扩展可以支持枚举类型的DorpDownList控件
我们有很多时候,都会定义一些枚举类型的变量,而我们用的时候,也习惯用DropDownList绑定,绑定的方式也有好多种,比如集合,泛型集合等等。
比如:我们定义一个图片后缀名的枚举类型
{
Jpg = 0,
Gif =1,
Png =2
}
如果我们想用这个枚举类型来作为DropDownList的数据源的话,我们可以这么做:
{
if(!Page.IsPostBack)
{
ddlEnumTypeBind();
}
}
private void ddlEnumTypeBind()
{
this.ddlenumtype.DataSource = Enum.GetNames(typeof(PicType));
this.ddlenumtype.DataBind();
}
但是用以上代码,DropDownList呈现的DataTextField和DataValueField都是枚举类型的字符表示也就是(Jpg,Gif..),得到它的Value ,我们可以这样:
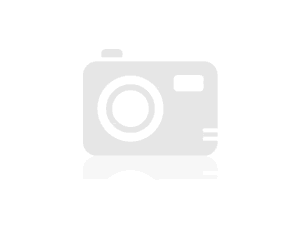
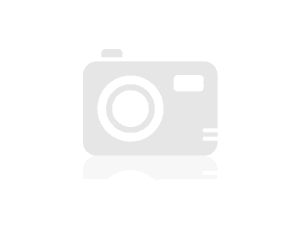
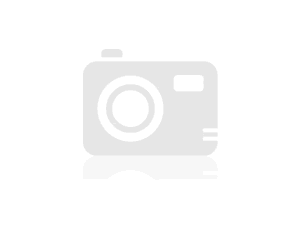



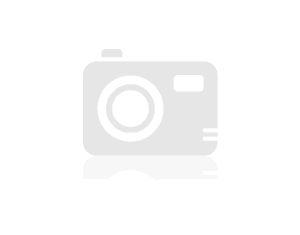
当然,如果我们想要得到枚举类型的数字值,我们可以用泛型(保证类型安全),集合等等。
泛型Code:
{
Type myEnumType = typeof(T);
if (myEnumType.BaseType != typeof(Enum))
{
throw new ArgumentException("Type T must inherit from System.Enum.");
}
SortedList<string, int> returnCollection = new SortedList<string, int>();
string[] enumNames = Enum.GetNames(myEnumType);
for (int i = 0; i < enumNames.Length; i++)
{
returnCollection.Add(enumNames[i],
(int)Enum.Parse(myEnumType, enumNames[i]));
}
return returnCollection;
}
集合Code:
{
ArrayList aList = new ArrayList();
foreach (int i in Enum.GetValues(type))
{
ListItem listItem = new ListItem(Enum.GetName(type, i), i.ToString());
aList.Add(listItem);
}
return aList;
}
在这里我只说明用泛型作为DropDownList的数据源,集合类似
{
this.ddlenumtype.DataSource = GetEnumDataSource<PicType>();
this.ddlenumtype.DataTextField = "Key";
this.ddlenumtype.DataValueField = "Value";
this.ddlenumtype.DataBind();
}
现在,我们写了一系列的代码,说明怎样用枚举类型作为DropDownlist的数据源,但是我们可以发现如果一个页面要用到许多这样的代码,或者多个页面也要用到这样的代码,那么我们的代码是不是有好多的冗余呢,又怎么管理呢?所以我们可以把这个封装到控件中,扩展一下DropDownList,使其支持枚举类型。
Code like the following:
using System.Data;
using System.Configuration;
using System.Web;
using System.Web.Security;
using System.Web.UI;
using System.Web.UI.WebControls;
using System.Web.UI.WebControls.WebParts;
using System.Web.UI.HtmlControls;
using System.Collections.Generic;
using System.ComponentModel;
namespace Xbf.Common
{
/// <summary>
/// EnumDorpDownList 的摘要说明
/// </summary>
public class EnumDorpDownListControl<T> : System.Web.UI.WebControls.DropDownList where T : struct
{
public EnumDorpDownListControl()
{
//
// TODO: 在此处添加构造函数逻辑
//
}
/// <summary>
/// 是否显示全部选项
/// </summary>
private bool _showAllOption = false;
public bool ShowAllOption
{
get
{
return _showAllOption;
}
set
{
_showAllOption = value;
}
}
public new T? SelectedValue
{
get
{
if (String.IsNullOrEmpty(this.SelectedItem.Text))
{
return null;
}
return (T)Enum.Parse(typeof(T), this.SelectedItem.Text);
}
}
public int? SelectedIntegerValue
{
get
{
if (ShowAllOption && this.SelectedIndex == 0)
{
return null;
}
if (String.IsNullOrEmpty(this.SelectedItem.Text))
{
return null;
}
return (int)Enum.Parse(typeof(T), this.SelectedValue.ToString());
}
}
protected override void OnInit(EventArgs e)
{
base.OnInit(e);
if (typeof(T).BaseType != typeof(Enum))
{
throw new ArgumentException("Type T must inherit from System.Enum.");
}
BindData();
}
/// <summary>
/// 数据绑定
/// </summary>
protected void BindData()
{
this.DataSource = GetEnumDataSource<T>();
this.DataTextField = "Key";
this.DataValueField = "Value";
this.DataBind();
if (ShowAllOption)
{
this.Items.Insert(0, new ListItem("All", ""));
}
}
public static SortedList<string, int> GetEnumDataSource<T>() where T : new()
{
Type myEnumType = typeof(T);
if (myEnumType.BaseType != typeof(Enum))
{
throw new ArgumentException("Type T must inherit from System.Enum.");
}
SortedList<string, int> returnCollection = new SortedList<string, int>();
string[] enumNames = Enum.GetNames(myEnumType);
for (int i = 0; i < enumNames.Length; i++)
{
returnCollection.Add(enumNames[i],
(int)Enum.Parse(myEnumType, enumNames[i]));
}
return returnCollection;
}
}
}
页面调用代码:
protected override void OnInit(EventArgs e)
{
base.OnInit(e);
ProposalStatusDropDownList1 = new EnumDorpDownListControl<PicType>();
this.PlaceHolder1.Controls.Add(ProposalStatusDropDownList1);
}
在这里,DropDownList被当作PlaceHolder的子控件显示在页面上,如果要取得其中的值可以这样:
{
this.Label1.Text = ProposalStatusDropDownList1.SelectedIntegerValue.ToString();
}
是不是比先前的那个好多了,但是还有一个不方便之处就是不支持拖放,因为自己才疏学浅,没有能把这个改造成可以拖放的,所以在此如果哪位能帮忙改造一下请在以下贴出或留下链接地址,在此先谢了。
文章出处:http://aspalliance.com/1514_Extending_the_DropDownList_to_Support_Enums.all
转载于:https://www.cnblogs.com/xbf321/archive/2008/02/04/extending-the-dorpdownlist-to-support-enum.html
扩展可以支持枚举类型的DorpDownList控件相关推荐
- java实体类中有枚举类型_实体类的枚举属性--原来支持枚举类型这么简单,没有EF5.0也可以...
通常,我们都是在业务层和界面层使用枚举类型,这能够为我们编程带来便利,但在数据访问层,不使用枚举类型,因为很多数据库都不支持,比如我们现在用的SqlServer2008就不支持枚举类型的列,用的时候也 ...
- 解决CPC撰写文档报错问题“无法获取“AxforApplication”控件的窗口句柄。不支持无窗口的 ActiveX 控件”
解决CPC撰写文档报错问题"无法获取"AxforApplication"控件的窗口句柄.不支持无窗口的 ActiveX 控件" 参考文章: (1)解决CPC撰写 ...
- firefox扩展开发(四) : 更多的窗口控件
firefox扩展开发(四) : 更多的窗口控件 2008-06-11 17:00 标签盒子 标签盒子是啥?大家都见过,就是分页标签: 对应的代码: <?xml version="1. ...
- (ESC IIS笔记)类型“TextBox”的控件“TextBox1”必须放在具有 runat=server 的窗体标记内
问题出现 在VisualStudio创建Web窗体并对页面内容进行删改时,出现这样的问题 "类型"TextBox"的控件"TextBox1"必须放在具 ...
- 类型“RadioButton”的控件“radTitle”必须放在具有 runat=server 的窗体标记内。
刚开始做东西,就遇到了一个小问题,类型"RadioButton"的控件"radTitle"必须放在具有 runat=server 的窗体标记内,查了下解决起来还 ...
- 类型“DropDownList”的控件“ContentPlaceHolder1_ddlDepartment”必须放在具有 runat=server 的窗体标记内。
小编今天在开发时运行出现报错黄页,图中提示信息为"类型"DropDownList"的控件"ContentPlaceHolder1_ddlDepartment&q ...
- 类型“GridView”的控件“GridView1”必须放在具有 runat=server 的窗体标记内
抛出异常:类型"GridView"的控件"GridView1"必须放在具有 runat=server 的窗体标记内 我个人的理解为:添加的GridView空间未 ...
- 类型“TextBox”的控件“email”必须放在具有 runat=server 的窗体标记内。
类型"TextBox"的控件"email"必须放在具有 runat=server 的窗体标记内. 说明: 执行当前 Web 请 ...
- 【Visual Studio 扩展工具】使用 ComponentOne迷你图控件,进行可视化数据趋势分析...
概述 迷你图 -- Sparklines是迷你的轻量级图表,有助于快速可视化数据. 它们是由数据可视化传奇人物Edward Tufte发明的,他将其描述为"数据密集,设计简单,字节大小的图形 ...
最新文章
- ubuntu18.04安装openresty
- 一段比较好的加1操作。能够防止简单的++造成的溢出。
- 【MySQL】性能优化之 Index Condition Pushdown
- shiro-cas------实现单点登出并自定义登出starter
- HTML常用特殊符号集
- Cocos开发中性能优化工具介绍(一):Xcode中Instruments工具使用
- php中smarty模板下载,Smarty模板下载|
- MongoDB 唯一索引
- 选择阿里云数据库HBase版十大理由
- python计算两点间距离_用python计算图像中两点之间的距离
- 全市场等权中位数_市场指数估值周报20200418
- 服务器系统获取最高权限,webshell+servu获取系统最高权限
- qq飞车鸿蒙车队,qq飞车手游鸿蒙版
- 计算机专业需要学习打字吗,电脑学习打字的最快方法是什么
- js 获取指定某一天的时间戳
- HDFS集群管理与运维+distcp工具的使用
- 502 问题怎么排查?
- Jquery监听radio的变化以及获取radio选中值
- 常见网络故障排查方法
- android系统广播汇总