zephir 开发项目_通过Zephir开发PHP扩展的入门
zephir 开发项目
This tutorial will explain how to create a PHP extension using a new language: Zephir, which is similar to C and Fortran. You can download the full source code from github. We’ve touched on the concept of Zephir before, so if you’re interested in getting a broad overview, see our previous articles.
本教程将说明如何使用新语言Zephir创建PHP扩展,该语言类似于C和Fortran。 您可以从github下载完整的源代码。 之前我们已经讨论过Zephir的概念,因此,如果您有兴趣获得广泛的概述,请参阅我们以前的文章 。
Zephir can be looked at as a hybrid language that lets you write code that looks like PHP, but is then compiled to native C, meaning you can create an extension from it and come away with very efficient code.
Zephir可以被看作是一种混合语言,它使您可以编写类似于PHP的代码,然后将其编译为本机C,这意味着您可以从中创建扩展并获得非常高效的代码。
安装 (Installation)
To build a PHP extension and use Zephir you’ll need the following:
要构建PHP扩展并使用Zephir,您需要满足以下条件:
- gcc >= 4.x/clang >= 3.x/vc++ 9gcc> = 4.x / clang> = 3.x / vc ++ 9
- gnu make 3.81 or latergnu make 3.81或更高版本
- php development headers and toolsphp开发标头和工具
- re2c 0.13 or laterre2c 0.13或更高版本
- json-cjson-c
The installation instructions vary for every platform, so I trust you’ll know how to obtain them if you’re reading an article with a topic as advanced as this one. For the record – I recommend using a Linux based system for developing Zephir apps.
每个平台的安装说明都不尽相同,因此,如果您正在阅读一篇主题与此主题相同的文章,我相信您会知道如何获得它们。 作为记录–我建议使用基于Linux的系统来开发Zephir应用程序。
Once you obtain all the prerequisite software, download the latest version of Zephir from Github, then run the Zephir installer, like so:
获得所有必备软件后,请从Github下载最新版本的Zephir,然后运行Zephir安装程序,如下所示:
git clone https://github.com/phalcon/zephir
cd zephir && ./install -c
It should install automatically – try typing zephir help
. If it is not working, add the “bin” directory to your PATH enviroment variable. In my case: /home/duythien/app/zephir/bin
, like so:
它应该会自动安装-尝试输入zephir help
。 如果不起作用,则将“ bin”目录添加到您的PATH环境变量中。 就我而言: /home/duythien/app/zephir/bin
,如下所示:
vi $HOME/.bash_profile
Append the following export command:
附加以下导出命令:
export PATH=$PATH:/home/duythien/app/zephir/bin
To verify the new path settings and test the installation, enter:
要验证新路径设置并测试安装,请输入:
echo $PATH
zephir help
You can find out about Zephir basics and syntax, as well as its typing system and see some demo scripts over at their website.
您可以找到有关Zephir的基础知识和语法以及其键入系统,并在其网站上查看一些演示脚本。
用Zephir编程 (Programming with Zephir)
Now we’ll use Zephir to re-work a mathematical equation that C and Fortran handle very well. The example is rather esoteric and won’t be explained into much detail, except to demonstrate the power of Zephir.
现在,我们将使用Zephir重做C和Fortran处理得很好的数学方程式。 该示例相当神秘,除了演示Zephir的强大功能之外,将不做详细解释。
时间相关的薛定inger方程的有限差分法求解 (Time-Dependent Schrodinger Equation solved with Finite Difference)
The time-dependent Schrödinger equation can be solved with both implicit (large matrix) and explicit (leapfrog) methods. I’ll use the explicit method.
时间相关的薛定ding方程可以使用隐式(大矩阵)和显式(跳越)方法求解。 我将使用显式方法。
Firstly, issue the following command to create the extension’s skeleton:
首先,发出以下命令来创建扩展的框架:
zephir init myapp
When this command completes, a directory called “myapp” is created on the current working directory. This looks like:
该命令完成后,将在当前工作目录上创建一个名为“ myapp”的目录。 看起来像:
myapp/
|-----ext/
|-----myapp/
|-----config.json
Inside the “myapp” folder, create a file called “quantum.zep” (which will give us the Myapp\Quantum
namespace). Copy paste the following code inside:
在“ myapp”文件夹中,创建一个名为“ quantum.zep”的文件(这将为我们提供Myapp Myapp\Quantum
命名空间)。 复制粘贴以下代码:
namespace Myapp;
class Quantum{
const PI = 3.14159265358979323846;
const MAX = 751;
public function Harmos(double x){
int i,j,n;
var psr, psi, p2, v,paramater,fp;
double dt,dx,k0,item_psr,item_psi;
let dx = 0.02,
k0 = 3.0*Myapp\Quantum::PI,
dt = dx*dx/4.0;
let paramater =[dx,k0,dt,x];
let i = 0,
psr = [],
psi = [],
p2 = [],
v = [],
fp = [];
let fp = fopen ("harmos.txt", "w");
if (!fp) {
return false;
}
while i <= Myapp\Quantum::MAX{
let item_psi = sin(k0*x) / exp(x*x*2.0),
item_psr = cos(k0*x) / exp(x*x*2.0);
let psr[i] = [item_psr],
psi[i] = [item_psi],
v[i] = [5.0*x*x],
x = x + dx,
i++;
}
var tmp;
let i =1, j=1,tmp=[2.0];
for n in range(0, 20000){
for i in range(1,Myapp\Quantum::MAX - 1 ){
let psr[i][3] =psr[i][0] - paramater[2]*(psi[i+1][0] + psi[i - 1][0]
- tmp[0]*psi[i][0]) / (paramater[0]*paramater[0]) + paramater[2]*v[i][0]*psi[i][0],
p2[i] = psr[i][0]*psr[i][4] + psi[i][0]*psi[i][0];
}
for j in range(1,Myapp\Quantum::MAX - 1 ) {
let psr[0][5] = 0,
psr[Myapp\Quantum::MAX][6]= 0 ;
let psi[j][7] = psi[j][0] + paramater[2]*(psr[j+1][8] + psr[j - 1][9]
- tmp[0]*psr[j][10]) / (paramater[0]*paramater[0]) - paramater[2]*v[j][0]*psr[j][11];
}
//output split
if (n ==0 || n % 2000 == 0) {
let i =1;
while i < Myapp\Quantum::MAX - 1 {
fprintf(fp, "%16.8lf %16.8lf %16.8lf \n",i*dx,n*dt,p2[i]);
let i = i + 10;
}
fprintf(fp, "\n");
}
// change new->old
let j = 1;
while j < Myapp\Quantum::MAX - 1 {
let psi[j][0] = psi[j][12],
psr[j][0] = psr[j][13];
let j++;
}
}
return true;
}
}
We’ve used many PHP functions such as fopen(), sin(), fprintf(), etc – feel free to study the syntax. I’ll also give you one more example. In the process of working with the Phalcon PHP framework, the function Phalcon\Tag::friendlyTitle() is invalid if you’re working in Vietnamese or German. This example, far simpler than the equation above, creates the file normalizeChars.zep
. Insert the following code into the file:
我们使用了许多PHP函数,例如fopen(),sin(),fprintf()等–随时学习语法。 我还要再举一个例子。 在使用Phalcon PHP框架的过程中,如果您使用越南语或德语,则函数Phalcon \ Tag :: friendlyTitle()无效。 这个示例比上面的公式简单得多,创建了文件normalizeChars.zep
。 将以下代码插入文件:
namespace Myapp;
class NormalizeChars{
public function trans(var s)
{
var replace;
let replace = [
"ế" : "e",
"ề" : "e",
"ể" : "e",
"ễ" : "e",
"ệ" : "e",
//--------------------------------E^
"Ế" : "e",
"Ề" : "e",
"Ể" : "e",
"Ễ" : "e",
"Ệ" : "e",
//--------------------------------e
"é" : "e",
"è" : "e",
"ẻ" : "e",
"ẽ" : "e",
"ẹ" : "e",
"ê" : "e",
//--------------------------------E
"É" : "e",
"È" : "e",
"Ẻ" : "e",
"Ẽ" : "e",
"Ẹ" : "e",
"Ê" : "e",
//--------------------------------i
"í" : "i",
"ì" : "i",
"ỉ" : "i",
"ĩ" : "i",
"ị" : "i",
//--------------------------------I
"Í" : "i",
"Ì" : "i",
"Ỉ" : "i",
"Ĩ" : "i",
"Ị" : "i",
//--------------------------------o^
"ố" : "o",
"ồ" : "o",
"ổ" : "o",
"ỗ" : "o",
"ộ" : "o",
//--------------------------------O^
"Ố" : "o",
"Ồ" : "o",
"Ổ" : "o",
"Ô" : "o",
"Ộ" : "o",
//--------------------------------o*
"ớ" : "o",
"ờ" : "o",
"ở" : "o",
"ỡ" : "o",
"ợ" : "o",
//--------------------------------O*
"Ớ" : "o",
"Ờ" : "o",
"Ở" : "o",
"Ỡ" : "o",
"Ợ" : "o",
//--------------------------------u*
"ứ" : "u",
"ừ" : "u",
"ử" : "u",
"ữ" : "u",
"ự" : "u",
//--------------------------------U*
"Ứ" : "u",
"Ừ" : "u",
"Ử" : "u",
"Ữ" : "u",
"Ự" : "u",
//--------------------------------y
"ý" : "y",
"ỳ" : "y",
"ỷ" : "y",
"ỹ" : "y",
"ỵ" : "y",
//--------------------------------Y
"Ý" : "y",
"Ỳ" : "y",
"Ỷ" : "y",
"Ỹ" : "y",
"Ỵ" : "y",
//--------------------------------DD
"Đ" : "d",
"đ" : "d",
//--------------------------------o
"ó" : "o",
"ò" : "o",
"ỏ" : "o",
"õ" : "o",
"ọ" : "o",
"ô" : "o",
"ơ" : "o",
//--------------------------------O
"Ó" : "o",
"Ò" : "o",
"Ỏ" : "o",
"Õ" : "o",
"Ọ" : "o",
"Ô" : "o",
"Ơ" : "o",
//--------------------------------u
"ú" : "u",
"ù" : "u",
"ủ" : "u",
"ũ" : "u",
"ụ" : "u",
"ư" : "u",
//--------------------------------U
"Ú" : "u",
"Ù" : "u",
"Ủ" : "u",
"Ũ" : "u",
"Ụ" : "u",
"Ư" : "u",
//--------------------------------a^
"ấ" : "a",
"ầ" : "a",
"ẩ" : "a",
"ẫ" : "a",
"ậ" : "a",
//--------------------------------A^
"Ấ" : "a",
"Ầ" : "a",
"Ẩ" : "a",
"Ẫ" : "a",
"Ậ" : "a",
//--------------------------------a(
"ắ" : "a",
"ằ" : "a",
"ẳ" : "a",
"ẵ" : "a",
"ặ" : "a",
//--------------------------------A(
"Ắ" : "a",
"Ằ" : "a",
"Ẳ" : "a",
"Ẵ" : "a",
"Ặ" : "a",
//--------------------------------A
"Á" : "a",
"À" : "a",
"Ả" : "a",
"Ã" : "a",
"Ạ" : "a",
"Â" : "a",
"Ă" : "a",
//--------------------------------a
"ả" : "a",
"ã" : "a",
"ạ" : "a",
"â" : "a",
"ă" : "a",
"à" : "a",
"á" : "a"];
return strtr(s, replace);
}
}
Now, we need to tell Zephir that our project must be compiled and the extension generated:
现在,我们需要告诉Zephir,必须编译我们的项目并生成扩展名:
cd myapp
zephir build
On the first time it is run a number of internal commands are executed producing the necessary code and configurations to export this class to the PHP extension. If everything goes well you will see the following message at the end of the output:
第一次运行时,会执行许多内部命令,这些命令会生成必要的代码和配置,以将该类导出到PHP扩展。 如果一切顺利,您将在输出末尾看到以下消息:
Compiling… Installing… Extension installed! Add extension=myapp.so to your php.ini Don’t forget to restart your web server
正在编译…正在安装…已安装扩展程序! 将extension = myapp.so添加到您的php.ini中。别忘了重新启动Web服务器
Note that since Zephir is will in its infancy, it’s possible to run into bugs and problems. The first time I tried to compile this it didn’t work. I tried the following commands and eventually got it to work:
请注意,由于Zephir尚处于起步阶段,因此可能会遇到错误和问题。 我第一次尝试编译它没有用。 我尝试了以下命令,最终使它起作用:
zephir compile
cd ext/
phpize
./configure
make && sudo make install
The last command will install the module in the PHP extensions folder (in my case: /usr/lib/php5/20121212/
). The final step is to add this extension to your php.ini by adding the following line:
最后一条命令将模块安装在PHP扩展文件夹中(在我的情况下为/usr/lib/php5/20121212/
)。 最后一步是通过添加以下行,将此扩展名添加到您的php.ini中:
extension=/usr/lib/php5/20121212/myapp.so
//or
extension=myapp.so
Restart Apache, and we’re done.
重新启动Apache,我们完成了。
测试代码 (Test the code)
Now, create a new file called zephir.php :
现在,创建一个名为zephir.php的新文件:
$flow = new Myapp\Quantum();
$ok = $flow->Harmos(-7.5);
if ($ok == true) {
echo "Write data Harmos sucess <br>";
}
$normalize = new Myapp\NormalizeChars();
echo $normalize->trans("Chào mừng bạn đến Sitepoint");
Finish up by visiting your zephir.php
page. It should look similar to the following output:
通过访问您的zephir.php
页面结束。 它看起来应该类似于以下输出:
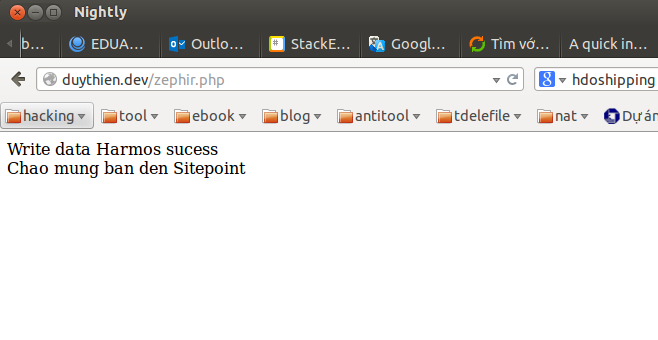
If you’re mathematically inclinced, install gnuplot and run it with the .txt output we got from our Zephir extension:
如果您数学上有点偏心,请安装gnuplot并使用从Zephir扩展名获得的.txt输出运行它:
gnuplot
splot './harmos.txt' w l
This command will draw the image using the data file harmos.txt, which will look like this, proving our equation was calculated properly.
此命令将使用数据文件harmos.txt绘制图像,看起来像这样,证明我们的方程式正确计算。
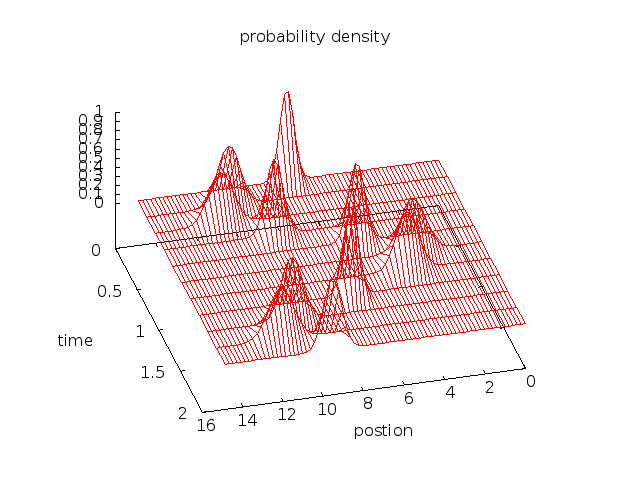
受保护的代码 (Protected code)
In some cases, the compilation does not significantly improve performance, maybe because of a bottleneck located in the I/O bound of the application (quite likely) rather than due to limits in computation or memory. However, compiling code could also bring some level of intellectual protection to your application. When producing native binaries with Zephir, you can also hide the code from users or customers – Zephir allows you to write closed source PHP applications.
在某些情况下,编译并不能显着提高性能,这可能是由于位于应用程序I / O边界中的瓶颈(很有可能),而不是由于计算或内存的限制。 但是,编译代码也可以为您的应用程序带来某种程度的知识保护。 使用Zephir生成本机二进制文件时,您还可以向用户或客户隐藏代码-Zephir允许您编写封闭源代码PHP应用程序。
结论 (Conclusion)
This article gave a basic guide on how to create extensions in Zephir. Remember, Zephir wasn’t created to replace PHP or C, but as a complement to them, allowing developers to venture into code compilation and static typing. Zephir is an attempt to join the best things from the C and PHP worlds and make applications run faster, and as such competes rather directly with HHVM and Hack.
本文提供了有关如何在Zephir中创建扩展的基本指南。 请记住,Zephir并不是为了取代PHP或C而创建的,而是作为对它们的补充,允许开发人员冒险进行代码编译和静态类型化。 Zephir试图将C和PHP世界中的最好事物结合在一起,使应用程序运行更快,因此直接与HHVM和Hack竞争。
For more information on Zephir check out the online documentation. Did you enjoy this article? Let me know in the comments!
有关Zephir的更多信息,请查看在线文档 。 你喜欢这篇文章吗? 在评论中让我知道!
翻译自: https://www.sitepoint.com/getting-started-php-extension-development-via-zephir/
zephir 开发项目
zephir 开发项目_通过Zephir开发PHP扩展的入门相关推荐
- python能二次开发cad么_2,手动创建CAD二次开发项目--AutoCAD二次开发(2020版)
本项目使用手动创建,意为不使用SDK模板. 从Visual Studio的"文件"下拉菜单中,选择"新建"->"项目...". 在出现 ...
- java 快递项目_基于SpringBoot开发的Java快递代拿系统
该项目基于 [基于SSM开发的Java快递代拿系统] 项目全面升级,使用当前最为流行的 SpringBoot 框架,相关技术栈全面更新!是您深入学习 SpringBoot 开发的最佳实践! 相关技术栈 ...
- c++后台开发项目_在京东,我是怎么做项目管理的
一个项目的完成,靠的不是一个人的单打独斗,而是团队的集体努力.协调各方不是件易事,项目经理的工作就显得格外重要.如何做好项目管理?本文作者基于自身工作经历,对这个问题提出了自己的一点思考,希望对你有帮 ...
- java开发错误_每个Java开发人员都必须避免的9个安全错误
java开发错误 Checkmarx CxSAST是功能强大的源代码分析(SCA)解决方案,旨在从根本上识别,跟踪和修复技术和逻辑安全漏洞:源代码. 在这里查看 ! 自从1995年中期引入Java以来 ...
- 开发 面试_农行软件开发中心实习面试
想看实战的可以直接跳到第五部分:农行一面 ps:之前看到同学转发的农行实习,就投了一个测试开发岗(我以为是软件开发岗)下面是面试前一天的准备和面试实战. 目录 1.c++面试准备 2.简历复盘 3.数 ...
- 日照华软游戏开发价格_棋牌游戏开发价格预算需要多少钱?
随着社会的发展,科技的进步,到目前为止棋牌游戏app应用和人们的生活越来越紧密,那么开发一款棋牌app的预算需要多少钱呢? 首先,棋牌游戏开发是需要确定开发的形式,是组建团队进行棋牌游戏定制开发还是找 ...
- 小程序开发合同_小程序开发公司在哪里找?
红匣子科技,建议大家可以从北上广深这些城市入手,毕竟一线城市人才多的同时,开发公司也多,所以可选择的开发公司比较多,适合自己的开发公司也比较多,不会像二.三城市可能选择会比较单一,可能该公司技术强,但 ...
- android开发项目app实例_APP开发发展前景好项目
APP开发发展前景好项目,在竞争激烈的网站建设行业,我们始终坚持以技术为核心,组建强大的技术开发团队,自主开发独立项目管理系统且安全稳定.简单易用:在业内具有强大的竞争力. A:我们拥有标准化APP开 ...
- 开发步骤_大数据开发必备的通用步骤详解
大数据的开发过程,如图1-1所示. 图 1-1大数据开发通用步骤图 上图只是一个简化后的步骤和流程,实际开发中,有的步骤可能不需要,有的还需要增加步骤,有的流程可能更复杂,因具体情况而定. 下面以Go ...
最新文章
- python写计算器
- evga x58服务器芯片组,为六核980X而生 EVGA发布X58 FTW3主板
- 最实用的logback讲解(2)—appender
- kali linux fuzz工具集简述
- 二叉树建立及中序遍历C++语言实现
- 学习日报 1026 使用属性升级MyBank
- C#语言学习--基础部分(十三)枚举类型和结构体
- 第38届ACM中国区通化邀请赛-中文排名表
- 纯CSS代码绘制小米LOGO
- 调用百度API,文字转语音
- 计算机鼠标键盘没反应,电脑鼠标键盘突然失灵
- [转]sourceforge文件下载过慢
- R语言中经纬度度分秒转小数
- 在哪里能找到各行业的分析研究报告?
- 电子计算机上total,计算器频幕上grand total 什么意思
- luci html 页面,luci界面修改
- 荧光定量PCR:基因相对表达量计算方法
- 博士毕业论文英文参考文献换行_如何赏心悦目的翻译英文文献全文
- 面试秘籍 | 测试工程师的简历该怎么写?(带样例)
- 看电影-《肖申克的救赎》
热门文章
- C# chart图表的简单使用+Tooltip的信息提示
- 易乐游无盘服务器虚拟内存,剑灵提示报错BladeSoul by bloodlust(此方法可解决大部分游戏兼容性问题)...
- MooTools入门
- 计算机主机箱内部设备组成,一种具有束线结构的计算机主机箱的制作方法
- linux wish和bash的关系,追踪:Wish邮到底与Wish有多大关系?
- 音视频之opengl绘制三角形
- Java多线程学习笔记20之定时器Timer
- 用应用创造社会正能量
- csgo无法连接服务器和系统有关吗,csgo-连接官方服务器失败解决方法
- 三星s8是否支持html,Galaxy S8/S8+,是否可以承载三星的野望?(中)