匕首线切割图纸下载_匕首击剑:更短更轻松!
匕首线切割图纸下载
学习Android开发 (Learning Android Development)
About more than 2 years ago, I was on a quest to make Dagger 2 as simple as possible, as it is such a complicated subject. So I came up with the below blog.
一个回合2年多前,我在寻求使匕首2尽可能的简单,因为它是这样一个复杂的课题。 所以我想出了以下博客。
The complication of Dagger has turned some developers away from it, especially from the iOS communities who highly recommend their Android counterpart in not using it.
Dagger的复杂性使一些开发人员远离了Dagger,尤其是iOS社区,他们强烈建议其Android同行不要使用它。
Google is fully aware of Dagger 2 complication of usage, and also the need to self inject itself into the Activities and Application. Last year, Google announced their quest to make it even better!
Google完全意识到Dagger 2使用的复杂性,也需要将自己自我注入到“活动和应用程序”中。 去年,Google宣布了他们的追求,以求更好!
A few weeks ago, I got to know about Dagger Hilt. Let’s look at it!
几周前,我结识了Dagger Hilt 。 让我们看看吧!
在此之前……让我们进行设置。 (Before that… let’s set it up.)
To setup, just add the following in your project build.gradle
要设置,只需在您的项目build.gradle
添加以下build.gradle
classpath 'com.google.dagger:hilt-android-gradle-plugin:2.28-alpha'
Then followed by the below in your app’s build.gradle
然后在应用程序的build.gradle
跟随以下build.gradle
apply plugin: 'dagger.hilt.android.plugin'apply plugin: 'kotlin-kapt'// ...implementation 'com.google.dagger:hilt-android:2.28-alpha'kapt 'com.google.dagger:hilt-android-compiler:2.28-alpha'
Ok now, let’s look at it.
好吧,让我们看看。
甚至更容易,更短。 (Even easier and shorter.)
If you have seen my previous blog, which I wrote with 20 lines of code to show how Dagger 2 works. Now it is 18 lines!! (well, although it is only 2 lines, but wait… it means a lot… let me explained later).
如果您看过我以前的博客 ,那么我用20行代码编写了该博客 ,以展示Dagger 2的工作原理。 现在是18行! (嗯,虽然只有2行,但是等等……这意味着很多……让我稍后解释)。
@HiltAndroidAppclass MainApplication: Application()@AndroidEntryPointclass MainActivity : AppCompatActivity() {@Inject lateinit var info: Infooverride fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) setContentView(R.layout.activity_main) text_view.text = info.text }}class Info @Inject constructor() { val text = "Hello Dagger Hilt"}
As you see, 2 annotation is introduced
如您所见,引入了2条注释
@HiltAndroidApp
: To tell Dagger Hilt, you’ll be using in your application@HiltAndroidApp
:要告诉Dagger Hilt,您将在应用程序中使用@AndroidEntryPoint
: Your activity needed to be injected with something@AndroidEntryPoint
:您的活动需要注入一些东西
Then all you need to do is to state @Inject lateinit var
on your needed injectable dependencies, then it will be available to you automatically without one injecting!
然后,您所需要做的就是在所需的可注入依赖项上声明@Inject lateinit var
,然后它将自动为您提供,而无需进行一次注入!

与以前的相比,看起来如何 (How does it look like when compares to previous)
If we’re to look at the previous shortest example I provided in my previous blog, we have dropped two things below.
如果要看一下我在前一个博客中提供的最短的例子,我们在下面做了两件事。
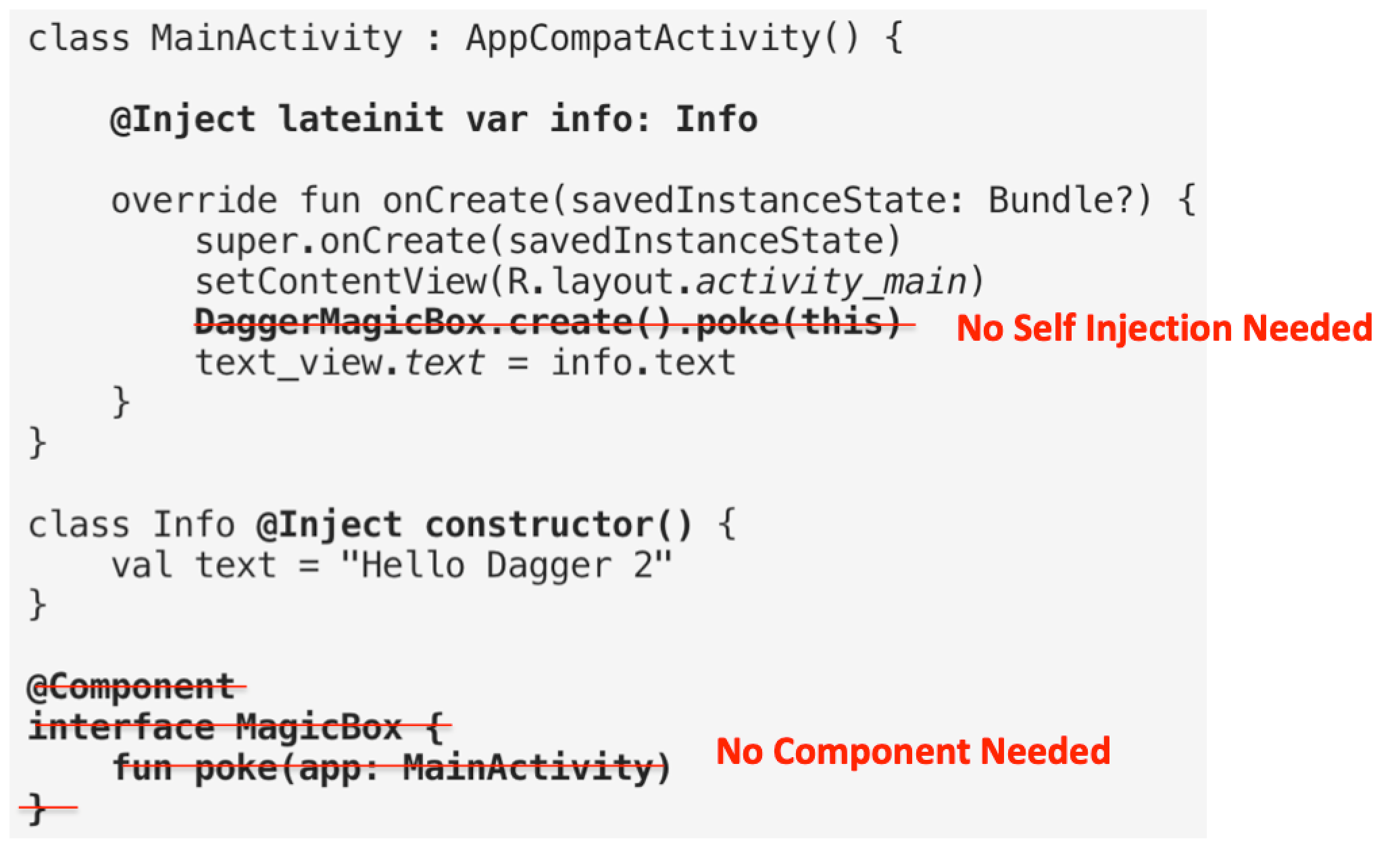
No more self-injection within the
MainActivity
. This is pretty cool, as now we feel more like a real Dependency Injection framework.MainActivity
不再需要自我注入。 这非常酷,因为现在我们感觉更像一个真正的依赖注入框架。- No need to explicitly declare a component, and create the component (MagicBox in the example above). The components are nothing but just a container to glue things together. It is now got hidden away by the Dagger Hilt!无需显式声明组件,也无需创建组件(上面示例中的MagicBox)。 这些组件不过是将事物粘合在一起的容器。 现在,它被匕首剑柄隐藏了!
With the additional two annotations, we are now done away with the self-injection and components, how nice!
有了另外两个注释,我们现在就完成了自我注入和组件,这太好了!
等等...如果我有更复杂的依赖关系呢? (Wait… what if I have more complicated dependencies?)
Some dependencies can’t be easily constructed by itself (e.g. dependency on interfaces that need a concrete implementation). In this case, we will still need Dagger’s @Module
and @Provides
to define them.
某些依赖关系本身无法轻易构建(例如,对需要具体实现的接口的依赖关系)。 在这种情况下,我们仍然需要Dagger的@Module
和@Provides
来定义它们。
Check out this blog if you like to learn about
@Module
and@Provides
如果您想了解
@Module
和@Provides
请查看此博客。
But it is much simpler since we no longer have @Component
.
但这要简单得多,因为我们不再有@Component
。
应用层模块 (Application Level Module)
Assuming you want a dependency to be available to everyone, then you need to be at the Application level. You just need to add @InstallIn(ApplicationComponent::class)
after the @Module
as shown below.
假设您希望所有人都可以使用依赖项,那么您需要处于应用程序级别。 您只需要添加@InstallIn(ApplicationComponent::class)
在@Module
,如下所示。
@Module@InstallIn(ApplicationComponent::class)object ApplicationModule { @Singleton @Provides fun provideApplicationWideDependency(some: SomeClass) = ApplicationWideDependency(some)}
Do note at this level, I can use @Singleton
if I wanted it to be one and only one copy throughout the life of the application
请注意这一级别,如果我希望它成为整个应用程序生命周期中的唯一副本,则可以使用@Singleton
活动级别模块 (Activity Level Module)
However, there are some dependencies you want it to be at Activity Level, (e.g. each Activity will need to be given its unique dependencies), then you just need to have @InstallIn(ActivityComponent::class)
after the @Module
as shown below.
但是,您希望它具有一些活动级别的依赖关系(例如,每个活动都需要被赋予其唯一的依赖关系),那么您只需要具有@InstallIn(ActivityComponent::class)
在@Module
,如下所示。
@Module@InstallIn(ActivityComponent::class)object ActivityModule { @ActivityScoped @Provides fun provideActivityWideDependency(some: SomeClass) = ActivityWideDependency(some)}
Do note at this level, I can use @ActivityScoped
, if I wanted it to be one and only one copy throughout the life of the activity (for each Activity, it will be a different copy).
请注意,在这个级别上,我可以使用@ActivityScoped
,如果我希望它在整个活动的生命周期中只有一个副本(对于每个Activity,它将是一个不同的副本)。
Unlike the original Dagger,
@ActivityScoped
is a provided@Scope
by Dagger Hilt.与原始Dagger不同,
@ActivityScoped
是Dagger Hilt提供的@Scope
。
In another word, by using @InstallIn(ApplicationComponent::class)
and @InstallIn(ActivityComponent::class)
, we have simplified the use of Dagger from needing to work with @Component
and @Subcomponent
.
换句话说,通过使用@InstallIn(ApplicationComponent::class)
和 @InstallIn(ActivityComponent::class)
,我们简化了Dagger的使用,而无需使用@Component
和@Subcomponent
。
The entire setup of @Component
and @Subcomponent
is part of the contribution of making using Dagger 2 complicated. Hence this simplifies the entire Dagger 2 usage for Android.
@Component
和@Subcomponent
的整个设置是使Dagger 2变得复杂的部分贡献。 因此,这简化了整个Dagger 2在Android上的使用。

但是……但是……我需要让每个Activity都具有一组依赖关系(模块等)。 (But… but… I need to have each Activity it’s set of dependencies (module etc).)
For now, Dagger Hilt is heading towards monolithic components (i.e. a single component shared across activities). This is with the purpose of simplifies its usage and many other reasons as shared by Google.
目前,Dagger Hilt正朝着单一组件(即跨活动共享的单个组件)发展。 这样做的目的是简化其用法以及Google共享的许多其他原因。
If you need the complexity of dependencies injection, you probably already have a good understanding of Dagger 2 itself, and comfortable using @Component
and @Subcomponent
. Perhaps you can consider continue using the original Dagger for your need.
如果您需要依赖项注入的复杂性,则可能已经对Dagger 2本身有了很好的了解,并且可以轻松使用@Component
和@Subcomponent
。 也许您可以考虑根据需要继续使用原始的Dagger。
Optionally, you can try Dagger Android, though I’m not a fan of it, as I think it complicates things more in order to avoid self-injection on Android components.
(可选)您可以尝试使用Dagger Android,尽管我不喜欢它,因为我认为它会使事情更加复杂,从而避免在Android组件上进行自我注入。
That’s it for now. If you like the code to play around, you can get it from
现在就这样。 如果您喜欢该代码,可以从
If you like this topic, perhaps the next one would be relevant to you as well.
如果您喜欢此主题,那么下一个主题可能也与您相关。
演示地址
翻译自: https://medium.com/mobile-app-development-publication/dagger-hilt-even-shorter-and-easier-881255ea54dd
匕首线切割图纸下载
http://www.taodudu.cc/news/show-3376392.html
相关文章:
- 匕首线切割图纸下载_真正的单身与匕首2
- 匕首线切割图纸下载_匕首击剑简介
- 匕首线切割图纸下载_干净匕首
- 匕首线切割图纸下载_匕首2-利用范围和子组件
- IOError: [Errno 2] No such file or directory的解决方法
- ArcEngine代码 打开文件地理库GDB中的ITable表
- 数据对齐 Tab 键
- ArcEngine代码 GP区域分析之面积制表(统计各行政区内的各土地利用类型面积)
- matlab图
- 在Python中如何优雅地创建表格
- 平均成绩和等级python_使用python3.6中的函数和列表计算平均成绩
- google velvet_LG Velvet 5G值得推出新的设计方向
- 昆明计算机学校有个爱什么,云南6个爱情传说,总有一个感动你
- 勇士屠熊,绿军射鹿,夕阳西下,人群散尽,唯有烈火燎原势不可挡
- Spring之IOC(一)
- java程序连接2个数据库,Java连接数据库(2)
- 第十一PickerView
- 一只眼睛的鹿
- php发送短信发送失败如何处理,php – 从我的网站发送短信(仅发送不接收)
- HarmonyOS(一) 快速开始学习鸿蒙开发,官方文档学习路线解析
- 数据库垂直拆分 水平拆分
- 用户行为分析大数据系统(实时统计每个分类被点击的次数,实时计算商品销售额,统计网站PV、UV )
- JavaScript大作业——美食餐饮网站设计与实现(HTML+CSS+JavaScript)
- 如何评估研发人员效能?软件工程师报告帮你看见每个人的贡献
- FreeBSD网站平台建设全过程
- wordpress 大网站_加快您的WordPress网站
- 使用Varnish为网站加速
- Mysql学习(一)架构原理
- php页面设置后缩放变形,网站网页缩放后变形
- 技术人如何利用 github+Jekyll ,搭建一个独立免费的技术博客
匕首线切割图纸下载_匕首击剑:更短更轻松!相关推荐
- 匕首线切割图纸下载_匕首击剑简介
匕首线切割图纸下载 Dependency injection (DI), in a nutshell, is a technique whereby one object provides or su ...
- 匕首线切割图纸下载_匕首2-利用范围和子组件
匕首线切割图纸下载 范围的含义是什么? (What is the meaning of scope?) Scope refers to the lifetime of an object. Consi ...
- 匕首线切割图纸下载_使用Robolectric测试带有匕首注入依赖性的类
匕首线切割图纸下载 It is common for Android code to use dependency injection (DI). And one of the tenets of D ...
- 匕首线切割图纸下载_干净匕首
匕首线切割图纸下载 重点 (Top highlight) A pragmatic guide to dependency injection on Android 在Android上进行依赖注入的实用 ...
- 匕首线切割图纸下载_真正的单身与匕首2
匕首线切割图纸下载 我之前写过有关Dagger 2的文章.但是,我仍然不了解每个角落. 尤其是@Singleton注释可能会引起误解,因为用户Zhuiden十分友善地指出 : 如果您每次注入都创建一个 ...
- 匕首线切割图纸下载_我们从匕首到科恩的旅程
匕首线切割图纸下载 When you think about Dependency Injection (DI) on Android, the first library you probably ...
- 钢铁侠头盔制作图纸下载_如何在10分钟内制作头盔图
钢铁侠头盔制作图纸下载 我每天的大部分时间都涉及创建,修改和部署Helm图表以管理应用程序的部署. Helm是Kubernetes的应用程序包管理器,负责协调应用程序的下载,安装和部署. Helm图表 ...
- kiel4.7下载_使用Kiel构建更好,干净的RecyclerView.Adapter
kiel4.7下载 Some years ago, we had only ListView and its Adapter. It had performance issues and these ...
- 迅为i.MX6ULL开发板资料下载,让Linux学习更轻松
迅为电子的 i.MX6ULL 核心板分为工业级和商业级两种.提供的接口是邮票孔方式. 开发板资料下载链接: 链接:https://pan.baidu.com/s/174ob7bzIaf_ls8_O4Q ...
最新文章
- layui 导航收缩代码_pycharm的十个小技巧,让你写代码效率翻倍
- 20110609 搭域控,布线,设计网络,杂事一堆啊
- Java案例:统计文本中所有整数之和
- fpga初始化错误_FPGA的ROM初始化问题讨论
- 10-4 用select进行调度
- 【Leetcode_easy】724. Find Pivot Index
- oracle 查看表空间及用户,oracle 表空间及查看所有用户的表空间
- 脚本、脚本语言、写脚本都是什么呀???
- Python学习笔记——python基础之python中for......else......的使用
- 【python技能树】python简介
- 技术赋能广告策略全升级,爱奇艺开启框内广告营销新篇章
- MySQL多表左右连接查询
- 大学生数学竞赛试题荟萃
- Jvm面试题总结及答案 300道(2022年最新版 )
- Windows Kits(Windows 工具包)
- obj转stl_STL转STP的方法视频教程,OBJ格式转STP或者IGS开模具格式的过程,STL转STP软件介绍...
- Mac电脑QuickTime Player不支持的播放格式
- Java eclipse控制台按任意键返回主菜单 控制台清屏
- MATLAB 基础知识 数据类型 表 添加、删除和重新排列表变量
- WGS84以及各种坐标系
热门文章
- 带感情的语音APP开发笔记(一)
- 东莞理工专插本计算机专业,东莞理工学院2021专插本专业,必看!专插本公办最容易录取的专业!是那些专业?...
- 如何部署会议室多屏同步显示系统
- linux 虚拟机 交叉,华恒s3c2440,虚拟机下redhat-linux交叉环境的搭建初体验
- 约瑟夫环问题(C语言循环链表)
- Node节点、NodeList节点列表
- UnrealText: Synthesizing Realistic Scene Text Images from the Unreal World(译)
- python-docx 页眉-清除原页眉图像插入自适应图像
- daysmatter安卓版_days matter最新版下载-Days Matter倒数日软件下载v2.2.1 安卓版-单机手游网...
- 双馈风力发电机模型研究与仿真(DFIG)控制策略Simulink,给定风速变化,电流电压等波形好用