使用opencv python进行手检测和手指计数
By seeing above image now you are very excited for implement it (like me). So not wasting too much time let’s jump to the code.
通过看到上面的图像,您现在很高兴实现它(像我一样)。 因此,不要浪费太多时间,让我们跳转到代码。
OpenCV (OpenCV)
OpenCV (Open Source Computer Vision Library) is an open source computer vision and machine learning software library. OpenCV was built to provide a common infrastructure for computer vision applications and to accelerate the use of machine perception in the commercial products.
OpenCV (开源计算机视觉库)是一个开源计算机视觉和机器学习软件库。 OpenCV构建旨在为计算机视觉应用程序提供通用的基础结构,并加速在商业产品中使用机器感知。
导入库 (Importing Libraries)
cv2: opencv [pip install opencv]
cv2: opencv [pip install opencv]
numpy: for handling arrays as well as for math [pip install numpy]
numpy:用于处理数组和数学[pip install numpy]
import cv2 as cvimport numpy as np
阅读影像 (Reading Image)
img_path = "data/palm.jpg"img = cv.imread(img_path)cv.imshow('palm image',img)

皮肤面具 (SkinMask)
It is used for highlighting specific color on image.
它用于突出显示图像上的特定颜色。
- hsvim : Change BGR (blue, green, red) image to HSV (hue, saturation, value).hsvim:将BGR(蓝色,绿色,红色)图像更改为HSV(色相,饱和度,值)。
- lower : lower range of skin color in HSV.较低:HSV中皮肤的颜色范围较低。
- upper : upper range of skin color in HSV.upper:HSV中皮肤颜色的上限。
- skinRegionHSV : Detect skin on the range of lower and upper pixel values in the HSV colorspace.skinRegionHSV:在HSV色彩空间的上下像素值范围内检测皮肤。
- blurred: bluring image to improve masking.模糊:使图像模糊以改善遮罩。
- thresh : applying threshing.脱粒:脱粒。
hsvim = cv.cvtColor(img, cv.COLOR_BGR2HSV)lower = np.array([0, 48, 80], dtype = "uint8")upper = np.array([20, 255, 255], dtype = "uint8")skinRegionHSV = cv.inRange(hsvim, lower, upper)blurred = cv.blur(skinRegionHSV, (2,2))ret,thresh = cv.threshold(blurred,0,255,cv.THRESH_BINARY)cv.imshow("thresh", thresh)

等高线 (Contours)
Now let’s finding contours on the image.
现在让我们在图像上找到轮廓。
contours, hierarchy = cv.findContours(thresh, cv.RETR_TREE, cv.CHAIN_APPROX_SIMPLE)contours = max(contours, key=lambda x: cv.contourArea(x))cv.drawContours(img, [contours], -1, (255,255,0), 2)cv.imshow("contours", img)

凸包 (Convex Hull)
hull = cv.convexHull(contours)cv.drawContours(img, [hull], -1, (0, 255, 255), 2)cv.imshow("hull", img)
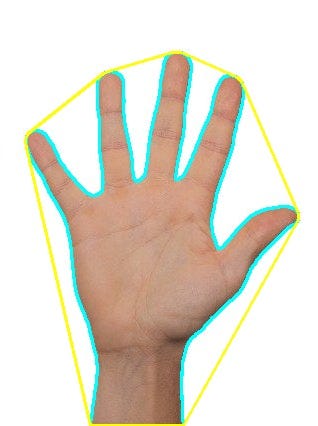
凸缺陷 (Convexity Defects)
Any deviation of the object from this hull can be considered as convexity defect.
物体与该船体的任何偏离都可以视为凸度缺陷。
hull = cv.convexHull(contours, returnPoints=False)defects = cv.convexityDefects(contours, hull)

余弦定理 (Cosine Theorem)
Now, this is Math time! Let’s understand cosine theorem.
现在,这是数学时间! 让我们了解余弦定理。
In trigonometry, the law of cosines relates the lengths of the sides of a triangle to the cosine of one of its angles. Using notation as in Fig. 1, the law of cosines states where γ denotes the angle contained between sides of lengths a and b and opposite the side of length c.
在三角学中,余弦定律将三角形边的长度与其角度之一的余弦相关。 使用如图1所示的符号表示,余弦定律表明,其中γ表示长度a和b的边之间的长度以及与长度c的边相对的角度。

式 (Formula)

By seeing this formula now we understand that if we have; a,b and gama then we also find c as well as if we have; a,b,c then we also find gamma (vice-versa)
通过现在看这个公式,我们知道如果有的话; a,b和gama然后我们也找到c以及是否有c ; a,b,c然后我们也找到伽玛(反之亦然)
For finding gamma this formula is used:
为了找到伽玛,使用以下公式:

使用余弦定理识别手指 (Using Cosine theorem to recognize fingers)

Sorry! for this dirty MS-Paint I am not a artist.
抱歉! 对于这个肮脏的MS-Paint,我不是艺术家。
In Fig. 2, I am draw a Side: a,b,c and angle: gamma. Now this gamma is always less than 90 degree, So we can say: If gamma is less than 90 degree or pi/2 we consider it as a finger.
在图2中,我画了一个Side: a,b,c和angle: gamma。 现在,该伽马始终小于90度,因此可以说:如果伽马小于90度或pi / 2 ,则将其视为手指。
数手指 (Counting Finger)
Note: if you not familiar with Convexity Defects please go and read this article by opencv docs. click here
注意:如果您不熟悉凸出缺陷,请转到opencv docs阅读本文。 点击这里
Convexity Defects returns an array where each row contains these values :
凸性缺陷返回一个数组,其中每一行都包含以下值:
start point
起点
end point
终点
farthest point
最远点
approximate distance to farthest point
到最远点的大概距离
By, this point we can easily derive Sides: a,b,c (see CODE) and from cosine theorem we can also derive gamma or angle between two finger. As you read earlier, if gamma is less than 90 degree we treated it as a finger. After knowing gamma we just draw circle with radius 4 in approximate distance to farthest point. And after we just simple put text in images we represent finger counts (cnt).
通过这一点,我们可以轻松得出Sides: a,b,c (请参见CODE),并且根据余弦定理,我们还可以得出两根手指之间的 伽马或角度。 如您先前所读,如果伽马小于90度,我们会将其视为手指。 知道伽玛后,我们只需画一个半径为4的圆, 到最远点的近似距离即可。 在将文本简单地放入图像中之后,我们就表示手指数(cnt)。
if defects is not None: cnt = 0for i in range(defects.shape[0]): # calculate the angle s, e, f, d = defects[i][0] start = tuple(contours[s][0]) end = tuple(contours[e][0]) far = tuple(contours[f][0]) a = np.sqrt((end[0] - start[0]) ** 2 + (end[1] - start[1]) ** 2) b = np.sqrt((far[0] - start[0]) ** 2 + (far[1] - start[1]) ** 2) c = np.sqrt((end[0] - far[0]) ** 2 + (end[1] - far[1]) ** 2) angle = np.arccos((b ** 2 + c ** 2 - a ** 2) / (2 * b * c)) # cosine theorem if angle <= np.pi / 2: # angle less than 90 degree, treat as fingers cnt += 1 cv.circle(img, far, 4, [0, 0, 255], -1)if cnt > 0: cnt = cnt+1cv.putText(img, str(cnt), (0, 50), cv.FONT_HERSHEY_SIMPLEX,1, (255, 0, 0) , 2, cv.LINE_AA)
让我们看看最终结果 (Let’s see our final result)
cv.imshow('final_result',img)

You can also do it for Videos, just by calling “cv.VideoCapture()”. If You want the code you can get in my GitHub: finger_counting_video.py
您也可以通过调用“ cv.VideoCapture()”来对视频执行此操作。 如果需要代码,可以在我的GitHub中获取: finger_counting_video.py
您也可以在YouTube中观看此教程视频。 (You can also watch this tutorial video in YouTube.)
翻译自: https://medium.com/analytics-vidhya/hand-detection-and-finger-counting-using-opencv-python-5b594704eb08
http://www.taodudu.cc/news/show-2858242.html
相关文章:
- html5跟随手指的小球,Android自定义圆形View实现小球跟随手指移动效果(详细介绍)...
- 基于OpenCV的手指只数检测
- Arduino使用手指测心跳模块
- 红米k30可以用鸿蒙系统吗,红米K30好用吗?Redmi K30上手评测
- php案例分析百度云_2019中国云手机市场红手指案例分析
- 云手机哪个好用最流畅?红手指、双子星、雷电云手机性价比推荐排行
- python 实用脚本_python实用脚本红手指导入
- javaweb成语接龙
- 8个成语接龙首尾相连_四字成语接龙连接8个
- Java成语接龙
- 成语接龙改版
- python 成语接龙
- 8个成语接龙首尾相连_八拜为交成语接龙
- python成语接龙到为所欲为_Python小伙用简单爬虫实现成语接龙小游戏!
- php 成语接龙api,再谈Weiphp公众平台开发——1、成语接龙插件
- python成语接龙到为所欲为_python成语接龙
- python中成语接龙游戏_python——成语接龙小游戏
- python成语接龙代码_实现成语接龙(Python)
- c语言成语接龙编程,C语言完成成语接龙小游戏
- 成语接龙php源码,weiphp——成语接龙插件的对接和使用
- python中成语接龙游戏_Python实现成语接龙
- JAVA设计模式之访问者模式
- sequelize模型关联_Node.js Sequelize 模型(表)之间的关联及关系模型的操作
- IDEA中Tomcat启动后提交表单,请求的资源[/servlet_demo2/book-add.html]不可用
- 【参赛作品29】基于openGauss数据库设计人力资源管理系统实验
- MySQL深入学习——第六章 查询优化批量导入操作学习笔记
- MYSQL面试总结
- MySQL高级2-优化分析
- 03.mysql调优--索引基本实现
- 《Entity Framework 6 Recipes》中文翻译系列 (32) ------ 第六章 继承与建模高级应用之TPH与TPT (1)...
使用opencv python进行手检测和手指计数相关推荐
- 基于 OpenCV 的手掌检测和手指计数
作者 | 努比 来源 | 小白学视觉 利用余弦定理使用OpenCV-Python实现手指计数与手掌检测. 手检测和手指计数 接下来让我们一起探索以下这个功能是如何实现的. OpenCV OpenCV( ...
- 【CV】基于OpenCV的手掌检测和手指计数
利用余弦定理使用OpenCV-Python实现手指计数与手掌检测. 手检测和手指计数 接下来让我们一起探索以下这个功能是如何实现的. OpenCV OpenCV(开源计算机视觉库)是一个开源计算机视觉 ...
- 基于OpenCV的手掌检测和手指计数
点击上方"小白学视觉",选择加"星标"或"置顶" 重磅干货,第一时间送达 利用余弦定理使用OpenCV-Python实现手指计数与手掌检测. ...
- OpenCV + python 实现人脸检测(基于照片和视频进行检测)
OpenCV + python 实现人脸检测(基于照片和视频进行检测) Haar-like 通俗的来讲,就是作为人脸特征即可. Haar特征值反映了图像的灰度变化情况.例如:脸部的一些特征能由矩形特征 ...
- 教你用OpenCV和Python实现手掌检测和手掌计数
点击上方"码农的后花园",选择"星标" 公众号 精选文章,第一时间送达 最近号主由于某些原因,并没有定期更新,但是一直记着你们呢,你们只要记住号主与你们同在 ...
- 基于Opencv+python的车流量检测项目
目录 项目介绍 整体流程 调试环境 项目流程 1.预处理 2.汽车识别--去背景算法(KNN/MOG2) 3.统计车流量数目 结尾 源代码 测试视频资料 流程图 项目介绍 本次项目主要采用了传统视觉的 ...
- OpenCV+python:人脸检测
1,人脸检测简介 人脸检测的模型主要有两类,一类是知识模型,根据眼睛.嘴.鼻子的相对位置或面部不同部位的颜色深度差异来检测人脸,另一类是统计模型,把海量的人脸数据转换成二维像素矩阵,从统计的观点出发构 ...
- OpenCV+python:圆检测
1,圆检测基本原理 对霍夫变换有了这样一种理解-----实际上就是坐标变换,是一种数学上的变换,然后再转换到参数坐标系进行讨论,最终确定待检测圆(或者其他形状)的数学方程.在极坐标系下,圆的数学表达式 ...
- OpenCV—python OCR文本检测
文章目录 一.形态学文本区域检测 1.1 直接匹配 1.2 多尺度匹配 二.MSER+NMS文本区域检测 2.1 MSER 2.2 NMS 一.形态学文本区域检测 图像形态学操作,包括膨胀.腐蚀基本操 ...
最新文章
- Shell tips
- linux中iso文件怎么安装系统文件,我有linux的iso文件,要怎么安装系统
- python if语句多个条件-python – if / elif语句的多个条件
- JS循环绑定对象或变量
- vb.net2019-Accord.Net机器学习库安装与SVM简单分类
- ASP.NET Core Razor 页面使用教程
- php dbutils 使用,dbutilsapi
- qt creator源码全方面分析(3-1)
- How to convert any valid date string to a DateTime.
- 21、方法的-、+符号
- Web测试-Web界面易用性测试
- 【项目实践】充电台灯电路拆解
- 图像CMYK模式转RGB模式
- 易基因 | 表观技术:单细胞及微量细胞全基因组重亚硫酸盐甲基化测序(scWGBS)
- ubuntu禁止指定软件包更新
- 空间数据库Topic推荐-AMiner
- 解决:Not creating XLA devices, tf_xla_enable_xla_devices not set
- 【DBC专题】-2-CAN Signal信号的Multiplexor多路复用在DBC中实现
- 解决GoLand上面出现的Couldn‘t copy unpacked SDK问题
- 不可小觑的吃内存大户