最长递增子序列 子串_最长递增子序列
最长递增子序列 子串
Description:
描述:
This is one of the most popular dynamic programming problems often used as building block to solve other problems.
这是最流行的动态编程问题之一,通常用作解决其他问题的基础。
Problem statement:
问题陈述:
Given a sequence A of size N, find the length of the longest increasing subsequence from the given sequence.
给定一个大小为N的序列A ,从给定序列中找到最长的递增子序列的长度 。
The longest increasing subsequence means to find a subsequence of a given sequence where the subsequence's elements are sorted in increasing order, and the subsequence is longest possible. This subsequence is not necessarily contiguous, or unique. Longest increasing subsequence is strictly increasing.
最长的增长子序列意味着找到给定序列的子序列,其中该子序列的元素按升序排序,并且该子序列可能最长。 该子序列不一定是连续的或唯一的。 最长增加的子序列严格增加。
Input:
N=7
Sequence:
{2, 3, 4, 0, 1, 2, 3, 8, 6, 4}
Output:
Length of Longest increasing subsequence is 5
Longest increasing subsequence= {0, 1, 2, 3, 8} or {0, 1, 2, 3, 4}
Explanation with example
举例说明
The possible increasing sub-sequences are,
可能增加的子序列是
Of Length 1 //each element itself is an increasing sequence
长度为1 //每个元素本身都是递增序列



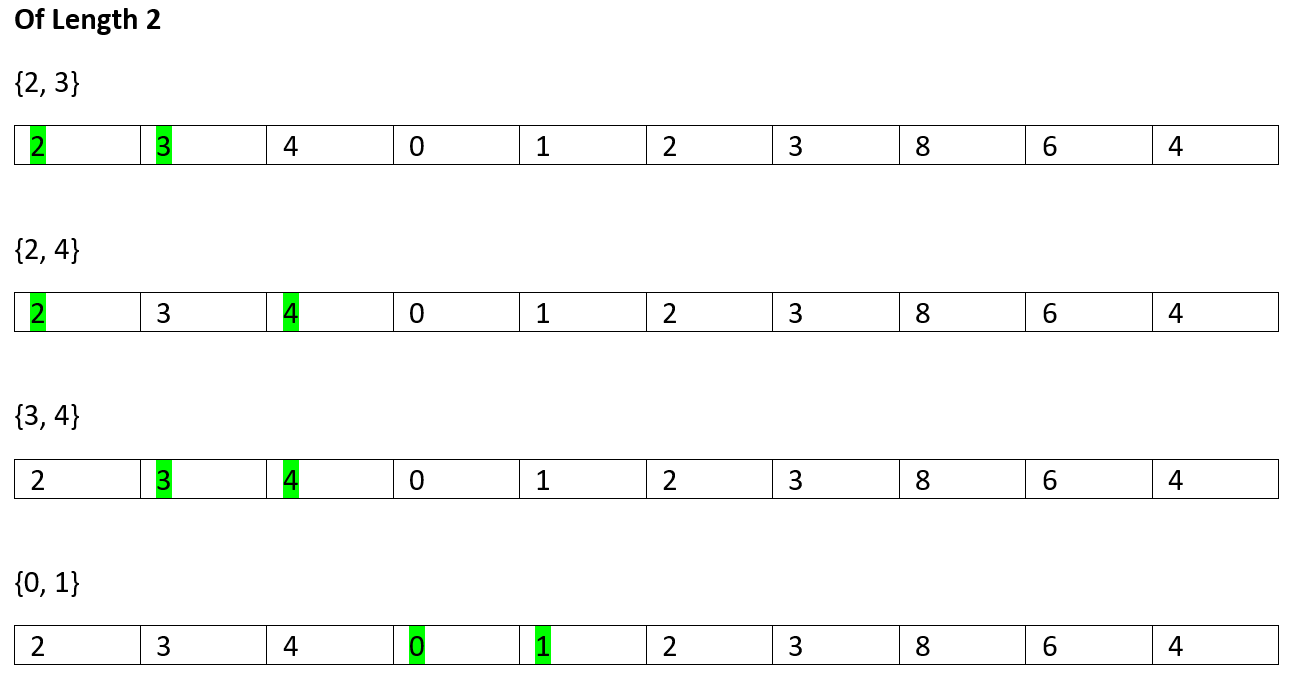

So, on...
所以...


So, on...
所以...

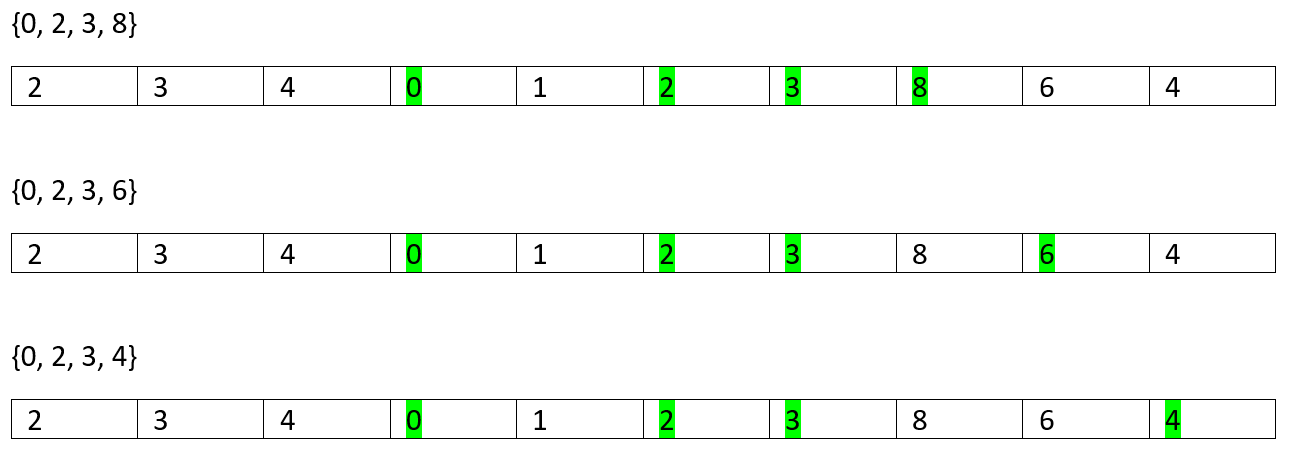
So, on...
所以...

No more
Of Length 6
None
不再
长度6
没有
So, the longest increasing subsequence length is 5.
因此,最长的递增子序列长度是5。
问题解决方法 (Problem Solution Approach)
Of course, in brute-force we can simply generate all increasing sequences and find the longest one. But it would take exponential time which is not a feasible solution. Hence, we choose Dynamic programming to solve.
当然,在蛮力作用下,我们可以简单地生成所有递增的序列并找到最长的序列。 但是,这将花费指数时间,这不是可行的解决方案。 因此,我们选择动态规划来解决。
We create a DP table to store longest increasing subsequence length.
It's intuitive that the minimum value would be 1 as each element represents the primitive sequence which is an increasing one.
我们创建一个DP表来存储最长的递增子序列长度。
直观的是,最小值将为1,因为每个元素代表原始序列,该序列是递增的。
So, the base value is 1.
因此,基准值为1。
Now,
现在,
Lis(i) = longest increasing subsequence starting from index 0 to index i
So,
To compute L(i) the recursion function is,
所以,
为了计算L(i) ,递归函数为

As, the base value is 1, for every index i, L(i) is at least 1.
这样,对于每个索引i ,基值为1, L(i)至少为1。
1) Create the DP array, Lis[n]
2) Initialize the DP array.
for i=0 to n-1
lis[i]=1;
3) Now, to compute the Lis[i]
for index i=1 to n-1
for previous index j=0 to i-1
// if (arr[i],arr[j]) is inceasing sequence
if(lis[i]<lis[j]+1 && a[i]>a[j])
lis[i]=lis[j]+1;
end for
end for
Initially DP table,
最初是DP表,
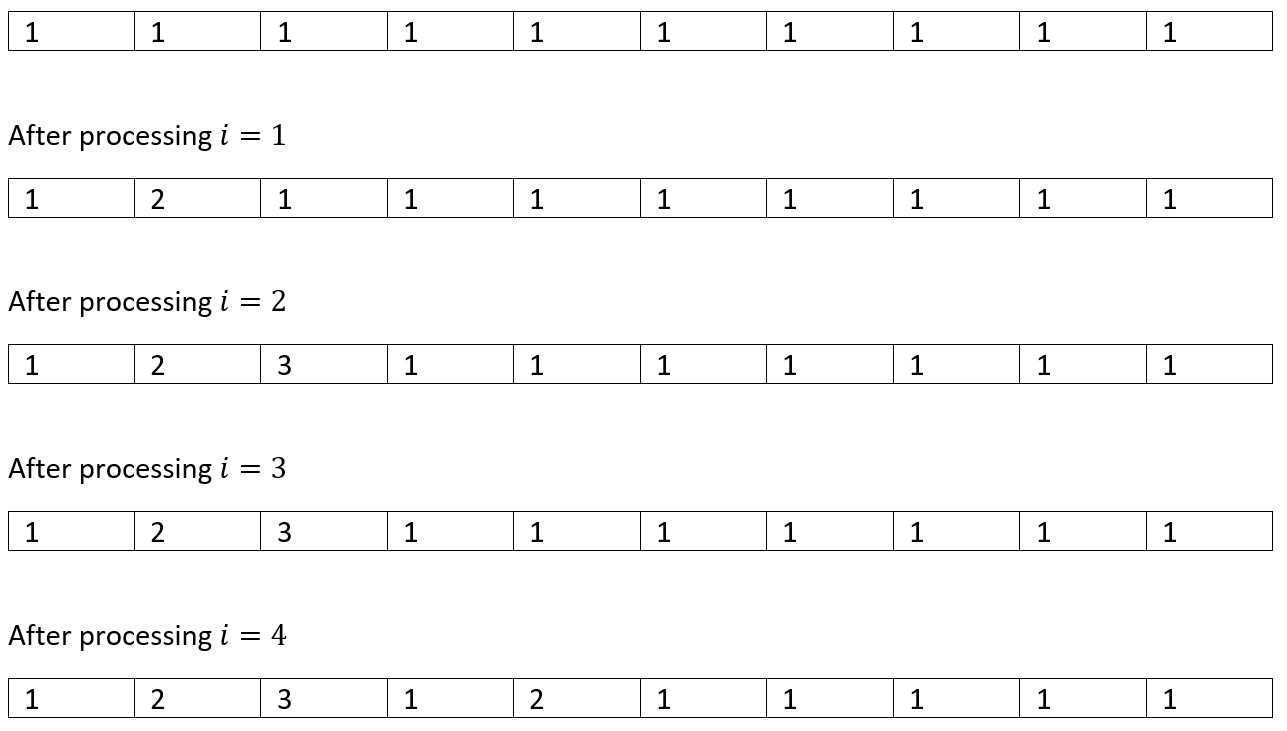
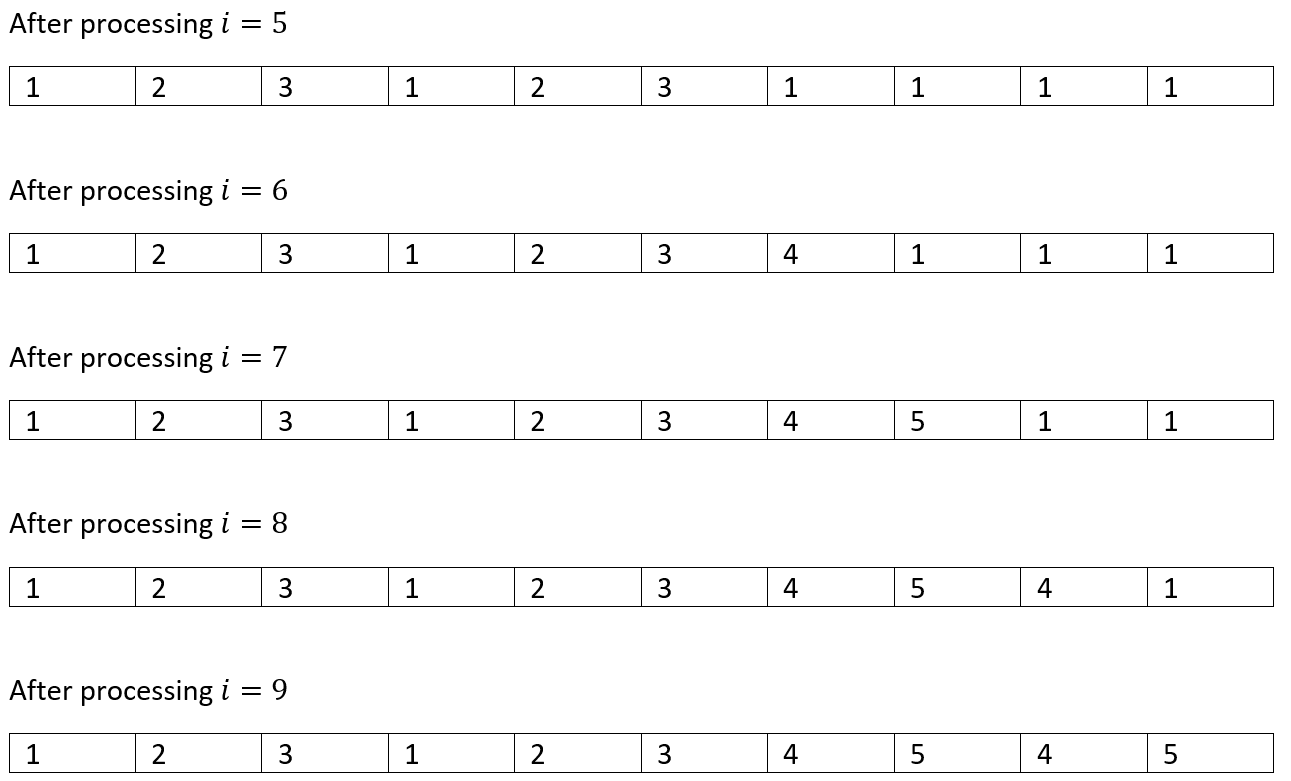
So, the maximum out of this is 5
Hence, LIS=5.
因此,最大数量为5
因此,LIS = 5。
C++ implementation:
C ++实现:
#include <bits/stdc++.h>
using namespace std;
int max(int a, int b)
{if (a > b)
return a;
else
return b;
}
int LIS(vector<int> a, int n)
{int lis[n];
//base case
for (int i = 0; i < n; i++)
lis[i] = 1;
//fill up table
for (int i = 1; i < n; i++) {for (int j = 0; j < i; j++) {if (lis[i] < lis[j] + 1 && a[i] > a[j])
lis[i] = lis[j] + 1;
}
}
//return LIS
return *max_element(lis, lis + n);
}
int main()
{int n, item;
cout << "Sequence size:\n";
scanf("%d", &n);
//input the array
vector<int> a;
cout << "Input sequence:\n";
for (int j = 0; j < n; j++) {scanf("%d", &item);
a.push_back(item);
}
cout << "Length of longest incresing subsequence is: " << LIS(a, n) << endl;
return 0;
}
Output
输出量
Sequence size:
10
Input sequence:
2 3 4 0 1 2 3 8 6 4
Length of longest incresing subsequence is: 5
翻译自: https://www.includehelp.com/icp/longest-increasing-subsequence.aspx
最长递增子序列 子串
最长递增子序列 子串_最长递增子序列相关推荐
- 最长递增子序列 子串_最长递增奇偶子序列
最长递增子序列 子串 Problem statement: 问题陈述: Given a sequence of numbers you have to find out the length of t ...
- 字符串最长回文子串_最长回文子串
字符串最长回文子串 Problem statement: 问题陈述: Given a string str, find the longest palindromic substring. A sub ...
- 数组字符串那些经典算法:最大子序列和,最长递增子序列,最长公共子串,最长公共子序列,字符串编辑距离,最长不重复子串,最长回文子串 (转)...
作者:寒小阳 时间:2013年9月. 出处:http://blog.csdn.net/han_xiaoyang/article/details/11969497. 声明:版权所有,转载请注明出处,谢谢 ...
- 最长回文子串与最长回文子序列
文章目录 最长回文子串与最长回文子序列 最长回文子串 题目描述 dp解法 从中心扩展 马拉车算法 最长回文子序列 题目描述 dp 解法 递归思想 最长回文子串与最长回文子序列 最长回文子串 LeetC ...
- python最大连续递增子列_最长递增子序列(LIS)解法详述
求数组中最长递增子序列(Longest Increasing Subsequence, LIS) LIS问题是算法中的经典题目,传统的解法是使用动态规划,时间复杂度是O(n^2):改进的方法时间复杂度 ...
- 最长回文子串和最长回文子序列
这两个题目我觉得可以放在一起讨论下: 字长回文子序列 首先是回文子序列的题目,涉及到子序列,一般都是不连续的问题: 那么首推动态规划,并且这个题目只要求返回长度,那么dp数组用来存子问题的最长回文串长 ...
- cstring判断是否包含子串_最长子串-滑动窗口
接下来我会找出LeetCode中一些比较有代表性的题,带来它的算法和讲解 很多题目,使用一般的暴力算法很多都能解出来,但时间复杂度可能是 O(n3),会比最优解慢很多,尤其是数据量变大时. 在我们实际 ...
- 最长回文子串_【每日编程142期】最长回文子串II
每日编程中遇到任何疑问.意见.建议请公众号留言或直接撩Q474356284(备注每日编程) 今日问题: 给定一个字符串 s,找到 s 中最长的回文子串.你可以假设 s 的最大长度为1000. 示例 1 ...
- python字符子串_子字符串和子序列(Python),子串,python
# -*- coding=utf-8 -*- ##### 1: 连续子串最大和 ##### def MaxSum(arr): res, s = arr[0], arr[0] for x in arr[ ...
最新文章
- 另类寻找百度文库下载地址
- 2021-03-09 Matlab RBF神经网络及其实例
- 小师妹学JavaIO之:NIO中那些奇怪的Buffer
- 5000字超干货|如何用数据分析驱动用户增长
- linux系统原理是什么意思,Linux系统原理之整体概述
- 面向对象(匿名内部类与有名字内部类的比较)
- 2020快手汽车行业数据价值报告
- 1.14 关于梯度检验实现的注记
- cass参考手册_什么?你还不会测绘?南方CASS教程+视频讲解+插件汇总,快来学!...
- [Serializable]在C#中的作用——实现.NET对象序列化
- Python Tricks(二十)—— 阶乘的极简实现
- 基于Qt5实现的截图工具分享(仿QQ截图功能)
- EasyExcel 背景颜色枚举
- Git学习笔记(二)——Git的分支管理、储藏和标签
- w ndows7与windows10区别,windows7和10区别
- 女朋友说“随便”到底是什么意思?
- Ant design pro入坑指南
- ​Python办公自动化之Excel最全整理
- [leetcode]Majority Element II
- uniapp 图片涂鸦、画笔 Ba-ImagePaint