vimeo下载_通过Vimeo的API喜欢,关注列表和上传
vimeo下载
In a previous post, we used the Vimeo API to build a rudimentary video application with Silex and Twig. We added login and user feed functionality and wrapped it all up with a video searching feature. In this one, we’ll add in liking a video, adding a video to a watchlist for later, and uploading videos via the Vimeo API.
在上一篇文章中,我们使用Vimeo API与Silex和Twig一起构建了基本的视频应用程序。 我们添加了登录和用户供稿功能,并通过视频搜索功能将其打包。 在这一部分中,我们将添加喜欢的视频,将视频添加到监视列表中以供以后使用,并通过Vimeo API上传视频。

先决条件 (Prerequisites)
To get up to speed, please skim through the code presented in the previous part and get it to run in your development environment (preferably Homestead Improved). Alternatively, just download the final code of the previous post here. Once you’re ready, continue with the content below.
为了加快速度,请略读上一部分中提供的代码,并使其在您的开发环境中运行(最好是Homestead Improvementd )。 或者,只需在此处下载上一篇文章的最终代码。 准备就绪后,请继续以下内容。
与视频互动 (Interacting with Videos)
In this section, you’re going to add the capability to like and add videos to be watched later. But before that, you first need to update the scopes for the Vimeo login (in the previous post, we only asked for public
and private
scope permissions). Add interact
as one of the items, and once that’s done, access the login page in the browser and try logging in again.
在本节中,您将添加喜欢的功能,并添加以后观看的视频。 但在此之前,您首先需要更新Vimeo登录的范围(在上一篇文章中,我们仅要求提供public
和private
范围权限)。 将interact
添加为其中一项,完成后,在浏览器中访问登录页面,然后尝试再次登录。
$scopes = array('public', 'private', 'interact');
$state = substr(str_shuffle(md5(time())), 0, 10);
$_SESSION['state'] = $state;
$url = $vimeo->buildAuthorizationEndpoint(REDIRECT_URI, $scopes, $state);
$page_data = array(
'url' => $url
);
It should now show an additional item under permissions. Check that to allow video interactions.
现在,它应该在权限下显示一个附加项目。 选中以允许视频互动。
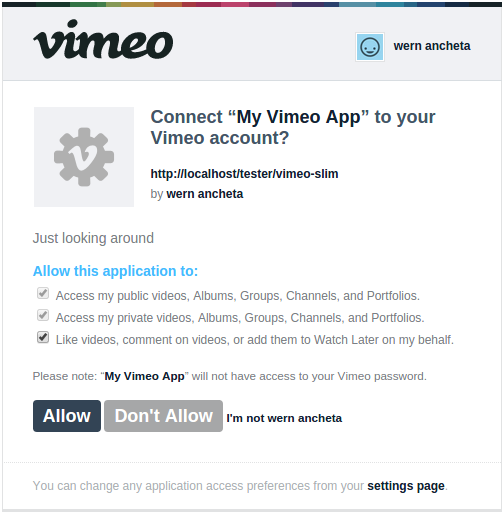
Open the videos.php
file in the templates
directory and add the buttons that the user will use for interacting with the video. Each button has the data-uri
attribute which stores the uri
of the video. You extract this value later on with JavaScript and pass it to the server. You can add this right below the video description.
打开templates
目录中的videos.php
文件,并添加用户将用于与视频进行交互的按钮。 每个按钮都有data-uri
属性,该属性存储视频的uri
。 您稍后可以使用JavaScript提取此值,并将其传递到服务器。 您可以在视频说明下方添加此内容。
<div>
<button class="like" data-uri="{{ row.uri }}">like</button>
<button class="watch-later" data-uri="{{ row.uri }}">watch later</button>
</div>
At the bottom of the page, add jQuery and the script where you will add the code for making an AJAX request to the server.
在页面底部,添加jQuery和脚本,您将在其中添加用于向服务器发出AJAX请求的代码。
<script src="http://cdnjs.cloudflare.com/ajax/libs/jquery/2.1.3/jquery.min.js"></script>
<script src="assets/js/videos.js"></script>
Create the videos.js
file in the assets/js
directory and add the following code.
在assets/js
目录中创建videos.js
文件,并添加以下代码。
$('.like').click(function(){
var self = $(this);
var uri = self.data('uri');
$.post(
'/tester/vimeo-slim/video/like',
{
'uri': uri
},
function(response){
if(response.status == '204'){
self.prop('disabled', true);
}
}
);
});
$('.watch-later').click(function(){
var self = $(this);
var uri = self.data('uri');
$.post(
'/tester/vimeo-slim/video/watchlater',
{
'uri': uri
},
function(response){
if(response.status == '204'){
self.prop('disabled', true);
}
}
);
});
What this does is extract the value of the data-uri
attribute and then makes an AJAX request to the server with the value. If the response is 204, this means that the server has fulfilled the request but there’s no response body. You can disable the button as a form of feedback to the user that the operation has been fulfilled.
这样做是提取data-uri
属性的值,然后使用该值向服务器发出AJAX请求。 如果响应为204,则表示服务器已满足请求,但没有响应正文。 您可以禁用该按钮,作为对用户已完成操作的一种反馈形式。
In the index.php
file, add the routes that would handle the AJAX requests. Each of these routes are similar, only the API endpoint is different. First, it checks if the uri
is passed along in the request and extracts the video ID by replacing the /videos
string with an empty one.
在index.php
文件中,添加将处理AJAX请求的路由。 这些路由均相似,仅API端点不同。 首先,它检查uri
是否在请求中传递,并通过将/videos
字符串替换为空字符串来提取视频ID。
Then, set the access token and make the request to the /me/likes/{video_id}
endpoint. You also need to pass in an empty array for the data since the request method expects an array of parameters as its second argument. Unlike in the previous example, the PUT
method is explicitly specified since the request uses POST
by default and the API requires the request to be a PUT
request. Lastly, set the content type of the response to json
and then return the status of the response. This would return ‘204’ if the request was successful.
然后,设置访问令牌并向/me/likes/{video_id}
端点发出请求。 您还需要为数据传递一个空数组,因为request方法期望将参数数组作为第二个参数。 与前面的示例不同,由于请求默认情况下使用POST
,并且API要求该请求为PUT
请求,因此显式指定了PUT
方法。 最后,将响应的内容类型设置为json
,然后返回响应的状态。 如果请求成功,它将返回“ 204”。
$app->post('/video/like', function () use ($app, $vimeo) {
if($app->request->post('uri')){
$video_id = str_replace('/videos/', '', $app->request->post('uri'));
$vimeo->setToken($_SESSION['user.access_token']);
$response = $vimeo->request('/me/likes/' . $video_id, array(), 'PUT');
$app->contentType('application/json');
echo '{"status": ' . json_encode($response['status']) . '}';
}
});
The route for marking the video to be watched later is similar, only this time you have to use /me/watchlater/{video_id}
as the endpoint.
标记稍后观看的视频的方法是相似的,只是这次您必须使用/me/watchlater/{video_id}
作为端点。
$app->post('/video/watchlater', function () use ($app, $vimeo) {
if($app->request->post('uri')){
$video_id = str_replace('/videos/', '', $app->request->post('uri'));
$vimeo->setToken($_SESSION['user.access_token']);
$response = $vimeo->request('/me/watchlater/' . $video_id, array(), 'PUT');
$app->contentType('application/json');
echo '{"status": ' . json_encode($response['status']) . '}';
}
});
上载影片 (Uploading Videos)
Uploading videos can also be done through the API, though you have to make a request to Vimeo for this functionality to be unlocked. If you’re planning to deploy an app which allows users to upload videos, you can request upload access from your app page.
上传视频也可以通过API完成,尽管您必须请求Vimeo才能解锁此功能。 如果您打算部署一个允许用户上传视频的应用程序,则可以从您的应用程序页面请求上传权限。
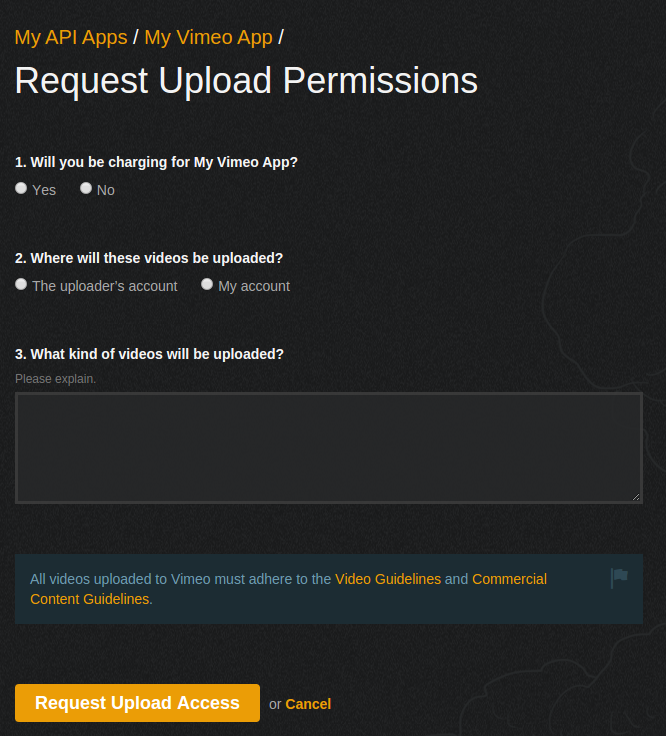
It could take up to 5 business days to receive the decision on your request. Vimeo provides a way for generating access tokens with specific scopes on it, and one of those is the upload scope. Unfortunately, even if an access token with the upload scope is generated, the upload wouldn’t work if the upload functionality hasn’t been approved by Vimeo.
您最多可能需要5个工作日才能收到您的决定。 Vimeo提供了一种生成具有特定作用域的访问令牌的方法,其中之一就是上传作用域。 不幸的是,即使生成了具有上载范围的访问令牌,如果上载功能未得到Vimeo的批准,上载也不起作用。

Here’s the code for rendering the upload view.
这是用于呈现上传视图的代码。
$app->get('/upload', function () use ($app, $vimeo) {
$page_data = array();
$app->render('upload.php', $page_data);
});
And here’s the upload view (templates/upload.php
). What it’s doing is checking if there are any errors in the flash session. If so, they’re shown to the user. Below that, create a form that allows the user to upload a video.
这是上传视图( templates/upload.php
)。 它正在做的是检查Flash会话中是否有任何错误。 如果是这样,它们会显示给用户。 在其下,创建一个允许用户上传视频的表单。
{% if flash.errors %}
<div>
error uploading file: {{ flash.errors[0] }}
</div>
{% endif %}
<form method="POST" enctype="multipart/form-data">
<input type="file" name="video" value=""/>
<button type="submit">upload</button>
</form>
Before moving on, install the Upload library for handling the video uploads.
在继续之前,请安装用于处理视频上载的上载库 。
composer require codeguy/upload
For the route that’s responsible for handling the uploads, set the access token.
对于负责处理上载的路由,设置访问令牌。
$app->post('/upload', function () use ($app, $vimeo) {
$vimeo->setToken($_SESSION['user.token']);
});
Next, set the upload directory. Be sure to create the directory and change the file permissions if necessary so that the upload can work correctly. Also set the input field which holds the file selected by the user.
接下来,设置上传目录。 确保创建目录并在必要时更改文件许可权,以便上载可以正常工作。 还要设置用于保存用户选择的文件的输入字段。
$storage = new \Upload\Storage\FileSystem('uploads');
$file = new \Upload\File('video', $storage);
Rename the file so you won’t have issues referring to it in the code.
重命名该文件,这样在代码中引用它就不会有问题。
$new_filename = uniqid();
$file->setName($new_filename);
Add the validation rules so that only mp4
files with a size not greater than 25Mb can be uploaded. You then wrap the code for uploading the file inside a try catch block. If an error occurs, flash the error into session. This is the value that’s being accessed earlier in the upload.php
view.
添加验证规则,以便只能上传大小不超过25Mb的mp4
文件。 然后,您将用于上传文件的代码包装在try catch块中。 如果发生错误,请将错误刷新到会话中。 这是先前在upload.php
视图中访问的值。
$file->addValidations(array(
new \Upload\Validation\Mimetype('video/mp4'),
new \Upload\Validation\Size('25M')
));
try {
$file->upload();
} catch (\Exception $e) {
$errors = $file->getErrors();
$app->flash('errors', $errors);
}
Finally, construct the path to the uploaded file and try to upload it to Vimeo. You do that by calling the upload
method on the Vimeo library. This accepts the path to the file as its argument. If an error occurs while uploading the file, flash it to the session.
最后,构建上传文件的路径,然后尝试将其上传到Vimeo。 您可以通过调用Vimeo库上的upload
方法来做到这一点。 这接受文件的路径作为其参数。 如果上传文件时发生错误,请将其刷新到会话中。
$new_filepath = 'uploads/' . $new_filename . '.' . $file->getExtension();
try {
$vimeo->upload($new_filepath);
} catch (\Exception $e) {
$app->flash('errors', array('error uploading to Vimeo'));
}
$app->redirect('upload');
结论 (Conclusion)
In this article, you’ve learned how to use the Vimeo API to perform different operations on a user’s Vimeo account. You’ve learned how give different permission to the app, interact with the videos, and then finally upload videos. All the code used in this article is available in this Github repo.
在本文中,您学习了如何使用Vimeo API对用户的Vimeo帐户执行不同的操作。 您已经了解了如何为应用赋予不同的权限,如何与视频进行交互,然后最终上传视频。 Github存储库中提供了本文中使用的所有代码。
Questions? Comments? Leave them below!
有什么问题吗 注释? 把它们留在下面!
翻译自: https://www.sitepoint.com/liking-watchlisting-and-uploading-through-vimeos-api/
vimeo下载
vimeo下载_通过Vimeo的API喜欢,关注列表和上传相关推荐
- 下载vimeo视频_使用Vimeo的API和Slim构建基本的视频搜索应用
下载vimeo视频 In this tutorial, you'll get to know the basics of the Vimeo API. With it, you can fetch i ...
- java文件客户端下载_使用Java写一个minio的客户端上传下载文件
标签:color ati tty java system wired format media param 前言: 确保已经安装了minio的服务端 代码: pom.x ...
- java流式上传下载_精讲RestTemplate第6篇-文件上传下载与大文件流式下载
C++Templates(第2版英文版) 123.24元 (需用券) 去购买 > 本文是精讲RestTemplate第6篇,前篇的blog访问地址如下: 精讲RestTemplate第1篇-在S ...
- live http工具下载_使用通用测试工具探索Blueworks Live REST API资源
live http工具下载 本系列的第1部分介绍了5个一般用例,它们是使用IBM®Blueworks Live的代表性状态转移(REST)应用程序编程接口(API)的最佳方法. 您还为每种用例学习了不 ...
- spotify音乐下载_使用Python和R对音乐进行聚类以在Spotify上创建播放列表。
spotify音乐下载 Spotify is one of the most famous Music Platforms to discover new music. The company use ...
- 匕首线切割图纸下载_干净匕首
匕首线切割图纸下载 重点 (Top highlight) A pragmatic guide to dependency injection on Android 在Android上进行依赖注入的实用 ...
- 魔兽怀旧网站模块下载_一个人的网站重新设计和怀旧
魔兽怀旧网站模块下载 Despite how I look, I'm the kind kind of person that loves to play old video games. (Full ...
- illustrator下载_平面设计:16个Illustrator快捷方式可加快工作流程
illustrator下载 I know, I know - keyboard shortcuts sound so nerdy, and you're a graphic designer, not ...
- figma下载_素描vs Figma困境
figma下载 I distinctly remember how much hatred I had in my heart when I lived through my first UI upd ...
最新文章
- [小明爬坑系列]AssetBundle原理介绍
- python装饰器教学_Python装饰器学习(九步入门)
- Excel多条件求和 SUMPRODUCT函数用法详解
- apache camel_Apache Camel请向我解释这些端点选项的含义
- dz去掉/forum.php_discuz如何去除url的forum.php
- 解决Fast api打印两次日志的问题
- android代码跳过锁屏,Android_android禁止锁屏保持常亮(示例代码),在播放video的时候在mediaplayer
m - phpStudy...
- 02205微型计算机原理与接口技术自考,2012年微型计算机原理与接口技术自考题模拟(2)...
- 编程基本功:工作中,高低境界如何迁就?
- 数学之美————每章小结
- 点击图标分享页面到QQ,微信,微博 等
- AI 入行那些事儿(13)人工智能的三类技术岗位
- 台式计算机的主流配置,现在台式电脑主流配置是什么配置?
- 多层板交期怎么才能有效把控?
- Java常见面试题 + 答案
- 余三码和8421码对比
- NI Vision:二值图像连通域标记算法
- 什么是有理数和无理数?
- Stlink固件更新问题“ST-Link is not in the dfu mode Please restart it“的解决方法
- qq气泡php接口,h5实现QQ聊天气泡的实例介绍