使用codeigniter_使用CodeIgniter解开MVC
使用codeigniter
When I first started programming the only type of code I wrote was procedural. You know the type, one thing leads to another with a thrown in function here and there and you have a working application. As I grew as a programmer, I began to find out that way of programming is fine for small projects but when I started to develop bigger applications my code became very disorganized and hard to read. To combat this I started writing my own classes to help me write better, reusable code that I could use in all my applications. Then I realized that although I was learning a lot by doing this, I was reinventing the wheel. Most of what I was writing had already been accomplished many times over in various PHP frameworks. I began looking into the almost endless selection of PHP frameworks and saw most of them were also based on something called MVC.
刚开始编程时,我编写的唯一类型的代码是过程代码。 您知道类型,一件事导致在另一处抛出功能,而您有一个正在运行的应用程序。 随着我成为一名程序员的成长,我开始发现这种编程方式适用于小型项目,但是当我开始开发更大的应用程序时,我的代码变得非常混乱而且难以阅读。 为了解决这个问题,我开始编写自己的类,以帮助我编写可以在所有应用程序中使用的更好,可重用的代码。 然后我意识到,尽管我从中学到了很多,但我还是在重新发明轮子。 我写的大部分内容已经在各种PHP框架中完成了许多次。 我开始研究几乎无穷无尽PHP框架,并发现其中大多数也基于称为MVC的东西。
Learning to program using MVC was a complete paradigm shift for me, but it was well worth the struggle. If you want to develop applications with sell-structured, readable code that you can quickly diagnose problems in, then MVC is for you. In this article I’ll untangle the mysteries of MVC for you using CodeIgniter, a PHP framework based on the MVC pattern. I’ll first present a high level overview of MVC, what it is and how it can help you to become a better programmer, and then guide you through writing a simple web form the CodeIgniter way so you can see how the pattern looks in action.
对我而言,使用MVC进行编程是一种完全的范式转变,但这值得我们为之奋斗。 如果您想使用可以快速诊断问题的销售结构,可读代码开发应用程序,那么MVC非常适合您。 在本文中,我将使用CodeIgniter(基于MVC模式PHP框架)为您解开MVC的奥秘。 我将首先概述MVC,它是什么,以及它如何帮助您成为更好的程序员,然后指导您以CodeIgniter方式编写简单的Web表单,以便您了解模式的实际效果。 。
什么是MVC? (What is MVC?)
MVC is another acronym you’ll want to add to your endless book of acronyms; it stands for Model-View-Controller and is an software architectural design pattern. Adhering to the MVC pattern of will separate your application’s logic from its data and presentation. If you have ever seen code like this:
MVC是另一个您想添加到无休止的首字母缩略词中的首字母缩略词。 它代表Model-View-Controller,是一种软件体系结构设计模式。 坚持MVC模式将把您的应用程序的逻辑与数据和表示分离。 如果您曾经看过这样的代码:
<?php if($somthing == $thisthing) echo "<p>Hello there</p>"; ?>
then you completely understand how a page full of mixed up PHP and HTML can become really hard to maintain and read. So let’s look at each part of MVC and see what each part represents.
那么您将完全理解如何将一个由PHP和HTML混合而成的页面变得很难维护和阅读。 因此,让我们看一下MVC的每个部分,看看每个部分代表什么。
Model – encapsulates your data management routines. Usually this is the part of your code that retrieves, inserts, updates, and deletes data in you database (or whatever else you’re using for a data store).
模型 –封装您的数据管理例程。 通常,这是代码的一部分,用于检索,插入,更新和删除数据库(或用于数据存储的其他任何数据)中的数据。
View – encapsulates the information that is presented to a user. This is the actual web page, RSS feed, or even a part of a page like a header of footer.
视图 –封装提供给用户的信息。 这是实际的网页,RSS提要,甚至是页面的一部分,例如页脚的页眉。
Controller – coordinates the Model and View parts of your application to respond to the request. A controller accepts input from the user and instructs the model and a view to perform actions based on that input; it controls the flow of information in the application.
控制器 –协调应用程序的“模型”和“视图”部分以响应请求。 控制器接受来自用户的输入,并指示模型和视图根据该输入执行操作; 它控制应用程序中的信息流。
To better understand how the MVC pattern works, let’s look at the steps you would take to code a PHP form using this pattern.
为了更好地理解MVC模式是如何工作的,让我们看一下使用该模式编写PHP表单所要执行的步骤。
- The user interacts with the View which presents a web form.用户与显示Web表单的视图进行交互。
- The user submits the form, and the Controller receives the POST request. It passes this data to the Model.用户提交表单,然后控制器收到POST请求。 它将这些数据传递给模型。
- The Model updates and queries the database and sends the result back to the Controller.模型更新并查询数据库,并将结果发送回控制器。
- The Controller passes the Model’s response to the View.控制器将模型的响应传递给视图。
- The View updates itself with the new data and is displayed to the user.视图将使用新数据进行更新,并显示给用户。
安装CodeIgniter (Installing CodeIgniter)
With so many MVC frameworks out there, how do you decide which one to choose – don’t they all provide similar functionality just differing slightly in their implementation and syntax? I had never used a framework before so I had to read through a lot of documentation and experiment with them to find out which one was right for me. I found that CodeIgniter had a much smaller learning curve then the others, which is probably why it has a reputation for being great for beginners. It’s documentation is very clear and provides many code examples to help you along the way. Although in this article I’ll be using CodeIgniter, the concept of MVC will be applicable to almost any framework you choose.
有这么多的MVC框架,您如何决定选择哪一个-它们是否都提供相似的功能,只是它们的实现和语法略有不同? 我以前从未使用过一种框架,因此我必须通读大量文档并对其进行实验,以找出最适合我的框架。 我发现CodeIgniter的学习曲线比其他的要小得多,这可能就是为什么它以对初学者而言出色而闻名的原因。 它的文档非常清晰,并提供了许多代码示例来帮助您。 尽管在本文中,我将使用CodeIgniter,但MVC的概念将几乎适用于您选择的任何框架。
CodeIgniter is a cinch to install and configure for you system. Follow these steps and you’ll be up and running in less then five minutes.
CodeIgniter是为您的系统安装和配置的工具。 按照以下步骤操作,您将在不到五分钟的时间内启动并运行。
Download the latest version of CodeIgniter. As of this writing, the latest version is 2.0.3.
下载最新版本的CodeIgniter 。 撰写本文时,最新版本为2.0.3。
- Uncompress the archive and place the uncompressed directory in your web root.解压缩档案并将未压缩的目录放置在您的Web根目录中。
Rename the directory from
CodeIgniter_2.0.3
toCI
将目录从
CodeIgniter_2.0.3
重命名为CI
Open
CI/application/config/config.php
and change the base URL to your server. In my case, it is:$config['base_url'] = "http://localhost/CI";
打开
CI/application/config/config.php
并将基本URL更改为服务器。 就我而言,它是:$config['base_url'] = "http://localhost/CI";
Go to the
CI
directory on the server using your web browser. Again, in my case it’shttp://localhost/CI
.使用Web浏览器转到服务器上的
CI
目录。 同样,在我的情况下,它是http://localhost/CI
。
You’ve now finished the CodeIgniter installation and should see a web page like the one below:
现在,您已经完成了CodeIgniter的安装,应该会看到一个类似于以下内容的网页:
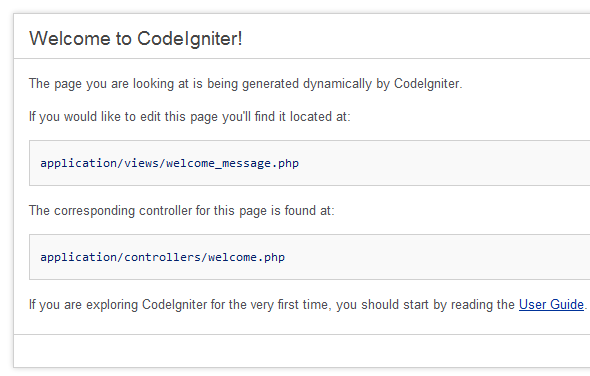
Now that you have everything installed you’ll have probably noticed all the directories that CodeIgniter has. Don’t be intimidated; you won’t have to deal with most of them since the majority of your work will take place in the system/application
directory.
现在,您已经安装了所有内容,您可能会注意到CodeIgniter拥有的所有目录。 不要被吓倒; 您无需处理大多数问题,因为大部分工作将在system/application
目录中进行。
Looking in the system/application
directory there are subdirectories named controllers
, models
, and views
. This is where you will place your files for your application. Your controller files will be in the controllers
directory, model files in the models
directory, and view files in the views
directory.
在system/application
目录中,有名为controllers
, models
和views
子目录。 在这里您将为应用程序放置文件。 您的控制器文件将在controllers
目录中,model文件在models
目录中,而view文件在views
目录中。
少说话,多代码 (Less Talk, More Code)
Now that you have a basic understanding of what the MVC architecture is and have CodeIgniter installed, let’s get down to some coding. In this example you’ll create a very simple form to collect email addresses using CodeIgniter’s form helper classes and form validation library. Let’s get started.
现在,您已经对MVC架构有了基本的了解,并安装了CodeIgniter,让我们开始一些编码。 在此示例中,您将创建一个非常简单的表单,以使用CodeIgniter的表单帮助器类和表单验证库来收集电子邮件地址。 让我们开始吧。
创建控制器 (Create the Controller)
Create a new file in your application/controllers
directory named sitepointform.php
that contains the following code:
在名为sitepointform.php
application/controllers
目录中创建一个新文件,其中包含以下代码:
<?php
class Sitepointform extends CI_Controller
{
public function index() {
// Load the Form helper which provides methods to assist
// working with forms.
$this->load->helper("form");
// Load the form validation classes
$this->load->library("form_validation");
// As you have loaded the validation classes, you can now
// apply rules to the fields you want validated. The
// functions below take three arguments:
// 1. The name of form field
// 2. The human name of the field to be displayed in
// the event of an error
// 3. The names of the validation rules to apply
$this->form_validation->set_rules("first", "First Name",
"required");
$this->form_validation->set_rules("last", "Last Name",
"required");
$this->form_validation->set_rules("email", "Email Address",
"required|valid_email");
// Check whether the form validates. If not, present the
// form view otherwise present the success view.
if ($this->form_validation->run() == false) {
$this->load->view("sitepointform_view");
}
else {
$this->load->view("formsuccess");
}
}
}
创建视图 (Create the Views)
Next, create a file in your application/views
directory called sitepointform_view.php
with this code:
接下来,使用以下代码在application/views
目录中创建一个名为sitepointform_view.php
文件:
<?php
// Display any form validation error messages
echo validation_errors();
// Using the form helper to help create the start of the form code
echo form_open("sitepointform");
?>
<label for="first">First Name</label>
<input type="text" name="first"><br>
<label for="last">Last Name</label>
<input type="text" name="last"><br>
<label for="email">Email Address</label>
<input type="text" name="email"><br>
<input type="submit" name="submit" value="Submit">
</form>
</html>
Create the file application/views/formsuccess_view.php
with this code which will be showed later when a form is successfully submitted:
使用以下代码创建文件application/views/formsuccess_view.php
,稍后成功提交表单时将显示该代码:
<html>
<head>
<title>Form Success</title>
</head>
<body>
<h3>Your form was successfully submitted!</h3>
<p><a href="http://localhost/CI/index.php/sitepointform">Enter a new
email address!</a></p>
</body>
</html>
Now visit http://localhost/CI/index.php/sitepointform/index
and you’ll see your newly created form:
现在访问http://localhost/CI/index.php/sitepointform/index
,您将看到新创建的表单:
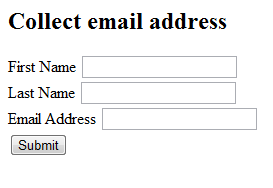
The URL follows this pattern in your application:
URL在您的应用程序中遵循以下模式:
http://yourwebroot/[controller-class]/[controller-method]/[agruments]
Here sitepointform
is the controller class, and index
is the desired method. If you were renamed the method to myForm()
, the URL would change like this:
这里sitepointform
是控制器类,而index
是所需的方法。 如果将方法重命名为myForm()
,则URL将会像这样更改:
http://localhost/CI/index.php/sitepointform/myForm
In the absence of a method in the URL, CodeIgniter will assume index
.
URL中没有方法时,CodeIgniter将采用index
。
Hit submit without any input in the text fields to try out the validation. You’ll see CodeIgniter enforcing the rules you set up earlier in the controller and displays the error messages.
在文本字段中未输入任何内容的情况下点击“提交”即可进行验证。 您将看到CodeIgniter强制执行您先前在控制器中设置的规则,并显示错误消息。
创建模型 (Create the Model)
Now that you have your form built, you need somewhere to store all the names and email addresses. Create a table addresses
with the following statement in your database:
现在您已经建立了表单,现在需要在某个地方存储所有名称和电子邮件地址。 在数据库中使用以下语句创建表addresses
:
CREATE TABLE addresses (
id INTEGER UNSIGNED NOT NULL AUTO_INCREMENT,
last_name VARCHAR(128) NOT NULL,
first_name VARCHAR(128) NOT NULL,
email VARCHAR(128) NOT NULL,
PRIMARY KEY (id)
);
With the database table in place, now you have to tell CodeIgniter where it is. Open up application/config/database.php
and enter your database credentials. Specify appropriate values for the hostname, username, password, database, and dbdriver keys.
有了数据库表之后,现在您必须告诉CodeIgniter它在哪里。 打开application/config/database.php
并输入数据库凭据。 为主机名 , 用户名 , 密码 , 数据库和dbdriver键指定适当的值。
Then, create application/models/sitepointform_model.php
with this code:
然后,使用以下代码创建application/models/sitepointform_model.php
:
<?php
class Sitepointform_model extends CI_Model
{
public function insert_address($data) {
$this->load->database();
$this->db->insert("addresses", $data);
}
}
The class has a method named insert_address()
that is accepts the array of values passed in from the controller. CodeIgniter has a database class built in so you don’t have to write any SQL at all; $this->db->insert()
will automatically generate and execute the SQL for you.
该类具有一个名为insert_address()
的方法,该方法接受从控制器传入的值的数组。 CodeIgniter内置了一个数据库类,因此您根本不必编写任何SQL。 $this->db->insert()
将自动为您生成并执行SQL。
试试看 (Try it Out)
To use the model from the controller, open up application/controllers/sitepointform.php
and update the index()
method as follows:
要从控制器使用模型,请打开application/controllers/sitepointform.php
并更新index()
方法,如下所示:
<?php
public function index() {
$this->load->helper("form");
$this->load->library("form_validation");
$this->form_validation->set_rules("first", "First Name",
"required");
$this->form_validation->set_rules("last", "Last Name",
"required");
$this->form_validation->set_rules("email", "Email Address",
"required|valid_email");
if ($this->form_validation->run() == false) {
$this->load->view("sitepointform_view");
}
else {
$first = $_POST["first"];
$last = $_POST["last"];
$email = $_POST["email"];
$data = array("first_name" => $first,
"last_name" => $last,
"email" => $email);
$this->load->model("sitepointform_model");
$this->sitepointform_model->insert_address($data);
$this->load->view("formsuccess");
}
}
The incoming data posted from the form is placed into an associative array to be passed into the model’s insert_address()
method. Note the keys in the array have the same names as the columns in the addresses table. This is important for the final step in creating the model. Then the model is loaded with $this->load->model()
, the data is passed to the insert_address()
method, and the success view is displayed.
从表单发布的传入数据被放置到关联数组中,以传递到模型的insert_address()
方法中。 请注意,数组中的键与地址表中的列具有相同的名称。 这对于创建模型的最后一步很重要。 然后,使用$this->load->model()
加载$this->load->model()
,将数据传递到insert_address()
方法,并显示成功视图。
Go back to http://localhost/CI/index.php/sitepointform
and enter some data. If you fill in all the text fields correctly you’ll see the success page and the data is now in your database.
返回http://localhost/CI/index.php/sitepointform
并输入一些数据。 如果正确填写所有文本字段,您将看到成功页面,并且数据现在已存在数据库中。
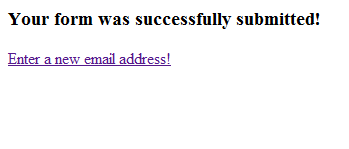
摘要 (Summary)
Hopefully you now understand what MVC is all about, a way of separating pieces of your code based on their function to keep everything more organized. Though CodeIgniter happens to be my framework of choice, knowledge of the pattern is applicable to almost any other framework you choose, and of course you can implement it in your own code without a framework as well.
希望您现在了解了MVC的全部含义,这是一种根据代码的功能将代码段分开以保持所有内容井井有条的方式。 尽管CodeIgniter恰好是我选择的框架,但是对模式的了解几乎适用于您选择的任何其他框架,当然您也可以在没有框架的情况下在自己的代码中实现它。
Image via Alexey Rozhanovsky / Shutterstock
图片来自Alexey Rozhanovsky / Shutterstock
翻译自: https://www.sitepoint.com/untangling-mvc-with-codeigniter/
使用codeigniter
使用codeigniter_使用CodeIgniter解开MVC相关推荐
- 使用codeigniter_使用CodeIgniter分页
使用codeigniter When you perform a search on Google, the results are displayed in a list and you have ...
- 使用codeigniter_使用CodeIgniter探索面向方面的编程,第2部分
使用codeigniter In the previous part of this series we learned what Aspect Oriented Programming (AOP) ...
- vs2017安装mvc框架_2017年PHP MVC框架的现状
vs2017安装mvc框架 This article was first published on ZenOfCoding, and re-published here with the author ...
- java ci框架_CI(CodeIgniter)框架介绍
CodeIgniter 是一个应用程序框架 1.免费:CodeIgniter是经过Apache/BSD-style开源许可授权的,只要你愿意就可以使用它. 2.简单:CodeIgniter是真正的轻量 ...
- CI(CodeIgniter)框架入门
什么是CI框架: CdoeIgniter是为PHP开发人员提供的一套Web应用程序工具包.他的目的是能够让你从零开始更加快速的完成项目,它提供了一套丰富的类库来满足我们的日常需求,并且提供了一个简单的 ...
- php 做app服务 开源,APP 与 PHP 的开源项目
展示类APP 其实就是 web 应用程序的外壳,他穿上了华丽的外衣,其肉体都是web应用程序后台的数据. PHP的开源WEB应用程序非常丰富.例如我们做博客类的网站可以选择wordpress 做CMS ...
- mvc路由原理 php_PHP实战002:CodeIgniter安装和入门使用
CodeIgniter简介 CodeIgniter 是一个基于MVC框架的PHP轻量级框架(开源),它的目标是让你能够更快速的开发,它提供了日常任务中所需的大量类库,以及简单的接口和逻辑结构.通过减少 ...
- php cli python,PHP MVC框架 CodeIgniter CLI模式简介
PHP MVC框架 CodeIgniter CLI模式简介 分类:PHP_Python| 发布:佚名| 查看: | 发表时间:2014/7/25 让我们以Hello World为例,首先创建一个简单的 ...
- codeigniter_如何在浏览器中查看CodeIgniter日志文件
codeigniter by Seun Matt 通过Seun Matt 如何在浏览器中查看CodeIgniter日志文件 (How to View CodeIgniter Log Files in ...
- 使用 CodeIgniter 框架快速开发 PHP 应用(五)
原文:使用 CodeIgniter 框架快速开发 PHP 应用(五) 简化 HTML 页面和表格设计 这一章介绍了又一个节约你的时间而且使你的代码更具安全性和逻辑性的领域. 第一,我们将会介绍创建视图 ...
最新文章
- OWASP top 10 (2017) 学习笔记--失效的身份验证
- Java Spring Boot VS .NetCore (七) 配置文件
- android 截屏指定区域,Android截图 截取ContentView 截取指定的View并且保存
- 二十八、Java中的Int和Integer的区别
- p1044与p1898
- css复合选择器 1205
- docker和数据卷问题探究
- Spring-MetadataReader接口
- oracle参数文件备份,备份的控制文件和新的数据文件
- K8S实战之部署java应用
- oracle网络加载错误怎么解决,Oracle加载数据库错误解决的方法详细教程
- C++连接MYSQL教程
- Windows10上安装EPLAN无法连接虚拟加密狗的解决方法
- java上传文件怎么设置成777权限_如何修改文件夹777权限
- PHP C#-QQ网站bkn算法
- dell设置从ssd启动_2. 戴尔电脑如何设置固态硬盘启动?
- Beyond Compare实现Class文件对比
- 历经一个月的时间,在大家的共同努力下新星计划圆满结束,让我们看一下详细数据吧!
- linux 找u盘,linux系统怎样找到U盘?
- 2021软件评测师真题