Python __dict__属性详解
由此可见, 类的静态函数、类函数、普通函数、全局变量以及一些内置的属性都是放在类__dict__里的
对象的__dict__中存储了一些属性
我们都知道Python一切皆对象,那么Python究竟是怎么管理对象的呢?
1、无处不在的__dict__
首先看一下类的__dict__属性和类对象的__dict__属性

# -*- coding: utf-8 -*-
class A(object):
“”"
Class A.
“”"
a </span>=<span style="color: #000000;"> 0
b </span>= 1<span style="color: #0000ff;">def</span> <span style="color: #800080;">__init__</span><span style="color: #000000;">(self):self.a </span>= 2<span style="color: #000000;">self.b </span>= 3<span style="color: #0000ff;">def</span><span style="color: #000000;"> test(self):</span><span style="color: #0000ff;">print</span> <span style="color: #800000;">'</span><span style="color: #800000;">a normal func.</span><span style="color: #800000;">'</span><span style="color: #000000;">@staticmethod
</span><span style="color: #0000ff;">def</span><span style="color: #000000;"> static_test(self):</span><span style="color: #0000ff;">print</span> <span style="color: #800000;">'</span><span style="color: #800000;">a static func.</span><span style="color: #800000;">'</span><span style="color: #000000;">@classmethod
</span><span style="color: #0000ff;">def</span><span style="color: #000000;"> class_test(self):</span><span style="color: #0000ff;">print</span> <span style="color: #800000;">'</span><span style="color: #800000;">a calss func.</span><span style="color: #800000;">'</span><span style="color: #000000;">
obj = A()
print A.dict
print obj.dict

运行结果如下:

{'a': 0, '__module__': '__main__', 'b': 1, 'class_test': <classmethod object at 0x00000000021882E8>, '__dict__': <attribute '__dict__' of 'A' objects>, '__init__': <function __init__ at 0x00000000023A5BA8>, 'test': <function test at 0x00000000023A5C18>, '__weakref__': <attribute '__weakref__' of 'A' objects>, '__doc__': '\n Class A.\n ', 'static_test': <staticmethod object at 0x00000000021881C8>} {'a': 2, 'b': 3}

由此可见, 类的静态函数、类函数、普通函数、全局变量以及一些内置的属性都是放在类__dict__里的
对象的__dict__中存储了一些self.xxx的一些东西
2、Python里什么没有__dict__属性
虽然说一切皆对象,但对象也有不同,就好比不是每个人的女朋友都是一个人一样,一些内置的数据类型是没有__dict__属性的,如下:

num = 3 ll = [] dd = {} print num.__dict__ print ll.__dict__ print dd.__dict__

当我们运行这样的代码时,解释器就会告诉我们

Traceback (most recent call last):File "f:\python\test.py", line 54, in <module>print num.__dict__ AttributeError: 'int' object has no attribute '__dict__'
Traceback (most recent call last):
File “f:\python\test.py”, line 55, in <module>
print ll.dict
AttributeError: ‘list’ object has no attribute ‘dict’
Traceback (most recent call last):
File “f:\python\test.py”, line 56, in <module>
print dd.dict
AttributeError: ‘dict’ object has no attribute ‘dict’

int, list, dict等这些常用的数据类型是没有__dict__属性的,其实这是可预料的,就算给了它们dict属性也没啥用,毕竟它们只是用来做数据容器的。
3、发生继承时候的__dict__属性
子类有自己的__dict__, 父类也有自己的__dict__,子类的全局变量和函数放在子类的dict中,父类的放在父类dict中。

# -*- coding: utf-8 -*-
class Parent(object):
a = 0
b = 1
<span style="color: #0000ff;">def</span> <span style="color: #800080;">__init__</span><span style="color: #000000;">(self):self.a </span>= 2<span style="color: #000000;">self.b </span>= 3<span style="color: #0000ff;">def</span><span style="color: #000000;"> p_test(self):</span><span style="color: #0000ff;">pass</span>
class Child(Parent):
a = 4
b = 5
<span style="color: #0000ff;">def</span> <span style="color: #800080;">__init__</span><span style="color: #000000;">(self):super(Child, self).</span><span style="color: #800080;">__init__</span><span style="color: #000000;">()# self.a </span>= 6<span style="color: #000000;"># self.b </span>= 7<span style="color: #0000ff;">def</span><span style="color: #000000;"> c_test(self):</span><span style="color: #0000ff;">pass</span><span style="color: #0000ff;">def</span><span style="color: #000000;"> p_test(self):</span><span style="color: #0000ff;">pass</span><span style="color: #000000;">
p = Parent()
c = Child()
print Parent.dict
print Child.dict
print p.dict
print c.dict

运行上面的代码,结果入下:

{'a': 0, '__module__': '__main__', 'b': 1, '__dict__': <attribute '__dict__' of 'Parent' objects>, 'p_test': <function p_test at 0x0000000002325BA8>, '__weakref__': <attribute '__weakref__' of 'Parent' objects>, '__doc__': None, '__init__': <function __init__ at 0x0000000002325B38>} {'a': 4, 'c_test': <function c_test at 0x0000000002325C88>, '__module__': '__main__', 'b': 5, 'p_test': <function p_test at 0x0000000002325CF8>, '__doc__': None, '__init__': <function __init__ at 0x0000000002325C18>} {'a': 2, 'b': 3} {'a': 2, 'b': 3}

1)上段输出结果中,用红色字体标出的是类变量和函数,由结果可知,每个类的类变量、函数名都放在自己的__dict__中
2) 再来看一下实力变量的__dict__中,由蓝色字体标识,父类和子类对象的__dict__是公用的
总结:
1) 内置的数据类型没有__dict__属性
2) 每个类有自己的__dict__属性,就算存着继承关系,父类的__dict__ 并不会影响子类的__dict__
3) 对象也有自己的__dict__属性, 存储self.xxx 信息,父子类对象公用__dict__
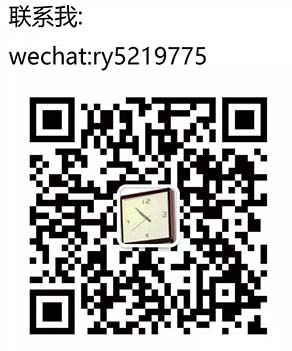
Python __dict__属性详解相关推荐
- __dict__属性详解
1.类的__dict__属性和类对象的__dict__属性 class Parent(object):a = 'a'b = 1def __init__(self):self.a = 'A'self.b ...
- python中文读音ndarray-numpy中的ndarray方法和属性详解
NumPy数组的维数称为秩(rank),一维数组的秩为1,二维数组的秩为2,以此类推.在NumPy中,每一个线性的数组称为是一个轴(axes),秩其实是描述轴的数量.比如说,二维数组相当于是一个一维数 ...
- Python元类详解
文章目录 Python元类详解 Python谜团 元类的本质 调用一个类时发生了什么 再探元类 自定义元类 彩蛋:跳过python解释器 Python元类详解 元类比99%的用户所担心的魔法要更深,如 ...
- windows上安装Anaconda和python的教程详解
一提到数字图像处理编程,可能大多数人就会想到matlab,但matlab也有自身的缺点: 1.不开源,价格贵 2.软件容量大.一般3G以上,高版本甚至达5G以上. 3.只能做研究,不易转化成软件. 因 ...
- python安装教程windows-windows上安装Anaconda和python的教程详解
一提到数字图像处理编程,可能大多数人就会想到matlab,但matlab也有自身的缺点: 1.不开源,价格贵 2.软件容量大.一般3G以上,高版本甚至达5G以上. 3.只能做研究,不易转化成软件. 因 ...
- Python 装饰器详解(下)
Python 装饰器详解(下) 转自:https://blog.csdn.net/qq_27825451/article/details/84627016,博主仅对其中 demo 实现中不适合pyth ...
- Python 装饰器详解(中)
Python 装饰器详解(中) 转自:https://blog.csdn.net/qq_27825451/article/details/84581272,博主仅对其中 demo 实现中不适合pyth ...
- Struts2零配置属性详解(2)
2019独角兽企业重金招聘Python工程师标准>>> Struts2 零配置属性详解 一.插件包 直接引入myEclipse里面的struts code 包即可. struts2- ...
- python协程详解
目录 python协程详解 一.什么是协程 二.了解协程的过程 1.yield工作原理 2.预激协程的装饰器 3.终止协程和异常处理 4.让协程返回值 5.yield from的使用 6.yield ...
最新文章
- Java Swing 探索(一)LayoutManager
- makefile中变量有哪些?
- golang 释放内存机制的探索
- 企业级实战03_真实项目实战SpringMVC整合ActiveMQ
- ftp搜索文件_Windows10下搭建FTP服务器详解(多图预警)
- Ubuntu crontab 定时 python 详细
- 微信公布6月朋友圈十大谣言 包括不打疫苗不让上飞机高铁等
- server2003 IIS6.0 网站不可用
- mysql safe 停止工作_mysql不明原因停止后不能启动,请高手分析
- ashly理器4.8软件汉化版_工程造价专业 常用广联达CAD等软件 推荐什么笔记本电脑?...
- 性能测试--jmeter中的HTTP信息头管理器的使用【8】
- Java游戏程序设计 第3章 游戏程序的基本框架
- ZeptoLab Code Rush 2015 B. Om Nom and Dark Park DFS
- python 图标字体_使用nerd-font/font-patcher为字体添加字体图标
- html网页中加入音乐播放器,如何在网页中插入音乐播放器
- java多人聊天_java编程实现多人聊天室功能
- html中自定义右键菜单功能,HTML中自定义右键菜单功能
- NFT新范式,OKALEIDO创新NFT聚合交易生态
- Android系统的VTS测试套件介绍
- SPI控制MS5614T
热门文章
- zeroclipboard 粘贴板的应用示例, 兼容 Chrome、IE等多浏览器
- 【C#】枚举_结构体_数组
- batch normalization
- HMM——维特比算法(Viterbi algorithm)
- LeetCode简单题之机器人能否返回原点
- TensorFlow反向传播算法实现
- 适用于AMD ROC GPU的Numba概述
- 点击事件如何传递到Activity中
- 2021年大数据Hive(一):​​​​​​​Hive基本概念
- 2021年大数据Hadoop(三):Hadoop国内外应用