POJ 1195 Mobile phones
链接:http://poj.org/problem?id=1195
Time Limit: 5000MS | Memory Limit: 65536K | |
Total Submissions: 16646 | Accepted: 7653 |
Description
Write a program, which receives these reports and answers queries about the current total number of active mobile phones in any rectangle-shaped area.
Input
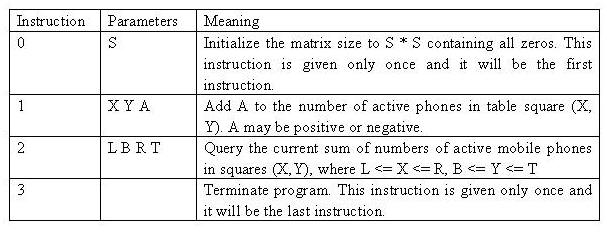
Table size: 1 * 1 <= S * S <= 1024 * 1024
Cell value V at any time: 0 <= V <= 32767
Update amount: -32768 <= A <= 32767
No of instructions in input: 3 <= U <= 60002
Maximum number of phones in the whole table: M= 2^30
Output
Sample Input
0 4 1 1 2 3 2 0 0 2 2 1 1 1 2 1 1 2 -1 2 1 1 2 3 3
Sample Output
3 4
Source
大意——给你一个n*n矩阵的区域,它的行列下标都是从0開始的。每一个矩阵元素代表一个基站。基站内的处于执行中的手机数量由于各种原因会不断变化。各个基站会不定期地向主基站报告,以便统计执行中的手机数量。你的任务是依据对应的命令完毕对应的操作。
当中基本的就是更新某一个矩阵元素的值和一个区域的矩阵元素值之和。
思路——非常显然这是一个裸的二维树状数组的题。
其操作就是更新某一个值和区间求和。因此。我们直接用一维树状数组推广过来就可以解决这个问题。注意:矩阵元素下标从0開始。
复杂度分析——时间复杂度:O((log(n))^2),空间复杂度:O(n^2)
附上AC代码:
#include <iostream>
#include <cstdio>
#include <string>
#include <cmath>
#include <iomanip>
#include <ctime>
#include <climits>
#include <cstdlib>
#include <cstring>
#include <algorithm>
#include <queue>
#include <vector>
#include <set>
#include <map>
//#pragma comment(linker, "/STACK:102400000, 102400000")
using namespace std;
typedef unsigned int li;
typedef long long ll;
typedef unsigned long long ull;
typedef long double ld;
const double pi = acos(-1.0);
const double e = exp(1.0);
const double eps = 1e-8;
const int maxn = 1030;
int mat[maxn][maxn];
int order; // 键入命令
int n; // 数组大小int lowbit(int x); // 求2^k,k表示x为二进制时末尾的零的个数
void update(int x, int y, int add); // 更新状态
int sum(int x, int y); // 求和int main()
{ios::sync_with_stdio(false);int x1, y1, x2, y2, add, ans;while (scanf("%d", &order)==1 && 3!=order){switch(order){case 0:scanf("%d", &n);memset(mat, 0, sizeof(mat)); // 初始化为0break;case 1:scanf("%d%d%d", &x1, &y1, &add);update(x1+1, y1+1, add); // x,y可能为0,所以加1。后面相似break;case 2:scanf("%d%d%d%d", &x1, &y1, &x2, &y2);ans = 0;ans += sum(x2+1, y2+1); // 总求和ans -= sum(x2+1, y1);ans -= sum(x1, y2+1); // 去掉多余部分ans += sum(x1, y1); // 多减掉一部分,加回来。绘图可知printf("%d\n", ans);break;default:printf("INPUT ERROR!\n");break;}}return 0;
}int lowbit(int x)
{return (x&(-x));
}void update(int x, int y, int add)
{ // x,y表示矩阵下标,此功能是把下标为x。y的数加上addfor (int i=x; i!=0&&i<=n; i+=lowbit(i))for (int j=y; j!=0&&j<=n; j+=lowbit(j))mat[i][j] += add;
}int sum(int x, int y)
{ // x。y表示矩阵下标,此功能是把下标小于x,y的矩阵元素值加起来int res = 0;for (int i=x; i>0; i-=lowbit(i))for (int j=y; j>0; j-=lowbit(j))res += mat[i][j];return res;
}
POJ 1195 Mobile phones相关推荐
- POJ 1195 Mobile phones(裸的二维树状数组)
http://poj.org/problem?id=1195 题意:给出一个矩阵,给某个格子加/减一个数,就某个子矩阵的和,1024*1024的范围,二维的树状数组 子矩阵(x1,y1,x2,y2)( ...
- POJ 1195 Mobile phones【 二维树状数组 】
题意:基础的二维数组,注意 0 + lowbit(0)会陷入无限循环----- 之前做一道一维的一直tle,就是因为这个-------------------------- 1 #include< ...
- hoj 1640 Mobile phones //poj 1195 Mobile phones 二维树状数组
/* (x1,y2) ____________ (x2,y2) | | | | | ...
- Take a Photo and Upload it on Mobile Phones with HTML5
In my daily life, I enjoy taking photos with my smartphone and uploading them to various websites. S ...
- POJ_1195 Mobile phones 【二维树状数组】
题目链接:http://poj.org/problem?id=1195 纯纯的二维树状数组,不解释,仅仅须要注意一点,由于题目中的数组从0開始计算,所以维护的时候须要加1.由于树状数组的下标是不能为1 ...
- poj1195 Mobile phones 二维线段树入门
二维线段树就是树套树,线段树套线段树... #include<iostream> #include<cstdio> #include<cstring> #inclu ...
- poj 1195(二维树状数组)
解题思路:这是一道很裸的二维树状数组 AC: #include<stdio.h> #include<string.h> #define N 1100 int c[N][N],n ...
- 【转载】树状数组题目
先提个注意点,由于Lowbit(0) = 0,这会导致x递增的那条路径发生死循环,所有当树状数组中可能出现0时,我们都全部加一,这样可以避免0带来的麻烦-- 简单: POJ 2299 Ul ...
- 如此好的树状数组学习资料
树状数组学习系列1 之 初步分析--czyuan原创 其实学树状数组说白了就是看那张图,那张树状数组和一般数组的关系的,看懂了基本就没问题了,推荐下面这个教程:http://www.topcoder. ...
最新文章
- nfs client高性能参数设置
- C++ 十进制转其他进制
- 2019\National _C_C++_C\试题 B: 递增序列
- 牛客 - 牛牛与牛妹的约会(贪心)
- Binary String Minimizing CodeForces - 1256D(贪心)
- 角落的开发工具集之Vs(Visual Studio)2017插件推荐
- Dynamics CRM 2015 站点地图公告配置实体显示名称的变更
- php如何检测键盘按键,js键盘事件,判断按下的是哪个键
- js 二叉树图形_js数据结构和算法(三)二叉树
- php pear mail 发送邮件,PHP用pear自带的mail类库发邮件
- NLP情感分析笔记(二):Updated情感分析
- [leetcode]Search in Rotated Sorted Array II
- eclipse tomcat cannot create a server using the...
- 阿里云服务器安装宝塔面板和配置安全组
- 深度学习图像分类(三): VggNet
- DICOM笔记-使用DCMTK读取DICOM文件保存DICOM文件
- 关于Web网页设计规范简述
- 【vbs消息轰炸代码】
- Doris export任务概率性cancelled第二种情况
- 微信智能机器人助手,基于hook技术,自动聊天机器人