sql动态sql给变量复值_在动态SQL中使用变量
sql动态sql给变量复值
Before we delve into these SQL concepts, note that I like to do all my development in SQL Management Studio. So if you want to follow along go ahead and open that up.
在深入研究这些SQL概念之前,请注意,我喜欢在SQL Management Studio中进行所有开发。 因此,如果您想继续前进,请打开它。
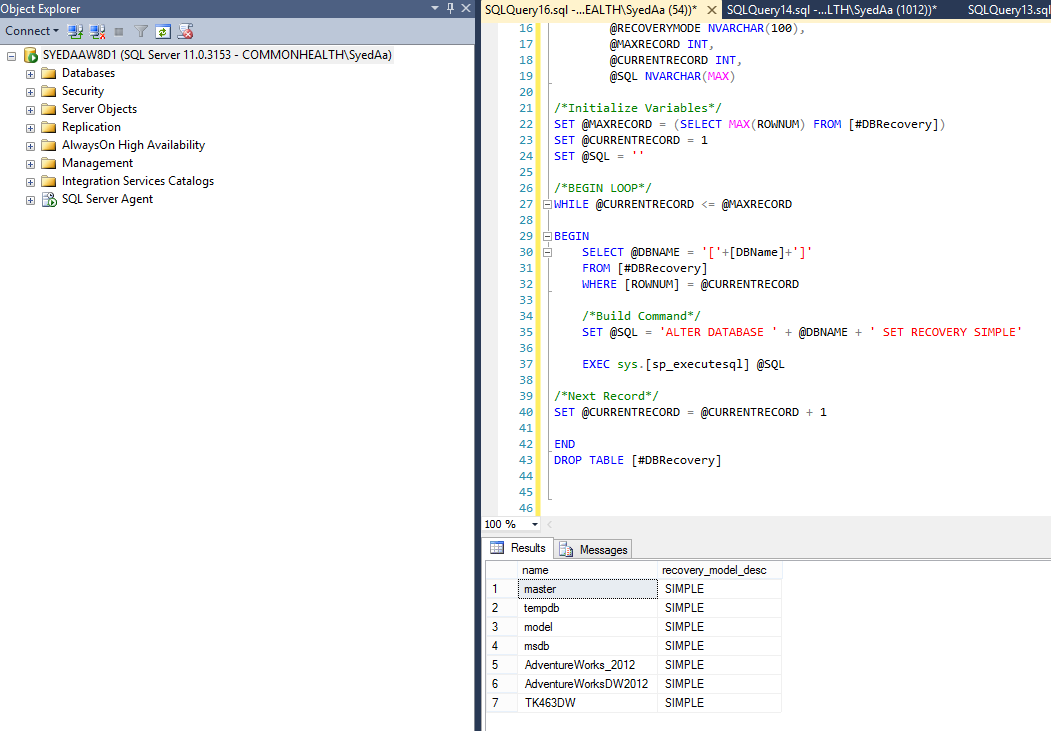
变数 (Variables)
Variables are extremely useful in SQL scripts. They offer the flexibility needed to create powerful tools for yourself. They are especially useful in dynamic SQL environments. They can, and are often used as counters for loops to control how many times you want the code inside the loop to run. A statement can use it to test the value of the data, or a stored procedure can use it to return a value.
变量在SQL脚本中非常有用。 它们提供了为自己创建强大工具所需的灵活性。 它们在动态SQL环境中特别有用。 它们可以并且通常用作循环的计数器,以控制您希望循环中的代码运行多少次。 语句可以使用它来测试数据的值,或者存储过程可以使用它来返回值。
We can use the DECLARE statement to declare one or more variables. From there we can utilize the SET command to initialize or assign a value to the variable. Here is a quick example:
我们可以使用DECLARE语句来声明一个或多个变量。 从那里我们可以利用SET命令来初始化变量或为变量赋值。 这是一个简单的示例:
DECLARE @MAXRECORD INT
You can declare multiple variables in the same DECLARE statement just separate them with a comma. Example would be:
您可以在同一DECLARE语句中声明多个变量,只需用逗号将它们分开即可。 例如:
/*Declare Variables*/
DECLARE @DBNAME NVARCHAR(100),@RECOVERYMODE NVARCHAR(100),@MAXRECORD INT,@CURRENTRECORD INT,@SQL NVARCHAR(MAX)
That seems simple enough.
这似乎很简单。
We have declared a variable called @maxrecord as an INT data type. Now we can use the SET operator to assign it a value. Like this:
我们已将名为@maxrecord的变量声明为INT数据类型。 现在,我们可以使用SET运算符为其分配一个值。 像这样:
SET @MAXRECORD = 10
We just assigned the INT variable @maxrecord with a value of 10. This can change throughout a SQL script, thus providing great flexibility.
我们刚刚为INT变量@maxrecord分配了值10。这可以在整个SQL脚本中更改,从而提供了极大的灵活性。
Think of a variable as a box that acts as a place holder for whatever value you want to put in it.
可以将变量想像成一个盒子,充当要放置在其中的任何值的占位符。

The SET statement can only be used on variable at a time. This can become cumbersome if you need to assign values to multiple attributes. But you do need to use multiple set statements.
SET语句一次只能在变量上使用。 如果您需要为多个属性分配值,则可能会变得很麻烦。 但是您确实需要使用多个set语句。
SET @MAXRECORD = (SELECT MAX(ROWNUM) FROM [#DBRecovery])
SET @CURRENTRECORD = 1
SET @SQL = ''
However you can use a select statement to make this process a little easier. (Largely depending on what you’re trying to accomplish). Here is a quick example of assigning values to variables in a select statement.
但是,您可以使用select语句使此过程更容易一些。 (很大程度上取决于您要完成的工作)。 这是在select语句中为变量分配值的快速示例。
SELECT @DBNAME = '['+[DBName]+']'
FROM [#DBRecovery]
WHERE [ROWNUM] = @CURRENTRECORD
动态SQL (Dynamic SQL)
I often think of Dynamic SQL as “code that thinks”. Although it’s not quite cognitive, it can be used to handle a lot of tasks that would likely be repetitive or have a high administrative overhead. The concept of Dynamic SQL is one that allows you to build a SQL statement or a full batch of SQL code and present it as a character string. Then you can take this string and execute it. SQL Server gives us a couple of options to execute character strings as T-SQL Code. One is via the simple EXECUTE command (I like to use “EXEC”) or the sp_executesql stored procedure.
我经常将动态SQL视为“思考的代码”。 尽管不是很容易理解,但是它可以用于处理很多可能是重复的或高管理开销的任务。 动态SQL的概念使您可以构建一条SQL语句或一整批SQL代码并将其显示为字符串。 然后,您可以使用此字符串并执行它。 SQL Server为我们提供了两种将字符串作为T-SQL代码执行的选项。 一种是通过简单的EXECUTE命令(我喜欢使用“ EXEC”)或sp_executesql存储过程。
So, again why would we ever want to use Dynamic SQL? One reason would be the automation of administrative tasks. Let’s say you have a non-production server with every limited space and you want to make sure that all the databases on your server are in simple recovery mode (to minimize the growth of the transaction logs). Rather than manually query the server to identify the databases that meet this parameter and then manually set them to the proper recovery model you can write a piece of code to execute periodically. This code can identify the databases that aren’t compliant with your set rules and make then make the change for you. Thus reducing your administrative overhead. This will save you time and allow you to focus on meeting real deadlines, projects and such.
那么,为什么我们还要使用动态SQL? 原因之一是管理任务的自动化。 假设您有一台具有有限空间的非生产服务器,并且您想确保服务器上的所有数据库都处于简单恢复模式(以最大程度地减少事务日志的增长)。 您可以编写一段代码来定期执行,而不是手动查询服务器以识别满足此参数的数据库,然后将它们手动设置为正确的恢复模型。 该代码可以识别不符合您设置的规则的数据库,然后为您进行更改。 从而减少您的管理开销。 这将节省您的时间,并使您可以专注于满足实际的截止日期,项目等。
了解EXEC命令 (Understanding the EXEC Command)
The EXEC command was around before the more robust sp_executesql stored procedure. Rather than placing Unicode strings into one of the parameters, you simply place it in parenthesis after exec.
在更强大的sp_executesql存储过程之前,存在EXEC命令。 无需将Unicode字符串放入参数之一,只需将其放在exec后面的括号中即可。
EXEC (sqlstatement)
EXEC(sqlstatement)
You can even place the piece of code into a variable and put the variable in parenthesis. (Note that EXEC allows the use of both regular character strings and Unicode character strings as input).
您甚至可以将一段代码放入一个变量中,并将该变量放在括号中。 (请注意,EXEC允许使用常规字符串和Unicode字符串作为输入)。
了解sp_executesql存储过程 (Understanding the sp_executesql Stored Procedure)
This is a great built-in stored procedure for SQL Server. It allows you to use input and output parameters allowing your dynamic SQL code to be secure and efficient. The Parameters not only serve a purpose for flexibility, but they also inhibit SQL Injection attacks since they appear as operands and not part of the actual code.
这是SQL Server的出色内置存储过程。 它允许您使用输入和输出参数,从而使动态SQL代码安全有效。 参数不仅起到灵活性的作用,而且还抑制SQL注入攻击,因为它们显示为操作数,而不是实际代码的一部分。
Also note that as long as the SQL statement itself remains the same, yet only the parameters change, the query optimizer will utilize the same cached execution plan.
还要注意,只要SQL语句本身保持不变,但是只有参数更改,查询优化器才会使用相同的缓存执行计划。
Let’s use the example above regarding databases and simple recovery model. I’m not going to write out the entire script for you here as it is beyond the scope of the article. But the snippets I provide should provide enough information to show how to use this method.
让我们使用上面有关数据库和简单恢复模型的示例。 我不会在这里为您写出整个脚本,因为这超出了本文的范围。 但是,我提供的摘录应提供足够的信息以显示如何使用此方法。
My goal is to find out if any databases are not in the simple recovery model. If they are, I want them to be put into the compliant mode.
我的目标是找出是否有任何数据库不在简单恢复模型中。 如果是这样,我希望将它们置于兼容模式下。
/*Insert Databases names into Temp Table*/
BEGIN TRY
DROP TABLE #DBRecovery
END TRY
BEGIN CATCH SELECT 1 END CATCHSELECT ROWNUM = ROW_NUMBER() OVER (ORDER BY sys.[databases]),DBName = [name],RecoveryModel = [recovery_model_desc]
INTO #DBRecovery
FROM sys.[databases]
WHERE [recovery_model_desc] NOT IN ('Simple')/*Declare Variables*/
DECLARE @DBNAME NVARCHAR(100),@RECOVERYMODE NVARCHAR(100),@MAXRECORD INT,@CURRENTRECORD INT,@SQL NVARCHAR(MAX)/*Initialize Variables*/
SET @MAXRECORD = (SELECT MAX(ROWNUM) FROM [#DBRecovery])
SET @CURRENTRECORD = 1
SET @SQL = ''/*BEGIN LOOP*/
WHILE @CURRENTRECORD <= @MAXRECORDBEGINSELECT @DBNAME = '['+[DBName]+']'FROM [#DBRecovery]WHERE [ROWNUM] = @CURRENTRECORD/*Build Command*/SET @SQL = 'ALTER DATABASE ' + @DBNAME + ' SET RECOVERY SIMPLE'EXEC sys.[sp_executesql] @SQL/*Next Record*/
SET @CURRENTRECORD = @CURRENTRECORD + 1END
DROP TABLE [#DBRecovery]
I like to take the criteria and place the databases that need to be altered into a temp table.
我喜欢采用标准并将需要更改的数据库放入临时表中。
SELECT ROWNUM = ROW_NUMBER() OVER (ORDER BY sys.[databases]),DBName = [name],RecoveryModel = [recovery_model_desc]
INTO #DBRecovery
FROM sys.[databases]
WHERE [recovery_model_desc] NOT IN ('Simple')
As you can see, I am using variables as they play an important role in creating dynamic SQL.
如您所见,我正在使用变量,因为它们在创建动态SQL中起着重要的作用。
DECLARE @DBNAME NVARCHAR(100),@RECOVERYMODE NVARCHAR(100),@MAXRECORD INT,@CURRENTRECORD INT,@SQL NVARCHAR(MAX)
I use SET to assign values to a few of the variables. In this case, I want the @maxrecord variable to know how many records in total we must loop through. The @currentrecord acts as our counter. Once @currentrecord is equal the @maxrecord the loop will end.
我使用SET将值分配给一些变量。 在这种情况下,我希望@maxrecord变量知道总共必须循环通过多少条记录。 @currentrecord充当我们的计数器。 一旦@currentrecord等于@maxrecord,循环将结束。
/*Initialize Variables*/
SET @MAXRECORD = (SELECT MAX(ROWNUM) FROM [#DBRecovery])
SET @CURRENTRECORD = 1
SET @SQL = ''
I use the @SQL variable to store the actual code that will execute. In this case, it is a simple command to change the recovery model of the database. Remember we already identified which databases are not in simple mode when we created the temp table.
我使用@SQL变量存储将执行的实际代码。 在这种情况下,更改数据库的恢复模型是一个简单的命令。 记住,我们在创建临时表时已经确定了哪些数据库不在简单模式下。
/*Build Command*/SET @SQL = 'ALTER DATABASE ' + @DBNAME + ' SET RECOVERY SIMPLE'
Then finally, I use the sp_executesql stored procedure to execute the command that is stored in the @SQL variable.
最后,我使用sp_executesql存储过程来执行存储在@SQL变量中的命令。
EXEC sys.[sp_executesql] @SQL
As the loop moves onto the next record in the table the @DBNAME will store the name of a different database, thus having the command execute on a different database. This is an example of SQL code acting dynamically.
当循环移至表中的下一个记录时,@ DBNAME将存储其他数据库的名称,从而使命令在其他数据库上执行。 这是动态执行SQL代码的示例。
Another way to visualize a loop:
可视化循环的另一种方法:

翻译自: https://www.sqlshack.com/using-variables-in-dynamic-sql/
sql动态sql给变量复值
sql动态sql给变量复值_在动态SQL中使用变量相关推荐
- python变量的理解_如何理解Python中的变量
在本篇文章里小编给大家分享的是关于Python中变量是什么意思的相关基础知识点,需要的朋友们可以学习下. 变量 在Python中,存储一个数据,需要定义一个变量 number1 = 1 #numbe1 ...
- 交换两个变量的值,不使用第三个变量的四种法方
交换两个变量的值,不使用第三个变量的四种法方 通常我们的做法是(尤其是在学习阶段):定义一个新的变量,借助它完成交换.代码如下: int a,b; a=10; b=15; int t; t=a; a= ...
- mysql绑定变量的值_关于绑定变量的SQL绑定什么值
当然这不是判断在哪里建立索引的依据. SELECT * FROM (SELECT A.*, SC.NAME, SC.POSITION AS "绑定变量在SQL语句中的位置", CA ...
- plsql怎么查看存储过程中long变量的值_面试官:详细说下基本数据类型与装箱拆箱的过程...
基本数据类型,有存放在栈中的,也有存放堆中的,这取决去基本类型声明的位置. 一:在方法中声明的变量,即该变量是局部变量,每当程序调用方法时,系统都会为该方法建立一个方法栈,其所在方法中声明的变量就放在 ...
- php比较两个变量的值_总结PHP不用第三个变量交换两个变量的值的几种方法
"PHP不用第三个变量交换两个变量的值"这个题看到过好多次了,看来面试确实喜欢考这道题.今天,对于这个题目,我自己总结了几种方法,可能不全,大家来互相补充. 有些仅适用于字符串,方 ...
- python中 是什么类型_浅谈python中的变量默认是什么类型
浅谈python中的变量默认是什么类型 1.type(变量名),输出的结果就是变量的类型: 例如 >>> type(6) 2.在Python里面变量在声明时,不需要指定变量的类型,变 ...
- python定义私有变量的方法_浅谈Python中的私有变量
私有变量表示方法 在变量前加上两个下划线的是私有变量.class Teacher(): def __init__(self,name,level): self.__name=name self.__l ...
- python私有变量什么意思_浅谈Python中的私有变量
私有变量表示方法 在变量前加上两个下划线的是私有变量. class Teacher(): def __init__(self,name,level): self.__name=name self.__ ...
- python中数据类型大小_详细解析Python中的变量的数据类型
变量是只不过保留的内存位置用来存储值.这意味着,当创建一个变量,那么它在内存中保留一些空间. 根据一个变量的数据类型,解释器分配内存,并决定如何可以被存储在所保留的内存中.因此,通过分配不同的数据类型 ...
最新文章
- 《机器学习与数据科学(基于R的统计学习方法)》——2.11 R中的SQL等价表述...
- kali linux安装ftp服务,CentOS7安装和配置FTP
- 一次线上生产问题的全面复盘 【定位-分析-解决】
- 比OCR更强大的PPT图片一键转文档重建技术
- clientHeight、offsetHeight 和 scrollHeight
- 令人惋惜的天才新秀:16岁上剑桥大学,27岁就出名,数学事业一路畅通无阻,但自从结婚后,人生从此翻天覆地······
- vaadin教程_Vaadin教程
- [Xcode 实际操作]五、使用表格-(11)调整UITableView的单元格顺序
- Container(容器)与 Injector(注入)
- 从Mixin到hooks,谈谈对React16.7.0-alpha中即将引入的hooks的理解
- 当对象转换成JSON的时候处理时间格式
- CF1110E Magic Stones(构造题)
- 【优化求解】基于matlab粒子群算法求解函数极值问题【含Matlab源码 1202期】
- linux下载tar包和rpm包以及镜像的地址分享一下
- 数据分析入门之2012美国大选政治献金项目
- springboot使用logback
- 微信小程序画布实现个人签名,并保存为图片
- 作为一名iOS开发者—面对音视频这个新风口应该怎样学习才能乘风而起?
- latex 大于小于大于等于小于等于
- Pycharm导入tabula模块包