ruby写的BT种子解析器
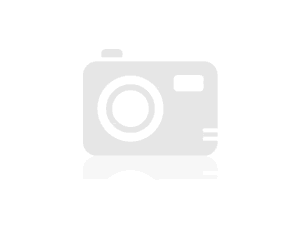
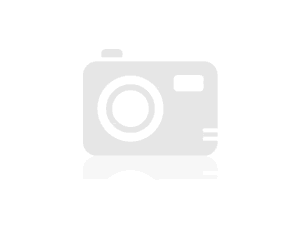
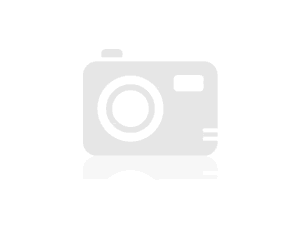
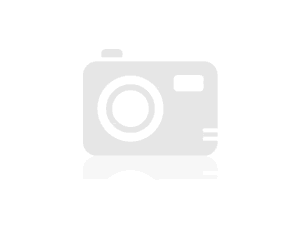
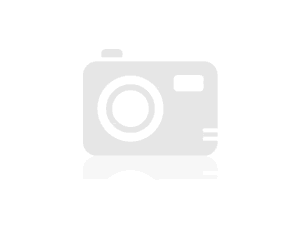
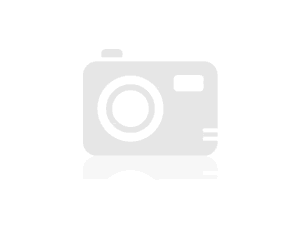
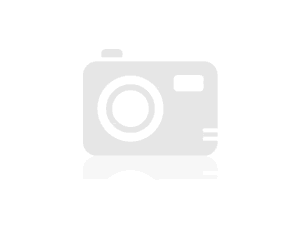
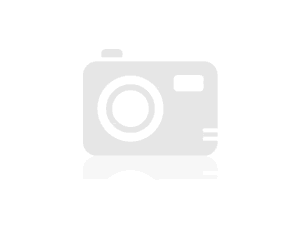
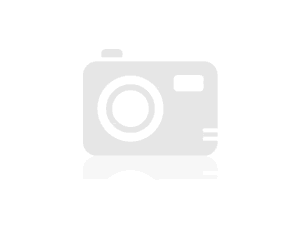
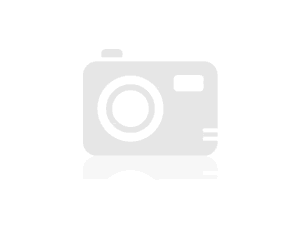
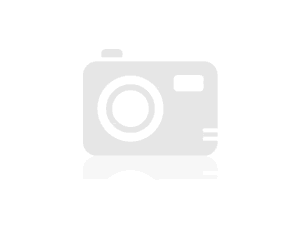
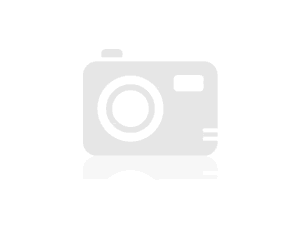
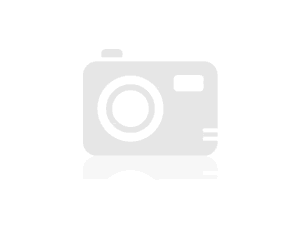
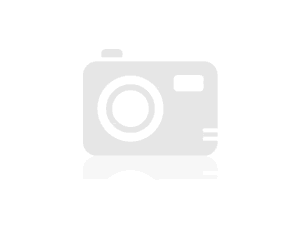
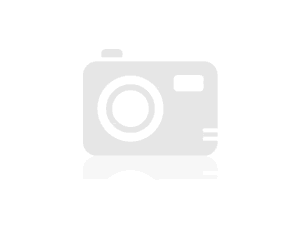
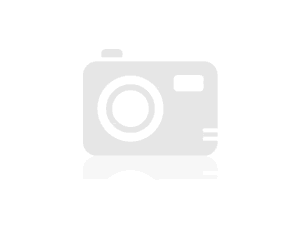
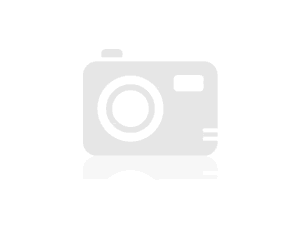
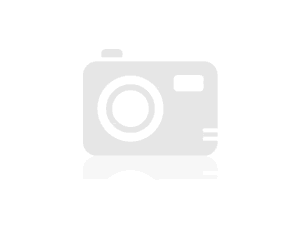
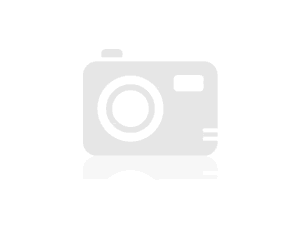
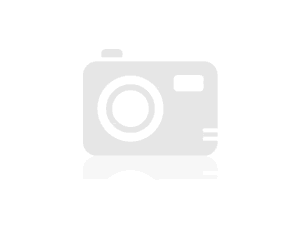
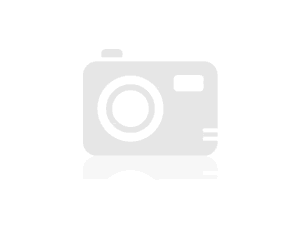
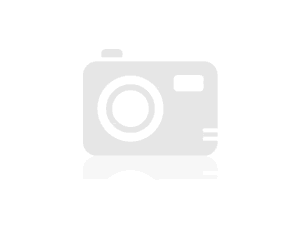
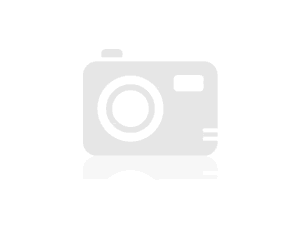
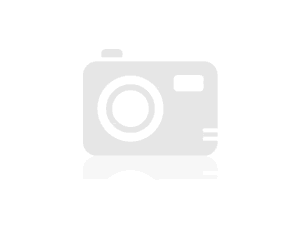
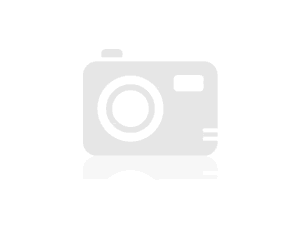
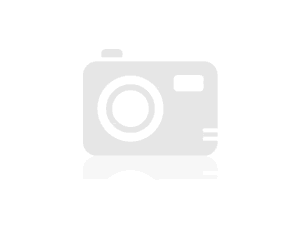
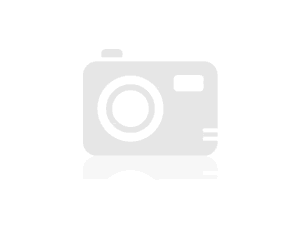
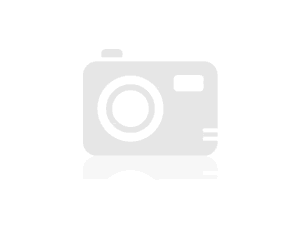
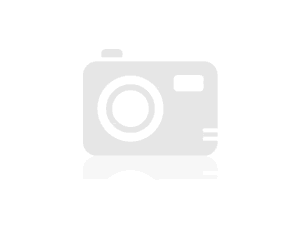
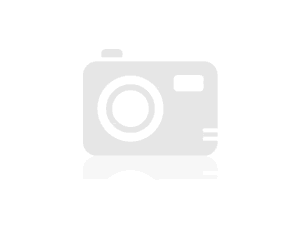
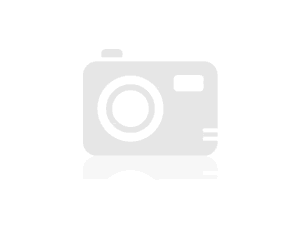
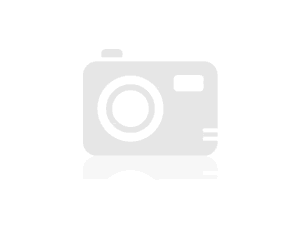
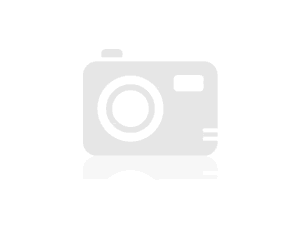
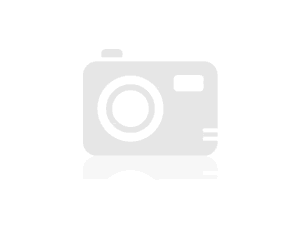
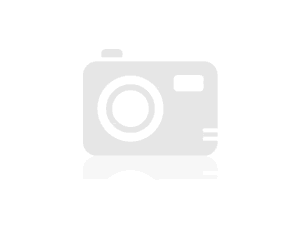
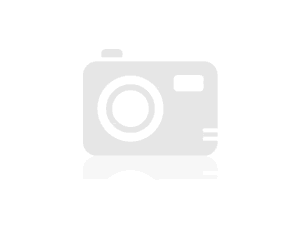
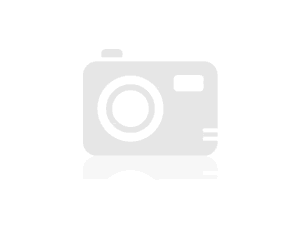
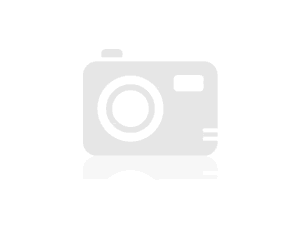
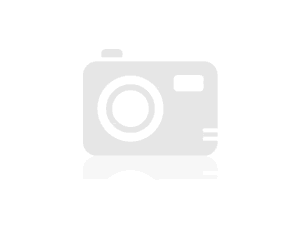
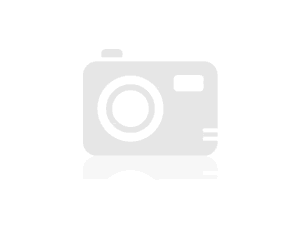
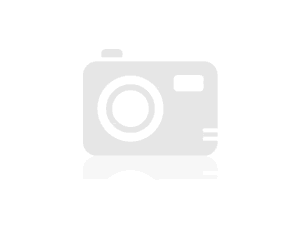
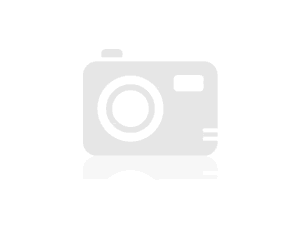
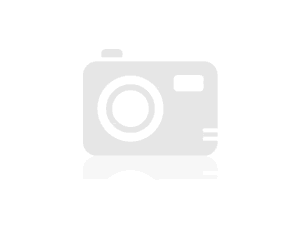
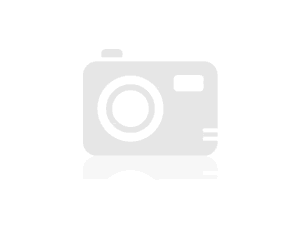
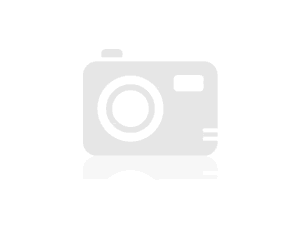
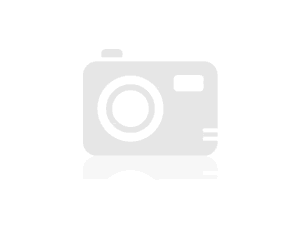
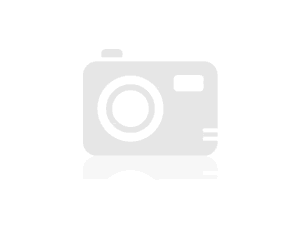
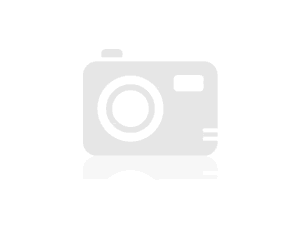
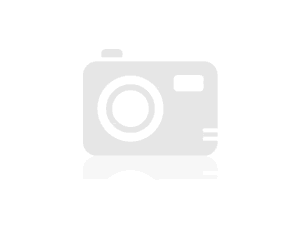
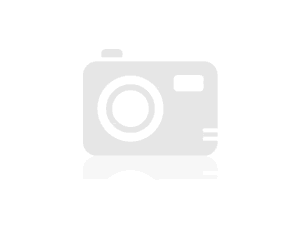
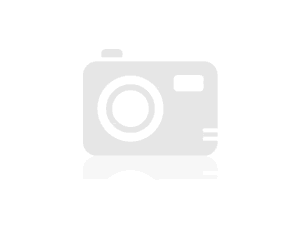
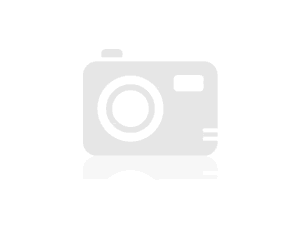
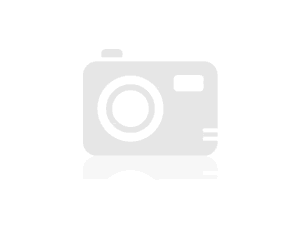
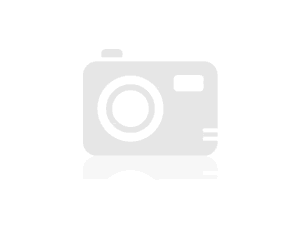
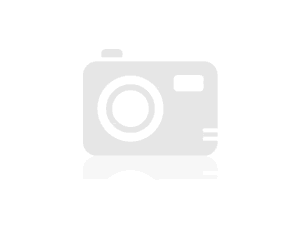
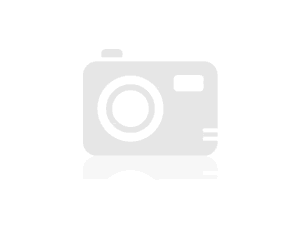
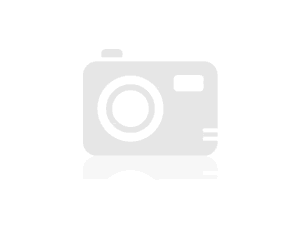
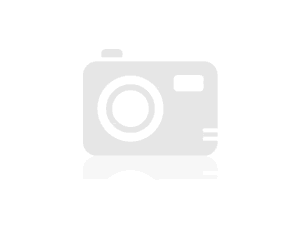
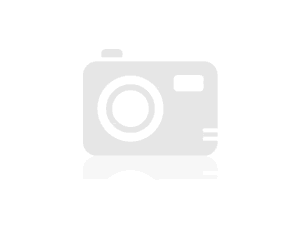
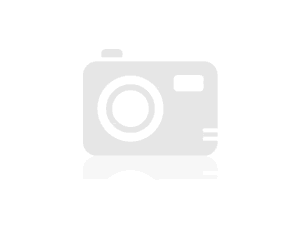
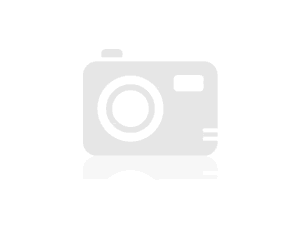
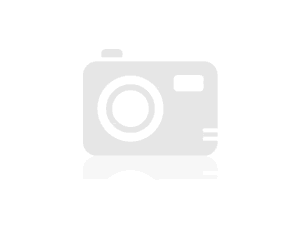
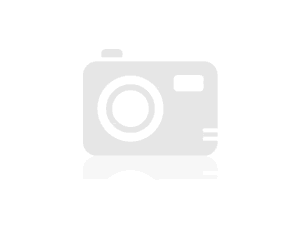
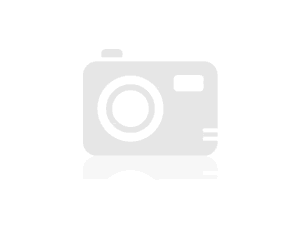
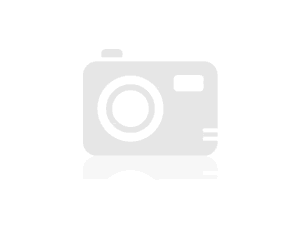
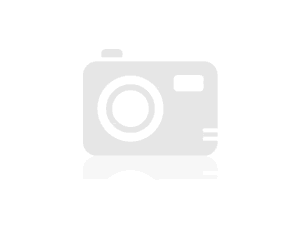
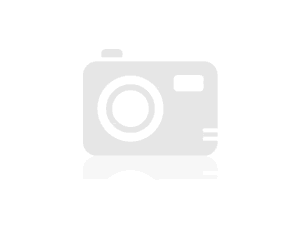
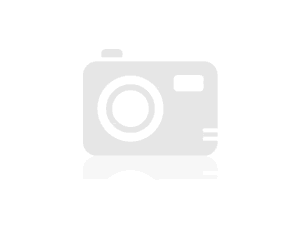
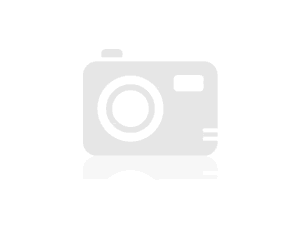
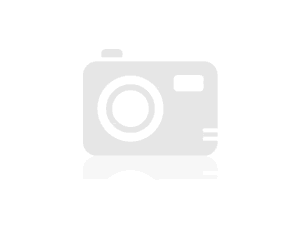
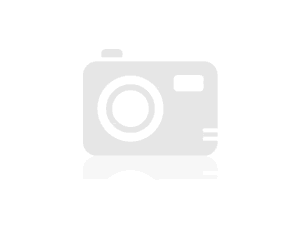
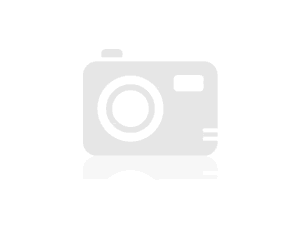
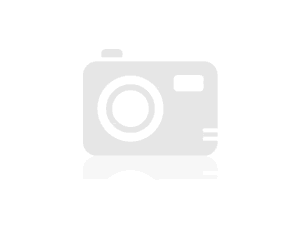
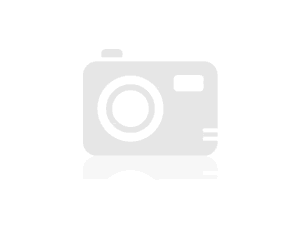
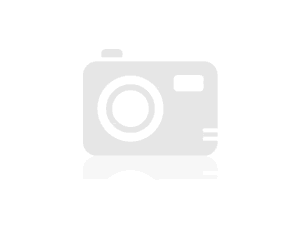
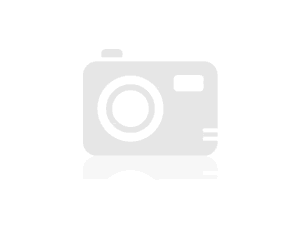
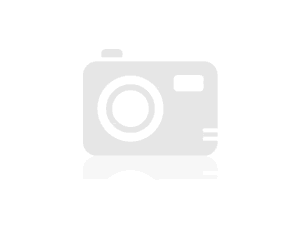
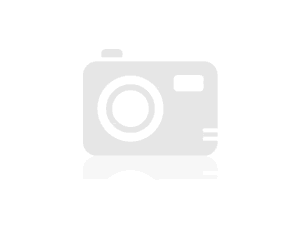
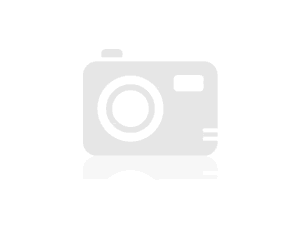
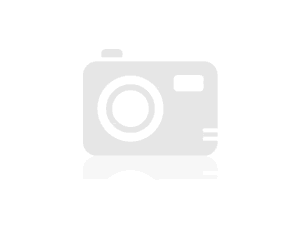
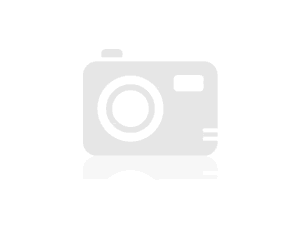
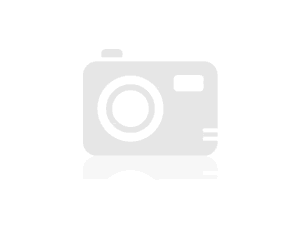
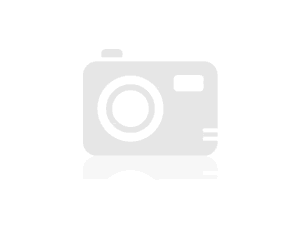
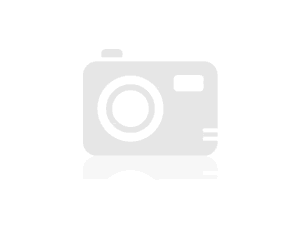
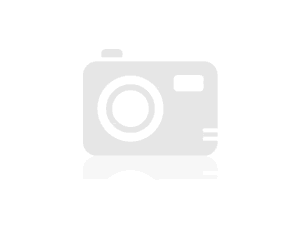
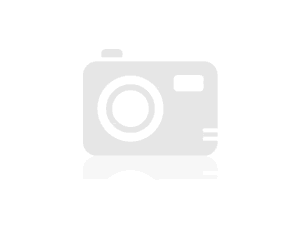
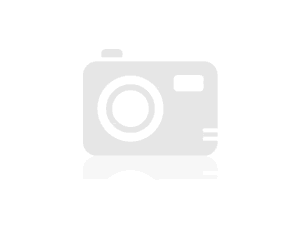
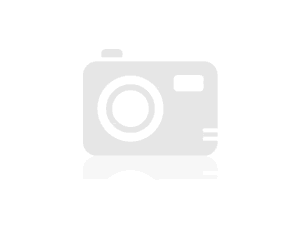
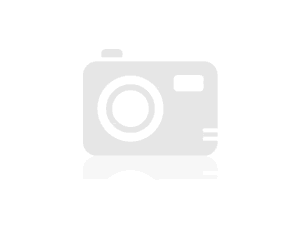
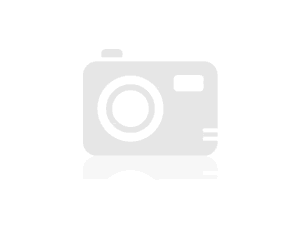
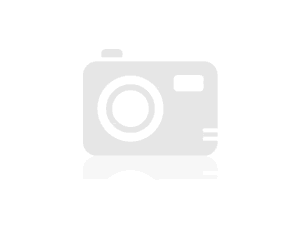
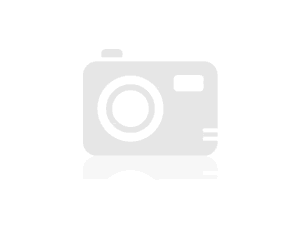
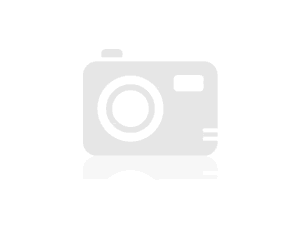
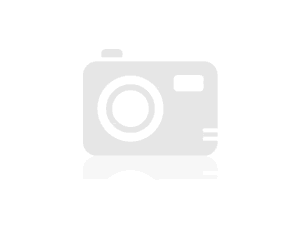
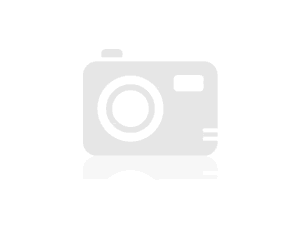
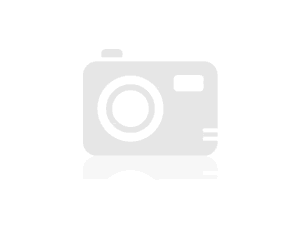
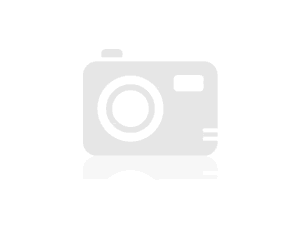
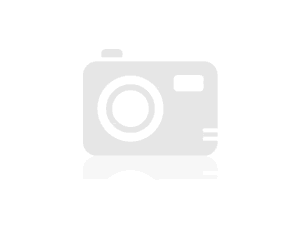
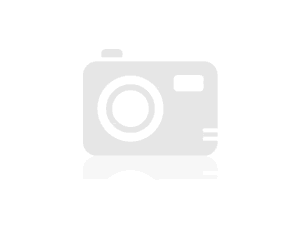
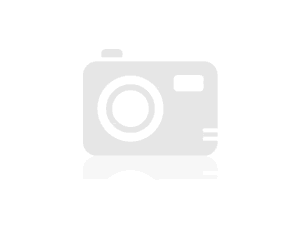
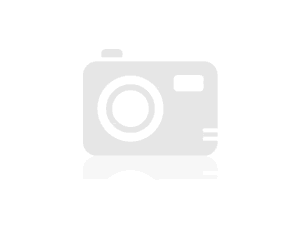
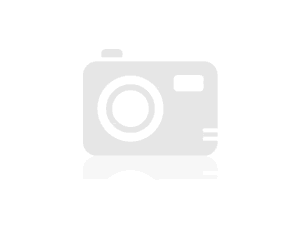
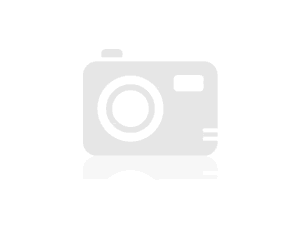
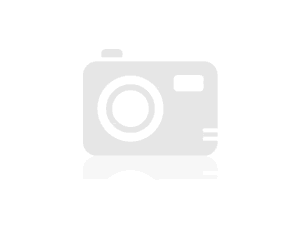
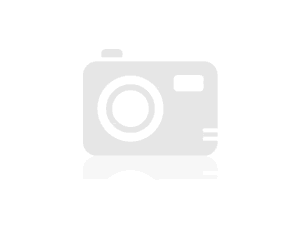
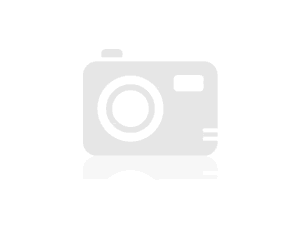
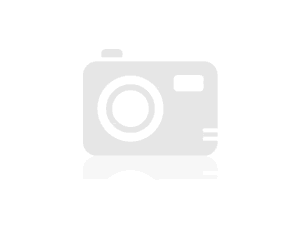
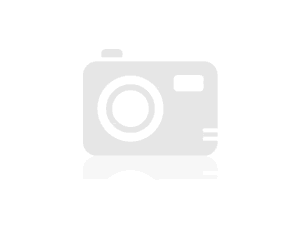
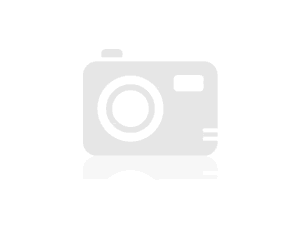
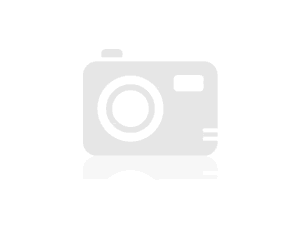
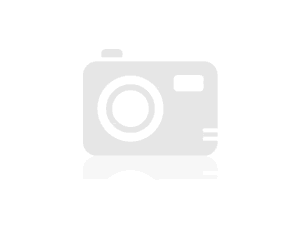
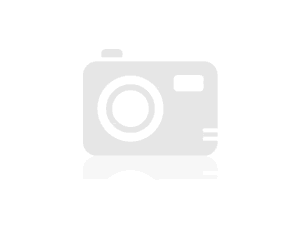
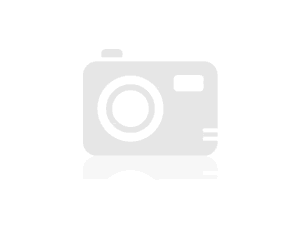
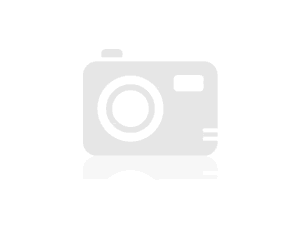
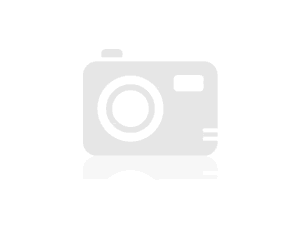
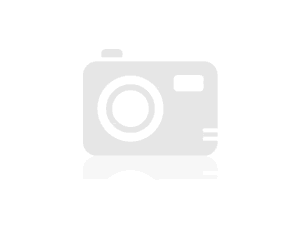
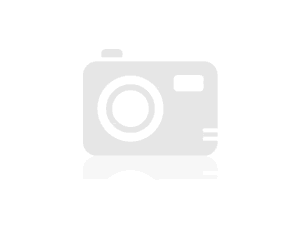
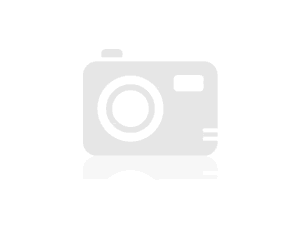
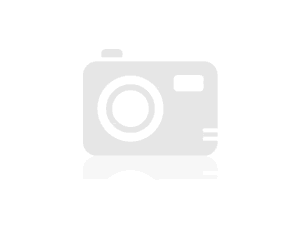
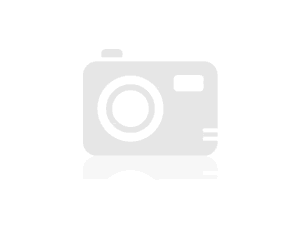
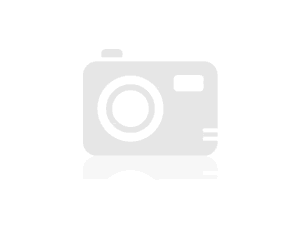
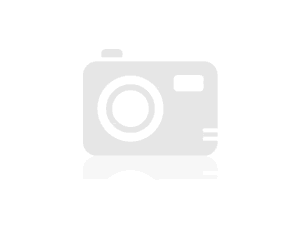
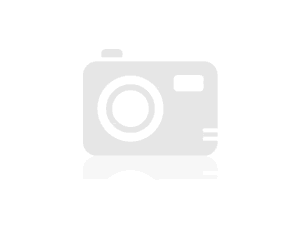
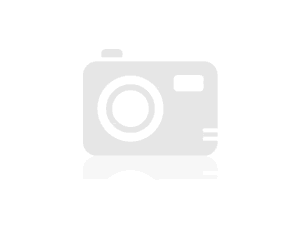
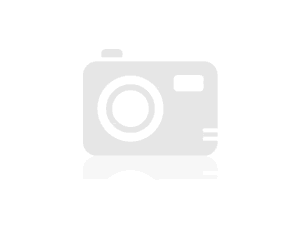
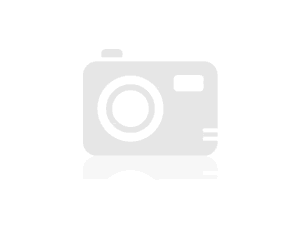
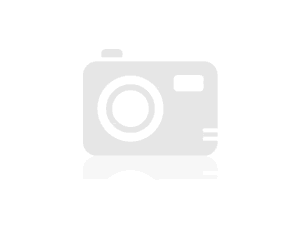
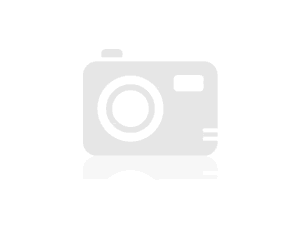
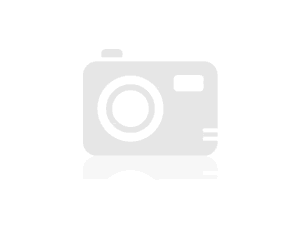
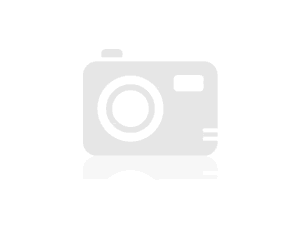
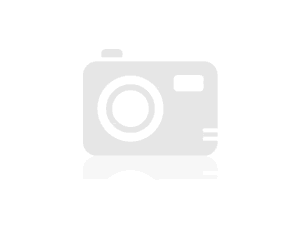
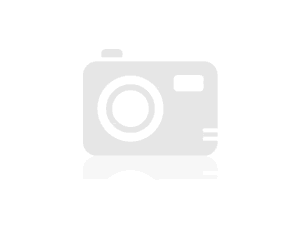
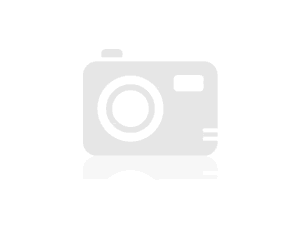
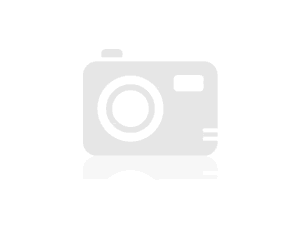
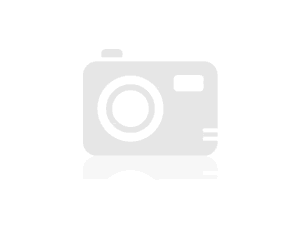
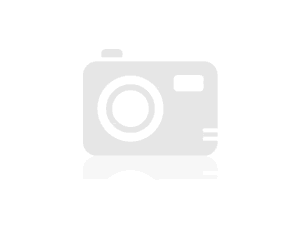
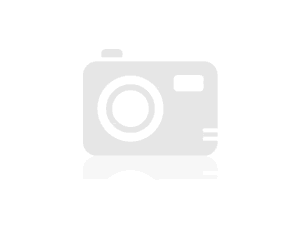
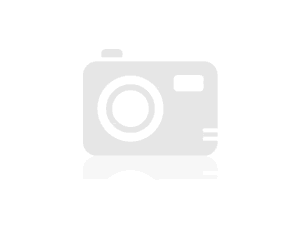
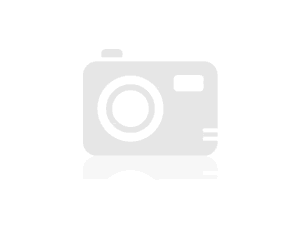
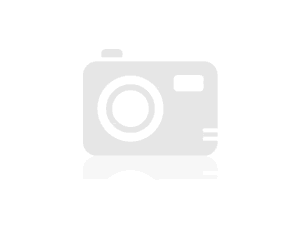
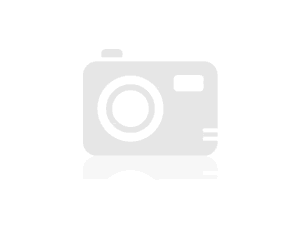
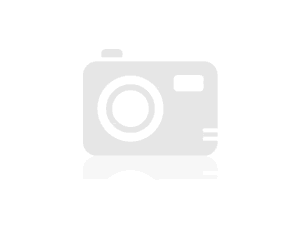
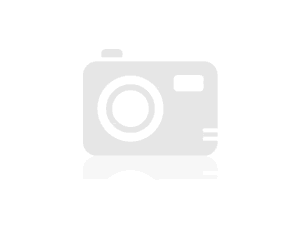
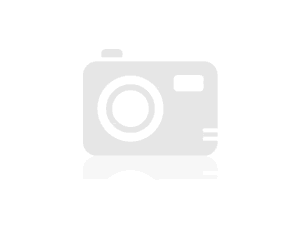
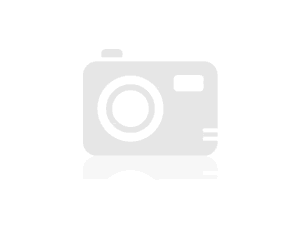
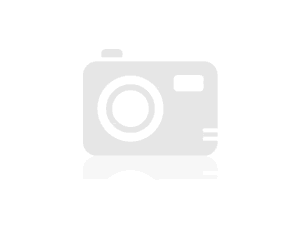
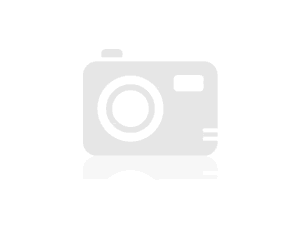
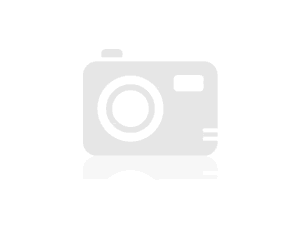
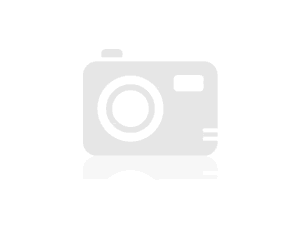
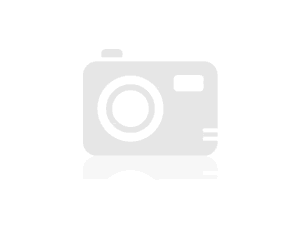
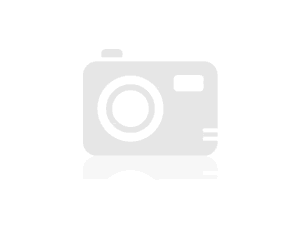
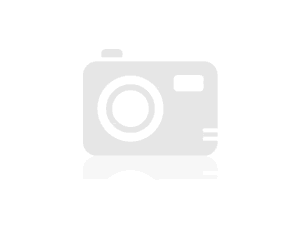
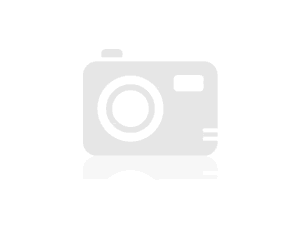
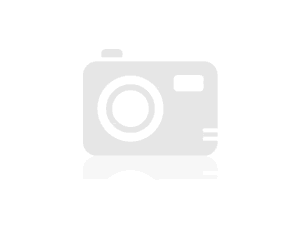
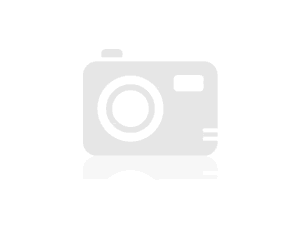
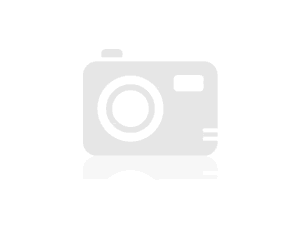
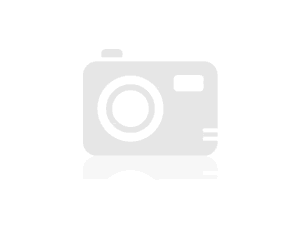
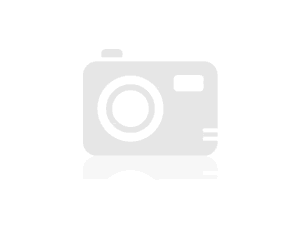
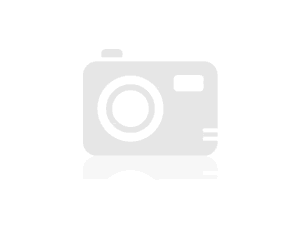
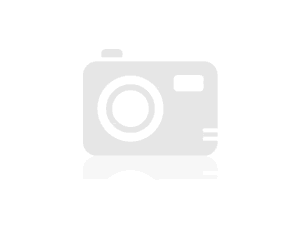
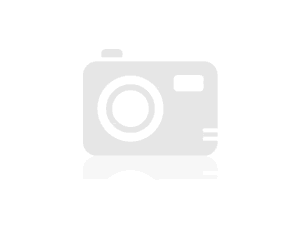
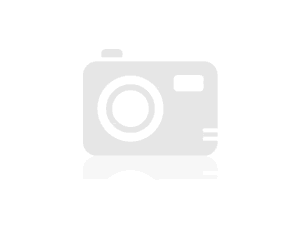
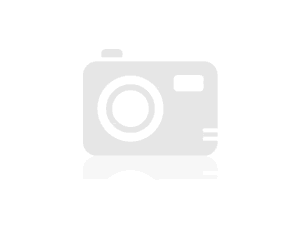
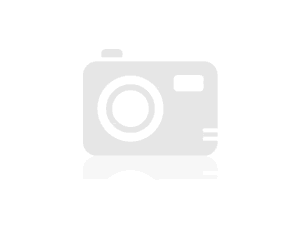
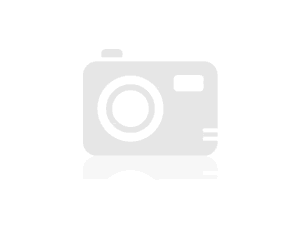
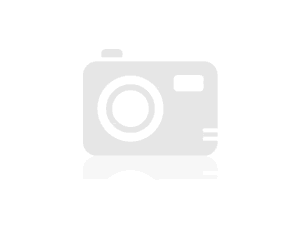
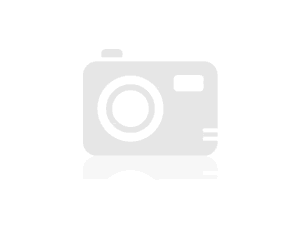
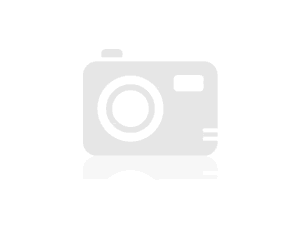
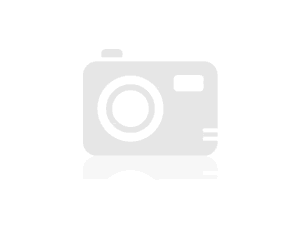
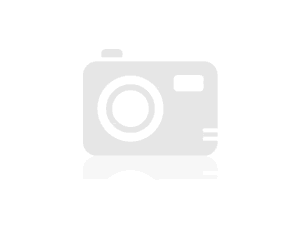
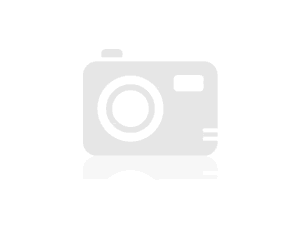
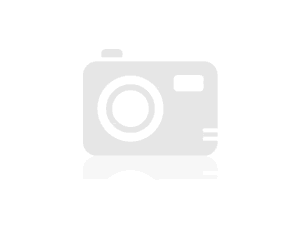
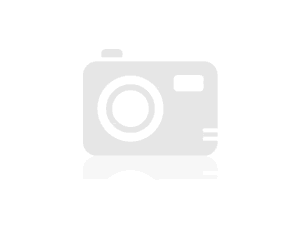
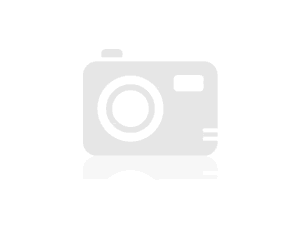
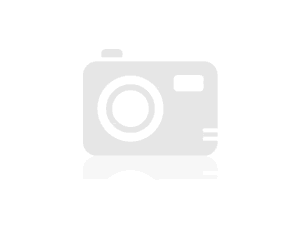
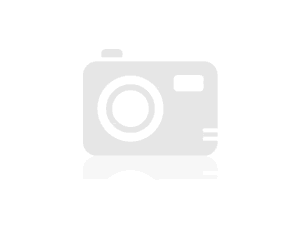
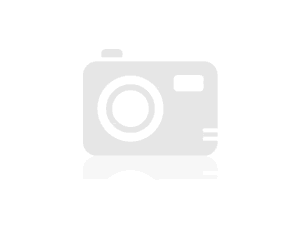
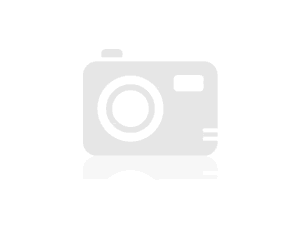
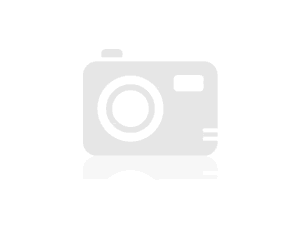
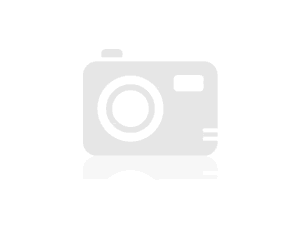
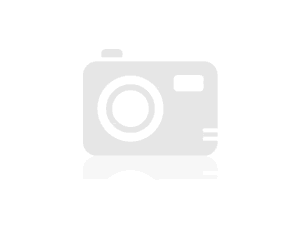
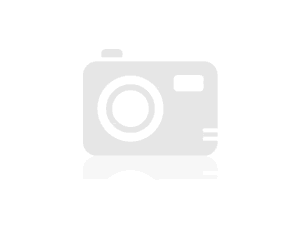
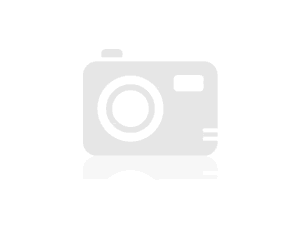
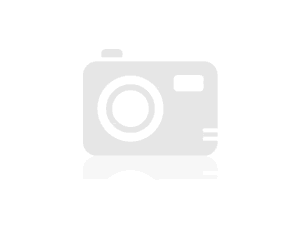
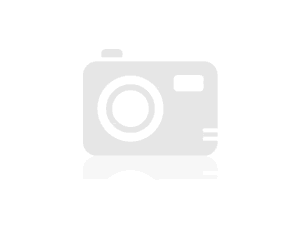
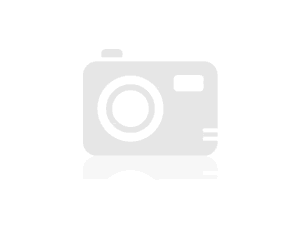
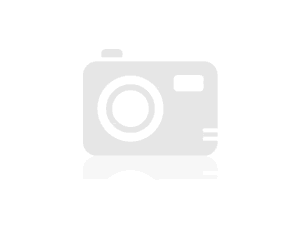
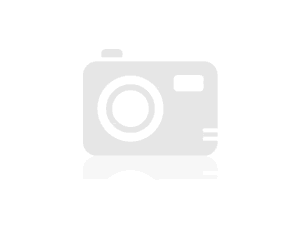
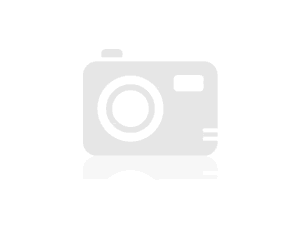
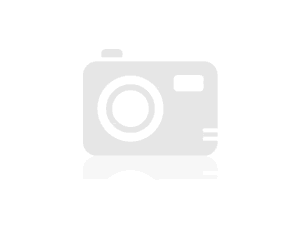
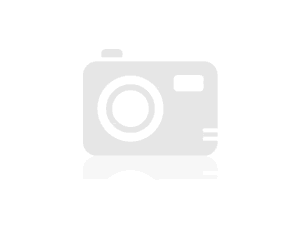
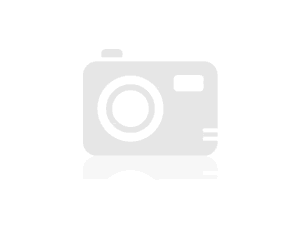
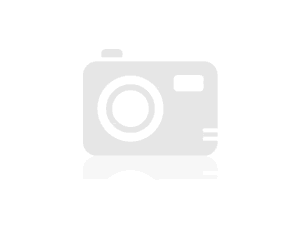
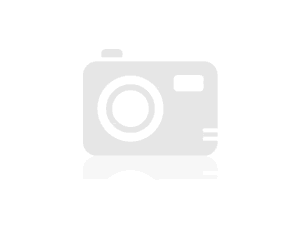
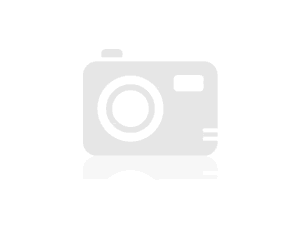
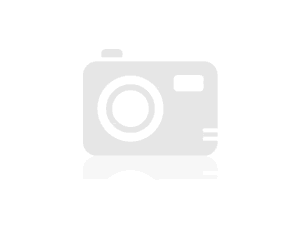
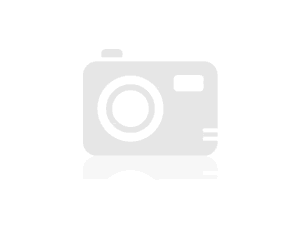
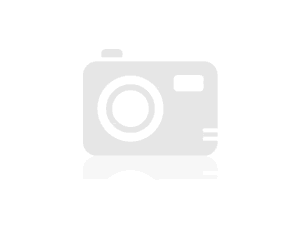
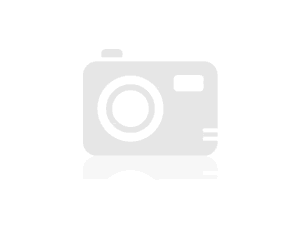
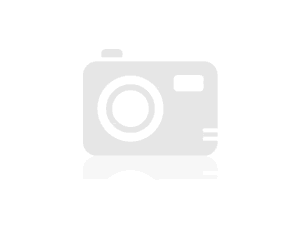
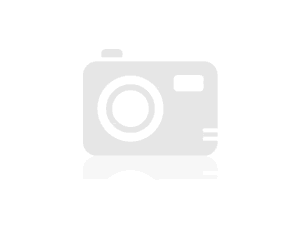
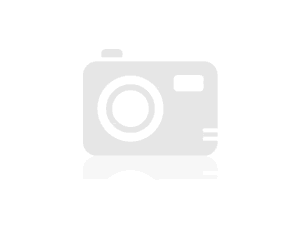
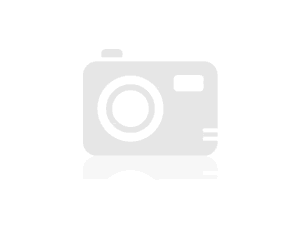
转载于:https://www.cnblogs.com/coderlee/archive/2008/04/08/1142788.html
ruby写的BT种子解析器相关推荐
- B编码与BT种子文件分析,以及模仿json-cpp写一个B编码解析器
B编码与BT种子文件分析,以及模仿json-cpp写一个B编码解析器 1.什么是B编码 2.B编码格式 3.种子文件结构 3.1.主文件结构 3.2.info结构 4.简单的例子了解一下种子文件和B编 ...
- bt种子播放器WebTorrent for Mac使用方法
WebTorrent for Mac这是一款Mac平台上非常好用的bt种子在线播放器,支持种子和边播放边下载.WebTorrent Mac版不仅可以用来BT下载,还可以边下边播,使用简单,拖动种子文件 ...
- 自己写的简单xml解析器
已经自我放逐好几年了.打算去上班得了.在最后的自由日子里,做点有意义的事吧... 先来下载地址 http://www.kuaipan.cn/file/id_12470514853353274.htm ...
- python 正则表达式语法大全_Python 之父撰文回忆:为什么要创造 pgen 解析器?
花下猫语: 近日,Python 之父在 Medium 上开通了博客,并发布了一篇关于 PEG 解析器的文章(参见我翻的 全文译文).据我所知,他有自己的博客,为什么还会跑去 Medium 上写文呢?好 ...
- 【LLM】Langchain使用[一](模型、提示和解析器、存储)
note 文章目录 note 零.LangChain介绍 一.模型.提示和解析器 1. 提示 2. 使用langchain写提示 3. 输出解析器 4. 链式思考推理(ReAct) 二.存储 1. 对 ...
- Go语言写的解析器(支持json,linq,sql,net,http等)
Monkey程序语言 Monkey v2.0版本已发布. monkey v2.0 增加了如下内容: 新增 short arrow(->)支持(类似C#的lambda表达式) 增加 列表推导和哈希 ...
- # 解析bt文件_磁力链接和BT种子使用方法
目前用的最多的是磁力链接和BT种子,不过好多人并不太会使用,因此写个教程给大家说明一下. 何为磁力链接:简单地说,磁力链接是一种特殊链接,但是它与传统基于文件的位置或名称的普通链接(如http://x ...
- python 接口测试 如何写配置文件_python接口自动化测试 - configparser配置文件解析器详细使用...
configparser简介 ConfigParser模块已在Python 3中重命名为configparser 该模块定义了ConfigParser类. ConfigParser类实现一种基本的配置 ...
- string 转 json_手写Json解析器学习心得
哦?从"{"开始,看来是个对象了! 一. 介绍 一周前,老同学阿立给我转了一篇知乎回答,答主说检验一门语言是否掌握的标准是实现一个Json解析器,网易游戏过去的Python入门培训 ...
- 手写token解析器、语法解析器、LLVM IR生成器(GO语言)
最近开始尝试用go写点东西,正好在看LLVM的资料,就写了点相关的内容 - 前端解析器+中间代码生成(本地代码的汇编.执行则靠LLVM工具链完成) https://github.com/daibinh ...
最新文章
- 股市币市:数据分析与交易所最新公告(20190302)
- zabbix系列~ 监控模式
- 宏基因组报名倒计时!报名线上课还可免费参加线下课
- ubuntu安装使用不同版本的gcc
- FW : 一只小青蛙的一生(图片连载)
- Maven---学习心得---maven的Dependency Mechanism(依赖关系机制)
- 图像局部显著性—点特征(Fast)
- mysql 全局不重复_php uniqid() 通过MYSQL实现全局不重复的唯一ID
- C语言课后习题(60)
- 微信电脑版重大更新,可以上班刷朋友圈摸鱼了
- SpringBoot实现抽奖大转盘
- 如何做好一场技术分享,100%纯实用技巧输出
- java碰撞检测代码_java 实现精确碰撞检测。
- 保镖机器人作文_暴力“保镖”作文800字
- 大中型 UGC 平台的反垃圾(anti-spam)工作
- 零基础微信小程序开发学习笔记(2.构建静态界面)
- 不怕加班狗有情绪,就怕加班狗有“武器”
- 深度学习模型轻量化(上)
- 2023年长沙Java培训机构排名前十新鲜出炉!有你心仪的机构吗?
- 昨夜西风凋碧树,独上高楼,望尽天涯路
热门文章
- Linux for Matlab中文注释乱码(亲测有效)
- sklearn 自定义函数转化器FunctionTransformer使用
- python基于scipy拟合构建所需统计分析模型,可视化分析展示
- 用PBD制作餐饮店KPI分析仪-入门篇
- 数据库事务 写偏斜write-skew
- 数据库学习笔记5-隔离级别 Repeatable Read
- Mac如何删除python Python cannot be opened because of a problem
- 基于SSM的教学质量系统
- 常见linux服务器存储空间,全面了解linux服务器的常用命令总结
- 华为 台积电 高通申请_华为表态愿意合作,台积电送来“神助攻”,高通:我太难了...