[content-description] find_element_by_accessibility_id 在 android 中的详解
出处:http://testerhome.com/topics/1034
最近 Appium 引入了一个新的 find element
方法:python client
为例
def find_element_by_accessibility_id(self, id): """Finds an element by accessibility id. :Args: - id - a string corresponding to a recursive element search using the Id/Name that the native Accessibility options utilize :Usage: driver.find_element_by_accessibility_id() """ return self.find_element(by=By.ACCESSIBILITY_ID, value=id)
文档里是这样说的:
Allows for elements to be found using the "Accessibility ID". The methods take a
string representing the accessibility id or label attached to a given element, e.g., for iOS the accessibility identifier and for Android the content-description. Adds the methods
driver.find_element_by_accessibility_id
andfind_elements_by_accessibility_id
.
意思就是可以使用 "Accessibility ID" 来定位元素。对于 iOS 而言就是 accessibility identifier。
对于 Android 就是 content-description
。
那什么是 content-description
呢?
官方是这样说的:
http://developer.android.com/training/accessibility/accessible-app.html
Android has several accessibility-focused features baked into the platform, which make it easy to optimize your application for those with visual or physical disabilities. However, it's not always obvious what the correct optimizations are, or the easiest way to leverage the framework toward this purpose. This lesson shows you how to implement the strategies and platform features that make for a great accessibility-enabled Android application.
这个属性,主要是为了一些有残障的人士准备的,方便他们使用程序,所以这个警告前面会有一个[Accessbility]。
举个例子,比如你有个 ImageView
放一张图片,这个图片可能包含很多物体和颜色,一些色弱或者色盲的人,他们可能会分不清这个图到底画的什么东西,所以这个时候 contentDescription
就会起作用。比如可能 Android 的一些程序可以用声音告诉使用者这个图片画的是什么,他们读的就是你 contentDescription
的内容。
这其实和 iOS 的 accessibility identifier
是一样的。
给一些需要加 contentDescription 的控件加 contentDescription 是一个良好的编程习惯,事实上 , ADT 的 Lint 检测也会提示你:
[Accessibility] Missing contentDescription attribute on image
所以给你的控件加上 android:contentDescription="@string/desc
, 这样也可以让 Appium 来定位。
如何判断是否加了 android:contentDescription?
- 第一种方法,看控件的 xml 是否加了 android:contentDescription 属性。
- 第二种,看代码,
myView.setContentDescription(desc);
- 第三种,使用 Hierarchy Viewer
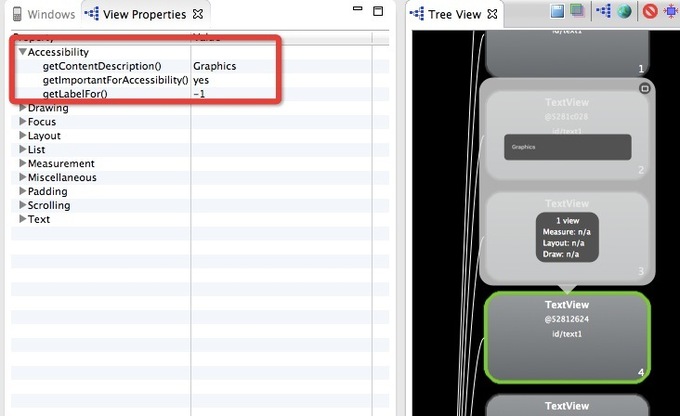
appium sample-code 里面的 android_complex.py 实际上得用 https://github.com/appium/android-apidemos 这个project 来导出 apk 来测试。
def test_find_elements(self): # pause a moment, so xml generation can occur sleep(2) els = self.driver.find_elements_by_xpath('//android.widget.TextView') self.assertEqual('API Demos', els[0].text) el = self.driver.find_element_by_xpath('//android.widget.TextView[contains(@text, "Animat")]') self.assertEqual('Animation', el.text) el = self.driver.find_element_by_accessibility_id("App") el.click() els = self.driver.find_elements_by_android_uiautomator('new UiSelector().clickable(true)') # there are more, but at least 10 visible self.assertLess(10, len(els)) # the list includes 2 before the main visible elements self.assertEqual('Action Bar', els[2].text) els = self.driver.find_elements_by_xpath('//android.widget.TextView') self.assertLess(10, len(els)) self.assertEqual('Action Bar', els[1].text)
其他
试用了下 genymotion, 非常好用,用来做 Appium 实验很棒。
使用模拟器的时候遇到了个问题:
java.lang.IllegalArgumentException: eglChooseConfig failed EGL_NOT_INITIALIZED 03-29 13:21:34.556: E/AndroidRuntime(4458): FATAL EXCEPTION: main 03-29 13:21:34.556: E/AndroidRuntime(4458): Process: com.example.news, PID: 4458 03-29 13:21:34.556: E/AndroidRuntime(4458): java.lang.IllegalArgumentException: eglChooseConfig failed EGL_NOT_INITIALIZED 03-29 13:21:34.556: E/AndroidRuntime(4458): at android.view.HardwareRenderer$GlRenderer.chooseEglConfig(HardwareRenderer.java:1173) 03-29 13:21:34.556: E/AndroidRuntime(4458): at android.view.HardwareRenderer$GlRenderer.loadEglConfig(HardwareRenderer.java:1135) 03-29 13:21:34.556: E/AndroidRuntime(4458): at android.view.HardwareRenderer$GlRenderer.initializeEgl(HardwareRenderer.java:1117) 03-29 13:21:34.556: E/AndroidRuntime(4458): at android.view.HardwareRenderer$GlRenderer.initialize(HardwareRenderer.java:1057) 03-29 13:21:34.556: E/AndroidRuntime(4458): at android.view.ViewRootImpl.performTraversals(ViewRootImpl.java:1550) 03-29 13:21:34.556: E/AndroidRuntime(4458): at android.view.ViewRootImpl.doTraversal(ViewRootImpl.java:1000) 03-29 13:21:34.556: E/AndroidRuntime(4458): at android.view.ViewRootImpl$TraversalRunnable.run(ViewRootImpl.java:5670) 03-29 13:21:34.556: E/AndroidRuntime(4458): at android.view.Choreographer$CallbackRecord.run(Choreographer.java:761) 03-29 13:21:34.556: E/AndroidRuntime(4458): at android.view.Choreographer.doCallbacks(Choreographer.java:574) 03-29 13:21:34.556: E/AndroidRuntime(4458): at android.view.Choreographer.doFrame(Choreographer.java:544) 03-29 13:21:34.556: E/AndroidRuntime(4458): at android.view.Choreographer$FrameDisplayEventReceiver.run(Choreographer.java:747) 03-29 13:21:34.556: E/AndroidRuntime(4458): at android.os.Handler.handleCallback(Handler.java:733) 03-29 13:21:34.556: E/AndroidRuntime(4458): at android.os.Handler.dispatchMessage(Handler.java:95) 03-29 13:21:34.556: E/AndroidRuntime(4458): at android.os.Looper.loop(Looper.java:136) 03-29 13:21:34.556: E/AndroidRuntime(4458): at android.app.ActivityThread.main(ActivityThread.java:5017) 03-29 13:21:34.556: E/AndroidRuntime(4458): at java.lang.reflect.Method.invokeNative(Native Method) 03-29 13:21:34.556: E/AndroidRuntime(4458): at java.lang.reflect.Method.invoke(Method.java:515) 03-29 13:21:34.556: E/AndroidRuntime(4458): at com.android.internal.os.ZygoteInit$MethodAndArgsCaller.run(ZygoteInit.java:779) 03-29 13:21:34.556: E/AndroidRuntime(4458): at com.android.internal.os.ZygoteInit.main(ZygoteInit.java:595) 03-29 13:21:34.556: E/AndroidRuntime(4458): at dalvik.system.NativeStart.main(Native Method)
Google了一下,关联到了opengl es,这个了解过,初步怀疑不是我应用的问题,最后查得居然是模拟器的问题,重启后解决问题。
转载于:https://blog.51cto.com/techgogogo/1608424
[content-description] find_element_by_accessibility_id 在 android 中的详解相关推荐
- android中getSystemService详解
原文地址:android中getSystemService详解作者:邹斌 http://blog.sina.com.cn/s/blog_71d1e4fc0100o8qr.html http://blo ...
- android中界面滑动延伸,android中ViewPager详解--视图滑动、界面卡等效果 (三)
GuideActivity.java引导界面: import java.util.ArrayList; import java.util.List; import android.app.Activi ...
- Android中menu详解(转)
Android中菜单的使用时非常频繁的,能够达到很好的使用效果,其实他的实现方法非常简单,下面为大家讲解,首先看图: JAVA代码: package com.smart; import android ...
- android中ViewPager详解--视图滑动、界面卡等效果 (三)
2019独角兽企业重金招聘Python工程师标准>>> GuideActivity.java引导界面: import java.util.ArrayList; import java ...
- Android中Context详解
给大家介绍下我们在应用开发中最熟悉而陌生的朋友-----Context类 ,说它熟悉,是应为我们在开发中时刻的在与它打交道,例如:Service.BroadcastReceiver.Activity等 ...
- android广播intent原理,Android中BroadcastReceiver详解
BroadcastReceiver是什么? Android app可以发送广播也可以接收系统或者其它app发送的广播,是发送/订阅的设计模式.这些广播被发送当重要的事件发生的时候.例如,安卓系统发送广 ...
- android 静态注册wifi广播,Android中BroadcastReceiver详解
BroadcastReceiver是什么? Android app可以发送广播也可以接收系统或者其它app发送的广播,是发送/订阅的设计模式.这些广播被发送当重要的事件发生的时候.例如,安卓系统发送广 ...
- Android中LaunchMode详解
越是做的时间越长,基础知识就忘的越干净,最近做一个项目中,发现启动的几个Activity居然重叠了,我ri--,再不回忆一下就要退出Android界了. 概念解释 Task Task叫做任务,这个简单 ...
- android asynctask 参数,Android中AsyncTask详解
定义 AsyncTask是一个抽象类,在使用时需要继承该类,实现其抽象方法protected abstract Result doInBackground(Params... params).其主要作 ...
最新文章
- Java8 lambda函数式编程
- nodejs(6)express学习
- QT-QPainter绘制曲线等基本图形
- python模块下载失败_python 安装tushare模块出错的一个解决方法
- leetcode589. N叉树的前序遍历
- 信息学奥赛C++语言:质因数分解
- scrapy-redis爬虫如何发送POST请求
- nao机器人行走速度_数计学院学子在2020世界机器人大赛总决赛中荣获佳绩!
- ubuntu搭建nginx服务器,并测试axel与wget的下载速度
- mysql配置文件my.cnf_MariaDB/MySQL配置文件my.cnf详解
- 树分解 宽度 如何分解 算法
- 使用Supermemo背单词6周年了
- 历史为什么选择C语言?事实证明:暮年的C语言,依旧宝刀未老!
- 简师网:考上教编才知道,小学教师和中学教师有这些差别!
- 7.2影像云阅片【斯纳克PACS医学影像云平台用户手册】
- JavaScript加密/解密与OpenAI的对接:生成加密对话的ChatGPT 4.0应用
- 智慧树工业机器人测试答案_智慧树工业机器人答案章节单元测试答案
- AI技术实用化 人脸识别协助线上实名认证
- [常微分方程的数值解法系列二] 欧拉法
- oracle中修改字段类型,字段值不为空
热门文章
- 百度:这次在AI领域我要做领头羊
- 对话科大讯飞刘聪:深度学习在中国是否“过火”?
- 干货丨深度学习和经典机器学习的全方位对比
- 一文概述2017年深度学习NLP重大进展与趋势
- 干货丨除了深度学习,你还应该了解这些发展方向
- 通过例子10分钟快速看懂pad_sequence、pack_padded_sequence以及pad_packed_sequence
- 费曼:所有科学知识都是不确定的
- 亚马逊首家“无人超市”系统存在bug?!开业当天,记者中途换装成功骗过摄像头...
- 新智能时代颠覆情报的未来
- 理性解读中国科技实力:不震惊式吹捧,也不全盘抹杀