phpquery使用_使用phpQuery的服务器端HTML处理
phpquery使用
In our day to day tasks of web development it is necessary for us to work with both client and server-side code. We write the business logic using PHP and generate the HTML to be displayed in the users’ browsers. Then we use frameworks such as jQuery or Prototype to provide client-side interactivity.
在Web开发的日常任务中,我们必须同时使用客户端和服务器端代码。 我们使用PHP编写业务逻辑,并生成要在用户浏览器中显示HTML。 然后,我们使用jQuery或Prototype之类的框架来提供客户端交互性。
Now think about how you can change and manipulate the generated HTML using server-side PHP code. phpQuery is the ultimate solution that will come to your mind. If you haven’t heard about phpQuery, you may be interested in this article since I am going to give you a brief introduction to phpQuery and explain how you can use it in real-world projects.
现在考虑如何使用服务器端PHP代码更改和操作生成HTML。 phpQuery是您想到的最终解决方案。 如果您还没有听说过phpQuery,那么您可能会对本文感兴趣,因为我将向您简要介绍phpQuery并解释如何在实际项目中使用它。
什么是phpQuery (What is phpQuery)
phpQuery is a server-side, chainable, CSS3 selector driven Document Object Model (DOM) API based on jQuery JavaScript Library.
phpQuery是基于jQuery JavaScript库的服务器端可链接CSS3选择器驱动的文档对象模型(DOM)API。
This is the definition given on the official phpQuery project page. If you have used jQuery, then you will have an idea of how it can simplify many tasks requiring DOM manipulation. phpQuery provides exactly the same functionalities to be used inside your server-side PHP code. You can say good bye to untidy HTML code generation using echo statements and similar methods.
这是在官方phpQuery项目页面上给出的定义。 如果您使用过jQuery,那么您将了解如何简化许多需要DOM操作的任务。 phpQuery提供与服务器端PHP代码内部完全相同的功能。 您可以告别使用echo语句和类似方法进行不整洁HTML代码生成。
You will have access to most of the functionality provided by jQuery in phpQuery, which can broadly be divided into the 4 tasks mentioned below:
您将可以使用jQuery在phpQuery中提供的大多数功能,这些功能可以大致分为以下4个任务:
- Creating DOM elements创建DOM元素
- Selecting and Manipulating elements选择和操作元素
- Iterating through the DOM遍历DOM
- Printing the output to the browser将输出打印到浏览器
You can execute the tasks using the features provided by phpQuery which is known as “ported jQuery sections.” Let’s see the features first:
您可以使用phpQuery提供的功能(称为“ jQuery移植部分”)执行任务。 首先让我们看一下这些功能:
Selectors – find elements based on given condition.
选择器 –根据给定条件查找元素。
Attributes – work with attributes of DOM elements.
属性 –使用DOM元素的属性。
Traversing – travel through list of selected elements.
遍历 – 遍历选定元素的列表。
Manipulation – add and remove content on selected elements.
操作 –添加和删除所选元素上的内容。
Ajax – create server side ajax requests.
Ajax –创建服务器端ajax请求。
Events – bind DOM events on selected elements.
事件 –在选定元素上绑定DOM事件。
Utilities – generic functions to support other features.
实用程序 –支持其他功能的通用功能。
You can download the phpQuery library from the project page at code.google.com/p/phpquery. Copy the folder to your web server and you are ready to go. Installation is simple as that, and you can execute the demo.php
file to get started.
您可以从项目页面code.google.com/p/phpquery下载phpQuery库。 将文件夹复制到Web服务器,即可开始使用。 这样安装很简单,您可以执行demo.php
文件开始。
如何使用phpQuery (How to Use phpQuery)
I’m going to show you how to create a two-column unordered list with headers and different row colors for odd and even rows, as shown in the image below:
我将向您展示如何创建一个两列无序列表,其标题和奇数行和偶数行的行颜色不同,如下图所示:
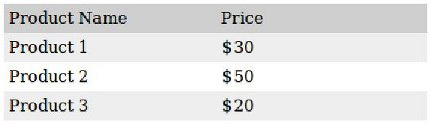
First, let’s create an HTML document using phpQuery:
首先,让我们使用phpQuery创建一个HTML文档:
<?php
require("phpQuery/phpQuery.php");
$doc = phpQuery::newDocument("<div/>");
The above code will create a basic HTML document with a div
tag. The library provides various methods of creating documents; I have used the simplest one, but you can find others in demo.php
and the documentation.
上面的代码将创建一个带有div
标签的基本HTML文档。 该库提供了多种创建文档的方法; 我使用了最简单的方法,但是您可以在demo.php
和文档中找到其他方法。
Now we need to create an unordered list and add it to our HTML document.
现在我们需要创建一个无序列表,并将其添加到我们HTML文档中。
<?php
...
$doc["div"]->append("<ul><li>Product Name</li><li>Price</li></ul>");
$products = array(
array("Product 1","$30"),
array("Product 2","$50"),
array("Product 3","$20"));
foreach($products as $key=>$product) {
$doc["div ul"]->append("<li>$product[0]</li><li>$product[1]</li>");
}
print $doc;
You can see that we have the unordered list now. But all the elements are in a single column, which is the default. We have to move the even elements of the list into a second column.
您可以看到我们现在有无序列表。 但是所有元素都在单个列中,这是默认设置。 我们必须将列表的偶数元素移到第二列。
<?php
...
$doc["div ul"]->attr("style", "width:420px;");
$doc["div ul"]->find("li:even")->attr("style","width:200px; float:left; padding:5px; list-style:none;");
$doc["div ul"]->find("li:odd")->attr("style","width:200px; float:left; padding:5px; list-style:none;");
I’m using the style attribute to define the CSS styles required for our example here, but it’s not recommended to use inline styles unless it’s really needed. Always use CSS classes to add styles.
我在这里使用style属性定义我们的示例所需CSS样式,但是除非确实需要,否则不建议使用内联样式。 始终使用CSS类添加样式。
Now, let’s highlight the header and even numbered rows using phpQuery methods.
现在,让我们使用phpQuery方法突出显示标题和偶数行。
<?php
...
$doc["div ul"]->find("li:nth-child(4n)")->attr("style","background:#EEE; width:200px; float:left; padding:5px; list-style:none;");
$doc["div ul"]->find("li:nth-child(4n-1)")->attr("style","background:#EEE; width:200px; float:left; padding:5px; list-style:none;");
$doc["div ul"]->find("li:lt(1)")->attr("style","background:#CFCFCF; width:200px; float:left; padding:5px; list-style:none;");
We have completed our simple example, and you should now have an idea of how phpQuery can be used to simplify HTML generation server-side. Everything we did is almost the same as would be done with jQuery, except we did all the actions against the $doc
object.
我们已经完成了简单的示例,现在您应该了解如何使用phpQuery简化服务器端HTML生成。 除了对$doc
对象执行了所有操作外,我们所做的一切与使用jQuery几乎相同。
phpQuery的重要性 (Importance of phpQuery)
Even though I explained the functionality of phpQuery, you must be wondering why we need the library when we have jQuery on the client-side. I’ll show the importance of phpQuery using a practical scenario.
即使我解释了phpQuery的功能,您仍然想知道为什么在客户端使用jQuery时为什么需要该库。 我将在实际情况中展示phpQuery的重要性。
Consider the following situation: assume we have a table like the following, which has all the information about web developers who went to an interview.
考虑以下情况:假设我们有一个类似于以下的表格,其中包含有关参加面试的Web开发人员的所有信息。
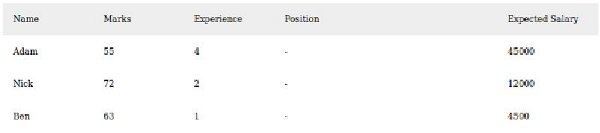
Now here’s the list of requirements we have to develop in this scenario:
现在,这是在这种情况下必须开发的需求列表:
- Applicants who got a mark over 60 for the exam should be highlighted in blue.考试成绩超过60分的申请人应以蓝色突出显示。
- Applicants with more than 3 years working experience should have a link in front labeled “Apply for Senior Software Engineer” and other applicants should have the link “Apply for Software Engineer”.具有3年以上工作经验的申请人应该在前面带有“申请高级软件工程师”的链接,而其他申请人应该具有“申请软件工程师”的链接。
The company has a salary structure based on experience:
该公司具有基于经验的薪资结构:
- 1 year – $5,000一年– $ 5,000
- 2 years – $10,0002年– 10,000美元
- 3 years – $20,0003年– $ 20,000
- more than 3 years – $50,0003年以上– 50,000美元
The company has a salary structure based on experience:
该公司具有基于经验的薪资结构:
The salary column should be highlighted in green for applicants who match the criteria.
对于符合条件的申请人,薪水列应以绿色突出显示。
This is how the output should look:
这是输出的外观:
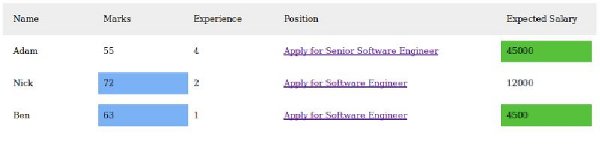
A developer might provide the following solution to meet the requirements using pure PHP code.
开发人员可能会使用纯PHP代码提供以下解决方案来满足要求。
<?php
// retrieve applicants from database
//...
echo <<<ENDHTML
<div id="main">
<div class="row_head">
<div>Name</div>
<div>Marks</div>
<div>Experience</div>
<div>Position</div>
<div>Expected Salary</div>
</div>
ENDHTML;
foreach ($applicants as $applicant) {
echo '<div class="row">';
echo "<div>" . $applicant["name"] . "</div>";
echo '<div class="' . marksClass($applicant["marks"]) . '">' . $applicant["marks"] . "</div>";
echo "<div>" . $applicant["experience"] . "</div>";
echo "<div>" . positionLink($applicant["experience"]) . "</div>";
echo '<div class="' . salaryClass($applicant["experience"], $applicant["salary"]) . '">' . $applicant["salary"] . "</div>";
echo "</div>";
}
echo "</div>";
function marksClass($info) {
return ($info > 60) ? "pass" : "fail";
}
function positionLink($experience) {
return ($experience > 3)
? '<a href="#">Apply for Senior Software Engineer</a>'
: '<a href="#">Apply for Software Engineer</a>';
}
function salaryClass($experience, $salary) {
switch ($experience) {
case 1:
return ($salary < 5000) ? "accept" : "reject";
case 2:
return ($salary < 10000) ? "accept" : "reject";
case 3:
return ($salary < 20000) ? "accept" : "reject";
default:
return ($salary < 50000) ? "accept" : "reject";
}
}
Now let’s do it using phpQuery and compare the code and advantages.
现在,让我们使用phpQuery进行比较,并比较代码和优点。
<?php
require("phpQuery/phpQuery.php");
$doc = phpQuery::newDocument('<div id="main"></div>');
phpQuery::selectDocument($doc);
// retrieve applicants from database
//...
$doc["#main"]->append('
<div id="main">
<div class="row_head">
<div>Name</div>
<div>Marks</div>
<div>Experience</div>
<div>Position</div>
<div>Expected Salary</div>
</div>');
foreach ($applicants as $key => $applicant) {
$doc["#main"]->append('<div class="row" id="app_' . $key . '"></div>');
foreach ($applicant as $field => $info) {
$doc["#main"]->find("#app_" . $key)->append('<div class="_' . $field . '">' . $info . "</div>");
if ($field == "experience") {
$doc["#main"]->find("#app_" . $key)->append('<div style="width:400px" class="_position">-</div>');
}
}
}
addMarksClass($doc);
addSalaryClass($doc);
addPositionLink($doc);
print $doc;
function addMarksClass(&$doc) {
$marks = pq("._marks");
foreach ($marks as $appMark) {
if (pq($appMark)->html() > 60) {
pq($appMark)->addClass("pass");
}
else {
pq($appMark)->addClass("fail");
}
}
}
function addSalaryClass(&$doc) {
$marks = pq("._salary");
foreach ($marks as $appMark) {
$experience = pq($appMark)->parent()->find("._experience" )->html();
$salary = pq($appMark)->html();
switch ($experience) {
case 1:
pq($appMark)->addClass(
($salary < 5000) ? "accept" : "reject"
);
break;
case 2:
pq($appMark)->addClass(
($salary < 10000) ? "accept" : "reject"
);
break;
case 3:
pq($appMark)->addClass(
($salary < 20000) ? "accept" : "reject"
);
break;
default:
pq($appMark)->addClass(
($salary < 50000) ? "accept" : "reject"
);
}
}
}
function addPositionLink(&$doc) {
$experience = pq("._experience");
foreach ($experiece as $appExp) {
if (pq($appExp)->html() > 3) {
pq($appExp)->parent()->find("._position")->html('<a href="#">Apply for Senior Software Engineer</a>');
}
else{
pq($appExp)->parent()->find("._position")->html('<a href="#">Apply for Software Engineer</a>');
}
}
}
phpQuery is easy if you have the knowledge of working with jQuery already. Most of the above code will be self-explanatory. I want to mention though that pq()
refers to the current document. All the others are jQuery functions.
如果您已经了解使用jQuery的知识,则phpQuery很容易。 上面的大多数代码都是不言自明的。 我想提一下,尽管pq()
是指当前文档。 所有其他都是jQuery函数。
And even though both look similar, the code which uses phpQuery provides better quality and extendibility. Think how brittle the original code can be if you have to add extra functionality later. Let’s assume we want to add additional validation on marks based on the working experience. In that scenario you’d have to add another method and assign the returned result inside the foreach
loop. That means you have to change already written code, violating the Open-Closed Principle:
即使两者看起来相似,使用phpQuery的代码也可以提供更好的质量和可扩展性。 考虑一下如果以后必须添加额外的功能,原始代码可能会很脆弱。 假设我们要根据工作经验在标记上添加其他验证。 在那种情况下,您将不得不添加另一个方法并在foreach
循环内分配返回的结果。 这意味着您必须更改已经编写的代码,从而违反开放式封闭原则 :
Software entities (classes, modules, functions, etc.) should be open for extension, but closed for modification.
软件实体(类,模块,功能等)应打开以进行扩展,但应关闭以进行修改。
With the second example which uses phpQuery, the code is first generated without any validation, and then we pass the table into each function and the changes are placed into the original table. Each function does not affect the other functions, so we can write a new function for any new requirements and use it outside the loop with the other functions. We don’t modify already existing code, which sounds good, right? This is called decoration:
在使用phpQuery的第二个示例中,代码首先在没有任何验证的情况下生成,然后将表传递给每个函数,并将所做的更改放入原始表中。 每个函数都不会影响其他函数,因此我们可以为任何新要求编写一个新函数,并将其与其他函数一起循环使用。 我们不修改已有的代码,听起来不错,对吗? 这称为装饰 :
Decorator pattern is a design pattern that allows behavior to be added to an existing object dynamically.
装饰器模式是一种设计模式,它允许将行为动态添加到现有对象。
摘要 (Summary)
We started this tutorial by introducing phpQuery features and its importance. After learning how to use phpQuery using a simple example, we moved to practical example where it became much more important in improving the quality of code. phpQuery has provided us with a new perspective to working with HTML in server side, and I hope you will use phpQuery in different ways and share your personal experiences in the comments below.
我们通过介绍phpQuery功能及其重要性开始了本教程。 在通过一个简单的示例学习了如何使用phpQuery之后,我们转到了实际示例,该示例在提高代码质量中变得越来越重要。 phpQuery为我们提供了在服务器端使用HTML的新视角,我希望您将以不同的方式使用phpQuery并在下面的评论中分享您的个人经验。
翻译自: https://www.sitepoint.com/server-side-html-handling-using-phpquery/
phpquery使用
phpquery使用_使用phpQuery的服务器端HTML处理相关推荐
- jboss url路径_在JBoss的服务器端正确解码URL参数
jboss url路径 我今天花了很多时间来弄清楚如何在运行在JBoss上的JSF应用程序中(使用JBoss 7 Final)强制正确解码编码的字符. 当您有例如通过URL传递中文字符时,就会发生此问 ...
- 《白帽子讲web安全》读书笔记_2021年7月16日(2)_第3篇 服务器端应用安全
第7章 注入攻击 7.1 SQL注入 注入攻击是web安全领域中一种最为常见的攻击方式. XSS本质上也是一种针对HTML的注入攻击. 在第1篇 我的安全世界观中,提出了一个安全设计原则--" ...
- p2p云服务是什么_先锋P2P云服务器端 V8.0.0.0正式版-完美软件下载
先锋P2P云服务器端是一个完整的P2P枢纽和加速网络中心,无缝协调大规模P2P网络集群.强大的分布式网络中心,让你自由解析,保证网络服务的有效性.完整的P2P网络生态系统万能格式分发功能强大的媒体管理 ...
- 接口报错500是什么意思_一次排查服务器端接口报500错误的经历
1 出现问题情景 该问题来自我实习期间完成的一个博客后端系统,具体如下:当我辛辛苦苦在编辑器里完成文章格式修改(字数较多,一般大于3000字:字数较少时不会出现问题),以及相关目录和标签的选定,点击提 ...
- python socket服务器_记-python socket服务器端四部曲
1.创建socket对象 s = socket.socket(socket.AF_INET, socket.SOCK_STREAM) #创建一个TCPsocket对象\ 2.设置socket选项 s. ...
- 极光推送java服务器端_极光推送服务器端(JAVA)
准备工作:appkey和secret两个码.(在极光官网管理页面新增相关应用,就会获得对应的appkey和secret,这部分由移动端同事完成) 1.在pom文件下添加这个包: 2.添加工具类JPus ...
- p2p云服务是什么_先锋p2p云服务器端(XfServer)下载 v8.0
XfServer是影音先锋官方推出的一款基于P2P技术的影音点播服务器,目前官方已发布XfServer云服务器64位版本,欢迎大家下载使用. 官方介绍: XfServer凭借先锋强大的P2P" ...
- phpQuery的使用
前言 为什么使用phpQuery phpQuery是基于php5新添加的DOMDocument.而DOMDocument则是专门用来处理html/xml.它提供了强大的xpath选择器及其他很多htm ...
- PHP获取客户端和服务器端IP
1. $ _ SERVER ['REMOTE_ADDR']客户端IP,有可能是用户的IP,也可能是代理的IP. 2. $ _ SERVER ['HTTP_CLIENT_IP']代理端的IP,可能存在可 ...
最新文章
- java接口防抖_前端性能优化:高频执行事件/方法的防抖
- java线程中等待_Java:线程中的Thread.sleep():没有等待
- oracle 创建带参数的视图
- 2016年科技阅读列表
- C++ static与const用法详解
- 将图片以流的形式保存到数据库(Image)以及从数据库中取出显示
- 自动驾驶全球产业链全景图
- uwb定位与wifi、蓝牙和RFID定位技术的区别
- C语言经典例23-输出菱形图案
- 学生计算机游戏代码,给计算机学院的学幼们贴一些游戏代码
- Oracle 使用xtts升级11g-to-19c
- python—最大公约数和最小公倍数
- android ui设计最新字体,ui用什么字体_安卓ui设计用什么字体
- 如何更改计算机名称及查看自己计算机的型号
- arcgis怎么关联excel表_arcgis中如何跟excel连接?
- Android中singleTask模式没起作用!!
- C/C++ 控制台高级操作(非常详细)
- 小工具:用C++读取TGA并输出数据到文本
- Bilibili支持了AV1编码,关于AV1编码你知道吗?
- 纯html css动画效果,8个超震撼的HTML5和纯CSS3动画源码
热门文章
- 谈谈 Raft 算法
- 【办公效率】更换笔记本屏幕后无法正常显示
- 没出货和人气可能问题在直播流程上
- java连接mysql数据库时出现乱码_java连接mysql数据库乱码怎么办
- bootstrapTable行内编辑----X-editable组件
- RandomErasing代码讲解与使用
- python-中介者模式
- CMAKE安装遇到的问题cannot find propriate Makefile Processor
- 【恩墨学院】迈向信息化2.0:贵州交警“互联网+智慧交通”云化架构的探索实践...
- JAVA实现类似淘宝物流路线图