UVa 10806 Dijkstra,Dijkstra(最小费用最大流)
裸的费用流.往返就相当于从起点走两条路到终点. 按题意建图,将距离设为费用,流量设为1.然后增加2个点,一个连向节点1,流量=2,费用=0;结点n连一条同样的弧,然后求解最小费用最大流.当且仅当最大流=2时,有solution,此时费用即answer.
--------------------------------------------------------------------------------
--------------------------------------------------------------------------------
Time Limit: 3000MS | Memory Limit: Unknown | 64bit IO Format: %lld & %llu |
Submit Status
Description
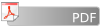
Dijkstra, Dijkstra.
Time Limit: 10 seconds
Dexter: "You don't understand. I can't walk... they've tied my shoelaces together." Topper Harley: "A knot. Bastards!" |
Jim Abrahams and Pat Proft,
"Hot Shots! Part Deux."
You are a political prisoner in jail. Things are looking grim, but fortunately, your jailmate has come up with an escape plan. He has found a way for both of you to get out of the cell and run through the city to the train station, where you will leave the country. Your friend will escape first and run along the streets of the city to the train station. He will then call you from there on your cellphone (which somebody smuggled in to you inside a cake), and you will start to run to the same train station. When you meet your friend there, you will both board a train and be on your way to freedom.
Your friend will be running along the streets during the day, wearing his jail clothes, so people will notice. This is why you can not follow any of the same streets that your friend follows - the authorities may be waiting for you there. You have to pick a completely different path (although you may run across the same intersections as your friend).
What is the earliest time at which you and your friend can board a train?
Problem, in short
Given a weighed, undirected graph, find the shortest path from S to T and back without using the same edge twice.
Input
The input will contain several test cases. Each test case will begin with an integer n (2<=n<=100) - the number of nodes (intersections). The jail is at node number 1, and the train station is at node number n. The next line will contain an integer m - the number of streets. The next m lines will describe the m streets. Each line will contain 3 integers - the two nodes connected by the street and the time it takes to run the length of the street (in seconds). No street will be longer than 1000 or shorter than 1. Each street will connect two different nodes. No pair of nodes will be directly connected by more than one street. The last test case will be followed by a line containing zero.
Output
For each test case, output a single integer on a line by itself - the number of seconds you and your friend need between the time he leaves the jail cell and the time both of you board the train. (Assume that you do not need to wait for the train - they leave every second.) If there is no solution, print "Back to jail".
Sample Input | Sample Output |
2 1 1 2 999 3 3 1 3 10 2 1 20 3 2 50 9 12 1 2 10 1 3 10 1 4 10 2 5 10 3 5 10 4 5 10 5 7 10 6 7 10 7 8 10 6 9 10 7 9 10 8 9 10 0 |
Back to jail 80 Back to jail |
转载于:https://www.cnblogs.com/JSZX11556/p/4384962.html
UVa 10806 Dijkstra,Dijkstra(最小费用最大流)相关推荐
- 使用dijkstra求解最小费用最大流网络
前言 在介绍如何使用dijkstra算法求解最小费用最大流问题的时候,假设看这篇博文的读者已经知道什么是最小费用最大流问题及熟悉dijkstra单源最短路径算法.在这篇博文里面,我并不会过多强调网络拓 ...
- 【最小费用最大流(改进Dijkstra)】2016 icpc 青岛 G - Coding Contest
题目:https://vjudge.net/contest/412116#problem/G 题意:t组样例,n个点,每个点有sis_isi个人和bib_ibi份物资.m条边,每条边从第二次开始, ...
- c语言最小费用流_策略算法工程师之路-图优化算法(一)(二分图amp;最小费用最大流)...
目录 1.图的基本定义 2.双边匹配问题 2.1 二分图基本概念 2.2 二分图最大匹配求解 2.3 二分图最优匹配求解 2.4 二分图最优匹配建模实例 2.4.1 二分图最优匹配在师生匹配中的应用 ...
- 最大流最小费用java_最小费用最大流及算法
最大流的网络,可看作为辅送一般货物的运输网络,此时,最大流问题仅表明运输网络运输货物的能力,但没有考虑运送货物的费用.在实际问题中,运送同样数量货物的运输方案可能有多个,因此从中找一个输出费用最小的的 ...
- 最大流,最小费用最大流:解析 + 各种板子
网络流初步 + Edmond-Karp算法 网络流的基本概念 源点,这个点只有流量的流出,没有流入. 汇点,这个点只有流量的流入,没有流出. 容量,每条有向边的最大可承受的流的理论大小. 流量,每条有 ...
- 经典网络流题目模板(P3376 + P2756 + P3381 : 最大流 + 二分图匹配 + 最小费用最大流)...
题目来源 P3376 [模板]网络最大流 P2756 飞行员配对方案问题 P3381 [模板]最小费用最大流 最大流 最大流问题是网络流的经典类型之一,用处广泛,个人认为网络流问题最具特点的操作就是建 ...
- P4043 [AHOI2014/JSOI2014]支线剧情(有源汇上下界最小费用可行流)
传送门 约束每个点至少要经过一次,因此是上下界网络流. 每经过边都需要相应的边权,且要求耗费边权之和最小,因此是最小费用流. 存在多个终点,需要建立汇点 ttt ,因此是有源汇网络流. 即:有源汇上下 ...
- 中转站有容量限制的运输问题(最小费用最大流)
初学,如有问题欢迎交流~ 问题详述 模型假设 线性规划 线性规划模型建立 线性规划模型求解 中转站增加容量限制 网络流 最小费用最大流模型建立 最小费用最大流模型求解 整体算法设计 贝尔曼-福特算法( ...
- 【图论】最小费用最大流(网络流进阶)
本文 前置基础:最大流问题(Dinic算法) && 单源最短路径(SPFA算法) 洛谷 P3381 [模板]最小费用最大流 所谓最小费用最大流,其实就是在最大流问题的基础上,再给边加上 ...
最新文章
- mxnet is not presented
- 初学web开发需要掌握哪些方面?
- SAP CRM text Transfer mode
- mysql基础表和修理表_MySQL基础知识——创建数据库和表
- postgresql主从备份_基于windows平台的postgresql主从数据库流备份配置
- Eclipse中要导出jar包中引用了第三方jar包怎么办
- 编译性语言、解释性语言和脚本语言
- ssh (安全外壳协议)Secure Shell 百度百科
- Linux进程管理:上帝视角看进程调度
- matlab生成不重复的随机数_怎么生成不重复随机数——《超级处理器》应用
- activiti mysql_基于MySQL的Activiti6引擎创建
- centos 6 编译emacs-24.5
- 微信小程序项目实践 项目范围及开发计划
- vue将文件/图片/视频批量打包成压缩包,并进行下载
- 计算机sci检索,计算机方向国内EI检索、SCI检索的期刊目录
- 【微处理器】基于FPGA的微处理器VHDL开发
- 74HC165基础篇(一)
- 七.getchar 和 scanf
- 运行内存数据加密加密
- illustrate插件--AI插件--印前插件--CADTools--导出表分析--界面检测(二)