsequence between the two strings. From "abcd" and "abxy" the longest common subsequence is "ab".
我们必须找出两个字符串之间最长的公共子序列。 从“ abcd”和“ abxy”开始,最长的公共子序列是“ ab”。
After getting the longest common subsequence we will add the non-common elements with the longest common subsequence. Non-common characters from "abcd" is "cd" and from "abxy" is "xy".
得到最长的公共子序列后,我们将添加具有最长的公共子序列的非公共元素。 来自“ abcd”的非常见字符为“ cd”,来自“ abxy”的非常见字符为“ xy”。
Therefore the length of the shortest common super sequence is:
因此,最短公共超序列的长度为:
length of the two strings-length of the longest common subsequencs
The length of the shortest common super sequence is6 (abcdxy) 6(abcdxy)
Using a dynamic programming algorithm to find out the longest common subsequence between two given string is very efficient and fast as compared to the recursion approach.
与递归方法相比,使用动态编程算法找出两个给定字符串之间最长的公共子序列非常有效且快速。
Let f(a,b) = count the number of common subsequences from the two string starting from 0 to position a and starting from 0 to position b.
令f(a,b) =计算两个字符串中从0开始到位置a以及从0开始到位置b的公共子序列数。
Considering the two facts:
考虑到两个事实:
If the character of string1 at index a and character of string1 at index b are the same then we have to count how many characters are the same between the two strings before these indexes? Therefore,
如果索引a的字符串 1的字符和索引b的字符串 1的字符相同,那么我们必须计算在这些索引之前两个字符串之间有多少个相同的字符? 因此,
f(a,b)=f(a-1,b-1)+1
If the character of string1 at index a and character of string1 at index b are not same then we have to calculate the maximum common character count between (0 to a-1 of string1 and 0 to b of string2) and (0 to a of string1 and 0 to b-1 of string2).
如果字符串 2中的索引和字符串1的字符索引b中的符不相同,则我们来计算之间的最大公共字符计数(0到字符串1的A-1和0至b 字符串2)和(0至a的string1和0到string2的 b-1 )。
f(a,b)=max((f(a-1,b),f(a,b-1))
For the two strings:
对于两个字符串:
str1 = "abcd"
str2 = "abxy"
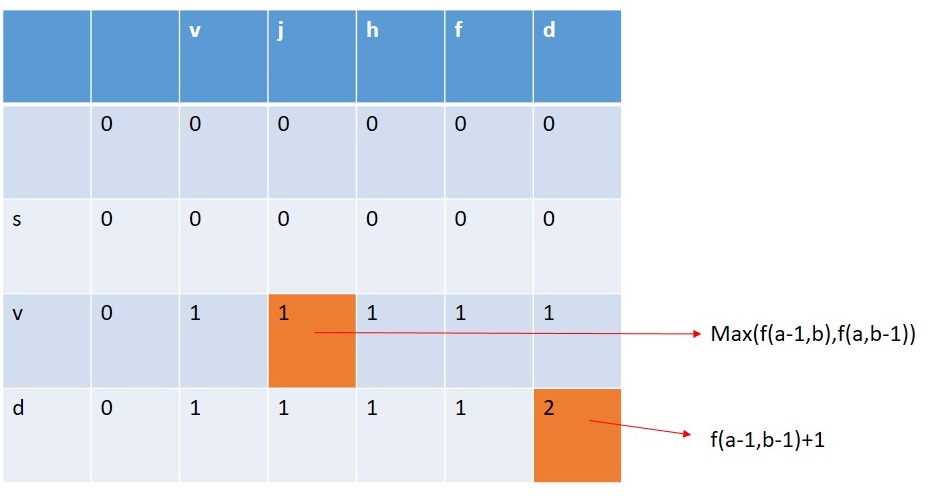
Problem solution:
问题方案:
Recursive algorithm:
递归算法:
int Function(str1,pos1,str2,pos2):
if pos1>=str1.length() || pos2>=str2.length()
return 0
for a=pos1 to 0
for b=pos1 to 0
if str[a]==str[a+l-1]
return Function(str1,a-1,str2,b-1)+1
else
return max(Function(str1,a,str2,b-1),Function(str1,a-1,str2,b));
DP conversion:
DP转换:
int Function(str1,str2):
arr[len1+1][len2+1]
for len=1 to str1.length()
for a=1 to str2.length()
if str[a]==str[b]
arr[a][b]=arr[a-1][b-1]+1
else
arr[a][b]=max(arr[a][b-1],arr[a-1][b])
return arr[len1-1][len2-1]
C++ Implementation:
C ++实现:
#include <bits/stdc++.h>
using namespace std;
int count_common_subsequence(string str1, string str2)
{int len1 = str1.length();
int len2 = str2.length();
int arr[len1 + 1][len2 + 1];
memset(arr, 0, sizeof(arr));
for (int i = 1; i <= len1; i++) {for (int j = 1; j <= len2; j++) {if (str1[i - 1] == str2[j - 1]) {arr[i][j] = arr[i - 1][j - 1] + 1;
}
else {arr[i][j] = max(arr[i - 1][j], arr[i][j - 1]);
}
}
}
return arr[len1][len2];
}
int main()
{int t;
cout << "Test Case : ";
cin >> t;
while (t--) {string str1, str2;
cout << "Enter the strings : ";
cin >> str1 >> str2;
cout << "Length of the longest common subsequence: " << count_common_subsequence(str1, str2) << endl;
}
return 0;
}
Output
输出量
Test Case : 3
Enter the strings : svd vjhfd
Length of the longest common subsequence: 2
Enter the strings : sghk rfgh
Length of the longest common subsequence: 2
Enter the strings : abcd abxy
Length of the longest common subsequence: 2
翻译自: https://www.includehelp.com/icp/shortest-common-super-sequence.aspx
最短公共子序列
最短公共子序列_最短公共超序列相关推荐
- 【算法设计与分析】最长公共子序列问题 动态规划算法 超详细
最长公共子序列问题描述 注意:最长公共子序列不一定是连续序列. 例如:"ASAFAGAHAJAK"与"AAAAAAA"的最长公共子序列为:AAAAAA 公共子序 ...
- 数据结构 - 字符串 - 最长公共子序列 + 最长公共子字符串 - 动态规划
最长公共子序列 /*** 最长公共子序列* 参考链接:http://blog.csdn.net/biangren/article/details/8038605* Created by 18710 o ...
- 动态规划算法之:最长公共子序列 最长公共子串(LCS)
1.先科普下最长公共子序列 & 最长公共子串的区别: 找两个字符串的最长公共子串,这个子串要求在原字符串中是连续的.而最长公共子序列则并不要求连续. 2.最长公共子串 其实这是一个序贯决策问题 ...
- 最长公共子序列算法 java_转【算法之动态规划(三)】动态规划算法之:最长公共子序列 最长公共子串(LCS)字符串相似度算法...
1.先科普下最长公共子序列 & 最长公共子串的区别: 找两个字符串的最长公共子串,这个子串要求在原字符串中是连续的.而最长公共子序列则并不要求连续. 2.最长公共子串 其实这是一个序贯决策问题 ...
- 最长公共子序列|最长公共子串|最长重复子串|最长不重复子串|最长回文子串|最长递增子序列|最大子数组和...
最长公共子序列|最长公共子串|最长重复子串|最长不重复子串|最长回文子串|最长递增子序列|最大子数组和 文章作者:Yx.Ac 文章来源:勇幸|Thinking (http://www.ahathi ...
- 动态规划最常见的习题 (最长公共子串、最长公共子序列、最短编辑距离)
(1)理论部分: (2)习题: 最长公共子串: 1 package month7.dp; 2 3 //https://www.nowcoder.com/questionTerminal/181a1a7 ...
- c语言最长公共子序列_序列比对(二十四)——最长公共子序列
原创: hxj7 本文介绍如何求解两个字符串的最长公共子序列. 最长公共子序列问题 前文<序列比对(二十三)--最长公共子字符串>介绍了如何求解两个字符串的最长公共子字符串,本文将介绍如何 ...
- java最长公共子序列_技术分享 | 最长公共子序列在比对工具的应用
即使如何1 在实际工作中,我们常常要对输出的文本和数据进行比对:以取证大师为例,取证大师导出的取证结果数据量很容易达到上万条.这类数据特点除了数量级大外,其实数据结构很相近.即使我们以无以伦比的细致和 ...
- 最长公共子序列_使用序列化查找对象中的脏字段
最长公共子序列 假设您正在开发一个将对象自动保存到数据库中的框架. 您需要检测两次保存之间所做的更改,以便仅保存修改的字段. 如何检测脏场. 最简单的方法是遍历原始数据和当前数据,并分别比较每个字段. ...
最新文章
- static的三种用法,定义静态变量,静态函数,静态代码块!
- 关于 SAP ABAP gateway OData 的一个诡异问题及解决办法
- python 分类变量xgboost_【转】XGBoost参数调优完全指南(附Python代码)
- vsftpd + Berkeley DB 创建基于虚拟用户的FTP
- [论文]论文的一般结构
- 用perl操作excel的介绍
- ansible远程在Windows server 2012 R2 安装vcredist(2008 2010 2012 2013)
- jquery html包含自身,jquery 获取 outerHtml 包含当前节点本身的代码
- 立创EDA超详细的PCB设计流程
- zotero word 调整样式 上标
- excel VBA自动化 - 多个工作簿自动合并到一个工作簿
- Fastadmin读取数据库配置
- Lzrs序數幻方集(32階七色彩虹)
- GBase 8c数据高可用技术
- 最好用的PDF阅读器,登陆华为应用市场首页
- POI-HSSFWorkbook合并单元格边框及文字居中问题
- 计算机网络物联网论文,物联网技术及其应用_计算机网络论文.doc
- 音视频基础(四)音频文件格式转换(支持重采样采样位数为24位)
- Cocos Creator 位图字体(艺术数字资源、BMFont、自定义位图字体、插件)
- zram disksize 设置
热门文章
- 有var d = new Date(‘20xx-m-09‘),可以设置为m+1月份的操作是?
- 腾讯手游助手android版本,腾讯游戏助手下载-腾讯游戏助手 安卓版v3.3.4.22-PC6安卓网...
- php order by where,无合适where条件过滤时尽量选择order by后的字段以驱动表进行查询...
- css React 单行省略和多行省略
- Selenium1 Selenium2 WebDriver
- 小程序在wxml页面中取整
- Google Chrome 浏览器JS无法更新解决办法
- Css3新属性:calc()
- matplotlib绘制饼状图
- 日期处理一之NSLalendar的使用