如何在JavaScript中为元素添加类名?
Adding class names using JavaScript can be often used to give certain functionalities to your web application. In this article, we will learn how to add class names to elements on the DOM through JavaScript?
使用JavaScript添加类名称通常可用于为您的Web应用程序提供某些功能。 在本文中,我们将学习如何通过JavaScript将类名称添加到DOM上的元素?
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>Document</title>
<!-- Compiled and minified CSS -->
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/materialize/1.0.0/css/materialize.min.css">
<!-- Compiled and minified JavaScript -->
<script src="https://cdnjs.cloudflare.com/ajax/libs/materialize/1.0.0/js/materialize.min.js"></script>
</head>
<style>
body {background: yellow;
}
.title {color: white;
}
</style>
<body>
<div class="container">
<h2>Blog Page </h2>
<div class="blog">
<h2 class="center title">How to learn JavaScript</h2>
<div class="card">
<p class="card-content">Lorem ipsum, dolor sit amet consectetur adipisicing elit. Libero, ut provident odit corporis quidem neque in facilis enim! Non possimus provident vitae culpa sed facere voluptatum expedita! Mollitia, rerum porro. Lorem ipsum, dolor sit amet consectetur adipisicing elit. Libero, ut provident odit corporis quidem neque in facilis enim! Non possimus provident vitae culpa sed facere voluptatum expedita! Mollitia, rerum porro. Lorem ipsum, dolor sit amet consectetur adipisicing elit. Libero, ut provident odit corporis quidem neque in facilis enim! Non possimus provident vitae culpa sed facere voluptatum expedita! Mollitia, rerum porro. Lorem ipsum, dolor sit amet consectetur adipisicing elit. Libero, ut provident odit corporis quidem neque in facilis enim! Non possimus provident vitae culpa sed facere voluptatum expedita! Mollitia, rerum porro. Lorem ipsum, dolor sit amet consectetur adipisicing elit. Libero, ut provident odit corporis quidem neque in facilis enim! Non possimus provident vitae culpa sed facere voluptatum expedita! Mollitia, rerum porro. Lorem ipsum, dolor sit amet consectetur adipisicing elit. Libero, ut provident odit corporis quidem neque in facilis enim! Non possimus provident vitae culpa sed facere voluptatum expedita! Mollitia, rerum porro.
</p>
</div>
<button class="left btn">Subscribe</button>
<button class="right btn">Mark as favorite</button>
</div>
</div>
</div>
</body>
<script>
</script>
</html>
Output
输出量
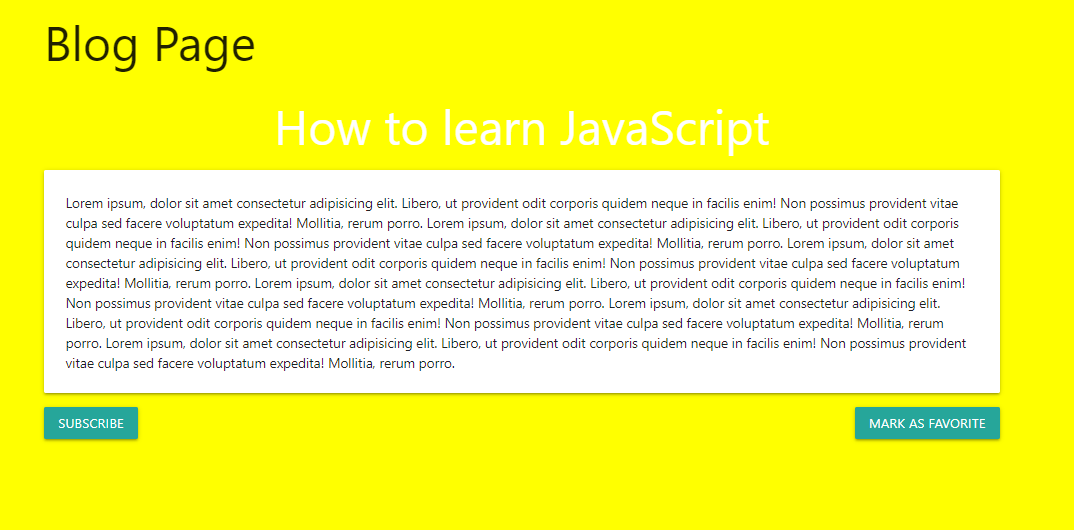
To demonstrate, let's add some materialize classes to some of our elements. I want the title to align in the center and the blog heading to have a black background. Also, I want both our buttons to be black.
为了演示,让我们向一些元素添加一些实现类。 我希望标题在中心对齐,并且博客标题具有黑色背景。 另外,我希望两个按钮都为黑色。
<script>
const title=document.querySelector('h2');
const heading=document.querySelector('.title');
const subBtn=document.querySelector('.sub');
const favBtn=document.querySelector('.fav');
title.classList.add('center');
heading.classList.add('black');
subBtn.classList.add('black');
favBtn.classList.add('black');
</script>
Output
输出量

Great! Our HTML looks much better now. We can add any CSS class to an element using JavaScript by getting a reference to that element, then using the classList property and calling then add method and pass the desired class name as a string inside. Let's say that you want to subscribe to this blog post. We'll add a class which disables the HTML and add this class when we hit the subscribe button.
大! 我们HTML现在看起来好多了。 我们可以使用JavaScript通过向元素添加任何CSS类,方法是获取对该元素的引用,然后使用classList属性并调用然后添加方法,然后将所需的类名称作为字符串传递给内部。 假设您要订阅此博客文章。 我们将添加一个禁用HTML的类,并在单击“订阅”按钮时添加此类。
.subscribed{display: none;
}
Inside our script tag
在我们的脚本标签内
subBtn.addEventListener('click',()=>{alert("You've now subscribed to this blog post!");
subBtn.classList.add('subscribed');
})
Now when we click the subscribe button we get an alert and after that the button vanishes!
现在,当我们单击“订阅”按钮时,我们将收到警报,然后该按钮消失!
Output
输出量

Cool! Let's hook up something to the favorite button as well. When you click it, I want to change its color to pink and give it a border. Can you think of how we'll do this?
凉! 让我们也将一些东西链接到“收藏夹”按钮上。 当您单击它时,我想将其颜色更改为粉红色并为其添加边框。 您能想到我们将如何做吗?
CSS:
CSS:
.fav-triggered {border: 2px solid pink;
color: salmon;
}
JavaScript:
JavaScript:
favBtn.addEventListener('click',()=>{favBtn.classList.add('fav-triggered');
})
Output
输出量

The button is styled differently on clicking!
单击时按钮的样式不同!
Thus loads of functionality can be implemented using add method to give our elements classes using JavaScript.
因此,可以使用add方法来实现功能负载,以使用JavaScript为元素类提供功能。
翻译自: https://www.includehelp.com/code-snippets/how-to-add-a-class-name-to-an-element-in-javascript.aspx
如何在JavaScript中为元素添加类名?相关推荐
- html中p元素添加超链接_如何在HTML中添加超链接
html中p元素添加超链接 How To Build a Website With HTML 如何使用HTML构建网站 This tutorial series will guide you thro ...
- 如何在JavaScript中实现链接列表
If you are learning data structures, a linked list is one data structure you should know. If you do ...
- 如何在javascript中使用多个分隔符分割字符串?
如何在JavaScript中使用多个分隔符拆分字符串? 我正在尝试在逗号和空格上进行拆分,但是AFAIK,JS的拆分功能仅支持一个分隔符. #1楼 对于那些想要在拆分功能中进行更多自定义的人,我编写了 ...
- 如何在JavaScript中区分深层副本和浅层副本
by Lukas Gisder-Dubé 卢卡斯·吉斯杜比(LukasGisder-Dubé) 如何在JavaScript中区分深层副本和浅层副本 (How to differentiate betw ...
- 如何在JavaScript中直观地设计状态
by Shawn McKay 肖恩·麦凯(Shawn McKay) 如何在JavaScript中直观地设计状态 (How to visually design state in JavaScript) ...
- html 获取文本框值,html - 如何在JavaScript中获取文本框值
html - 如何在JavaScript中获取文本框值 我正在尝试使用JavaScript从HTML文本框中获取值,但值不是在空格之后 例如: 我只得到:上面的"软件". 我正在使 ...
- regexp 好汉字符串_如何在JavaScript中使用RegExp确认字符串的结尾
regexp 好汉字符串 by Catherine Vassant (aka Codingk8) 由凯瑟琳·瓦森(Catherine Vassant)(又名Codingk8) 如何在JavaScrip ...
- !! javascript_产量! 产量! 生成器如何在JavaScript中工作。
!! javascript by Ashay Mandwarya ?️?? 由Ashay Mandwarya提供吗? 产量! 产量! 生成器如何在JavaScript中工作. (Yield! Yiel ...
- javascript案例_如何在JavaScript中使用增强现实-一个案例研究
javascript案例 by Apurav Chauhan 通过Apurav Chauhan 如何在JavaScript中使用增强现实-一个案例研究 (How to use Augmented Re ...
最新文章
- Keil5 STM32F系列 安装 安装包
- 刚刚,我国智能科学技术最高奖揭晓!
- 记录一下HALCON基于可变形,利用CAD画dxf模板进行模板匹配(二)
- TCP快速重传为什么是三次冗余ack,这个三次是怎么定下来的?
- c#中怎样取得某坐标点的颜色
- 解题:POI 2004 String
- requests库提示警告:InsecureRequestWarning: Unverified HTTPS request is being made. Adding certificate ver
- centos重置系统_双系统下Linux系统无法启动及其引导丢失之解决
- vue下拉框值改变事件_vue和element ui 下拉框select的change事件
- Qt学习笔记-简单的UDP广播包聊天室
- [转]context-param和init-param区别 context-param和in...
- [NPOI2.0] 使用NPOI读取和导出Excel文件
- 高淇Struts2.0教程之视频笔记(4)
- 各种格式的文件使用工具打开
- Hadoop,HBASE启动命令
- Secure CRT 最大显示行数的设置
- c语言 四层电梯算法,电梯算法c语言
- ccProxy软件实现网络共享
- 毕业设计 - 题目:基于FP-Growth的新闻挖掘算法系统的设计与实现
- Exchange邮箱服务器后利用
热门文章
- 安装paddle 出错
- “lib32ncurses5 : Depends: libc6-i386 (>= 2.18) but it is not going to be installed”类似问题解决办法
- dpkg was interrupted, you must manually run 'dpkg --configure -a'
- 机器学习中如何评价模型的好坏
- 微软 Win快捷键大全
- windows快捷键自定义_在Windows中创建自定义Windows键盘快捷键
- Python标准库和第三方库简介
- Docker从安装到卸载
- Python urlencode编码和urldecode解码
- 电路噪声的产生以及抑制噪声的方法