POJ1673 EXOCENTER OF A TRIANGLE(三角形垂心)
题目链接:
http://poj.org/problem?id=1673
题目描述:
Description
On each side of ABC, construct a square (ABDE, BCHJ and ACFG in the figure below).
Connect adjacent square corners to form the three Extriangles (AGD, BEJ and CFH in the figure).
The Exomedians of ABC are the medians of the Extriangles, which pass through vertices of the original triangle,extended into the original triangle (LAO, MBO and NCO in the figure. As the figure indicates, the three Exomedians intersect at a common point called the Exocenter (point O in the figure).
This problem is to write a program to compute the Exocenters of triangles.
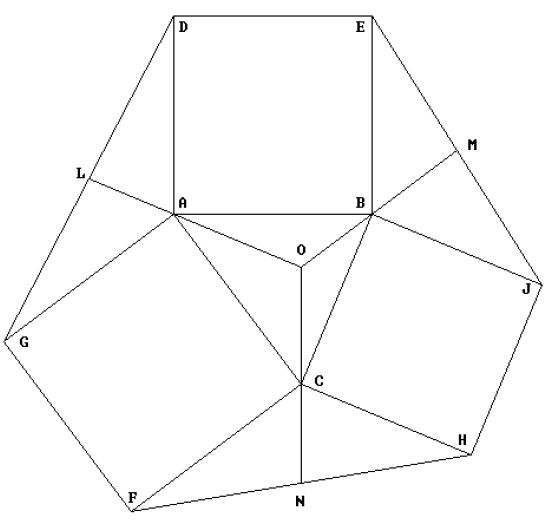
Input
Output
Sample Input
2 0.0 0.0 9.0 12.0 14.0 0.0 3.0 4.0 13.0 19.0 2.0 -10.0
Sample Output
9.0000 3.7500 -48.0400 23.3600
题目大意:
如图,将三边延拓成正方形,然后连线取中点,连线求交点
思路:
证明点O为三角形垂心
然后可知 CO ⊥ AB
另两条边同理
求垂心即可
PS:输出需要加一个EPS,避免-0.000
代码:
1 #include <iostream> 2 #include <cstdio> 3 #include <cstring> 4 #include <algorithm> 5 #include <cmath> 6 using namespace std; 7 8 const double EPS = 1e-8; //精度系数 9 const double PI = acos(-1.0); //π 10 11 struct Point { 12 double x, y; 13 Point(double x = 0, double y = 0) :x(x), y(y) {} 14 const bool operator < (Point A)const { 15 return x == A.x ? y < A.y : x < A.x; 16 } 17 }; //点的定义 18 19 typedef Point Vector; //向量的定义 20 21 Vector operator + (Vector A, Vector B) { return Vector(A.x + B.x, A.y + B.y); } //向量加法 22 Vector operator - (Vector A, Vector B) { return Vector(A.x - B.x, A.y - B.y); } //向量减法 23 Vector operator * (Vector A, double p) { return Vector(A.x*p, A.y*p); } //向量数乘 24 25 Vector Rotate(Vector A, double rad) { 26 return Vector(A.x*cos(rad) - A.y*sin(rad), A.x*sin(rad) + A.y*cos(rad)); 27 } //逆时针旋转rad度 28 29 Point LineIntersectionPoint(Point A1, Point B1, Point A2, Point B2) { 30 double t1 = ((A1.y - A2.y)*(B2.x - A2.x) - (A1.x - A2.x)*(B2.y - A2.y)) / 31 ((B1.x - A1.x)*(B2.y - A2.y) - (B1.y - A1.y)*(B2.x - A2.x)); 32 return A1 + (B1 - A1)*t1; 33 } //返回直线交点 34 35 int main() { 36 Point A, B, C; 37 int n; 38 cin >> n; 39 while (n--) { 40 scanf("%lf%lf%lf%lf%lf%lf", &A.x, &A.y, &B.x, &B.y, &C.x, &C.y); 41 Vector v = Rotate(B - A, PI / 2), u = Rotate(C - B, PI / 2); 42 Point ans = LineIntersectionPoint(C, C + v, A, A + u); 43 printf("%.4lf %.4lf\n", ans.x + EPS, ans.y + EPS); 44 } 45 }
转载于:https://www.cnblogs.com/hyp1231/p/7094273.html
POJ1673 EXOCENTER OF A TRIANGLE(三角形垂心)相关推荐
- poj pku 1673 EXOCENTER OF A TRIANGLE 三角形 垂心
[b][color=blue]题目描述:[/color][/b][url]http://poj.org/problem?id=1673[/url][size=large][/size] 该题重点是求证 ...
- poj1673 EXOCENTER OF A TRIANGLE
地址:http://poj.org/problem?id=1673 题目: EXOCENTER OF A TRIANGLE Time Limit: 1000MS Memory Limit: 100 ...
- POJ 1673 EXOCENTER OF A TRIANGLE(证明+求三角形垂心)
POJ 1673 EXOCENTER OF A TRIANGLE(证明+求三角形垂心) http://poj.org/problem?id=1673 题意: ZOJ 1821 有一个三角形ABC,扩展 ...
- POJ 1673 EXOCENTER OF A TRIANGLE(求三角形的垂心)
博客原文地址:http://blog.csdn.net/xuechelingxiao/article/details/40654421 EXOCENTER OF A TRIANGLE 题目大意:一个三 ...
- OpenGL绘制Triangle三角形
OpenGL绘制Triangle三角形 前期知识准备 顶点输入 顶点着色器 编译着色器 片段着色器 着色器程序 链接顶点属性 顶点数组对象 我们一直期待的三角形 索引缓冲对象 前期知识准备 在Open ...
- OpenGL 9点圆 三角形垂心/垂足 三角形中线
OpenGL 9点圆 三角形垂心/垂足 三角形中线 点此下载: http://rorger.download.csdn.net/ //rorger,2011 //night points circle ...
- POJ 1673 EXOCENTER OF A TRIANGLE(解三角形重心)
题目链接:http://poj.org/problem?id=1673 AC代码: #include<cstdio> #include<cmath> #include<a ...
- 120. Triangle 三角形最小路径和
Title 给定一个三角形,找出自顶向下的最小路径和.每一步只能移动到下一行中相邻的结点上. 相邻的结点 在这里指的是 下标 与 上一层结点下标 相同或者等于 上一层结点下标 + 1 的两个结点. 例 ...
- 粒子群 多目标 matlab_matlab 粒子群求解三角形垂心位置
续 https://www.toutiao.com/i6766960319995576843/ 设定三角形A顶点的坐标为 (x1,y1);(x2,y2);(x3,y3);随机初始化:计算得知垂心到三个 ...
最新文章
- WPF:Documents文档--Annomation批注(3)
- POJ2406简单KMP
- 线程池的使用(线程池重点解析)
- 2017.7.18可变/不可变类型,符号运算及其流程控制
- python 倒叙 数组_Python函数合集:68个内置函数请收好!
- git分支添加访问权限
- 蓝桥杯 ADV-16 算法提高 和最大子序列
- JMX监测JVM报错
- cocos2dx 3.1从零学习(三)——Touch事件(回调,反向传值)
- 高效管理CrossOver容器里的程序
- HDU1054 Strategic Game —— 最小点覆盖 or 树形DP
- PS使用:windows解决Adobe Photoshop 2020(PS2020)闪退
- (java)五大常用算法
- 加速Web开发的9款知名HTML5框架
- 聊天类APP的测试点
- 知识兔课程揭秘2021抖音卖货代运营的新骗局,你中招了吗?
- 2016杭州云栖大会回顾网址
- 初级程序员与高级程序员
- 锐捷交换机删除vlan
- Linux信号量 sem_t简介
热门文章
- xp打印机驱动安装不了_解决Windows XP安装过程中的“安装程序找不到任何硬盘驱动器”
- java sleep异常_sleep方法要求处理中断异常:InterruptedException
- Codeforces 1263D(Secret Passwords )
- 放下助人情结,尊重他人命运
- VBA提高篇_16 传递Range提升自定义函数,巧用属分辨公式型内容
- 手机屏幕常见故障_【最新推荐】手机触摸屏常见问题及解决方法-范文模板 (3页)...
- html实现富文本编辑器,前端程序员福利,6款轻量级富文本编辑器,轻松实现富文本编辑...
- Spring Validation及消息国际化.md
- 威马汽车:跃马扬鞭未竟,鞍马劳顿难行?
- 如何搭建一个属于自己的网站(使用宝塔面板)