tt作曲家简谱打谱软件_掌握作曲家的技巧和窍门
tt作曲家简谱打谱软件
Composer has revolutionized package management in PHP. It upped the reusability game and helped PHP developers all over the world generate framework agnostic, fully shareable code. But few people ever go beyond the basics, so this post will cover some useful tips and tricks.
Composer彻底改变了PHP中的程序包管理。 它提高了可重用性游戏,并帮助全世界PHP开发人员生成了与框架无关的,可完全共享的代码。 但是很少有人会超越基础知识,因此本文将涵盖一些有用的技巧和窍门。
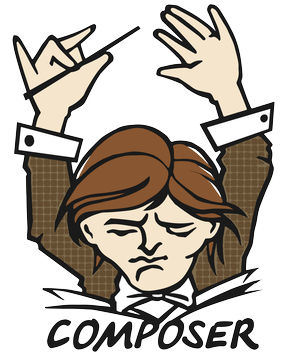
全球 (Global)
Although it’s clearly defined in the documentation, Composer can (and in most cases should) be installed globally. Global installation means that instead of typing out
尽管在文档中对其进行了明确定义,但Composer可以(并且在大多数情况下应该是)全局安装。 全局安装意味着无需输入
php composer.phar somecommand
you can just type out
你可以输入
composer somecommand
in any project whatsoever. This makes starting new projects with, for example, the create-project
command dead easy in any location on your filesystem.
在任何项目中。 这使得使用例如create-project
命令启动新项目在文件系统上的任何位置都很容易。
To install Composer globally, follow these instructions.
要全局安装Composer,请遵循以下说明 。
在里面 (Init)
To create a new composer.json
file in a project (and thus initialize a new Composer-powered project), you can use:
要在项目中创建一个新的composer.json
文件(从而初始化一个新的由Composer驱动的项目),可以使用:
composer init
You can also pass in some options as defaults.
您还可以将某些选项作为默认值传递。
正确安装软件包 (Installing packages the right way)
When reading tutorials or README files of projects, many will say something like:
阅读项目的教程或自述文件时,许多人会说:
Just add the following to your composer.json file:
{
"require": {
"myproject": "someversion"
}
}
But this has several downsides. One, the copy-pasting may introduce some errors. Two, for a newbie, figuring out where to place the code if you already have an extensive composer.json
file in your project can be tedious and also introduce errors. Finally, many people will be encountering Composer for the first time and in a command line, so covering all the use cases in which they may find themselves isn’t feasible (do they have a GUI text editor or are they on the command line? If it’s the latter, do they have a text editor installed, and if so which? Do you explain the editing procedure or just leave it? What if the file doesn’t exist in their projects? Should you cover the creation of the file, too?).
但这有几个缺点。 第一,复制粘贴可能会引入一些错误。 第二,对于一个新手来说,弄清楚如果您的项目中已经有一个广泛的composer.json
文件,那么将代码放置在何处可能会很麻烦,而且还会引入错误。 最后,许多人将是第一次在命令行中遇到Composer,因此涵盖所有可能发现自己的用例是不可行的(他们是否具有GUI文本编辑器,或者是否在命令行上?如果是后者,他们是否安装了文本编辑器,如果是的话,是什么呢?您解释编辑过程还是只保留它?如果文件在他们的项目中不存在该怎么办?您应该涵盖文件的创建,太?)。
The best way to add a new requirement to a composer.json
file is with the require command
:
向composer.json
文件添加新需求的最好方法是使用require command
:
composer require somepackage/somepackage:someversion
This adds everything that’s needed into the file, bypassing all manual intervention.
这会将所需的所有内容添加到文件中,从而绕过所有手动干预。
If you need to add packages to require-dev
, add the --dev
option, like so:
如果需要将软件包添加到require-dev
,请添加--dev
选项,如下所示:
composer require phpunit/phpunit --dev
The require
command supports adding several packages at once, just separate them with a space. Note that there is no need to specify the version in this approach, as seen in the code snippet above – installing a package this way automatically grabs the most recent version of a package, and tells you which one it picked.
require
命令支持一次添加多个软件包,只需用空格分隔即可。 请注意,无需使用这种方法来指定版本,如上面的代码片段所示–以这种方式安装软件包会自动获取软件包的最新版本,并告诉您选择了哪个版本。
锁定档案 (Lock Files)
The composer.lock
file saves the list of currently installed packages, so that when another person clones your project at a date when the dependencies may have been updated, they still get the old versions installed. This helps make sure everyone who grabs your project has the exact same package environment as you did when the project was developed, avoiding any bugs that may have been created due to version updates.
composer.lock
文件保存当前安装的软件包的列表,以便当其他人在依赖项可能已更新的日期克隆您的项目时,他们仍然会安装旧版本。 这有助于确保抓住项目的每个人都具有与开发项目时完全相同的程序包环境,避免了由于版本更新而造成的任何错误。
composer.lock
should almost always be committed to version control. Maybe.
composer.lock
应该几乎总是致力于版本控制。 也许吧 。
composer.lock
also contains the hash of the composer.json
file, so if you update just the project author, or some contact info, or a description, you’ll get a warning about the lock file not matching the json
file – when that’s the case, running composer update --lock
will help things, updating only the lock
file and not touching anything else.
composer.lock
还包含composer.json
文件的哈希,因此,如果您仅更新项目作者,某些联系信息或说明,则将收到有关锁文件与json
文件不匹配的警告。在这种情况下,运行composer update --lock
会有所帮助,仅更新lock
文件,而不接触其他任何东西。
版本标志 (Version flags)
When defining package versions, one can use exact matches (1.2.3
), ranges with operators (<1.2.3
), combinations of operators (>1.2.3 <1.3
), best available (1.2.*
), tilde (~1.2.3
) and caret (^1.2.3
).
定义软件包版本时 ,可以使用精确匹配( 1.2.3
),带运算符的范围( <1.2.3
),运算符的组合( >1.2.3 <1.3
),最佳( 1.2.*
)和波浪号( ~1.2.3
)和脱字号( ^1.2.3
)。
The latter two might warrant further explanation:
后两个可能需要进一步说明:
tilde (
~1.2.3
) will go up to version1.3
(not included), because in semantic versioning that’s when new features get introduced. Tilde fetches the highest known stable minor version. As the docs say, we can consider it as only the last digit specified being allowed to change.tilde(
~1.2.3
)将会升级到1.3
版(不包括在内),因为在语义版本控制中 ,是引入了新功能的时候。 Tilde获取已知的最高稳定次版本。 正如文档所说,我们可以将其视为仅允许更改的最后一位数字。caret (
^1.2.3
) means “only be careful of breaking changes”, and will thus go up to version2.0
. According to semver, that’s when breaking changes are introduced, so1.3
,1.4
and1.9
are fine, while2.0
is not.脱字号(
^1.2.3
)的意思是“仅注意打破更改”,因此将升级到2.0
。 据semver,被引入重大更改的时候,所以1.3
,1.4
和1.9
的罚款,而2.0
则不是。
Unless you know you need a specific version, I recommend always using the ~1.2.3
format – it’s your safest bet.
除非您知道需要特定版本,否则我建议始终使用~1.2.3
格式–这是您最安全的选择。
配置和全局配置 (Configuration and Global Configuration)
The default values are not fixed in stone. See the full config
reference for details.
默认值不是一成不变的。 有关详细信息,请参见完整的config
参考 。
For example, by specifying:
例如,通过指定:
{
"config": {
"optimize-autoloader": true
}
}
you force Composer to optimize the classmap after every installation/update, or in other words, whenever the autoload file is being generated. This is a little bit slower than generating the default autoloader, and slows down as the project grows.
您会在每次安装/更新后(或换句话说,每当生成自动加载文件时)强制Composer优化类映射。 这比生成默认的自动加载器要慢一些,并且随着项目的增长而降低。
Another useful option might be the cache-files-maxsize
– in enormous projects like eZ Publish or Symfony, the cache might get full pretty fast. Increasing the size would keep Composer fast longer.
另一个有用的选项可能是cache-files-maxsize
在eZ Publish或Symfony之类的庞大项目中,缓存可能很快就满了。 增加大小将使Composer保持更快的速度。
Note that configuration can be set globally, too, so it’s consistent across projects. See here for how. For example, to add the cache size setting to our global configuration, we either edit ~/.composer/config.json
or execute:
请注意,配置也可以全局设置,因此在项目之间是一致的。 有关详情,请参见此处 。 例如,要将缓存大小设置添加到我们的全局配置中,我们可以编辑~/.composer/config.json
或执行以下命令:
composer config --global cache-files-maxsize "2048MiB"
简介和详细 (Profile and Verbose)
You can add a --profile
flag to any command you execute on the command line with Composer, and it’ll produce not only a final output like this:
您可以在使用Composer在命令行上执行的任何命令中添加--profile
标志,它不仅会产生如下最终输出:
[174.6MB/54.70s] Memory usage: 174.58MB (peak: 513.47MB), time: 54.7s
but also prefix each line it outputs with the exact total duration of the command’s execution so far, plus the memory usage:
还要在其输出的每一行之前加上命令执行的确切总持续时间,再加上内存使用量:
[175.9MB/54.64s] Installing assets for Sensio\Bundle\DistributionBundle into web/bundles/sensiodistribution
I use this command often to identify the bottleneck packages and to observe how the stats improve or degrade on different versions of PHP.
我经常使用此命令来识别瓶颈软件包,并观察统计信息如何在不同版本PHP上改进或降低。
Likewise, the --verbose
flag will make sure Composer outputs more information with each operation it performs, helping you understand exactly what’s going on. Some people have even aliased their composer
command to include composer --verbose --profile
by default.
同样,-- --verbose
标志将确保Composer对其执行的每个操作输出更多信息,从而帮助您准确了解正在发生的事情。 甚至有人默认使用composer --verbose --profile
作为别名的composer
命令。
自订来源 (Custom Sources)
Sometimes, you just want to install from a Github repo if your project isn’t yet on Packagist. Maybe it’s under development, maybe it’s locally hosted, who knows. To do that, see our guide.
有时,如果您的项目尚未安装在Packagist上,则只想从Github存储库进行安装。 谁知道呢,也许它正在开发中,或者它是本地托管的。 为此,请参见我们的指南 。
Likewise, if you have your own version of a popular project that another part of your project depends on, you can use custom sources in combination with inline aliasing to fake the version constraint like Matthieu Napoli did here.
同样,如果您拥有自己的流行项目的版本,而该项目的另一部分依赖于该版本,则可以结合使用自定义源和内联别名来伪造版本约束,就像Matthieu Napoli 在此处所做的那样 。
加快作曲家的速度 (Speeding up Composer)
As per this excellent trick by Mark Van Eijk, you can speed up Composer’s execution by making it run on HHVM.
按照Mark Van Eijk的出色技巧,可以通过使Composer在HHVM上运行来加快其执行速度。
Another way is forcing it to use --prefer-dist
which downloads a stable, packaged version of a project rather than cloning it from the version control system it’s on (much slower). This is on by default, though, so you shouldn’t need to specify it on stable projects. If you want to download the sources, use the --prefer-source
flag. More info about this in the options of the install
command here.
另一种方法是强制它使用--prefer-dist
,它会下载项目的稳定,打包版本,而不是从项目所在的版本控制系统中克隆它(速度要慢得多)。 不过,默认情况下此功能处于启用状态,因此您无需在稳定的项目中指定它。 如果要下载源,请使用--prefer-source
标志。 有关此内容的选项更多信息install
命令在这里 。
使您的Composer项目更轻便 (Making your Composer project lighter)
If you’re someone who develops Composer-friendly projects, you might want to do your part, too. Based on this Reddit thread, you can use a .gitattributes
file to ignore some of the files and folders during packaging for the --prefer-dist
mode above.
如果您是开发对Composer友好的项目的人,那么您可能也想尽自己的一份力量。 基于此Reddit线程 ,您可以使用.gitattributes
文件在上述--prefer-dist
模式的打包过程中忽略某些文件和文件夹。
/docs export-ignore
/tests export-ignore
/.gitattributes export-ignore
/.gitignore export-ignore
/.travis.yml export-ignore
/phpunit.xml export-ignore
How does this work? When you upload a project to Github, it automatically makes available the “Download zip” button which you can use to download an archive of your project. What’s more, Packagist uses these auto-generated archives to pull in the --prefer-dist
dependencies, and then unarchives them once downloaded (much faster than cloning). If you thus ignore your tests, docs and other logically irrelevant files by listing them in .gitattributes
, the archives won’t contain them, becoming much, much lighter.
这是如何运作的? 当您将项目上传到Github时,它会自动使“下载zip”按钮可用,您可以使用该按钮下载项目的存档。 更重要的是,Packagist使用这些自动生成的存档来提取--prefer-dist
依赖关系,然后在下载后取消存档(比克隆要快得多)。 如果您因此通过将它们列出在.gitattributes
而忽略了它们的测试,文档和其他与逻辑无关的文件,则归档将不包含它们,从而使它们变得轻巧得多。
Naturally, people who want to debug your library or run its tests should then specify the --prefer-source
flag.
自然, 想要调试您的库或运行其测试的人应该然后指定--prefer-source
标志。
The PhpLeague has adopted this approach and included it in their Package skeleton, so any project based on that is automatically “dist friendly”.
PhpLeague已采用此方法并将其包含在其Package框架中 ,因此基于此的任何项目都将自动“远程友好”。
表演 (Show)
If you ever forget what version of PHP or its extensions you’re running, or need a list of all the projects (and their descriptions) that you’ve installed inside the current project and their versions, you can use the show
command with the --platform
(short -p
) and --installed
(short -i
) flags respectively:
如果您忘记了正在运行PHP版本或其扩展名,或者需要列出当前项目及其版本中已安装的所有项目(及其说明)的列表,可以将show
命令与--platform
(short -p
)和--installed
(short -i
)标志:
$ composer show --installed
behat/behat v3.0.15 Scenario-oriented BDD framework for PHP 5.3
behat/gherkin v4.3.0 Gherkin DSL parser for PHP 5.3
behat/mink v1.5.0 Web acceptance testing framework for PHP 5.3
behat/mink-browserkit-driver v1.1.0 Symfony2 BrowserKit driver for Mink framework
behat/mink-extension v2.0.1 Mink extension for Behat
behat/mink-goutte-driver v1.0.9 Goutte driver for Mink framework
behat/mink-sahi-driver v1.1.0 Sahi.JS driver for Mink framework
behat/mink-selenium2-driver v1.1.1 Selenium2 (WebDriver) driver for Mink framework
behat/sahi-client dev-master ce7bfa7 Sahi.js client for PHP 5.3
behat/symfony2-extension v2.0.0 Symfony2 framework extension for Behat
behat/transliterator v1.0.1 String transliterator
components/bootstrap 3.3.2 The most popular front-end framework for developing responsive, mobile first projects on the web.
components/jquery 2.1.3 jQuery JavaScript Library
doctrine/annotations v1.2.4 Docblock Annotations Parser
doctrine/cache v1.4.1 Caching library offering an object-oriented API for many cache backends
doctrine/collections v1.3.0 Collections Abstraction library
doctrine/common v2.5.0 Common Library for Doctrine projects
doctrine/dbal v2.5.1 Database Abstraction Layer
doctrine/doctrine-bundle v1.4.0 Symfony DoctrineBundle
doctrine/doctrine-cache-bundle v1.0.1 Symfony2 Bundle for Doctrine Cache
doctrine/inflector v1.0.1 Common String Manipulations with regard to casing and singular/plural rules.
doctrine/instantiator 1.0.4 A small, lightweight utility to instantiate objects in PHP without invoking their constructors
doctrine/lexer v1.0.1 Base library for a lexer that can be used in Top-Down, Recursive Descent Parsers.
egulias/listeners-debug-command-bundle 1.9.1 Symfony 2 console command to debug listeners
ezsystems/behatbundle dev-master bd95e1b Behat bundle for help testing eZ Bundles and projects
ezsystems/comments-bundle dev-master 8f95bc7 Commenting system for eZ Publish
ezsystems/demobundle dev-master c13fb0b Demo bundle for eZ Publish Platform
ezsystems/demobundle-data v0.1.0 Data for ezsystems/demobundle
ezsystems/ezpublish-kernel dev-master 3d6e48d eZ Publish API and kernel. This is the heart of eZ Publish 5.
ezsystems/platform-ui-assets-bundle v0.5.0 External assets dependencies for PlatformUIBundle
ezsystems/platform-ui-bundle dev-master 4d0442d eZ Platform UI Bundle
ezsystems/privacy-cookie-bundle v0.1 Privacy cookie banner integration bundle into eZ Publish/eZ Platform
fabpot/goutte v1.0.7 A simple PHP Web Scraper
friendsofsymfony/http-cache 1.3.1 Tools to manage cache invalidation
friendsofsymfony/http-cache-bundle 1.2.1 Set path based HTTP cache headers and send invalidation requests to your HTTP cache
guzzle/guzzle v3.9.3 PHP HTTP client. This library is deprecated in favor of https://packagist.org/packages/guzzlehttp/guzzle
hautelook/templated-uri-bundle 2.0.0 Symfony2 Bundle that provides a RFC-6570 compatible router and URL Generator.
hautelook/templated-uri-router 2.0.1 Symfony2 RFC-6570 compatible router and URL Generator
imagine/imagine 0.6.2 Image processing for PHP 5.3
incenteev/composer-parameter-handler v2.1.0 Composer script handling your ignored parameter file
instaclick/php-webdriver 1.0.17 PHP WebDriver for Selenium 2
jdorn/sql-formatter v1.2.17 a PHP SQL highlighting library
knplabs/knp-menu v1.1.2 An object oriented menu library
knplabs/knp-menu-bundle v1.1.2 This bundle provides an integration of the KnpMenu library
kriswallsmith/assetic v1.2.1 Asset Management for PHP
kriswallsmith/buzz v0.13 Lightweight HTTP client
league/flysystem 0.5.12 Many filesystems, one API.
liip/imagine-bundle 1.2.6 This Bundle assists in imagine manipulation using the imagine library
monolog/monolog 1.13.1 Sends your logs to files, sockets, inboxes, databases and various web services
nelmio/cors-bundle 1.3.3 Adds CORS (Cross-Origin Resource Sharing) headers support in your Symfony2 application
ocramius/proxy-manager 0.5.2 A library providing utilities to generate, instantiate and generally operate with Object Proxies
oneup/flysystem-bundle v0.4.2 Integrates Flysystem filesystem abstraction library to your Symfony2 project.
pagerfanta/pagerfanta v1.0.3 Pagination for PHP 5.3
phpdocumentor/reflection-docblock 2.0.4
phpspec/prophecy v1.4.1 Highly opinionated mocking framework for PHP 5.3+
phpunit/php-code-coverage 2.0.16 Library that provides collection, processing, and rendering functionality for PHP code coverage information.
phpunit/php-file-iterator 1.4.0 FilterIterator implementation that filters files based on a list of suffixes.
phpunit/php-text-template 1.2.0 Simple template engine.
phpunit/php-timer 1.0.5 Utility class for timing
phpunit/php-token-stream 1.4.1 Wrapper around PHP's tokenizer extension.
phpunit/phpunit 4.6.4 The PHP Unit Testing framework.
phpunit/phpunit-mock-objects 2.3.1 Mock Object library for PHPUnit
psr/log 1.0.0 Common interface for logging libraries
qafoo/rmf 1.0.0 Very simple VC framework which makes it easy to build HTTP applications / REST webservices
sebastian/comparator 1.1.1 Provides the functionality to compare PHP values for equality
sebastian/diff 1.3.0 Diff implementation
sebastian/environment 1.2.2 Provides functionality to handle HHVM/PHP environments
sebastian/exporter 1.2.0 Provides the functionality to export PHP variables for visualization
sebastian/global-state 1.0.0 Snapshotting of global state
sebastian/recursion-context 1.0.0 Provides functionality to recursively process PHP variables
sebastian/version 1.0.5 Library that helps with managing the version number of Git-hosted PHP projects
sensio/distribution-bundle v3.0.21 Base bundle for Symfony Distributions
sensio/framework-extra-bundle v3.0.7 This bundle provides a way to configure your controllers with annotations
sensio/generator-bundle v2.5.3 This bundle generates code for you
sensiolabs/security-checker v2.0.2 A security checker for your composer.lock
swiftmailer/swiftmailer v5.4.0 Swiftmailer, free feature-rich PHP mailer
symfony-cmf/routing 1.3.0 Extends the Symfony2 routing component for dynamic routes and chaining several routers
symfony/assetic-bundle v2.6.1 Integrates Assetic into Symfony2
symfony/monolog-bundle v2.7.1 Symfony MonologBundle
symfony/swiftmailer-bundle v2.3.8 Symfony SwiftmailerBundle
symfony/symfony v2.6.6 The Symfony PHP framework
tedivm/stash v0.12.3 The place to keep your cache.
tedivm/stash-bundle v0.4.2 Incorporates the Stash caching library into Symfony.
twig/extensions v1.2.0 Common additional features for Twig that do not directly belong in core
twig/twig v1.18.1 Twig, the flexible, fast, and secure template language for PHP
white-october/pagerfanta-bundle v1.0.2 Bundle to use Pagerfanta with Symfony2
whiteoctober/breadcrumbs-bundle 1.0.2 A small breadcrumbs bundle for Symfony2
zendframework/zend-code 2.2.10 provides facilities to generate arbitrary code using an object oriented interface
zendframework/zend-eventmanager 2.2.10
zendframework/zend-stdlib 2.2.10
zetacomponents/base 1.9 The Base package provides the basic infrastructure that all packages rely on. Therefore every component relies on this package.
zetacomponents/feed 1.4 This component handles parsing and creating RSS1, RSS2 and ATOM feeds, with support for different feed modules (dc, content, creativeCommons, geo, iTunes).
zetacomponents/mail 1.8.1 The component allows you construct and/or parse Mail messages conforming to the mail standard. It has support for attachments, multipart messages and HTML mail. It also interfaces with SMTP to send mail or IMAP, P...
zetacomponents/system-information 1.1 Provides access to common system variables, such as CPU type and speed, and the available amount of memory.
空跑 (Dry Runs)
To just see if an installation of new requirements would go well, you can use the --dry-run
flag with Composer’s install
and update
command. This will throw all the potential problems at you, without actually causing them – no changes will really be made. Excellent for testing big requirement and setup changes before actually committing to them.
要仅查看新要求的安装是否顺利,可以在Composer的install
和update
命令中使用--dry-run
标志。 这将把所有潜在问题扔给您,而不会真正引起它们-不会真正进行更改。 非常适合测试大型需求并在实际提交之前进行设置更改。
composer update --dry-run --profile --verbose
建立专案 (Create Project)
Last but not least, we must mention the create-project
command, applicable to anything and everything.
最后但并非最不重要的一点是,我们必须提到适用于所有事物的create-project
命令 。
Create project takes a package name as the argument, then clones the package and executes composer install
inside it. This is fantastic for bootstrapping projects – no more finding out the exact Github URL of the package you want, then cloning, then manually going into the folder and executing install
.
Create project将软件包名称作为参数,然后克隆该软件包并在其中执行composer install
。 这对于引导项目非常有用–无需再查找所需软件包的确切Github URL,然后克隆,然后手动进入文件夹并执行install
。
Major projects such as Symfony and Laravel use this approach to bootstrap a skeleton application, and many others are jumping on board.
Symfony和Laravel等主要项目都使用这种方法来引导框架应用程序,许多其他项目也正在加入。
With Laravel, for example, it’s used like this:
以Laravel为例,它的用法如下:
composer create-project laravel/laravel --prefer-dist --profile --verbose
The create-project
command also accepts two parameters. The first is the path into which to install. If omitted, the project’s name is used. The second is the version. If omitted, the latest version is used.
create-project
命令还接受两个参数。 第一个是安装路径。 如果省略,则使用项目的名称。 第二个是版本。 如果省略,则使用最新版本。
结论 (Conclusion)
Hope this list of tips and tricks has been helpful! If we missed some, do tell us and we’ll update the post! And remember – if you forget about some of the commands or switches, just check out the cheatsheet. Happy Composing!
希望此提示和技巧列表对您有所帮助! 如果我们错过了一些,请告诉我们,我们将更新帖子! 记住-如果您忘记了某些命令或开关,只需查看备忘单 。 快乐作曲!
翻译自: https://www.sitepoint.com/mastering-composer-tips-tricks/
tt作曲家简谱打谱软件
tt作曲家简谱打谱软件_掌握作曲家的技巧和窍门相关推荐
- tt作曲家简谱打谱软件_作曲家入门指南
tt作曲家简谱打谱软件 Since its release on March 1st, 2012 Composer has gained widespread popularity for bring ...
- tt作曲家简谱打谱软件_作曲家全球需求被认为有害吗?
tt作曲家简谱打谱软件 We've discussed Composer best practices before, and I've always advocated using composer ...
- vi和vt的区别小窍门_十大vi技巧和窍门
vi和vt的区别小窍门 vi编辑器是Unix和类似Unix的系统(例如Linux)上最受欢迎的文本编辑器之一. 无论您是初次接触vi还是只是想复习一下,这11个技巧都将增强您的使用方式. 编辑中 编辑 ...
- kaggle比赛数据_表格数据二进制分类:来自5个Kaggle比赛的所有技巧和窍门
kaggle比赛数据 This article was originally written by Shahul ES and posted on the Neptune blog. 本文最初由 Sh ...
- html多张图片组合成一张,如何把多张图片快速拼接组合到一起成一张_微信图文排版技巧?...
原标题:如何把多张图片快速拼接组合到一起成一张_微信图文排版技巧? 如何把多张图片快速拼接组合到一起成一张_微信图文排版技巧? 微信公众号图文编辑排版技巧又开课了,这次跟大家分享一个排版技巧,多张照片 ...
- 简谱打谱软件音乐梦想家与作曲大师有什么不同
最近小编了解到,很多作曲大师的老用户对音乐梦想家软件不了解.下面小编就和大家讲一下音乐梦想家就和作曲大师究竟有什么不同. 音乐梦想家与作曲大师其实是同一款软件,但是音乐梦想家是作曲大师的升级版本,在功 ...
- figma下载_在Figma中进行原型制作的技巧和窍门
figma下载 自定义过渡和微交互 (Custom transitions and micro-interactions) Yep, I know that there are a lot of us ...
- sql优化技巧_使用这些查询优化技巧成为SQL向导
sql优化技巧 成为SQL向导! (Become an SQL Wizard!) It turns out storing data by rows and columns is convenient ...
- cmd指令大全指令_数控加工中心编程技巧及指令大全,请转给需要的数控人!
大家好,人生最可贵的两个词,一个叫认真,一个叫坚持,认真的人改变了自己,坚持的人改变了命运.有些事情,不是看到了希望才去坚持,而是坚持了才有希望.如果你相信我,我有能力,有实力把你教会.只要你想学习, ...
最新文章
- GPU、AI芯片技术市场分析
- 永远不要在 MySQL 中使用“utf8”
- CUBA在查询语句如何添加参数
- pandas 排序一个变量
- c# asp.net mvc 开发的正方教务助手(一)
- n个字符串按照字典序排列
- IDEA Tomcat Catalina Log出现乱码
- wince手机投屏代码_除了 iOS,这些手机系统你肯定没用过
- php程序控制结构,PHP 控制结构
- Codeblocks 中文乱码解决方法
- 设计模式——单例模式(饿汉式、懒汉式和DCL)
- 计算机教室戴尔电脑网络同传,DELL商用台式电脑如何作网络同传
- 一级计算机基础试题答案,计算机一级计算机基础试题及答案
- 互联网行业的颜值担当,李彦宏!
- 华创期货恒生网上交易
- 把烂土豆砸到古永锵的脸上
- android 设置应用权限管理,几种安卓权限管理应用介绍
- 深度学习用于股票预测_用于自动股票交易的深度强化学习
- 减治法(Decrease and Conquer)
- 零基础学习C语言的第一天