easyui04:datagrid数据查询
在easyui03上再加了些方法
com.zking.entitypackage com.zking.entity;import java.io.Serializable;/*** 实体类:书籍类* @author zjjt**/
public class Book implements Serializable{private int bid;private String bname;private double bprice;private String btype;public int getBid() {return bid;}public void setBid(int bid) {this.bid = bid;}public String getBname() {return bname;}public void setBname(String bname) {this.bname = bname;}public double getBprice() {return bprice;}public void setBprice(double bprice) {this.bprice = bprice;}public String getBtype() {return btype;}public void setBtype(String btype) {this.btype = btype;}public Book() {// TODO Auto-generated constructor stub}public Book(int bid, String bname, double bprice, String btype) {this.bid = bid;this.bname = bname;this.bprice = bprice;this.btype = btype;}public Book(String bname, double bprice, String btype) {this.bname = bname;this.bprice = bprice;this.btype = btype;}@Overridepublic String toString() {return "Book [bid=" + bid + ", bname=" + bname + ", bprice=" + bprice + ", btype=" + btype + "]";}}
数据库插入的新数据的脚本create table tb_book (bid number not null,bname varchar2(50) not null,bprice float not null,btype varchar2(40) not null,primary key(bid)
)-- 测试数据
insert into TB_BOOK (bid, bname, bprice, btype)
values (1, '西游记', 180, '名著');
insert into TB_BOOK (bid, bname, bprice, btype)
values (2, '红楼梦1', 110.08, '名著');
insert into TB_BOOK (bid, bname, bprice, btype)
values (3, '倚天屠龙记', 150.16, '武侠');
insert into TB_BOOK (bid, bname, bprice, btype)
values (4, '聊斋志异1', 100.12, '玄幻');
insert into TB_BOOK (bid, bname, bprice, btype)
values (5, '永生', 110.11, '玄幻');
insert into TB_BOOK (bid, bname, bprice, btype)
values (6, '武动乾坤', 90.89, '玄幻');
insert into TB_BOOK (bid, bname, bprice, btype)
values (7, '完美世界1', 100, '玄幻');
insert into TB_BOOK (bid, bname, bprice, btype)
values (8, '万域之王', 56.5, '玄幻');
insert into TB_BOOK (bid, bname, bprice, btype)
values (9, '遮天1', 130.9, '玄幻');
insert into TB_BOOK (bid, bname, bprice, btype)
values (10, '凡人修仙传1', 200, '修仙');
insert into TB_BOOK (bid, bname, bprice, btype)
values (11, '倚天屠龙记', 150.16, '武侠');
insert into TB_BOOK (bid, bname, bprice, btype)
values (12, '斗破苍穹', 115.07, '玄幻');
insert into TB_BOOK (bid, bname, bprice, btype)
values (13, '超级兵王1', 145, '言情');
insert into TB_BOOK (bid, bname, bprice, btype)
values (14, '武极天下', 45.55, '玄幻');
insert into TB_BOOK (bid, bname, bprice, btype)
values (15, '聊斋志异', 100.12, '玄幻');
insert into TB_BOOK (bid, bname, bprice, btype)
values (16, '永生1', 110.11, '玄幻');
insert into TB_BOOK (bid, bname, bprice, btype)
values (17, '武动乾坤', 90.89, '玄幻');
insert into TB_BOOK (bid, bname, bprice, btype)
values (18, '完美世界', 100, '玄幻');
insert into TB_BOOK (bid, bname, bprice, btype)
values (19, '万域之王', 56.5, '玄幻');
insert into TB_BOOK (bid, bname, bprice, btype)
values (20, 'Java', 1000, '修仙');
insert into TB_BOOK (bid, bname, bprice, btype)
values (21, '娃哈哈', 100, '玄幻');
insert into TB_BOOK (bid, bname, bprice, btype)
values (22, '呼啸山庄1', 123, '哈哈');
insert into TB_BOOK (bid, bname, bprice, btype)
values (23, '平凡的世界', 123, '哈哈');
insert into TB_BOOK (bid, bname, bprice, btype)
values (24, '大红底1', 12, '哈哈');
insert into TB_BOOK (bid, bname, bprice, btype)
values (25, '屌丝的逆袭1', 34.67, '哈哈');
insert into TB_BOOK (bid, bname, bprice, btype)
values (26, '嗨害嗨1', 22.3, '哈哈');
commit;
com.zking.daopackage com.zking.dao;import java.util.List;import com.zking.entity.Book;public interface IBookDao {/*** 带模糊查询的分页* @param pageIndex 页数* @param pageSize 每页几条数据* @param str 名称的关键字* @return 结果集合*/public List<Book> getAllByPage(int pageIndex,int pageSize,String str,String col);/*** 获得总行数* @param str 表名等* @return 总行数*/public int getRows(String str);}package com.zking.dao;import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.util.ArrayList;
import java.util.List;import com.zking.entity.Book;
import com.zking.util.DBHelper;public class BookDao implements IBookDao{//三兄弟Connection con = null;PreparedStatement ps = null;ResultSet rs = null;@Overridepublic List<Book> getAllByPage(int pageIndex, int pageSize, String str,String col) {List<Book> list = new ArrayList<Book>();int a =(pageIndex-1)*pageSize+1;int b = pageIndex*pageSize;try {//获得连接con=DBHelper.getCon();//定义SQL语句String sql = "select * from (select a.*,rownum as rid from tb_book a where "+col+" like '%"+str+"%') b where b.rid between ? and ?";//获得执行对象ps=con.prepareStatement(sql);//给占位符赋值ps.setInt(1, a);ps.setInt(2, b);//获得结果集rs=ps.executeQuery();while(rs.next()) {Book bb = new Book(rs.getInt(1), rs.getString(2), rs.getDouble(3), rs.getString(4));//加到集合里面去list.add(bb);}} catch (Exception e) {e.printStackTrace();}finally {DBHelper.myClo(con, ps, rs);}return list;}@Overridepublic int getRows(String str) {int n = 0;try {con=DBHelper.getCon();//定义SQL语句String sql = "select count(*) from "+str;//获得执行对象ps=con.prepareStatement(sql);//获得结果集rs=ps.executeQuery();if(rs.next()) {n=rs.getInt(1);}} catch (Exception e) {e.printStackTrace();}finally {DBHelper.myClo(con, ps, rs);}return n;}}
com.zking.bizpackage com.zking.biz;import java.util.List;import com.zking.entity.Book;public interface IBookBiz {/*** 带模糊查询的分页* @param pageIndex 页数* @param pageSize 每页几条数据* @param str 名称的关键字* @return 结果集合*/public List<Book> getAllByPage(int pageIndex,int pageSize,String str,String col);/*** 获得总行数* @param str 表名等* @return 总行数*/public int getRows(String str);}package com.zking.biz;import java.util.List;import com.zking.dao.BookDao;
import com.zking.dao.IBookDao;
import com.zking.entity.Book;
/*** 业务逻辑层* @author zjjt**/
public class BookBiz implements IBookBiz{IBookDao ibd = new BookDao();@Overridepublic List<Book> getAllByPage(int pageIndex, int pageSize, String str,String col) {return ibd.getAllByPage(pageIndex, pageSize, str,col);}@Overridepublic int getRows(String str) {return ibd.getRows(str);}// public static void main(String[] args) {
// System.out.println(ibd.getAllByPage(1, 10, ""));
// }}
com.zking.servletpackage com.zking.servlet;import java.io.IOException;
import java.io.PrintWriter;
import java.util.HashMap;
import java.util.List;
import java.util.Map;import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;import com.alibaba.fastjson.JSON;
import com.zking.biz.BookBiz;
import com.zking.biz.IBookBiz;
import com.zking.entity.Book;/*** Servlet implementation class BookListServlet*/
@WebServlet("/BookListServlet")
public class BookListServlet extends HttpServlet {protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {doPost(request, response);}protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {//设置编码方式request.setCharacterEncoding("utf-8");response.setCharacterEncoding("utf-8");response.setContentType("text/html; charset=UTF-8");//获得outPrintWriter out = response.getWriter();int pageIndex=1;int pageSize=10;//接收前台传递过来的值 pageString pid = request.getParameter("page");//当前页码if(pid!=null) {pageIndex=Integer.parseInt(pid);}//接收前台传递过来的值 sizeString size = request.getParameter("rows");//每页多少条if(size!=null) {pageSize=Integer.parseInt(size);}/*//接收文本框关键词String bname = request.getParameter("bname");//关键字if(bname==null) {bname="";//相当于查询全部}*///下拉框String bname = request.getParameter("bname");if(bname==null) {bname="bname";}//System.out.println(bname+"=========");//关键字 文本框String str = request.getParameter("str");if(str==null) {str="";//相当于查询全部}//System.out.println(str+"=========");//servlet调用bizIBookBiz ibb = new BookBiz();//获取总行数int rows = ibb.getRows(" tb_book where "+bname+" like '%"+str+"%'");//拿到分页的集合List<Book> ls = ibb.getAllByPage(pageIndex, pageSize,str,bname);//相当于查询所有//前台的JSON数据需要的两个参数: total:总行数 rows:书籍集合Map<String, Object> mym = new HashMap<String, Object>();//放两对值mym.put("total",rows);mym.put("rows", ls);//把map集合变成Jason格式的对象字符串String b = JSON.toJSONString(mym);//输送到页面out.write(b);out.flush();out.close();}}
index.jsp<%@ page language="java" contentType="text/html; charset=UTF-8"pageEncoding="UTF-8"%><!-- 引入公共页面 -->
<%@ include file="common/head.jsp" %>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
<script type="text/javascript">$(function(){$('#myTree').tree({ url:ctx+'/moduleServlet' ,//请求地址 Ajaxanimate:true ,//折叠或者展开的时候是否有动画效果onDblClick: function(node){//alert(node.text); // 在用户双击的时候提示//alert(node.url);//路径//拿到后代节点的集合var cs = $('#myTree').tree('getChildren',node.target);//alert(cs);if(cs.length==0){//没有后代节点var f = $('#myTab').tabs('exists',node.text);//判断是否存在if(!f){//说明不存在//新打开一个选项卡(tab页)$('#myTab').tabs('add',{ title:node.text,//标题content:'<iframe scrolling="no" frameborder="0px" width="99%" height="99%" src="'+node.url+'" ></iframe>', //内容 closable:true, //是否可关闭/* tools:[{ iconCls:'icon-ok', handler:function(){ alert('想干啥就干啥'); } }]*/iconCls:node.iconCls}); }else{//说明存在 让其对应选中$('#myTab').tabs('select',node.text);}}}}); })
</script>
</head>
<body class="easyui-layout"> <div data-options="region:'north',split:true" style="height:85px;text-align:center;"><h1>后台书籍管理</h1></div> <div data-options="region:'south',split:true" style="height:60px;text-align:center;"><b>©玉渊工作室所有,未经允许不可擅自使用</b></div> <div data-options="region:'west',title:'功能导航',split:false" style="width:150px;"><!--左侧 tree控件 --><ul id="myTree" class="easyui-tree"> </ul> </div> <div data-options="region:'center'" style="padding:5px;background:#fff;"><!--中间的tab控件 --><div id="myTab" data-options="pill:true" class="easyui-tabs" style="width:100%;height:100%;"> <div data-options="iconCls:'icon-application-home'" title="首页" style="padding:20px;display:none;"> <img src="data:images/1.jpeg" width="100%" height="100%"> </div> </div> </div>
</body>
</html>
效果图:
easyui04:datagrid数据查询相关推荐
- .easyui(DataGrid数据查询)
1.DataGrid 1.1 DataGrid基本属性 1.1.1 基本属性 url : '', // 初始化请求路径 fitcolumns : false, ...
- easyui_04.DataGrid数据查询
1.创建一个表代码在下面 create table t_book (id integer not null,bookname varchar(50) not null,price float not ...
- Easyui之Datagrid(数据表格)
Datagrid 前言 1.概念 2.常见使用案例 3.datagrid属性 4.datagrid事件 5.datagrid方法 6.案例演示 7.为什么使用datagrid 总结 前言 今天为大家分 ...
- 关于EasyUI Datagrid 数据网格使用策略
每天一小步前进一大步,记录一下前段时间使用的EasyUI数据网格.话不多说,上图. 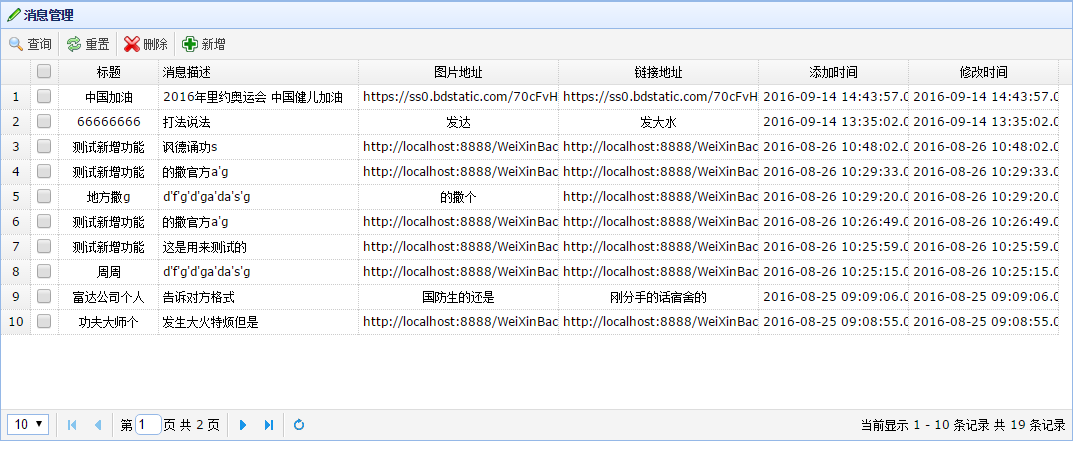 ![第二页] ...
- easyui04---datagrid数据查询
一.datagrid 首先这是一个基本的表格代码 <table id="tt" class="easyui-treegrid" style="w ...
- 合肥工业大学—SQL Server数据库实验七:数据查询
数据查询 1. 单表查询 2. 多表连接查询 1. 单表查询 1. 查询全体学生的信息: -- 查询全体学生的信息 select * from student 2. 根据专业编号(21)查询学生的学号 ...
- Java网页数据采集器[下篇-数据查询]【转载】
本期概述 上一期我们学习了如何将html采集到的数据存储到MySql数据库中,这期我们来学习下如何在存储的数据中查询我们实际想看到的数据. 数据采集页面 2011-2012赛季英超球队战绩 如果是初学 ...
- 用python做一个数据查询软件_Python实现功能简单的数据查询及可视化系统
欢迎点击右上角关注小编,除了分享技术文章之外还有很多福利,私信学习资料可以领取包括不限于Python实战演练.PDF电子文档.面试集锦.学习资料等. image.png 前言 数据时代,数据的多源集成 ...
- 查询两张表 然后把数据并在一起_工作表数据查询时,类似筛选功能LIKE和NOT LIKE的应用...
大家好,我们继续讲解VBA数据库解决方案,今日讲解第53讲内容:工作表查询时,类似于筛选功能的LIKE和NOT LIKE 的应用.大家在工作的时候,利用EXCEL操作,筛选是必不可少的工具之一.例如我 ...
最新文章
- iPhone读取plist文件
- 为什么计算机系统安全具有整体性质,操作系统全局性质的形式化描述和验证
- 3.IDA-数据显示窗口(导出窗口、导入窗口、String窗口、...窗口)
- 树莓派3B+,我要跑.NET CORE
- (转)基因芯片数据GO和KEGG功能分析
- 字符串正反连接java_字符串正反连接(Java实现,超简单)
- 使用spring的JavaMailSender发送邮件
- 用sed替换文件中的空格
- 华三服务器linux系统安装u盘,华三H3CR4900服务器安装linux系统
- linux设计引物探针,恳请相助:Taq man引物和探针设计
- 项目管理-Visio可以绘制甘特图编辑操作极方便
- 蓝屏代码0x00000109 错误分析
- ps自定义(新建)图框工具
- angularJS与IE8整合简介
- 模拟与仿真两个词的区别
- win10计算机未连接到网络适配器,Windows 10 Hyper-V网络适配器未连接
- AUTOSAR CanNm Nm Configuration
- 可信安全网络 —— 安全左移之DDoS对抗
- Hive中如何统计用户三个月或者以上的行为数据
- 大厂面试系列(七):数据结构与算法等