在Python中使用OpenCV(CV2)对图像进行边缘检测
Modules used:
使用的模块:
For this, we will use the opencv-python module which provides us various functions to work on images.
为此,我们将使用opencv-python模块,该模块为我们提供了处理图像的各种功能。
Download opencv-python
下载opencv-python
General Way:
pip install opencv-python
Pycharm Users:
Go to the project Interpreter and install this module from there.
opencv-python Module:
opencv-python模块:
opencv-python is a python library that will solve the Computer Vision Problems and provides us various functions to edit the Images.
opencv-python是一个python库,它将解决计算机视觉问题并为我们提供编辑图像的各种功能。
Note: The edge Detection is possible only in grayscale Image.
注意:只能在灰度图像中进行边缘检测。
What we will do in this script?
我们将在此脚本中做什么?
To detect the edges of the images we will use opencv-python various Functions and Provide thresholds.
为了检测图像的边缘,我们将使用opencv-python的各种功能并提供阈值。
In this article we will detect the edge of the Image with the help of various functions and the accuracy of edge increases as we go down,
在本文中,我们将借助各种功能来检测图像的边缘,并且当我们下降时边缘的精度会提高,
Sobel Function: This Function will create the Horizontal and vertical edges and after that, we will use the Bitwise or operator to combine them
Sobel函数 :此函数将创建水平边缘和垂直边缘,然后,我们将使用按位或运算符将它们组合
Laplacian Function: This Function is the simplest Function in which we just have to put the Grayscale Variable into it, and we will get the edge detected image.
拉普拉斯函数 :此函数是最简单的函数,只需要将灰度变量放入其中,就可以得到边缘检测到的图像。
Canny Function: This is the most powerful function for edge detection and most accurate.
Canny功能 :这是边缘检测功能最强大且最准确的功能。
Let's see the code:
让我们看一下代码:
1)使用Sobel函数 (1) Using Sobel Function)
# importing the module
import cv2
# read the image and store the data in a variable
image=cv2.imread("/home/abhinav/PycharmProjects/untitled1/b.jpg")
# make it grayscale
Gray=cv2.cvtColor(image,cv2.COLOR_BGR2GRAY)
# Make it with the help of sobel
# make the sobel_horizontal
# For horizontal x axis=1 and yaxis=0
# for vertical x axis=0 and y axis=1
Horizontal=cv2.Sobel(Gray,0,1,0,cv2.CV_64F)
# the thresholds are like
# (variable,0,<x axis>,<y axis>,cv2.CV_64F)
Vertical=cv2.Sobel(Gray,0,0,1,cv2.CV_64F)
# DO the Bitwise operation
Bitwise_Or=cv2.bitwise_or(Horizontal,Vertical)
# Show the Edged Image
cv2.imshow("Sobel Image",Bitwise_Or)
cv2.imshow("Original Image",Gray)
cv2.waitKey(0)
cv2.destroyAllWindows()
Output:
输出:
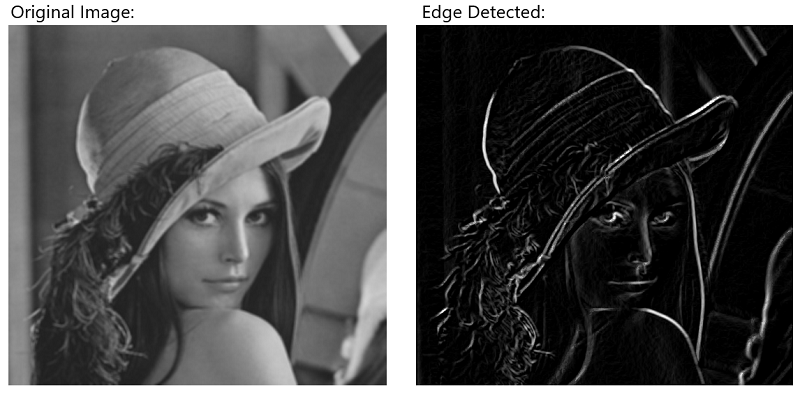
2)拉普拉斯函数 (2) Laplacian Function)
# importing the module
import cv2
# read the image and store the data in a variable
image=cv2.imread("/home/abhinav/PycharmProjects/untitled1/b.jpg")
# make it grayscale
Gray=cv2.cvtColor(image,cv2.COLOR_BGR2GRAY)
# Make Laplacian Function
Lappy=cv2.Laplacian(Gray,cv2.CV_64F)
cv2.imshow("Laplacian",Lappy)
cv2.imshow("Original",Gray)
cv2.waitKey(0)
cv2.destroyAllWindows()
Output:
输出:
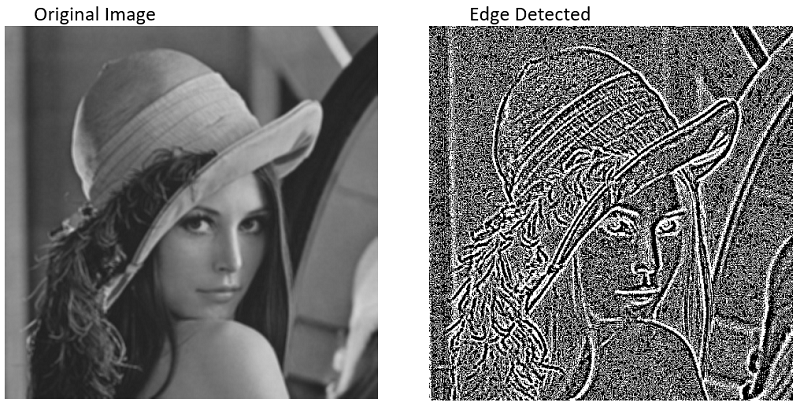
3)使用Canny函数 (3) Using Canny Function)
# importing the module
import cv2
# read the image and store the data in a variable
image=cv2.imread("/home/abhinav/PycharmProjects/untitled1/b.jpg")
# make it grayscale
Gray=cv2.cvtColor(image,cv2.COLOR_BGR2GRAY)
# Make canny Function
canny=cv2.Canny(Gray,40,140)
# the threshold is varies bw 0 and 255
cv2.imshow("Canny",canny)
cv2.imshow("Original",Gray)
cv2.waitKey(0)
cv2.destroyAllWindows()
Output:
输出:
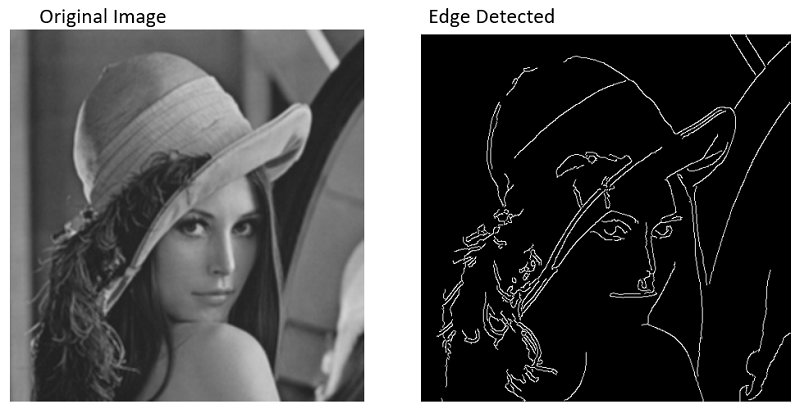
翻译自: https://www.includehelp.com/python/edge-detection-of-image-using-opencv-cv2.aspx
在Python中使用OpenCV(CV2)对图像进行边缘检测相关推荐
- 使用Python中的OpenCV降噪功能增强图像的3个步骤
点击上方"小白学视觉",选择加"星标"或"置顶" 重磅干货,第一时间送达 在本文中,我们将展示如何通过三个简单的步骤来实现降噪.我们将使用机 ...
- 在 Python 中使用 OpenCV 高斯模糊我这张的丑脸
@Author:Runsen 谁都无法否认,长得好看的人就是更具有吸引力,赏心悦目谁都喜欢.好看的人无论在职场或情场,都一定更占优势. 但是,此「颜值」非彼「颜值」.一说到「颜值」,大部分想到的是脸蛋 ...
- 在python中使用opencv自带函数转换转换RBG和BGR
在python中使用opencv自带函数转换图像的R通道和B通道 RGB -> BGR img_bgr = cv2.cvtColor(img_rgb, cv2.COLOR_RGB2BGR) BG ...
- opencv检测图片失焦 python_如何在Python中使用OpenCV执行模糊检测
如何在Python中使用OpenCV执行模糊检测 目标检测 最后更新 2020-10-12 14:23 阅读 154 最后更新 2020-10-12 14:23 阅读 154 目标检测 ##FlyAI ...
- 在Python中使用OpenCV将RGB格式的图像转换为HSV格式的图像
An HSV is another type of color space in which H stands for Hue, S stands for Saturation and V stand ...
- Python中利用Opencv进行车牌号检测
初学Python.Opencv,想用它做个实例解决车牌号检测. 车牌号检测需要分为四个部分:1.车辆图像获取.2.车牌定位.3.车牌字符分割和4.车牌字符识别 在百度查到了车牌识别部分车牌定位和车牌字 ...
- python中安装opencv一直说不是内部或外部文件_Window系统下Python如何安装OpenCV库
关于OpenCV简介 OpenCV是一个基于BSD许可(开源)发行的跨平台计算机视觉库,可以运行在Linux.Windows.Android和Mac OS操作系统上.它轻量级而且高效--由一系列 C ...
- python linux usb摄像头,树莓派用python中的OpenCV输出USB摄像头画面
本文实例为大家分享了python OpenCV来表示USB摄像头画面的具体代码,供大家参考,具体内容如下 确认Python版本 $ python Python 2.7.13 (default, Jan ...
- python cv.rectangle_Python OpenCV cv2.rectangle()用法及代码示例
OpenCV-Python是旨在解决计算机视觉问题的Python绑定库.cv2.rectangle()方法用于在任何图像上绘制矩形. 用法: cv2.rectangle(image, start_po ...
最新文章
- JSBinding+SharpKit / 菜单介绍
- idea怎么调成黑色页面
- SFTP 命令用法介绍
- linux 中国-新手村,从新手村开始,手把手带你入门梳理内核代码
- FPGA设计技巧总结
- vSphere 7融合Kubernetes,构建现代化应用的平台
- 剑指offer——二叉搜索树的后序遍历序列
- 话说这发表日志跟聊天似的简单很啊。
- python项目结构目录结构_python 项目目录结构
- 2022系统软件开发公司排行榜
- 【51单片机】基于51单片机的时钟电子锁设计
- 可以替代树莓派4(raspberry pi 4B)的tinker board 2
- PHP生成DataMatrix二维条码
- 一个小蜜蜂游戏的源代码
- 超图导入ArcGIS数据文件
- Android如何定制主题
- 怎么在网易云或者QQ音乐上上传自己翻唱的歌
- java安装步骤(java安装步骤视频)
- HashSet里的元素是不能重复的,那用什么方法来区分重复与否呢?
- Maya/3DMax/RV的集成插件下载
热门文章
- c#string倒数第二位插入字符_c#string倒数第二位插入字符_C#利用String类的IndexOf、LastIndexOf、...
- java中 下列不合法的语句_在Java中,下列( )是不合法的赋值语句。_学小易找答案...
- db2和mysql性能优化_DB2数据库性能调优的十个办法
- Maplace.js – 小巧实用的 jQuery 谷歌地图插件
- angular 拼接html 事件无效
- 写博客的这几个月,获益良多
- 【移动端 Web】怎么循序渐进地开发一个移动端页面
- scss的使用方式(环境搭建)
- VUE.js 中取得后台原生HTML字符串 原样显示问题
- 如何判断两个时间段是否有交集