t–sql pl–sql_SQL View –完整的介绍和演练
t–sql pl–sql
In relational databases, data is structured using various database objects like tables, stored procedure, views, clusters etc. This article aims to walk you through ‘SQL VIEW’ – one of the widely-used database objects in SQL Server.
在关系数据库中,数据是使用各种数据库对象(例如表,存储过程,视图,群集等)构造的。本文旨在引导您了解“ SQL VIEW”(SQL Server中广泛使用的数据库对象之一)。
It is a good practice to organize tables in a database to reduce redundancy and dependency in SQL database. Normalization is a database process for organizing the data in the database by splitting large tables into smaller tables. These multiple tables are linked using the relationships. Developers write queries to retrieve data from multiple tables and columns. In the query, we might use multiple joins and queries could become complicated and overwhelming to understand. Users should also require permissions on individual objects to fetch the data.
最好在数据库中组织表以减少SQL数据库中的冗余和依赖性。 规范化是一个数据库过程,用于通过将大型表拆分为较小的表来组织数据库中的数据。 这些多个表使用关系进行链接。 开发人员编写查询以从多个表和列中检索数据。 在查询中,我们可能使用多个联接,并且查询可能变得复杂且难以理解。 用户还应该要求对单个对象具有权限才能获取数据。
Let’s go ahead and see how SQL VIEW help to resolve these issues in SQL Server.
让我们继续看看SQL VIEW如何帮助解决SQL Server中的这些问题。
介绍 (Introduction )
A VIEW in SQL Server is like a virtual table that contains data from one or multiple tables. It does not hold any data and does not exist physically in the database. Similar to a SQL table, the view name should be unique in a database. It contains a set of predefined SQL queries to fetch data from the database. It can contain database tables from single or multiple databases as well.
SQL Server中的VIEW就像一个虚拟表,其中包含一个或多个表中的数据。 它不保存任何数据,也不存在于数据库中。 与SQL表类似,视图名称在数据库中应该唯一。 它包含一组预定义SQL查询,以从数据库中获取数据。 它也可以包含来自单个或多个数据库的数据库表。
In the following image, you can see the VIEW contains a query to join three relational tables and fetch the data in a virtual table.
在下图中,您可以看到VIEW包含一个查询以连接三个关系表并获取虚拟表中的数据。
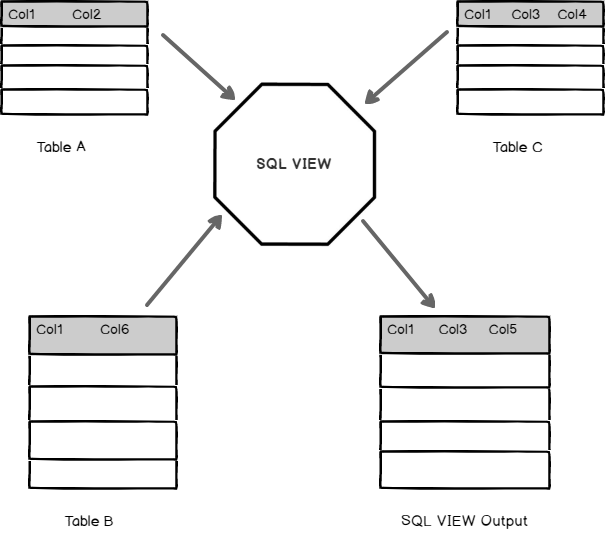
A VIEW does not require any storage in a database because it does not exist physically. In a VIEW, we can also control user security for accessing the data from the database tables. We can allow users to get the data from the VIEW, and the user does not require permission for each table or column to fetch data.
VIEW不需要数据库中的任何存储,因为它实际上并不存在。 在VIEW中,我们还可以控制用户安全性以访问数据库表中的数据。 我们可以允许用户从VIEW获取数据,并且用户不需要每个表或列的权限即可获取数据。
Let’s explore user-defined VIEW in SQL Server.
让我们探索SQL Server中用户定义的VIEW。
Note: In this article, I am going to use sample database AdventureWorks for all examples.
注意:在本文中,我将为所有示例使用示例数据库AdventureWorks 。
创建一个SQL视图 (Create a SQL VIEW)
The syntax to create a VIEW is as follows:
创建VIEW的语法如下:
CREATE VIEW Name AS
Select column1, Column2...Column N From tables
Where conditions;
示例1:SQL VIEW获取表的所有记录 (Example 1: SQL VIEW to fetch all records of a table)
It is the simplest form of a VIEW. Usually, we do not use a VIEW in SQL Server to fetch all records from a single table.
它是VIEW的最简单形式。 通常,我们不使用SQL Server中的VIEW从单个表中获取所有记录。
CREATE VIEW EmployeeRecords
ASSELECT *FROM [HumanResources].[Employee];
Once a VIEW is created, you can access it like a SQL table.
创建VIEW后,您可以像访问SQL表一样对其进行访问。

示例2:SQL VIEW来获取表的几列 (Example 2: SQL VIEW to fetch a few columns of a table)
We might not be interested in all columns of a table. We can specify required column names in the select statement to fetch those fields only from the table.
我们可能对表的所有列都不感兴趣。 我们可以在select语句中指定所需的列名称,以仅从表中获取这些字段。
CREATE VIEW EmployeeRecords
ASSELECT NationalIDNumber,LoginID,JobTitle FROM [HumanResources].[Employee];
示例3:SQL VIEW获取表的几列并使用WHERE子句过滤结果 (Example 3: SQL VIEW to fetch a few columns of a table and filter results using WHERE clause)
We can filter the results using a Where clause condition in a Select statement. Suppose we want to get EmployeeRecords with Martial status ‘M’.
我们可以在Select语句中使用Where子句条件过滤结果。 假设我们要获取武术状态为“ M”的EmployeeRecords。
CREATE VIEW EmployeeRecords
ASSELECT NationalIDNumber, LoginID, JobTitle, MaritalStatusFROM [HumanResources].[Employee]WHERE MaritalStatus = 'M';
示例4:SQL VIEW从多个表中获取记录 (Example 4: SQL VIEW to fetch records from multiple tables)
We can use VIEW to have a select statement with Join condition between multiple tables. It is one of the frequent uses of a VIEW in SQL Server.
我们可以使用VIEW在多个表之间使用带有Join条件的select语句。 它是SQL Server中VIEW的常用用法之一。
In the following query, we use INNER JOIN and LEFT OUTER JOIN between multiple tables to fetch a few columns as per our requirement.
在以下查询中,我们根据需要在多个表之间使用INNER JOIN和LEFT OUTER JOIN来获取几列。
CREATE VIEW [Sales].[vStoreWithContacts]
ASSELECT s.[BusinessEntityID], s.[Name], ct.[Name] AS [ContactType], p.[Title], p.[FirstName], p.[MiddleName], p.[LastName], p.[Suffix], pp.[PhoneNumber], ea.[EmailAddress], p.[EmailPromotion]FROM [Sales].[Store] sINNER JOIN [Person].[BusinessEntityContact] bec ON bec.[BusinessEntityID] = s.[BusinessEntityID]INNER JOIN [Person].[ContactType] ct ON ct.[ContactTypeID] = bec.[ContactTypeID]INNER JOIN [Person].[Person] p ON p.[BusinessEntityID] = bec.[PersonID]LEFT OUTER JOIN [Person].[EmailAddress] ea ON ea.[BusinessEntityID] = p.[BusinessEntityID]LEFT OUTER JOIN [Person].[PersonPhone] pp ON pp.[BusinessEntityID] = p.[BusinessEntityID];
GO
Suppose you need to execute this query very frequently. Using a VIEW, we can simply get the data with a single line of code.
假设您需要非常频繁地执行此查询。 使用VIEW,我们只需用一行代码即可获取数据。
select * from [Sales].[vStoreWithContacts]
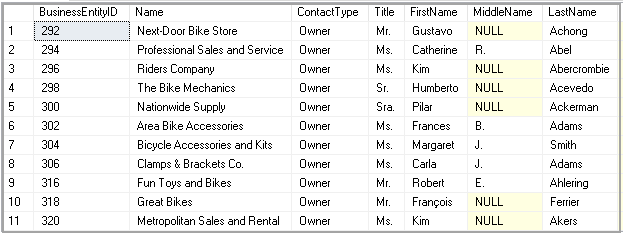
示例5:SQL VIEW获取特定列 (Example 5: SQL VIEW to fetch specific column )
In the previous example, we created a VIEW with multiple tables and a few column from those tables. Once we have a view, it is not required to fetch all columns from the view. We can select few columns as well from a VIEW in SQL Server similar to a relational table.
在前面的示例中,我们创建了一个VIEW,其中包含多个表以及这些表中的几列。 一旦有了视图,就不需要从视图中获取所有列。 我们也可以从SQL Server的VIEW中选择一些与关系表类似的列。
In the following query, we want to get only two columns name and contract type from the view.
在以下查询中,我们只想从视图中获取两列名称和合同类型。
SELECT Name, ContactType
FROM [Sales].[vStoreWithContacts];
示例6:使用Sp_helptext检索VIEW定义 (Example 6: Use Sp_helptext to retrieve VIEW definition)
We can use sp_helptext system stored procedure to get VIEW definition. It returns the complete definition of a SQL VIEW.
我们可以使用sp_helptext系统存储过程来获取VIEW定义。 它返回SQL VIEW的完整定义。
For example, let’s check the view definition for EmployeeRecords VIEW.
例如,让我们检查EmployeeRecords VIEW的视图定义。

We can use SSMS as well to generate the script for a VIEW. Expand database -> Views -> Right click and go to Script view as -> Create To -> New Query Editor Window.
我们也可以使用SSMS为VIEW生成脚本。 展开数据库->视图->右键单击,然后以->创建到->新建查询编辑器窗口转到脚本视图。

示例7:sp_refreshview更新SQL VIEW的元数据 (Example 7: sp_refreshview to update the Metadata of a SQL VIEW)
Suppose we have a VIEW on a table that specifies select * statement to get all columns of that table.
假设我们在一个表上有一个VIEW,该表指定select *语句以获取该表的所有列。
CREATE VIEW DemoView
ASSELECT *FROM [AdventureWorks2017].[dbo].[MyTable];
Once we call the VIEW DemoView, it gives the following output.
一旦我们调用VIEW DemoView,它就会给出以下输出。

Let’s add a new column in the table using the Alter table statement.
让我们使用Alter table语句在表中添加一个新列。
Alter Table [AdventureWorks2017].[dbo].[MyTable] Add City nvarchar(50)
Rerun the select statement to get records from VIEW. It should display the new column as well in the output. We still get the same output, and it does not contain the newly added column.
重新运行select语句以从VIEW获取记录。 它也应该在输出中显示新列。 我们仍然得到相同的输出,并且其中不包含新添加的列。

By Default, SQL Server does not modify the schema and metadata for the VIEW. We can use the system stored procedure sp_refreshview to refresh the metadata of any view.
默认情况下,SQL Server不会修改VIEW的架构和元数据。 我们可以使用系统存储过程sp_refreshview刷新任何视图的元数据。
Exec sp_refreshview DemoView
Rerun the select statement to get records from VIEW. We can see the City column in the output.
重新运行select语句以从VIEW获取记录。 我们可以在输出中看到“城市”列。

示例8:模式绑定SQL视图 (Example 8: Schema Binding a SQL VIEW)
In the previous example, we modify the SQL table to add a new column. Suppose in the production instance, and you have a view in the application. You are not aware of the changes in the table design for the new column. We do not want any changes to be made in the tables being used in the VIEW. We can use SCHEMABINDING option to lock all tables used in the VIEW and deny any alter table statement against those tables.
在前面的示例中,我们修改了SQL表以添加新列。 假设在生产实例中,您在应用程序中有一个视图。 您不知道新列的表设计中的更改。 我们不希望在VIEW中使用的表中进行任何更改。 我们可以使用SCHEMABINDING选项锁定VIEW中使用的所有表,并针对这些表拒绝任何alter table语句。
Let’s execute the following query with an option SCHEMABINDING.
让我们使用SCHEMABINDING选项执行以下查询。
CREATE VIEW DemoView
WITH SCHEMABINDING
ASSELECT *FROM [AdventureWorks2017].[dbo].[MyTable];
It gives an error message.
它给出一个错误信息。
Msg 1054, Level 15, State 6, Procedure DemoView, Line 4 [Batch Start Line 2] Syntax ‘*’ is not allowed in schema-bound objects.
消息1054,级别15,状态6,过程DemoView,第4行[批处理开始第2行]架构绑定的对象中不允许使用语法'*'。
We cannot call all columns (Select *) in a VIEW with SCHEMABINDING option. Let’s specify the columns in the following query and execute it again.
我们无法在带有SCHEMABINDING选项的VIEW中调用所有列(选择*)。 让我们在以下查询中指定列,然后再次执行。
CREATE VIEW DemoView
WITH SCHEMABINDING
ASSELECT TableID, ForeignID ,Value, CodeOneFROM [AdventureWorks2017].[dbo].[MyTable];
We again get the following error message.
我们再次收到以下错误消息。
Msg 4512, Level 16, State 3, Procedure DemoView, Line 5 [Batch Start Line 1] Cannot schema bind VIEW ‘DemoView’ because of the name ‘AdventureWorks2017.dbo.MyTable’ is invalid for schema binding. Names must be in a two-part format, and an object cannot reference itself.
消息4512,级别16,状态3,过程DemoView,第5行[批处理开始第1行]由于名称'AdventureWorks2017.dbo.MyTable'对架构绑定无效,因此无法进行架构绑定VIEW'DemoView'。 名称必须采用两部分格式,并且对象不能引用自身。
In my query, I used a three-part object name in the format [DBName.Schema.Object]. We cannot use this format with SCHEMABINDING option in a VIEW. We can use the two-part name as per the following query.
在查询中,我使用了三部分的对象名称,格式为[DBName.Schema.Object]。 我们不能在VIEW中将此格式与SCHEMABINDING选项一起使用。 我们可以根据以下查询使用由两部分组成的名称。
CREATE VIEW DemoView
WITH SCHEMABINDING
ASSELECT TableID, ForeignID ,Value, CodeOneFROM [dbo].[MyTable];
Once you have created a VIEW with SCHEMABINDING option, try to add a modify a column data type using Alter table command.
创建带有SCHEMABINDING的VIEW选项后,尝试使用Alter table命令添加修改列数据类型。
ALTER TABLE dbo.MyTable ALTER COLUMN ForeignID BIGINT;

We need to drop the VIEW definition itself along with other dependencies on that table before making a change to the existing table schema.
在更改现有表架构之前,我们需要删除VIEW定义本身以及该表上的其他依赖项。
示例8:SQL VIEW ENCRYPTION (Example 8: SQL VIEW ENCRYPTION)
We can encrypt the VIEW using the WITH ENCRYPTION clause. Previously, we checked users can see the view definition using the sp_helptext command. If we do not want users to view the definition, we can encrypt it.
我们可以使用WITH ENCRYPTION子句对VIEW进行加密。 以前,我们检查用户是否可以使用sp_helptext命令查看视图定义。 如果我们不希望用户查看该定义,则可以对其进行加密。
CREATE VIEW DemoView
WITH ENCRYPTION
ASSELECT TableID, ForeignID ,Value, CodeOneFROM [dbo].[MyTable];
Now if you run the sp_helptext command to view the definition, you get the following error message.
现在,如果运行sp_helptext命令以查看定义,则会收到以下错误消息。
Exec sp_helptext DemoView
The text for the object ‘DemoView’ is encrypted.
对象“ DemoView”的文本已加密。
示例9:DML(更新,删除和插入)查询SQL VIEW (Example 9: SQL VIEW for DML (Update, Delete and Insert) queries)
We can use SQL VIEW to insert, update and delete data in a single SQL table. We need to note the following things regarding this.
我们可以使用SQL VIEW在单个SQL表中插入,更新和删除数据。 我们需要注意以下几点。
- We can use DML operation on a single table only 我们只能在单个表上使用DML操作
- VIEW should not contain Group By, Having, Distinct clauses VIEW不应包含Group By,Having,Distinct子句
- We cannot use a subquery in a VIEW in SQL Server 我们不能在SQL Server的VIEW中使用子查询
- We cannot use Set operators in a SQL VIEW 我们不能在SQL VIEW中使用Set运算符
Use the following queries to perform DML operation using VIEW in SQL Server.
使用以下查询在SQL Server中使用VIEW执行DML操作。
Insert into DemoView values(4,'CC','KK','RR')
Delete from DemoView where TableID=7
Update DemoView set value='Raj' where TableID=5
示例10:SQL VIEW和检查选项 (Example 10: SQL VIEW and Check Option)
We can use WITH CHECK option to check the conditions in VIEW are inline with the DML statements.
我们可以使用WITH CHECK选项来检查VIEW中的条件是否与DML语句内联。
- It prevents to insert rows in the table where the condition in the Where clause is not satisfied 它防止在不满足Where子句条件的表中插入行
- If the condition does not satisfy, we get an error message in the insert or update statement 如果条件不满足,我们会在插入或更新语句中收到错误消息
In the following query, we use the CHECK option, and we want only values starting with letter F in the [Codeone] column.
在以下查询中,我们使用CHECK选项,并且只需要[Codeone]列中以字母F开头的值。
CREATE VIEW DemoView
ASSELECT *FROM [dbo].[MyTable]WHERE [Codeone] LIKE 'F%'
WITH CHECK OPTION;
If we try to insert a value that does not match the condition, we get the following error message.
如果我们尝试插入与条件不匹配的值,则会收到以下错误消息。
Insert into DemoView values (5,'CC','Raj','Raj')

示例11:删除SQL VIEW (Example 11: Drop SQL VIEW)
We can drop a VIEW using the DROP VIEW statement. In the following query, we want to drop the VIEW demoview in SQL Server.
我们可以使用DROP VIEW语句删除VIEW。 在以下查询中,我们要在SQL Server中删除VIEW demoview 。
DROP VIEW demoview;
示例12:更改SQL视图 (Example 12: Alter a SQL VIEW)
We can change the SQL statement in a VIEW using the following alter VIEW command. Suppose we want to change the condition in the where clause of a VIEW. Execute the following query.
我们可以使用以下alter VIEW命令更改VIEW中SQL语句。 假设我们要更改VIEW的where子句中的条件。 执行以下查询。
Alter VIEW DemoView
ASSELECT *FROM [dbo].[MyTable]WHERE [Codeone] LIKE 'C%'
WITH CHECK OPTION;
Starting from SQL Server 2016 SP1, we can use the CREATE or ALTER statement to create a SQL VIEW or modify it if already exists. Prior to SQL Server 2016 SP1, we cannot use both CREATE or Alter together.
从SQL Server 2016 SP1开始,我们可以使用CREATE或ALTER语句创建SQL VIEW或对其进行修改(如果已存在)。 在SQL Server 2016 SP1之前,我们不能同时使用CREATE或Alter。
CREATE OR ALTER VIEW DemoView
AS SELECT *FROM [dbo].[MyTable]WHERE [Codeone] LIKE 'C%'
WITH CHECK OPTION;
结论 (Conclusion)
In this article, we explored SQL View with various examples. You should be familiar with the view in SQL Server as a developer or DBA as well. Further, you can learn more on how to create view in SQL Server and SQL Server indexed view. If you have any comments or questions, feel free to leave them in the comments below.
在本文中,我们通过各种示例探索了SQL View。 您还应该以开发人员或DBA的身份熟悉SQL Server中的视图。 此外,您可以了解有关如何在SQL Server和SQL Server索引视图中 创建视图的更多信息。 如果您有任何意见或疑问,请随时将其留在下面的评论中。
翻译自: https://www.sqlshack.com/sql-view-a-complete-introduction-and-walk-through/
t–sql pl–sql
t–sql pl–sql_SQL View –完整的介绍和演练相关推荐
- t–sql pl–sql_SQL Server处理器性能指标–第1部分–最重要的CPU指标
t–sql pl–sql Starting with this article, we will present the most important CPU metrics, describe th ...
- t–sql pl–sql_SQL存储过程–终极指南
t–sql pl–sql Hey, folks! In this article, we will be focusing on SQL Stored Procedures. 嘿伙计! 在本文中,我们 ...
- t–sql pl–sql_SQL Server性能疑难解答的DBA指南–第1部分–问题和性能指标
t–sql pl–sql It doesn't mean that every SQL Server slowdown is a performance problem. Some specific ...
- t–sql pl–sql_SQL Server处理器性能指标–第3部分–指示硬件组件问题的指标
t–sql pl–sql Part 1 and 第1部分和Part 2 of the SQL Server processor performance metrics series, we descr ...
- t–sql pl–sql_SQL Server处理器性能指标–第4部分–处理器指标有助于更深入的调查和故障排除
t–sql pl–sql In the previous part of this series, we presented the processor metrics that indicate h ...
- t–sql pl–sql_SQL Server性能疑难解答的DBA指南–第2部分–监视实用程序
t–sql pl–sql SQL Server探查器 (SQL Server Profiler ) SQL Server Profiler is a monitoring tool that you ...
- t–sql pl–sql_SQL串联正确完成–第1部分–可疑做法
t–sql pl–sql This article is a part of three articles series to explore SQL Concatenation techniques ...
- t–sql pl–sql_SQL日期时间–您应该知道的15个重要功能!
t–sql pl–sql Hey, folks! Hope you all are doing well. Today, in this article, we will be highlightin ...
- t–sql pl–sql_SQL Server –在T-SQL中使用最低的度量单位
t–sql pl–sql A client recently discovered a discrepancy on one of our reports that showed an improve ...
最新文章
- 四百元值不值——论小米2A与2S
- 清华大学唐杰老师组:自监督学习最新研究进展
- 一张图了解javaJwt
- Java数据结构和算法(六)——前缀、中缀、后缀表达式
- bootstrap学习(五)代码
- Javascript s08
- arcgis已知两点投影坐标求距离
- LeetCode 1876. 长度为三且各字符不同的子字符串
- .NET Core 3.0中的Cookie身份验证
- Hudson安装配置文档
- 同志,云原生了解一下?
- 全球三大计算机病毒爆发事件,全球爆发勒索病毒 十大恐怖电脑病毒排行榜(3)...
- 单片机复位电路,隐藏着这么多门道
- 笔记本安装固态硬盘ssd教程
- 【java校招你不知道的那些事儿】java校招有没有考点大纲?不能拿面试补缺
- 【计算机网络】第四部分 网络层(21) 地址映射、差错报告和多播
- CV2逐步学习-2:cv2.GaussianBlur()详解
- 整理38款国外的一些免费虚拟主机,云计算,看看你使用过哪几个
- java查找_用Java如何实现搜索功能?
- 化工企业安全生产管理监控预警系统软件
热门文章
- jq post 表单提交文件_Power Query 中使用POST方法进行网络抓取的尝试
- python3 表情符号编码
- *2.3.2_加入env
- 【网络流24题----01】飞行员配对方案问题
- GCD三部曲之一---辗转相除法
- Andrew Stankevich#39;s Contest (1)
- nvm管理node版本
- LeetCode(872)——叶子相似的树(JavaScript)
- LeetCode(344)——反转字符串(JavaScript)
- HIT Software Construction Review Notes(1-2 Quality Objectives of Software Construction)