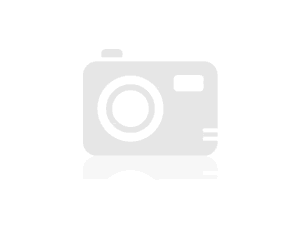
该类适应读写如下格式的.xml,.config文档
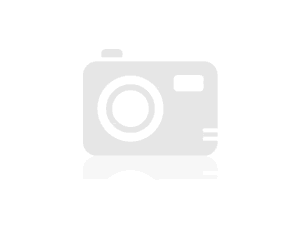
<?xml version="1.0" encoding="utf-8" standalone="no"?>
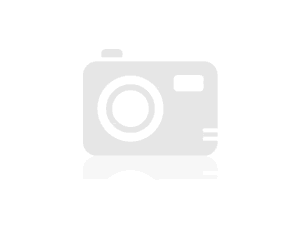
<!--This is a config file ,writer by furenjun-->
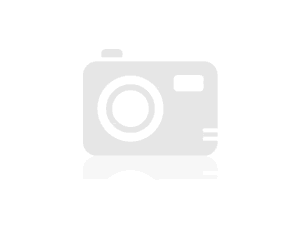
<configuration>
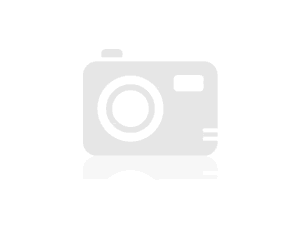
<appSettings>
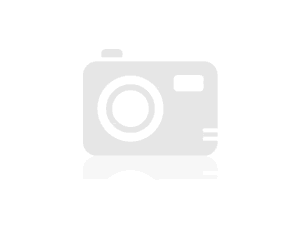
<add key="SqlStr0" value="0" />
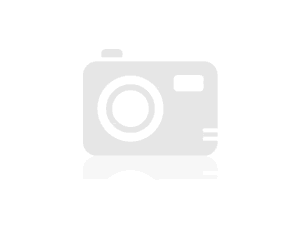
<add key="SqlStr1" value="1" />
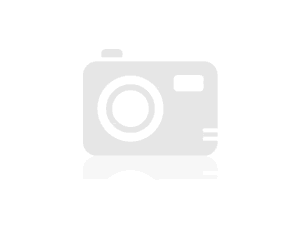
<add key="2006-7-3 16:05:08" value="1" />
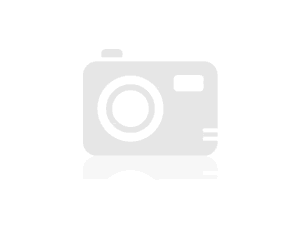
</appSettings>
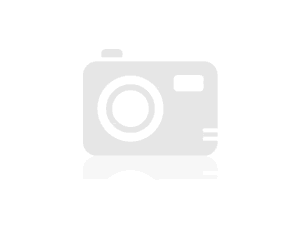
</configuration>
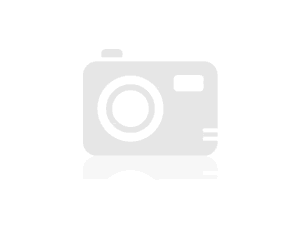
using System;
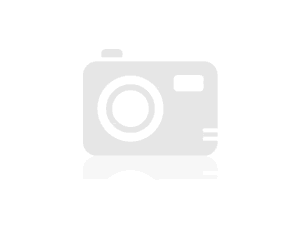
using System.Configuration ;
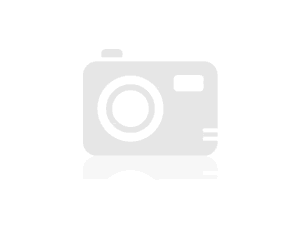
using System.Reflection ;
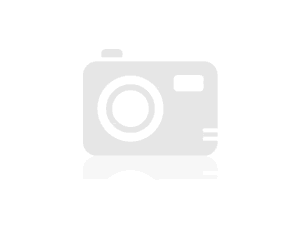
using System.Xml;
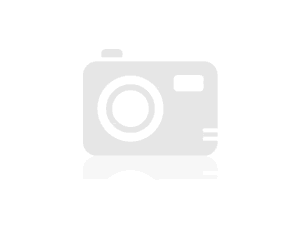
using System.IO;
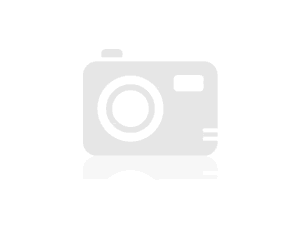
using System.Collections.Specialized;
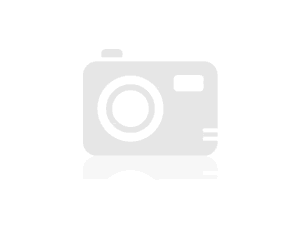
using System.Collections;
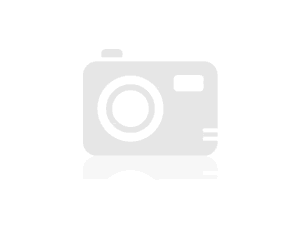
using System.Data ;
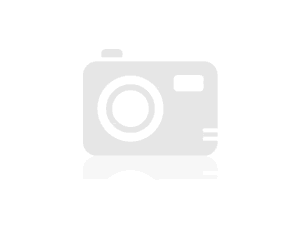
using System.Diagnostics;
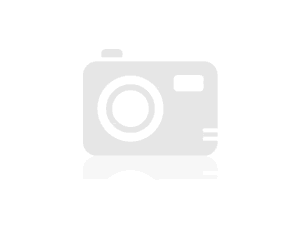
using System.Windows.Forms;
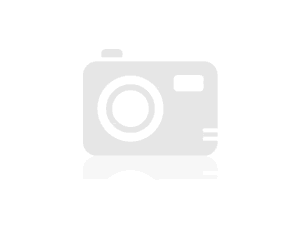
namespace 读写配置文件
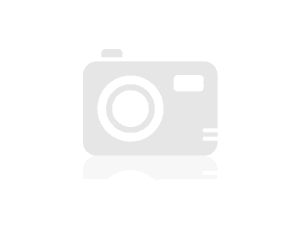
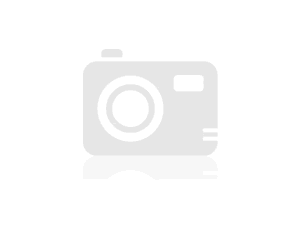

{

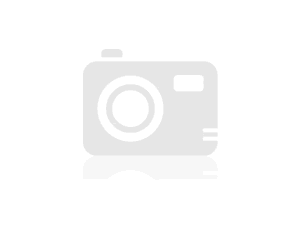
/**//// <summary>

/// MrfuRWXmlFile 的摘要说明。

/// </summary>

public class MrfuRWXmlFile


{

public static string ConfigFullName="";

private NameValueCollection appSettings = null;

public MrfuRWXmlFile()


{

//

// TODO: 在此处添加构造函数逻辑

//

// Assembly MyAssembly= Assembly.GetExecutingAssembly();

// string ConfigLocPath=MyAssembly.Location;

// string strDireName=Path.GetDirectoryName(ConfigLocPath) ;

// //MessageBox.Show(ConfigLocPath+"\n"+strDireName) ;

// ConfigFullName=ConfigLocPath+".config";

ConfigFullName=Application.StartupPath+@"\myProject.config" ;

//MessageBox.Show(ConfigFullName) ;

}

private static void CreateNewXmlFile()


{

XmlTextWriter myXmlTextWriter = new XmlTextWriter (ConfigFullName, System.Text.Encoding.UTF8 );

myXmlTextWriter.Formatting = Formatting.Indented;

myXmlTextWriter.WriteStartDocument(false); //设置standalone="no" //当为(true)时 <?xml version="1.0" standalone="yes" ?>

// myXmlTextWriter.WriteDocType("bookstore", null, "books.dtd", null);

myXmlTextWriter.WriteComment("This is a config file ,writer by furenjun");

myXmlTextWriter.WriteStartElement("configuration");

myXmlTextWriter.WriteStartElement("appSettings", null);
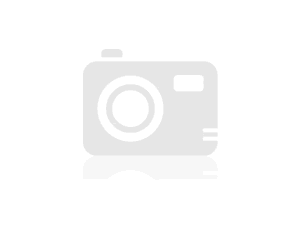

'写入其它元素'#region '写入其它元素'

// myXmlTextWriter.WriteAttributeString("genre","autobiography");

// myXmlTextWriter.WriteAttributeString("publicationdate","1979");

// myXmlTextWriter.WriteAttributeString("ISBN","0-7356-0562-9");

// myXmlTextWriter.WriteElementString("title", null, "The Autobiography of Mark Twain");

// myXmlTextWriter.WriteStartElement("Author", null);

// myXmlTextWriter.WriteElementString("first-name", "Mark");

// myXmlTextWriter.WriteElementString("last-name", "Twain");

// myXmlTextWriter.WriteEndElement();

// myXmlTextWriter.WriteElementString("price", "7.99");

#endregion

myXmlTextWriter.WriteEndElement();

myXmlTextWriter.WriteEndElement();

//Write the XML to file and close the myXmlTextWriter

myXmlTextWriter.Flush();

myXmlTextWriter.Close();

}

public MrfuRWXmlFile(string ConfigFilePath)


{

//

// TODO: 在此处添加构造函数逻辑

//

ConfigFullName=ConfigFilePath;

}

public string GsConfigFilePath


{

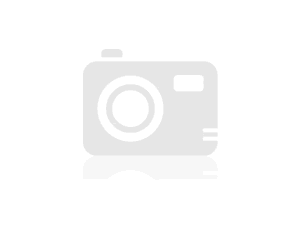
get

{return ConfigFullName;}

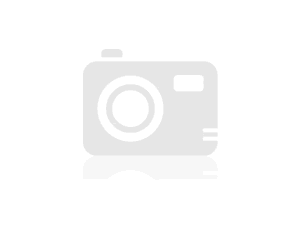
set

{ConfigFullName=value;}

}

//初始化xml文档

//首先请右击"项目"-->添加一个新项-->选择"应用程序配置文件"-->点击确定

public static bool CreateXmlFile()


{

if(!File.Exists(ConfigFullName) )

CreateNewXmlFile();

bool bflag=true;

ConfigXmlDocument configXmlDoc=new ConfigXmlDocument();// XmlDocument();

configXmlDoc.Load(ConfigFullName) ;
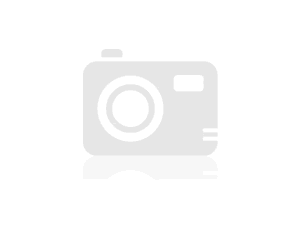

//检查结点是否存在#region //检查结点是否存在

// //XmlNodeList xList=configXmlDoc.SelectNodes ("//appSettings");//可以根据实际需要更改它的查找范围.如SelectNodes("//User")

// XmlNodeList xList=configXmlDoc.DocumentElement.ChildNodes ;

//

//

// if (xList.Count> 0)

// {

//

//

// foreach(XmlNode node in xList)

// {

// if(node.Name =="appSettings")

// MessageBox.Show ("找到");

// return;

// }

// }

// else

// {

// MessageBox.Show("no nodes") ;

// }

//

// XmlNode root2 = configXmlDoc.FirstChild;

//

// //Display the contents of the child nodes.

// if (root2.HasChildNodes)

// {

// string strs="";

// for (int i=0; i<root2.ChildNodes.Count; i++)

// {

// strs+=(root2.ChildNodes[i].InnerText)+"\n";

// }

// MessageBox.Show(strs) ;

// }

#endregion

XmlNode appSettingsNode;

appSettingsNode = configXmlDoc.SelectSingleNode("configuration/appSettings");

if(appSettingsNode.LocalName=="appSettings") //或者(appSettingNode!=null)


{

//如果xml文档中已存在该结点则采用选择定位该结点的方法

appSettingsNode.RemoveAll();

//MessageBox.Show(appSettingsNode.LocalName) ;

}

else


{

// Create a new element node.

XmlNode newElem;

newElem = configXmlDoc.CreateNode(XmlNodeType.Element, "appSettings", "");

//newElem.InnerText = "290";

//"Add the new element to the document

"

XmlElement root = configXmlDoc.DocumentElement;

root.AppendChild(newElem);

//("Display the modified XML document

");

//Console.WriteLine(configXmlDoc.OuterXml);

appSettingsNode =newElem;

}

try


{

for (int i = 0; i < 2; i++)


{

string key = "SqlStr"+i.ToString() ;

string val = i.ToString() ;

//创建结点

XmlNode node = configXmlDoc.CreateNode(XmlNodeType.Element, "add", "");

//创建属性"key"并为其赋值

XmlAttribute attr = configXmlDoc.CreateAttribute("key");

attr.Value = key;

node.Attributes.Append(attr);

//创建属性"value"并为其赋值

attr = configXmlDoc.CreateAttribute("value");

attr.Value = val;

node.Attributes.Append(attr);

appSettingsNode.AppendChild(node);

}

configXmlDoc.Save(ConfigFullName);

//MessageBox.Show("创建Xml文档结点成功.","系统提示",MessageBoxButtons.OK ,MessageBoxIcon.Information ) ;

}

catch (Exception ex)


{

bflag=false;

throw new ConfigurationException(ex.ToString() );

}

return bflag;

}

private void LoadFromFile()


{

//if (appSettings == null)

//{

try


{

//string filePath=@"E:\淄博市鲁中机动车检测中心数据联网软件\FromHlk\读写配置文件\ConfigurationSettingsRW_demo\ConfigurationSettingsRW_demo\ConfigurationSettingsRW.exe.config";

ConfigXmlDocument configXml = new ConfigXmlDocument();// XmlDocument();

//configXml.Load(filePath);//this.configFilePath);

configXml.Load(ConfigFullName);

//MessageBox.Show(ConfigFullName ) ;

XmlNode appSettingsNode = configXml.SelectSingleNode("configuration/appSettings");

//MessageBox.Show(appSettingsNode.LocalName) ;

if(appSettingsNode.LocalName=="appSettings")


{

NameValueSectionHandler handler = new NameValueSectionHandler();

appSettings = new NameValueCollection((NameValueCollection)handler.Create(null, null, appSettingsNode));

}

}

catch (Exception ex)


{

throw new ConfigurationException("Error while loading appSettings. Message: " + ex.Message, ex);

}

//}

}

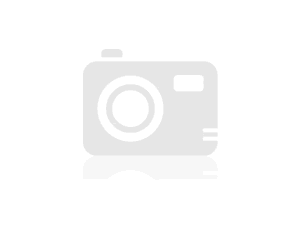
/**//// <summary>

/// 获取配置文件中的结点"appSettings"中的值

/// </summary>

/// <returns></returns>

public DataTable GetSettingsValue()


{

LoadFromFile();

DataTable tblAppSettings=new DataTable("Settings") ;

tblAppSettings.Columns.Add("key", typeof(System.String));

tblAppSettings.Columns.Add("value", typeof(System.String));

for (int i = 0; i < appSettings.Count; i++)


{

string key = appSettings.GetKey(i);

string val = appSettings.Get(i);

DataRow row = tblAppSettings.NewRow();

row["key"] = key;

row["value"] = val;

tblAppSettings.Rows.Add(row);

}

return tblAppSettings;

}

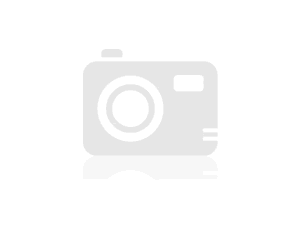
/**//// <summary>

/// 添加一条新的纪录

/// </summary>

/// <param name="strKey"></param>

/// <param name="strValue"></param>

/// <returns></returns>

public bool AddNewRecorder(string strKey,string strValue)


{

bool bflag=true;

XmlDocument configXmlDoc=new XmlDocument();

configXmlDoc.Load(ConfigFullName) ;

XmlNode appSettingsNode;

appSettingsNode = configXmlDoc.SelectSingleNode("configuration/appSettings");

if(appSettingsNode==null)


{

// Create a new element node.

XmlNode newElem;

newElem = configXmlDoc.CreateNode(XmlNodeType.Element, "appSettings", "");

//newElem.InnerText = "290";

//"Add the new element to the document

"

XmlElement root = configXmlDoc.DocumentElement;

root.AppendChild(newElem);

//("Display the modified XML document

");

//Console.WriteLine(configXmlDoc.OuterXml);

appSettingsNode =newElem;

}

try


{

string key = strKey;

string val = strValue;

//创建结点

XmlNode node = configXmlDoc.CreateNode(XmlNodeType.Element, "add", "");

//创建属性"key"并为其赋值

XmlAttribute attr = configXmlDoc.CreateAttribute("key");

attr.Value = key;

node.Attributes.Append(attr);

//创建属性"value"并为其赋值

attr = configXmlDoc.CreateAttribute("value");

attr.Value = val;

node.Attributes.Append(attr);

appSettingsNode.AppendChild(node);

configXmlDoc.Save(ConfigFullName);

//MessageBox.Show("创建Xml文档结点成功.","系统提示",MessageBoxButtons.OK ,MessageBoxIcon.Information ) ;

}

catch (Exception ex)


{

bflag=false;

throw new ConfigurationException(ex.ToString() );

}

return bflag;

}

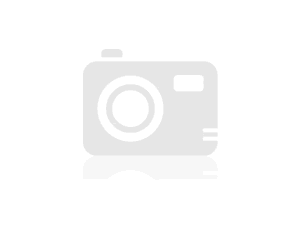
/**//// <summary>

/// Loops through the <code>appSettings</code> NameValueCollection

/// and recreates the XML nodes of the <appSettings> config

/// section accordingly. It saves the configuration file afterwards.

/// </summary>

private void SaveToFile()


{

if (appSettings != null)


{

try


{

XmlDocument configXml = new XmlDocument();

configXml.Load(ConfigFullName);

XmlNode appSettingsNode = configXml.SelectSingleNode("configuration/appSettings");

appSettingsNode.RemoveAll();

for (int i = 0; i < appSettings.Count; i++)


{

string key = appSettings.GetKey(i);

string val = appSettings.Get(i);

XmlNode node = configXml.CreateNode(XmlNodeType.Element, "add", "");

XmlAttribute attr = configXml.CreateAttribute("key");

attr.Value = key;

node.Attributes.Append(attr);

attr = configXml.CreateAttribute("value");

attr.Value = val;

node.Attributes.Append(attr);

appSettingsNode.AppendChild(node);

}

configXml.Save(ConfigFullName);

}

catch (Exception ex)


{

throw new ConfigurationException("Error while saving appSettings.", ex);

}

}

}

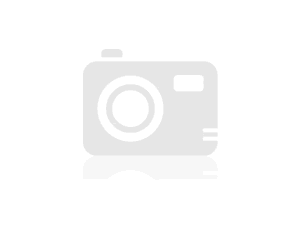
/**//// <summary>

/// 更新一条纪录

/// </summary>

/// <param name="strKey"></param>

/// <param name="strNewValue"></param>

/// <returns></returns>

public bool UpdateRecorder(string strKey,string strNewValue)


{

bool flag=true;

try


{

appSettings[strKey]=strNewValue;

SaveToFile();

}

catch(System.Exception err)


{

flag=false;

throw new Exception(err.ToString() ) ;

}

return flag;

}

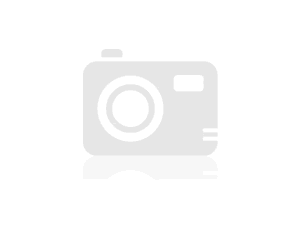
/**//// <summary>

/// 读取一个值

/// </summary>

/// <param name="strKey"></param>

/// <returns></returns>

public string ReadSettingsValue(string strKey)


{

LoadFromFile();

return appSettings[strKey].ToString();

}

public bool SaveDataGridChange(DataTable myTable)


{

bool flag=true;

myTable.AcceptChanges();

try


{

// Recreating the config.AppSettings collection with the data contained in appSettings DataGrid.

appSettings.Clear();

foreach (DataRow row in myTable.Rows)


{

appSettings[row["key"].ToString()] = row["value"].ToString();

}

// Saving the appSettings data into the configuration file.

SaveToFile();

}

catch(System.Exception err)


{

flag=false;

throw new Exception (err.ToString() );

}

return flag;

}
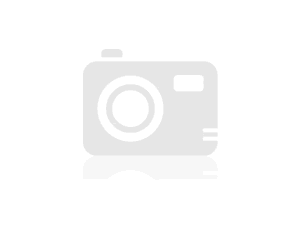

// private void getTableChange(DataTable table)#region// private void getTableChange(DataTable table)

// {

//

// string missingFields = "";

// StringCollection columnNames = new StringCollection();

// foreach (DataColumn col in table.Columns)

// {

//

// if (missingFields.Length == 0)

// missingFields = col.ColumnName;

// else

// missingFields += ", " + col.ColumnName;

//

// }

//

// if (missingFields.Length > 0)

// {

// throw new Exception("Unable to update nodes with datatable because the nodes are missing the fields: "+missingFields);

// }

//

// ///

// /// Remove nodes that got deleted from datatable

// ///

// DataTable currTable = table.GetChanges(DataRowState.Deleted);

// if (currTable != null)

// {

//

// Trace.WriteLine("Rows Deleted:");

// foreach (DataRow row in table.Rows)

// {

// string keyValue = row[0].ToString();

//

// Trace.WriteLine(keyValue);

//

// }

// }

//

// ///

// /// Update nodes with changes made on the datatable

// ///

// currTable = table.GetChanges(DataRowState.Modified);

// if (currTable != null)

// {

//

// Trace.WriteLine("Rows Changed:");

// foreach (DataRow row in currTable.Rows)

// {

//

// }

// }

//

// ///

// /// Add new nodes to match new rows added to datatable

// ///

// currTable = table.GetChanges(DataRowState.Added);

// if (currTable != null)

// {

//

// string keyValue;

//

// Trace.WriteLine("Rows Added:");

// foreach (DataRow row in currTable.Rows)

// {

//

// }

// }

// table.AcceptChanges();

//

//

// }

#endregion

}
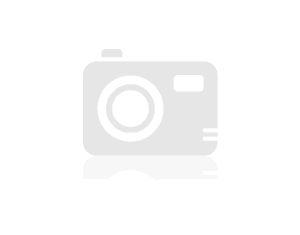
}
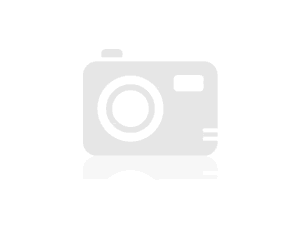
使用:
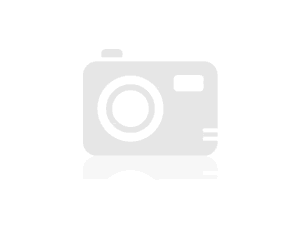
MrfuRWXmlFile mrfurw=new MrfuRWXmlFile();
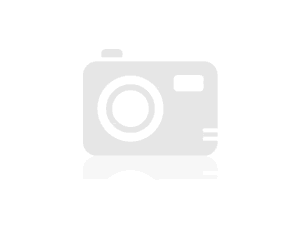
private void button_Create_Click(object sender, System.EventArgs e)
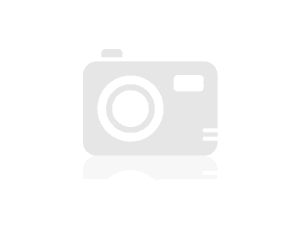

{

MrfuRWXmlFile.CreateXmlFile();

this.button_read.PerformClick();
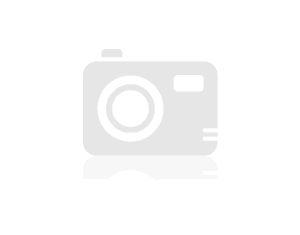
}
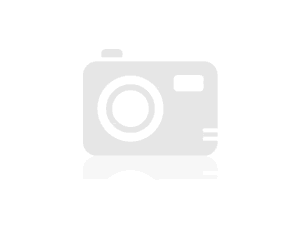
private void button_read_Click(object sender, System.EventArgs e)
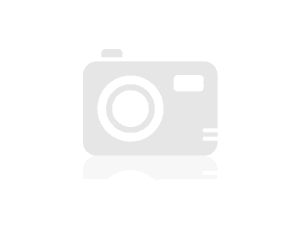

{

this.dataGrid1.DataSource=mrfurw.GetSettingsValue();
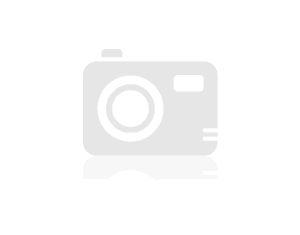
}
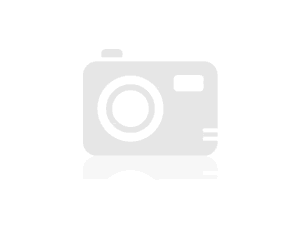
private void button_Update_Click(object sender, System.EventArgs e)
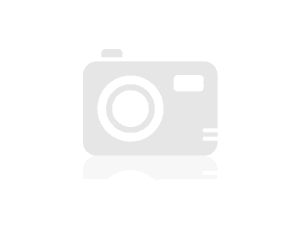

{

if(this.textBox_Value.Text.Trim()=="")

return;

mrfurw.UpdateRecorder("SqlStr0",this.textBox_Value.Text.Trim());

this.button_read.PerformClick();
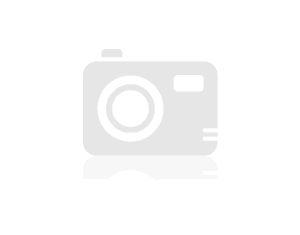
}
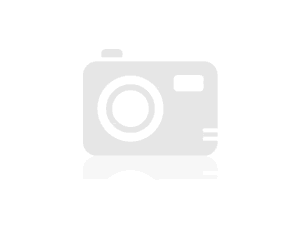
int i=0;
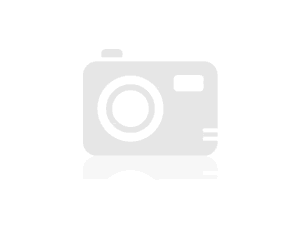
private void button_Add_Click(object sender, System.EventArgs e)
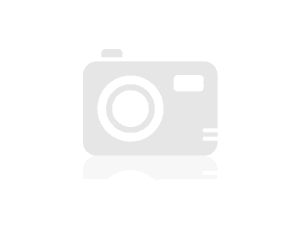

{

i+=1;

mrfurw.AddNewRecorder( System.DateTime.Now.ToString(),i.ToString());

this.button_read.PerformClick();
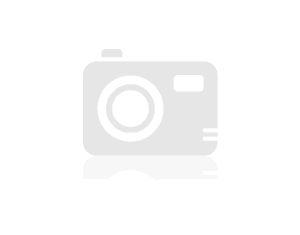
}
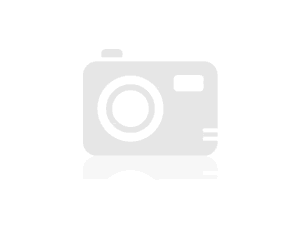
private void button1_Click(object sender, System.EventArgs e)
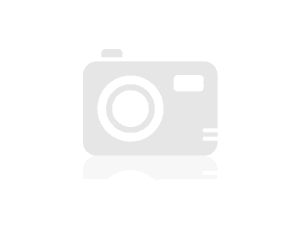

{

MessageBox.Show (mrfurw.ReadSettingsValue("SqlStr0" ));
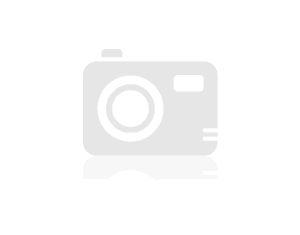
}
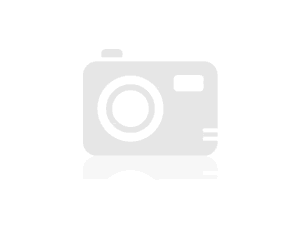
private void button2_Click(object sender, System.EventArgs e)
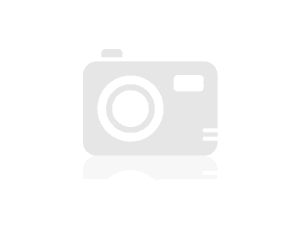

{

DataTable myTable=(DataTable)dataGrid1.DataSource;

mrfurw.SaveDataGridChange(myTable);
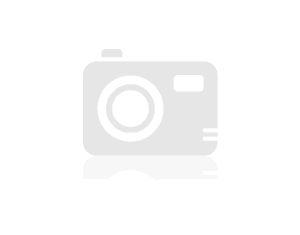
}