使用SQL Coalesce函数查询数据
We all know that a Null value is a field with no value. The statements that we are running daily will have to deal with Null values, especially when it comes to strings concatenation (adding strings together).
我们都知道Null值是没有值的字段。 我们每天运行的语句将必须处理Null值,尤其是在涉及字符串连接(将字符串加在一起)时。
Let’s start with the SQL Coalesce definition. The Coalesce SQL function returns first nonnull expression among its arguments. The rule of thumb here is that Null plus anything equals zero. It’s like zero times anything equals zero. For example, the big problem here is when working with things like addresses. To be more specific, it’s a problem when we have to combine e.g. a street name with a postal code, additional address line, etc. So, using the logic from above, if one of those has a Null value, the whole thing is going to be Null.
让我们从SQL Coalesce定义开始。 Coalesce SQL函数返回其参数中的第一个非空表达式。 经验法则是Null加任何东西等于零。 就像零乘以零一样。 例如,这里最大的问题是使用地址之类的东西时。 更具体地说,当我们必须将街道名称与邮政编码,附加地址行等结合使用时,这是一个问题。因此,使用上面的逻辑,如果其中一个具有Null值,那么整个过程就在进行为空。
Now, if you are not so familiar with the SQL Coalesce expression, it’s probably best to get familiar with the Case expression first and its formats because the Coalesce SQL expression is a syntactic shortcut for the Case expression. We will explain this using a simple example later on but feel free to check out the following article for more details on the Case expression: Querying data using the SQL Case statement
现在,如果您不太熟悉SQL Coalesce表达式,则最好先熟悉Case表达式及其格式,因为Coalesce SQL表达式是Case表达式的语法快捷方式。 稍后,我们将使用一个简单的示例对此进行解释,但是请随时查看以下文章以获取有关Case表达式的更多详细信息: 使用SQL Case语句查询数据
If we want to prove the Coalesce SQL definition from above, consider the following example that uses the “AdventureWorks2012” database:
如果要从上面证明Coalesce SQL定义,请考虑以下使用“ AdventureWorks2012”数据库的示例:
SELECT p.Title, p.FirstName, p.MiddleName, p.FirstName+' '+p.LastName AS FirstLastName, COALESCE(p.FirstName+' '+p.MiddleName+' '+p.LastName, p.FirstName+' '+p.LastName, p.FirstName, p.LastName) AS FullName
FROM Person.Person AS p;
Before we run this query, let’s take a look at the columns from Object Explorer. Note that both “FirstName” and “LastName” are not null which means that they cannot be empty:
在运行此查询之前,让我们看一下对象浏览器中的列。 请注意,“ FirstName”和“ LastName”都不为空,这意味着它们不能为空:

For the sake of the example simplicity, execute the script below to nullify the “LastName” column:
为了简单起见,请执行以下脚本以使“ LastName”列无效:
ALTER TABLE Person.Person ALTER COLUMN LastName NVARCHAR(50) NULL;
GO
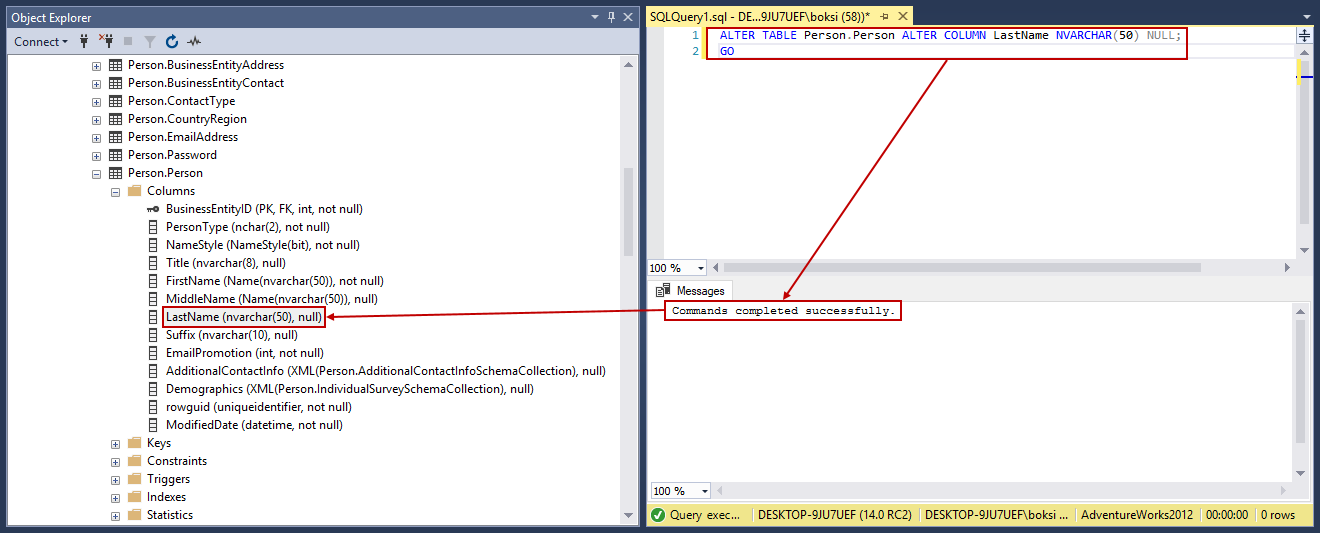
Right-click the “Person” table from Object Explorer and choose the Edit Top 200 Rows command. Change the “LastName” value from “Sánchez” to Null of the very first record:
右键单击“对象资源管理器”中的“人员”表,然后选择“编辑前200行”命令。 将“ LastName”值从“Sánchez”更改为第一条记录的Null:

We just made sure that this record has the first name but not the last name. So, right now if we are to do just first name plus last name part of our initial query the result will be Null:
我们只是确保该记录具有名字而不是姓氏。 因此,现在,如果我们只执行初始查询的名字和姓氏部分,结果将为Null:
SELECT p.Title, p.FirstName, p.MiddleName, p.FirstName+' '+p.LastName AS FirstLastName
FROM Person.Person AS p;
Here is the result:
结果如下:
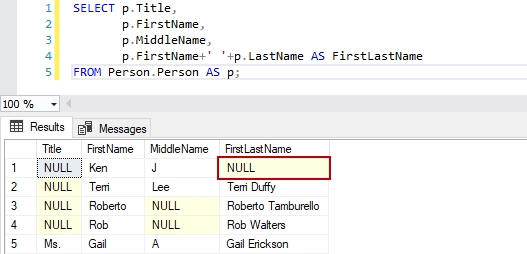
As we mentioned at the beginning, this is a problem. But, if we run the query with the SQL Server Coalesce expression, by the Coalesce SQL definition it will return the first non-Null value:
正如我们一开始提到的,这是一个问题。 但是,如果我们使用SQL Server Coalesce表达式运行查询,则根据Coalesce SQL定义,它将返回第一个非null值:
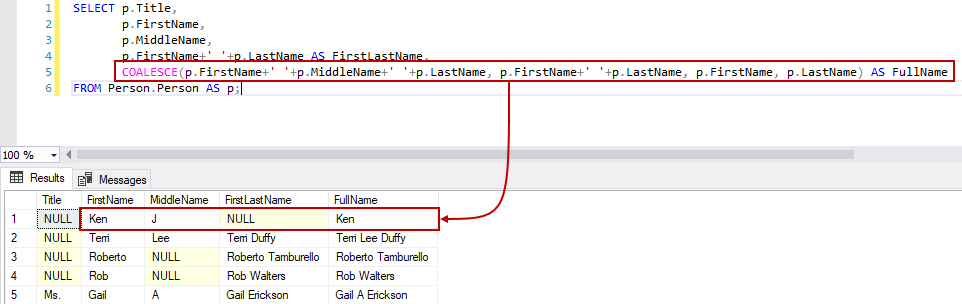
As it can be seen from the query, we can do an expression one, comma, expression two, etc. We are basically saying add them all together using the SQL Coalesce expressions:
从查询中可以看出,我们可以执行表达式1,逗号,表达式2等。我们基本上是说要使用SQL Coalesce表达式将它们全部加在一起:
- First name, middle name, last name – if any of those is Null, go to the next one 名,中间名,姓氏 -如果其中任何一个为Null,则转到下一个
- First name, last name – same rules apply as above as well for below 名,姓 –上面和下面的规则相同
- First name – this is where we hit the Coalesce and return the first non-Null value (Ken) 名字 –这是我们击中Coalesce并返回第一个非null值(肯)的地方
- Last name – the last one will not get hit 姓氏 -姓氏不会被击中
We can even go an extra mile and say if all of the above is Null (first name, middle name, last name), provide a default value saying “No name”:
我们甚至可以加倍努力,说出上述所有内容是否都为Null(名字,中间名,姓氏),并提供一个默认值“ No name”:
SELECT p.Title, p.FirstName, p.MiddleName, p.FirstName+' '+p.LastName AS FirstLastName, COALESCE(p.FirstName+' '+p.MiddleName+' '+p.LastName, p.FirstName+' '+p.LastName, p.FirstName, p.LastName, 'No name') AS FullName
FROM Person.Person AS p;
And get something like this if the first, middle, and last name are Null:
如果名字,中间名和姓氏为Null,则得到如下所示的内容:
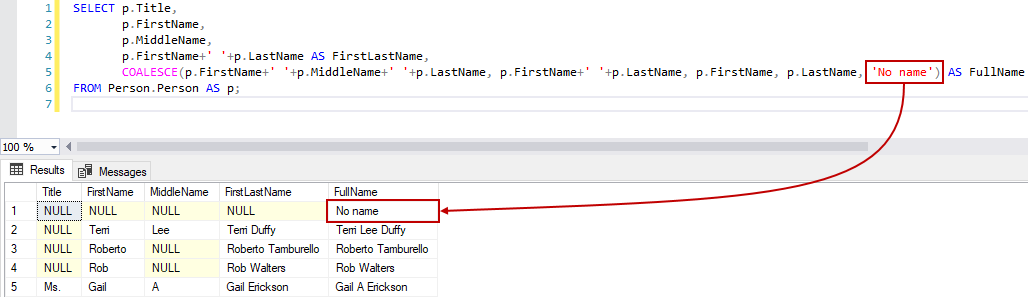
To wrap things up, hopefully you’ve seen how handy SQL Coalesce can be when you have to combine many fields and when some of them are Null. You can play with the output and basically see how it would look like if they are all there, what it would look like if a couple of them are there, and you can go all the way down the list to make all the possible combinations including the case when all of the fields have Null value and ensure that the result/output will not be a Null using the SQL Coalesce function.
总结一下,希望您已经了解了当必须合并多个字段并且其中一些字段为Null时SQL Coalesce可以多么方便。 您可以使用输出,基本上可以看到它们都在那里时的样子,如果其中有几个就可以看到它的样子,您可以一直沿列表向下进行以进行所有可能的组合,包括所有字段均具有Null值并使用SQL Coalesce函数确保结果/输出不会为Null的情况。
Furthermore, note that the SQL Server Coalesce expression and IsNull function have a similar purpose but behave differently. SQL Coalesce can be used on anything that can return a Null value and not just characters as shown in this article, but number too, etc. The IsNull function has only two parameters, where SQL Coalesce has X parameters and it can go for as long as you can keep adding expressions.
此外,请注意,SQL Server Coalesce表达式和IsNull函数具有相似的用途,但行为不同。 SQL Coalesce可以用于可以返回Null值的任何对象,不仅可以返回本文中显示的字符,还可以返回数字,等等。IsNull函数只有两个参数,其中SQL Coalesce具有X参数,并且可以使用很长时间因为您可以继续添加表达式。
I hope this has been informative for you and you have learned something about the Coalesce SQL function. Thanks for reading.
我希望这对您有所帮助,并且您已了解有关Coalesce SQL函数的知识。 谢谢阅读。
翻译自: https://www.sqlshack.com/querying-data-using-the-sql-coalesce-function/
使用SQL Coalesce函数查询数据相关推荐
- 在SQL Server中使用SQL Coalesce函数
This article explores the string manipulation using SQL Coalesce function in SQL Server. 本文探讨了在SQL S ...
- SQL COALESCE函数和NULL
目录 什么是COALESCE? 比较SQL的COALESCE和CASE 在处理NULL时,知道何时使用SQL COALESCE函数是一个救生员. 如您所知,NULL是一个棘手的概念,似乎NULL在表达 ...
- 云小课|使用SQL加密函数实现数据列的加解密
摘要:数据加密作为有效防止未授权访问和防护数据泄露的技术,在各种信息系统中广泛使用.作为信息系统的核心,GaussDB(DWS)数仓也提供数据加密功能,包括透明加密和使用SQL函数加密. 本文分享自华 ...
- 使用hibernate的this.getSession().createSQLQuery(sql).list();方法查询数据时出现查到的数据和想象的不一致,很是郁闷,诡异...
今天 使用hibernate的this.getSession().createSQLQuery(sql).list();方法查询数据时出现查到的数据和想象的不一致的问题,郁闷我很长一段时间 执行的方法 ...
- SQL COALESCE 函数
SQL COALESCE 函数 https://docs.oracle.com/cd/B28359_01/server.111/b28286/functions023.htm#SQLRF00617 C ...
- sql coalesce()函数、datalength()函数介绍及应用
SQL常用的日期格式转换方法 Posted by 欧阳振华 on 2008-10-3 8:46:20 select CONVERT(varchar(12) , getdate(), 101 ) 09/ ...
- SQL Server(三)-查询数据(2)
--函数与分组查询数据 (一) 系统函数 在SQL Server 2008中系统函数是指在SQL Server 2008中自带的函数,主要分为聚合函数.数据类型转换函数.日期函数.数学函数及其他一些常 ...
- 使用 SQL 加密函数实现数据列的加解密
数据加密作为有效防止未授权访问和防护数据泄露的技术,在各种信息系统中广泛使用.作为信息系统的核心,GaussDB (DWS) 数仓也提供数据加密功能,包括透明加密和使用 SQL 函数加密.这里主要讨论 ...
- C#连接SQL Server并查询数据
用C#连接本地SQL Server 查询信息 using System; using System.Collections.Generic; using System.Linq; using Syst ...
最新文章
- java正则替换标点
- matplotlib.pyplot绘制函数图像希腊字母latex效果设置
- 设计模式之_动态代理_06
- java学到什么程度才有用处_如何自学Java?Java学到什么程度才可以找工作?
- linux git 发邮件,gitlab发邮件基于sendmail
- 京东月薪8万招聘HR,岗位要求只有这3个字
- oracle基础教程(第四天)数据库管理
- 北京飞马贸易借沟通CTBS实现总部与分公司同步做帐
- vue axios封装
- oracle所有自带系统表,oracle常用系统表
- 程序员是做什么的?未来计算机变得智能,就不需要程序员了吗?
- 神经网络:Epoch、Batch Size和迭代
- 来自资深会员管理人的深度思考
- NF-ResNet:去掉BN归一化,值得细读的网络信号分析 | ICLR 2021
- 主板有电无法启动_电脑主板有电 但是就是开不了机
- 微信对账单接口返回值解析
- IO操作中flush()方法作用
- python之购物车(详解list tupe 循环)
- 【人工智能+区块链项目Cortex首尔举办韩国首场线下活动】
- CSDN文章markdown图片居中以及调整大小(超级简单)
热门文章
- 三、Springmvc之Controller层方法返回值
- Linux 系统的IP与域名解析文件[局域网的DNS]
- ACM 竞赛高校联盟 练习赛 第六场 韩梅梅的抽象画(图论水题)
- 根据指定的commit查找对应的log
- Comet杀人游戏开发日志-1(问题记录-于核心功能测试成功转向实际开发阶段)
- 【零基础学Java】—对象的向上和向下转型(二十七)
- 【Vue2.0】—表单事件数据绑定(六)
- 谷歌浏览器安装Postman插件 亲测有效!!!
- 前端—每天5道面试题(十一)
- access数据库窗体设计实验报告_来自窗体控件的数值条件(VBA)