运算符重载,以及迭代器[foreach]示例
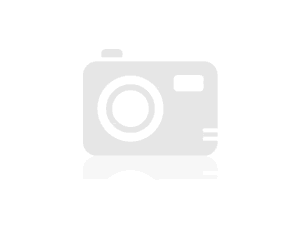
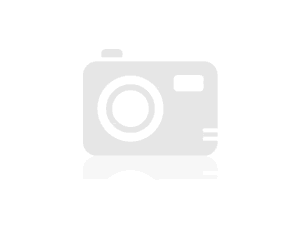
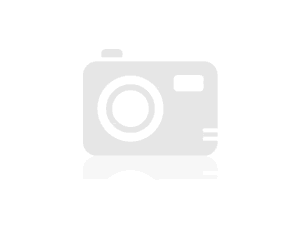
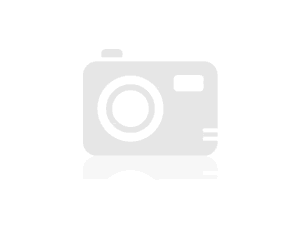
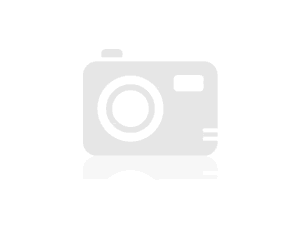
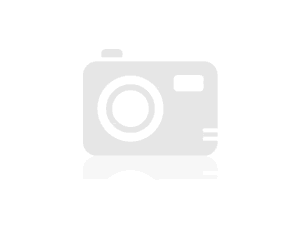
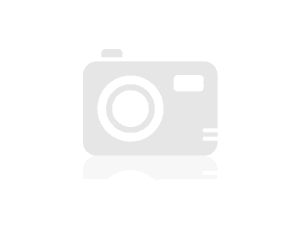
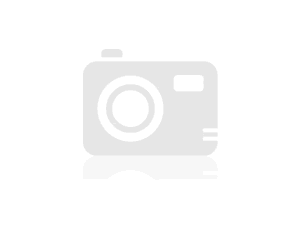



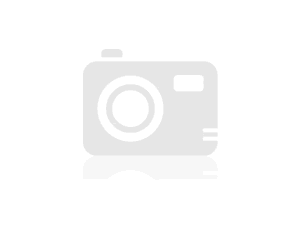



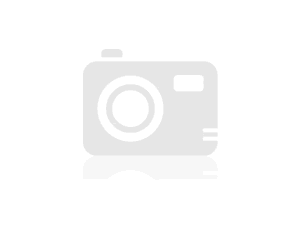
















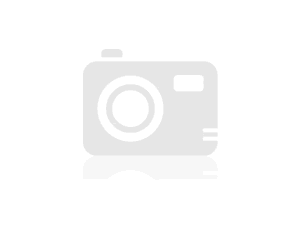








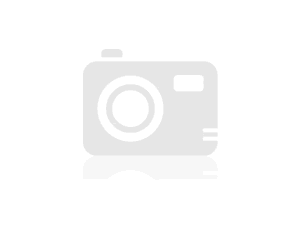




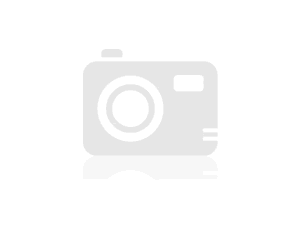





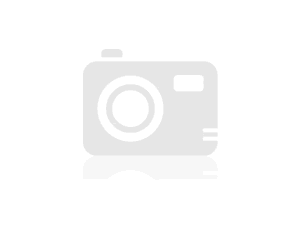









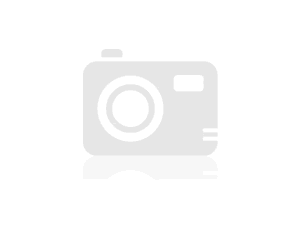





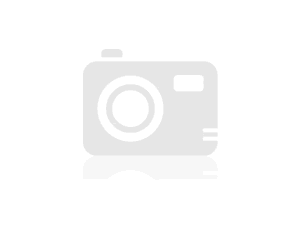







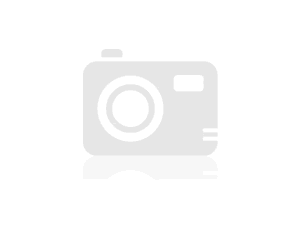





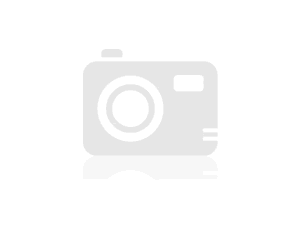






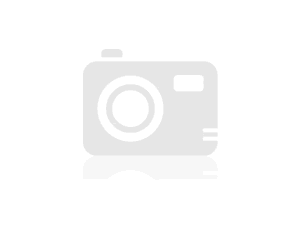







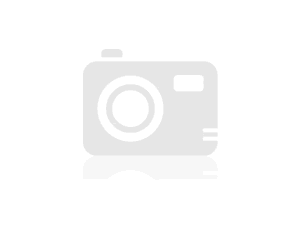






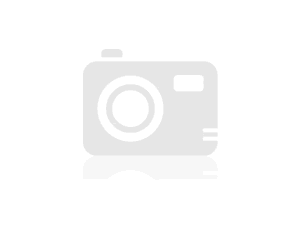



















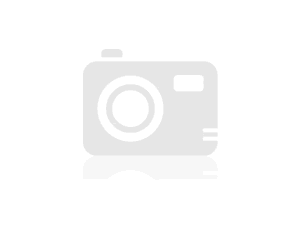






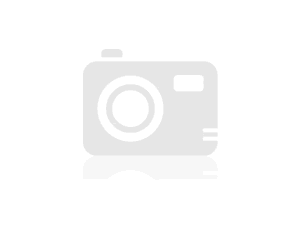



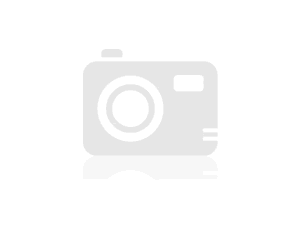



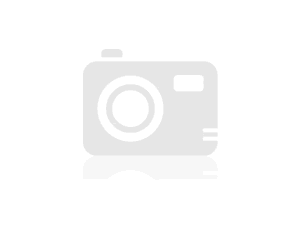














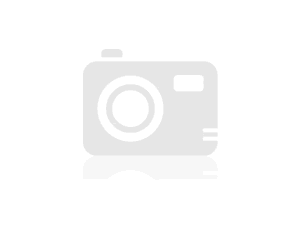



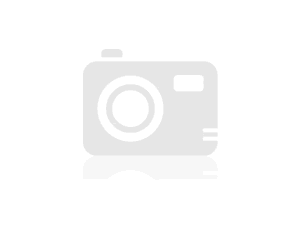


















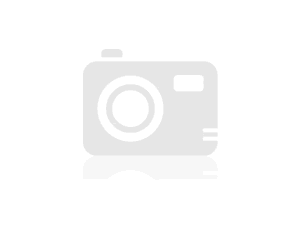













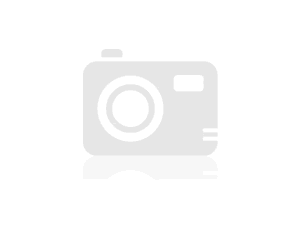







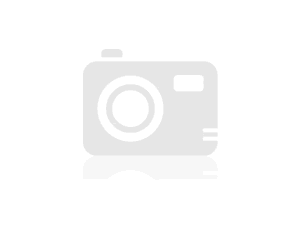










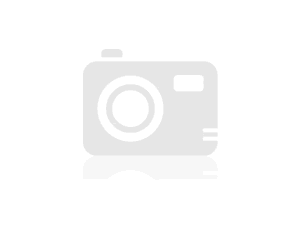







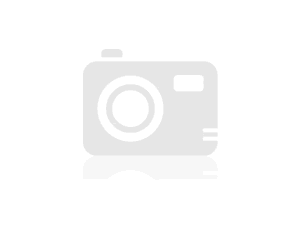






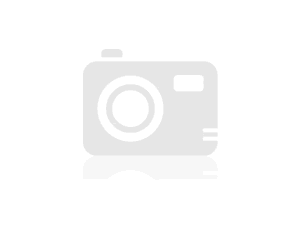







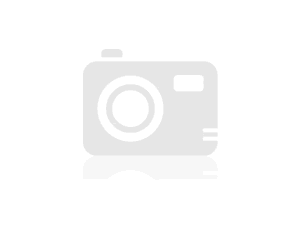









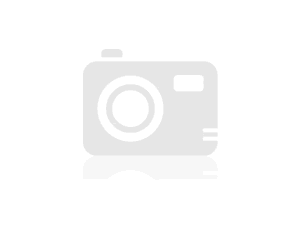







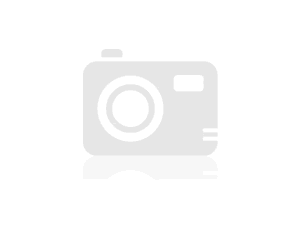





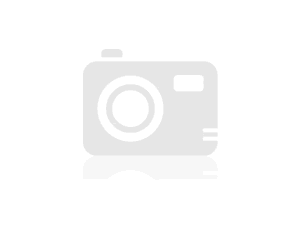







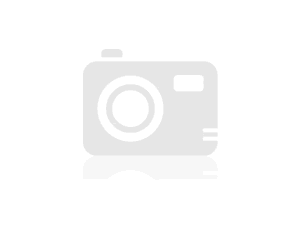





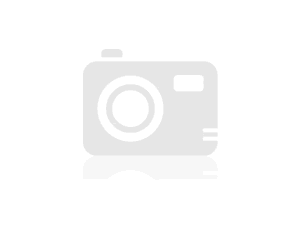







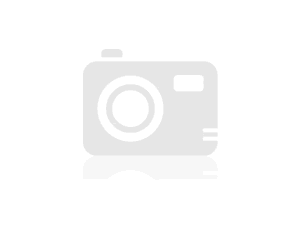





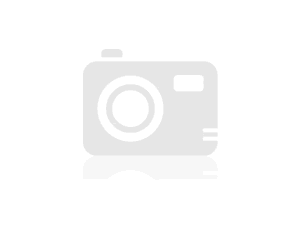





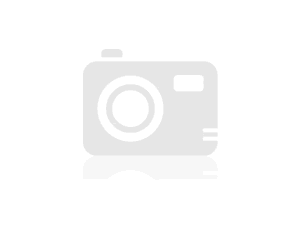





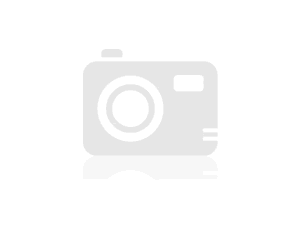






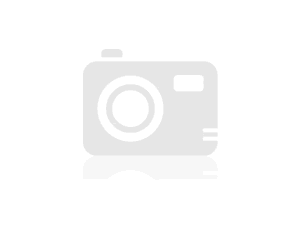





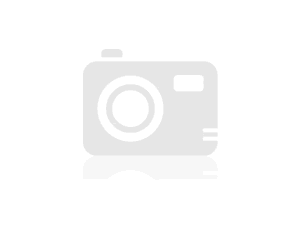





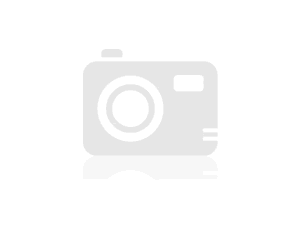




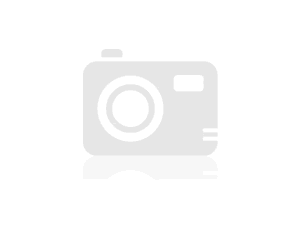




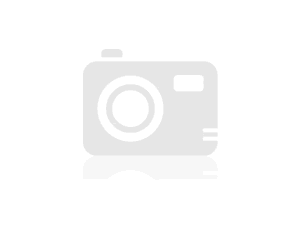







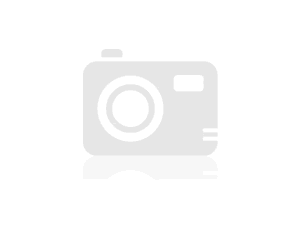






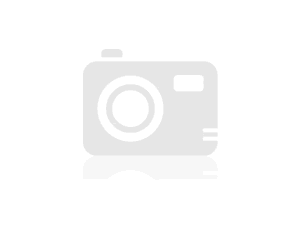







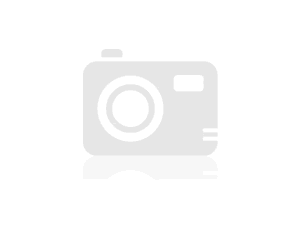







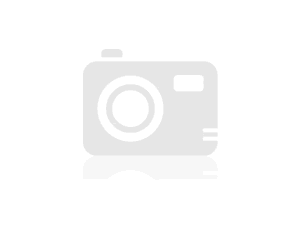



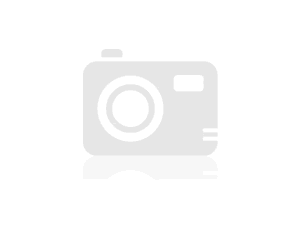











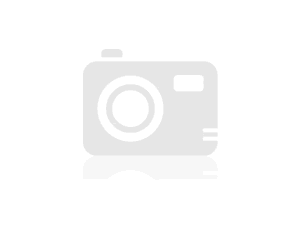






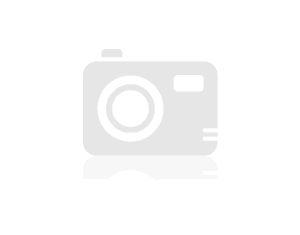
运行结果:
( 1 , 2 , 5 )
1 i + 2 j + 5 k
( 1.000000E+000, 2.000000E+000, 5.000000E+000 )
False
20
1
2
5
转载于:https://www.cnblogs.com/yjmyzz/archive/2007/12/16/996979.html
运算符重载,以及迭代器[foreach]示例相关推荐
- 十个 C++ 运算符重载示例,看完不懂打我...
下面是一些 C++ 运算符重载示例,包括算术运算符.赋值运算符.逻辑运算符.成员运算符.关系运算符等等,这些都是使用频率较高的几个运算符重载案例. ⭐️ 所有示例代码均存放于 GitHub: geti ...
- Python-特殊方法(迭代器,生成器,内建方法,运算符重载)
Python是一门独特的语言,力求简洁,它甚至不像某些语言(如Java)提供接口语法,Python语言采用的是"约定"规则,它提供了大量具有特殊意义的方法,这些方法有些可以直接使用 ...
- 计算时间:一个运算符重载示例
题目 今天早上在Priggs的账户上花费2小时35分钟,下午又花费了2小时40分钟,则总共花了多少时间. mytime0.h #ifndef MYTIME0_H #define MYTIME0_Hcl ...
- delphi 操作符重载_Delphi XE2中的运算符重载示例
delphi 操作符重载 In my programming career I have only very rarely run into situations where operator ove ...
- Java为什么不提供运算符重载?
从C ++到Java,一个显而易见的未解决问题是Java为什么不包括运算符重载? 不是Complex a, b, c; a = b + c;吗Complex a, b, c; a = b + c; C ...
- python 运算符重载_Python3面向对象-运算符重载
1:运算符重载介绍 运算符重载,就是在某个类的方法中,拦截其内置的操作(比如:+,-,*,/,比较,属性访问,等等),使其实例的行为接近内置类型. 当类的实例出现在内置操作中时(比如:两个实例相加 + ...
- 笔记②:牛客校招冲刺集训营---C++工程师(面向对象(友元、运算符重载、继承、多态) -- 内存管理 -- 名称空间、模板(类模板/函数模板) -- STL)
0618 C++工程师 第5章 高频考点与真题精讲 5.1 指针 & 5.2 函数 5.3 面向对象(和5.4.5.5共三次直播课) 5.3.1 - 5.3.11 5.3.12-14 友元 友 ...
- 运算符重载的基本规则和习语是什么?
问: 注意:答案是按特定顺序给出的,但是由于许多用户根据投票而不是给出的时间对答案进行排序,所以这里是一个按最有意义的顺序排列的答案索引: C++中运算符重载的一般语法 C++中运算符重载的三个基本规 ...
- C++中的运算符重载
1.Cpp中的重载运算符和重载函数 C++允许在同一作用域中的某个函数和运算符指定多个定义,分别称为函数重载和运算符重载.重载声明是指一个与之前已经在该作用域内声明过的函数或方法具有相同名称的声明,但 ...
最新文章
- 大学生创业难?现在已不是问题!
- c语言spi测试代码,C语言程序SPI
- Hadoop2.8集群安装详细教程
- CSS:display和visibility隐藏的区别
- opencv图像分析与处理(15)- 图像压缩中的编码方法:霍夫曼编码、Golomb编码、Rice编码、算术编码及其实现
- Atitit mysql insert perf enhance 批量插入数据库性能 目录 1.1. 案一:使用ignore关键字	1 2. 异步插入	2 2.1. 其它关键:DELAYED 做为
- 基于python爬虫数据分析论文_基于Python的招聘网站信息爬取与数据分析
- 成功解决android 网络视频边下载变播放。
- 基于QT(c++)的家庭财务管理系统
- 计算机怎么查询隐藏的字体,Win10怎么隐藏不使用的字体?隐藏字体的方法
- Windows 2003访问https失败
- 英特尔SST音频驱动导致Windows11电脑蓝屏,驱动人生带来电脑蓝屏解决方案
- windows10系统解除微软账户和本地账户绑定
- VS2010调试时出现“0x7556d36f 处最可能的异常: 0x000006BA: RPC 服务器不可用”的解决方法
- 关系型数据库RDBMS
- pc端调试使用微信环境
- 先前的pytorch各个版本的地址
- CAJviewer7.2无法添加进打开方式的列表,在打开方式中“浏览”无法选择
- 计算机基础之 计算机存储单位(比特/位 bit、字节Byte、兆MB...)换算关系
- 目标检测主要数据格式的转换
热门文章
- hive 字段不包含某个字符_hive之面试必问 hive调优
- js变量后面加问号是什么_js没那么简单(1)-- 执行上下文
- JDK1.8中的HashMap,HashTable,ConcurrentHashMap有什么区别?
- 自考护理学计算机考试时间,护理学专业2019年10月江苏自考科目及考试时间安排...
- java中间缓存变量机制_Java中间缓存变量机制
- Axure RP 8.0软件安装教程
- 航空频率表 2020_航空波段+调频、中波、短波,这个美国TR608收音机值40美元吗?...
- python实现api server_使用Python的http.server实现一个简易的Web Api对外提供HanLP拼音转换服务...
- win11什么时候发布的_2021年初级会计师考试大纲什么时候发布?
- 华为云计算之ebackup了解