geometry-api-java 学习笔记(四)多线段 polyline
Polyline
- API Reference
A polyline is an ordered collection of paths. Each path is a collection of contiguous line segments.A line segment is defined by a pair of consecutive points.
多线段,就是path的一个集合。
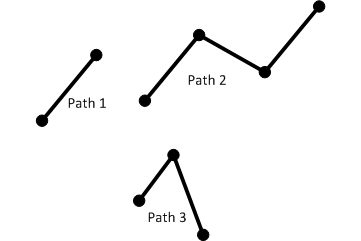
Boundary, Interior & Exterior
The boundary of a polyline is the set of start and end points of each path. Theinterior of a polylineis the set of points in the polyline that are not in the boundary. Theexterior of a polyline is the set ofpoints that are not in the boundary or the interior. It is important to understand the boundary, interior and exteriorof a geometry when using the various operators. The relational operators, for example, rely heavily on theseconcepts.
For the polyline shown below, the set of points comprising the boundary is shown in red. The interior of the polyline is shown in black.
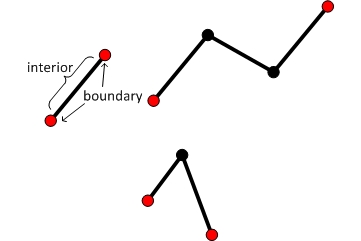
If a vertex is contained in several paths, how do we determine if it is in the boundary of the polyline?To determine if a vertex is in the boundary of a polyline count the number of segments in which it is itis contained. If that number is even, then the vertex is not in the boundary. If that number is odd, thenthe vertex is in the boundary.
For example, consider the polyline shown below.
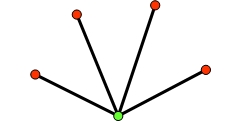
Clearly, the red vertices are in the boundary of the polyline. But what about the green one? The answeris that the green vertex is not in the boundary since it is contained in four segments.
Here is another example.
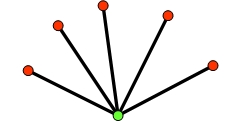
In this case, since the green vertex is contained in five segments, it is in the boundary of the polyline.
One way to remember this rule is to think of a single path with three vertices.
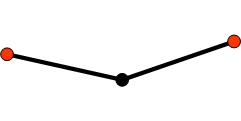
It is easy to see that the red vertices are in the boundary and the black vertex is not. Notice that thenumber of segments containing each of the red vertices is one, an odd number. Now count the number of segmentscontaining the black vertex. Two, an even number.
Valid polylines
A valid polyline is such that every path has at least two distinct points. Tolerance is used to determine iftwo points are distinct. In other words, if the distance between two points is less than the tolerance specifiedby the spatial reference, then the points are considered equal.
A closed path is a path with more than two distinct points and the start point equals the end point.A closed path is a valid polyline.
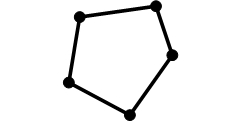
A valid polyline is said to be simple.See the Simplify operator for a more detailed specificationof simple polylines. Note that a simple polyline may not be OGC compliant. See theSimplify operator with OGC restrictions.
Examples
Let's look at some examples of polylines.
In the first group of examples, the green circles are the vertices of the polyline.
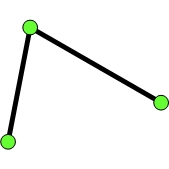
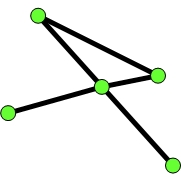
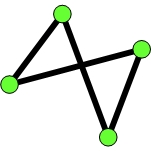
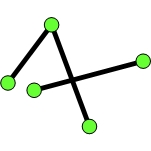
This example shows polylines which represent some of the major highways in the United States.
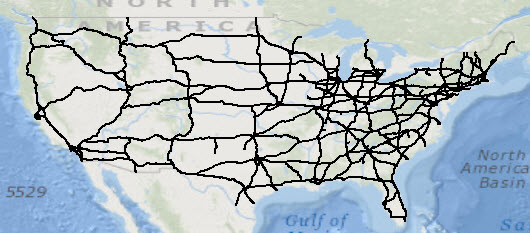
JSON format
A polyline can be represented as a JSON string. A polyline in JSON format contains an array ofpaths
and an optional spatialReference
. A polyline can also have boolean-valuedhasM
andhasZ
fields. The default value is false for both thehasM
and hasZ
fields.
Each path is represented by an array of points. Each point in a path is represented as an array of numbers.See the description ofJSON multipoints for details on theinterpretation of the point arrays.
An empty polyline is represented with an empty array for the paths
field.
Syntax
{"hasZ" : true | false,"hasM" : true | false,"paths": [[[<x11>,<y11>,<z11>,<m11>],[<x12>,<y12>,<z12>,<m12>], ... ,[<x1j>,<y1j>,<z1j>,<m1j>]],... ,[[<xn1>,<yn1>,<zn1>,<mn1>],[<xn2>,<yn2>,<zn2>,<mn2>], ... ,[<xnk>,<ynk>,<znk>,<mnk>]]],"spatialReference" : {"wkid" : <wkid>} }
2D polyline
{"paths": [[[32462,-57839],[43892,-49160],[35637,-65035],[46009,-60379]],[[52570,-77736],[39870,-83027],[46644,-99114]]],"spatialReference" : {"wkid" : 54004} }
3D polyline with Ms
Note that the third point does not have a z-value, and the second ring does not have any m-values.
{"hasZ" : true,"hasM" : true,"paths": [[[32462,-57839,20,1],[43892,-49160,25,2],[35637,-65035,null,3],[46009,-60379,22,4]],[[52570,-77736,30],[39870,-83027,32],[46644,-99114,35]]],"spatialReference" : {"wkid" : 54004} }
Empty polyline
{"paths": []}
Creating a polyline
To create a polyline, we can use the Polyline
class methods or one of the import operators.
Each of the code samples below creates a polyline with three paths.
The polyline looks like this:
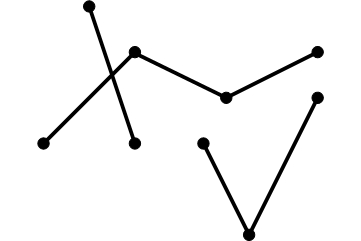
Polyline
class methods
To begin each path, we call the startPath
method and then successsive calls to thelineTo
method.
这里创建用到2个方法:startPath
表示起点,lineTo
连接线。
static Polyline createPolyline1() {Polyline line = new Polyline();// Path 1line.startPath(6.9, 9.1);line.lineTo(7, 8.8);// Path 2line.startPath(6.8, 8.8);line.lineTo(7, 9);line.lineTo(7.2, 8.9);line.lineTo(7.4, 9);// Path 3line.startPath(7.4, 8.9);line.lineTo(7.25, 8.6);line.lineTo(7.15, 8.8);return poly; }
Import from JSON
We first create the JSON string which represents the polyline. Note that the order of the paths doesn'tmatter. Only the order of the points within each path is important. We then call theexecute
method of OperatorImportFromJson
.
static Polyline createPolygonFromJson() throws JsonParseException, IOException {String jsonString = "{\"paths\":[[[6.8,8.8],[7,9],[7.2,8.9],[7.4,9]],"+ "[[7.4,8.9],[7.25,8.6],[7.15,8.8]],[[6.9, 9.1],[7, 8.8]]],"+ "\"spatialReference\":{\"wkid\":4326}}";MapGeometry mapGeom = OperatorImportFromJson.local().execute(Geometry.Type.Polyline, jsonString);return (Polyline)mapGeom.getGeometry(); }
Import from GeoJSON
We first create the GeoJSON string which represents the polyline. We then call theexecute
method of OperatorImportFromGeoJson
.
The code shown below creates the same polygon as in the previous examples.
static Polyline createPolylineFromGeoJson() throws JsonParseException, IOException {String geoJsonString = "{\"type\":\"MultiLineString\","+ "\"coordinates\":[[[6.8,8.8],[7,9],[7.2,8.9],[7.4,9]],"+ "[[7.4,8.9],[7.25,8.6],[7.15,8.8]],[[6.9, 9.1],[7, 8.8]]],"+ "\"crs\":\"EPSG:4326\"}";MapGeometry mapGeom = OperatorImportFromGeoJson.local().execute(GeoJsonImportFlags.geoJsonImportDefaults, Geometry.Type.Polyline, geoJsonString, null);return (Polyline)mapGeom.getGeometry(); }
Import from WKT
We first create the WKT string which represents the polyline. We then call theexecute
method of OperatorImportFromWkt
.
The code shown below creates the same polygon as in the previous examples.
static Polyline createPolylineFromWKT() throws JsonParseException, IOException {String wktString = "MULTILINESTRING ((6.9 9.1,7 8.8),(6.8 8.8,7 9,7.2 8.9,7.4 9),(7.4 8.9,7.25 8.6,7.15 8.8))";Geometry geom = OperatorImportFromWkt.local().execute(WktImportFlags.wktImportDefaults, Geometry.Type.Polyline, wktString, null);return (Polyline)geom; }
geometry-api-java 学习笔记(四)多线段 polyline相关推荐
- JAVA学习笔记(四十九)- Swing相关组件
JFrame组件 import java.awt.Color;import javax.swing.JFrame; import javax.swing.JPanel;/** Swing是在AWT基础 ...
- java学习笔记(四)----对象、数组作为参数传递,静态变量、静态方法的使用,内部类,使用文档注释
***对象作为参数传递*** class passparam { int x; public static void main(String[] args) { passparam obj = ...
- Java学习笔记四:static关键字
1.static表示什么? static表示"全局"或者"静态"的意思.在Java中没有全局变量的概念,static 可以简单的理解为全局的,独立的一个功能. ...
- 【Java学习笔记四】Java中的包
包的声明和引入:在Java语言系统中,Java编译器为每一个类生成一个字节码文件(.class),为了对类文件进行分层和按用途分类管理,同时也为了解决相同类名的文件冲突的问题,Java提供了包机制来管 ...
- Java学习笔记四(可视化的基基基基础)
不算是完整的代码,便于自己理解的写法. import java.awt.Container; import java.awt.FlowLayout; import java.awt.event.Act ...
- java学习笔记四:正则表达式
今天接触了正则表达式,我们在编写处理字符串的程序或网页时,经常会有查找符合某些复杂规则的字符串的需要,正则表达式就可以很好地描述这些规则.换句话说,正则表达式就是记录文本规则的代码. 现在用一个实例来 ...
- JAVA学习笔记(四)城堡游戏
城堡游戏 我们在尝试了之前的简单媒体库构造之后,试着整合一下之前学到的关于类,继承,多态等知识,制作一个简单的城堡游戏,城堡游戏是一个简单的文字游戏,通过输入命令可以在地图上不同的房间进行移动. 目录 ...
- Java学习笔记(四)——接口
Java学习笔记(四)--接口 1.格式 2.接口的特点 3.接口举例 1.格式 接口:初期理解,可以认为是一个特殊的抽象类.当抽象类中的方法都是抽象的,那么该类 可以通过接口的方式来表示. clas ...
- java学习笔记13--反射机制与动态代理
本文地址:http://www.cnblogs.com/archimedes/p/java-study-note13.html,转载请注明源地址. Java的反射机制 在Java运行时环境中,对于任意 ...
- java学习笔记16--I/O流和文件
本文地址:http://www.cnblogs.com/archimedes/p/java-study-note16.html,转载请注明源地址. IO(Input Output)流 IO流用来处理 ...
最新文章
- ubuntu16.04 opencv多版本管理与切换
- SQL Server 系统存储过程
- Matplotlib 中文用户指南 3.3 使用 GridSpec 自定义子图位置
- Linux操作Oracle(2)——Oracle导出exp导出用户报错:EXP-00006: 出现内部不一致的错误 EXP-00000: 导出终止失败
- python为list实现find方法
- 黑马程序员C++学习笔记(第二阶段核心:面向对象)(一)
- 科学计算器 java_用Java编写的标准计算器、科学计算器、时间转换。
- 关于MD5和salt盐值加密后破解方法
- 微软应用商城下载ShareX老出错
- 利用Tensorflow构建RNN并对序列数据进行建模
- flink yarn模式HA部署
- Gunicorn-使用详解
- 【UOJ311】【UNR #2】积劳成疾
- Linux GPIO操作分析 - Exynos 5260
- 操作系统bootloader是什么
- 【BUUCTF】reverse2
- 内网渗透-内网信息收集
- Excel作图-多层环图制作
- 使用JD-GUI批量反编译Java文件
- Java开发面试题!mysqlfront导出数据库
热门文章
- android ndk使用c 11,使用c 11 std :: async在android ndk中使用不完整类型无效
- 华为交换机配置Telnet步骤
- python中lambda函数对时间排序_python – 使用lambda函数排序()
- yolov3权重_目标检测之 YOLOv3 (Pytorch实现)
- python小案例_Python的应用小案例
- hdfs数据节点分发什么协议_HDFS主要节点解说(一)节点功能
- 64位linux安装mysql数据库吗_CentOS7 64位安装mysql教程
- inc指令是什么意思_mips指令集与cpu架构(一)
- 小学计算机打字基础知识,浅谈小学计算机教学技巧5篇
- android volley 上传图片 和参数,android Volley 上传文件上传图片