pion webrtc 示例代码解析 一
pion webrtc
pion 是go语言写的webrtc的开发库套装
DTLS 协议
我们对DTLS 协议要适当的解释,TLS是用于TCP的,而DTLS是用于数据报的,很多应用运行在TCP之上,但是目前这种情况正在改变。现在不断增长的应用层协议,比如SIP (session initial protocol),RTP (real time protocol), MGCP (the Media Gateway Control Protocol),还有许多游戏协议都基于UDP传输而设计,而HTTP协议也正悄然改变,HTTP3可能也会转移到udp上。那么webrtc的datachannel也是基于这个协议的。
DataChannel的数据通过boringssl加密后再通过udp发送给对端,实现了DTLS,发送datachannel数据和音视频数据的事实上是同一个sock。不过不一样的是:音视频数据传输使用了RTP协议,并且通过NACK和FEC算法来抗丢包,而DataChannel底层使用了SCTP(stream control transmission protocol)协议,SCTP和TCP类似,具备流量控制、拥塞控制,可以说是一个可靠性传输协议,因此可以不采取其它措施即,就已经实现DataChannel数据的可靠传输。webrtc采用了第三方开源库usrsctplib来实现SCTP协议。
pion示例代码
这里,我们会对pion的示例一一解析,首先:启动 go run examples.go, ,example.go 是一个http协议的服务器,默认在80端口启动协议,这里我们打开网页后,打开datachannel示例:
点击run javascript后,得到Browser base64 Session Description框里的数据,复制里面的base64到一个文件中,保存名字为a.txt 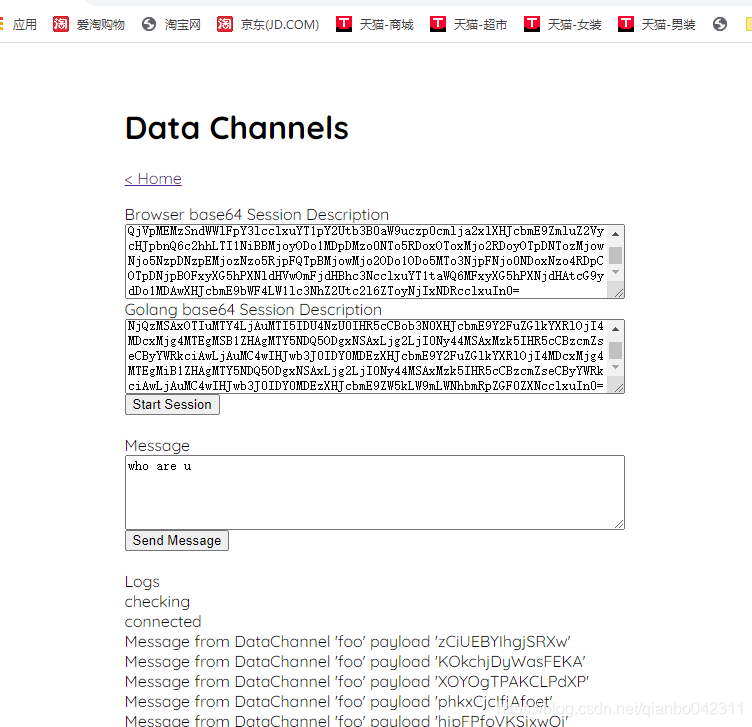 然后进入datachannel 目录 go run main.go < a.txt 就会得到自己的sdp信息,将这个sdp信息复制到页面中, 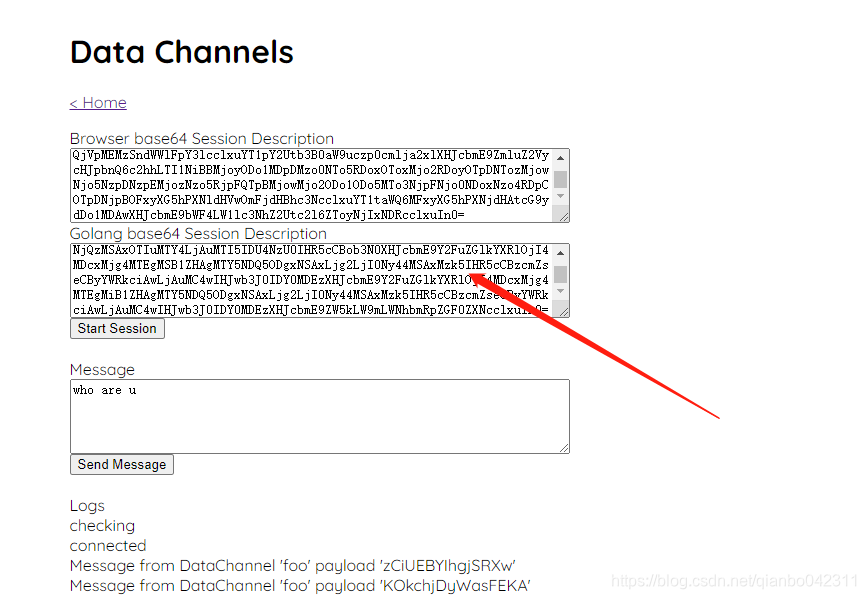 点击Start session, 网页和客户端可以开始互相发送信息。
示例datachannel
offer := webrtc.SessionDescription{}
signal.Decode(signal.MustReadStdin(), &offer)
singal 需要对输入进行读取,所以要从参数上输入offer,offer是一串码,sdp信息,我们来看看解码后的字符串:
{
offer v=0
o=- 203838700267639233 2 IN IP4 127.0.0.1
s=-
t=0 0
a=group:BUNDLE 0
a=extmap-allow-mixed
a=msid-semantic: WMS
m=application 3002 UDP/DTLS/SCTP webrtc-datachannel
c=IN IP4 1.86.247.81
a=candidate:2026329132 1 udp 2122260223 192.168.0.129 51720 typ host generation 0 network-id 1
a=candidate:910469340 1 tcp 1518280447 192.168.0.129 9 typ host tcptype active generation 0 network-id 1
a=candidate:4186919576 1 udp 1686052607 1.86.247.81 3002 typ srflx raddr 192.168.0.129 rport 51720 generation 0 network-id 1
a=ice-ufrag:y7zo
a=ice-pwd:vYIYqME4lmB5i0C3JwVZQicy
a=ice-options:trickle
a=fingerprint:sha-256 A2:28:50:C3:45:9D:19:12:6D:29:C5:32:06:97:C7:D2:37:9F:EA:A2:02:68:58:91:76:E6:44:17:8D:B9:C6:A8
a=setup:actpass
a=mid:0
a=sctp-port:5000
a=max-message-size:262144
}
这下看的很清晰了
package mainimport ("fmt""os""time""github.com/pion/webrtc/v3""github.com/pion/webrtc/v3/examples/internal/signal"
)func main() {// Everything below is the Pion WebRTC API! Thanks for using it ❤️.// Prepare the configurationconfig := webrtc.Configuration{ICEServers: []webrtc.ICEServer{{URLs: []string{"stun:stun.l.google.com:19302"},},},}// Create a new RTCPeerConnectionpeerConnection, err := webrtc.NewPeerConnection(config)if err != nil {panic(err)}defer func() {if cErr := peerConnection.Close(); cErr != nil {fmt.Printf("cannot close peerConnection: %v\n", cErr)}}()// Set the handler for Peer connection state// This will notify you when the peer has connected/disconnectedpeerConnection.OnConnectionStateChange(func(s webrtc.PeerConnectionState) {fmt.Printf("Peer Connection State has changed: %s\n", s.String())if s == webrtc.PeerConnectionStateFailed {// Wait until PeerConnection has had no network activity for 30 seconds or another failure. It may be reconnected using an ICE Restart.// Use webrtc.PeerConnectionStateDisconnected if you are interested in detecting faster timeout.// Note that the PeerConnection may come back from PeerConnectionStateDisconnected.fmt.Println("Peer Connection has gone to failed exiting")os.Exit(0)}})// Register data channel creation handlingpeerConnection.OnDataChannel(func(d *webrtc.DataChannel) {fmt.Printf("New DataChannel %s %d\n", d.Label(), d.ID())// Register channel opening handlingd.OnOpen(func() {fmt.Printf("Data channel '%s'-'%d' open. Random messages will now be sent to any connected DataChannels every 5 seconds\n", d.Label(), d.ID())for range time.NewTicker(5 * time.Second).C {message := signal.RandSeq(15)fmt.Printf("Sending '%s'\n", message)// Send the message as textsendErr := d.SendText(message)if sendErr != nil {panic(sendErr)}}})// Register text message handlingd.OnMessage(func(msg webrtc.DataChannelMessage) {fmt.Printf("Message from DataChannel '%s': '%s'\n", d.Label(), string(msg.Data))})})// Wait for the offer to be pastedoffer := webrtc.SessionDescription{}signal.Decode(signal.MustReadStdin(), &offer)// Set the remote SessionDescriptionerr = peerConnection.SetRemoteDescription(offer)if err != nil {panic(err)}// Create an answeranswer, err := peerConnection.CreateAnswer(nil)if err != nil {panic(err)}// Create channel that is blocked until ICE Gathering is completegatherComplete := webrtc.GatheringCompletePromise(peerConnection)// Sets the LocalDescription, and starts our UDP listenerserr = peerConnection.SetLocalDescription(answer)if err != nil {panic(err)}// Block until ICE Gathering is complete, disabling trickle ICE// we do this because we only can exchange one signaling message// in a production application you should exchange ICE Candidates via OnICECandidate<-gatherComplete// Output the answer in base64 so we can paste it in browserfmt.Println(signal.Encode(*peerConnection.LocalDescription()))// Block foreverselect {}
}
网页和客户端
网页和客户端的api 非常接近,可以看出pion是非常友好的,可以根据这个 示例来制作点对点的聊天程序。
pion webrtc 示例代码解析 一相关推荐
- mongoose框架示例代码解析(一)
mongoose框架示例代码解析(一) 参考: Mongoose Networking Library Documentation(Server) Mongoose Networking Librar ...
- 通过调试微信小程序示例代码解析flex布局参数功能(一)
通过调试微信小程序示例代码解析flex布局参数功能 官方示例小程序源代码下载地址:https://github.com/wechat-miniprogram/miniprogram-demo 通过调试 ...
- 【Android应用开发】 Universal Image Loader ( 使用简介 | 示例代码解析 )
作者 : 韩曙亮 转载请注明出处 : http://blog.csdn.net/shulianghan/article/details/50824912 相关地址介绍 : -- Universal I ...
- 【TensorFlow】多GPU训练:示例代码解析
使用多GPU有助于提升训练速度和调参效率. 本文主要对tensorflow的示例代码进行注释解析:cifar10_multi_gpu_train.py 1080Ti下加速效果如下(batch=128) ...
- 单图像超分辨率重建示例代码解析
昨天发了单图像超分辨率重建示例,今天来对里面主要的Python代码进行讲解,如果有补充或指正,欢迎大家在评论区留言.PS:这里的代码不要直接复制粘贴使用,由于注释的关系可能会报错,建议到示例给出的gi ...
- TSINGSEE青犀视频构建pion webrtc运行broadcast示例的步骤
上一篇我们介绍了TSINGSEE青犀团队关于WebRTC Pion音频模块的开发,有兴趣的朋友可以阅读一下:如何添加音频模块并通过浏览器播放.对于Pion WebRTC的开发,我们仍在探索当中,本文将 ...
- webrtc入门:14.pion webrtc中Data Channels示例
Data Channels 在pion webrtc 中有非常多的示例,Data Channels 就是其中的一个,当我们第一次打开pion webrtc的示例时,可能会有点不知所措,不知道他要让我们 ...
- php解析命令行参数选项,PHP 命令行参数解析工具类的示例代码
PHP 命令行参数解析工具类的示例代码 /** * 命令行参数解析工具类 * @author guolinchao */ class CommandLine { // 临时记录短选项的选项值 priv ...
- beego学习与代码示例WebIM解析-Ali0th
Author : Ali0th Date : 2019-4-26 安装 go get github.com/astaxie/beego # beego go install # 在beego目录下安装 ...
最新文章
- 解决QT无法调试问题-----the cdb process terminated
- EAS BOS 发布
- LeetCode 1801. 积压订单中的订单总数(map)
- Written English-书面-现在完成时
- python算法应用(四)——多维缩放
- 如何获取类(接口)的成员
- windows10计算机用户密码,忘记Windows 10系统密码?教你重置
- 链表的基本操作Basic Operation of LinkList
- android 15.6寸平板,关于HUAWEI 华为M6 10.8英寸平板的槽点,不吐不快
- 智能机械按摩椅的改进设计
- html+css实现自定义图片上传按钮
- 解决第三方dll出现:找不到指定模块(非路径错误)
- ./与../和/的区别
- Python手把手实现远程控制桌面
- NIST伪随机测试出现igamc:UNDERFLOW的原因以及测试文件的格式
- 材料专业转行,可以做什么
- 【转载】5年内从3500元到700万的过程,有爱情、有奋斗、有。。。泪水
- 旅行社软件测试培训,旅行社管理系统软件测试总结分析方案(65页)-原创力文档...
- 批处理是什么?能干什么?
- 【js实现手写签名板】canvas