python利用opencv标注bounding box
http://blog.csdn.net/xieqiaokang/article/details/60780608
1. 函数
用 OpenCV 标注 bounding box 主要用到下面两个工具——cv2.rectangle() 和 cv2.putText()。用法如下:
# cv2.rectangle()
# 输入参数分别为图像、左上角坐标、右下角坐标、颜色数组、粗细
cv2.rectangle(img, (x,y), (x+w,y+h), (B,G,R), Thickness)# cv2.putText()
# 输入参数为图像、文本、位置、字体、大小、颜色数组、粗细
cv2.putText(img, text, (x,y), Font, Size, (B,G,R), Thickness)
- 1
- 2
- 3
- 4
- 5
- 6
- 7
2. Example
import cv2fname = '001.jpg'
img = cv2.imread(fname)
# 画矩形框
cv2.rectangle(img, (212,317), (290,436), (0,255,0), 4)
# 标注文本
font = cv2.FONT_HERSHEY_SUPLEX
text = '001'
cv2.putText(img, text, (212, 310), font, 2, (0,0,255), 1)
cv2.imwrite('001_new.jpg', img)
documents:
https://docs.opencv.org/3.1.0/dc/da5/tutorial_py_drawing_functions.html
Goal
- Learn to draw different geometric shapes with OpenCV
- You will learn these functions : cv2.line(), cv2.circle() , cv2.rectangle(), cv2.ellipse(), cv2.putText() etc.
Code
In all the above functions, you will see some common arguments as given below:
- img : The image where you want to draw the shapes
- color : Color of the shape. for BGR, pass it as a tuple, eg: (255,0,0) for blue. For grayscale, just pass the scalar value.
- thickness : Thickness of the line or circle etc. If **-1** is passed for closed figures like circles, it will fill the shape. default thickness = 1
- lineType : Type of line, whether 8-connected, anti-aliased line etc. By default, it is 8-connected. cv2.LINE_AA gives anti-aliased line which looks great for curves.
Drawing Line
To draw a line, you need to pass starting and ending coordinates of line. We will create a black image and draw a blue line on it from top-left to bottom-right corners.
Drawing Rectangle
To draw a rectangle, you need top-left corner and bottom-right corner of rectangle. This time we will draw a green rectangle at the top-right corner of image.
Drawing Circle
To draw a circle, you need its center coordinates and radius. We will draw a circle inside the rectangle drawn above.
Drawing Ellipse
To draw the ellipse, we need to pass several arguments. One argument is the center location (x,y). Next argument is axes lengths (major axis length, minor axis length). angle is the angle of rotation of ellipse in anti-clockwise direction. startAngle and endAngle denotes the starting and ending of ellipse arc measured in clockwise direction from major axis. i.e. giving values 0 and 360 gives the full ellipse. For more details, check the documentation of cv2.ellipse(). Below example draws a half ellipse at the center of the image.
Drawing Polygon
To draw a polygon, first you need coordinates of vertices. Make those points into an array of shape ROWSx1x2 where ROWS are number of vertices and it should be of type int32. Here we draw a small polygon of with four vertices in yellow color.
- Note
- If third argument is False, you will get a polylines joining all the points, not a closed shape.
- cv2.polylines() can be used to draw multiple lines. Just create a list of all the lines you want to draw and pass it to the function. All lines will be drawn individually. It is a much better and faster way to draw a group of lines than calling cv2.line() for each line.
Adding Text to Images:
To put texts in images, you need specify following things.
- Text data that you want to write
- Position coordinates of where you want put it (i.e. bottom-left corner where data starts).
- Font type (Check cv2.putText() docs for supported fonts)
- Font Scale (specifies the size of font)
- regular things like color, thickness, lineType etc. For better look, lineType = cv2.LINE_AA is recommended.
We will write OpenCV on our image in white color.
Result
So it is time to see the final result of our drawing. As you studied in previous articles, display the image to see it.
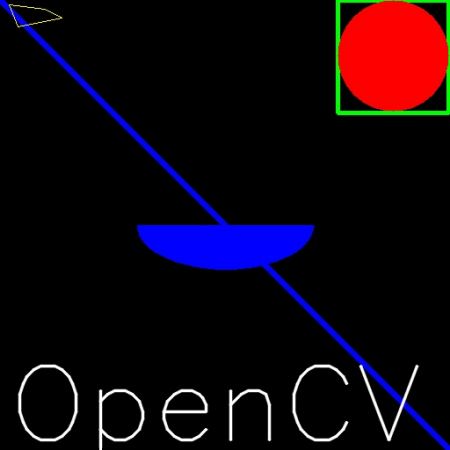
Additional Resources
- The angles used in ellipse function is not our circular angles. For more details, visit this discussion.
Exercises
- Try to create the logo of OpenCV using drawing functions available in OpenCV.
python利用opencv标注bounding box相关推荐
- python利用opencv进行相机标定获取参数,并根据畸变参数修正图像附有全部代码(流畅无痛版)
python利用opencv进行相机标定获取参数,并根据畸变参数修正图像附有全部代码 一.前言 今天的低价单孔摄像机(照相机)会给图像带来很多畸变.畸变主要有两 种:径向畸变和切想畸变.如下图所示,用 ...
- AI 质检学习报告——实践篇——第三步:python利用OpenCV打开摄像头截图后实现图片识字
AI 质检学习报告--实践篇--第一步:python利用OpenCV打开摄像头并截图 AI 质检学习报告--实践篇--第二步:实现图片识字 前边两篇实践已经分别实现了利用OpenCV打开摄像头并截图和 ...
- AI 质检学习报告——实践篇——第一步:python利用OpenCV打开摄像头并截图
写在前边 想要做一个AI质检的项目,一点一点来,首先:python利用OpenCV打开摄像头并拍照. 效果 设摄像头像素不咋地,实际的产品肯定不会是这个像素,必须安排一个专业的. 实现代码 impor ...
- python利用opencv去除图片logo_利用python和opencv批量去掉图片黑边
import os import cv2 import numpy as np from scipy.stats import mode import time import concurrent.f ...
- python利用opencv去除图片logo_python 基于opencv去除图片阴影
一.前言 如果你自己打印过东西,应该有过这种经历.如果用自己拍的图片,在手机上看感觉还是清晰可见,但是一打印出来就是漆黑一片.比如下面这两张图片: 因为左边的图片有大片阴影,所以打印出来的图片不堪入目 ...
- 基于Python利用OpenCV实现Hough变换的形状检测
点击上方"小白学视觉",选择加"星标"或"置顶" 重磅干货,第一时间送达 今天我们将学习如何借助霍夫变换技术来检测图像中的直线和圆. 什么是 ...
- Python利用OpenCV去除图片水印
点击上方"何俊林",马上关注,每天早上8:50准时推送 真爱,请置顶或星标 这两天公司来了一个新的需求--去除水印,对于我一个从未接触过的这种事情的人来说,当时我是蒙的.不过首先我 ...
- python利用opencv进识别行红绿灯
目录 一.使用步骤 1.引入库 2.读入数据 3.获取帧率 4.创建函数将鼠标在图片中点击的坐标储存 5.逐帧采集视频并显示一帧图片来选择roi区域 6.最后逐帧采集视频并且放入循环一帧一帧的判断感兴 ...
- python利用opencv自带的颜色查找表(LUT)进行色彩风格变换
1 LUT颜色查找表介绍 Look Up Table(LUT)查找表 1.颜色查找表就是一种像素值映射的表,如下是一个对比度改变图像的查找表,从图中可以知道: 原图中像素值为40的像素,经过查找表映射 ...
最新文章
- java程序中的图片与数值关联_Java从图片中读取图片的元数据Exif信息
- MySql 踩坑小记
- Bit-Z 项目评审中心开启首次投票币竞选
- github推送错误:已经有此代码,不允许覆盖的解决方法
- 批量处理文件,除了 Python,不妨试试 VIM!
- C++中的抽象类以及接口的区别联系
- P1368-工艺【最小表示法】
- 【C语言】数据结构C语言版 实验3 带头结点的单链表
- 使用JWT的ASP.NET CORE令牌身份验证和授权(无Cookie)——第2部分
- java 输出视频文件格式_java – 如何从各种视频文件格式中提取元数据?
- Flex RIA的ArcIMS WebGIS之路(一)--胸中的那棵竹
- 震惊,CSDN居然可以打出爱心?
- ehcache缓存原理_Mybatis-09-缓存
- TechNet Magazine/TechNet杂志
- 转:基于Spark的电影推荐系统(包含爬虫项目、web网站、后台管理系统以及spark推荐系统)
- windows server 2008 远程终端激活
- vscode修改背景
- 使用tornado的异步非阻塞
- 充电桩(charging station)是什么?
- 昆石VOS3000/VOS2009 Web手机管理说明
热门文章
- 【C语言简单说】三:整数变量和输出扩展(2)
- 安卓模拟器获取服务器信息出错,安卓模拟器客户端与服务器不同步
- linux nginx线程池,nginx使用线程池提升9倍性能
- java3n 1_1005 继续(3n+1)猜想(JAVA)
- linux中mpich的运行线程,贝叶斯法构建进化树:MrBayes
- 产品运行所需的信息检索失败_禁煤后用什么替代锅炉?看看三种热源运行费用对比就知道了...
- 批作业是小学老师的一大乐趣 | 今日最佳
- 从生物神经网络到人工神经网络
- modbus 台达a2_驱控智造未来 台达重磅发布多款工业自动化新品
- 计算机技术级生活中的应用,人工智能技术在计算机中的发展与应用