Http Invoker的Spring Remoting支持
Spring通过HttpInvokerProxyFactoryBean和HttpInvokerServiceExporter支持HTTP调用程序基础结构。 HttpInvokerServiceExporter,它将指定的服务bean导出为HTTP调用程序服务端点,可通过HTTP调用程序代理访问。 HttpInvokerProxyFactoryBean是用于HTTP调用程序代理的工厂bean。
此外,还提供了有关Spring Remoting简介和RMI Service&Client示例项目的Spring Remoting支持和RMI文章。
让我们看一下Spring Remoting Support,以开发Http Invoker Service&Client。
二手技术:
- JDK 1.6.0_31
- 春天3.1.1
- Tomcat 7.0
- Maven的3.0.2
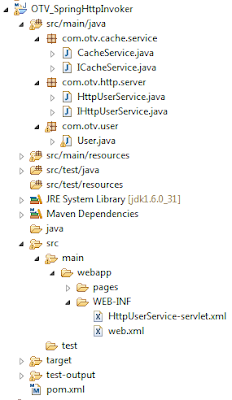
步骤1:建立已完成的专案
创建一个Maven项目,如下所示。 (可以使用Maven或IDE插件来创建它)。
步骤2:图书馆
Spring依赖项已添加到Maven的pom.xml中。
<!-- Spring 3.1.x dependencies -->
<properties><spring.version>3.1.1.RELEASE</spring.version>
</properties><dependencies><dependency><groupId>org.springframework</groupId><artifactId>spring-core</artifactId><version>${spring.version}</version></dependency><dependency><groupId>org.springframework</groupId><artifactId>spring-context</artifactId><version>${spring.version}</version></dependency><dependency><groupId>org.springframework</groupId><artifactId>spring-remoting</artifactId><version>2.0.8</version></dependency><dependency><groupId>org.springframework</groupId><artifactId>spring-web</artifactId><version>${spring.version}</version></dependency><dependency><groupId>org.springframework</groupId><artifactId>spring-webmvc</artifactId><version>${spring.version}</version></dependency>
<dependencies>
步骤3:建立使用者类别
创建一个新的用户类。
package com.otv.user;import java.io.Serializable;/*** User Bean** @author onlinetechvision.com* @since 24 Feb 2012* @version 1.0.0**/
public class User implements Serializable {private long id;private String name;private String surname;/*** Get User Id** @return long id*/public long getId() {return id;}/*** Set User Id** @param long id*/public void setId(long id) {this.id = id;}/*** Get User Name** @return long id*/public String getName() {return name;}/*** Set User Name** @param String name*/public void setName(String name) {this.name = name;}/*** Get User Surname** @return long id*/public String getSurname() {return surname;}/*** Set User Surname** @param String surname*/public void setSurname(String surname) {this.surname = surname;}@Overridepublic String toString() {StringBuilder strBuilder = new StringBuilder();strBuilder.append("Id : ").append(getId());strBuilder.append(", Name : ").append(getName());strBuilder.append(", Surname : ").append(getSurname());return strBuilder.toString();}
}
步骤4:建立ICacheService介面
创建了代表远程缓存服务接口的ICacheService接口。
package com.otv.cache.service;import java.util.concurrent.ConcurrentHashMap;import com.otv.user.User;/*** Cache Service Interface** @author onlinetechvision.com* @since 10 Mar 2012* @version 1.0.0**/
public interface ICacheService {/*** Get User Map** @return ConcurrentHashMap User Map*/public ConcurrentHashMap<Long, User> getUserMap();}
步骤5:创建CacheService类
CacheService类是通过实现ICacheService接口创建的。 它提供对远程缓存的访问…
package com.otv.cache.service;import java.util.concurrent.ConcurrentHashMap;import com.otv.user.User;/*** Cache Service Implementation** @author onlinetechvision.com* @since 10 Mar 2012* @version 1.0.0**/
public class CacheService implements ICacheService {//User Map is injected...ConcurrentHashMap<Long, User> userMap;/*** Get User Map** @return ConcurrentHashMap User Map*/public ConcurrentHashMap<Long, User> getUserMap() {return userMap;}/*** Set User Map** @param ConcurrentHashMap User Map*/public void setUserMap(ConcurrentHashMap<Long, User> userMap) {this.userMap = userMap;}}
步骤6:建立IHttpUserService接口
创建了代表Http用户服务接口的IHttpUserService。 此外,它为Http客户端提供了远程方法。
package com.otv.http.server;import java.util.List;import com.otv.user.User;/*** Http User Service Interface** @author onlinetechvision.com* @since 10 Mar 2012* @version 1.0.0**/
public interface IHttpUserService {/*** Add User** @param User user* @return boolean response of the method*/public boolean addUser(User user);/*** Delete User** @param User user* @return boolean response of the method*/public boolean deleteUser(User user);/*** Get User List** @return List user list*/public List<User> getUserList();}
步骤7:创建HttpUserService类
HttpUserService类是通过实现IHttpUserService接口创建的。
package com.otv.http.server;import java.util.ArrayList;
import java.util.List;
import org.apache.log4j.Logger;import com.otv.cache.service.ICacheService;
import com.otv.user.User;/*** Http User Service Implementation** @author onlinetechvision.com* @since 10 Mar 2012* @version 1.0.0**/
public class HttpUserService implements IHttpUserService {private static Logger logger = Logger.getLogger(HttpUserService.class);//Remote Cache Service is injected...ICacheService cacheService;/*** Add User** @param User user* @return boolean response of the method*/public boolean addUser(User user) {getCacheService().getUserMap().put(user.getId(), user);logger.debug("User has been added to cache. User : "+getCacheService().getUserMap().get(user.getId()));return true;}/*** Delete User** @param User user* @return boolean response of the method*/public boolean deleteUser(User user) {getCacheService().getUserMap().remove(user.getId());logger.debug("User has been deleted from cache. User : "+user);return true;}/*** Get User List** @return List user list*/public List<User> getUserList() {List<User> list = new ArrayList<User>();list.addAll(getCacheService().getUserMap().values());logger.debug("User List : "+list);return list;}/*** Get Remote Cache Service** @return ICacheService Remote Cache Service*/public ICacheService getCacheService() {return cacheService;}/*** Set Remote Cache Service** @param ICacheService Remote Cache Service*/public void setCacheService(ICacheService cacheService) {this.cacheService = cacheService;}}
步骤8:创建HttpUserService-servlet.xml
HttpUserService应用程序上下文如下所示。 该xml必须命名为your_servlet_name-servlet.xml
<beans xmlns="http://www.springframework.org/schema/beans"xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"xmlns:util="http://www.springframework.org/schema/util"xsi:schemaLocation="http://www.springframework.org/schema/beanshttp://www.springframework.org/schema/beans/spring-beans-3.0.xsdhttp://www.springframework.org/schema/utilhttp://www.springframework.org/schema/util/spring-util-3.0.xsd"><!-- User Map Declaration --><bean id="UserMap" class="java.util.concurrent.ConcurrentHashMap" /><!-- Cache Service Declaration --><bean id="CacheService" class="com.otv.cache.service.CacheService"><property name="userMap" ref="UserMap"/></bean> <!-- Http User Service Bean Declaration --><bean id="HttpUserService" class="com.otv.http.server.HttpUserService" ><property name="cacheService" ref="CacheService"/></bean><!-- Http Invoker Service Declaration --><bean id="HttpUserServiceExporter" class="org.springframework.remoting.httpinvoker.HttpInvokerServiceExporter"><!-- service represents Service Impl --><property name="service" ref="HttpUserService"/><!-- serviceInterface represents Http Service Interface which is exposed --><property name="serviceInterface" value="com.otv.http.server.IHttpUserService"/></bean><!-- Mapping configurations from URLs to request handler beans --><bean id="urlMapping" class="org.springframework.web.servlet.handler.SimpleUrlHandlerMapping"><property name="mappings"><props><prop key="/HttpUserService">HttpUserServiceExporter</prop></props></property></bean></beans>
步骤9:创建web.xml
web.xml的配置如下:
<?xml version="1.0" encoding="UTF-8"?>
<web-app xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns="http://java.sun.com/xml/ns/javaee" xmlns:web="http://java.sun.com/xml/ns/javaee/web-app_2_5.xsd" xsi:schemaLocation="http://java.sun.com/xml/ns/javaee http://java.sun.com/xml/ns/javaee/web-app_2_5.xsd" id="WebApp_ID" version="2.5"><display-name>OTV_SpringHttpInvoker</display-name><!-- Spring Context Configuration' s Path definition --><context-param><param-name>contextConfigLocation</param-name><param-value>/WEB-INF/HttpUserService-servlet.xml</param-value></context-param><!-- The Bootstrap listener to start up and shut down Spring's root WebApplicationContext. It is registered to Servlet Container --><listener><listener-class>org.springframework.web.context.ContextLoaderListener</listener-class></listener><!-- Central dispatcher for HTTP-based remote service exporters. Dispatches to registered handlers for processing web requests.--><servlet><servlet-name>HttpUserService</servlet-name><servlet-class>org.springframework.web.servlet.DispatcherServlet</servlet-class><load-on-startup>2</load-on-startup></servlet><!-- Servlets should be registered with servlet container and mapped with url for the http requests. --><servlet-mapping><servlet-name>HttpUserService</servlet-name><url-pattern>/HttpUserService</url-pattern></servlet-mapping><welcome-file-list><welcome-file>/pages/index.xhtml</welcome-file></welcome-file-list></web-app>
步骤10:创建HttpUserServiceClient类
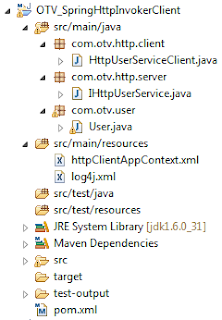
HttpUserServiceClient类已创建。 它调用远程Http用户服务并执行用户操作。
package com.otv.http.client;import org.apache.log4j.Logger;
import org.springframework.context.ApplicationContext;
import org.springframework.context.support.ClassPathXmlApplicationContext;import com.otv.http.server.IHttpUserService;
import com.otv.user.User;/*** Http User Service Client** @author onlinetechvision.com* @since 24 Feb 2012* @version 1.0.0**/
public class HttpUserServiceClient {private static Logger logger = Logger.getLogger(HttpUserServiceClient.class);/*** Main method of the Http User Service Client**/public static void main(String[] args) {logger.debug("Http User Service Client is starting...");//Http Client Application Context is started...ApplicationContext context = new ClassPathXmlApplicationContext("httpClientAppContext.xml");//Remote User Service is called via Http Client Application Context...IHttpUserService httpClient = (IHttpUserService) context.getBean("HttpUserService");//New User is created...User user = new User();user.setId(1);user.setName("Bruce");user.setSurname("Willis");//The user is added to the remote cache...httpClient.addUser(user);//The users are gotten via remote cache...httpClient.getUserList();//The user is deleted from remote cache...httpClient.deleteUser(user);logger.debug("Http User Service Client is stopped...");}
}
步骤11:创建httpClientAppContext.xml
Http客户端应用程序上下文如下所示:
<beans xmlns="http://www.springframework.org/schema/beans"xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"xsi:schemaLocation="http://www.springframework.org/schema/beanshttp://www.springframework.org/schema/beans/spring-beans-3.0.xsd"><!-- Http Invoker Client Declaration --><bean id="HttpUserService" class="org.springframework.remoting.httpinvoker.HttpInvokerProxyFactoryBean"><!-- serviceUrl demonstrates Http Service Url which is called--><property name="serviceUrl" value="http://remotehost:port/OTV_SpringHttpInvoker-0.0.1-SNAPSHOT/HttpUserService"/><!-- serviceInterface demonstrates Http Service Interface which is called --><property name="serviceInterface" value="com.otv.http.server.IHttpUserService"/></bean></beans>
步骤12:部署项目
将OTV_SpringHttpInvoker Project部署到Tomcat之后,将启动Http用户服务客户端,并且输出日志如下所示:
....
15.03.2012 21:26:41 DEBUG (DispatcherServlet.java:819) - DispatcherServlet with name 'HttpUserService' processing POST request for [/OTV_SpringHttpInvoker-0.0.1-SNAPSHOT/HttpUserService]
15.03.2012 21:26:41 DEBUG (AbstractUrlHandlerMapping.java:124) - Mapping [/HttpUserService] to HandlerExecutionChain with handler [org.springframework.remoting.httpinvoker.HttpInvokerServiceExporter@f9104a] and 1 interceptor
15.03.2012 21:26:41 DEBUG (RemoteInvocationTraceInterceptor.java:73) - Incoming HttpInvokerServiceExporter remote call: com.otv.http.server.IHttpUserService.addUser
15.03.2012 21:26:41 DEBUG (HttpUserService.java:33) - User has been added to cache. User : Id : 1, Name : Bruce, Surname : Willis
15.03.2012 21:26:41 DEBUG (RemoteInvocationTraceInterceptor.java:79) - Finished processing of HttpInvokerServiceExporter remote call: com.otv.http.server.IHttpUserService.addUser
15.03.2012 21:26:41 DEBUG (DispatcherServlet.java:957) - Null ModelAndView returned to DispatcherServlet with name 'HttpUserService': assuming HandlerAdapter completed request handling
15.03.2012 21:26:41 DEBUG (FrameworkServlet.java:913) - Successfully completed request
15.03.2012 21:26:41 DEBUG (DispatcherServlet.java:819) - DispatcherServlet with name 'HttpUserService' processing POST request for [/OTV_SpringHttpInvoker-0.0.1-SNAPSHOT/HttpUserService]
15.03.2012 21:26:41 DEBUG (AbstractUrlHandlerMapping.java:124) - Mapping [/HttpUserService] to HandlerExecutionChain with handler [org.springframework.remoting.httpinvoker.HttpInvokerServiceExporter@f9104a] and 1 interceptor
15.03.2012 21:26:41 DEBUG (RemoteInvocationTraceInterceptor.java:73) - Incoming HttpInvokerServiceExporter remote call: com.otv.http.server.IHttpUserService.getUserList
15.03.2012 21:26:41 DEBUG (HttpUserService.java:57) - User List : [Id : 1, Name : Bruce, Surname : Willis]
15.03.2012 21:26:41 DEBUG (RemoteInvocationTraceInterceptor.java:79) - Finished processing of HttpInvokerServiceExporter remote call: com.otv.http.server.IHttpUserService.getUserList
15.03.2012 21:26:41 DEBUG (DispatcherServlet.java:957) - Null ModelAndView returned to DispatcherServlet with name 'HttpUserService': assuming HandlerAdapter completed request handling
15.03.2012 21:26:41 DEBUG (FrameworkServlet.java:913) - Successfully completed request
15.03.2012 21:26:41 DEBUG (DispatcherServlet.java:819) - DispatcherServlet with name 'HttpUserService' processing POST request for [/OTV_SpringHttpInvoker-0.0.1-SNAPSHOT/HttpUserService]
15.03.2012 21:26:41 DEBUG (AbstractUrlHandlerMapping.java:124) - Mapping [/HttpUserService] to HandlerExecutionChain with handler [org.springframework.remoting.httpinvoker.HttpInvokerServiceExporter@f9104a] and 1 interceptor
15.03.2012 21:26:41 DEBUG (RemoteInvocationTraceInterceptor.java:73) - Incoming HttpInvokerServiceExporter remote call: com.otv.http.server.IHttpUserService.deleteUser
15.03.2012 21:26:41 DEBUG (HttpUserService.java:45) - User has been deleted from cache. User : Id : 1, Name : Bruce, Surname : Willis
15.03.2012 21:26:41 DEBUG (RemoteInvocationTraceInterceptor.java:79) - Finished processing of HttpInvokerServiceExporter remote call: com.otv.http.server.IHttpUserService.deleteUser
15.03.2012 21:26:41 DEBUG (DispatcherServlet.java:957) - Null ModelAndView returned to DispatcherServlet with name 'HttpUserService': assuming HandlerAdapter completed request handling
15.03.2012 21:26:41 DEBUG (FrameworkServlet.java:913) - Successfully completed request
...
步骤13:下载
OTV_SpringHttpInvoker
参考: Online Technology Vision博客上的JCG合作伙伴 Eren Avsarogullari 提供的Http Invoker的Spring Remoting支持 。
翻译自: https://www.javacodegeeks.com/2012/04/spring-remoting-support-with-http.html
Http Invoker的Spring Remoting支持相关推荐
- http invoker_Http Invoker的Spring Remoting支持
http invoker Spring HTTP Invoker是Java到Java远程处理的重要解决方案. 该技术使用标准的Java序列化机制通过HTTP公开服务,并且可以看作是替代方法,而不是He ...
- Spring远程支持和开发RMI服务
Spring远程支持简化了启用远程服务的开发. 当前,Spring支持以下远程技术:远程方法调用(RMI),HTTP调用程序,Hessian,Burlap,JAX-RPC,JAX-WS和JMS. 远程 ...
- Spring Remoting: Remote Method Invocation (RMI)--转
原文地址:http://www.studytrails.com/frameworks/spring/spring-remoting-rmi.jsp Concept Overview Spring pr ...
- Spring Remoting: HTTP Invoker--转
原文地址:http://www.studytrails.com/frameworks/spring/spring-remoting-http-invoker.jsp Concept Overview ...
- MyEclipse中删除对Struts、hibernate、spring的支持
注:本文转载自:http://blog.csdn.net/j04110414/article/details/9900033,版权归其所有 一.首先是撤消MyEclipse对Struts的支持 关键的 ...
- Spring Remoting: Burlap--转
原文地址:http://www.studytrails.com/frameworks/spring/spring-remoting-burlap.jsp Concept Overview In the ...
- Spring Remoting: Hessian--转
原文地址:http://www.studytrails.com/frameworks/spring/spring-remoting-hessian.jsp Concept Overview The p ...
- Spring测试支持和上下文缓存
Spring为单元测试和集成测试提供了全面的支持-通过注释来加载Spring应用程序上下文,并与JUnit和TestNG等单元测试框架集成. 由于为每个测试加载大型应用程序上下文需要时间,因此Spri ...
- weblogic各个版本对JDK和Spring的支持度
weblogic各个版本对JDK和Spring的支持度 现在很多人在使用SSH架构(Spring, Struts, Hibernate)开发, Tomcat 上开发完了, 认为往WebLogic上一放 ...
最新文章
- spring中environment设计与实现
- python 利用pandas库实现 读写 .csv文件
- Django非常简单的安装方法
- SAP CRM BP contact detail - workAddress
- zblog php 七牛缩略图,zblog中Gravatar头像不显示解决方法
- duration java_Java Duration类| 带示例的dividBy()方法
- Javascript预解析、作用域、作用域链
- Java 8 Optional 类 学习
- 浙江商人立下的22条规矩
- 样本量估算:随机对照试验(两组均数)比较的样本量计算方法
- 使用 ASP.NET 制作一个音乐网站
- 杭州电子科技大学acm--2005
- Go使用绘图的库(go-charts、go-echarts)
- 手眼标定_全面细致的推导过程
- 洛谷 P4208 [JSOI2008]最小生成树计数 矩阵树定理
- IDEA-Warring:Add Author to custom tags
- 分布式之数据库和缓存双写一致性方案解析
- 汉字动图动态图gif格式,无水印 4500个汉字
- Vue前端框架的使用
- 做产品和运营必须深参这5大人性弱点
热门文章
- java流与文件——java生成解压缩文件(夹)
- java代码识别_识别Java中的代码气味
- 版本交付_连续交付友好的Maven版本
- 英文连词_连词我们…讨厌
- web.xml.jsf_JSF 2.2在30秒内创建一个自定义Hello World组件
- 众神进入瓦尔哈拉_一时冲动:“通往瓦尔哈拉之路的冒险”
- jooq 配置oracle_jOOQ配置
- 通过Main的Checkpoint Restore加快Java启动速度
- H2数据库的Spring Boot
- 服务器日志记录_5种改善服务器日志记录的技术