在WooCommerce中设置最低结账要求
Chances are that you want to set some kind of minimum requirements on your WooCommerce store before your customers checkout. What follows is a guide on how to set these requirements and restrictions, without needing to use any plugins at all:
您可能希望在客户结帐之前在WooCommerce商店上设置某种最低要求。 以下是无需设置任何插件即可如何设置这些要求和限制的指南:
- Setting A Minimum Weight Requirement Per Order设置每个订单的最小重量要求
- Setting A Minimum Number Of Products Required Per Order设置每个订单所需的最小产品数量
- Setting A Minimum Quantity Per Product设置每个产品的最小数量
- Setting A Minimum Dollar Amount Per Order设置每笔订单的最低金额
本文使用的方法 (Methods Used In This Article)
There is always more than one way of setting minimum requirements in WooCommerce; the results might even be identical. But I consider the methods described below to be the right (or better) way of doing it. Any suggestions on how you accomplish these tasks or further improvements are always welcome and received well.
在WooCommerce中,总是存在不止一种设置最低要求的方法。 结果甚至可能是相同的。 但我认为以下所述的方法是正确的(或更好的)方法。 始终欢迎并收到有关如何完成这些任务或进一步改进的任何建议。
The following code has been tested in the latest versions available for WordPress (3.9.1) and WooCommerce (2.1.12). We’ll be using the dummy data provided for WooCommerce when you install the plugin. The code should go in your theme’s functions.php file and is heavily commented so it’s easier to follow and/or modify if needed.
以下代码已经过WordPress(3.9.1)和WooCommerce(2.1.12)可用的最新版本的测试。 安装插件时,我们将使用为WooCommerce提供的虚拟数据。 该代码应该放在主题的functions.php文件中,并带有大量注释,因此如果需要,可以更轻松地遵循和/或修改。
We are going to be using the woocommerce_check_cart_items
action provided by WooCommerce to run our functions and execute our code. Visit the following link for a complete list of WooCommerce actions and filters, also known as hooks.
我们将使用WooCommerce提供的woocommerce_check_cart_items
操作来运行我们的函数并执行我们的代码。 请访问以下链接,获取WooCommerce操作和过滤器 (也称为钩子)的完整列表。
设置每个订单的最小重量要求 (Setting A Minimum Weight Requirement Per Order)
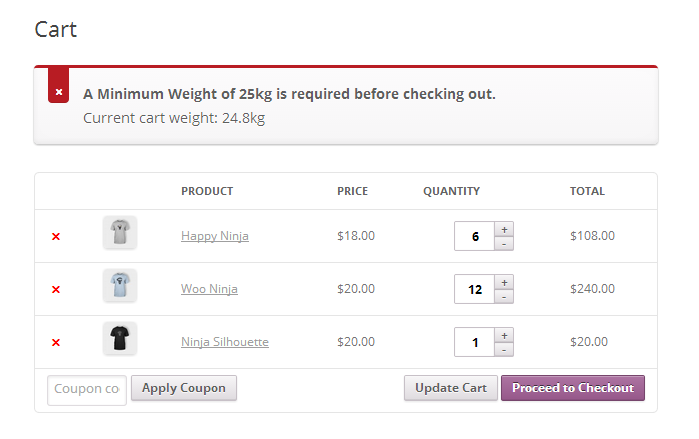
It is often useful to restrict your customer(s) from completing the checkout process without having met a minimum weight requirement. Minium weight requirements can help make your shipping costs more manageable, and the shipping process more streamlined. Don’t forget to change the minimum weight requirement to whatever works best for you, and keep in mind that weight is calculated in whatever weight unit you have set under WooCommerce -> Settings -> Products.
在不满足最低重量要求的情况下,限制客户完成结帐流程通常很有用。 最低重量要求可以帮助您简化运输成本,简化运输流程。 不要忘记将最小重量要求更改为最适合您的重量,并记住,重量是根据您在WooCommerce->设置->产品下设置的任何重量单位计算的。
// Set a minimum weight requirement before checking out
add_action( 'woocommerce_check_cart_items', 'spyr_set_weight_requirements' );
function spyr_set_weight_requirements() {
// Only run in the Cart or Checkout pages
if( is_cart() || is_checkout() ) {
global $woocommerce;
// Set the minimum weight before checking out
$minimum_weight = 25;
// Get the Cart's content total weight
$cart_contents_weight = WC()->cart->cart_contents_weight;
// Compare values and add an error is Cart's total weight
// happens to be less than the minimum required before checking out.
// Will display a message along the lines of
// A Minimum Weight of 25kg is required before checking out. (Cont. below)
// Current cart weight: 12.5kg
if( $cart_contents_weight < $minimum_weight ) {
// Display our error message
wc_add_notice( sprintf('<strong>A Minimum Weight of %s%s is required before checking out.</strong>'
. '<br />Current cart weight: %s%s',
$minimum_weight,
get_option( 'woocommerce_weight_unit' ),
$cart_contents_weight,
get_option( 'woocommerce_weight_unit' ),
get_permalink( wc_get_page_id( 'shop' ) )
),
'error' );
}
}
}
设置每个订单所需的最小产品数量 (Setting A Minimum Number Of Products Required Per Order)
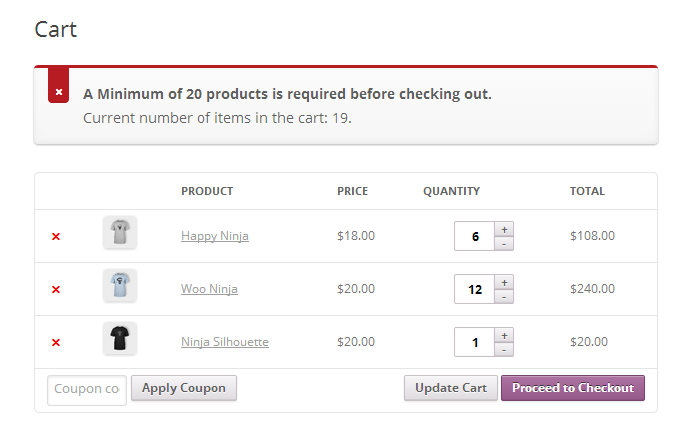
Another valid scenario is setting a minimum number of products that have to be ordered at a time before allowing the customer to fully pay for his order and shipped. Change ’20’ to whatever works best for your needs. As in the previous example, you want to make sure that you only run this code on the cart and checkout pages. This is we use is_cart() and is_checkout(), which return ‘true’ whenever we are on those two specific pages. Learn more about WooCommerce conditionals Tags.
另一个有效的方案是设置一次必须订购的最小数量的产品,然后才能让客户全额支付其订单并发货。 将“ 20”更改为最适合您的需求。 与前面的示例一样,您要确保仅在购物车和结帐页面上运行此代码。 这是因为我们使用is_cart()和is_checkout(),只要我们在这两个特定页面上,它们都将返回“ true”。 了解有关WooCommerce条件标签的更多信息。
// Set a minimum number of products requirement before checking out
add_action( 'woocommerce_check_cart_items', 'spyr_set_min_num_products' );
function spyr_set_min_num_products() {
// Only run in the Cart or Checkout pages
if( is_cart() || is_checkout() ) {
global $woocommerce;
// Set the minimum number of products before checking out
$minimum_num_products = 20;
// Get the Cart's total number of products
$cart_num_products = WC()->cart->cart_contents_count;
// Compare values and add an error is Cart's total number of products
// happens to be less than the minimum required before checking out.
// Will display a message along the lines of
// A Minimum of 20 products is required before checking out. (Cont. below)
// Current number of items in the cart: 6
if( $cart_num_products < $minimum_num_products ) {
// Display our error message
wc_add_notice( sprintf( '<strong>A Minimum of %s products is required before checking out.</strong>'
. '<br />Current number of items in the cart: %s.',
$minimum_num_products,
$cart_num_products ),
'error' );
}
}
}
设置每个产品的最小数量 (Setting A Minimum Quantity Per Product)
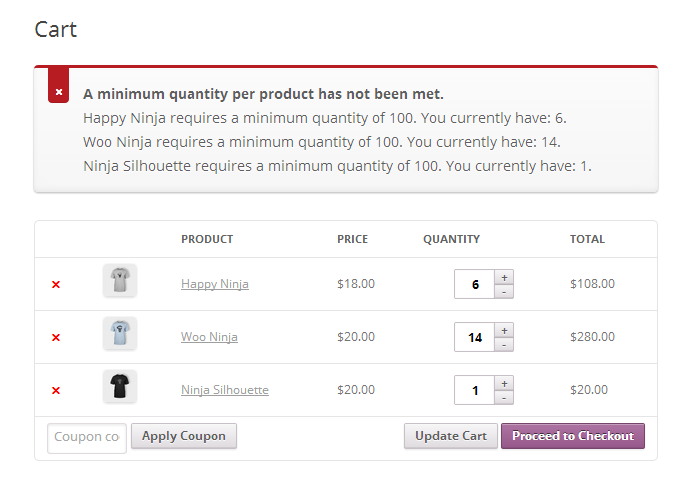
Setting a minimum quantity per product is a common requirement for WooCommerce stores, specially if you are selling wholesale. Setting a minimum quantity will restrict your customer(s) from purchasing a specific product in lesser quantities. For us to check for the minimum quantities, we need to loop through every single product in the cart and check it against our minimum quantity per product requirements set in place.
WooCommerce商店通常会要求为每种产品设置最小数量,特别是如果您批发销售。 设置最小数量将限制您的客户购买数量较少的特定产品。 为了让我们检查最小数量,我们需要遍历购物车中的每个产品,并根据已设置的每个产品的最小数量要求对其进行检查。
To set these restrictions, you need to create an array which holds your rules/restrictions inside another array. Be careful when editing this array, and make sure that all code is entered accurately in order to prevent errors and unexpected results. The format you need to use is as follows:
要设置这些限制,您需要创建一个将规则/限制保存在另一个数组中的数组。 编辑此数组时要小心,并确保正确输入所有代码,以防止出现错误和意外结果。 您需要使用的格式如下:
// Product Id and Min. Quantities per Product
// id = Product ID
// min = Minimum quantity
$product_min_qty = array(
array( 'id' => 47, 'min' => 100 ),
array( 'id' => 37, 'min' => 100 ),
array( 'id' => 34, 'min' => 100 ),
array( 'id' => 31, 'min' => 100 ),
);
Here is where the magic happens.
这就是魔术发生的地方。
// Set minimum quantity per product before checking out
add_action( 'woocommerce_check_cart_items', 'spyr_set_min_qty_per_product' );
function spyr_set_min_qty_per_product() {
// Only run in the Cart or Checkout pages
if( is_cart() || is_checkout() ) {
global $woocommerce;
// Product Id and Min. Quantities per Product
$product_min_qty = array(
array( 'id' => 47, 'min' => 100 ),
array( 'id' => 37, 'min' => 100 ),
array( 'id' => 34, 'min' => 100 ),
array( 'id' => 31, 'min' => 100 ),
);
// Will increment
$i = 0;
// Will hold information about products that have not
// met the minimum order quantity
$bad_products = array();
// Loop through the products in the Cart
foreach( $woocommerce->cart->cart_contents as $product_in_cart ) {
// Loop through our minimum order quantities per product
foreach( $product_min_qty as $product_to_test ) {
// If we can match the product ID to the ID set on the minimum required array
if( $product_to_test['id'] == $product_in_cart['product_id'] ) {
// If the quantity required is less than than the quantity in the cart now
if( $product_in_cart['quantity'] < $product_to_test['min'] ) {
// Get the product ID
$bad_products[$i]['id'] = $product_in_cart['product_id'];
// Get the Product quantity already in the cart for this product
$bad_products[$i]['in_cart'] = $product_in_cart['quantity'];
// Get the minimum required for this product
$bad_products[$i]['min_req'] = $product_to_test['min'];
}
}
}
// Increment $i
$i++;
}
// Time to build our error message to inform the customer
// About the minimum quantity per order.
if( is_array( $bad_products) && count( $bad_products ) > 1 ) {
// Lets begin building our message
$message = '<strong>A minimum quantity per product has not been met.</strong><br />';
foreach( $bad_products as $bad_product ) {
// Append to the current message
$message .= get_the_title( $bad_product['id'] ) .' requires a minimum quantity of '
. $bad_product['min_req']
.'. You currently have: '. $bad_product['in_cart'] .'.<br />';
}
wc_add_notice( $message, 'error' );
}
}
}
设置每笔订单的最低金额 (Setting A Minimum Dollar Amount Per Order)
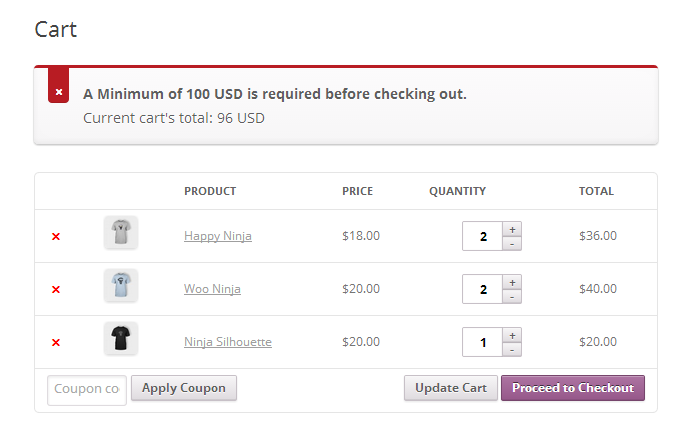
Last but not least, you may want to set a minimum dollar amount that must be spent before your customer is allowed to checkout. Please note that we are calculating the total value using the subtotal, before shipping and taxes are added to the final order total.
最后但并非最不重要的一点是,您可能希望设置在允许客户结帐之前必须花费的最低金额。 请注意,我们将使用小计计算总价值,然后将运费和税金添加到最终订单总额中。
// Set a minimum dollar amount per order
add_action( 'woocommerce_check_cart_items', 'spyr_set_min_total' );
function spyr_set_min_total() {
// Only run in the Cart or Checkout pages
if( is_cart() || is_checkout() ) {
global $woocommerce;
// Set minimum cart total
$minimum_cart_total = 10;
// Total we are going to be using for the Math
// This is before taxes and shipping charges
$total = WC()->cart->subtotal;
// Compare values and add an error is Cart's total
// happens to be less than the minimum required before checking out.
// Will display a message along the lines of
// A Minimum of 10 USD is required before checking out. (Cont. below)
// Current cart total: 6 USD
if( $total <= $minimum_cart_total ) {
// Display our error message
wc_add_notice( sprintf( '<strong>A Minimum of %s %s is required before checking out.</strong>'
.'<br />Current cart\'s total: %s %s',
$minimum_cart_total,
get_option( 'woocommerce_currency'),
$total,
get_option( 'woocommerce_currency') ),
'error' );
}
}
}
结论 (Conclusion)
Are you can see, WooCommerce allows you to use actions and filters to change the normal checkout process. All stores are different and being able to set these restrictions when needed is crucial. For us developers, who need to accomplish tasks like these, knowing how to do it is vital.
可以看到,WooCommerce允许您使用操作和过滤器来更改正常的结帐过程。 所有商店都不尽相同,能够在需要时设置这些限制至关重要。 对于需要完成此类任务的开发人员,知道如何做到这一点至关重要。
Thanks for reading, comments or suggestions on how to improve the code are always welcome. If you have ideas for a specific WooCommerce modification, don’t be shy and post a link to it in the comments for discussion.
感谢您的阅读,欢迎随时提出有关改进代码的意见或建议。 如果您有关于特定WooCommerce修改的想法,请不要害羞,并在评论中发布指向其的链接以进行讨论。
翻译自: https://www.sitepoint.com/minimum-checkout-requirements-in-woocommerce/
在WooCommerce中设置最低结账要求相关推荐
- 如何在WooCommerce中设置运费(免费功能)
这篇WooCommerce教程,我们使用WooCommerce自带的免费的运费配置功能来讲解如何设置运费.基本思路是:1.设置配送区域,2.向这些配送区域添加配送方法,3.在配送方法中添加运费费率.你 ...
- 如何在WooCommerce中创建智能优惠券
Several of our readers have asked us how to create smart coupons in WooCommerce to extend the defaul ...
- TensorFlow中设置学习率的方式
目录 1. 指数衰减 2. 分段常数衰减 3. 自然指数衰减 4. 多项式衰减 5. 倒数衰减 6. 余弦衰减 6.1 标准余弦衰减 6.2 重启余弦衰减 6.3 线性余弦噪声 6.4 噪声余弦衰减 ...
- java按时间范围过滤_按日期范围在WooCommerce中过滤产品
我需要按日期范围搜索WooCommerce中的产品,确切地说是每个月 . 我为使用ACF创建的产品设置了2个自定义字段(acf-season_from和acf-season_to) . 我尝试使用pr ...
- 如何在WooCommerce中添加免费送货栏
Do you offer free shipping on your WooCommerce store? 你们在WooCommerce商店提供免费送货吗? Offering free shippin ...
- Android 中设置线程优先级的正确方式(2种方法)
Android 中设置线程优先级的正确方式(2种方法) 在 Android 中,有两种常见的设置线程优先级的方式: 第一种,使用 Thread 类实例的 setPriority 方法,来设置线程优先级 ...
- 在Dockerfile中设置G1垃圾回收器参数
在Dockerfile中设置G1垃圾回收器参数 ENV JAVA_OPTS="\ -server \ -XX:SurvivorRatio=8 \ -XX:+DisableExplicitGC ...
- idea中设置指向源代码Scala
1.到官网下载scala源代码 点击如下链接下载源码:http://www.scala-lang.org/download/all.html 选择需要的版本点击进行下载,我选择的是2.11.8版本,如 ...
- 转--Android如何在java代码中设置margin
======== 3 在Java代码里设置button的margin(外边距)? 1.获取按钮的LayoutParams LinearLayout.LayoutParams layoutParams ...
最新文章
- Nginx源码分析:master/worker工作流程概述
- 卫星的性能和服务器比较,卫星通信的常用频段的详细对比
- SAP MM ME29N 试图取消审批报错 - Document has already been outputed(function not possible) -
- TCP_Wrappers 基于TCP的安全控制
- BQ24296充电管理芯片使用过程中的注意事项
- Android build.gradle 获取Git 仓库数据
- VirtualBox虚拟机共享剪贴板无效之新解决思路
- 如何在EdrawMax中同时画有箭头和没箭头的直线
- USB驱动分析(二)
- java 苹果cms 萌果_MacCMS8.x(苹果CMS8.x)整合Ckplayer6.4
- Unity3D 官方案例实现类似红警的移动
- Vue3——Suspense组件
- java 中vo、po、dto、bo、pojo、entity、mode如何区分
- shell下删除文件末尾的空行
- 浏览器类应用后台耗电解析 教你三招让手机更省电!
- 立创EDA助力2021全国电赛数百万奖学金!
- 【python】20行代码实现有道翻译api接口调用
- ivm 无法播放 解决
- C++设计:关于CMatrix类的相关操作
- springboot_vue校园二手交易平台java毕业设计
热门文章
- 计算机考研边工作边二战,考研失败,要不要边工作边准备二战?
- 转载 同龄人2016年的一篇博文 用来自励
- 360 电脑重装系统服务器,360!系统重装大师重装完后C盘空间不足解决方法
- 服务器被挖矿重装系统可以吗,电脑被挖矿了需要重装系统_我网上买了一个重装系统U盘重装系统之后电脑里有一个恶意挖矿软件请问把这个卸载了就可以了吗_区块链百科...
- Linq中datetime的处理以及asp.net下拉列表控件的selectitem,text等的设置显示处理
- zookeeper环境搭建中的几个坑[Error contacting service. It is probably not running]的分析及解决
- python调整图表的宽度_Matplotlib表格格式列宽
- 【转】ECharts各种图表文字样式修改大全
- ICT 2017 | 赛迪顾问总裁孙会峰: 信息技术变革开创数字经济的时代
- 三坐标检测之测针标定