python飞机大战类_Python版飞机大战
前面学了java用java写了飞机大战这次学完python基础后写了个python版的飞机大战,有兴趣的可以看下。
父类是飞行物类是所有对象的父类,setting里面是需要加载的图片,你可以换称自己的喜欢的图片,敌机可以分为敌机和奖励,enemy为普通敌人的父类,award为奖励敌机的父类。
各个类的基本属性
主类的大概逻辑
具体的代码:
settings配置
import pygame
class Settings(object):
"""设置常用的属性"""
def __init__(self):
self.bgImage = pygame.image.load('img/background.png') # 背景图
self.bgImageWidth = self.bgImage.get_rect()[2] # 背景图宽
self.bgImageHeight = self.bgImage.get_rect()[3] # 背景图高
self.start=pygame.image.load("img/start.png")
self.pause=pygame.image.load("img/pause.png")
self.gameover=pygame.image.load("img/gameover.png")
self.heroImages = ["img/hero.gif",
"img/hero1.png", "img/hero2.png"] # 英雄机图片
self.airImage = pygame.image.load("img/enemy0.png") # airplane的图片
self.beeImage = pygame.image.load("img/bee.png") # bee的图片
self.heroBullet=pygame.image.load("img/bullet.png")# 英雄机的子弹
飞行物类
import abc
class FlyingObject(object):
"""飞行物类,基类"""
def __init__(self, screen, x, y, image):
self.screen = screen
self.x = x
self.y = y
self.width = image.get_rect()[2]
self.height = image.get_rect()[3]
self.image = image
@abc.abstractmethod
def outOfBounds(self):
"""检查是否越界"""
pass
@abc.abstractmethod
def step(self):
"""飞行物移动一步"""
pass
def shootBy(self, bullet):
"""检查当前飞行物是否被子弹bullet(x,y)击中"""
x1 = self.x
x2 = self.x + self.width
y1 = self.y
y2 = self.y + self.height
x = bullet.x
y = bullet.y
return x > x1 and x < x2 and y > y1 and y < y2
def blitme(self):
"""打印飞行物"""
self.screen.blit(self.image, (self.x, self.y))
英雄机
from flyingObject import FlyingObject
from bullet import Bullet
import pygame
class Hero(FlyingObject):
"""英雄机"""
index = 2 # 标志位
def __init__(self, screen, images):
# self.screen = screen
self.images = images # 英雄级图片数组,为Surface实例
image = pygame.image.load(images[0])
x = screen.get_rect().centerx
y = screen.get_rect().bottom
super(Hero,self).__init__(screen,x,y,image)
self.life = 3 # 生命值为3
self.doubleFire = 0 # 初始火力值为0
def isDoubleFire(self):
"""获取双倍火力"""
return self.doubleFire
def setDoubleFire(self):
"""设置双倍火力"""
self.doubleFire = 40
def addDoubleFire(self):
"""增加火力值"""
self.doubleFire += 100
def clearDoubleFire(self):
"""清空火力值"""
self.doubleFire=0
def addLife(self):
"""增命"""
self.life += 1
def sublife(self):
"""减命"""
self.life-=1
def getLife(self):
"""获取生命值"""
return self.life
def reLife(self):
self.life=3
self.clearDoubleFire()
def outOfBounds(self):
return False
def step(self):
"""动态显示飞机"""
if(len(self.images) > 0):
Hero.index += 1
Hero.index %= len(self.images)
self.image = pygame.image.load(self.images[int(Hero.index)]) # 切换图片
def move(self, x, y):
self.x = x - self.width / 2
self.y = y - self.height / 2
def shoot(self,image):
"""英雄机射击"""
xStep=int(self.width/4-5)
yStep=20
if self.doubleFire>=100:
heroBullet=[Bullet(self.screen,image,self.x+1*xStep,self.y-yStep),Bullet(self.screen,image,self.x+2*xStep,self.y-yStep),Bullet(self.screen,image,self.x+3*xStep,self.y-yStep)]
self.doubleFire-=3
return heroBullet
elif self.doubleFire<100 and self.doubleFire > 0:
heroBullet=[Bullet(self.screen,image,self.x+1*xStep,self.y-yStep),Bullet(self.screen,image,self.x+3*xStep,self.y-yStep)]
self.doubleFire-=2
return heroBullet
else:
heroBullet=[Bullet(self.screen,image,self.x+2*xStep,self.y-yStep)]
return heroBullet
def hit(self,other):
"""英雄机和其他飞机"""
x1=other.x-self.width/2
x2=other.x+self.width/2+other.width
y1=other.y-self.height/2
y2=other.y+self.height/2+other.height
x=self.x+self.width/2
y=self.y+self.height
return x>x1 and xy1 and y
enemys
import abc
class Enemy(object):
"""敌人,敌人有分数"""
@abc.abstractmethod
def getScore(self):
"""获得分数"""
pass
award
import abc
class Award(object):
"""奖励"""
DOUBLE_FIRE = 0
LIFE = 1
@abc.abstractmethod
def getType(self):
"""获得奖励类型"""
pass
if __name__ == '__main__':
print(Award.DOUBLE_FIRE)
airplane
import random
from flyingObject import FlyingObject
from enemy import Enemy
class Airplane(FlyingObject, Enemy):
"""普通敌机"""
def __init__(self, screen, image):
x = random.randint(0, screen.get_rect()[2] - 50)
y = -40
super(Airplane, self).__init__(screen, x, y, image)
def getScore(self):
"""获得的分数"""
return 5
def outOfBounds(self):
"""是否越界"""
return self.y < 715
def step(self):
"""移动"""
self.y += 3 # 移动步数
Bee
import random
from flyingObject import FlyingObject
from award import Award
class Bee(FlyingObject, Award):
def __init__(self, screen, image):
x = random.randint(0, screen.get_rect()[2] - 60)
y = -50
super(Bee, self).__init__(screen, x, y, image)
self.awardType = random.randint(0, 1)
self.index = True
def outOfBounds(self):
"""是否越界"""
return self.y < 715
def step(self):
"""移动"""
if self.x + self.width > 480:
self.index = False
if self.index == True:
self.x += 3
else:
self.x -= 3
self.y += 3 # 移动步数
def getType(self):
return self.awardType
主类
import pygame
import sys
import random
from setting import Settings
from hero import Hero
from airplane import Airplane
from bee import Bee
from enemy import Enemy
from award import Award
START=0
RUNNING=1
PAUSE=2
GAMEOVER=3
state=START
sets = Settings()
screen = pygame.display.set_mode(
(sets.bgImageWidth, sets.bgImageHeight), 0, 32) #创建窗口
hero=Hero(screen,sets.heroImages)
flyings=[]
bullets=[]
score=0
def hero_blitme():
"""画英雄机"""
global hero
hero.blitme()
def bullets_blitme():
"""画子弹"""
for b in bullets:
b.blitme()
def flyings_blitme():
"""画飞行物"""
global sets
for fly in flyings:
fly.blitme()
def score_blitme():
"""画分数和生命值"""
pygame.font.init()
fontObj=pygame.font.Font("SIMYOU.TTF", 20) #创建font对象
textSurfaceObj=fontObj.render(u'生命值:%d\n分数:%d\n火力值:%d'%(hero.getLife(),score,hero.isDoubleFire()),False,(135,100,184))
textRectObj=textSurfaceObj.get_rect()
textRectObj.center=(300,40)
screen.blit(textSurfaceObj,textRectObj)
def state_blitme():
"""画状态"""
global sets
global state
if state==START:
screen.blit(sets.start, (0,0))
elif state==PAUSE:
screen.blit(sets.pause,(0,0))
elif state== GAMEOVER:
screen.blit(sets.gameover,(0,0))
def blitmes():
"""画图"""
hero_blitme()
flyings_blitme()
bullets_blitme()
score_blitme()
state_blitme()
def nextOne():
"""生成敌人"""
type=random.randint(0,20)
if type<4:
return Bee(screen,sets.beeImage)
elif type==5:
return Bee(screen,sets.beeImage) #本来准备在写几个敌机的,后面没找到好看的图片就删了
else:
return Airplane(screen,sets.airImage)
flyEnteredIndex=0
def enterAction():
"""生成敌人"""
global flyEnteredIndex
flyEnteredIndex+=1
if flyEnteredIndex%40==0:
flyingobj=nextOne()
flyings.append(flyingobj)
shootIndex=0
def shootAction():
"""子弹入场,将子弹加到bullets"""
global shootIndex
shootIndex +=1
if shootIndex % 10 ==0:
heroBullet=hero.shoot(sets.heroBullet)
for bb in heroBullet:
bullets.append(bb)
def stepAction():
"""飞行物走一步"""
hero.step()
for flyobj in flyings:
flyobj.step()
global bullets
for b in bullets:
b.step()
def outOfBoundAction():
"""删除越界的敌人和飞行物"""
global flyings
flyingLives=[]
index=0
for f in flyings:
if f.outOfBounds()==True:
flyingLives.insert(index,f)
index+=1
flyings=flyingLives
index=0
global bullets
bulletsLive=[]
for b in bullets:
if b.outOfBounds()==True:
bulletsLive.insert(index,b)
index+=1
bullets=bulletsLive
j=0
def bangAction():
"""子弹与敌人碰撞"""
for b in bullets:
bang(b)
def bang(b):
"""子弹与敌人碰撞检测"""
index=-1
for x in range(0,len(flyings)):
f=flyings[x]
if f.shootBy(b):
index=x
break
if index!=-1:
one=flyings[index]
if isinstance(one,Enemy):
global score
score+=one.getScore() # 获得分数
flyings.remove(one) # 删除
if isinstance(one,Award):
type=one.getType()
if type==Award.DOUBLE_FIRE:
hero.addDoubleFire()
else:
hero.addLife()
flyings.remove(one)
bullets.remove(b)
def checkGameOverAction():
if isGameOver():
global state
state=GAMEOVER
hero.reLife()
def isGameOver():
for f in flyings:
if hero.hit(f):
hero.sublife()
hero.clearDoubleFire()
flyings.remove(f)
return hero.getLife()<=0
def action():
x, y = pygame.mouse.get_pos()
blitmes() #打印飞行物
for event in pygame.event.get():
if event.type == pygame.QUIT:
sys.exit()
if event.type == pygame.MOUSEBUTTONDOWN:
flag=pygame.mouse.get_pressed()[0] #左键单击事件
rflag=pygame.mouse.get_pressed()[2] #右键单击事件
global state
if flag==True and (state==START or state==PAUSE):
state=RUNNING
if flag==True and state==GAMEOVER:
state=START
if rflag==True:
state=PAUSE
if state==RUNNING:
hero.move(x,y)
enterAction()
shootAction()
stepAction()
outOfBoundAction()
bangAction()
checkGameOverAction()
def main():
pygame.display.set_caption("飞机大战")
while True:
screen.blit(sets.bgImage, (0, 0)) # 加载屏幕
action()
pygame.display.update() # 重新绘制屏幕
# time.sleep(0.1) # 过0.01秒执行,减轻对cpu的压力
if __name__ == '__main__':
main()
``` 写这个主要是练习一下python,把基础打实些。pygame的文本书写是没有换行,得在新建一个对象去写,为了简单我把文本都书写在了一行。
**background.png**
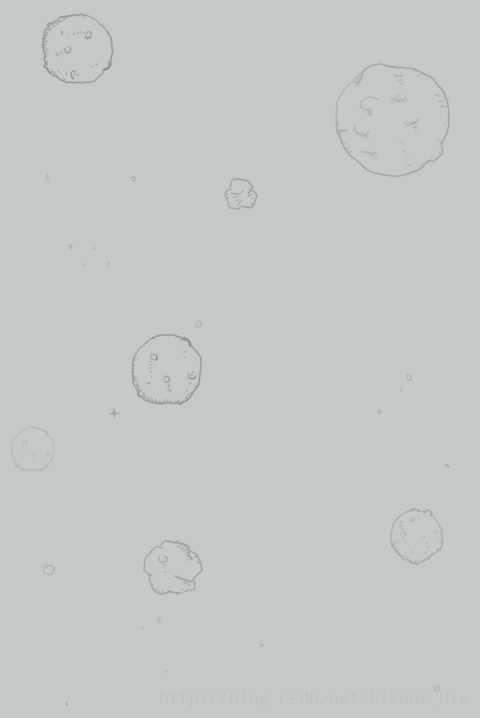
**hero.gif**
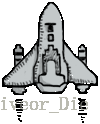
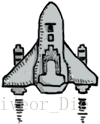
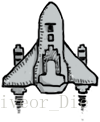
**bee.png**

**enemy.png**

**gameover.png**
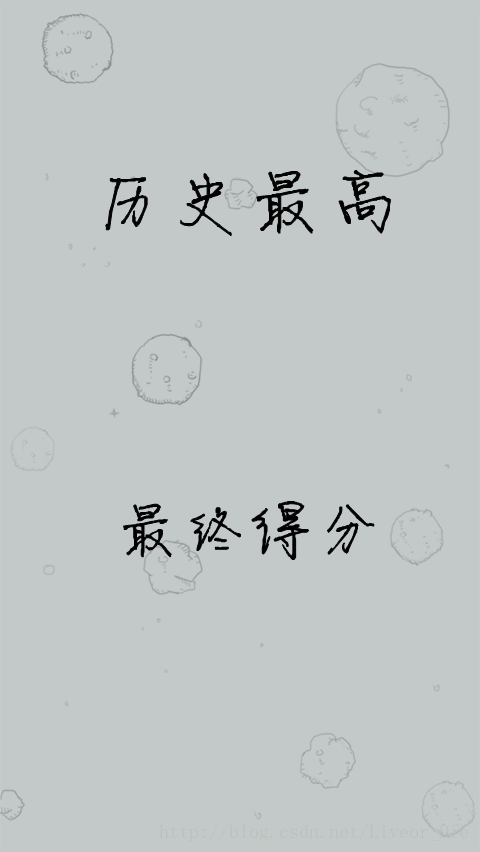
**start.png**
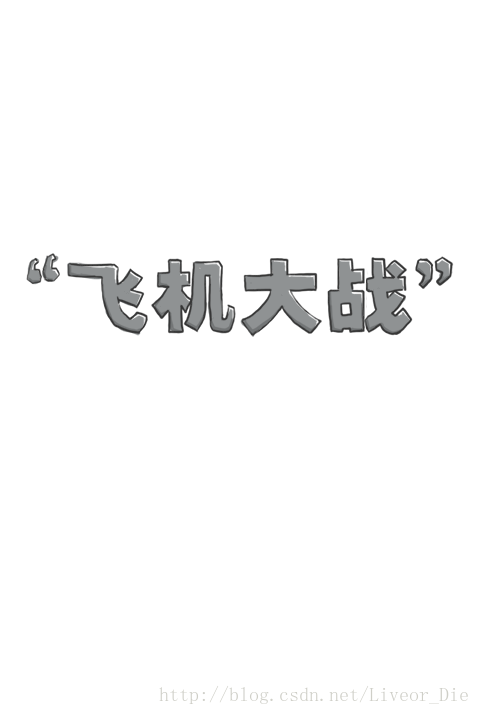
python飞机大战类_Python版飞机大战相关推荐
- C语言程序飞机大战,C语言版飞机大战游戏
C语言版飞机大战,供大家参考,具体内容如下 不多说直接上代码 #include #include #include #include #include using namespace std; /*= ...
- python如何定义类_Python 面向对象
Python 面向对象 Python从设计之初就已经是一门面向对象的语言,正因为如此,在Python中创建一个类和对象是很容易的.本章节我们将详细介绍Python的面向对象编程. 如果你以前没有接触过 ...
- python中定制类_python定制类__str__(实例详解)
在接下来的文章中,让我们明白什么是python中的自定义类.学习什么是python的自定义类,python定制类可以扮演何种角色在python编程.当你看到像__xxx__ __slots__变量或函 ...
- python编写ATM类_Python中编写类的各种技巧和方法
有关 Python 内编写类的各种技巧和方法(构建和初始化.重载操作符.类描述.属性访问控制.自定义序列.反射机制.可调用对象.上下文管理.构建描述符对象.Pickling).你可以把它当作一个教程, ...
- python如何定义类_python中定义类
广告关闭 腾讯云11.11云上盛惠 ,精选热门产品助力上云,云服务器首年88元起,买的越多返的越多,最高返5000元! 类的定义python中,定义类是通过class关键字,例如我们定义一个存储学生信 ...
- python写飞机大战游戏_python实现飞机大战游戏
飞机大战(Python)代码分为两个python文件,工具类和主类,需要安装pygame模块,能完美运行(网上好多不完整的,调试得心累.实现出来,成就感还是满满的),如图所示: 完整代码如下: 1.工 ...
- python做飞机大战游戏_python实现飞机大战游戏
飞机大战(Python)代码分为两个python文件,工具类和主类,需要安装pygame模块,能完美运行(网上好多不完整的,调试得心累.实现出来,成就感还是满满的),如图所示: 完整代码如下: 1.工 ...
- python制作飞机大战代码_python实现飞机大战完整代码,可运行
我发现很多python代码飞机大战在互联网上,但几乎没有一个是完整的.所以我做了一个完整的人.python代码分为两个文件,工具类和主类.python版本,pygame模块需要安装.完整的代码如下.1 ...
- python如何实现飞机上下移动_python实现飞机大战
本文实例为大家分享了python实现飞机大战的具体代码,供大家参考,具体内容如下 实现的效果如下: 主程序代码如下: import pygame from plane_sprites import * ...
最新文章
- Python __dict__属性详解
- 使用bottle进行web开发:get的参数传递,form数据传递等
- PCL—点云分割(基于凹凸性) 低层次点云处理
- Spring Boot入门(9)网页版计算器
- python 服务注册_python注册Windows服务
- insertRole attempted to return null from a method with a primitive return type
- 成功解决1406, “Data too long for column ‘txt‘ at row 1“
- Kotlin基础(五)Kotlin的类型系统
- linux 进程 线程 优先级,Linux编程-线程优先级的设定
- 【Latex系列】括号用法总结
- 前端学习路线,如何学习前端
- 达芬奇影视后期处理4K/8K图形工作站、存储完美2021配置推荐
- Python网络爬虫中图片下载简单实现
- Ubuntu 18.04 安装后的美化与软件安装
- There was a problem confirming the ssl certificate: [SSL: CERTIFICATE_VERIFY_FAILED]
- 芝诺志愿者走进养老院送温暖
- Softmax及其损失函数求导推导过程
- Robot framework中支持360浏览器测试
- B/S聊天室(websocket)
- AD导出3D模型的各种方法——AD转SW(MCAD插件一键生成),也适用于Fusion360、Inventor等三维建模软件
热门文章
- Java集合如何遍历删除指定元素
- html 语言 回车 替换,标记语言——图片替换
- Ubuntu16.04 挂载vivoZ3手机存储器
- html毕业论文的摘要,毕业论文摘要格式及范文
- Elasticsearch报错: received plaintext http traffic on an https channel, closing connection ...
- 抖音热门BGM爬虫下载
- python图像批量导入
- zbrush 使用dynamic mesh zremesh 进行快速拓扑
- mysql语句delete报错_MySQL delete语句的问题
- js字符串转数字遇到的问题