在Python中使用OpenCV裁剪图像
What is Cropping?
什么是播种?
Cropping is the removal of unwanted outer areas from a photographic or illustrated image. The process usually consists of the removal of some of the peripheral areas of an image to remove extraneous trash from the picture, to improve its framing, to change the aspect ratio, or to accentuate or isolate the subject matter from its background.
裁剪是从摄影或插图图像中去除不需要的外部区域。 该过程通常包括去除图像的某些外围区域,以从图片中去除多余的垃圾,以改善其取景,改变纵横比,或者使主题与背景突出或隔离。
We will be using these functions of OpenCV - python(cv2),
我们将使用OpenCV的这些功能-python(cv2),
imread(): This function is like it takes an absolute path of the file and reads the whole image, and after reading the whole image it returns us the image and we will store that image in a variable.
imread() :此函数就像它采用文件的绝对路径并读取整个图像一样,在读取整个图像之后,它将返回图像并将图像存储在变量中。
imshow(): This function will be displaying a window (with a specified window name) which contains the image that is read by the imread() function.
imshow() :此函数将显示一个窗口(具有指定的窗口名称),该窗口包含由imread()函数读取的图像。
shape: This function will return the height, width, and layer of the image
形状 :此函数将返回图像的高度 , 宽度和层
Let’s take an example,
让我们举个例子
Let there be a list a=[1,2,3,4,5,6,7,8,9]Now, here I just wanted the elements between 4 and 8
(including 4 and 8) so what we will do is :print(a[3:8])
The result will be like : [4,5,6,7,8]
裁剪图像的Python程序 (Python program to crop an image)
# importing the module
import cv2
img=cv2.imread("/home/abhinav/PycharmProjects/untitled1/a.jpg")
# Reading the image with the help of
# (specified the absolute path)
# imread() function and storing it in the variable img
cv2.imshow("Original Image",img)
# Displaying the Original Image Window
# named original image
# with the help of imshow() function
height,width=img.shape[:2]
# storing height and width with the help
# of shape function as shape return us
# three things(height,width,layer) in the form of list
# but we wanted only height and width
start_row,start_col=int(width*0.25),int(height*0.25)
end_row,end_col=int(width*0.75),int(height*0.75)
# start_row and start_col are the cordinates
# from where we will start cropping
# end_row and end_col is the end coordinates
# where we stop
cropped=img[start_row:end_row,start_col:end_col]
# using the idexing method cropping
# the image in this way
cv2.imshow("Cropped_Image",cropped)
# using the imshow() function displaying
# another window of
# the cropped picture as cropped contains
# the part of image
cv2.waitKey(0)
cv2.destroyAllWindows()
Output:
输出:
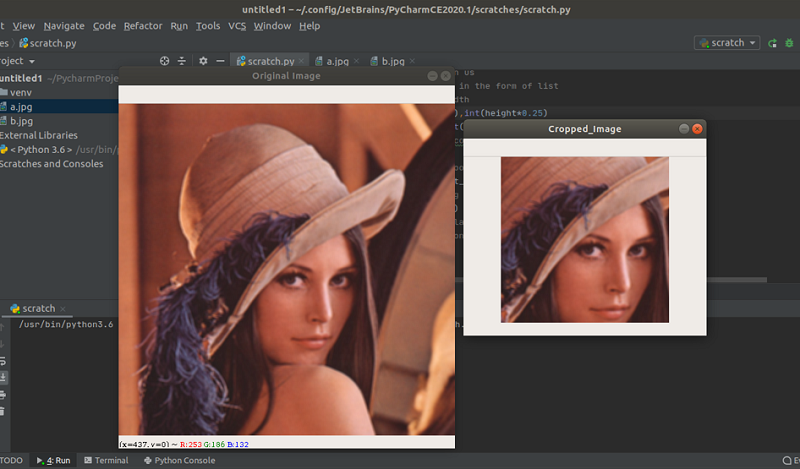
You can see the Cropped Image and the Original Image(the cropping is done like 0.25 to 0.75 with row and with column 0.25 to 0.75, and you can change the number).
您可以看到“裁剪的图像”和“原始图像”(裁剪的过程类似于0.25到0.75行和0.25到0.75列,并且可以更改数字)。
翻译自: https://www.includehelp.com/python/cropping-an-image-using-opencv.aspx
在Python中使用OpenCV裁剪图像相关推荐
- OpenCV入门(C++/Python)-使用OpenCV裁剪图像(四)
裁剪是为了从图像中删除所有不需要的物体或区域.甚至突出显示图像的特定功能. 使用OpenCV裁剪没有特定的功能,NumPy数组切片是工作.读取的每个图像都存储在2D数组中(对于每个颜色通道).只需指定 ...
- 如何在 Python 中使用 OpenCV 在图像上添加水印
简介 水印是企业和在线内容信用标记的重要组成部分.它可以以标志.签名或印章的形式出现,对创作者来说是独一无二的.在向数字世界中的对象创建者提供所有权或信用时,它是一个非常重要的工具. 大多数专业人士使 ...
- python图片截取特定部分_如何在Python中使用OpenCV提取图像的特定部分?
我正在尝试通过执行精明的边缘检测来提取图像的一部分.我已经成功地创建了该对象的掩码.但是,当我对原始图像执行bitwise_and操作以提取前景部分时,会出现以下错误.OpenCV Error: As ...
- 8.openCV 裁剪图像
8.openCV 裁剪图像 一.使用 OpenCV 裁剪图像 二.项目结构和代码讲解 1.项目结构 2.代码讲解 三.代码下载 一.使用 OpenCV 裁剪图像 在本教程的第一部分,我们将讨论如何将 ...
- 在 Python 中使用 OpenCV 高斯模糊我这张的丑脸
@Author:Runsen 谁都无法否认,长得好看的人就是更具有吸引力,赏心悦目谁都喜欢.好看的人无论在职场或情场,都一定更占优势. 但是,此「颜值」非彼「颜值」.一说到「颜值」,大部分想到的是脸蛋 ...
- OpenCV裁剪图像任意区域
目录 1.获取选定图中的矩形ROI 2.裁剪任意多边形 1.获取选定图中的矩形ROI 利用矩形 Rect 框定,指定其左上角坐标(构造函数前两个参数)和矩形的 长宽(后两个参数) //定义一个 Mat ...
- 在python中使用opencv自带函数转换转换RBG和BGR
在python中使用opencv自带函数转换图像的R通道和B通道 RGB -> BGR img_bgr = cv2.cvtColor(img_rgb, cv2.COLOR_RGB2BGR) BG ...
- python中matplotlib自定义设置图像标题使用的字体类型:获取默认的字体族及字体族中对应的字体、自定义设置图像标题使用的字体类型
python中matplotlib自定义设置图像标题使用的字体类型:获取默认的字体族及字体族中对应的字体.自定义设置图像标题使用的字体类型 目录
- python matplotlib 显示opencv的图像
python matplotlib 显示opencv的图像 首先需要import import cv2 import numpy as np from matplotlib import pyplot ...
最新文章
- Linux 操作系统原理 — loop 伪设备
- gitblit无法安装windows服务或者启动服务失败:Failed creating java
- Assigning to Classes CodeForces - 1300B
- p2p 源码 linux,我们打算开发一个WiFi功能的P2P文件共享系统在Linux平台…
- php界面框架luy_LazyPHP
- flask mysql环境配置_Flask教程4:数据库
- python科学计算-01程序包和API简介
- 嵌入式Linux开发工具
- 【微信小程序】解决代码上传超过大小限制,小程序分包
- 打开计算机硬盘有声音,电脑硬盘有响声总吱吱响的解决方法
- agp计算机组装什么意思,教你怎么组装电脑
- 算法学习:501.二叉搜索树中的众数
- BT技术概念 — 一些术语的意思
- 小米9 淘宝 618列车加油 脚本 Android10 MIUI11
- 如何下载金融街街道卫星地图高清版大图
- 十进制浮点数的表示方法
- Axure 9 案例教程进阶篇之课程简介(带你玩转高交互设计)
- Linux Alsa声卡驱动(2):代码分析
- centos7-汉化vim帮助指令文档
- docker容器添加微软雅黑字体
热门文章
- strtotime()加半个小时_椰子鸡这样做太好吃了,一滴水不用加,鲜香嫩滑,做法非常简单...
- dokcer mysql修改编码_默认支持utf8编码的mysql docker镜像
- unity着色器和屏幕特效开发秘笈_Oculus研发分享:开发移动VR内容时应避免的PC渲染技术...
- nginx中的location指令
- Docker原理之UnionFS
- Epson C1100报错“Service Req E511”的处理方法
- PowerDesigner导出表为Excel(转)
- 《Web安全之机器学习入门》一 第3章 机器学习概述
- 《Spark核心技术与高级应用》——3.2节构建Spark的开发环境
- 【HM】第2课:JavaScript基础