remix使用_使用Remix展平合同和调试
remix使用
Smart contracts on the Ethereum main-net use real money, so building error-free smart contracts is crucial and requires special tools like debuggers.
以太坊主网上的智能合约使用真实资金,因此构建无错误的智能合约至关重要,并且需要调试器之类的特殊工具。
Remix IDE is the most fully-featured IDE for Solidity. Among other tools, it has an excellent step-by-step debugger. You can perform various tasks such as moving in time, changing the current step, exploring local variables and current state by expanding various panels.
Remix IDE是Solidity中功能最齐全的IDE。 在其他工具中,它具有出色的逐步调试器。 您可以执行各种任务,例如移动时间,更改当前步骤,通过展开各种面板来浏览局部变量和当前状态。
You can also set breakpoints to move between different points in the code. And the terminal allows you to display transactions executed from Remix and debug them.
您还可以设置断点以在代码中的不同点之间移动。 并且终端允许您显示从Remix执行的事务并调试它们。
Throughout this tutorial, we’ll use Truffle and OpenZeppelin to build a simple custom token. We’ll see why and how to flatten the contract, and finally we’ll use Remix IDE to start debugging the contract.
在整个教程中,我们将使用Truffle和OpenZeppelin构建简单的自定义令牌。 我们将了解为什么以及如何展平合同,最后,我们将使用Remix IDE开始调试合同。
为什么要展平智能合约? (Why Flatten a Smart Contract?)
Flattening a smart contract refers to combining all Solidity code in one file instead of multiple source files such that, instead of having imports, the imported code is embedded in the same file. We need to flatten smart contracts for various reasons, such as manually reviewing the contract, verifying the contract on Etherscan, or debugging the contract with Remix IDE, as it currently doesn’t support imports.
展平智能合约是指将所有Solidity代码组合在一个文件中,而不是多个源文件中,从而使导入的代码被嵌入同一文件中,而不是进行导入。 由于各种原因,我们需要展平智能合约,例如手动检查合约,在Etherscan上验证合约或使用Remix IDE调试合约,因为该合约目前不支持导入。
使用Truffle和OpenZeppelin编写简单令牌 (Writing a Simple Token Using Truffle and OpenZeppelin)
Remix IDE is officially recommended for building small contracts or for the sake of learning Solidity, but once you need to build a larger contract or need advanced compilation options, you’ll have to use the Solidity compiler or other tools/frameworks such as Truffle.
正式建议使用Remix IDE来构建小型合同或为了学习Solidity,但是一旦您需要构建较大的合同或需要高级编译选项,则必须使用Solidity编译器或其他工具/框架(例如Truffle)。
Besides error-free contracts, security is also a crucial part of building a smart contract. For this reason, using battle-tested frameworks like OpenZeppelin that provides reusable, well-tested, community-reviewed smart contracts, will help you reduce the vulnerabilities in your Dapps.
除了无差错合同,安全性也是构建智能合同的关键部分。 因此,使用经过测试的框架(如OpenZeppelin)提供可重复使用,经过良好测试,经过社区审查的智能合约,将帮助您减少Dapps中的漏洞。
Let’s now see how we can use Truffle and OpenZeppelin to create a simple custom Token that extends the standard token from OpenZeppelin.
现在,让我们看看如何使用Truffle和OpenZeppelin创建一个简单的自定义令牌,该令牌扩展了OpenZeppelin的标准令牌。
要求 (Requirements)
This tutorial requires some knowledge of Truffle, Ethereum and Solidity. You can read the Blockchain Introduction and Ethereum Introduction.
本教程要求对松露,以太坊和坚实性有所了解。 您可以阅读区块链简介和以太坊简介 。
You also need to have Node.js 5.0+ and npm installed on your system. Refer to their download page for instructions.
您还需要在系统上安装Node.js 5.0+和npm。 有关说明,请参阅其下载页面 。
安装松露 (Installing Truffle)
Using your terminal, run the following command to install Truffle:
使用您的终端,运行以下命令来安装Truffle:
npm install -g truffle
创建一个新的松露项目 (Creating a New Truffle Project)
Start by creating a new directory for your project. Let’s call it simpletoken
and navigate into it:
首先为您的项目创建一个新目录。 让我们将其称为simpletoken
并导航至其中:
mkdir simpletoken
cd simpletoken
Next, create the actual project files using:
接下来,使用以下命令创建实际的项目文件:
truffle init
This command will create multiple folders, such as contracts/
and migrations/
, and files that will be used when deploying the contract to the blockchain.
该命令将创建多个文件夹,例如contracts/
和migrations/
,以及在将合同部署到区块链时将使用的文件。
Next, we’ll install OpenZeppelin:
接下来,我们将安装OpenZeppelin:
npm install openzeppelin-solidity
创建一个简单的代币合约 (Creating a Simple Token Contract)
Inside the contracts/
folder, create a file named SimpleToken.sol
and add the following content:
在contracts/
文件夹中,创建一个名为SimpleToken.sol
文件,并添加以下内容:
pragma solidity ^0.4.23;
import 'openzeppelin-solidity/contracts/token/ERC20/StandardToken.sol';
contract SimpleToken is StandardToken {
address public owner;
string public name = 'SimpleToken';
string public symbol = 'STt';
uint8 public decimals = 2;
uint public INITIAL_SUPPLY = 10000;
constructor() public {
totalSupply_ = INITIAL_SUPPLY;
balances[owner] = INITIAL_SUPPLY;
}
}
This is the contract that we’re going to flatten and debug. We’re importing the OpenZeppelin StandardToken.sol
contract and declaring our SimpleToken
contract which extends StandardToken.sol
using the is
operator.
这是我们要展平和调试的合同。 我们正在导入OpenZeppelin StandardToken.sol
合同,并声明使用is
运算符扩展了StandardToken.sol
SimpleToken
合同。
Our contract will inherit a bunch of variables and functions, which need to be overridden in order to customize the contract.
我们的合同将继承一堆变量和函数,需要对其进行重写以自定义合同。
For the sake of completing our Truffle project, inside the migrations/
folder of your project, create a file called 2_deploy_contract.js
and add the following content:
为了完成我们的Truffle项目,请在项目的migrations/
文件夹中创建一个名为2_deploy_contract.js
的文件,并添加以下内容:
var SimpleToken = artifacts.require("SimpleToken");
module.exports = function(deployer) {
deployer.deploy(SimpleToken);
};
Using this file, we can deploy/migrate our smart contract into the blockchain, but we don’t actually need this for this example, because we’re going to use Remix IDE to deploy the smart contract after flattening it.
使用此文件,我们可以将智能合约部署/迁移到区块链中,但是在本示例中我们实际上并不需要它,因为在扁平化之后,我们将使用Remix IDE来部署智能合约。
使用松露压平器压平合同 (Flattening the Contract Using Truffle Flattener)
Truffle Flattener is an npm utility that flattens or combines Solidity files developed under Truffle with all of their dependencies in the right order.
Truffle Flattener是一个npm实用程序,可以按正确的顺序对Truffle下开发的Solidity文件及其所有依赖项进行展平或合并。
First, start by installing truffle-flattener
from npm globally using:
首先,首先使用以下命令从npm全局安装truffle-flattener
:
npm install -g truffle-flattener
Next, inside your Truffle project, run the following command to flatten the SimpleToken.sol
file:
接下来,在您的Truffle项目中,运行以下命令以展平SimpleToken.sol
文件:
truffle-flattener contracts/SimpleToken.sol > FlattenedSimpleToken.sol
truffle-flattener
outputs the flattened contract into the standard output or the terminal. Using the >
operator we save the output in the FlattenedSimpleToken.sol
file inside the current folder.
truffle-flattener
器将展平合约输出到标准输出或终端中。 使用>
运算符,我们将输出保存在当前文件夹内的FlattenedSimpleToken.sol
文件中。
使用Remix IDE编译和部署合同 (Compiling and Deploying the Contract Using Remix IDE)
You can access your flattened smart contract from the FlattenedSimpleToken.sol
file. Open the file and copy its content.
您可以从FlattenedSimpleToken.sol
文件访问展平的智能合约。 打开文件并复制其内容。
Next, open Remix IDE from https://remix.ethereum.org and paste the flattened smart contract into a new file in the IDE.
接下来,从https://remix.ethereum.org打开Remix IDE,然后将展平的智能合约粘贴到IDE中的新文件中。
If you get an error saying Mock compiler : Source not found, make sure to select a newer compiler version from Settings tab > Solidity version pane > select new compiler version dropdown.
如果出现错误提示“ 模拟编译器:找不到源” ,请确保从“设置”选项卡>“实体版本”窗格中选择一个较新的编译器版本,然后选择“新编译器版本”下拉列表 。
If Auto compile is disabled, click on the Start to compile button in the Compile panel.
如果禁用了自动编译 ,请在“ 编译”面板中单击“ 开始编译”按钮。
Next, activate the Run panel. First make sure you have JavaScript VM selected for environment. On the second box, select the SimpleToken contract from the drop-down, then click on the red Deploy button, right below the drop-down.
接下来,激活“ 运行”面板。 首先,请确保已为环境选择JavaScript VM 。 在第二个框中,从下拉菜单中选择SimpleToken合同,然后单击下拉菜单正下方的红色Deploy按钮。
Your contract is deployed and running on the JavaScript VM, which simulates a blockchain. We can now start debugging it in different ways.
您的合同已部署并在模拟区块链JavaScript VM上运行。 现在,我们可以用不同的方式开始调试它。
调试自定义令牌合同 (Debugging the Custom Token Contract)
To see how we can debug the contract, we’ll first introduce some errors.
为了了解如何调试合同,我们将首先介绍一些错误。
You can start debugging smart contracts in Remix IDE using two different ways, depending on the use case.
您可以根据使用情况使用两种不同的方式在Remix IDE中开始调试智能合约。
When you first deploy your smart contract or perform any transaction afterwards, some information will be logged in the terminal and you can start debugging it from the white Debug button next to the log. Let’s see that in action.
首次部署智能合约或之后执行任何交易时,一些信息将记录在终端中,您可以从日志旁边的白色“ 调试”按钮开始对其进行调试 。 让我们看看实际情况。
使用assert() (Using assert())
SafeMath is an OpenZeppelin library for Math operations with safety checks that throw an error. The sub(a,b)
function subtracts two numbers and returns an unsigned number. The first parameter should be greater than the second parameter so we can always get a positive number. The function enforces this rule using an assert() function. This is the code for the sub function:
SafeMath是用于数学运算的OpenZeppelin库,具有引发错误的安全检查。 sub(a,b)
函数将两个数字相减并返回一个无符号数字。 第一个参数应大于第二个参数,以便我们始终可以获得正数。 该函数使用assert()函数强制执行此规则。 这是子函数的代码:
function sub(uint256 a, uint256 b) internal pure returns (uint256) {
assert(b <= a);
return a - b;
}
As long as you provide values for a and b that verify the condition b <= a, the execution continues without problems, but once you provide a value of b greater that a, the assert() function will throw an error that says:
只要提供a和b的值来验证条件b <= a ,执行就不会出现问题,但是一旦您提供的b值大于a ,则assert()函数将抛出错误:
VM error: invalid opcode. invalid opcode The execution might have thrown. Debug the transaction to get more information.
So let’s add a call to the sub() with wrong parameters in the SimpleToken contract:
因此,让我们在SimpleToken合同中使用错误的参数添加对sub()的调用:
constructor() public {
totalSupply_ = INITIAL_SUPPLY;
balances[owner] = INITIAL_SUPPLY;
SafeMath.sub(10, 1000);
}
If you redeploy the contract, you’re going to get the the invalid opcode error in the terminal:
如果重新部署合同,您将在终端中看到无效的操作码错误:
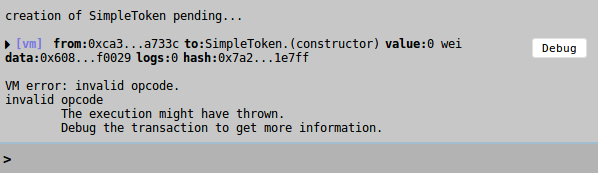
Once you click the Debug button you’ll be taken to the Debugger panel where you can start debugging your code.
单击“ 调试”按钮后,将转到“ 调试器”面板,您可以在其中开始调试代码。
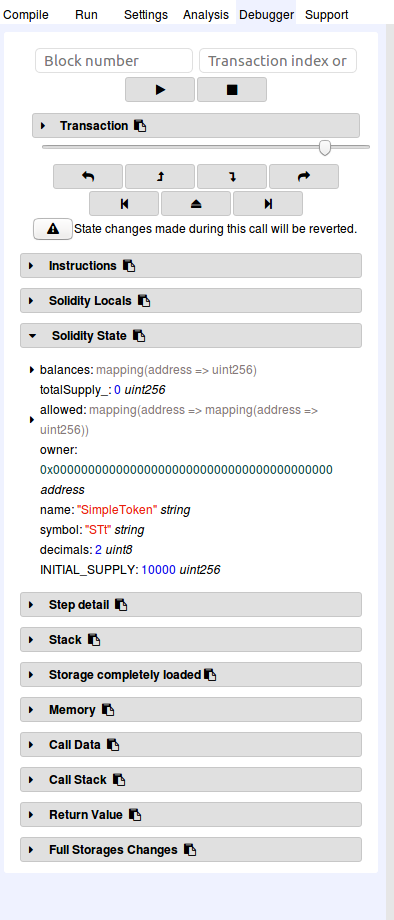
In the code editor, the first line/instruction will be highlighted marking where we are currently at the code.
在代码编辑器中,第一行/指令将突出显示,以标记我们当前在代码中的位置。
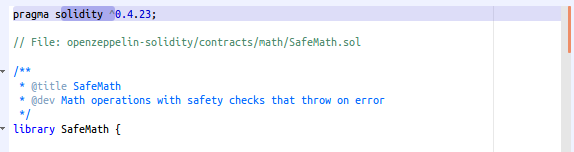
Click on the Step over forward button to step through the code.
单击逐步前进按钮以逐步浏览代码。
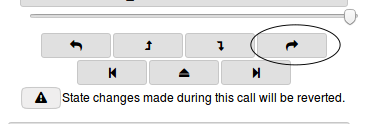
You can also jump right in the exception using the Jump to exception button.
您也可以使用“ 跳转到例外”按钮在例外中直接跳转 。
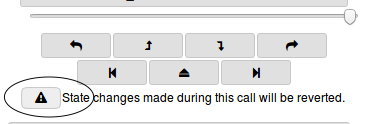
Whatever way you’re using, the debugger will take you to the code causing the problem, and will then stop.
无论使用哪种方式,调试器都会带您到导致问题的代码,然后停止。

You can inspect the state of the local variables at the current step.
您可以在当前步骤检查局部变量的状态。
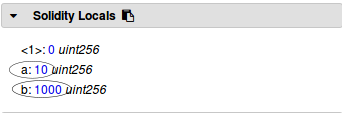
The Solidity Locals panel displays local variables associated with the current context.
“ Solidity Locals”面板显示与当前上下文关联的局部变量。
From the source code and Solidity Locals, you can conclude that the source of the problem is related to the assert() method and the value of b being greater than the value of a.
从源代码和密实度当地人 ,可以得出这样的结论问题的源极相关的断言()方法和B是更大的比a的值的值。
You can stop debugging using the stop debugging button.
您可以使用“ 停止调试”按钮停止调试 。
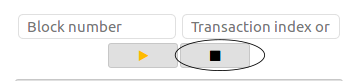
It’s also worth looking at the other panels of the debugger.
同样值得一看的是调试器的其他面板。
使用说明 (Instructions)
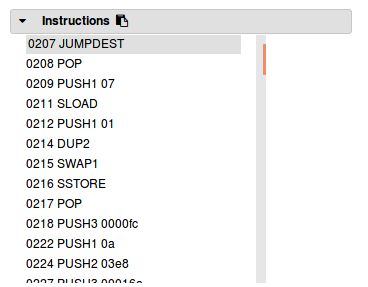
The Instructions panel displays the byte-code of the debugged contract. The byte-code of the current step is highlighted.
“ 指令”面板显示调试合约的字节码。 当前步骤的字节码突出显示。
固结状态 (Solidity State)
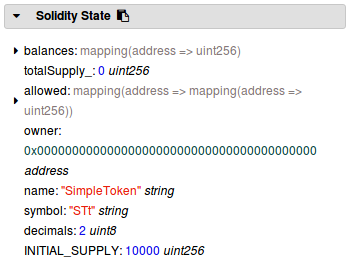
The Solidity State panel displays state variables of the currently debugged contract.
Solidity State面板显示当前调试合同的状态变量。
低层面板 (Low Level Panels)
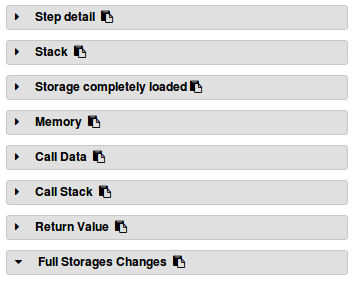
These panels display low level information about the execution such as step details, memory, stack and return value of functions.
这些面板显示有关执行的低级信息,例如步骤详细信息,内存,堆栈和函数的返回值。
结论 (Conclusion)
In this tutorial, we’ve used Truffle and OpenZeppelin to build a simple token, then used truffle-flattener to flatten the custom contract and Remix IDE to start debugging the contract for errors.
在本教程中,我们使用Truffle和OpenZeppelin构建了一个简单的令牌,然后使用truffle-flattener扩展了自定义合同,并使用Remix IDE开始调试该合同中的错误。
Hopefully this will help you fearlessly dive into step-by-step debugging of your own contracts. Let us know how it works out for you!
希望这将帮助您无所畏惧地逐步调试自己的合同。 让我们知道如何为您服务!
翻译自: https://www.sitepoint.com/flattening-contracts-debugging-remix/
remix使用
remix使用_使用Remix展平合同和调试相关推荐
- Python计算两个numpy数组的交集(Intersection)实战:两个输入数组的交集并排序、获取交集元素及其索引、如果输入数组不是一维的,它们将被展平(flatten),然后计算交集
Python计算两个numpy数组的交集(Intersection)实战:两个输入数组的交集并排序.获取交集元素及其索引.如果输入数组不是一维的,它们将被展平(flatten),然后计算交集 目录
- pytorch Flatten展平
经过测试,cpu模式中,用Module的方式比直接在推理中展平平均快1~5ms from torch import nn import torch import mathclass Flatten(M ...
- sql 数组三 展平数组
展平数组 如需将 ARRAY 转换为一组行(即执行展平操作),请使用 UNNEST 运算符.UNNEST 获取一个 ARRAY,然后返回一个表,ARRAY 中的每个元素均占该表的一行. 由于 UNNE ...
- flatMap() :对每个元素执行映射函数并将结果展平
JavaScript Array flatMap() 方法介绍 flat() 方法创建一个新数组,其中连接了子数组的元素. map() 方法创建一个新数组,其元素是映射函数的结果. flatMap() ...
- Maya角色UV展平技巧笔记
Maya角色UV展平技巧笔记 一 展平uv注意事项 二 展平uv步骤 1 头部uv展开 2 手臂uv展开 3 手部uv展开 4 身体uv展开 4 腿部uv展开 5 脚部uv展开 三 排布uv壳 参考 ...
- js算法数组flat展平的几种方法
数组的展平,主要是指的是数组嵌套数组转为一维数组,总结了一下几种方法 es6原生的方法 链接: es6中的flat let arr = [1, 2, [3, 4]].flat() console.lo ...
- Python数据处理Tips多层嵌套Json数据展平到DataFrame
在工作中需要处理嵌套数据(尤其是无模式的 MongoDB 日志等)或者是网络爬虫抓取下来的多层嵌套数据需要展平处理. 如果将它放在 BigQuery 中则很容易通过使用 WITHIN 等的查询将其更改 ...
- 《Python数据分析基础教程:NumPy学习指南(第2版)》笔记6:第三章 常用函数2——中位数、方差、日期、展平
本章将介绍NumPy的常用函数.具体来说,我们将以分析历史股价为例,介绍怎样从文件中载入数据,以及怎样使用NumPy的基本数学和统计分析函数.这里还将学习读写文件的方法,并尝试函数式编程和NumPy线 ...
- SolidWorks如何放样锥面展平下料,你该这样做
有的正在上班的也正在使用SolidWorks的小伙伴是不是经常遇到工件展平下料操作,不知道您是怎么样的,方法很多,下面我写一个简单的锥面展平下料实例 1.先画一个锥面零件模型,尺寸你顺便画,如图 曲面 ...
最新文章
- linux ramdisk与tmpfs的深入分析
- kotlin学习笔记——sqlite(anko)
- 这是我在网上安的第一个窝!
- python ev3图形化编程软件下载_mPython(图形化编程软件)
- hive转16进制unhex_Java 进制的转换
- ECCV 2020 论文大盘点-遥感与航空影像处理识别篇
- oracle字符串使用函数,oracle函数大全-字符串处理函数
- 把Python项目打包成exe文件
- 双目测距(五)--匹配算法对比
- SharePoint 2010 中有个新的列表模板“导入电子表格”可以直接导入Excel数据并创建为列表 ....
- Windows Mobile Web方式下载文件
- 【Visual Assist X】VAssistX的安装和使用
- 终极算法 机器学习和人工智能如何重塑世界
- 含耦合电感元件的电路分析
- python方法怎么调用_python函数怎么调用自身?
- ubuntu 16.04软件源
- 关于gitlab报错 would clobber existing tag 的解决办法
- 计量单位报错:消息号BM302 “未使用语言 ZH 创建单位 XXX”
- 使用vue+div+svg实现审批流程图功能,可生成JSON格式
- 鸿蒙系统源代码解析,鸿蒙内核源码分析(系统调用篇) | 图解系统调用全貌
热门文章
- linux 虚拟硬盘释放,虚拟机的磁盘碎片清理
- NVIDIA英伟达jetson xavier nx怎么进入Recovery模式
- 安装SQL Server 2000时“以前的某个程序安装已在安装计算机上创建挂起的文件操作。运行安装程序之前必须重新启动计算机”错误的排除
- 田野调查手记·浮山摩崖石刻(八)
- mysql的cpu使用率突然增高_mysql cpu使用率过高解决方法
- remote: pingfan443: Incorrect username or password (access token) fatal: Authentication failed for
- 转:C++资源之不完全导引
- 小程序抢购页面倒计时定时器
- matlab 与cla的区别clf,何时使用cla(),clf()或close()清除matplotlib中的图?...
- Python 位运算