vue 画布插件_一个Vue.js插件,用于使用EaselJS控制HTML5画布
vue 画布插件
vue-easeljs (vue-easeljs)
A Vue.js plugin to control an HTML5 canvas using EaselJS.
一个Vue.js插件,用于使用EaselJS控制HTML5画布。
入门 (Getting Started)
安装vue-easeljs (Install vue-easeljs)
On the command line:
在命令行上:
npm install vue-easeljs --save
In app.js:
在app.js中:
Vue.use(require('vue-easeljs'));
In your Vue.js code start with an easel-canvas
component. Other components reside within it.
在您的Vue.js代码中,以easel-canvas
组件开始。 其他组件也位于其中。
The earliest components are hidden by later components whenever they overlap.
每当最早的组件重叠时,它们就会被后面的组件隐藏。
<easel-canvas width="400" height="300"><easel-shape:x="200":y="150"form="circle"fill="blue":dimensions="20":align="['center','center']"></easel-shape>
</easel-canvas>

See Live Demo 观看现场演示
组件 (Components)
画架位图 (easel-bitmap)
Show a static image.
显示静态图像。
Attributes:
属性:
align - array, controls what point of the image the x and y refer to. Default: ['top', 'left'].
align-array,控制x和y指向图像的哪个点。 默认值:['top','left']。
alpha - 0 to 1, controls the opacity of the image. Default: 1, completely opaque.
alpha-0至1,控制图像的不透明度。 默认值:1,完全不透明。
flip - 'horizontal'|'vertical'|'both'|'', flips the image.
flip-'horizontal'|'vertical'|'both'|'',翻转图像。
image - relative or absolute URL to an image file. Required.
image-图像文件的相对或绝对URL。 需要。
rotation - degrees, rotates the image. Default: 0.
rotation-度,旋转图像。 默认值:0
scale - number, resizes the image. Default: 1.
scale-数字,调整图像大小。 默认值:1。
shadow - array, cast an image-shaped shadow. Format: [color, xOffset, yOffset, amountOfBluriness]. Default: null.
shadow-数组,投射图像形状的阴影。 格式:[颜色,xOffset,yOffset,amountOfBluriness]。 默认值:null。
x - number, horizontal position based on the origin of the parent component. Default: 0.
x-数字,基于父组件原点的水平位置。 默认值:0
y - number, vertical position based on the origin of the parent component. Default: 0.
y-数字,基于父组件原点的垂直位置。 默认值:0
Example:
例:
<easel-bitmapimage="/images/awesome-background.jpg":x="0":y="0":align="['left','top']"
>
</easel-bitmap>
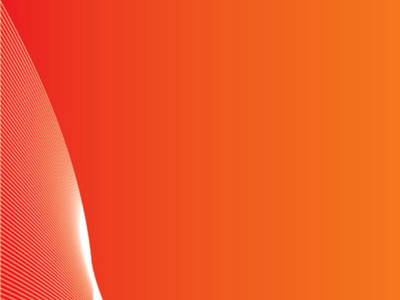
See Live Demo 观看现场演示
画架画布 (easel-canvas)
Give the vue-easeljs components a place to live.
给vue-easeljs组件一个居住的地方。
Attributes:
属性:
anti-alias: boolean, whether or not edges should be smoothed on scaled images. Default: true.
抗锯齿:布尔值,是否应在缩放的图像上对边缘进行平滑处理。 默认值:true。
height: number, the pixel height of the canvas on the page. Default: 300.
height:数字,页面上画布的像素高度。 默认值:300。
width: number, the pixel width of the canvas on the page. Default: 150.
width:数字,页面上画布的像素宽度。 默认值:150
Example:
例:
<easel-canvas width="500" height="100"><easel-shapeform="rect":dimensions="[500,100]":x="0":y="0"fill="#CCCCFF"></easel-shape><easel-text text="This is so easy!":x="250":y="75"font="70px Calibri"color="white":shadow="['#000088',3,2,3]":align="['center', 'alphabetical']"></easel-text>
</easel-canvas>
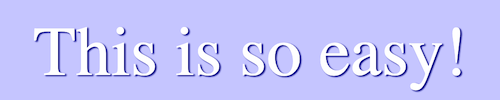
See Live Demo 观看现场演示
画架容器 (easel-container)
Group other vue-easel components together and manipulate them as one.
将其他vue-easel组件组合在一起,然后将它们作为一个组件进行操作。
Attributes:
属性:
alpha - 0 to 1, controls the opacity of the container. Default: 1, completely opaque.
alpha-0到1,控制容器的不透明度。 默认值:1,完全不透明。
flip - 'horizontal'|'vertical'|'both'|'', flips the container.
flip-'horizontal'|'vertical'|'both'|'',翻转容器。
rotation - degrees, rotates the container. Default: 0.
rotation-度,旋转容器。 默认值:0
scale - number, resizes the container. Default: 1.
scale-数字,调整容器大小。 默认值:1。
shadow - array, cast a shadow of all contained components. Format: [color, xOffset, yOffset, amountOfBluriness]. Default: null.
shadow-数组,为所有包含的组件投射阴影。 格式:[颜色,xOffset,yOffset,amountOfBluriness]。 默认值:null。
x - number, horizontal position based on the origin of the parent component. Default: 0.
x-数字,基于父组件原点的水平位置。 默认值:0
y - number, vertical position based on the origin of the parent component. Default: 0.
y-数字,基于父组件原点的垂直位置。 默认值:0
Example:
例:
<easel-containerflip="horizontal"scale=".5":x="250":y="50"
><easel-bitmapimage="/images/wooden-sign-texture.png"></easel-bitmap><easel-text text="Dan's Left Shoe Emporium"font="50px 'Times New Roman'":y="25"></easel-text>
</easel-container>
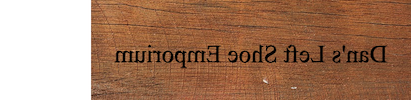
See Live Demo 观看现场演示
画架形状 (easel-shape)
Show a shape.
显示形状。
Attributes:
属性:
align - array, controls what point of the shape the x and y refer to. Default: ['top', 'left'].
align-array,控制x和y指向形状的哪个点。 默认值:['top','left']。
alpha - 0 to 1, controls the opacity of the shape. Default: 1, completely opaque.
alpha-0到1,控制形状的不透明度。 默认值:1,完全不透明。
dimensions - Depends on the form. See below. Required.
尺寸-取决于形式。 见下文。 需要。
fill - color, the inside of the shape
填充-颜色,形状的内部
flip - 'horizontal'|'vertical'|'both'|'', flips the shape.
flip-'水平'|'垂直'|'都'|'',翻转形状。
form - 'circle'|'ellipse'|'rect'|'star'. Required.
形式-'circle'|'椭圆'|'rect'|'star'。 需要。
rotation - degrees, rotates the shape. Default: 0.
rotation-度,旋转形状。 默认值:0
scale - number, resizes the shape. Default: 1.
scale-数字,调整形状的大小。 默认值:1。
shadow - array, cast a same-shape shadow. Format: [color, xOffset, yOffset, amountOfBluriness]. Default: null.
shadow-数组,投射相同形状的阴影。 格式:[颜色,xOffset,yOffset,amountOfBluriness]。 默认值:null。
stroke - color, the outline of the shape.
笔触-颜色,形状的轮廓。
x - number, horizontal position based on the origin of the parent component. Default: 0.
x-数字,基于父组件原点的水平位置。 默认值:0
y - number, vertical position based on the origin of the parent component. Default: 0.
y-数字,基于父组件原点的垂直位置。 默认值:0
Dimensions for:
尺寸:
circle - number, the radius of the circle
circle-数字,圆的半径
ellipse - array, the width and height of the ellipse. Format: [width, height].
ellipse-数组,椭圆的宽度和高度。 格式:[宽度,高度]。
rect - array, the width and height of the rectangle. Optionally include the radius of rounded corners. Format: [width, height], or [width, height, allRadiuses], or [width, height, topLeftRadius, topRightRadius, bottomRightRadius, bottomLeftRadius].
rect-数组,矩形的宽度和高度。 (可选)包括圆角半径。 格式:[宽度,高度]或[宽度,高度,所有半径]或[宽度,高度,topLeftRadius,topRightRadius,bottomRightRadius,bottomLeftRadius]。
star - array, the radius, sides count, and point size of a "star". Use point size 0 to draw a simple polygon. Max point size is 1.
星形-数组,“星形”的半径,边数和点大小。 使用点大小0绘制一个简单的多边形。 最大点数为1。
Example, to draw a blue triangle with red stroke:
例如,用红色笔划绘制一个蓝色三角形:
<easel-shapeform="star":dimensions="[100, 3, 0]"fill="blue"stroke="red":x="100":y="100"
>

See Live Demo 观看现场演示
画架精灵 (easel-sprite)
Show a moving image. An easel-sprite
must reside in an easel-sprite-sheet
node. The easel-sprite-sheet
defines the animations that can be used by the easel-sprite
.
显示运动图像。 easel-sprite
必须位于easel-sprite-sheet
节点中。 easel-sprite-sheet
定义了可以由easel-sprite
使用的动画。
Attributes:
属性:
align - array, controls what point of the image the x and y refer to. Default: ['top', 'left'].
align-array,控制x和y指向图像的哪个点。 默认值:['top','left']。
alpha - 0 to 1, controls the opacity of the image. Default: 1, completely opaque.
alpha-0至1,控制图像的不透明度。 默认值:1,完全不透明。
animation - string, name of the animation to run from the
easel-sprite-sheet
. Required.animation-string,从
easel-sprite-sheet
运行的动画的名称。 需要。flip - 'horizontal'|'vertical'|'both'|'', flips the image.
flip-'horizontal'|'vertical'|'both'|'',翻转图像。
rotation - degrees, rotates the image. Default: 0.
rotation-度,旋转图像。 默认值:0
scale - number, resizes the image. Default: 1.
scale-数字,调整图像大小。 默认值:1。
shadow - array, cast an image-shaped shadow. Format: [color, xOffset, yOffset, amountOfBluriness]. Default: null.
shadow-数组,投射图像形状的阴影。 格式:[颜色,xOffset,yOffset,amountOfBluriness]。 默认值:null。
x - number, horizontal position based on the origin of the parent component. Default: 0.
x-数字,基于父组件原点的水平位置。 默认值:0
y - number, vertical position based on the origin of the parent component. Default: 0.
y-数字,基于父组件原点的垂直位置。 默认值:0
Example:
例:
See the easel-sprite-sheet
example below.
请参阅下面的easel-sprite-sheet
例。
画架精灵表 (easel-sprite-sheet)
Define image animations for use in a sprite.
定义要在精灵中使用的图像动画。
Attributes:
属性:
animations - object, defines names for animations. Each animation is a series of frames. Format: {<animationName>: [frame1, frame2, ...], ...}.
animations-对象,定义动画的名称。 每个动画都是一系列帧。 格式:{<animationName>:[frame1,frame2,...],...}。
framerate - number, the speed the animation should play at.
帧速率-数字,动画播放的速度。
frames - mixed, usually an object with format: {width: width, height: height}. Required.
帧-混合的,通常是以下格式的对象:{width:width,height:height}。 需要。
images - array, relative or absolute URL's to image files. Required.
images-图片文件的数组,相对URL或绝对URL。 需要。
In-depth:
深入:
EaselJS provides a lot of options for defining sprite sheets, to allow you to format the images in whatever way suits you.
EaselJS提供了许多用于定义精灵表的选项,以允许您以适合自己的任何方式格式化图像。
A sprite sheet is a single image with a set of images on it that will be used in rotation to show an animation.
Sprite工作表是单张图像,上面带有一组图像,这些图像将旋转使用以显示动画。
The friendliest approach is to layout the images in a grid. For example, if a character requires 32x32 pixels to show, a sprite sheet might have 10 images of the character in a 320x32 pixel image. (Or a 32x320 image. Or a 160x64 image. EaselJS will figure it out.)
最友好的方法是将图像布置在网格中。 例如,如果一个角色需要显示32x32像素,则子画面可以在320x32像素的图像中包含10个该角色的图像。 (或者是32x320的图像。或者是160x64的图像。EaselJS会找出来的。)
In that case the following definition will do nicely:
在这种情况下,以下定义会很好地执行:
<easel-sprite-sheet:images="['/images/character.png']":frames="{width:32,height:32}"...
>
But sometimes frames have space between them or margins around them. In these cases, you'll need to specify more information.
但是有时框架之间有空间或周围有空白。 在这些情况下,您需要指定更多信息。
In this example, there is space and margin between the frames.
在此示例中,帧之间有空间和边距。
<easel-sprite-sheet:images="['/images/lots-of-characters.png']":frames="{width:32,height:32,spacing:5,margin:10}"...
>
Other times, the frames are different sizes or are on different images.
有时,框架的大小不同或在不同的图像上。
In that case, this format will be required:
在这种情况下,将需要以下格式:
<easel-sprite-sheet:images="['/images/thomasChugging.png','/images/thomasBraking.png']":frames="[// x, y, width, height, imageIndex[0, 0, 64, 32, 0],[0, 32, 64, 32, 0],...]"...
>
Animations give names to a series of frames. The name is used in the easel-sprite
component to determine what to show.
动画为一系列框架命名。 该名称用于在easel-sprite
组件中确定要显示的内容。
The value of an animation is a number, array, or object.
动画的值是数字,数组或对象。
If the animation is just one frame, a number is appropriate.
如果动画只有一帧,则数字是合适的。
If the animation is multiple frames laid out in order in the sprite sheet, then the array short form can be used. The first two values are the start and end of the animation. The third value is an optional next animation to begin when this one is done. The fourth is speed.
如果动画是在精灵表中按顺序排列的多个帧,则可以使用数组缩写形式。 前两个值是动画的开始和结束。 第三个值是可选的下一个动画,此动画将在完成后开始。 第四是速度。
If the animation uses frames that are not in order, use an object with the field frames
and the optional next
and speed
.
如果动画使用的帧顺序不正确,则将一个对象与场frames
以及可选的next
和speed
。
animations: {stand: 0,run: [1, 4, "stand"],fall: {frames: [5, 1, 0, 2],},
}
Example:
例:
<easel-sprite-sheet:images="['images/lastguardian-all.png']":frames="{width:32,height:32}":animations="{stand: 7,walk: [6, 7],walkAndStand: [6, 7, 'stand'],confusion: {frames: [5, 1, 0, 2],},}":framerate="4"
><easel-sprite:x="32":y="32"animation="walkAndStand"></easel-sprite>
>
</easel-sprite-sheet>

See Live Demo 观看现场演示
画架文本 (easel-text)
Show some text.
显示一些文字。
Attributes:
属性:
align - array, controls what point of the text the x and y refer to. Default: ['top', 'left'].
align-array,控制x和y指向文本的哪一点。 默认值:['top','left']。
alpha - 0 to 1, controls the opacity of the text. Default: 1, completely opaque.
alpha-0到1,控制文本的不透明度。 默认值:1,完全不透明。
color - color, the color to use for the text.
color-颜色,用于文本的颜色。
flip - 'horizontal'|'vertical'|'both'|'', flips the text.
flip-'horizontal'|'vertical'|'both'|'',翻转文本。
font - string, size and family of the font. Format: "Npx family".
字体-字符串,字体大小和字体系列。 格式:“ Npx系列”。
rotation - degrees, rotates the text. Default: 0.
rotation-度,旋转文本。 默认值:0
scale - number, resizes the text. Default: 1.
scale-数字,调整文本大小。 默认值:1。
shadow - array, cast a text-shaped shadow. Format: [color, xOffset, yOffset, amountOfBluriness]. Default: null.
shadow-数组,投射文本形状的阴影。 格式:[颜色,xOffset,yOffset,amountOfBluriness]。 默认值:null。
text - string, the text to display.
text-字符串,要显示的文本。
x - number, horizontal position based on the origin of the parent component. Default: 0.
x-数字,基于父组件原点的水平位置。 默认值:0
y - number, vertical position based on the origin of the parent component. Default: 0.
y-数字,基于父组件原点的垂直位置。 默认值:0
Example:
例:
<easel-texttext="The Ran In Span Falls Manly On The Plan":x="250":y="32"font="20px Arial"color="red"
>
</easel-text>

See Live Demo 观看现场演示
对齐属性 (Align attribute)
All visible components can accept an align
attribute. The align attribute defaults to ['left', 'top']
.
所有可见组件都可以接受align
属性。 align属性默认为['left', 'top']
。
The field is formatted as [horizontal-alignment, vertical-alignment]
.
该字段的格式为[horizontal-alignment, vertical-alignment]
。
Alignment options:
对齐选项:
horizontal: left, center, right
水平:左,中,右
vertical: top, center, bottom
垂直:上,中,下
The 'easel-text' component has some special alignment options:
“ easel-text”组件具有一些特殊的对齐选项:
horizontal: start, end, left, right, center
水平:开始,结束,向左,向右,居中
vertical: top, hanging, middle, alphabetic, ideographic, bottom
垂直:顶部,悬挂,中间,字母,表意,底部
These are described in the whatwg spec for HTML5 canvases.
这些在HTML5画布的whatwg规范中进行了描述。
大事记 (Events)
All visible components and the canvas itself emit Vue.js events with an event object.
所有可见组件和画布本身都会通过事件对象发出Vue.js事件。
added - Fired when the component is added to its parent.
添加-将组件添加到其父项时触发。
animationend - (easel-sprite only) - Fired when an animation completes.
animationend-(仅限easel-sprite)-动画完成时触发。
change - (easel-sprite only) - Fired when an animation changes.
change-(仅用于easel-sprite)-动画更改时触发。
click - Fired when the component is clicked or tapped.
click-单击或轻击组件时触发。
dblclick - Fired when the component is double-clicked or tapped.
dblclick-双击或点击组件时触发。
mousedown - Fired when the component is clicked down.
mousedown-按下组件时触发。
mouseout - Fired when the mouse leaves a component's hit area.
mouseout-当鼠标离开组件的点击区域时触发。
mouseover - Fired when the mouse enters a component's hit area.
mouseover-当鼠标进入组件的点击区域时触发。
pressmove - Fired when the component is dragged.
pressmove-拖动组件时触发。
pressup - Fired when the component is unclicked.
pressup-取消单击组件时触发。
removed - Fired when the component is removed from its parent.
已移除-当组件从其父级移除时触发。
rollout - Fired when the mouse leaves a component's hit area.
卷展栏-鼠标离开组件的点击区域时触发。
rollover - Fired when the mouse enters a comopnent's hit area.
翻转-当鼠标进入伙伴的点击区域时触发。
tick - Fired many times a second to keep the components in sync. Using this event can impact performance.
tick-每秒触发多次,以使组件保持同步。 使用此事件可能会影响性能。
Modifiers such as .stop
usually work with the events.
诸如.stop
类的.stop
通常与事件配合使用。
These events will be made available in the future.
这些事件将在将来提供。
drawend
支出
drawstart
画龙点睛
mouseenter
鼠标输入
mouseleave
鼠标离开
stagemousedown
暂停鼠标
stagemousemove
舞台表演
stagemouseup
舞台鼠标
tickend
tickend
tickstart
节拍开始
待定 (Pending)
These plans are merely dreams:
这些计划只是梦想:
Percentages
百分比
Caching shapes
缓存形状
Pre-load images
预加载图像
Masks
口罩
Mouse cursors
鼠标光标
Hit areas
热门地区
There are no plans to implement these features:
没有计划实现这些功能:
Filters
筛选器
BitmapText
位图文本
Buttons
纽扣
Complex Vector Paths
复数向量路径
MovieClip
电影剪辑
翻译自: https://vuejsexamples.com/a-vue-js-plugin-to-control-an-html5-canvas-using-easeljs/
vue 画布插件
vue 画布插件_一个Vue.js插件,用于使用EaselJS控制HTML5画布相关推荐
- vue 图片引入_一个Vue的时间插件
安装 npm install -S hzqing-vue-timeline 如何引入 main.js: // 全局注册import hzqingVueTimeline from 'hzqing-vue ...
- baidumap vue 判断范围_一个Vue引发的性能问题
笔者最近在一个Vue项目里面引入了一个动画库,但是发现性能有点异常,项目里面使用的CPU是在一个demo页面的3.5倍左右,我已经把项目里所有其它干扰的东西都给删掉了,但是CPU就是降不下去,如下图所 ...
- html组态插件_组态 web组态 插件 编辑器 使用说明书
一.插件目录 init.json 插件初始化配置 initConfig.js 插件初始化方法 module_config.json 所有的组件配置都放在这里,要添加组件需要在这里面添加. exampl ...
- html中画布中怎么画一条直线,使用EaselJS在html5画布中绘制线条
我对画架和HTML5本身非常新颖.我正试图在使用EaselJS的画布上绘制一条线. X坐标纵坐标固定为100,Y坐标纵坐标从数组列表中获得.我写的代码如下.可以请别人让我知道我哪里出错了?使用Ease ...
- chrome vue插件_「Vue学习记录一」开发环境准备
1.开发工具 - VS Code ❝ 选择 VS Code 是因为这是一款很容易上手的工具,在 VS Code 中找到的每个功能都完成一项出色的工作,构建了一些简单的功能集,包括语法高亮.智能补全.集 ...
- Vue插件_自己封装插件_以及使用自定义插件---vue工作笔记0017
然后我们再来看插件的使用. vue官网上也提供了,怎么样来自己开发插件. 我们自己开发一个插件,并且使用 首先我们把插件包括在一个函数中去, (function(){ })()
- vue连线 插件_基于vue的网页标尺辅助线工具(vue-ruler-tool)
原文首发于我的博客,欢迎点击查看获得更好的阅读体验~ 标尺辅助线.gif vue-ruler-tool 最近在网上找到一个网页制作辅助工具-jQuery标尺参考线插件,觉得在现在的一个项目中能用的上, ...
- js清空文本框的值_一个Vue.js实例控制字变大变小,含样式操作,flex布局。「603」...
这是一个用vue.js对css操作完成的实例. 当然用了flex简单布局. 一.先创建一个html文件,记得添加vue库文件. 二.创建一盒容器vmdiv,用vue绑定它,测试vue绑定后的插入值te ...
- vue脚手架vue数据交互_学习Vue:3分钟的交互式Vue JS教程
vue脚手架vue数据交互 Vue.js is a JavaScript library for building user interfaces. Last year, it started to ...
最新文章
- 首发 | 13篇京东CVPR 2019论文!你值得一读~ 技术头条
- css border制作小三角形状及应用(兼容IE6)
- linux 脚本 变量为空,Shell脚本中判断输入变量或者参数是否为空的方法
- csu 1976: 搬运工小明
- v9更新栏目缓存提示PHP has encountered a Stack overflow解决方法
- linux boost 卸载,Ubuntu下boost库的编译安装步骤及卸载方法详解
- Kendo UI开发教程(8): Kendo UI 特效概述
- 如何使用trace模式运行BRF+应用
- 加速你的Hibernate引擎(上)
- 让你的 Linux 远离黑客(二):另外三个建议
- 小米路由器3无线网连接到服务器,小米路由器3设置完成后,手机能用,电脑没法上网...
- cck8graphpad作图_北京cck8数据作图「上海儒安生物科技供应」
- python投资组合有效边界,【python量化】如何用Python找到投资时的最佳组合比例
- dbt-tidb 1.2.0 尝鲜
- Handing Incomplete Heterogeneous Data using VAEs
- Readhub客户端
- perl 产生随机数
- Vue子组件触发父组件事件
- 双链路热备份(负载分担)实验
- Linux服务器集群部署
热门文章
- Java岗大厂面试百日冲刺 - 日积月累,每日三题【Day20】—— MyBatis2
- KMP算法中的next数组求解
- 计算机主板大小性能区别,主板对CPU的影响大吗?聊聊主板对电脑性能影响有多大...
- 透过8个行业,带你了解Web 3.0区块链网络时代的颠覆性,你的行业会被颠覆吗?...
- skyeye模拟uboot启动linux(initrd方式)
- 中望CAD调用lisp在哪_中望CAD加载LISP程序
- 在PyBullet中进行机械臂的强化学习
- 人工智能的经济学和效益
- 一段通过java 得到svn log 然后过滤一些文字, 最后打印出来的shell
- 图新地球工程版|同豪路易BIM设计成果、CAD、倾斜模型、纬地数据、影像地形等多源项目数据融合展示汇报