uva10534 hdu2198 双向LIS问题
LIS问题算是DP中很基础很简单的一类问题,其经典算法时间复杂度为O(n^2);利用它本身的特点,结合二分法,即可设计出时间复杂度为O(n*log n)的优化算法。LIS的变形好像不太多,下面是两个很相似的双向LIS问题,其实第二个题的代码是直接用第一个题的代码改的。
uva10543:http://uva.onlinejudge.org/index.php?option=com_onlinejudge&Itemid=8&category=17&page=show_problem&problem=1475
Problem D
Wavio Sequence
Input: Standard Input
Output: Standard Output
Time Limit: 2 Seconds
Wavio is a sequence of integers. It has some interesting properties.
· Wavio is of odd length i.e. L = 2*n + 1.
· The first (n+1) integers of Wavio sequence makes a strictly increasing sequence.
· The last (n+1) integers of Wavio sequence makes a strictly decreasing sequence.
· No two adjacent integers are same in a Wavio sequence.
For example 1, 2, 3, 4, 5, 4, 3, 2, 0 is an Wavio sequence of length 9. But 1, 2, 3, 4, 5, 4, 3, 2, 2 is not a valid wavio sequence. In this problem, you will be given a sequence of integers. You have to find out the length of the longest Wavio sequence which is a subsequence of the given sequence. Consider, the given sequence as :
1 2 3 2 1 2 3 4 3 2 1 5 4 1 2 3 2 2 1.
Here the longest Wavio sequence is : 1 2 3 4 5 4 3 2 1. So, the output will be 9.
Input
The input file contains less than 75 test cases. The description of each test case is given below: Input is terminated by end of file.
Each set starts with a postive integer, N(1<=N<=10000). In next few lines there will be N integers.
Output
For each set of input print the length of longest wavio sequence in a line.
Sample Input Output for Sample Input
10 1 2 3 4 5 4 3 2 1 10 19 1 2 3 2 1 2 3 4 3 2 1 5 4 1 2 3 2 2 1 5 1 2 3 4 5 |
9 9 1 |
#include <cstdio>
#include <algorithm>
using namespace std;
int LIS[10010];
int LDS[10010];
int minlis[10010];
int data[10010];
int binary(int l, int r , int x){int mid; while(l <= r){mid = (l + r) >> 1; if(minlis[mid] < x) l = mid + 1; else r = mid -1;} return l;
}
int main(){int n, maxn, len;while(~scanf("%d", &n)){for(int i = 1; i <= n; i++) scanf("%d", data + i); minlis[1] = data[1]; LIS[1] = 1; len = 1;for(int i = 2; i <= n; i++){LIS[i] = binary(1, len, data[i]); minlis[LIS[i]] = data[i]; if(LIS[i] > len) len++;}minlis[1] = data[n]; LDS[n] = 1; len = 1;for(int i = n-1; i > 0; i--){LDS[i] = binary(1, len, data[i]); minlis[LDS[i]] = data[i]; if(LDS[i] > len) len++;}maxn = 0;for(int i = 1; i <= n; i++)maxn = max(maxn , 2 * min(LIS[i] , LDS[i]) - 1); printf("%d\n", maxn);}return 0;
}
How many elements you must throw out?
Time Limit: 1000/1000 MS (Java/Others) Memory Limit: 32768/32768 K (Java/Others)
Total Submission(s): 383 Accepted Submission(s): 152
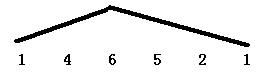
You are given a sequence of numbers. Output the minimal number of elements you must throw out from the given sequence such that the remaining subsequence satisfies the condition described above.
#include <cstdio>
#define max(a , b) ((a) > (b) ? (a) : (b))
int LIS[55];
int LDS[55];
long long minlis[55];
long long data[55];
int binary(int l, int r , int x){int mid; while(l <= r){mid = (l + r) >> 1;if(minlis[mid] < x) l = mid + 1; else r = mid -1;} return l;
}
int main(){int n, maxn, len;while(~scanf("%d", &n) && n != 0){for(int i = 1; i <= n; i++) scanf("%lld", data + i); minlis[1] = data[1]; LIS[1] = 1; len = 1;for(int i = 2; i <= n; i++){LIS[i] = binary(1, len, data[i]); minlis[LIS[i]] = data[i]; if(LIS[i] > len) len++;}minlis[1] = data[n]; LDS[n] = 1; len = 1;for(int i = n-1; i > 0; i--){LDS[i] = binary(1, len, data[i]); minlis[LDS[i]] = data[i]; if(LDS[i] > len) len++;}maxn = 0;for(int i = 1; i <= n; i++)maxn = max(maxn , LIS[i] + LDS[i] - 1); printf("%d\n", n - maxn);}return 0;
}
uva10534 hdu2198 双向LIS问题相关推荐
- UVA10534 Wavio Sequence【LIS+DP】
Wavio is a sequence of integers. It has some interesting properties. • Wavio is of odd length i.e. L ...
- this指向、数据双向流、传递参数、JSX中循环、React中样式、路由、引入资源的其它方式、create-react-app脚手架、事件处理、获取数据、UI框架推荐、pc桌面应用electronjs
改变this指向的几种方式: //1.使用箭头函数代替原始函数写法:getState=()=>{}//2.在函数调用时给函数名加bind(this)方法:(bind中第一个参数表示修改this指 ...
- 区域云LIS检验系统源码 商业级LIS全套源代码 预留标准HIS、仪器数据接入接口
商业级高端云LIS系统源码,可直接上手项目,正版授权. 系统概述: 系统完全采用B/S架构模式,扩展性强.整个系统的运行基于WEB层面,只需要在对应的工作台安装一个浏览器软件有外网即可访问. 私信我了 ...
- Vue.js 基础语法 入门语句 Vue学习笔记 v-model 双向数据绑定
Vue.js 基础语法,入门语句,Vue学习笔记 学习网站:https://www.bilibili.com/video/BV15741177Eh vue 的体验 响应式:数据一旦改变,视图就会响应改 ...
- lis通道号_LIS系统功能模块和技术参数
1 南华县中医院 LIS 系统功能模块和技术参数 检验申请系统: 1. 通过和 HIS 建立无缝连接,可以直接读取 HIS 中的检验申请单. 2. 支持各工作站录入申请单. 3. 可打印检验申请单 ...
- lis双工常见设置!
LIS仪器双工手册 LIS仪器双工手册 1 化学发光Bayer Centaur 1 化学发光 罗氏 Roche ES2010 2 化学发光 罗氏E411 2 生化仪 奥林巴斯 AU400 2 生化仪 ...
- LIS系统通讯程序原理与实现
一.BSLIS仪器数据采集方法 BSLIS对检验仪器的数据采集主要通过串行口通讯.USB端口通讯.TCP/IP通讯.定时监控数据库和手工录入等几种方法.串行口通讯最为普遍,采用RS-232C标准,一般 ...
- 医院检验管理系统lis系统源码
子系统 功能模块 功能描述 检验 医生 工作 平台 检验 录入 处理 模块 界面集成录入.审核.报告.打印.质控图功能.一个界面能完成大部分检验等相关工作.方便使用. 提供自定义病人资料显示列,审核和 ...
- 医院LIS系统解决方案
要求检验项目直接从 LIS 系统发送到检验设备 (同时发送标本编号与仪器架号相对应) , 仪器结果出来后又会以同样的对应号发送回 LIS 上,就不需要读取条形码也不存在输错项目 或漏检项目的问题,对于 ...
最新文章
- sudo找不到命令:修改sudo的PATH路径
- python get方法请求参数_python中requests库get方法带参数请求
- Oracle sql 中的字符(串)替换与转换[转载]
- boost random library的使用
- 最小生成树的Kruskal算法实现
- ElasticSearch搜索引擎常见面试题总结
- window8下安装RabbitMQ
- Kafka-batch.size属性
- 小米高管:已投大量精力研发手机AI芯片,造不造还没定
- Spring事件监听机制
- 监听pda扫描_uniapp App监听PDA扫描工具数据
- Oracle用户管理命令
- TOP Network技术总监Justin:TOP公链已率先实现多层状态分片
- 支付宝基金自选管理系统Springboot + Vue 实现
- Android 版本号和分支查看
- 电脑连接手机热点后无法上网或提示找不到服务器IP地址(DNS错误)
- MATLAB绘制“问题儿童表情包”动图2
- cocos creator 3D | 拇指投篮 | 3D项目入门实战
- ★互联网告别免费时代,准备…
- java跳格子不同跳发_(算法)跳格子
热门文章
- 云集微店怎么做 我的第一份生意经
- 软件测试市场前景怎么样,软件测试的发展前景怎么样?
- 多边形区域填充算法--扫描线填充算法(有序边表法)
- 最全C++知识点--重载运算与类型转换
- 2022危险化学品经营单位主要负责人特种作业证考试题库及答案
- 基于小波的图像边缘检测,小波变换边缘检测原理
- 2021年CCPC河南省赛部分题解
- android脚本实现自动捉妖,一起来捉妖自动秒杀脚本下载-一起来捉妖自动秒杀辅助 最新版_爱下手机站...
- opcache php7,让子弹飞~利用 OPcache 扩展提升 PHP7 性能 | Laravel 篇
- 理解yolov3的anchor、置信度