python 面试问题_值得阅读的30个Python面试问题
python 面试问题
Interview questions are quite tricky to predict. In most cases, even peoples with great programming ability fail to answer some simple questions. Solving the problem with your code is not enough. Often, the interviewer will expect you to have depth knowledge in a particular domain. One must have knowledge of internal mechanisms of the programming concepts. This article will help you to understand the most important concepts in Python.
面试问题很难预测。 在大多数情况下,即使编程能力强的人也无法回答一些简单的问题。 解决您的代码问题还不够。 面试官通常会希望您对特定领域有深入的了解。 必须了解编程概念的内部机制。 本文将帮助您了解Python中最重要的概念。
Let’s get started with the questions.
让我们开始思考这些问题。
1.为什么Python被称为解释语言? (1. Why Python is called as Interpreted language?)
Many books state that Python is an interpreted language. A programming language follows any one of the two approaches to implement the code. The approaches are compilation and interpretation. Some languages follow both concepts.
许多书指出Python是一种解释语言。 编程语言遵循两种方法中的任何一种来实现代码。 这些方法是编译和解释。 某些语言遵循这两个概念。

During compilation, the entire source code is converted into machine code. Machine code is understandable by the CPU. The program will be executed only if the entire code is compiled successfully.
在编译期间,整个源代码都将转换为机器代码。 CPU可以理解机器代码。 仅在成功编译整个代码后,程序才会执行。
In interpretation, the code is executed line by line. Each line of source code in Python is translated into machine code during the execution. There is a Virtual Machine available to interpret the python code. The compilation and interpretation runtime structure is given in the above diagram.
在解释中,代码是逐行执行的。 在执行过程中,Python中的每一行源代码都会转换为机器代码。 有一个虚拟机可用于解释python代码。 上图中给出了编译和解释运行时结构。
2.什么是动态类型语言? (2. What is dynamically typed language?)
It is one of the behaviors of high level programming language to check the type of data stored in a variable during the execution. In programming languages such as C, we must declare the type of data before using it.
在执行过程中检查存储在变量中的数据类型是高级编程语言的行为之一。 在诸如C的编程语言中,我们必须在使用数据之前声明数据的类型。

Python do not requires and declaration of data type. The python interpreter will know the data type when we assign a value to a variable. This is why python is known as dynamically typed language.
Python不需要数据类型的声明。 当我们给变量赋值时,python解释器会知道数据类型。 这就是为什么python被称为动态类型语言的原因。
3. Python中的PEP8是什么? (3. What is PEP8 in Python?)
Python known for its readability. In most cases, we can understand the python code better than any other programming languages. Writing beautiful and readable code is an art. PEP8 is an official style guide given by the community to improve the readability to the top.
Python以可读性着称。 在大多数情况下,我们可以比其他任何编程语言更好地理解python代码。 编写漂亮且可读的代码是一门艺术。 PEP8是社区提供的官方样式指南,旨在提高顶部的可读性。
“The code is read much more often than it is written”
“代码被读取的次数要多于其编写的次数”
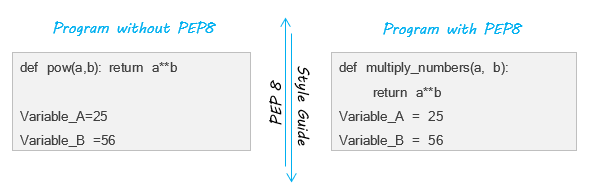
PEP8 enables you to write the python code more effective. Some naming conventions and comments will be helpful to share your code with other people to understand the code better.
PEP8使您可以更有效地编写python代码。 一些命名约定和注释将有助于与其他人共享您的代码以更好地理解代码。
4. Python的内存管理系统如何工作? (4. How Python’s memory management system works?)
A python program will contain many objects and data types in it. Allocating memory for each object is must. In python heap spaces are used to store data chunks. CPython contains a memory manager that manages the heap space in memory.
python程序中将包含许多对象和数据类型。 必须为每个对象分配内存。 在python中,堆空间用于存储数据块。 CPython包含一个内存管理器,用于管理内存中的堆空间。
Managing memory is an important thing in a program. The memory allocation determines the performance of the program. Python’s memory manager handles sharing of information, data caching and garbage collection, etc. This memory manager contains automatic garbage collection.
管理内存在程序中很重要。 内存分配决定了程序的性能。 Python的内存管理器处理信息共享,数据缓存和垃圾回收等。此内存管理器包含自动垃圾回收。
5.什么是可变性? 每种数据类型有何不同? (5. What is mutability? How it differs in each data types?)
The ability of a python object to change itself is called mutability. All objects in python are either mutable or immutable. Once created the immutable objects cannot be changed. But, the mutable objects can be changed.
python对象改变自身的能力称为可变性。 python中的所有对象都是可变的或不可变的。 一旦创建了不变的对象就无法更改。 但是,可变对象可以更改。
Let us try to check the mutability of two different type of objects in Python.
让我们尝试检查Python中两种不同类型的对象的可变性。
List - Mutable object
列表-可变对象
my_list = [1, 2, 3, 4, 5]my_list[2] = 7print(my_list)
Output
输出量
[1, 2, 7, 4, 5]
Tuple - Immutable object
元组-不可变对象
my_tuple = (1, 2, 3, 4, 5)my_tuple[2] = 7print(my_tuple)
Output
输出量
Traceback (most recent call last): File "main.py", line 2, in <module> my_tuple[2] = 7TypeError: 'tuple' object does not support item assignment
6. Python中的命名空间是什么? (6. What is Namespace in Python?)
Name is an identifier given to an object in Python. Every object in python is associated with a particular name. For example, when we assign the value 9 to a variable name number
, the object that holds the number is associated with the name number
.
名称是在Python中赋予对象的标识符。 python中的每个对象都与一个特定的名称相关联。 例如,当我们将值9分配给变量名称number
,保存该数字的对象与name number
相关联。
Namespace is a collection of names associated with every object in Python. The namespace contains all the isolated names and created when we executing the program. As the namespace contains the useful keywords and methods, we can access the , methods easily. The namespace stack controls the scope of variables in python.
命名空间是与Python中每个对象关联的名称的集合。 命名空间包含所有隔离的名称,并在我们执行程序时创建。 由于名称空间包含有用的关键字和方法,因此我们可以轻松地访问方法。 命名空间堆栈控制python中变量的范围。
7. System中的空执行是什么? (7. What is null execution in System?)
In python, the keyword pass
is used to create a statement that do nothing. If someone need to create a function with null operation then we can use the pass
statement.
在python中,关键字pass
用于创建不执行任何操作的语句。 如果有人需要使用null操作创建函数,则可以使用pass
语句。
We can create null function to check whether it works or not. If you are going to develop the implementation steps in a function in future, we can use pass
statement in it.
我们可以创建null函数来检查它是否有效。 如果将来要在某个函数中开发实现步骤,则可以在其中使用pass
语句。
Don’t get confused with comments. Comments and pass statements are different things. The interpreter ignores the comments in a program whereas pass
statement do null operation.
不要与评论混淆。 注释和通过语句是不同的东西。 解释器忽略程序中的注释,而pass
语句则执行空操作。
The following program is using pass statement in it.
以下程序在其中使用pass语句。
def function(): passfunction()
8.什么是字符串切片? (8. What is string slicing?)
String slicing is the process of making subsets from strings. We can pass a range in the following syntax to slice the string.
字符串切片是从字符串中产生子集的过程。 我们可以使用以下语法传递范围以对字符串进行切片。
Syntax: string[start:end:step]
语法: 字符串[开始:结束:步骤]
Consider the string “Hello World”. We can slice the string using the range values. To print ‘Hello’ from the string. Our range should be 0 to 5. The slicing creates sub string from start
index to end-1
th index.
考虑字符串“ Hello World”。 我们可以使用范围值对字符串进行切片。 从字符串中打印“ Hello”。 我们的范围应为0到5。切片将创建从start
索引到第end-1
个end-1
索引的子字符串。
string = "Hello World"print(string[0:5:1])print(string[6:11:1])
Output
输出量
HelloWorld
9.用Python连接两个字符串的方法是什么? 哪个更好? (9. What are the ways to concatenate two strings in Python? Which is better?)
We can use join()
or +
for concatenating two or more strings. The following codes will explain how to use those methods in Python.
我们可以使用join()
或+
连接两个或多个字符串。 以下代码将说明如何在Python中使用这些方法。
string_1 = "Hello"string_2 = "World"print(string_1 + string_2)print(''.join([string_1, string_2]))
Output
输出量
HelloWorldHello World
Comparing the both methods using join
method is good choice. When we need to create a sentence from more than two strings we should use +
each time. Join method is helpful to join an iterable with more strings in it.
比较使用join
方法的两种方法是不错的选择。 当我们需要用两个以上的字符串创建一个句子时,我们应该每次使用+
。 Join方法有助于连接其中包含更多字符串的迭代器。
10. Python中的装饰器是什么? (10. What is decorator in Python?)
Decorator is tool that allows us to modify the behavior of a function or a class. The decorator objects are called just before the function that you want modify.
装饰器是允许我们修改函数或类的行为的工具。 装饰器对象在要修改的函数之前调用。
11. Python中的生成器是什么? (11. What is generator in Python?)
Generator is a method to create iterable objects in python. Creating generators are same as creating functions. Instead of return statement we have to use yield in generator function. It returns iterating object each time it is called.
Generator是在python中创建可迭代对象的方法。 创建生成器与创建函数相同。 代替return语句,我们必须在生成器函数中使用yield。 每次调用它都会返回迭代对象。
12. Python中的lambda表达式是什么? (12. What is lambda expression in Python?)
Lambda is an anonymous function that helps us to create functions in single line of code. This is an important thing in functional programming. The lambda function should contains only one expressions in it.
Lambda是一个匿名函数,可以帮助我们在单行代码中创建函数。 这在函数式编程中很重要。 lambda函数中应仅包含一个表达式。
Syntax: lambda arguments : expression
语法: lambda参数:表达式
Y = lambda X : X*5print(Y(7))
Output
输出量
35
13.如何用逗号分隔的输入列出清单? (13. How to make a list from comma separated inputs?)
To make a list from a comma separated values or string we can use split
method. Split method splits the comma separated values by taking comma as dilimeter.
要使用逗号分隔的值或字符串列出列表,我们可以使用split
方法。 拆分方法将逗号分隔为逗号分隔值。
a = "A,B,C,D,E"print(a.split(','))
Output
输出量
['A', 'B', 'C', 'D', 'E']
14.普通分隔和地板分隔有什么区别? (14. What is the difference between normal division and floor division?)
The arithmetic operators contains two different types of division operators. One is normal division \
another one is \\
. The normal division returns the float value as result. The floor division returns the whole number as result.
算术运算符包含两种不同类型的除法运算符。 一个是普通除法\
另一个是\\
。 普通除法返回浮点值作为结果。 楼层划分返回整数结果。
a = 5b = 2print(a/b)print(a//b)
Output
输出量
2.52
15. Python中'is'和'=='有什么区别? (15. What is the difference between ‘is’ and ‘==’ in Python?)
We use is
and ==
to comparing to objects. But both works in different manner. The ==
compares the values of two objects whereas the is
keyword checks whether two objects are same or not.
我们使用is
和==
与对象进行比较。 但是两者的工作方式不同。 ==
比较两个对象的值,而is
关键字检查两个对象是否相同。
Using ==
in Python
在Python中使用==
a = [1, 3, 5]b = [1, 3, 5]c = a #Copy of a in cprint(a==b) #Same Values but different objectsprint(a==c) #Same Values Same objects
Output
输出量
TrueTrue
Using is
in Python
使用is
在Python
a = [1, 3, 5]b = [1, 3, 5]c = a #Copy of a in cprint(a is b) #Same Values but different objectsprint(a is c) #Same Values Same Objects
Output
输出量
FalseTrue
16. Python中的List和Tuple有什么区别? (16. What are the difference between List and Tuple in Python?)
- List is enclosed by brackets whereas the tuples are created using parenthesis.列表用方括号括起来,而元组使用括号创建。
- The effectiveness of tuple is higher than the list. So it works faster.元组的有效性高于列表。 因此它工作更快。
- The list is mutable object whereas the tuples are immutable objects.该列表是可变对象,而元组是不可变对象。
17.什么是Python中的负取整? (17. What is negative rounding in Python?)
We know that the round
keyword in python is used to to rounding of decimal places. It can be used to round off whole numbers sing negative rounding. Let the value of the variable X be 1345. To make is 1300 we have use negative rounding.
我们知道python中的round
关键字用于舍入小数位。 可以使用负数舍入来舍入整数。 假设变量X的值为1345。为了使值为1300,我们使用了负取整。
X = 1345print(round(X,-2))
Output
输出量
1300
18.如何防止以下代码异常? (18. How do you prevent the following code from exception?)
def function(): a = 5function()print(a)
The scope of the variable a is inside the function. To access the variable across the program we have declare it as global variable.
变量a的范围在函数内部。 要在程序中访问变量,我们已将其声明为全局变量。
def function(): global a a = 5function()print(a)
Output
输出量
5
19.在Python中交换两个变量的独特方法是什么? (19. What is the unique way to swap two variables in Python?)
Python has unique way to swap two variables. The swapping can be done in one line of code. Let the values of the variables a and b be 5 and 6 respectively. The swapping program will be the following.
Python具有交换两个变量的独特方法。 交换可以在一行代码中完成。 令变量a和b的值分别为5和6。 交换程序如下。
a = 5b = 6a,b = b,aprint(a)print(b)
Output
输出量
65
20. Python中的迭代器是什么? (20. What are Iterators in Python?)
The objects in python that implements the iterator protocols are called iterators. iter
is the keyword used to create the iterable objects. It iterates throough various iterable objects such as List, dictionary.
python中实现迭代器协议的对象称为迭代器。 iter
是用于创建可迭代对象的关键字。 它遍历各种可迭代对象,例如List,Dictionary。
my_list = [1, 2, 3, 4, 5]my_iterator = iter(my_list)print(next(my_iterator))print(next(my_iterator))print(next(my_iterator))
Output
输出量
123
21. Python中的浅表复制和深表复制有什么区别? (21. What is the difference between shallow copy and deep copy in Python?)
In python, when we assign a value of a variable to another variable using assignment operator we are just creating a new variable associated with the old object. The copy
module in python contains two different type of copying method called copy
and deepcopy
.
在python中,当我们使用赋值运算符将一个变量的值分配给另一个变量时,我们只是在创建一个与旧对象关联的新变量。 python中的copy
模块包含两种不同类型的复制方法,分别称为copy
和deepcopy
。
Shallow Copy
浅拷贝
The shallow copy works same as the assignment operator. It just create a new variable that is associated with the old object.
浅表副本的工作方式与赋值运算符相同。 它只是创建一个与旧对象关联的新变量。
import copymy_list = [[1, 1, 1], [2, 2, 2], [3, 3, 3]]new_list = copy.copy(my_list)new_list[2][2] = 50print(my_list)print(new_list)
Output
输出量
[[1, 1, 1], [2, 2, 2], [3, 3, 50]][[1, 1, 1], [2, 2, 2], [3, 3, 50]]
Deep Copy
深拷贝
The deep copy method creates independent copy of an object. If we edit the element in a copied object, that does not affect the other object.
深层复制方法创建对象的独立副本。 如果我们在复制的对象中编辑元素,则不会影响其他对象。
import copymy_list = [[1, 1, 1], [2, 2, 2], [3, 3, 3]]new_list = copy.deepcopy(my_list)new_list[2][2] = 50print(my_list)print(new_list)
Output
输出量
[[1, 1, 1], [2, 2, 2], [3, 3, 3]][[1, 1, 1], [2, 2, 2], [3, 3, 50]]
22.如何在Python中生成随机数? (22. How to generate random numbers in Python?)
There is no in-bulit function in python to generate random numbers We can use random
module for this purpose. We can create random number from a range or list. randint
is the simple method used to create a random number between two numbers.
python中没有inbulit函数来生成随机数。为此,我们可以使用random
模块。 我们可以从范围或列表中创建随机数。 randint
是用于在两个数字之间创建随机数的简单方法。
import randomprint( random.randint(0,9))
Output
输出量
1
23.如何禁用字符串中的转义序列? (23. How to disable the escape sequences in a string?)
The escape sequences in a string can be disabled using regular expression in Python. The raw output of string can be printed using formatting. The following code will show you the process of printing raw string.
可以使用Python中的正则表达式禁用字符串中的转义序列。 字符串的原始输出可以使用格式打印。 以下代码将向您展示打印原始字符串的过程。
import reprint(r'Hi.\nThis is \t a String')
Output
输出量
Hi.\nThis is \t a String
24.什么是负索引? (24. What is negative indexing?)
Python allows us to access the string and list objects using negative indexes. The negative index of 0th index is -len(iterable). If a string contains five characters then the first character can be accessed with the help of 0 and -5. The last character of the string points to -1.
Python允许我们使用负索引访问字符串和列表对象。 第0个索引的负索引是-len(iterable)。 如果字符串包含五个字符,则可以使用0和-5访问第一个字符。 字符串的最后一个字符指向-1。
my_string = "Hello"print(my_string[-1])print(my_string[-5])
Output
输出量
oH
26.如何在Python中创建多行字符串? (26. How to create multi line string in Python?)
We can use the escape character \n to split the strings in the output console. But, to write a string in multiple lines inside the code we need to use triple quotes.
我们可以使用转义符\ n在输出控制台中分割字符串。 但是,要在代码内的多行中编写字符串,我们需要使用三引号。
my_string = """Hello.This is a multi line string"""print(my_string)
Output
输出量
Hello.This is a multi line string
27.如何在Python中创建私有数据? (27. How to create private data in Python?)
Not like other object oriented programming languages such as java an C++ , Python does not have any keywords to define public and protected data. Normally, the objects created in python can be accessed by public members.
与其他面向对象的编程语言(例如Java C ++)不同,Python没有任何关键字来定义公共数据和受保护数据。 通常,公共成员可以访问在python中创建的对象。
Protected members can be accessed only by the class and its sub classes. The following code examples shows that how the private and public members works in python.
受保护的成员只能由该类及其子类访问。 以下代码示例显示了私有成员和公共成员如何在python中工作。
Program with public member
与公众成员一起计划
class emp: def __init__(self, s): self.salary = st = emp(25000)t.salary = 30000print(t.salary)
Output
输出量
30000
Program with private member
私人会员计划
class emp: def __init__(self, s): self.__salary = st = emp(25000)print(t.__salary)
Output
输出量
Traceback (most recent call last): File "main.py", line 5, in <module> print(t.__salary)AttributeError: 'emp' object has no attribute '__salary'
28.什么是酸洗和酸洗? (28. What is pickling and unpickling?)
Pickle is a module in Python that converts a python object and converts the object into string representation and the string is further converted into a file using dump method.
Pickle是Python中的一个模块,可转换python对象并将对象转换为字符串表示形式,然后使用dump方法将字符串进一步转换为文件。
Unpickling is the exact opposite to the process of pickling. The file created is converted into the old version of python object. The following code contains both pickling and unpickling methods.
与酸洗过程完全相反。 创建的文件将转换为旧版本的python对象。 以下代码包含酸洗和解酸方法。
import picklemy_list = [1, 2, 3, 4, 5]with open("my_file.out", "wb") as f: pickle.dump(my_list,f)with open("my_file.out", "rb") as f: my_new_list = pickle.load(f)if(my_list == my_new_list): print("The objects are same")
Output
输出量
The objects are same
29. Python中的Frozenset是什么? (29. What is frozenset in Python?)
The immutable and hashable version of a normal set is called frozenset. It possess all the properties of normal sets in python. The updating the set is not possible in frozenset.
普通集的不可变和可哈希化版本称为Frozenset。 它具有python中常规集的所有属性。 在Frozenset中无法更新集。
30. Python提供了哪些函数式编程概念? (30. What are the functional programming concepts available in Python?)
Splitting a program into multiple parts is called functional programming. It is one of the widely used programming paradigm. The functional programming concepts that are available as in built features in Python are zip
, map
, filter
and reduce
.
将程序分为多个部分称为功能编程。 它是广泛使用的编程范例之一。 Python的内置功能中可用的功能编程概念是zip
, map
, filter
和reduce
。
Thank you all for reading this article. I hope it will help you to learn something.
谢谢大家阅读本文。 我希望它将帮助您学习一些东西。
翻译自: https://towardsdatascience.com/30-python-interview-questions-that-worth-reading-63b868e682e5
python 面试问题
http://www.taodudu.cc/news/show-997409.html
相关文章:
- 机器学习模型 非线性模型_机器学习:通过预测菲亚特500的价格来观察线性模型的工作原理...
- pytorch深度学习_深度学习和PyTorch的推荐系统实施
- 数据库课程设计结论_结论:
- 网页缩放与窗口缩放_功能缩放—不同的Scikit-Learn缩放器的效果:深入研究
- 未越狱设备提取数据_从三星设备中提取健康数据
- 分词消除歧义_角色标题消除歧义
- 在加利福尼亚州投资于新餐馆:一种数据驱动的方法
- 近似算法的近似率_选择最佳近似最近算法的数据科学家指南
- 在Python中使用Seaborn和WordCloud可视化YouTube视频
- 数据结构入门最佳书籍_最佳数据科学书籍
- 多重插补 均值插补_Feature Engineering Part-1均值/中位数插补。
- 客户行为模型 r语言建模_客户行为建模:汇总统计的问题
- 多维空间可视化_使用GeoPandas进行空间可视化
- 机器学习 来源框架_机器学习的秘密来源:策展
- 呼吁开放外网_服装数据集:呼吁采取行动
- 数据可视化分析票房数据报告_票房收入分析和可视化
- 先知模型 facebook_Facebook先知
- 项目案例:qq数据库管理_2小时元项目:项目管理您的数据科学学习
- 查询数据库中有多少个数据表_您的数据中有多少汁?
- 数据科学与大数据技术的案例_作为数据科学家解决问题的案例研究
- 商业数据科学
- 数据科学家数据分析师_站出来! 分析人员,数据科学家和其他所有人的领导和沟通技巧...
- 分析工作试用期收获_免费使用零编码技能探索数据分析
- 残疾科学家_数据科学与残疾:通过创新加强护理
- spss23出现数据消失_改善23亿人口健康数据的可视化
- COVID-19研究助理
- 缺失值和异常值的识别与处理_识别异常值-第一部分
- 梯度 cv2.sobel_TensorFlow 2.0中连续策略梯度的最小工作示例
- yolo人脸检测数据集_自定义数据集上的Yolo-V5对象检测
- 图深度学习-第2部分
python 面试问题_值得阅读的30个Python面试问题相关推荐
- python 优点 英文_值得关注的 10 个 Python 英文博客
1. 第一个博客是 Planet python , 它无疑是最出名的python博客之一.去看看吧.我希望你会发现它很有用.它今天还更新过. 2. 第二个博客是 lucumr . 一个 Flask 开 ...
- Python灰帽子_黑客与逆向工程师的Python编程之道
收藏自用 链接:Python灰帽子_黑客与逆向工程师的Python编程之道
- 值得收藏的30道Python练手题(附详解)
今天给大家分享30道Python练习题,建议大家先独立思考一下解题思路,再查看答案. 1. 已知一个字符串为 "hello_world_yoyo",如何得到一个队列 [" ...
- python后端开发面试简历_【Atman爱特曼Python后端工程师实习生面试】我是在boss直聘上投的简历,从简历筛选到拿到offer一共有如下步骤-看准网...
我是在boss直聘上投的简历,从简历筛选到拿到offer一共有如下步骤: 1.简历筛选:职位上有985/211优先,虽然我是双非,但我还是给HR发了消息.第二天HR就要了简历,然后等了4天简历过了(能 ...
- python zope 工作流_使用C语言来扩展Python程序和Zope服务器的教程
有几个原因使您可能想用 C 扩展 Zope.最可能的是您有一个已能帮您做些事的现成的 C 库,但是您对把它转换成 Python 却不感兴趣.此外,由于 Python 是解释性语言,所以任何被大量调用的 ...
- python清华教程_清华教授整理的全套Python 400集视频教程,速拿!
Python是世界上功能最多,功能最强大的编程语言之一.如果你一直想学Python,但是不知道如何入手,那就别犹豫了.这篇文章就是为你写的. 清华教授整理的Python全集视频教程,这就是你需要的 如 ...
- python图像计数_计算机视觉:利用OpenCV和Python进行车辆计数详细步骤
本教程我将分享几个简单步骤解释如何使用OpenCV进行Python对象计数. 需要安装一些软件: Python 3 OpennCV 1.了解Opencv从摄像头获得视频的Python脚本import ...
- python就业发展前景_如何参与蓬勃发展的Python就业市场
python就业发展前景 From finance to artificial intelligence, data science to web development, there isn't a ...
- 怎么学python知乎_你们都是怎么学 Python 的?
自学确实是比较难得,没有一个好的规划,好的学习路线图,你不会知道自己下一步该怎么办. 今天我就帮你来解决,分享2020年黑马程序员Python学习路线图,包含学习路线图,学习视频,学习工具,你都可以找 ...
最新文章
- python3 语法再学习
- CF Vicious Keyboard 构造水题
- compress()方法
- 测量软件应用系统的聚合复杂度【翻译稿】
- 世界33种名车标志及来历
- Netflix Media Database - 起源和数据模型
- [自动化] 如果电脑不会自己看网课,就应该用Python教会它
- 光通量发光强度照度亮度关系_什么是光通量、光强、亮度和照度?它们之间的关系是什么?...
- ETI工作-测试文件多层folder树生成
- python列表去掉逗号_python – Scrapy crawler,从字符串中删除逗号
- alm系统的使用流程_Polarion ALM—涵盖您所需的一切于整体统一的 ALM 解决方案之中...
- GraphPad Prism 列联表教程
- 使用条件分布模态流进行多变量概率时序预测
- python numpy读取数据_Python numpy数据的保存和读取
- HTML ===> 向右侧展开div
- ai推理_人工智能推理
- 微信小程序-公廉租房维保系统
- 几款虚拟打印机的奇妙用途
- tomcat 配置域名
- U盘 移动硬盘提示格式化 怎么办 属性为0字节了 文件格式变RAW了
热门文章
- idea出现找不到实体类
- Put-Me-Down项目Postmortem2
- iOS: TableView如何刷新指定的cell 或section
- 一个经典实例理解继承与多态原理与优点(附源码)---面向对象继承和多态性理解得不够深刻的同学请进...
- Elk7.2 Docker
- org.activiti.engine.ActivitiOptimisticLockingException updated by another transaction concurrently
- 初识Activiti
- yum配置中driver-class-name: com.mysql.jdbc.Driver报错
- telnet命令发送邮件
- html - meta name=viewport content=XX/ 标签常见属性及说明