SQL OUTER JOIN概述和示例
This article will provide a full overview, with examples of the SQL Outer join, including the full, right and left outer join as well as cover the union between SQL left and right outer joins.
本文将提供完整的概述,并提供SQL外连接示例,包括完整,右侧和左侧外部连接,以及SQL左侧和右侧外部连接之间的联合。
It is essential to understand the process to get the data from the multiple tables. A beginner might not have the idea of Joins in SQL Server. In this tip, we will take an overview of the SQL joins, learn SQL OUTER JOIN along with its syntax, examples, and use cases.
必须了解从多个表中获取数据的过程。 初学者可能没有在SQL Server中加入连接的想法。 在本文中,我们将概述SQL连接,学习SQL OUTER JOIN及其语法,示例和用例。
In a relational database system, it is best practice to follow the principles of Normalization, in which, basically, we split large tables into the smaller tables. In a select statement, we can retrieve the data from these tables using joins. We can join the tables and get the required fields from these tables in the result set. These tables should have some common field to relate with each other. You might find data split across multiple databases and sometimes it is a very complex structure as well. With Joins, we can join the data together from the multiple tables, databases into a user-friendly way and represent this data in the application.
在关系数据库系统中,最佳实践是遵循规范化原则,在该原则中,我们基本上将大表拆分为较小的表。 在select语句中,我们可以使用联接从这些表中检索数据。 我们可以联接表并从结果集中的这些表中获取必填字段。 这些表应具有一些相互关联的公共字段。 您可能会发现数据分散在多个数据库中,有时它也是一个非常复杂的结构。 使用Joins,我们可以将来自多个表,数据库的数据连接在一起,以一种用户友好的方式进行,并在应用程序中表示该数据。
We can represent a SQL JOIN using the following image
我们可以使用下图表示一个SQL JOIN

We can many SQL Join types in SQL Server. In the following image, you can see SQL Joins categories
我们可以在SQL Server中使用许多SQL Join类型。 在下图中,您可以看到SQL Joins类别

Let’s explore SQL Outer Join in details in the upcoming section.
让我们在接下来的部分中详细探讨SQL外连接。
SQL OUTER JOIN概述 (Overview of the SQL OUTER JOIN)
We use the SQL OUTER JOIN to match rows between tables. We might want to get match rows along with unmatched rows as well from one or both of the tables. We have the following three types of SQL OUTER JOINS.
我们使用SQL OUTER JOIN来匹配表之间的行。 我们可能想从一个或两个表中获取匹配行以及不匹配行。 我们有以下三种类型SQL OUTER JOINS。
- SQL Full Outer Join SQL Full外连接
- SQL Left Outer Join SQL左外部联接
- SQL Right Outer Join SQL右外部联接
Let’s explore each of SQL Outer Join with examples.
让我们通过示例探索每个SQL Outer Join。
SQL Full外连接 (SQL Full Outer Join)
In SQL Full Outer Join, all rows from both the tables are included. If there are any unmatched rows, it shows NULL values for them.
在SQL Full Outer Join中,两个表中的所有行都包括在内。 如果有任何不匹配的行,则显示它们的NULL值。
We can understand efficiently using examples. Let’s create a sample table and insert data into it.
我们可以使用示例来有效地理解。 让我们创建一个示例表并将数据插入其中。
CREATE TABLE [dbo].[Employee]([EmpID] [int] IDENTITY(1,1) PRIMARY KEY CLUSTERED,[EmpName] [varchar](50) NULL,[City] [varchar](30) NULL,[Designation] [varchar](30) NULL]
)CREATE TABLE Departments
(EmpID INT PRIMARY KEY CLUSTERED , DepartmentID INT, DepartmentName VARCHAR(50)
);
You can refer the following data model of both the tables.
您可以参考以下两个表的数据模型。

Insert data into the Employee table with the following script.
使用以下脚本将数据插入Employee表。

USE [SQLShackDemo];
GO
SET IDENTITY_INSERT [dbo].[Employee] ON;
GO
INSERT INTO [dbo].[Employee]
([EmpID], [EmpName], [City], [Designation]
)
VALUES
(1, N'Charlotte Robinson', N'Chicago', N'Consultant'
);
GO
INSERT INTO [dbo].[Employee]
([EmpID], [EmpName], [City], [Designation]
)
VALUES
(2, N'Madison Phillips', N'Dallas', N'Senior Analyst'
);
GO
INSERT INTO [dbo].[Employee]
([EmpID], [EmpName], [City], [Designation]
)
VALUES
(3, N'Emma Hernandez', N'Phoenix', N'Senior Analyst'
);
GO
INSERT INTO [dbo].[Employee]
([EmpID], [EmpName], [City], [Designation]
)
VALUES
(4, N'Samantha Sanchez', N'San Diego', N'Principal Conultant'
);
GO
INSERT INTO [dbo].[Employee]
([EmpID], [EmpName], [City], [Designation]
)
VALUES
(5, N'Sadie Ward', N'San Antonio', N'Consultant'
);
GO
INSERT INTO [dbo].[Employee]
([EmpID], [EmpName], [City], [Designation]
)
VALUES
(6, N'Savannah Perez', N'New York', N'Principal Conultant'
);
GO
INSERT INTO [dbo].[Employee]
([EmpID], [EmpName], [City], [Designation]
)
VALUES
(7, N'Victoria Gray', N'Los Angeles', N'Assistant'
);
GO
INSERT INTO [dbo].[Employee]
([EmpID], [EmpName], [City], [Designation]
)
VALUES
(8, N'Alyssa Lewis', N'Houston', N'Consultant'
);
GO
INSERT INTO [dbo].[Employee]
([EmpID], [EmpName], [City], [Designation]
)
VALUES
(9, N'Anna Lee', N'San Jose', N'Principal Conultant'
);
GO
INSERT INTO [dbo].[Employee]
([EmpID], [EmpName], [City], [Designation]
)
VALUES
(10, N'Riley Hall', N'Philadelphia', N'Senior Analyst'
);
GO
SET IDENTITY_INSERT [dbo].[Employee] OFF;
GO
Insert Data into the Departments table
将数据插入“部门”表

USE [SQLShackDemo];
GO
INSERT INTO [dbo].[Departments]
([EmpID], [Department_ID], [DepartmentName]
)
VALUES
(1, 0, N'Executive'
);
GO
INSERT INTO [dbo].[Departments]
([EmpID], [Department_ID], [DepartmentName]
)
VALUES
(2, 1, N'Document Control'
);
GO
INSERT INTO [dbo].[Departments]
([EmpID], [Department_ID], [DepartmentName]
)
VALUES
(3, 2, N'Finance'
);
GO
INSERT INTO [dbo].[Departments]
([EmpID], [Department_ID], [DepartmentName]
)
VALUES
(4, 3, N'Engineering'
);
GO
INSERT INTO [dbo].[Departments]
([EmpID], [Department_ID], [DepartmentName]
)
VALUES
(5, 4, N'Facilities and Maintenance'
);
GO
INSERT INTO [dbo].[Departments]
([EmpID], [Department_ID], [DepartmentName]
)
VALUES
(6, 2, N'Finance'
);
GO
INSERT INTO [dbo].[Departments]
([EmpID], [Department_ID], [DepartmentName]
)
VALUES
(10, 4, N'Facilities and Maintenance'
);
GO
We can represent a logical relationship between two tables using a Venn diagram. In a Venn diagram contains multiple overlapping circles and each circle represents an entity or table. The common area or overlapping area in Venn diagram represents the common values between both tables.
我们可以使用维恩图表示两个表之间的逻辑关系。 在维恩图中,包含多个重叠的圆圈,每个圆圈代表一个实体或表格。 Venn图中的公共区域或重叠区域表示两个表之间的公共值。
For example, in the following screenshot, we have two overlapping circles. Each circle resent a table (Employee and Departments). Let’s understand the FULL Outer Join using the following example.
例如,在以下屏幕截图中,我们有两个重叠的圆圈。 每个圈子都寄出一张表格(员工和部门)。 让我们使用以下示例了解“完全外部联接”。
We have a common field ( EmpID) in both the tables; therefore, we can join the table with this column. In the following query, we defined the FULL OUTER JOIN between departments and Employee table on the EMPID column of both the table.
两个表中都有一个公共字段(EmpID)。 因此,我们可以将此表与该列连接。 在以下查询中,我们在两个表的EMPID列上的部门和Employee表之间定义了FULL OUTER JOIN。
SELECT *
FROM EmployeeFULL OUTER JOIN Departments ON Employee.EmpID = Departments.EmpID;

SQL Full Outer Join gives following rows in an output
SQL Full Outer Join在输出中给出以下行
- Matching Rows between both the tables 两个表之间的匹配行
- Unmatched Rows from both the tables (NULL values) 两个表中不匹配的行(NULL值)
Let’s execute this query to return Full Outer Join query output. We get the following output.
让我们执行此查询以返回“完全外部联接”查询输出。 我们得到以下输出。

We can see a few records with NULL values as well. Let’s understand this in a better way using a Venn diagram.
我们还可以看到一些带有NULL值的记录。 让我们使用维恩图更好地理解这一点。
In the following screenshot, you can see the following information
在以下屏幕截图中,您可以看到以下信息
- EmpID 1, 2,3,4,5,6,10 exists in both Employee and Departments table. In Full Outer Join query output, we get all these rows with data from both the tables 雇员表和部门表中都存在EmpID 1、2、3、4、5、6、10。 在“完全外部联接”查询输出中,我们从两个表中获取了所有这些行以及数据
- EmpID 7, 8, 9 exists in the Employee table but not in the Departments table. It does not include any matching rows in the departments table; therefore; we get NULL values for those records EmpID 7、8、9存在于Employee表中,但不存在于Departments表中。 它在Departments表中不包含任何匹配的行; 因此; 我们获得这些记录的NULL值
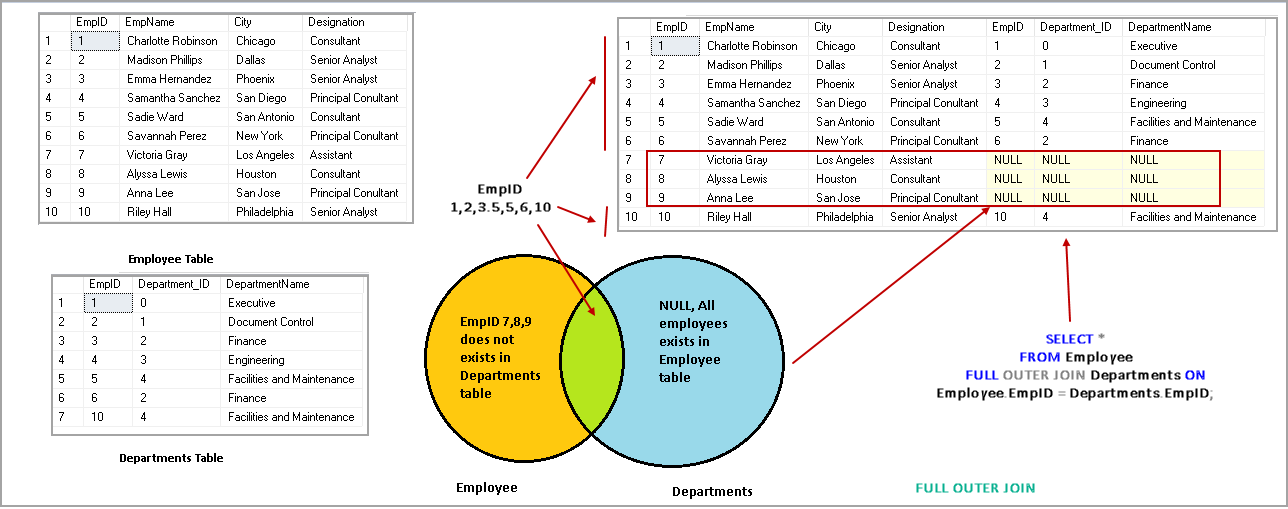
Now, for demo purpose let’s insert one more record in Departments tables. In this query, we insert EmpID 11 that does not exist in the Employee table.
现在,出于演示目的,让我们在Departments表中再插入一条记录。 在此查询中,我们插入Employee表中不存在的EmpID 11。
INSERT INTO [dbo].[Departments]
([EmpID], [Department_ID], [DepartmentName]
)
VALUES
(11, 4, N'Facilities and Maintenance'
);
GO
Rerun the SQL Full Outer Join query. In the following image, you get one additional row with NULL values. We do not have any matching row for EmpID 11 in the employee table. Due to this, we get NULL values for it in the output.
重新运行SQL Full Outer Join查询。 在下图中,您将获得另外一行具有NULL值的行。 在employee表中没有与EmpID 11相匹配的行。 因此,我们在输出中获得了NULL值。

As a summary, we can represent the SQL Full Outer Join using the following Venn diagram. We get what is represented in the highlighted area in the output of Full Outer Join.
概括来说,我们可以使用以下维恩图表示SQL Full Outer Join。 我们得到“完全外部联接”输出中突出显示的区域中表示的内容。
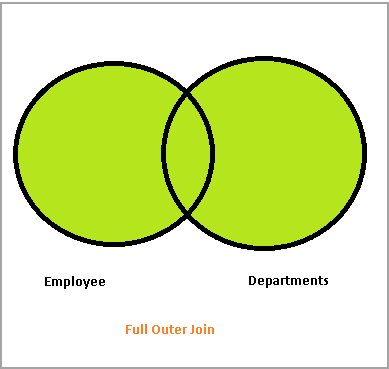
SQL FULL OUTER JOIN和WHERE子句 (SQL FULL OUTER JOIN and WHERE clause )
We can add a WHERE clause with a SQL FULL OUTER JOIN to get rows with no matching data between the both Join tables.
我们可以添加带有SQL FULL OUTER JOIN的WHERE子句,以获取两个Join表之间没有匹配数据的行。
In the following query, we add a where clause to show only records having NULL values.
在以下查询中,我们添加where子句以仅显示具有NULL值的记录。
SELECT *
FROM EmployeeFULL OUTER JOIN Departments ON Employee.EmpID = Departments.EmpID
WHERE Employee.EmpID IS NULLOR Departments.EmpID IS NULL;
Execute this command and view the output. It only returns rows that do not match either in Employee or Departments table.
执行此命令并查看输出。 它仅返回在Employee或Departments表中不匹配的行。

SQL左外连接 (SQL LEFT OUTER JOIN)
In a SQL Left Outer Join, we get following rows in our output.
在SQL左外部联接中,我们在输出中得到以下行。
- It gives the output of the matching row between both the tables 它给出两个表之间匹配行的输出
- If no records match from the left table, it also shows those records with NULL values 如果左表中没有记录匹配,则还会显示那些具有NULL值的记录
Execute the following code to return SQL LEFT OUTER JOIN output
执行以下代码以返回SQL LEFT OUTER JOIN输出
SELECT *
FROM EmployeeLEFT OUTER JOIN Departments ON Employee.EmpID = Departments.EmpID
In the following image, you can see we have NULL values for EmpID 7,8 and 9. These EmpID does not exist in the right side Department table.
在下图中,您可以看到EmpID 7,8和9具有NULL值。这些EmpID在右侧Department表中不存在。
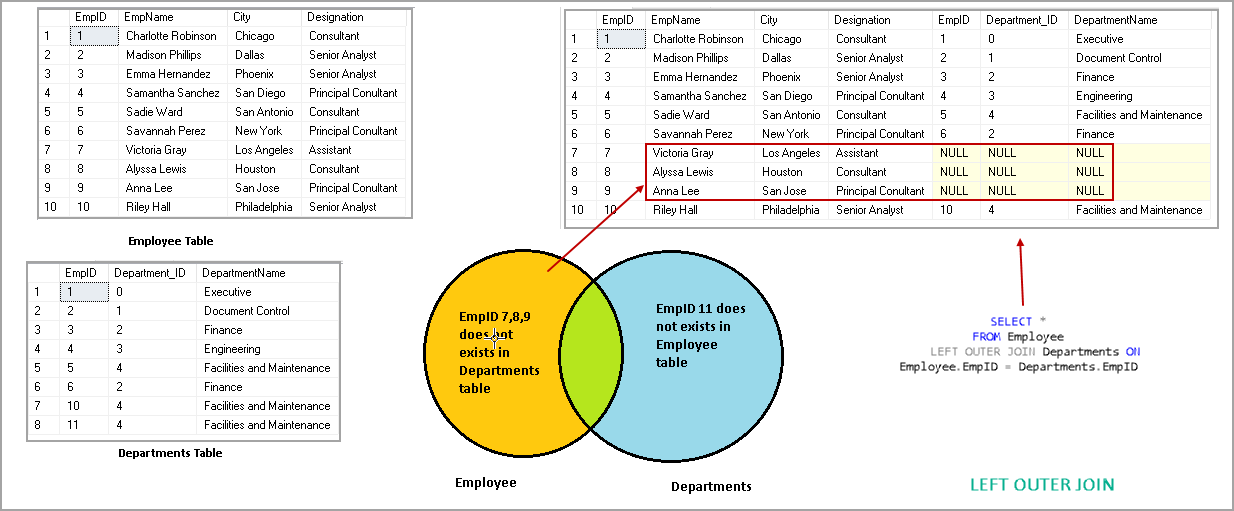
We need to note the table placement position in the Join statement. Currently, we have an Employee table on the left side and Departments table in Right side.
我们需要注意Join语句中表的放置位置。 当前,我们在左侧有一个Employee表,在右侧有Departments表。
Let’s rewrite query and swap the position of tables in query. In this query, we have the Department table in left position, so the Left Outer Join should check the values for this table and return a NULL value in case of a mismatch.
让我们重写查询并交换表在查询中的位置。 在此查询中,我们的Department表位于左侧,因此Left Outer Join应该检查该表的值,并在不匹配的情况下返回NULL值。
In the following screenshot, you can see that only one NULL value for EmpID 11. It is because EmpID 11 is not available in the Employee table.
在下面的屏幕快照中,您可以看到EmpID 11只有一个NULL值。这是因为EmpID 11在Employee表中不可用。

As a summary, we can represent SQL Left Outer Join using the following Venn diagram. We get the highlighted area in the output of SQL Left Outer Join.
作为总结,我们可以使用下面的维恩图表示SQL Left Outer Join。 我们在SQL Left Outer Join的输出中获得了突出显示的区域。

SQL Right外连接 (SQL Right OUTER JOIN)
In SQL Right Outer Join, we get the following rows in our output.
在SQL Right Outer Join中,我们在输出中获得以下行。
- It gives the output of the matching row between both the tables 它给出两个表之间匹配行的输出
- If no records match from the right table, it also shows those records with NULL values 如果右表中没有记录匹配,则还显示那些具有NULL值的记录
Execute the following query to get the output of Right Outer Join
执行以下查询以获取Right Outer Join的输出
SELECT *
FROM EmployeeRIGHT OUTER JOIN Departments ON Employee.EmpID = Departments.EmpID
In the following image, you can see we get all matching rows along with one row with NULL values. Null value row has EmpID 11 because it does not exist in the Employee table. You can also notice the position of the Department table is in the right position in Join. Due to this, we do not get values from the Employee table (left position) which does not match with Department table (Right side).
在下图中,您可以看到我们得到了所有匹配的行以及带有NULL值的一行。 空值行的EmpID为11,因为它在Employee表中不存在。 您还可以注意到“部门”表的位置在“联接”中的正确位置。 因此,我们无法从Employee表(左侧)获得的值与Department表(右侧)不匹配。
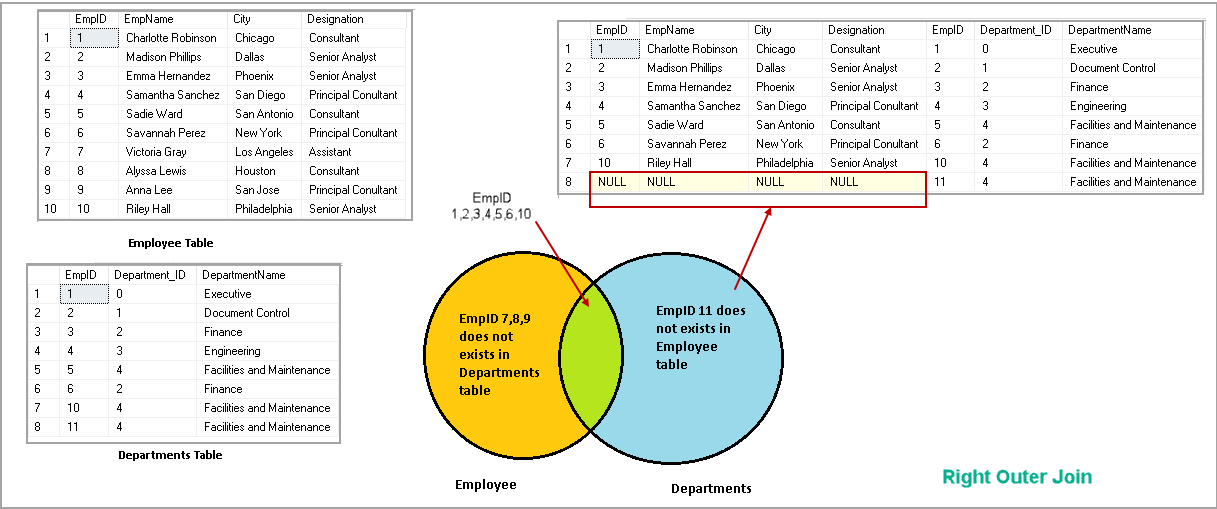
As highlighted earlier, the table position is important in the JOIN statement. If we change the table positions, we get different output. In the following query, we have Departments table (Left) and Employee table (Right).
如前所述,表位置在JOIN语句中很重要。 如果更改表的位置,则会得到不同的输出。 在以下查询中,我们具有Departments表(左)和Employee表(右)。
SELECT *
FROM DepartmentsRIGHT OUTER JOIN Employee ON Departments.EmpID = Employee.EmpID
You can notice the difference in Right Outer Join after swapping tables positions in above query.
在上述查询中交换表位置后,您会注意到在右外部联接中的差异。

As a summary, we can represent the SQL Right Outer Join using the following Venn diagram. We get highlighted area in the output of SQL Right Outer Join.
作为总结,我们可以使用下面的维恩图表示SQL Right Outer Join。 我们在SQL Right Outer Join的输出中突出显示了区域。

SQL左外部联接和SQL右外部联接之间的联合 (The union between SQL Left Outer Join and SQL Right Outer Join)
In the previous examples, we explored the SQL Left Outer Join, and the SQL Right Outer Join with different examples. We can do a Union of the result of both SQL Left Outer Join and SQL Right Outer Join. It gives the output of SQL Full Outer Join.
在前面的示例中,我们使用不同的示例探讨了SQL Left Outer Join和SQL Right Outer Join。 我们可以对SQL Left Outer Join和SQL Right Outer Join的结果进行并集。 它给出了SQL Full Outer Join的输出。
Execute the following query as an alternative to SQL Full Outer Join.
执行以下查询,以替代SQL Full Outer Join。
SELECT *
FROM EmployeeLEFT OUTER JOIN Departments ON Employee.EmpID = Departments.EmpIDUNION ALLSELECT *
FROM EmployeeRIGHT OUTER JOIN Departments ON Employee.EmpID = Departments.EmpID
In the following output, we get all matching records, unmatch records from the left table and unmatch records from the right table. It is similar to an output of SQL Full Outer Join.
在以下输出中,我们获得所有匹配的记录,左表中的不匹配记录和右表中的不匹配记录。 它类似于SQL Full Outer Join的输出。
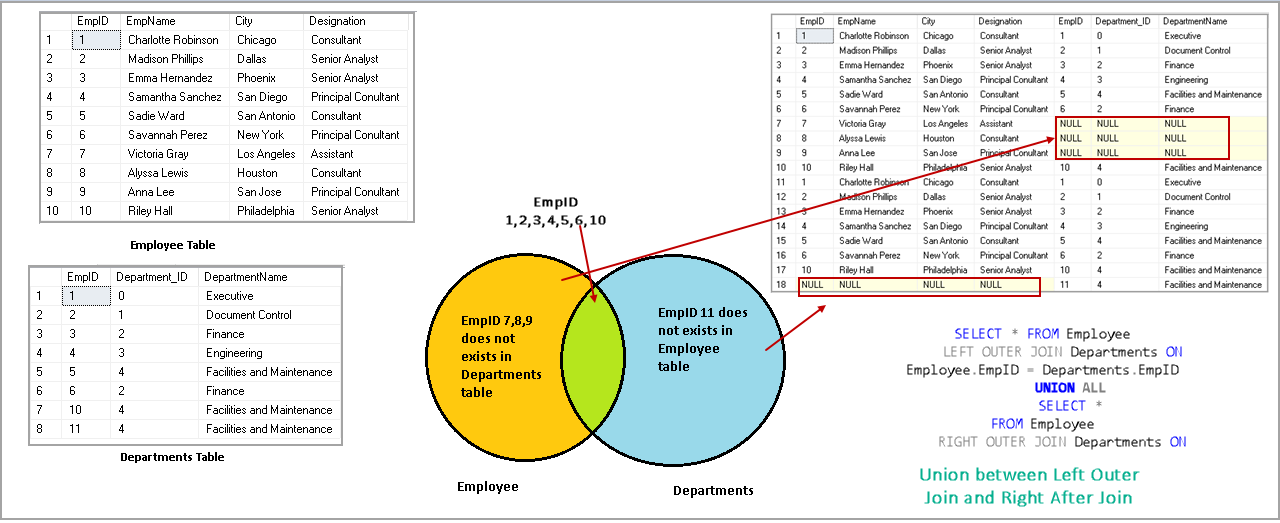
结论 (Conclusion)
In this article, we explored the SQL Outer Join and its types along with examples. I hope you found this article helpful. Feel free to provide feedback in the comments below.
在本文中,我们探讨了SQL外连接及其类型以及示例。 希望本文对您有所帮助。 请随时在下面的评论中提供反馈。
翻译自: https://www.sqlshack.com/sql-outer-join-overview-and-examples/
SQL OUTER JOIN概述和示例相关推荐
- oracle sql outer join,解答Oracle LEFT JOIN和LEFT OUTER JOIN的区别
问:我一直在对不同种类的Oracle连接做研究,目前还搞不清楚LEFT JOIN和LEFT OUTER JOIN之间有什么区别.Outer这个词是可选的么?因为Oracle中的LEFT JOIN是默认 ...
- sql中join类型_SQL Join类型概述和教程
sql中join类型 This article will provide an overview of the SQL Join and cover all of the SQL join types ...
- 如何在 Excel 里将两部分数据进行横向合并(即 Outer Join)?
不通过编程,实现 Excel 数据的 Outer Join 合并.总体思路是先从两块数据中找出具备相同内容的列作为 Key 列,然后把两块数据的 Key 列内容纵向串接在一起,找出重复值并删除相应的行 ...
- Spark SQL JOIN操作代码示例
title: Spark SQL JOIN操作 date: 2021-05-08 15:53:21 tags: Spark 本文主要介绍 Spark SQL 的多表连接,需要预先准备测试数据.分别创建 ...
- 干货 | SQL 外部联接 Outer Join
外部联接(Outer Join)是所有 SQL 联接类型中最不为人知的.也许是因为与其他联接类型相比,外部联接的需求较少.无论如何,外部联接本身并没有什么奇特的.正如我们将在这篇文章中看到的几个外部联 ...
- SQL学习之full outer join关键字
目录 参考源 SQL full outer join 关键字 图例 full outer join语法 示例数据 full outer join使用 参考源 简单教程 https://www.twle ...
- SQL FULL OUTER JOIN
FULL OUTER JOIN关键字只要左表(table1)和右表(table2)其中一个表中存在匹配,则返回行. FULL OUTER JOIN关键字结合了LEFT JOIN和RIGHT JOIN的 ...
- 零基础自学SQL课程 | OUTER JOIN外连接
大家好,我是宁一. 今天讲解SQL教程第12课:OUTER JOIN外连接. 外连接是左外连接(LEFT OUTER JOIN).右外连接(RIGHT OUTER JOIN).全外连接(FULL OU ...
- sql sum条件求和_SQL进阶7外连接(outer join)
本文中介绍的SQL中行列转换和嵌套式侧栏的生成,将SQL语句查询的结果转换成我们想要的结果. 行转列,制作交叉表头 列转行 实现行列转换(行->列):制作交叉表 需求 根据下面的表Courses ...
最新文章
- mysql 多个if_mysql if else 多条件
- 表格数据清空还能恢复吗_数据恢复大师:清空回收站文件如何恢复?
- RDD:基于内存的集群计算容错抽象
- 工具--Eclipse/MarkDown/XMind文章分类目录
- 为你的集成需求选择合适的ESB
- 数理统计-5.1 总体与样本
- JAX-RS和JSON-P集成
- hive udaf_Hive UDAF 函数的编写
- ssm指的是什么_什么是RESTful?RESTfule风格又是啥?
- android集成华为push 6003错误,以及华为低版本crash问题
- Photoshop-选区的应用
- winform直接控制云台_比 2 代便宜的灵眸手机云台 3,竟然还多了 15 条新亮点!...
- 双曲线matlab函数拟合,matlab怎么拟合双曲线
- 2017国产品牌台式计算机,2017三大热门国产平板电脑推荐
- 1000个苹果要分到10个箱子中去 两种分析方式
- Icons8 Pichon Crack,超链接或快捷方式的功能
- 通过Webkit远程调试协议监听网页崩溃
- 基于层次化LSTM的篇章级别情感分析方法
- c语言编程因子和,用c语言求一个数的所有因子
- VO、DTO、DO、PO的概念、区别和用处