poj 1556 (Dijkstra + Geometry 线段相交)
链接:http://poj.org/problem?id=1556
Time Limit: 1000MS | Memory Limit: 10000K | |
Total Submissions: 6216 | Accepted: 2495 |
Description
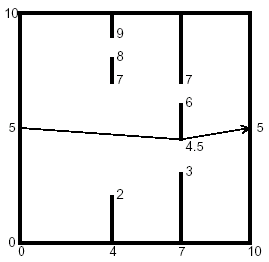
Input
2
4 2 7 8 9
7 3 4.5 6 7
The first line contains the number of interior walls. Then there is a line for each such wall, containing five real numbers. The first number is the x coordinate of the wall (0 < x < 10), and the remaining four are the y coordinates of the ends of the doorways in that wall. The x coordinates of the walls are in increasing order, and within each line the y coordinates are in increasing order. The input file will contain at least one such set of data. The end of the data comes when the number of walls is -1.
Output
Sample Input
1 5 4 6 7 8 2 4 2 7 8 9 7 3 4.5 6 7 -1
Sample Output
10.00 10.06 这题处理起来挺难的,要把输入的点存到图里,用Dijkstra求出最短路径,存图的过程是,判断任意两点连成的线,横坐标不能相同,并且如果,横坐标与线上的横坐标不相同,就要判断是否相交,相交则行不通否则存图,用Dijkstra搜出最短的路径即可还有,要注意细节


1 #include <stdio.h> 2 #include <string.h> 3 #include <stdlib.h> 4 #include <iostream> 5 #include <math.h> 6 #include <algorithm> 7 8 #define eps 1e-6 9 #define INF 1000000000 10 typedef struct point 11 { 12 double x,y; 13 }point; 14 15 typedef struct beline 16 { 17 point st,ed; 18 }beline; 19 20 using namespace std; 21 22 point p[1005]; 23 double mp[1005][1005]; 24 double d[1005]; 25 int visit[1005]; 26 27 bool dy(double x,double y){ return x > y+eps; } 28 bool xy(double x,double y){ return x < y-eps; } 29 bool dyd(double x,double y){ return x > y-eps; } 30 bool xyd(double x,double y){ return x < y+eps; } 31 bool dd(double x,double y){ return fabs(x - y)<eps; } 32 33 double crossProduct(point a,point b,point c) 34 { 35 return (c.x-a.x)*(b.y-a.y)-(c.y-a.y)*(b.x-a.x); 36 } 37 double Dist(point a,point b) 38 { 39 return sqrt((a.x-b.x)*(a.x-b.x)+(a.y-b.y)*(a.y-b.y)); 40 } 41 42 bool onSegment(point a,point b,point c) 43 { 44 double maxx=max(a.x,b.x); 45 double maxy=max(a.y,b.y); 46 double minx=min(a.x,b.x); 47 double miny=min(a.y,b.y); 48 if(dd(crossProduct(a,b,c),0.0)&&dy(c.x,minx)&&xy(c.x,maxx) 49 &&dy(c.y,miny)&&xy(c.y,maxy)) 50 return true; 51 return false; 52 } 53 54 bool segIntersect(point p1,point p2,point p3,point p4) 55 { 56 double d1 = crossProduct(p3,p4,p1); 57 double d2 = crossProduct(p3,p4,p2); 58 double d3 = crossProduct(p1,p2,p3); 59 double d4 = crossProduct(p1,p2,p4); 60 if(xy(d1*d2,0.0)&&xy(d3*d4,0.0)) 61 return true; 62 if(dd(d1,0.0)&&onSegment(p3,p4,p1)) 63 return true; 64 if(dd(d2,0.0)&&onSegment(p3,p4,p2)) 65 return true; 66 if(dd(d3,0.0)&&onSegment(p1,p2,p3)) 67 return true; 68 if(dd(d4,0.0)&&onSegment(p1,p2,p4)) 69 return true; 70 return false; 71 } 72 73 void Dijkstra(int n) 74 { 75 int i,y; 76 memset(visit,0,sizeof(visit)); 77 for(i=0; i<n; i++) 78 d[i] = mp[0][i]; 79 d[0] = 0; 80 for(i=0; i<n; i++) 81 { 82 int m=INF,x; 83 { 84 for(y=0; y<n; y++) 85 { 86 if(!visit[y] && d[y]<=m) 87 { 88 m = d[ x = y ]; 89 } 90 } 91 visit[x]=1; 92 for(y=0; y<n; y++) 93 { 94 if(!visit[y] && d[y] > d[x]+mp[x][y]) 95 { 96 d[y] = d[x] + mp[x][y]; 97 } 98 } 99 } 100 } 101 } 102 103 int main() 104 { 105 int n,m,i,j,k,t; 106 double a,b,c,d1,e; 107 beline li[10005]; 108 beline tmp; 109 p[0].x=0;p[0].y=5;//freopen("in.txt","r",stdin); 110 while(scanf("%d",&n)!=EOF && n!=-1) 111 { 112 for(i=0; i<1005; i++) 113 for(j=0; j<1005; j++) 114 mp[i][j] = INF; 115 int cas=1,css=0; 116 for(i=0; i<n; i++) 117 { 118 scanf("%lf%lf%lf%lf%lf",&a,&b,&c,&d1,&e); 119 li[css].st.x=a; 120 li[css].st.y=0; 121 p[cas].x=a; li[css].ed.x=a; 122 p[cas++].y=b;li[css++].ed.y=b; 123 p[cas].x=a; li[css].st.x=a; 124 p[cas++].y=c;li[css].st.y=c; 125 p[cas].x=a; li[css].ed.x=a; 126 p[cas++].y=d1;li[css++].ed.y=d1; 127 p[cas].x=a; li[css].st.x=a; 128 p[cas++].y=e;li[css].st.y=e; 129 li[css].ed.x=a; 130 li[css++].ed.y=10; 131 } 132 p[cas].x=10.0;p[cas].y=5.0; 133 for(i=0; i<=cas; i++) 134 { 135 for(j=i+1; j<=cas; j++) 136 { 137 int ok=0; 138 for(k=0; k<css; k++) 139 { 140 if(dd(p[i].x,p[j].x)||!dd(p[i].x,li[k].st.x)&&!dd(p[j].x,li[k].st.x)&&(segIntersect(p[i],p[j],li[k].st,li[k].ed))) 141 { 142 ok=1; 143 break; 144 } 145 } 146 if(!ok) 147 { 148 mp[j][i] = mp[i][j] = Dist(p[i],p[j]);//printf("%d %d %lf ^^\n",i,j,mp[i][j]); 149 } 150 } 151 } 152 Dijkstra(cas+1); 153 printf("%.2lf\n",d[cas]); 154 } 155 return 0; 156 }
View Code
转载于:https://www.cnblogs.com/ccccnzb/p/3893820.html
poj 1556 (Dijkstra + Geometry 线段相交)相关推荐
- 简单几何(线段相交+最短路) POJ 1556 The Doors
题目传送门 题意:从(0, 5)走到(10, 5),中间有一些门,走的路是直线,问最短的距离 分析:关键是建图,可以保存所有的点,两点连通的条件是线段和中间的线段都不相交,建立有向图,然后用Dijks ...
- 【POJ - 1556】The Doors (计算几何,线段相交)
题干: You are to find the length of the shortest path through a chamber containing obstructing walls. ...
- HDU1086You can Solve a Geometry Problem too(判断线段相交)
You can Solve a Geometry Problem too Time Limit: 2000/1000 MS (Java/Others) Memory Limit: 65536/3 ...
- Segments POJ 3304 直线与线段是否相交
题目大意:给出n条线段,问是否存在一条直线,使得n条线段在直线上的投影有至少一个公共点. 题目思路:如果假设成立,那么作该直线的垂线l,该垂线l与所有线段相交,且交点可为线段中的某两个交点 证明:若有 ...
- POJ 2653 Pick-up sticks (线段相交)
题意:给你n条线段依次放到二维平面上,问最后有哪些没与前面的线段相交,即它是顶上的线段 题解:数据弱,正向纯模拟可过 但是有一个陷阱:如果我们从后面向前枚举,找与前面哪些相交,再删除前面那些相交的线段 ...
- POJ 1410 Intersection 判断线段交和点在矩形内 【计算几何】
ACM博客_kuangbin POJ 1410 Intersection(判断线段交和点在矩形内) Intersection Time Limit: 1000MS Memory Limit: 10 ...
- POJ 1556 The Doors(计算几何+最短路)
这题就是,处理出没两个点.假设能够到达,就连一条边,推断可不能够到达,利用线段相交去推断就可以.最后求个最短路就可以 代码: #include <cstdio> #include < ...
- poj3304(线段相交问题)
题意:是否存在这样一条直线,使这条直线和所有的线段相交. #include<iostream> #include<algorithm> #include<cstring& ...
- poj1410(线段相交问题判断)
题意:给一个线段的两个端点坐标(确定线段),再给一个矩形的左上角的坐标和右下角的坐标(确定矩形),问判断该线段是否和矩形相交,在矩形内也算和矩形相交.直接用线段和矩形的四条边判断是否相交即可. #in ...
最新文章
- 在ASP.NET 2.0中使用样式、主题和皮肤
- ThreadLocal源码分析
- bzoj2154 Crash的数字表格
- Unity3D中使用KiiCloud总结一
- java中过滤器、监听器、拦截器的区别
- c# 获取machineguid_C#中怎么生成和获取GUID
- Unity 实现物体破碎效果(转)
- 【C#学习笔记】使用C#中的Dispatcher
- ROOBO公布A轮1亿美元融资 发布人工智能机器人系统
- linux c++应用程序内存高或者占用CPU高的解决方案_20161213
- 逻辑运算map函数filter函数reduce函数
- 数据结构 --- 线性表学习(php模拟)
- php遍历path树,php 递归遍历文件树代码_PHP教程
- 主成分分析 与 因子分析
- 如何保证分布式系统数据一致性
- 防雷击和浪涌电路设计以及放电管、压敏电阻、TVS管对比
- 我的深圳真实驾考经历
- 为什么计算机打不开优盘,U盘打不开,且8G的U盘只报64M,为什么?
- 海底捞成功的全套培训体系(收藏)
- 区块链技术发展现状与展望 论文阅读摘要(袁勇、王飞跃)
热门文章
- c++:opencv读图后mat矩阵的基本操作
- VMD_test matlab仿真
- 100行java电路程序_easyopen原理解析——不到100行代码实现一个最精简的easyopen
- iPhone音频播放后台控制
- SpringMVC---数据校验
- delphi 16 网页缩放
- XT910开通了GPRS却上不了网的原因--“数据漫游”功能关闭导致的
- 加密和解密.net配置节
- 什么是 ANSI C 和 GNU C
- C# System.Runtime.InteropServices 相关学习总结