java生成酷炫霸气叼二维码
java生成二维码
文章目录
- java生成二维码
- pom依赖
- 第一种类型
- 第二种类型
- 完整pom文件
pom依赖
<!--生成二维码依赖--><!-- https://mvnrepository.com/artifact/com.google.zxing/javase --><dependency><groupId>com.google.zxing</groupId><artifactId>javase</artifactId><version>3.3.1</version></dependency><!-- https://mvnrepository.com/artifact/com.google.zxing/core --><dependency><groupId>com.google.zxing</groupId><artifactId>core</artifactId><version>3.3.1</version></dependency><!--生成二维码依赖--><!--生成可定制二维码--><!-- 请注意groupId和github的方式有一些区别哦 --><dependency><groupId>com.github.liuyueyi.quick-media</groupId><artifactId>qrcode-plugin</artifactId><version>2.5</version></dependency><!--生成可定制二维码-->
第一种类型
package com.ims.common.utils;import com.google.zxing.BarcodeFormat;
import com.google.zxing.EncodeHintType;
import com.google.zxing.MultiFormatWriter;
import com.google.zxing.common.BitMatrix;
import com.google.zxing.qrcode.decoder.ErrorCorrectionLevel;import javax.imageio.ImageIO;
import java.awt.*;
import java.awt.image.BufferedImage;
import java.io.File;
import java.io.FileOutputStream;
import java.io.IOException;
import java.util.HashMap;
import java.util.Map;import static java.awt.image.ImageObserver.HEIGHT;
import static java.awt.image.ImageObserver.WIDTH;public class QRCodeUtil1 {private static final int BLACK = 0xFF000000;private static final int WHITE = 0xFFFFFFFF;/*** 把生成的二维码存入到图片中* @param matrix zxing包下的二维码类* @return*/public static BufferedImage toBufferedImage(BitMatrix matrix) {int width = matrix.getWidth();int height = matrix.getHeight();BufferedImage image = new BufferedImage(width, height, BufferedImage.TYPE_INT_RGB);for (int x = 0; x < width; x++) {for (int y = 0; y < height; y++) {image.setRGB(x, y, matrix.get(x, y) ? BLACK : WHITE);}}return image;}/*** 生成二维码并写入文件* @param content 扫描二维码的内容* @param format 图片格式 jpg* @param file 文件* @throws Exception*/public static void writeToFile(String content, String format, File file)throws Exception {MultiFormatWriter multiFormatWriter = new MultiFormatWriter();@SuppressWarnings("rawtypes")Map hints = new HashMap();//设置UTF-8, 防止中文乱码hints.put(EncodeHintType.CHARACTER_SET, "UTF-8");//设置二维码四周白色区域的大小hints.put(EncodeHintType.MARGIN,10);//设置二维码的容错性hints.put(EncodeHintType.ERROR_CORRECTION, ErrorCorrectionLevel.H);//画二维码BitMatrix bitMatrix = multiFormatWriter.encode(content, BarcodeFormat.QR_CODE, 300, 300, hints);BufferedImage image = toBufferedImage(bitMatrix);if (!ImageIO.write(image, format, file)) {throw new IOException("Could not write an image of format " + format + " to " + file);}}/*** 给二维码图片加上文字* @param pressText 文字* @param qrFile 二维码文件* @param fontStyle* @param color* @param fontSize*/public static void pressText(String pressText, File qrFile, int fontStyle, Color color, int fontSize) throws Exception {pressText = new String(pressText.getBytes(), "utf-8");Image src = ImageIO.read(qrFile);int imageW = src.getWidth(null);int imageH = src.getHeight(null);BufferedImage image = new BufferedImage(imageW, imageH, BufferedImage.TYPE_INT_RGB);Graphics g = image.createGraphics();g.drawImage(src, 0, 0, imageW, imageH, null);//设置画笔的颜色g.setColor(color);//设置字体Font font = new Font("宋体", fontStyle, fontSize);FontMetrics metrics = g.getFontMetrics(font);//文字在图片中的坐标 这里设置在中间
// int startX = (WIDTH - metrics.stringWidth(pressText)) / 2;
// int startY = HEIGHT/2;g.setFont(font);g.drawString(pressText, 235, 100);g.dispose();FileOutputStream out = new FileOutputStream(qrFile);ImageIO.write(image, "JPEG", out);out.close();System.out.println("image press success");}public static void main(String[] args) throws Exception {File qrcFile = new File("e:/tmp/","google.jpg");writeToFile("www.google.com.hk", "jpg", qrcFile);pressText("谷歌", qrcFile, 5, Color.RED, 32);}}
第二种类型
package com.ims.common.utils;import java.awt.*;
import java.awt.image.BufferedImage;
import java.io.File;
import java.io.IOException;
import java.util.Date;
import java.util.HashMap;
import java.util.Map;import javax.imageio.ImageIO;import com.github.hui.quick.plugin.qrcode.wrapper.QrCodeGenWrapper;
import com.google.zxing.*;
import com.google.zxing.client.j2se.BufferedImageLuminanceSource;
import com.google.zxing.common.BitMatrix;
import com.google.zxing.common.HybridBinarizer;
/*** Google zxing二维码工具类** @2017年12月20日* @author yinxf**/
public class QRCodeUtil {//二维码颜色private static final int BLACK = 0xFF000000;//二维码颜色private static final int WHITE = 0xFFFFFFFF;public static void main(String[] args) throws Exception {// zxingCodeCreate("http://www.baidu.com", 300, 300, "e:/tmp/qrcode.jpg", "jpg");
// zxingCodeAnalyze("e:/tmp/qrcode.jpg");
// testQrCode("你好再见","e:/tmp/cqr.png");}/*** 定制二维码生成*/public static void testQrCode(String msg,String outPutPath){try {long stime = System.currentTimeMillis();
// String msg = "https://weixin.qq.com/r/FS9waAPEg178rUcL93oH";boolean ans = QrCodeGenWrapper.of(msg).asFile(outPutPath);System.out.println(System.currentTimeMillis()-stime);} catch (IOException e) {e.printStackTrace();} catch (WriterException e) {e.printStackTrace();}}/*** 生成二维码** @param text 二维码内容* @param width 二维码宽* @param height 二维码高* @param outPutPath 二维码生成保存路径* @param imageType 二维码生成格式*/public static void zxingCodeCreate(String text, int width, int height, String outPutPath, String imageType) {Map<EncodeHintType, Object> his = new HashMap<EncodeHintType, Object>();//设置编码字符集his.put(EncodeHintType.CHARACTER_SET, "utf-8");his.put(EncodeHintType.MARGIN,1);try {//1、生成二维码BitMatrix encode = new MultiFormatWriter().encode(text, BarcodeFormat.QR_CODE, width, height, his);//2、获取二维码宽高int codeWidth = encode.getWidth();int codeHeight = encode.getHeight();//3、将二维码放入缓冲流BufferedImage image = new BufferedImage(codeWidth, codeHeight, BufferedImage.TYPE_INT_RGB);for (int i = 0; i < codeWidth; i++) {for (int j = 0; j < codeHeight; j++) {//4、循环将二维码内容定入图片image.setRGB(i, j, encode.get(i, j) ? BLACK : WHITE);}}File outPutImage = new File(outPutPath);//如果图片不存在创建图片if (!outPutImage.exists())outPutImage.createNewFile();//5、将二维码写入图片ImageIO.write(image, imageType, outPutImage);} catch (WriterException e) {e.printStackTrace();System.out.println("二维码生成失败");} catch (IOException e) {e.printStackTrace();System.out.println("生成二维码图片失败");}}/*** 二维码解析** @param analyzePath 二维码路径* @return* @throws IOException*/@SuppressWarnings({"rawtypes", "unchecked"})public static String zxingCodeAnalyze(String analyzePath) throws Exception {MultiFormatReader formatReader = new MultiFormatReader();String resultStr = null;try {File file = new File(analyzePath);if (!file.exists()) {return "二维码不存在";}BufferedImage image = ImageIO.read(file);LuminanceSource source = new BufferedImageLuminanceSource(image);Binarizer binarizer = new HybridBinarizer(source);BinaryBitmap binaryBitmap = new BinaryBitmap(binarizer);Map hints = new HashMap();hints.put(EncodeHintType.CHARACTER_SET, "UTF-8");Result result = formatReader.decode(binaryBitmap, hints);resultStr = result.getText();System.out.println(resultStr);} catch (NotFoundException e) {e.printStackTrace();}return resultStr;}}
完整pom文件
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/maven-v4_0_0.xsd"><!-- 配置下载镜像 --><repositories><repository><id>jitpack.io</id><url>https://jitpack.io</url></repository></repositories><!-- 配置下载镜像 --><modelVersion>4.0.0</modelVersion><groupId>com.demodata</groupId><artifactId>sm</artifactId><packaging>jar</packaging><version>0.0.1-SNAPSHOT</version><build><plugins><plugin><artifactId>maven-resources-plugin</artifactId><version>3.1.0</version><executions><execution><id>copy-webapp</id><phase>process-sources</phase><goals><goal>copy-resources</goal></goals><configuration><encoding>UTF-8</encoding><outputDirectory>${basedir}/target/classes/webapp</outputDirectory><resources><resource><directory>${basedir}/src/main/webapp</directory><includes><!-- <include>**/*.xml</include> --><!-- <include>**/**</include> --></includes></resource></resources></configuration></execution></executions></plugin><plugin><groupId>org.apache.maven.plugins</groupId><artifactId>maven-shade-plugin</artifactId><version>3.2.1</version><executions><execution><phase>package</phase><goals><goal>shade</goal></goals><configuration><finalName>ims</finalName><transformers><transformerimplementation="org.apache.maven.plugins.shade.resource.ManifestResourceTransformer"><mainClass>com.ims.common.CoreConfig</mainClass></transformer><!--下面的配置支持排除指定文件打包到 jar 之中,可以用于排除需要修改的配置文件以便于在外部的 config 目录下的同名配置文件生效,建议使用 Prop.appendIfExists(xxx_pro.txt) 在外部放一个非同名配置来覆盖开发环境的配置则可以不用使用下面的配置,文档参考:http://maven.apache.org/plugins/maven-shade-plugin/examples/resource-transformers.html#DontIncludeResourceTransformer--><transformerimplementation="org.apache.maven.plugins.shade.resource.DontIncludeResourceTransformer"><resources><!-- <resource>demo-config-dev.txt</resource> --><!-- <resource>.PDF</resource> --><!-- <resource>READ.md</resource> --></resources></transformer></transformers></configuration></execution></executions></plugin><plugin><groupId>org.apache.maven.plugins</groupId><artifactId>maven-compiler-plugin</artifactId><configuration><source>1.8</source><target>1.8</target><encoding>UTF-8</encoding></configuration></plugin></plugins></build><dependencies><dependency><groupId>com.jfinal</groupId><artifactId>jfinal-undertow</artifactId><version>1.6</version></dependency><dependency><groupId>com.jfinal</groupId><artifactId>jfinal</artifactId><version>4.2</version></dependency><dependency><groupId>com.esen.jdbc</groupId><artifactId>oscarJDBC</artifactId><version>16</version></dependency><dependency><groupId>commons-beanutils</groupId><artifactId>commons-beanutils</artifactId><version>1.9.3</version></dependency><dependency><groupId>it.sauronsoftware.cron4j</groupId><artifactId>cron4j</artifactId><version>2.2.5</version></dependency><dependency><groupId>commons-cli</groupId><artifactId>commons-cli</artifactId><version>1.4</version></dependency><dependency><groupId>commons-codec</groupId><artifactId>commons-codec</artifactId><version>1.10</version></dependency><dependency><groupId>org.apache.commons</groupId><artifactId>commons-collections4</artifactId><version>4.1</version></dependency><dependency><groupId>commons-configuration</groupId><artifactId>commons-configuration</artifactId><version>1.6</version><exclusions><exclusion><artifactId>commons-beanutils</artifactId><groupId>commons-beanutils</groupId></exclusion><exclusion><artifactId>commons-logging</artifactId><groupId>commons-logging</groupId></exclusion><exclusion><artifactId>commons-collections</artifactId><groupId>commons-collections</groupId></exclusion></exclusions></dependency><dependency><groupId>commons-io</groupId><artifactId>commons-io</artifactId><version>2.4</version></dependency><dependency><groupId>com.jfinal</groupId><artifactId>cos</artifactId><version>2017.5</version></dependency><dependency><groupId>com.alibaba</groupId><artifactId>druid</artifactId><version>1.1.17</version></dependency><dependency><groupId>com.alibaba</groupId><artifactId>fastjson</artifactId><version>1.2.47</version></dependency><dependency><groupId>com.fasterxml.jackson.core</groupId><artifactId>jackson-core</artifactId><version>2.8.5</version></dependency><dependency><groupId>net.sf.json-lib</groupId><artifactId>json-lib</artifactId><version>2.4</version><classifier>jdk15</classifier><exclusions><exclusion><artifactId>commons-lang</artifactId><groupId>commons-lang</groupId></exclusion><exclusion><artifactId>commons-logging</artifactId><groupId>commons-logging</groupId></exclusion><exclusion><artifactId>commons-beanutils</artifactId><groupId>commons-beanutils</groupId></exclusion><exclusion><artifactId>commons-collections</artifactId><groupId>commons-collections</groupId></exclusion></exclusions></dependency><dependency><groupId>com.google.guava</groupId><artifactId>guava</artifactId><version>15.0</version></dependency><dependency><groupId>org.codehaus.jackson</groupId><artifactId>jackson-mapper-asl</artifactId><version>1.9.13</version></dependency><dependency><groupId>log4j</groupId><artifactId>log4j</artifactId><version>1.2.17</version></dependency><dependency><groupId>io.netty</groupId><artifactId>netty-all</artifactId><version>4.1.36.Final</version></dependency><dependency><groupId>com.belerweb</groupId><artifactId>pinyin4j</artifactId><version>2.5.1</version></dependency><dependency><groupId>org.apache.poi</groupId><artifactId>poi</artifactId><version>3.17</version></dependency><dependency><groupId>org.apache.poi</groupId><artifactId>poi-ooxml</artifactId><version>3.17</version></dependency><dependency><groupId>org.apache.poi</groupId><artifactId>poi-scratchpad</artifactId><version>3.17</version></dependency><dependency><groupId>org.apache.poi</groupId><artifactId>ooxml-schemas</artifactId><version>1.1</version></dependency><dependency><groupId>org.slf4j</groupId><artifactId>slf4j-api</artifactId><version>1.7.26</version></dependency><dependency><groupId>org.freemarker</groupId><artifactId>freemarker</artifactId><version>2.3.28</version></dependency><dependency><groupId>junit</groupId><artifactId>junit</artifactId><version>3.8.1</version><scope>test</scope></dependency><!--生成二维码依赖--><!-- https://mvnrepository.com/artifact/com.google.zxing/javase --><dependency><groupId>com.google.zxing</groupId><artifactId>javase</artifactId><version>3.3.1</version></dependency><!-- https://mvnrepository.com/artifact/com.google.zxing/core --><dependency><groupId>com.google.zxing</groupId><artifactId>core</artifactId><version>3.3.1</version></dependency><!--生成二维码依赖--><!--生成可定制二维码--><!-- 请注意groupId和github的方式有一些区别哦 --><dependency><groupId>com.github.liuyueyi.quick-media</groupId><artifactId>qrcode-plugin</artifactId><version>2.5</version></dependency><!--生成可定制二维码--></dependencies></project>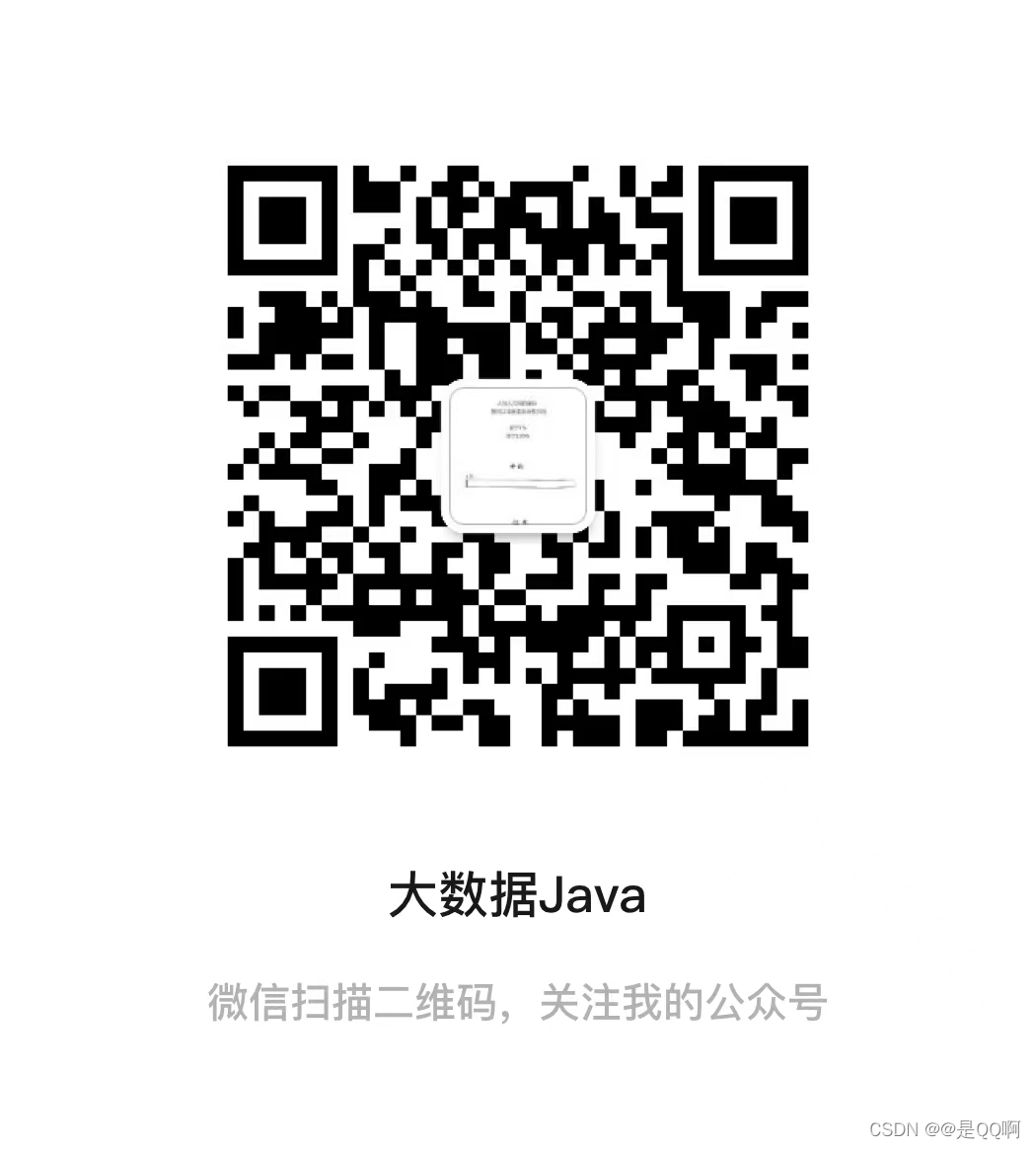
java生成酷炫霸气叼二维码相关推荐
- java生成自定义标志、大小的二维码
为什么80%的码农都做不了架构师?>>> 前段时间没事突然看到有些宣传海报上面打印了带log的二维码,于是在网上查找了生成二维码的方法,自己进行了写修改,下面直接贴出代码供参考 ...
- JAVA生成带图片带名称的二维码
maven引入 com.google.zxing package com.util.qrCode;import com.alibaba.druid.util.Base64; import com.gi ...
- Java实现生成可跳转指定页面的二维码
Java实现生成可跳转指定页面的二维码 package test; import java.awt.BasicStroke; import java.awt.Graphics; import java ...
- 微信公众号开发之生成并扫描带参数的二维码(无需改动)
首先把参考的博文罗列出来: 1.微信公众号开发之生成并扫描带参数的二维码: https://blog.csdn.net/qq_23543983/article/details/80228558 2.由 ...
- 小程序之 保存canvas生成商品图片附加小程序二维码 分享到朋友圈
小程序之 保存canvas生成商品图片附加小程序二维码 分享到朋友圈 一.概述 需要用到的生成二维码组件(可自行下载添加到小程序根目录utils里):https://github.com/demi52 ...
- C#生成带背景和文字的二维码图片
/// <summary> /// 生成带背景和文字的二维码图片 /// </summary> /// <param na ...
- Python学习之生成带logo背景图的二维码(静态和动态图)
前言 二维码简称 QR Code(Quick Response Code),学名为快速响应矩阵码,是二维条码的一种,由日本的 Denso Wave 公司于 1994 年发明.现随着智能手机的普及,已广 ...
- Java:利用qrcode工具类对二维码进行解析或生成
1.引用jar包 <dependency><groupId>com.github.binarywang</groupId><artifactId>qrc ...
- python制作炫酷吊炸天的二维码
import PySimpleGUI as sg from MyQR import myqrsg.change_look_and_feel("LightBlue") layout ...
最新文章
- 从0开始搭建编程框架——主框架和源码
- 独家 | 使用机器学习对非结构化数据加速查询-第2部分(具有统计保证的近似选择查询)...
- 防止程序重复执行的单元
- Roger Ver:BCH也可成为价值储备,前提是它被用起来
- ZNNT-5NM 扭矩测量模块
- 自考计算机和行政管理哪个好考,自考行政管理好考吗?自考行政管理都考哪些科目?...
- mysql例子 restful_Gin实战:Gin+Mysql简单的Restful风格的API
- android 手机固定mac,Android之获取手机MAC
- case --when
- 如何备份服务器日志到其他服务器_KIWI Syslog日志服务器搭建及配置
- Android系统架构图
- sae java 开发环境_新浪开放平台 sae环境 java主机使用感受
- 大数据BI可视化软件在企业的应用
- Spring read-only=true 只读事务的一些概念
- Office 365系列(6)------Stage Migrate 搬迁方式至O365上来方法及步骤总结
- c语言编程学习宝典,C语言学习宝典app
- 米游社-原神每日签到含DS算法
- 【LeetCode】算法初涉
- 春风吹又生(1年工作经验感悟)
- 医院挂号系统代码_智慧医院中心是怎样做的?分诊叫号系统如何正确使用!
热门文章
- JAVA毕设项目慧学IT精品课程网站(Vue+Mybatis+Maven+Mysql+sprnig+SpringMVC)
- B样条基函数:1.引言
- ORB_SLAM2代码阅读及总结使用(二)
- ubuntu 16.10下软件记录
- 多地复工复产复销,泰禾集团正在“上岸”
- mysql字符串逆时针旋转180度_mysql字符串操作
- c实战开发前奏之指针常用参数传递 三板斧头到家
- 小程序云开发出坑系列(一)
- Openstack glance 安装 403错误
- 国仁猫哥:微信视频号的快速迭代;狙击抖音快手的最后一张底牌。