Microsoft Updater Application Block 1.4.3 KeyValidator类设计 [翻译]
译者:Tony Qu
KeyValidator类提供一个基于对称密钥的验证器,该章节将介绍KeyValidator设计的以下几个方面:
问题描述
设计目标、权衡和问题
解决方案描述
具体实现
问题描述
一个通常使用的验证方法是使用对称密钥进行签名和验证数据。为了支持这项技术,必须实现一个基于对称密钥的验证器。
设计目标、权衡和问题
1. KeyValidator应该支持由.net的KeyedHashAlgorithm类支持的对称密钥。
2. 开发人员应该能够在应用程序升级器的配置文件中指定密钥,该密钥应该用base64字符串存储。如果没有密钥被指定,KeyValidator会抛出一个异常。
解决方案描述
KeyValidator类派生自IValidator接口,它使用.net的KeyedHashAlgorithm类基于一个对称密钥生成和验证签名。
具体实现
KeyValidator类由Microsoft.ApplicationBlocks.ApplicationUpdater.Validators命名空间实现。
实现KeyValidator需要考虑四个方面:
1. 密钥初始化
2. 签名
3. 验证
4. KeyValidator配置
密钥初始化
1. Init方法用于从应用程序升级器配置文件传递<validator>元素给KeyValidator类。该元素应该包含<key>子元素,该元素中以base64字符串存储密钥。<key>元素用于初始化验证密钥,该初始化过程代码在下面的代码片断中。
[VB.NET]
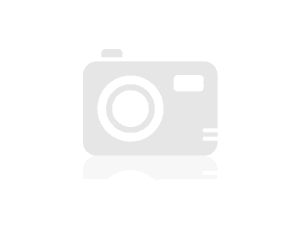
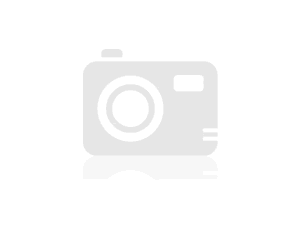





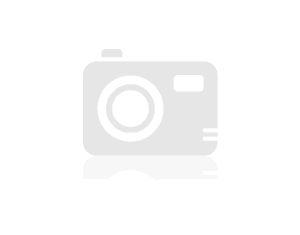
[C#]
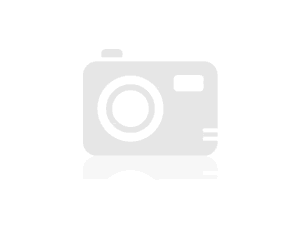
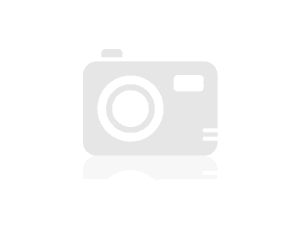
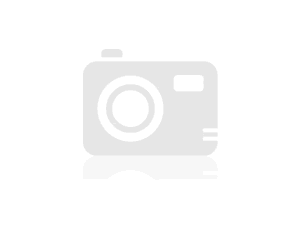





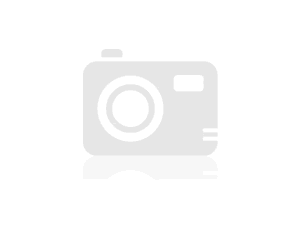



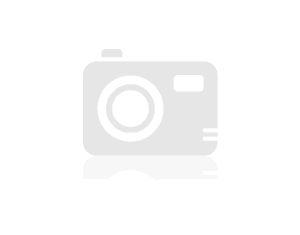
2. Init方法使用一个ExtractKeyFromNode私有函数解析位于配置文件中的base64加密的字符串,并且返回一个byte数组。下面的代码展示了ExtractKeyFromNode函数。
[VB.NET]
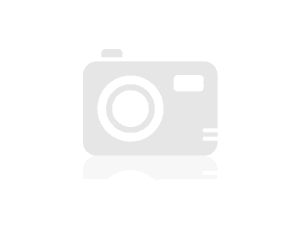
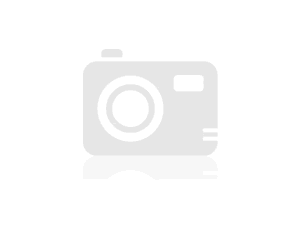










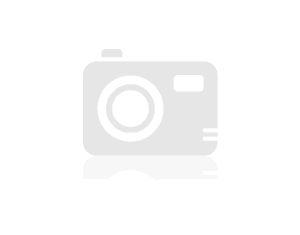
[C#]
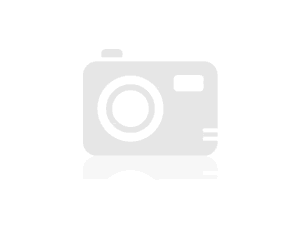
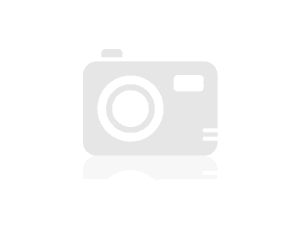
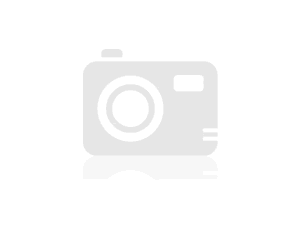




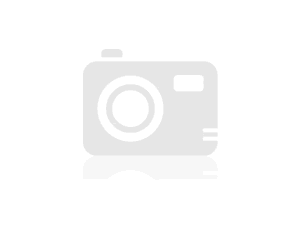





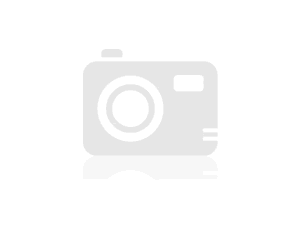





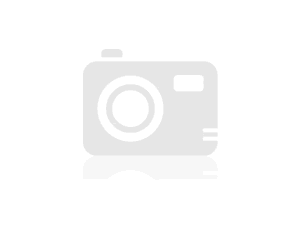




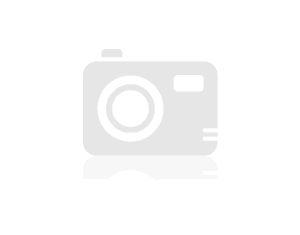
签名
重载的Sign方法使用KeyedHashAlgorithm类生成hashed签名,下面的代码片断展示了这一过程:
[VB.NET]
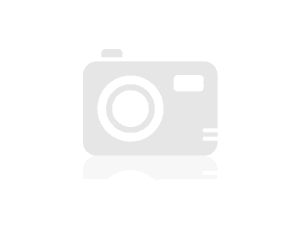
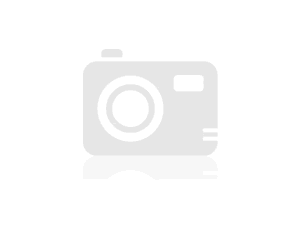
















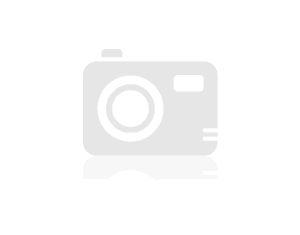
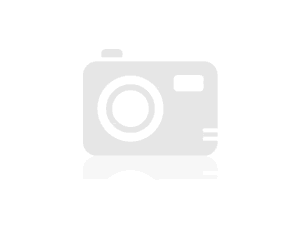
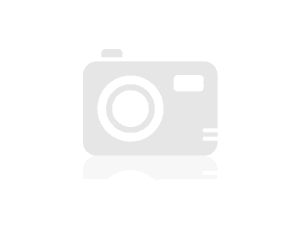
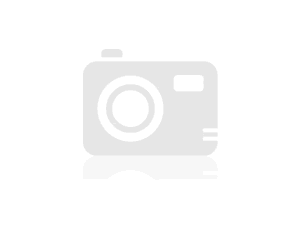












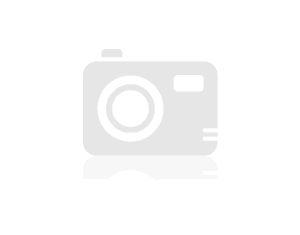
[C#]
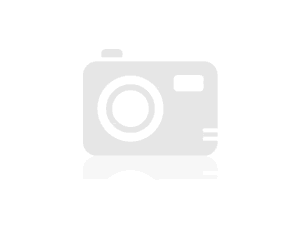
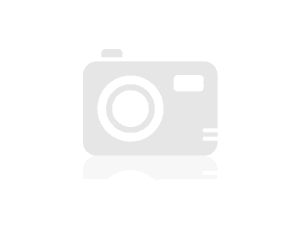
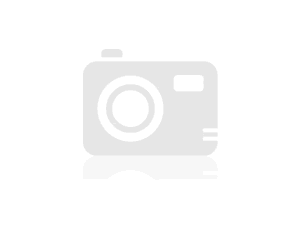





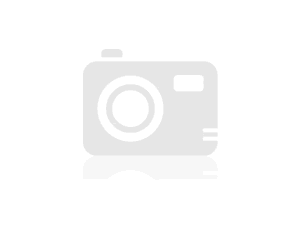








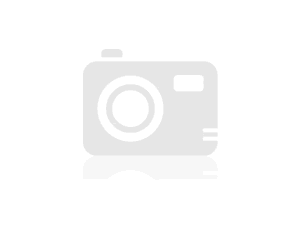






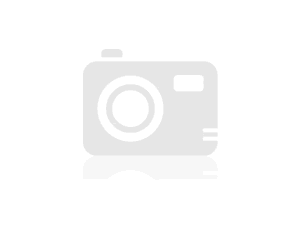



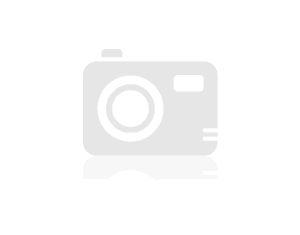
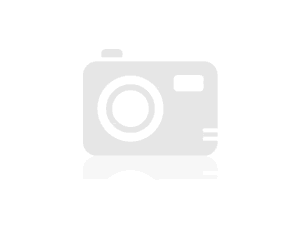
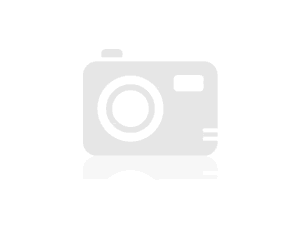
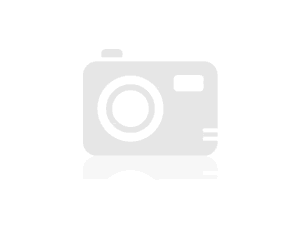
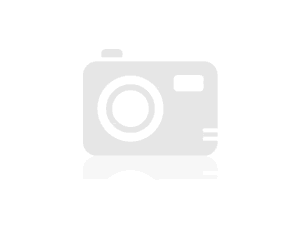
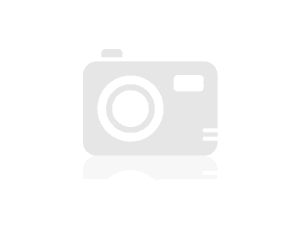
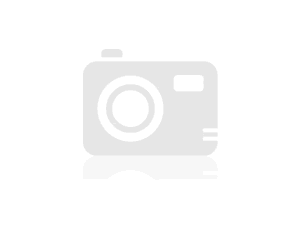






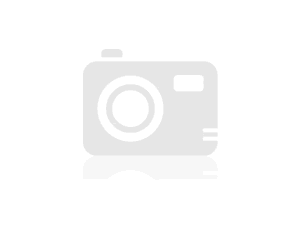







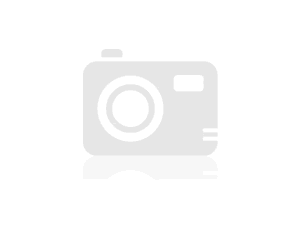




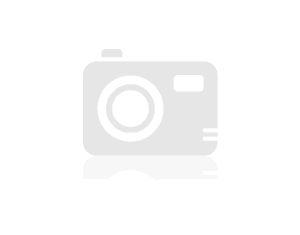
验证
Validate方法使用相同的KeyedHashAlgorithm验证签名和密钥,该密钥用于生成签名。Validate方法在下面的代码片断中实现:
[VB.NET]
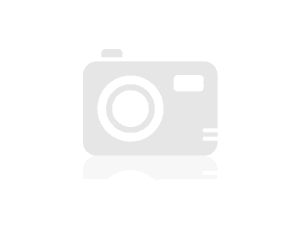
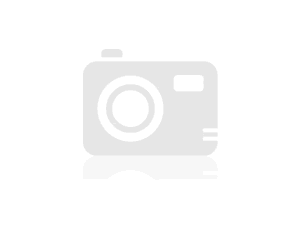














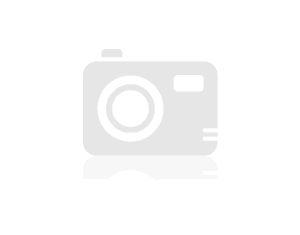
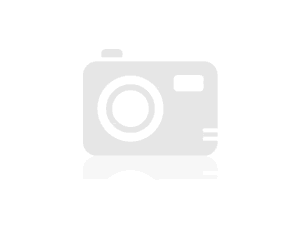
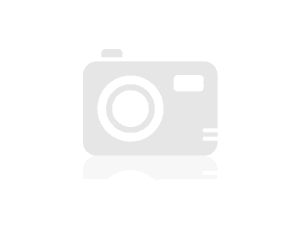
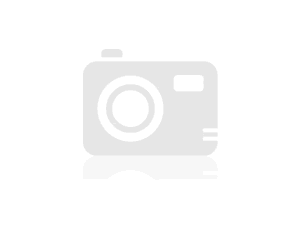











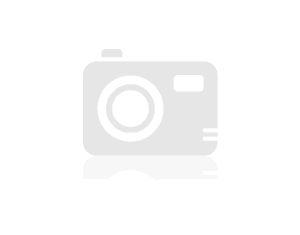
[C#]
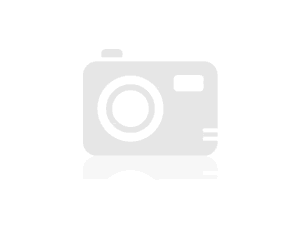
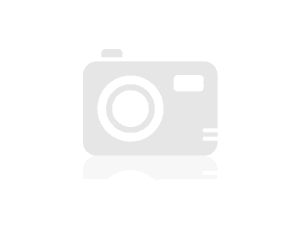
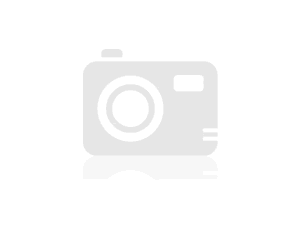






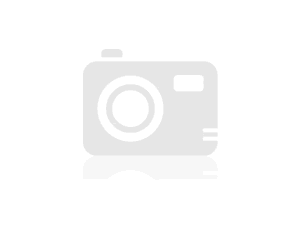






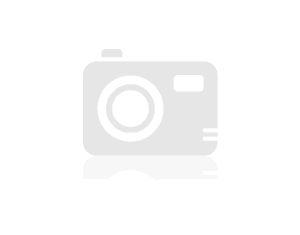
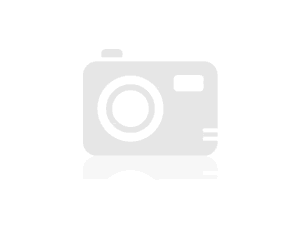
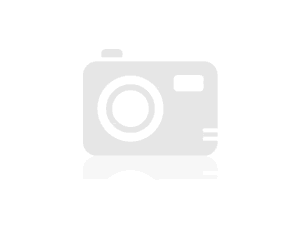
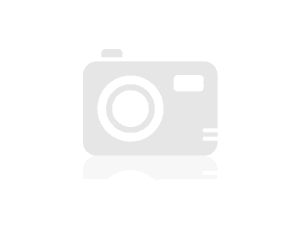
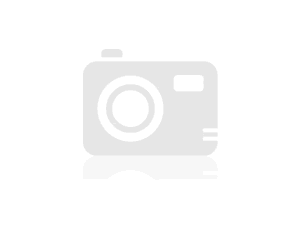










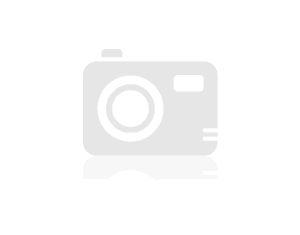
这两个Validate的重载方法使用一个私有的compareKeys来确认签名匹配。compareKeys函数在下面的代码中实现:
[VB.NET]
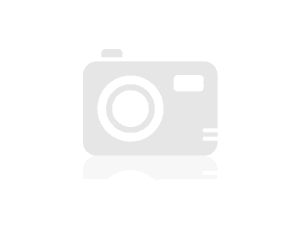
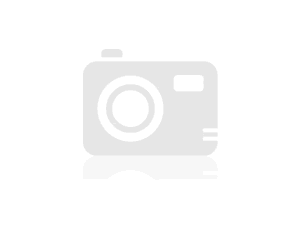











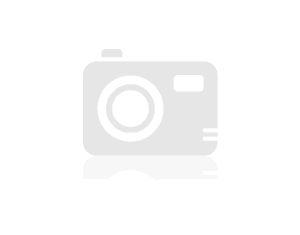
[C#]
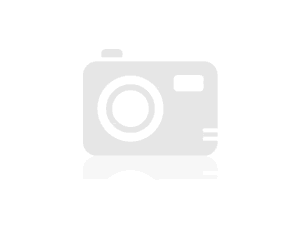
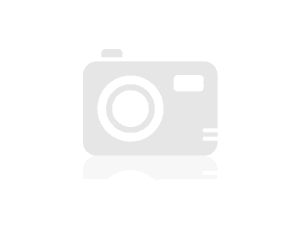
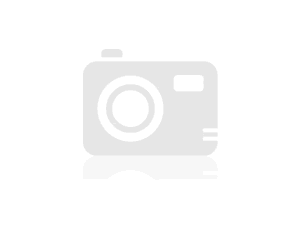




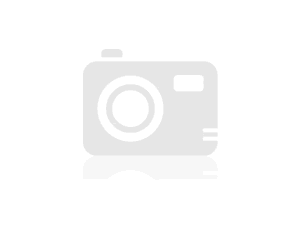




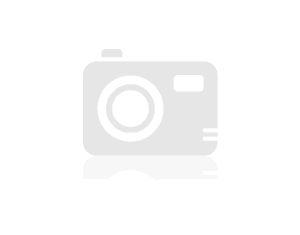
KeyValidator配置
为了使用KeyValidator,你必须在应用程序升级器配置文件中包含一个<validator>元素,指定KeyValidator集合和类型的全名,以及你要使用的base64加密密钥。一个<validator>配置元素的例子如下所示:
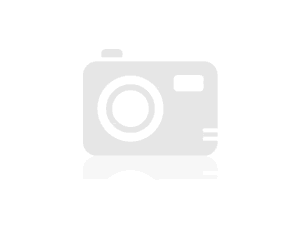
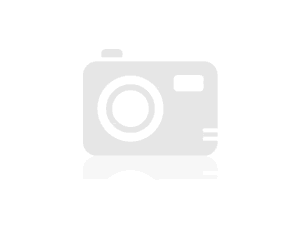
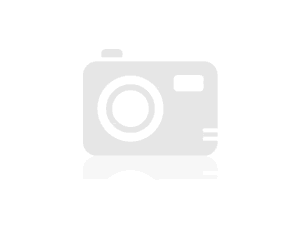
Microsoft Updater Application Block 1.4.3 KeyValidator类设计 [翻译]相关推荐
- Microsoft Updater Application Block 1.5.3 服务器端manifest文件设计 [翻译]
Microsoft Updater Application Block 服务器端manifest文件设计 译者:Tony Qu Manfest文件用于列出一次升级所需要的所有的文件,它与验证签名相关联 ...
- Microsoft Updater Application Block 1.2.1 核心设计(core design) [翻译]
Microsoft Updater Application Block 核心设计(core design) 译者:Tony Qu Updater Application Block由一个核心应用程序升 ...
- Updater Application Block for .NET
Introduction Do you need to deploy updates to .NET applications across multiple desktops? Would you ...
- Updater Application Block v1.0 翻译文档目录
为方便大家阅读有关Updater Application Block v1.0 的翻译文章,在本文中列出最近翻译的相关文章目录. Updater Application Block v1.0 介绍 U ...
- Updater Application Block for .net 2.0 发布了
Updater Application Block for .net 2.0 终于发布了,做smart client的兄弟来下载吧! 另外 Smart Client Application Block ...
- 近期工作:Updater Application Block (UAB)继续
阅读: 1.Using Updater Block by Praveen Nayak http://www.codeproject.com/csharp/VanillaUpdaterBlock.a ...
- 黄聪:Microsoft Enterprise Library 5.0 系列教程(九) Policy Injection Application Block
代理对象(Proxy Object)会通过Handler链定位到真实对象(Real Object),而Policy则被注入到代理对象和真实对象中.整个流程如图: 我个人对Policy Injectio ...
- 黄聪:Microsoft Enterprise Library 5.0 系列教程(二) Cryptography Application Block (高级)
原文:黄聪:Microsoft Enterprise Library 5.0 系列教程(二) Cryptography Application Block (高级) 本章介绍的是企业库加密应用程序模块 ...
- 黄聪:Microsoft Enterprise Library 5.0 系列教程(四) Logging Application Block
企业库日志应用程序模块工作原理图: 从上图我们可以看清楚企业库日志应用程序模块的工作原理,其中LogFilter,Trace Source,Trace Listener,Log Formatter ...
最新文章
- dbartisan mysql_Sybase数据库安全
- IIC原理及简单流程
- python 定时器_按键精灵定时器介绍和使用,不会的小伙伴速速看看精辟
- php monolith,单体架构(Monolith)与微服务架构(MicroService)
- sae java 开发环境_新浪开放平台 sae环境 java主机使用感受
- LR运行负载测试场景-笔记
- WAMP(windows+apache+mysql+php)
- 03 - 雷达的基本组成
- 剧本创作时的标准格式,让你的剧本轻松得到制片公司青睐
- 有多少旅游企业入驻了抖音平台?有多少抖音用户喜欢看旅游视频?
- Justinmind,为移动设计而生
- 【转】ORA-28040: 没有匹配的验证协议
- java生成自己的Doc文档
- Word: 是否将更改保存到WordCmds.dot中?
- 对称矩阵到三对角矩阵的Lanczos推导(python,数值积分)
- 浅析B树、B+树插入删除操作(附代码实现)
- 屏幕录像软件使用心得
- 利用python处理Excel表格中的销售数据
- python 警告:simplify chained comparison
- 计算机考试题画图板,9月全国计算机一级Photoshop考试试题操作题
热门文章
- php解析定时任务格式,php 实现定时任务简单实现
- linux查看usb设备名称,Linux系统下查看USB设备名及使用USB设备
- 苹果6重置系统后无服务器,iphone6总是无服务,恢复初始设置就好了,然后一两天又不行了,怎么处理...
- 通过build.xml在Eclipse中导入工程
- 支持access的php框架,NginX友好的PHP框架
- matlab 离散点求导_Matlab的离散点曲线导数曲率数值模拟方法
- flink读取不到文件_flink批处理从0到1
- python删除文件代码_python2.7删除文件夹和删除文件代码实例
- tomcat ajp协议安全限制绕过漏洞_Apache tomcat 文件包含漏洞复现(CVE20201938)
- 评分卡模型开发(四)--定量指标筛选