C# 注册表控制++ Autorun after windows
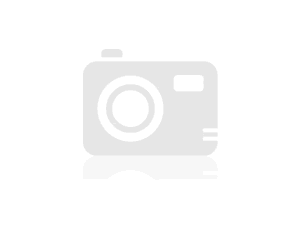
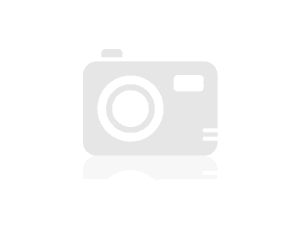
1
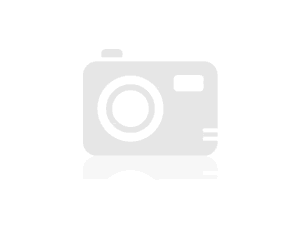
2
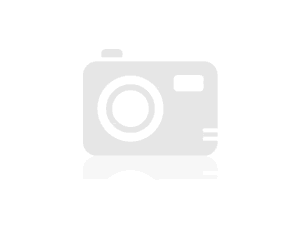
3
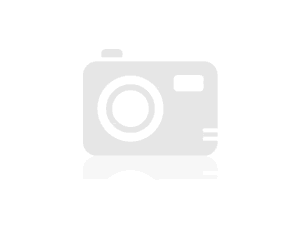
4
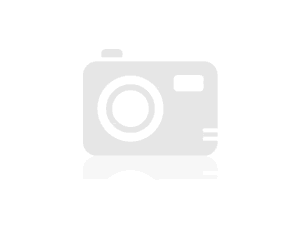
5
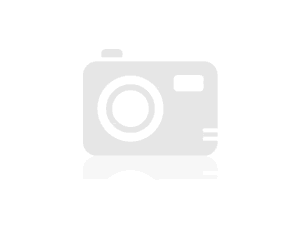
6
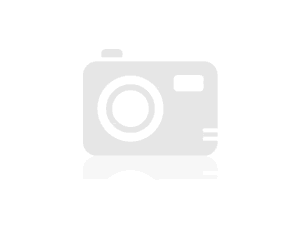
7
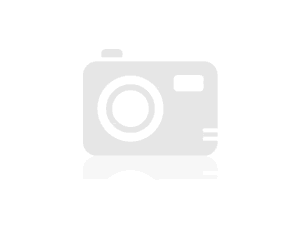
8
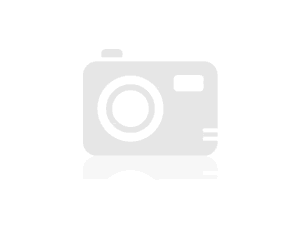
9
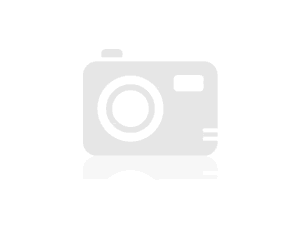
10
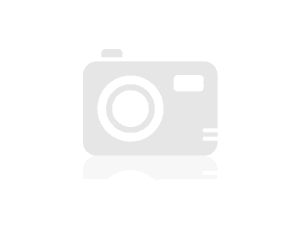
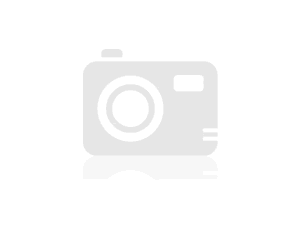
11

12

13

14
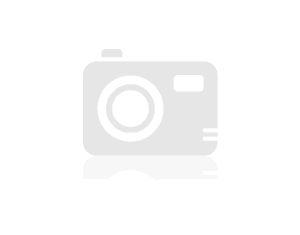
15
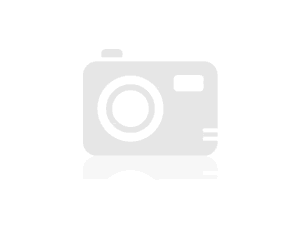
16
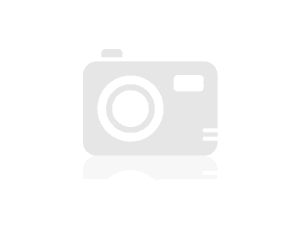
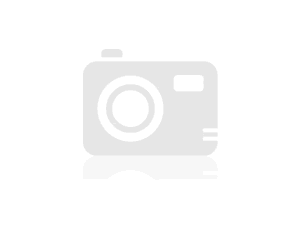

17

18

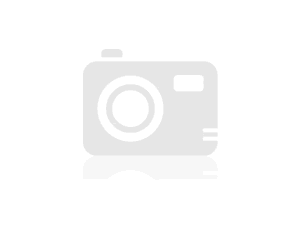

19

20

21

22

23

24

25

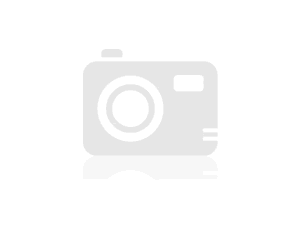

26

27

28

29

30

31

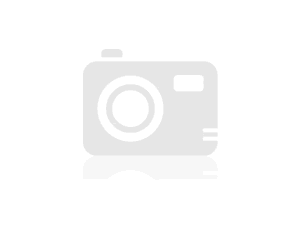

32

33

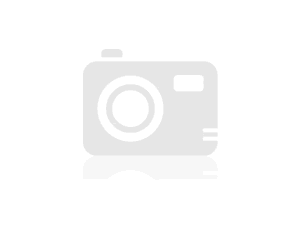

34

35

36

37

38

39

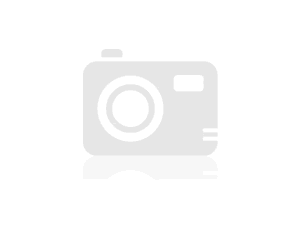

40

41

42

43

44

45

46

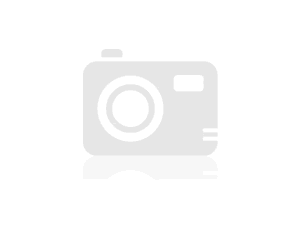

47

48

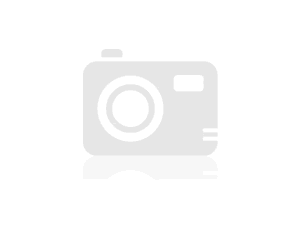

49

50

51

52

53

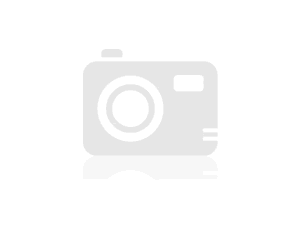

54

55

56

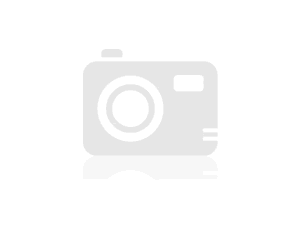

57

58

59

60

61

62

63

64

65

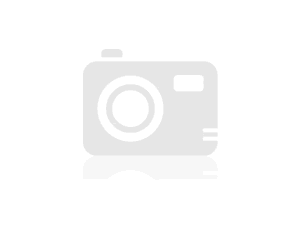

66

67

68

69

70

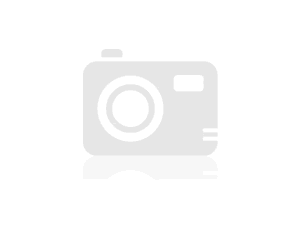
71

72

73

74

75

76

77

78

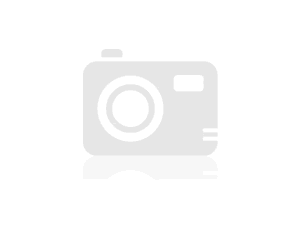

79

80

81

82

83

84

85

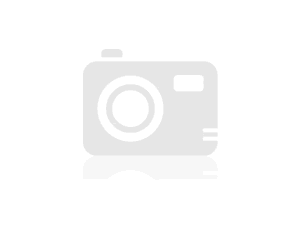

86

87

88

89

90

91

92

93

94

95

96

97

98

99

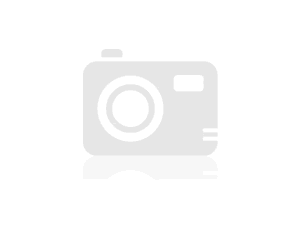

100

101

102

103

104

105

106

107

108
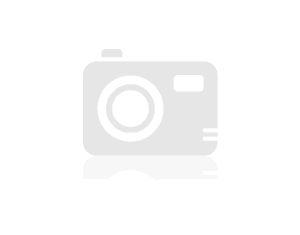
转载于:https://www.cnblogs.com/josephshi/archive/2007/12/13/993745.html
C# 注册表控制++ Autorun after windows相关推荐
- IE选项注册表控制大全(转)
IE选项注册表控制大全(转) 以上是几个大项,小项的解释在下面: HKEY_CURRENT_USERSoftwarePoliciesMicrosoftInternet ExplorerControl ...
- 注册表 关闭打印机服务器,Windows 2016 server部署打印机服务器后 重启服务器,出现“重定向的打印机”,如何来删除?...
你好, 请问你登录的账户是否有本地管理员权限? 请检查了再次尝试. 重定向也可能是RDP会话的原因,为用户使用的Rdp连接禁用打印机本地资源选项. 或者通过注册表来删除 首先手动停止打印机服务,然后 ...
- java 删除注册表_java – 如何从Windows注册表中删除JRE条目?
JDK本身不使用Windows注册表来运行. 在某些情况下,使用系统注册表运行的JRE是如Applet或以WebStart技术开始的程序. 最后,如果从Windows系统目录(例如C:/winnt/s ...
- oracle注册表重建,一次Windows 注册表中注册表项目丢失导致的Oracle 数据库启动问题。...
一次Windows 注册表中注册表项目丢失导致的Oracle 数据库启动问题. 环境说明: 1.windows 2008操作系统 x64bit 2.Oracle database 11.2.0.1 ...
- [网络转载]IE选项注册表控制大全
//------仅供参考--------/ 近来,很多网友在各大BBS提出一个相同的问题----IE选项全部或其中某几个不能使用,怎么办?本来有关IE的选项的问题Solon已经发表过相关的文章,但是还 ...
- IE选项注册表控制大全
近来,很多网友在各大BBS提出一个相同的问题----IE选项全部或其中某几个不能使用,怎么办?本来有关IE的选项的问题Solon已经发表过相关的文章,但是还是有很多不是很了解注册表的朋友看不懂,在这种 ...
- C++ 无法将值写入注册表 HKEY_LOCAL_MACHINE\Sofeware\Microsoft\Windows\CurrentVersion\Run以实现开机自启动应用程序
C++中通过写入注册表实现应用程序开机自启动时,如果应用程序本身以非管理员权限启动的话,是写入machine级别的注册表的,如果以管理员权限启动应用程序,可以写入machine级别的注册表,但是默认以 ...
- Windows注册表修改实例完全手册(下)
注 册表是Windows操作系统的核心.它实质上是一个庞大的数据库,存放有计算机硬件和全部配置信息.系统和应用软件的初始化信息.应用软件和文档文件的 关联关系.硬件设备说明以及各种网络状态信息和数据. ...
- 渗透知识- Windows系统目录、服务、端口、注册表
1.系统目录 Windows:是Windows系统安装文件所在文件夹,一般用来存储系统安装文件和硬件驱动程序等内容,是系统正常运转的必要保证. Program Files:指的是程序文件,是Windo ...
最新文章
- 只有变强大,才能照亮他人
- 计算机协会成立活动简报,“中国计算机学会CCF走进高校”活动在我校举行
- 每日一套codeforce集训1119E[贪心],821C[栈模拟],645D[拓扑排序]
- iodine免费上网——本质就是利用dns tunnel建立tcp,然后tcp proxy来实现通过访问虚拟dns0网卡来访问你的dns 授权server...
- php和java整合开发实战_PHP和Java强强联合 PHP与Java集成开发详解
- SQLServer之事务简介
- python前端调用后端模型_前端调用后端的方法(基于restful接口的mvc架构)
- [转载] [Python] np.ones_like(ndarray)和np.zeros_like(ndarray)
- 计算机软驱的连接方式,岛精仿真软驱、斯托尔USB软驱、斯坦格电脑横机软盘改U盘...
- 【Proteus仿真】波形信号发生器(4种波形可选,频率可调)
- ReactNative--控件小记-更新中
- 写尽自己一个人的孤独,却写不出心里的寂寞
- 使用UltraISO软碟通离线安装Centos8.3.2011过程中遇到的问题
- (转)《浪潮之巅》作者吴军前言:有幸见证历史(内有目录和作者简介)
- 苹果进入品牌价值衰减期
- 站内搜寻引擎 php mysql_迅搜(xunsearch) - 开源免费中文全文搜索引擎|PHP全文检索|mysql全文检索|站内搜索...
- ORB-SLAM3:单目+imu 详细代码解读
- 台式计算机无法启动不了,台式机和笔记本电脑主机启动不了常见原因解决方法...
- NULL与nullptr
- 十几年经验的原华为海思工程师亲述ic验证如何转ic设计
热门文章
- 改进了一下这个游戏的输出及思路,是不是好玩多了??:)
- jquery源码解析:proxy,access,swap,isArraylike详解
- KlayGE 4.4中渲染的改进(五):OpenGL 4.4和OpenGLES 3
- UVA 11825 Hackers' Crackdown 状态DP
- linux下文件删除的原理精华讲解(考试题答案系列)
- petalinux板卡没有ip address_海思板卡端访问服务器的文件(一)
- 可变化的鸿蒙武器,DNF2018史诗改版大全 武器套装改版属性介绍
- 常用选择器(CSS+JQuery)
- java 区块链开发_Java开发人员的区块链入门
- linus为什么开源_Linus Torvalds谈个性崇拜,美国最好的开源学校等等