matlab拔河比赛_拔河比赛
matlab拔河比赛
Description:
描述:
This is a standard interview problem to divide a set of number to two different set where both the subset contains same number of element and have a minimum difference of sum between them using backtracking.
这是一个标准的采访问题,它是将一组数字划分为两个不同的集合,其中两个子集都包含相同数量的元素,并且使用回溯,它们之间的总和差异最小。
Problem statement:
问题陈述:
There is a set contains N number of elements. We have to divide this set into two different sets where each of the subsets contains the same number of elements and has a minimum difference between them.
有一个包含N个元素的集合。 我们必须将此集合分为两个不同的集合,其中每个子集包含相同数量的元素,并且它们之间的差异最小。
If N is even then each of the subsets will contain N/2 number of elements.
如果N为偶数,则每个子集将包含N / 2个元素。
If N is odd then one of the subsets will contain (N+1)/2 and others will contain (N-1)/2 number of elements.
如果N为奇数,则子集中的一个子集将包含(N + 1)/ 2个元素,而其他子集将包含(N-1)/ 2个元素。
Input:
Test case T
//T no. of line with the value of N and corresponding values.
E.g.
3
3
1 2 3
4
1 2 3 4
10
1 4 8 6 -7 -10 87 54 16 100
1<=T<=100
1<=N<=100
Output:
Print the two subsets.
Example
例
T=3
N=3
1 2 3
Output: set1: {1, 2} set2: {3}
N=4
1 2 3 4
Output: set1: {1, 4} set2: {2, 3}
N=10
1 4 8 6 -7 -10 87 54 16 100
Output: set1: {{ 4, -7, -10, 87, 54 } set2: {1, 8, 6, 16, 100 }
Explanation with example
举例说明
Let, there is N no. of values, say e1, e2, e3, ..., en and N is even.
让我们没有N。 e 1 ,e 2 ,e 3 ,...,e n和N的值是偶数。
Subset1: { N/2 no. of values }
Subset2: { N/2 no. of values }
The process to insert the elements to the Subsets is a problem of combination and permutation. To get the result we use the backtracking process. Here we take the sum of all the elements
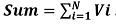
将元素插入子集的过程是组合和排列的问题。 为了获得结果,我们使用了回溯过程。 这里我们取所有元素的总和
The ith element is a part of the subset1.
第i 个元素是subset1的一部分。
The ith element is not a part of the subset1.
第i 个元素不是subset1的一部分。
After getting N/2 number of elements into the subset1. We will check,
在将N / 2数量的元素放入子集1之后 。 我们会检查

If the value is less than then Difference we update the value of the Difference and make a note of the elements that are in the subset1.
如果该值小于那么差 ,我们更新之差的值并记录是在SUBSET1元素。
After getting the elements for those the value of Difference is less we check the elements of the main set and find out the elements that are not in the subset1 and put them in subset2.
获得元素对于那些经过差值小于我们检查的主要集合中的元素,并找出不在SUBSET1的元素,并把它们放在SUBSET2。
Set: {1, 2, 3}
Sum=6
1 is in the subset1 therefore Subset1: {1} and Difference = abs(3-1) = 2
1在子集1中,因此子集1:{1},并且差= abs(3-1)= 2
2 is in the subset1 therefore Subset1: {1,2} and Difference = abs(3-3) = 0
2在子集1中,因此子集1:{1,2},且差= abs(3-3)= 0
Therefore, Difference is minimum.
因此, 差异最小。
Subset1: {1,2}
Subset2: {3}
C++ implementation:
C ++实现:
#include <bits/stdc++.h>
using namespace std;
void traverse(int* arr, int i, int n, int no_of_selected, int sum, int& diff, bool* take, vector<int>& result, vector<int> current, int current_sum)
{//if the current position is greater than or equals to n
if (i >= n)
return;
//if the value of difference is greater than
//Sum/2-current sum of the elements of Subset no.1
if ((diff > abs(sum / 2 - current_sum)) && (no_of_selected == (n + 1) / 2 || no_of_selected == (n - 1) / 2)) {diff = abs(sum / 2 - current_sum);
//store the subset no. 1
result = current;
}
//taking the elemets after the current element one by one
for (int j = i; j < n; j++) {take[j] = true;
current.push_back(arr[j]);
traverse(arr, j + 1, n, no_of_selected + 1, sum, diff, take, result, current, current_sum + arr[j]);
current.pop_back();
take[j] = false;
}
}
void find(int* arr, int n)
{//array to distinguished the elements those are in subset no. 1
bool take[n];
int sum = 0;
for (int i = 0; i < n; i++) {sum += arr[i];
take[i] = false;
}
int diff = INT_MAX;
int no_of_selected = 0;
vector<int> result;
vector<int> current;
int current_sum = 0;
int i = 0;
traverse(arr, i, n, no_of_selected, sum, diff, take, result, current, current_sum);
set<int> s;
//elements those are in subset no.1
cout << "Set1 : { ";
for (int j = 0; j < result.size(); j++) {cout << result[j] << " ";
s.insert(result[j]);
}
cout << "}" << endl;
//elements those are in subset no.2
cout << "Set2 : { ";
for (int j = 0; j < n; j++) {if (s.find(arr[j]) == s.end()) {cout << arr[j] << " ";
}
}
cout << "}" << endl;
}
int main()
{int t;
cout << "Test case : ";
cin >> t;
while (t--) {int n;
cout << "Enter the value of n : ";
cin >> n;
int arr[n];
cout << "Enter the values: ";
//taking the set elements
for (int i = 0; i < n; i++) {cin >> arr[i];
}
find(arr, n);
}
return 0;
}
Output
输出量
Test cases : 3
Enter the value of n : 3
Enter the values: 1 2 3
Set1 : { 1 2 }
Set2 : { 3 }
Enter the value of n : 4
Enter the values: 1 2 3 4
Set1 : { 2 3 }
Set2 : { 1 4 }
Enter the value of n : 10
Enter the values: 1 4 8 6 -7 -10 87 54 16 100
Set1 : { 4 -7 -10 87 54 }
Set2 : { 1 8 6 16 100 }
翻译自: https://www.includehelp.com/icp/tug-of-war.aspx
matlab拔河比赛
matlab拔河比赛_拔河比赛相关推荐
- 第八届育才杯机器人比赛_赛场、名单公布!南海区第八届“献血者杯”羽毛球公开赛“羽”你相约本周六...
主办单位:南海区献血办.南海血站协办单位:南海区羽毛球协会为进一步扩大无偿献血宣传,感恩南海区献血者,活跃无偿献血者的文体生活,打造一个南海区无偿献血者交流的平台,共同营造"运动.健康.献血 ...
- 设计比赛-我图杯原创设计比赛_我图网www.ooopic.com_第一期:中国风原创设计比赛
设计比赛-我图杯原创设计比赛_我图网www.ooopic.com_第一期:中国风原创设计比赛 详细到:http://a.ooopic.com/ 第一期奖品设置: 金牌获得者:1名,奖品:苹果 ipod ...
- python预测足球比赛_机器学习算法预测足球赛事的模型比较(一)
本文是作者前一段时间关注足球赛事时做的一点分析,内容涉及基于足球数据进行描述性统计分析.基于机器学习建模与传统的泊松模型建模三大部分,由于文章较长,将分两部分进行展现. 第一部分为前言与述性分析部分1 ...
- 山东栋梁机器人比赛_谁是最强“移动机器人”?来深技师这场全国大赛一决高下!...
9月24日 第一届全国技能大赛世赛移动机器人项目 全国机械行业选拔赛 在深圳技师学院闭幕! 为期三天的赛程中 来自全国职业院校.机械行业相关企业的 40支参赛队伍.130多名选手和专家教练 相聚在深技 ...
- python分析比赛_世界杯:用Python分析热门夺冠球队-(附源代码)
2018年,火热的世界杯即将拉开序幕.在比赛开始之前,我们不妨用 Python 来对参赛队伍的实力情况进行分析,并大胆的预测下本届世界杯的夺冠热门球队. 通过数据分析,可以发现很多有趣的结果,比如: ...
- python编程比赛_用Python编程分析4W场球赛后,2018世界杯冠军竟是…
比赛已经开始,我们不妨用 Python 来对参赛队伍的实力情况进行分析,并大胆的预测下本届世界杯的夺冠热门球队吧! 通过数据分析,可以发现很多有趣的结果,比如: 找出哪些队伍是首次进入世界杯的黑马队伍 ...
- 天池比赛物流比赛_比赛树及其属性
天池比赛物流比赛 比赛树 (Tournament Tree) Tournament tree is a complete binary tree n external nodes and n-1 in ...
- 乒乓球十一分制比赛规则_乒乓球比赛11 分制规定
1 关于新规则 1 . 1 大球 2000 年 2 月, 在马来西亚举行的国际乒联代表大会上通过了决议, 把原 38 毫米直径的乒 乓球增加两毫米,为 40 毫米,俗称 " 大球 " ...
- python分析比赛_最强观战指南 | Python分析热门夺冠球队(附源代码)
2018年,火热的世界杯即将拉开序幕.在比赛开始之前,我们不妨用 Python 来对参赛队伍的实力情况进行分析,并大胆的预测下本届世界杯的夺冠热门球队. 通过数据分析,可以发现很多有趣的结果,比如: ...
最新文章
- Python调整图像亮度和饱和度
- LOL手游上线!同步专属限量游戏红包封面,还不快来拿?
- B+树与LSM树的区别与联系
- 基于Hadoop的数据分析平台搭建
- Filebeat常用配置
- C# Socket系列二 简单的创建 socket 通信
- Uboot中start.S源码的指令级的详尽解析(转)
- 您好GroovyFX
- 一步一步教你在CentOS6.0下安装NS2(ns-allinone-2.34.tar.gz)模拟仿真工具
- EasyPR 环境配置
- KUKA库卡机器人零点失效维修案例
- Android打开项目一直build的问题解决
- 在电子电路中,为什么要进行电气隔离?
- ubuntu 18.04-设置合上笔记本盖子不休眠
- 都2021年了,你还在考虑电赛飞行器赛题,备赛是否有必要用基于TI处理芯片的飞控问题?
- Sharding Sphere ~ Sharding-jdbc分库分表、读写分离
- 揭露强奸犯的黑客被判有罪?审视CFAA计算机欺诈法
- C# 添加Word页眉、页脚
- 系统变量和用户变量的区别
- FPGA - 7系列 FPGA内部结构之CLB -01- CLB资源概述
热门文章
- python句柄无效_subprocess.Popen 运行windows命令出现“句柄无效”报错的解决方法
- SpringBoot-探索回顾Spring框架本质
- typedef 的四个用途和两大陷阱
- Linux集群架构(LVS DR模式搭建、keepalived + LVS)
- Android 撸起袖子,自己封装 DialogFragment
- Android-TextView跑马灯效果
- JDK各版本新增的主要特性
- 在SQL Server中为什么不建议使用Not In子查询
- mongodb replicaset shard 集群性能测试
- 永中向香港博览会主办方演示云办公(转载)