一个OOP的课程设计,不难实现,贴出来请大家指正。
提高1:用TDD的方法该如何写这个类呢?希望有朋友来讲一讲。
提高2:重构该如何进行?很多朋友问起过这个,希望有朋友可以稍微详细讲一下重构的一些基础知识和心得,最好针对这段代码有个例子。
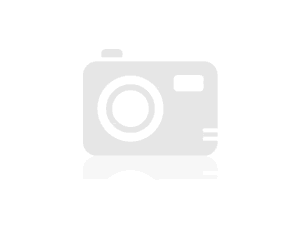
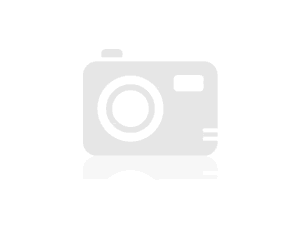
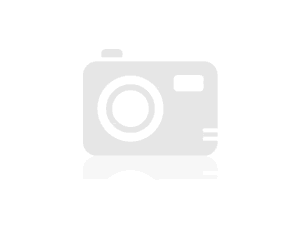
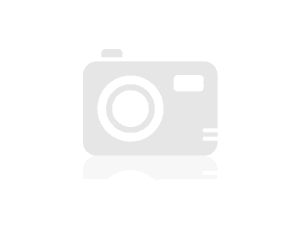
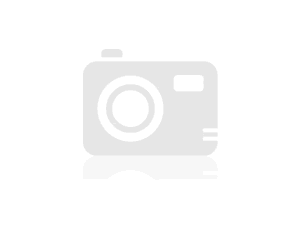


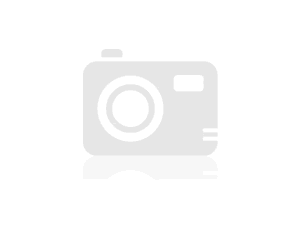









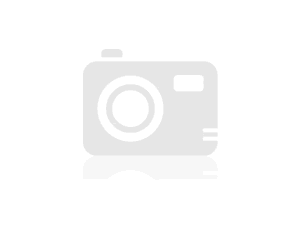











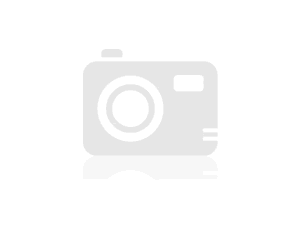



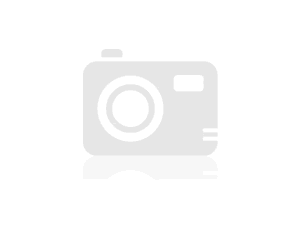








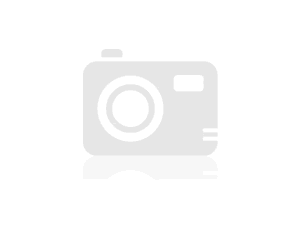













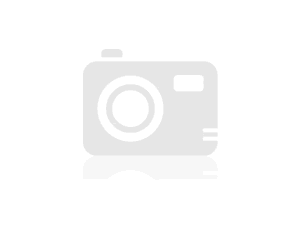







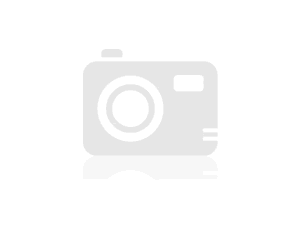





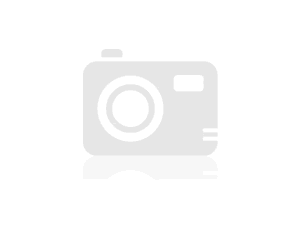







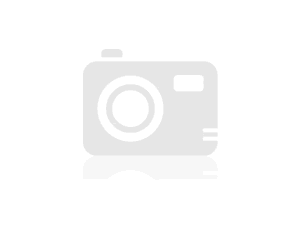






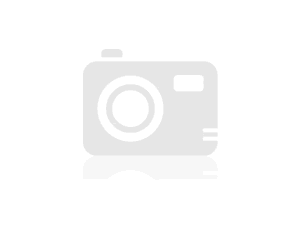










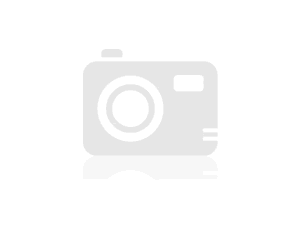



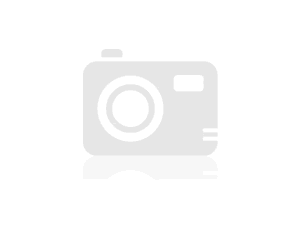





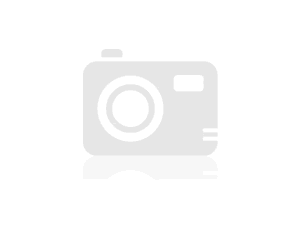









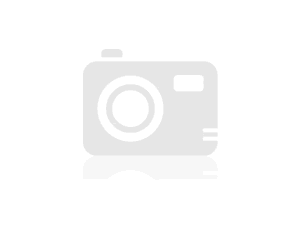



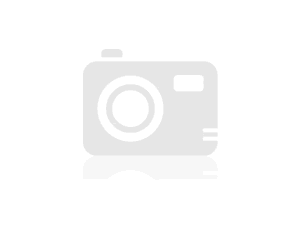





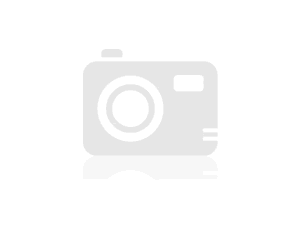









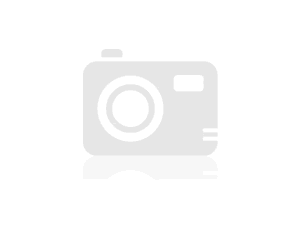



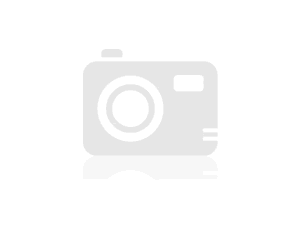






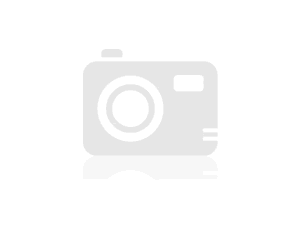





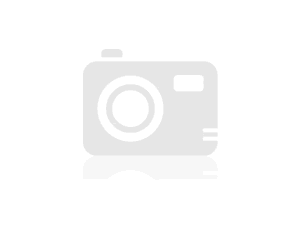










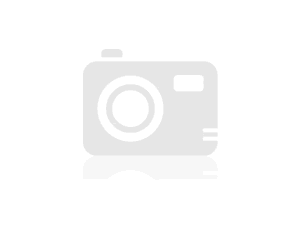












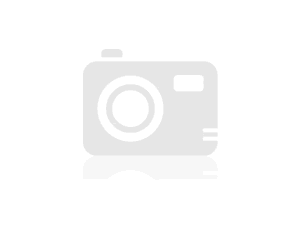








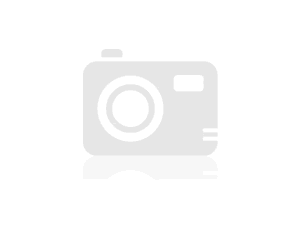




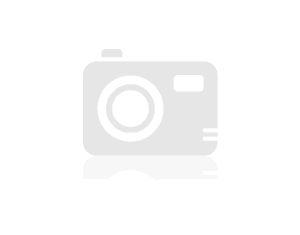
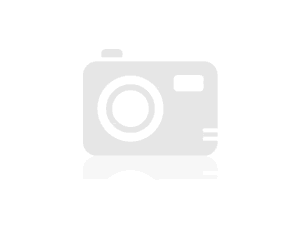
一个OOP的课程设计,不难实现,贴出来请大家指正。相关推荐
- java 设计一个动物类_Java课程设计(动物换位)
[实例简介] 是一个关于Java课程设计的一个游戏,这是一个动物换位的游戏,是在前人的基础上改进的.不好请见谅! [实例截图] [核心代码] 112df6fb-1189-4bc0-a501-6dd58 ...
- c语言课程设计报告-计算器的实现,C语言课程设计--一个简易计算器的设计与实现.doc...
C语言课程设计--一个简易计算器的设计与实现 扬 州 大 学 ------------------1 程序设计内容:------------------1 课程设计所补充的内容:补充的函数或算法--- ...
- 关于课程设计、毕业设计的一些总结与思考
研究生期间陆续帮一些老师带了一些本科生的课或者课程设计,今年也帮老师带了几十个学生的毕业设计,参与了毕业设计的检查和验收,因而有机会接触了更多的同学,也从很多更为优秀的同学那里学习到了很多东西.可能和 ...
- 计算机课程设计结业感言,课程设计感言
微课程的设计与制作培训感言.微课程的设计与制作培训感言. 课程设计感言2017-07-29 19:45:41 | #1楼回目录 将近一个月的课程设计结束了,在这次的课程设计中不仅检验了我所学习的知识, ...
- c语言程序设计 在线课程设计,c语言程序设计 本科课程设计
<c语言程序设计 本科课程设计>由会员分享,可在线阅读,更多相关<c语言程序设计 本科课程设计(11页珍藏版)>请在人人文库网上搜索. 1.河北农业大学本 科 课 程 设 计课 ...
- c语言课程设计报告停车系统,停车场管理系统C语言课程设计
<停车场管理系统C语言课程设计>由会员分享,可在线阅读,更多相关<停车场管理系统C语言课程设计(27页珍藏版)>请在人人文库网上搜索. 1.计算机科学与技术系课程设计报告20 ...
- java设计五子棋_JAVA课程设计+五子棋(团队博客)
JAVA课程设计 利用所学习的JAVA知识设计一个五子棋小游戏 1.团队名称.团队成员介绍(菜鸟三人组) 杨泽斌[组长]:201521123049 网络1512 叶文柠[组员]:20152112305 ...
- 【计算机毕业设计】50.课程设计管理系统
一.系统截图(需要演示视频可以私聊) 摘 要 网络的广泛应用给生活带来了十分的便利.所以把课程设计管理与现在网络相结合,利用JSP技术建设课程设计管理系统,实现课程设计管理的信息化.则对于进一步提高 ...
- 计算机专业课程设计论文,课程设计学生论文,关于计算机专业课程设计教学改进相关参考文献资料-免费论文范文...
导读:本论文可用于课程设计学生论文范文参考下载,课程设计学生相关论文写作参考研究. 孙克雷 吴观茂 (安徽理工大学计算机科学与工程学院 安徽淮南 232001) 摘 要:计算机专业开设课程设计是培养学 ...
最新文章
- 【论文相关】盘点AAAI2020中的四篇推荐系统好文
- 前端教程之Intro.js轻松实现新手引导效果
- ES6的导入和导出模块
- 光纤通道如何过渡到SAN
- 创业冲突的五种解决方法是_失眠原因不同,中医五种调理方法解决问题!
- kotlin 查找id_Kotlin程序查找平行四边形的区域
- C语言错误处理方法、C++异常处理方法(throw, try, catch)简介
- linux suse 安装redis,suse 安装redis(示例代码)
- 转ORA-28002: the password will expire within 7 days 解决方法
- 8个经典智能穿戴设备优选电路方案合辑
- (vue)h5 通过高德地图(原生) 获取当前位置定位
- 位偏移 java_时区和偏移类 / Zone and Offset
- 可中心可边缘,云计算“罗马大路”需要什么样的超融合新基建?
- 淘宝首页幻灯片(二) 居中按钮源代码
- OPPO小布4.0:软件定义硬件,智能定义“助手”
- [026] 深度学习--学习笔记(4)Back-propagation反向传播链式法则理论推导
- 我数星星...宝宝,你智商差点,就数月亮吧
- 青蛙现象、鳄鱼法则、鲇鱼效应、羊群效应、刺猬法则、手表定律、破窗理论、二八定律、木桶理论、马太效应
- 2022年双十一洗面奶选购指南
- ImageWatch2019下载和安装