vue nodejs 构建_如何使用nodejs后端打字稿版本开发和构建vue js应用
vue nodejs 构建
There are so many ways we can build Vue.js apps and ship for production. One way is to create the Vue app with NodeJS or Java, and another way is to develop and serve that static content with the NGINX web server. With NodeJS, we have to deal with the server code as well; for example, you need to load the index.html page with node.
我们可以使用多种方法来构建Vue.js应用并交付生产。 一种方法是使用NodeJS或Java创建Vue应用程序,另一种方法是使用NGINX Web服务器开发并提供静态内容。 使用NodeJS,我们还必须处理服务器代码。 例如,您需要使用节点加载index.html页面。
In this post, we will see the details and implementation with the NodeJS. We will go through step by step with an example.
在本文中,我们将看到NodeJS的详细信息和实现。 我们将逐步举例。
Introduction
介绍
Prerequisites
先决条件
Example Project
示例项目
Just Enough NodeJS For This Project
就足够这个项目的NodeJS
Development Phase
开发阶段
How To Build Project For Production
如何建立生产项目
How To Package Project For Production
如何打包生产项目
Summary
摘要
Conclusion
结论
介绍 (Introduction)
Vue.js is a Progressive JavaScript Framework for building web apps, and it doesn’t load itself in the browser. We need some mechanism that loads the index.html (single page) of Vue application with all the dependencies(CSS and js files) in the browser. In this case, we are using node as the webserver, which loads Vue assets and accepts any API calls from the Vue UI app.
Vue.js是用于构建Web应用程序的渐进式JavaScript框架,并且不会将自身加载到浏览器中。 我们需要某种机制来加载浏览器中所有依赖项(CSS和js文件)的Vue应用程序的index.html (单页)。 在这种情况下,我们使用节点作为Web服务器,该服务器加载Vue资产并接受来自Vue UI应用程序的所有API调用。
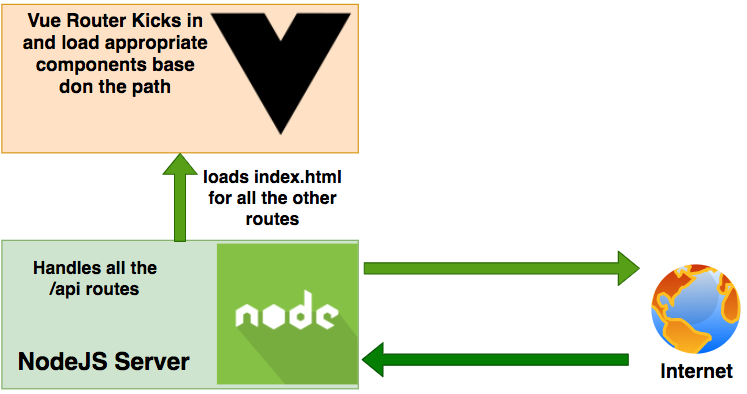
If you look at the above diagram, all the web requests without the /api will go to Vue routing, and the Vue Router kicks in and loads components based on the path. The Node server itself will handle all the paths that contain /api.
如果您查看上图,所有不带/ api的Web请求都将转到Vue路由,并且Vue路由器将启动并根据路径加载组件。 节点服务器本身将处理所有包含/ api的路径。
In this post, we are going to develop the Vue app with NodeJS (Written in Typescript) and see how to build for production.
在本文中,我们将使用NodeJS(用Typescript编写)开发Vue应用程序,并了解如何为生产而构建。
先决条件 (Prerequisites)
There are some prerequisites for this article. It would help if you had nodejs installed on your laptop and know-how Http works. If you want to practice and run this on your laptop, you need to have these on your machine.
本文有一些先决条件。 如果您的笔记本电脑上安装了nodejs并且知道Http的工作原理,这将有所帮助。 如果您想在笔记本电脑上练习并运行它,则需要在计算机上安装它们。
NodeJS
节点JS
Typescript
打字稿
Vue CLI
Vue CLI
VSCode
VSCode
BootstrapVue
BootstrapVue
示例项目 (Example Project)
This is a simple project which demonstrates developing and running Vue application with NodeJS. We have a simple app in which we can add users, count, and display them at the side, and retrieve them whenever you want.
这是一个简单的项目,演示了如何使用NodeJS开发和运行Vue应用程序。 我们有一个简单的应用程序,可以在其中添加用户,计数并在侧面显示它们,并在需要时检索它们。

As you add users, we are making an API call to the nodejs server to store them and get the same data from the server when we retrieve them. You can see network calls in the following video.
添加用户时,我们正在对nodejs服务器进行API调用以存储它们,并在检索用户时从服务器获取相同的数据。 您可以在以下视频中看到网络通话。
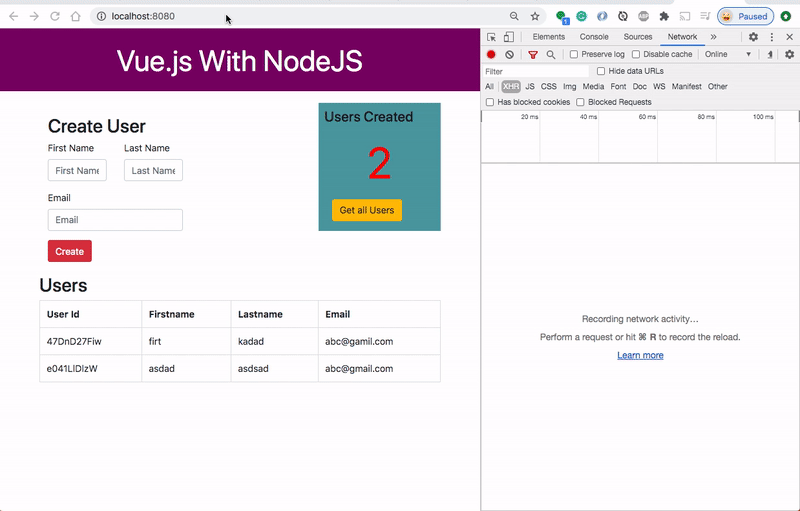
Here is a Github link to this project. You can clone it and run it on your machine.
这是该项目的Github链接。 您可以克隆它并在计算机上运行它。
// clone the projectgit clone https://github.com/bbachi/vuejs-nodejs-typescript-example.git//Vue Codecd my-appnpm installnpm run serve// API codecd apinpm installnpm run start:dev
就足够这个项目的NodeJS (Just Enough NodeJS For This Project)
If you are new to NodeJS, don’t worry. This short description is enough to get you started with this project. If you are already aware of NodeJS, you can skip this section.
如果您不熟悉NodeJS,请不用担心。 简短的描述足以使您开始该项目。 如果您已经知道NodeJS,则可以跳过本节。
NodeJS is an asynchronous event-driven javascript runtime environment for server-side applications. The current version of the nodejs is 12, and you can install it from this link here. You can click on any LTS link, and the NodeJS package is downloaded, and you can install it on your laptop.
NodeJS是用于服务器端应用程序的异步事件驱动的javascript运行时环境。 当前的nodejs版本是12,您可以在此链接中安装它。 您可以单击任何LTS链接,并下载NodeJS软件包,然后将其安装在笔记本电脑上。
You can check the version of the Node with this command node -v.
You can run javascript on the node REPL by typing the command node
on the CLI.
您可以使用此命令node -v.
检查Node的版本node -v.
您可以通过在CLI上键入命令node
在节点REPL上运行javascript。

The installation of a node is completed and you know how to run javascript. You can even run javascript files. For example, Let’s put the above commands in the sample.js file and run it with this command node sample.js.
节点的安装已完成,您知道如何运行javascript。 您甚至可以运行javascript文件。 例如,让我们将以上命令放入sample.js文件,并使用此命令node sample.js.
运行它node sample.js.
var x = 10;
var y = 20;
var z = x + y;
console.log(z);
开发阶段 (Development Phase)
Usually, the way you develop and the way you build and run in production are completely different. Thatswhy, I would like to define two phases: The development phase and the production phase.
通常,您的开发方式以及在生产中构建和运行的方式是完全不同的。 因此,我想定义两个阶段:开发阶段和生产阶段。
In the development phase, we run the nodejs server and the Vue app on completely different ports. It’s easier and faster to develop that way. If you look at the following diagram the Vue app is running on port 8080 with the help of a webpack dev server and the nodejs server is running on port 3080.
在开发阶段,我们在完全不同的端口上运行nodejs服务器和Vue应用程序。 这样开发起来更容易,更快捷。 如果您查看下图,则在Webpack开发服务器的帮助下Vue应用程序在端口8080上运行,而nodejs服务器在端口3080上运行。
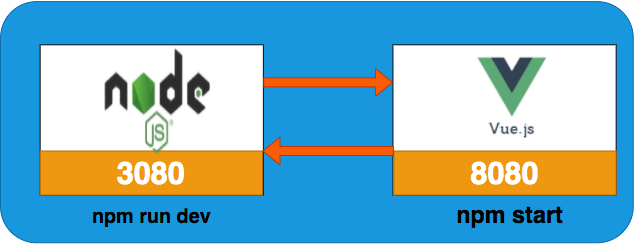
项目结构 (Project Structure)
Let’s understand the project structure for this project. We will have two package.json: one for the Vue and another for nodejs API. It’s always best practice to have completely different node_modules for each one. In this way, you won’t get merging issues or any other problems regarding web and server node modules collision.
让我们了解该项目的项目结构。 我们将有两个package.json:一个用于Vue ,另一个用于nodejs API 。 始终最好的做法是每个节点都有完全不同的node_modules 。 这样,您将不会遇到合并问题或有关Web和服务器节点模块冲突的任何其他问题。

If you look at the above project structure, all the Vue app resides under the my-app folder and nodejs API resides under the api folder.
如果您查看上述项目结构,则所有Vue应用程序都位于my-app文件夹下,而nodejs API则位于api文件夹下。
NodeJS API (NodeJS API)
We use the express and nodemon on the server-side. Express is the Fast, unopinionated, minimalist web framework for NodeJS and nodemon is the library which makes your API reloads automatically whenever there is a change in the files. Let’s install these two dependencies. nodemon is only used for development so install this as a dev dependency.
我们在服务器端使用express和nodemon。 Express是用于NodeJS的快速,不受限制的,极简的Web框架,而nodemon是一个库,该库可以使您的API在文件发生更改时自动重新加载。 让我们安装这两个依赖项。 nodemon仅用于开发,因此请将其安装为dev依赖项。
Since it is written in typescript you need some other dependencies as well. Let’s install all the dependencies.
由于它是用打字稿编写的,因此您还需要一些其他依赖项。 让我们安装所有依赖项。
npm install express --savenpm install @types/express nodemon ts-node typescript --save-devnpm install webpack webpack-cli --save-dev
Here is the package.json of nodejs API.
这是nodejs API的package.json 。
{"name": "api","version": "1.0.0","description": "","main": "index.js","scripts": {"start": "ts-node index.ts","start:dev": "./node_modules/nodemon/bin/nodemon.js","test": "echo \"Error: no test specified\" && exit 1","tslint": "tslint --project tsconfig.json --config tslint.json","tslint-fix": "tslint --project tsconfig.json --config tslint.json --fix","build": "webpack"},"keywords": [],"author": "Bhargav Bachina","license": "ISC","dependencies": {"express": "^4.17.1"},"devDependencies": {"@types/express": "^4.17.7","nodemon": "^2.0.4","ts-loader": "^8.0.2","ts-node": "^8.10.2","typescript": "^3.9.7","webpack": "^4.44.1","webpack-cli": "^3.3.12"}
}
We need to import express and define two routes: /api/users and /api/user and the server listening on the port 3080. Here are the app.ts, routes.ts, user.ts files. We use body-parser to handle data in the http request object.
我们需要导入express并定义两个路由: / api / users和/ api / user以及侦听端口3080的服务器。这是app.ts,routes.ts和user.ts文件。 我们使用body-parser处理http请求对象中的数据。
import * as bodyParser from "body-parser";
import * as express from "express";
import { Logger } from "./logger/logger";
import Routes from "./routes/routes";class App {public express: express.Application;public logger: Logger;// array to hold userspublic users: any[];constructor() {this.express = express();this.middleware();this.routes();this.users = [];this.logger = new Logger();}// Configure Express middleware.private middleware(): void {this.express.use(bodyParser.json());this.express.use(bodyParser.urlencoded({ extended: false }));}private routes(): void {this.express.get("/", (req, res, next) => {res.send("Typescript App works!!");});// user routethis.express.use("/api", Routes);// handle undefined routesthis.express.use("*", (req, res, next) => {res.send("Make sure url is correct!!!");});}
}export default new App().express;
We are using nodemon in the development environment. Here is the configuration nodemon.json file. Any file that ends with ts will be watched and all the node_modules are ignored.
我们在开发环境中使用nodemon。 这是配置nodemon.json文件。 任何以ts结尾的文件都会被监视,所有的node_modules都会被忽略。
{"ignore": [".git", "node_modules"],"watch": ["./"],"exec": "npm start","ext": "ts"
}
You need to start the nodejs API with this command npm run start:dev
and the moment you change any file, the server will be automatically restarted.
您需要使用此命令npm run start:dev
启动nodejs API,并且在更改任何文件时,服务器将自动重启。

Vue用户界面 (Vue UI)
Now the nodejs API is running on port 3080. Now it’s time to look at the Vue UI. The entire Vue app is under the folder my-app. You can create with this command vue create my-app
I am not going to put all the files here you can look at the entire files in the above Github link or here.
现在,nodejs API在端口3080上运行。现在是时候查看Vue UI了。 整个Vue应用程序位于my-app文件夹下。 您可以使用此命令vue create my-app
我不会将所有文件放在此处,您可以在上面的Github链接中或此处查看整个文件。
Let’s see some important files here. Here is the service file which calls node API. This is a service file with two async functions that use fetch API to make the API calls.
让我们在这里看到一些重要的文件。 这是调用节点API的服务文件。 这是一个具有两个异步函数的服务文件,这些函数使用访存API进行API调用。
export async function getAllUsers() {const response = await fetch('/api/users');return await response.json();
}export async function createUser(data) {const response = await fetch(`/api/user`, {method: 'POST',headers: {'Content-Type': 'application/json'},body: JSON.stringify({user: data})})return await response.json();
}
Here is the Dashboard component that calls this service and gets the data from the API. Once we get the data from the API we set the state accordingly and renders the appropriate components again to pass the data down the component tree. You can find other components here.
这是调用此服务并从API获取数据的仪表板组件。 从API获取数据后,我们将相应地设置状态,并再次渲染适当的组件以将数据传递到组件树。 您可以在此处找到其他组件。
<template><div class="hello"><Header /><div class="container mrgnbtm"><div class="row"><div class="col-md-8"><CreateUser @createUser="userCreate($event)" /></div><div class="col-md-4"><DisplayBoard :numberOfUsers="numberOfUsers" @getAllUsers="getAllUsers()" /></div></div></div><div class="row mrgnbtm"><Users v-if="users.length > 0" :users="users" /></div></div>
</template><script>
import Header from './Header.vue'
import CreateUser from './CreateUser.vue'
import DisplayBoard from './DisplayBoard.vue'
import Users from './Users.vue'
import { getAllUsers, createUser } from '../services/UserService'export default {name: 'Dashboard',components: {Header,CreateUser,DisplayBoard,Users},data() {return {users: [],numberOfUsers: 0}},methods: {getAllUsers() {getAllUsers().then(response => {console.log(response)this.users = responsethis.numberOfUsers = this.users.length})},userCreate(data) {console.log('data:::', data)createUser(data).then(response => {console.log(response);this.getAllUsers();});}},mounted () {this.getAllUsers();}
}
</script>
Vue UI和Node API之间的交互 (Interaction between Vue UI and Node API)
In the development phase, the Vue app is running on port 8080 with the help of a Vue CLI and nodejs API running on port 3080.
在开发阶段,借助于在端口3080上运行的Vue CLI和nodejs API,Vue应用程序将在端口8080上运行。
There should be some interaction between these two. We can proxy all the API calls to nodejs API. Vue CLI provides some inbuilt functionality and to tell the development server to proxy any unknown requests to your API server in development, we just need to add this file at the root where package.json resides and configures the appropriate API paths.
两者之间应该有一些互动。 我们可以将所有API调用代理到nodejs API。 Vue CLI提供了一些内置功能,并告诉开发服务器将任何未知请求代理到开发中的API服务器,我们只需要将此文件添加到package.json所在的根目录并配置适当的API路径即可。
module.exports = {devServer: {proxy: {'^/api': {target: 'http://localhost:3080',changeOrigin: true},}}}
With this in place, all the calls start with /api will be redirected to http://localhost:3080 where the nodejs API running.
有了这个,所有以/ api开头的调用将被重定向到http:// localhost:3080 运行nodejs API的位置。
Once this is configured, you can run the Vue app on port 8080 and nodejs API on 3080 still make them work together.
配置完成后,您可以在端口8080上运行Vue应用程序,而在3080上的nodejs API仍然可以使它们协同工作。
// nodejs API (Terminal 1)cd api (change it to API directory)npm run dev// Vue app (Terminal 2)cd my-app (change it to app directory)npm run serve
如何建立生产项目 (How To Build Project For Production)
The Vue app runs on the port 8080 with the help of a Vue CLI. This is not the case for running in production. We have to build the Vue project and load those static assets with the node server. Let’s see those step by step here.
Vue应用程序借助Vue CLI在端口8080上运行。 在生产中运行不是这种情况。 我们必须构建Vue项目,并将这些静态资产加载到节点服务器。 让我们逐步了解这些内容。
First, we need to build the Vue project with this command npm run build
or vue-cli-service build
and all the built assets will be put under the dist folder.
首先,我们需要使用此命令npm run build
或vue-cli-service build
来构建Vue项目,所有构建的资产都将放在dist文件夹下。
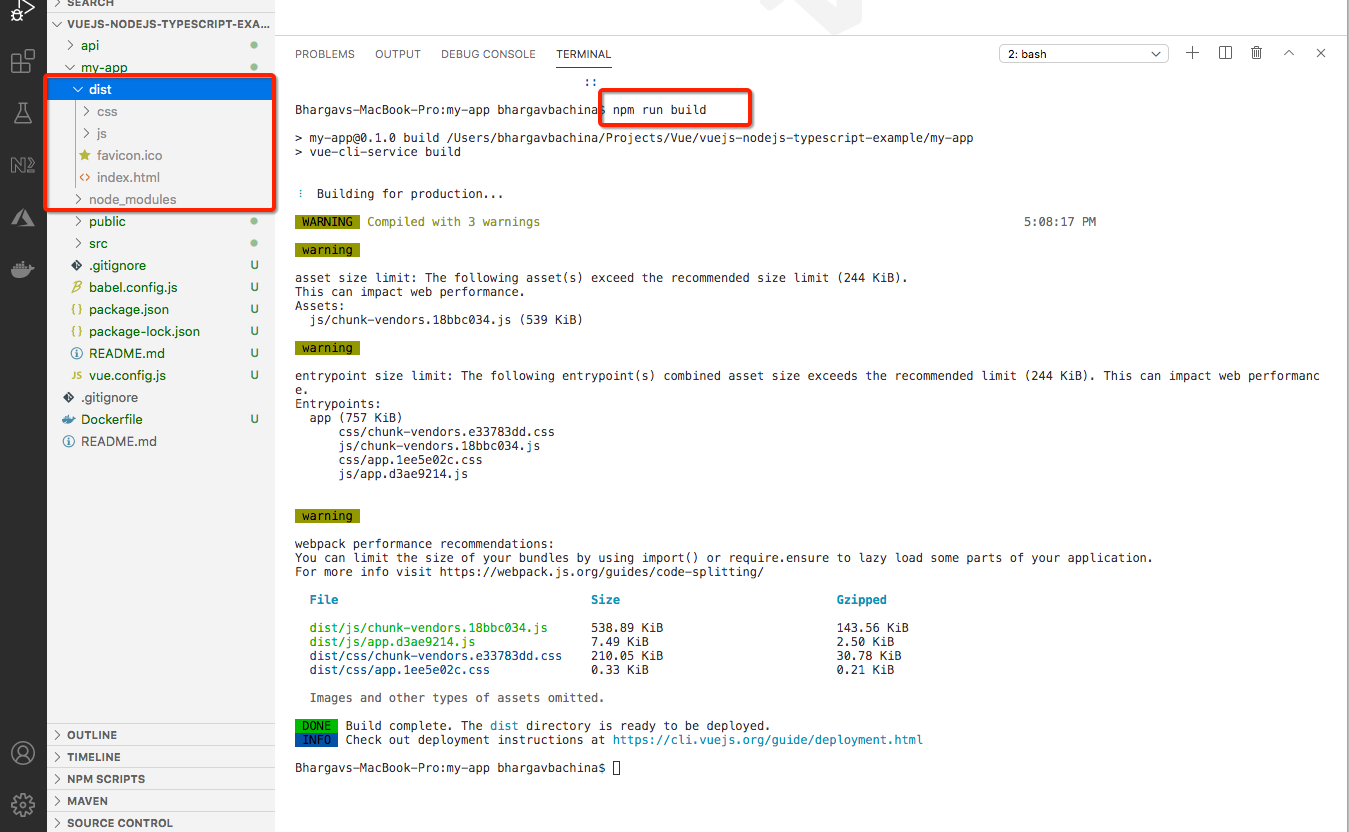
Second, we need to make some changes on the server-side. Here is the modified app.ts file.
其次,我们需要在服务器端进行一些更改。 这是修改后的app.ts文件。
We have to use express.static at line number 27 to let express know there are a dist folder and assets of the Angular build.
我们必须在第27行使用express.static来使express知道有dist文件夹和Angular构建的资产。
Load index.html for the default route / at line number 33
在第33行加载index.html作为默认路由/
import * as bodyParser from "body-parser";
import * as express from "express";
import { Logger } from "./logger/logger";
import Routes from "./routes/routes";
const path = require('path');class App {public express: express.Application;public logger: Logger;// array to hold userspublic users: any[];constructor() {this.express = express();this.middleware();this.routes();this.users = [];this.logger = new Logger();}// Configure Express middleware.private middleware(): void {this.express.use(bodyParser.json());this.express.use(bodyParser.urlencoded({ extended: false }));this.express.use(express.static(path.join(__dirname, "../my-app/dist/")));}private routes(): void {this.express.get("/", (req, res, next) => {res.sendFile(path.join(__dirname, "../my-app/dist/index.html"));});// user routethis.express.use("/api", Routes);// handle undefined routesthis.express.use("*", (req, res, next) => {res.send("Make sure url is correct!");});}
}export default new App().express;
Once you are done with the above changes, you can actually run the whole app with the nodejs server running on port 3080 like below as nodejs acts as a web server as well.
完成上述更改后,您实际上可以使用运行在端口3080上的nodejs服务器运行整个应用程序,如下所示,因为nodejs也可以充当Web服务器。

如何打包生产项目 (How To Package Project For Production)
Packaging this application for production needs other tools such as webpack, gulp. We need a webpack to transpile the NodeJS API into javascript and bundle it in a single server file.
打包此应用程序以进行生产需要其他工具,例如webpack,gulp。 我们需要一个webpack将NodeJS API转换为javascript并将其捆绑在一个服务器文件中。
We use the gulp tool to copy all the built files and put them in the appropriate folders and finally make them a deployable artifact or zip file.
我们使用gulp工具复制所有构建的文件,并将它们放置在适当的文件夹中,最后使它们成为可部署的工件或zip文件。
摘要 (Summary)
- There are so many ways we can build Vue apps and ship for production.我们可以通过多种方式来构建Vue应用并交付生产。
- One way is to build Vue with NodeJS.一种方法是使用NodeJS构建Vue。
- We have seen how to build the NodeJS API in typescript version我们已经看到了如何在打字稿版本中构建NodeJS API
In the development phase, we can run Vue and Nodejs on separate ports. Vue on 8080 and NodeJS server on port 3080.
在开发阶段,我们可以在单独的端口上运行Vue和Nodejs。 8080上的Vue和端口3080上的NodeJS服务器。
- The interaction between these two happens with proxying all the calls to API.这两者之间的交互是通过代理所有对API的调用而发生的。
- In the production phase, you can build the Vue app and put all the assets in the dist folder and load it with the node server.在生产阶段,您可以构建Vue应用并将所有资产放入dist文件夹中,然后将其与节点服务器一起加载。
- Nodejs act as a web server as well in the above case. You need to let express know where are all the Vue assets are.在上述情况下,Node.js也充当Web服务器。 您需要让express知道所有Vue资产在哪里。
- We can package the application in a number of ways.我们可以通过多种方式打包应用程序。
- You can deploy the packaged zip folder in any node environment.您可以在任何节点环境中部署打包的zip文件夹。
结论 (Conclusion)
This is one way of building and shipping Vue apps. This is really useful when you want to do server-side rendering or you need to do some processing. NodeJS is non-blocking IO and it is very good for normal websites as well. In future posts, I will discuss more on deploying strategies for this kind of architecture.
这是构建和交付Vue应用程序的一种方法。 当您要进行服务器端渲染或需要进行一些处理时,这确实很有用。 NodeJS是非阻塞IO,对于普通网站也非常有用。 在以后的文章中,我将讨论有关这种架构的部署策略的更多信息。
翻译自: https://medium.com/bb-tutorials-and-thoughts/how-to-develop-and-build-vue-js-app-with-nodejs-backend-typescript-version-2eeb0f10e87f
vue nodejs 构建
http://www.taodudu.cc/news/show-3263474.html
相关文章:
- AC自动机及KMP练习
- 计算机英语口语练习,计算机英语会话(MP3+中英字幕) 第22期:办公自动化(1)
- PAT练习笔记——4.2 散列
- 手动输入计算机在线,2001计算机英文录入反复练习
- 电脑打字前面的那条线叫什么_我29岁那年就学会了打字。这值得吗?
- 使用c#制作打字游戏_使用打字稿iii绘制网格构建游戏4 5
- VScode 主题和打字特效配置,让你的VScode活“”起来
- 打字软件带倒计时_使用VueJS创建打字速度效果-第2部分:计时器和计分板
- 什么软件可以听力打字测试,雅思听力1 - 在线打字测试(dazi.kukuw.com)
- 机器学习 伪标签_伪英语—机器学习打字练习
- Mysql 8.0修改密码
- openstack修改密码
- sourceTree 更改密码
- 使用saltstack批量修改密码
- linux root密码修改失败,【转】Linux root修改密码失败
- linux修改密码策略
- 让word文档中的代码更美观
- word中如何美观插入代码?
- c#窗体美观原则
- 如何做出美观高大上的前端页面
- 一个简单而又美观的 beamer 模板制作
- PyQt如何使界面按钮更加美观
- 写出一个美观的表单页
- qt如何设计界面更美观_8个更好的界面设计的黄金法则
- 如何让你的网页看起来更美观
- 在word中如何美观地插入代码
- 【oh-my-zsh】打造强大又美观的linux终端
- 巧用Vscode编辑器,快速格式化代码,让你的代码变得整洁又美观
- 使用Qt绘制一个简约美观的界面 【使用QSS简单美化】(笔记)
- 怎么写出美观,可读性高的代码?
vue nodejs 构建_如何使用nodejs后端打字稿版本开发和构建vue js应用相关推荐
- angular 模块构建_如何通过11个简单的步骤从头开始构建Angular 8应用
angular 模块构建 Angular is one of the three most popular frameworks for front-end development, alongsid ...
- nodejs入门_如何在NodeJS中使用套接字创建专业的Chat API解决方案[入门级]
nodejs入门 Have you ever wondered how chat applications work behind the scenes? Well, today I am going ...
- Vue 合同模板_【开源】后端开发也很容易上手的前端框架模板
作为一个后端开发,说实话每次看到vue,react等等框架都头大.看着很漂亮,但是我这三脚猫的前端水平真是玩不转啊. 今天发现了一个bootstrap的前端模板框架.对我来说友好多了.和Thymele ...
- 使用c#制作打字游戏_使用打字稿iii绘制网格构建游戏4 5
使用c#制作打字游戏 Chapter III in the series of tutorials on how to build a game from scratch with TypeScrip ...
- vue 背景透明度_一款媒体小白喜爱的视频编辑软件,vue视频编辑APP,想学就来...
大家好,我今天利用这半个多小时的时间里,我们共同学习下一款手机剪辑软件,名字叫 VUE Vlog视频剪辑软件. 在学习之前,我想首先感谢让我们相遇在这个互联网时代,再要感谢这个平台 ...
- ejb构建_如何使用单例EJB,Ehcache和MBean构建和清除参考数据缓存
ejb构建 在本文中,我将介绍如何使用单例EJB和Ehcache在Java EE中构建简单的参考数据缓存. 高速缓存将在给定的时间段后重置自身,并且可以通过调用REST端点或MBean方法" ...
- vue ui框架_「webAPP」记录几款比较好用的vue 移动端的ui框架
有时在做项目时,不同场景的项目既要有网站,又要有手机端,为了快速开发,如果功能简单,要求不高的话,我们一般会用H5进行移动端的适配. 如果采用纯html进行书写手机端的样式,往往UI的体验感非常差.为 ...
- vue导入音乐_【vlog制作】不经电脑,如何在VUE中导入自定义音乐
VUE按不同风格.节气/节日.每月精选和流行曲集设置了多个音乐库,用户在剪辑视频的时候可以在库中挑选BGM,省掉了找音乐的麻烦,对于新手用户尤为贴心.然而对于频繁使用VUE剪辑视频的用户来说,原配音乐 ...
- web自动化构建_通过在真实设备上进行自动测试来构建更好的Web
web自动化构建 This article was originally published on Medium. 本文最初发表在Medium上 . My work is entirely dedic ...
最新文章
- centos7快速搭建LAMP
- ubuntu中安装ffmpeg+mencoder转换flv -
- cesium 隐藏entity_cesium entity创建各类实体
- PHP 多维数组搜索 PHP multi dimensional array search
- [leetcode]Palindrome Number @ Python
- Tosca:键盘输入字符串
- dependency报错
- 宜家开发中心东亚区完成在中国的全新升级;牙科巨头卡瓦集团上海创新卓越中心正式启动 | 美通企业日报...
- 7z文件格式及其源码的分析(六)-完结篇
- win10纯净版安装教程
- 跨平台应用开发进阶(十一) :uni-app 实现IOS原生APP-云打包集成极光推送(JG-JPUSH)详细教程
- vscode的c_cpp_properties.json
- 生产者-消费者-管程法(java代码示例)
- 8.遍历二叉树、线索二叉树、森林
- 高级宏观经济学公式整理
- 肖特基二极管检波电路设计与分析
- 计算机病毒主动传播途径,蠕虫病毒的传播方式是什么
- 罗生门:一个简单查询实现引发的思考
- donet 微服务开发 学习-Docker环境搭建 win7 docker 环境配置
- macos\Linux下使用fcrackzip破解zip压缩文件密码