01.自定义View(ArcView弧形进度条)
开始重新学习一下自定义View的相关知识,借鉴了一些网上的文章,目前在跟这位博主学习,大家可以关注一下
作者:红橙Darren
链接:https://www.jianshu.com/p/0a32e81c3c89
來源:简书
ArcView
实现效果
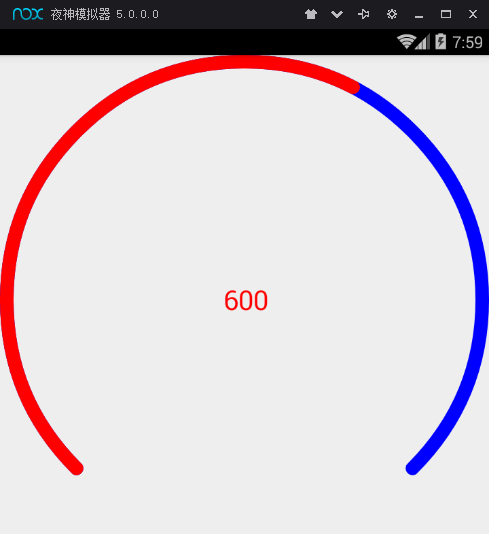
package com.example.mylibrary.view;import android.content.Context;
import android.content.res.TypedArray;
import android.graphics.Canvas;
import android.graphics.Color;
import android.graphics.Paint;
import android.graphics.Rect;
import android.graphics.RectF;
import android.support.annotation.Nullable;
import android.util.AttributeSet;
import android.view.View;import com.example.mylibrary.R;/*** Created by renzhenming on 2018/3/22.* 圆弧形的进度条*/public class ArcView extends View{private final Paint mBackgroundPaint;private final Paint mForegroundPaint;private final Paint mTextPaint;//中间字体颜色private int mCenterTextColor = Color.RED;//中间字体大小private int mCenterTextSize = 40;//进度条宽度private float mBorderWidth = 20;//进度条已完成部分颜色private int mForegroundColor = Color.RED;//进度条进行中部分颜色private int mBackgroundColor = Color.BLUE;//总量和当前量private float mTotalPercent = 0f;private float mCurrentPercent = 0f;public ArcView(Context context) {this(context,null);}public ArcView(Context context, @Nullable AttributeSet attrs) {this(context, attrs,-1);}public ArcView(Context context, @Nullable AttributeSet attrs, int defStyleAttr) {super(context, attrs, defStyleAttr);TypedArray typedArray = context.obtainStyledAttributes(attrs, R.styleable.ArcView);mBackgroundColor = typedArray.getColor(R.styleable.ArcView_backgroundColor, mBackgroundColor);mForegroundColor = typedArray.getColor(R.styleable.ArcView_foregroundColor, mForegroundColor);mBorderWidth = typedArray.getDimension(R.styleable.ArcView_borderWidth,mBorderWidth);mCenterTextSize = typedArray.getDimensionPixelSize(R.styleable.ArcView_centerTextSize,mCenterTextSize);mCenterTextColor = typedArray.getColor(R.styleable.ArcView_centerTextColor,mCenterTextColor);typedArray.recycle();mBackgroundPaint = new Paint();mBackgroundPaint.setAntiAlias(true);mBackgroundPaint.setStrokeWidth(mBorderWidth);mBackgroundPaint.setColor(mBackgroundColor);//STROKE 画出来的圆弧不是封闭的,FILL是封闭的mBackgroundPaint.setStyle(Paint.Style.STROKE);mBackgroundPaint.setDither(true);//Paint.Cap.ROUND设置圆弧首尾位置是ROUND的,不是直角//Paint.Cap.SQUARE默认的是直角mBackgroundPaint.setStrokeCap(Paint.Cap.ROUND);mForegroundPaint = new Paint();mForegroundPaint.setAntiAlias(true);mForegroundPaint.setStrokeWidth(mBorderWidth);mForegroundPaint.setDither(true);mForegroundPaint.setColor(mForegroundColor);//STROKE 画出来的圆弧不是封闭的,FILL是封闭的mForegroundPaint.setStyle(Paint.Style.STROKE);//Paint.Cap.ROUND设置圆弧首尾位置是ROUND的,不是直角//Paint.Cap.SQUARE默认的是直角mForegroundPaint.setStrokeCap(Paint.Cap.ROUND);mTextPaint = new Paint();mTextPaint.setAntiAlias(true);mTextPaint.setDither(true);mTextPaint.setColor(mCenterTextColor);mTextPaint.setTextSize(mCenterTextSize);}@Overrideprotected void onMeasure(int widthMeasureSpec, int heightMeasureSpec) {super.onMeasure(widthMeasureSpec, heightMeasureSpec);//如果宽度和高度不一样,取最小值,确保是个正方形int width = MeasureSpec.getSize(widthMeasureSpec);int height = MeasureSpec.getSize(heightMeasureSpec);setMeasuredDimension(width>height?height:width,width>height?height:width);}@Overrideprotected void onDraw(Canvas canvas) {super.onDraw(canvas);//画背景,RectF rectF = new RectF(mBorderWidth / 2, mBorderWidth / 2, getWidth() - mBorderWidth / 2, getHeight() - mBorderWidth / 2);//false表示圆弧不闭合,绘制的宽度也要占空间,所以RectF的设置上下左右要考虑去掉宽度的一般,猜测,//绘制的时候是从边缘宽度的中心开始计算的canvas.drawArc(rectF, 135, 270, false, mBackgroundPaint);//画进行中的进度if (mTotalPercent ==0 )return;if ( mCurrentPercent > mTotalPercent){mCurrentPercent = mTotalPercent;}float sweepAngle = mCurrentPercent/mTotalPercent;canvas.drawArc(rectF,135,sweepAngle*270,false,mForegroundPaint);//画文字String s = ((int)mCurrentPercent) + "";Rect bounds = new Rect();mTextPaint.getTextBounds(s,0,s.length(),bounds);//获取文字的起始位置,文字位于控件中间,所以起始位置等于控件宽度的//一半减去文字宽度的一半int start = getWidth()/2-bounds.width()/2;Paint.FontMetrics metrics = mTextPaint.getFontMetrics();//文字高度//文字中心点距离底部的距离int dy = (int) ((metrics.bottom-metrics.top)/2-metrics.bottom);//获取基线baselineint baseLine = getHeight()/2+dy;canvas.drawText(s,start,baseLine,mTextPaint);}/*** 设置总量* @param totalProgress*/public synchronized void setTotalProgress(int totalProgress){this.mTotalPercent = totalProgress;}/*** 设置当前量* @param currentProgress*/public synchronized void setCurrentProgress(int currentProgress){this.mCurrentPercent = currentProgress;invalidate();}
}
attrs
<!--半圆弧进度条--><declare-styleable name="ArcView"><attr name="backgroundColor" format="color"/><attr name="foregroundColor" format="color"/><attr name="borderWidth" format="dimension"/><attr name="centerTextSize" format="dimension"/><attr name="centerTextColor" format="color"/></declare-styleable>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"xmlns:tools="http://schemas.android.com/tools"android:layout_width="match_parent"android:layout_height="match_parent"tools:context="com.app.rzm.test.TextCustomeViewActivity"><com.example.mylibrary.view.ArcViewandroid:id="@+id/arcView"android:layout_width="match_parent"android:layout_height="match_parent" />
</LinearLayout>
测试
public class TextCustomeViewActivity extends AppCompatActivity {@Overrideprotected void onCreate(Bundle savedInstanceState) {super.onCreate(savedInstanceState);setContentView(R.layout.activity_text_custormer_view);final ArcView mArcView = (ArcView) findViewById(R.id.arcView);mArcView.setTotalProgress(1000);ValueAnimator animator = ValueAnimator.ofFloat(0,2000);animator.setDuration(2000);animator.start();animator.addUpdateListener(new ValueAnimator.AnimatorUpdateListener() {@Overridepublic void onAnimationUpdate(ValueAnimator animation) {float currentProgress = (float) animation.getAnimatedValue();mArcView.setCurrentProgress((int) currentProgress);}});}
}
01.自定义View(ArcView弧形进度条)相关推荐
- android自定义view 模仿win10进度条
android自定义view 模仿win10进度条 本文已授权微信公众号:鸿洋(hongyangAndroid)在微信公众号平台原创首发. PS:有朋友反映动画无法播放,那是因为PathMeasure ...
- android自定义view圆环,Android自定义View实现圆环进度条
本文实例为大家分享了android自定义view实现圆环进度条的具体代码,供大家参考,具体内容如下 效果展示 动画效果 view实现 1.底层圆环是灰色背景 2.上层圆环是红色背景 3.使用动画画一条 ...
- Android自定义view之圆形进度条
本节介绍自定义view-圆形进度条 思路: 根据前面介绍的自定义view内容可拓展得之: 1:新建类继承自View 2:添加自定义view属性 3:重写onDraw(Canvas canvas) 4: ...
- 自定义View加载进度条首页面
1.主页面布局 <?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:an ...
- Android自定义View之圆弧形进度条,支持背景图片设置
Android下自定义的一款圆弧形进度条,支持中心图片设置,有兴趣的朋友可以尝试下. Github地址点击打开链接 package com.julyapp.progressdemo.view;impo ...
- android 自定义进度条 水量,Android自定义带水滴的进度条样式(带渐变色效果)...
一.直接看效果 二.直接上代码 1.自定义控件部分 package com.susan.project.myapplication; import android.app.Activity; impo ...
- android 动态改变进度条,Android条纹进度条的实现(调整view宽度仿进度条)
Android条纹进度条的实现(调整view宽度仿进度条) 发布时间:2020-10-03 16:14:24 来源:脚本之家 阅读:89 作者:RustFisher 前言 本文主要给大家介绍了关于An ...
- 弧形进度条(动画版)
本博客只要没有注明"转",那么均为原创,转贴请注明本博客链接链接 我们先把问题分解为下面3个小问题. 1.如何画一个弧形 2.如何让弧形带有加载过程 3.如何让进度值随着圆弧一起转 ...
- php怎样获取视频播放的进度条,小程序如何实现视频或音频自定义可拖拽进度条...
本篇文章给大家带来的内容是关于小程序如何实现视频或音频自定义可拖拽进度条,有一定的参考价值,有需要的朋友可以参考一下,希望对你有所帮助. 小程序原生组件的音频播放时并没有进度条的显示,而此次项目中,鉴 ...
- android 自定义view滚动条,Android自定义View实现等级滑动条的实例
Android自定义View实现等级滑动条的实例 实现效果图: 思路: 首先绘制直线,然后等分直线绘制点: 绘制点的时候把X值存到集合中. 然后绘制背景图片,以及图片上的数字. 点击事件down的时候 ...
最新文章
- 苹果禁止使用热更新 iOS开发程序员新转机来临
- C 语言——字符串和格式化输入/输出
- 2008R2文件服务器迁移到2012R2
- 在php中单引号和双引号的区别错误的是___________,浅谈PHP中单引号和双引号到底有啥区别呢?...
- 上云、微服务化和DevOps,少走弯路的办法
- import Vue form 'vue’的意思
- Kick Start 2019 Round D
- MySQL高级 - 锁 - InnoDB行锁 - 介绍及背景知识
- android sdk eclipse没导入,Android—新的eclipse导入SDK出错解决办法
- P1791-[国家集训队]人员雇佣【最大权闭合图】
- React开发(201):react代码分割之打包导出
- 记录一个美丽的小县城
- 2017.0704.《计算机组成原理》-动态RAM
- 攻略:简易病毒制作(Windows)
- 加好友饥荒服务器没有响应,饥荒进不去别人的服务器 | 手游网游页游攻略大全...
- 从“Liskov替换原则”和“Refused Bequest”看“正方形为什么不能继承长方形”
- 12306对抢票软件“下手”了 1
- 从招式与内功谈起——设计模式概述(三)
- 网页连接至数据库(asp->mdb)
- 【NOIP模拟题】【DP】【LIS】【中缀表达式】2016.11.15 第一题 小L的二叉树 题解