解压缩文件并将其中的excel文件汇总
首先我有一个压缩文件,如下图所示:
package com.excel;import org.apache.commons.compress.archivers.sevenz.SevenZArchiveEntry;
import org.apache.commons.compress.archivers.sevenz.SevenZFile;
import org.apache.poi.ss.usermodel.CellType;
import org.apache.poi.xssf.usermodel.*;import java.io.*;
import java.time.LocalDateTime;public class Uncompress7z {/*** zip解压** @param inputFile 待解压文件名* @param destDirPath 解压路径*/public static void Uncompress(String inputFile, String destDirPath) throws Exception {File srcFile = new File(inputFile);//获取当前压缩文件// 判断源文件是否存在if (!srcFile.exists()) {throw new Exception(srcFile.getPath() + "所指文件不存在");}//开始解压SevenZFile zIn = new SevenZFile(srcFile);SevenZArchiveEntry entry;File file;boolean firstRowWrite = false;XSSFWorkbook resultWorkbook = new XSSFWorkbook();XSSFSheet resultWorkBookFirstSheet = resultWorkbook.createSheet("Detail Return Inquiry");int targetRowNum = 1;while ((entry = zIn.getNextEntry()) != null) {if (!entry.isDirectory()) {file = new File(destDirPath, entry.getName());if (!file.exists()) {new File(file.getParent()).mkdirs();}System.out.println("正在处理excel:" + entry.getName());OutputStream out = new FileOutputStream(file);BufferedOutputStream bos = new BufferedOutputStream(out);int len;byte[] buf = new byte[1024];while ((len = zIn.read(buf)) != -1) {bos.write(buf, 0, len);}// 关流顺序,先打开的后关闭bos.close();out.close();FileInputStream fileInputStream = new FileInputStream(file);XSSFWorkbook tempWorkbook = new XSSFWorkbook(fileInputStream);XSSFSheet tempFirstSheet = tempWorkbook.getSheetAt(0);XSSFCellStyle cellStyle = resultWorkbook.createCellStyle();//获取Excel文档中的第一个表单if (!firstRowWrite) {XSSFRow firstResourceFirstRow = tempFirstSheet.getRow(0);XSSFRow row = resultWorkBookFirstSheet.createRow(0);row.setRowStyle(cellStyle);for (int i = firstResourceFirstRow.getFirstCellNum(); i < firstResourceFirstRow.getLastCellNum(); i++) {XSSFCell cell = row.createCell(i);XSSFCell firstResourceFirstRowIndexCell = firstResourceFirstRow.getCell(i);String firstResourceFirstRowIndexCellStringCellValue = firstResourceFirstRowIndexCell.getStringCellValue();cell.setCellValue(firstResourceFirstRowIndexCellStringCellValue);}firstRowWrite = true;}// 循环除了第一行以外的数据for (int i = 1; i <= tempFirstSheet.getLastRowNum(); i++) {XSSFRow resourceRow = tempFirstSheet.getRow(i);XSSFRow targetRow = resultWorkBookFirstSheet.createRow(targetRowNum);targetRowNum++;for (int j = resourceRow.getFirstCellNum(); j < resourceRow.getLastCellNum(); j++) {XSSFCell cell = targetRow.createCell(j);XSSFCell resourceRowCell = resourceRow.getCell(j);if (resourceRowCell == null) {continue;}CellType cellType = resourceRowCell.getCellType();if (cellType.compareTo(CellType.STRING) == 0) {cell.setCellValue(resourceRowCell.getStringCellValue());}if (cellType.compareTo(CellType.NUMERIC) == 0) {cell.setCellValue(resourceRowCell.getNumericCellValue());}}}}}LocalDateTime now = LocalDateTime.now();//Create file system using specific nameFileOutputStream out = new FileOutputStream(new File("/home/shenlinnan/Downloads/汇总_202012041931.xlsx"));
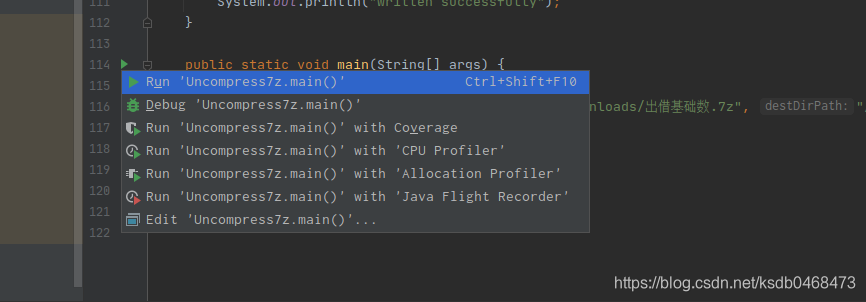//write operation workbook using file out objectresultWorkbook.write(out);out.close();System.out.println("written successfully");}public static void main(String[] args) {try {Uncompress("/home/shenlinnan/Downloads/出借基础数.7z", "/home/shenlinnan/Downloads");} catch (Exception e) {e.printStackTrace();}}
}
通过上面的代码可以直接解压缩文件,并且得到压缩的excel文件.
执行之后如下图所示:
逐步调整代码,首先这个需求是要在一个客户的电脑上直接执行这个操作,所以不能让人家直接在 IDE 里面去执行,所以只能是打成 jar 包.
现在使用Maven进行打包,如下图所示:
点击 install 文件,可以直接产生一个 jar 文件,如下图所示:
然后是如何执行这个 jar 文件呢,可以使用 java -jar 命令来执行一个 jar 文件:
在 shell 中输入 java 命令可以看到相关的提示,如上图所示.所以我们使用上面的命令执行该 jar 文件.
shenlinnan@shenlinnan-PC:~$ java -jar /home/shenlinnan/IdeaProjects/excel1/target/excel1-1.0-SNAPSHOT.jar
/home/shenlinnan/IdeaProjects/excel1/target/excel1-1.0-SNAPSHOT.jar中没有主清单属性
首先清单文件就是 jar 中的 META-INF 下的 MAINFEST.MF 文件.
可以看到在 shell 中给出了提示,没有主清单属性,首先要弄明白什么是主清单属性.
这里可以参考在 CSDN 上其他人的帖子,通读之后搞懂,这里给一个链接地址:
https://blog.csdn.net/qq_36838191/article/details/91381780
从其中得知:Main-Class是该 jar 的入口类,该类必须是一个可执行的类,我理解可执行必须得有:
public static void main(String[] args) {......
}
因为只有该代码的类才能执行.很显然我们是没有配置 Main-Class 属性才导致上面的报错的,我于是在工程中创建如下文件:
如图所示,我自己创建了一个 MANIFEST.MF 文件,但是我再次尝试打包,包中的清单文件内容还是和上面的内容一致,所以说明我这个清单文件根本没有起作用,参考该文档:
maven在MANIFEST.MF文件添加参数
弄清楚了,原来使用 Maven 来打包的话,需要配置 Maven 的插件.
下面是 Maven 官网中有关这个插件的说明:
Apache Maven JAR Plugin
我自己在 CSDN 上面进行了翻译,也可以直接看我翻译后的文档:
Apache Maven JAR Plugin官方文档
读过官网之后比较清晰了,插件使用Maven Archiver来处理jar内容和清单配置。
所以要去读官网有关 Maven Archiver 的部分.地址在超链接中已经给出了,下面是我自己的翻译官网 CSDN 的地址,要读官网还是我的翻译都可以.
中文翻译
查看了官方文档,自然就知道该如何配置了,按照官网上的配置.
但是现在面临着一个新的问题,pom文件中,这部分代码写到哪里?现在的pom文件如下截图:
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0"xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"><modelVersion>4.0.0</modelVersion><groupId>org.example</groupId><artifactId>excel1</artifactId><version>1.0-SNAPSHOT</version><dependencies><!-- https://mvnrepository.com/artifact/org.apache.poi/poi --><dependency><groupId>org.apache.poi</groupId><artifactId>poi</artifactId><version>4.1.2</version></dependency><!-- https://mvnrepository.com/artifact/org.apache.poi/poi-ooxml-schemas --><dependency><groupId>org.apache.poi</groupId><artifactId>poi-ooxml-schemas</artifactId><version>4.1.2</version></dependency><!-- https://mvnrepository.com/artifact/org.apache.poi/poi-ooxml --><dependency><groupId>org.apache.poi</groupId><artifactId>poi-ooxml</artifactId><version>4.1.2</version></dependency><!-- https://mvnrepository.com/artifact/org.apache.commons/commons-compress --><dependency><groupId>org.apache.commons</groupId><artifactId>commons-compress</artifactId><version>1.20</version></dependency><!-- https://mvnrepository.com/artifact/org.tukaani/xz --><dependency><groupId>org.tukaani</groupId><artifactId>xz</artifactId><version>1.8</version></dependency></dependencies><build><plugins><plugin><groupId>org.apache.maven.plugins</groupId><artifactId>maven-compiler-plugin</artifactId><version>2.3.2</version><configuration><source>1.8</source><target>1.8</target><encoding>utf-8</encoding></configuration></plugin></plugins></build></project>
这里还是要看Apache Maven JAR Plugin的那篇文档,完善了 pom 文件之后如下所示:
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0"xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"><modelVersion>4.0.0</modelVersion><groupId>org.example</groupId><artifactId>excel1</artifactId><version>1.0-SNAPSHOT</version><dependencies><!-- https://mvnrepository.com/artifact/org.apache.poi/poi --><dependency><groupId>org.apache.poi</groupId><artifactId>poi</artifactId><version>4.1.2</version></dependency><!-- https://mvnrepository.com/artifact/org.apache.poi/poi-ooxml-schemas --><dependency><groupId>org.apache.poi</groupId><artifactId>poi-ooxml-schemas</artifactId><version>4.1.2</version></dependency><!-- https://mvnrepository.com/artifact/org.apache.poi/poi-ooxml --><dependency><groupId>org.apache.poi</groupId><artifactId>poi-ooxml</artifactId><version>4.1.2</version></dependency><!-- https://mvnrepository.com/artifact/org.apache.commons/commons-compress --><dependency><groupId>org.apache.commons</groupId><artifactId>commons-compress</artifactId><version>1.20</version></dependency><!-- https://mvnrepository.com/artifact/org.tukaani/xz --><dependency><groupId>org.tukaani</groupId><artifactId>xz</artifactId><version>1.8</version></dependency></dependencies><build><plugins><plugin><groupId>org.apache.maven.plugins</groupId><artifactId>maven-compiler-plugin</artifactId><version>2.3.2</version><configuration><source>1.8</source><target>1.8</target><encoding>utf-8</encoding></configuration></plugin><plugin><groupId>org.apache.maven.plugins</groupId><artifactId>maven-jar-plugin</artifactId><version>3.2.0</version><configuration><archive><manifest><mainclass>com.excel.Uncompress7z</mainclass></manifest></archive></configuration></plugin></plugins></build></project>
我们再来打包看看是否把main-class的配置加上去了.
这里执行失败了,是因为mainclass这个属性错误了,应该是 mainClass.
修改后从新打包就可以了.可以看到main-class属性了,如下图所示:
再次执行看看结果如何:
~$ java -jar /home/shenlinnan/IdeaProjects/excel1/target/excel1-1.0-SNAPSHOT.jar
错误: 无法初始化主类 com.excel.Uncompress7z
原因: java.lang.NoClassDefFoundError: org/apache/poi/ss/usermodel/CellStyle
这是很明显的错误,肯定是 用于excel的jar包没有打进去,经过查看确实没有,我们再看看刚才的文档.
首先尝试着加入:
<addClasspath>true</addClasspath>
来试一试,因为在文档上面说该值代表着的意思是,是否创建 Class-Path 清单项。默认值为false。现在设置成true,应该会自动创建的.
果然自动创建了Class-Path,这些 jar 包都是在 pom 中引入的,再执行一次看看.
shenlinnan@shenlinnan-PC:~$ java -jar /home/shenlinnan/IdeaProjects/excel1/target/excel1-1.0-SNAPSHOT.jar
错误: 无法初始化主类 com.excel.Uncompress7z
原因: java.lang.NoClassDefFoundError: org/apache/poi/ss/usermodel/CellStyle
结果还是依旧不行,看看打出来的 jar 包里面确实是没有这些 jar.
目前这篇文档上我们已经无法正确的设置 class-path了,所以看一下另外这篇文档,就在刚才那片官网的如下位置:
我自己的翻译位置如下:
Set Up The Classpath中文官方文档
解压缩文件并将其中的excel文件汇总相关推荐
- 多個excel文件合并到一個excel文件
各位大俠下午好: 小弟在把多個由水晶報表導出的excel文件合并到一個excel文件的時候發現有一下問題: 圖片和表格重疊在一起,也就是說報表的圖形遮住了表格文字.如果 ...
- php原生读取excel文件夹,原生php实现excel文件读写的方法分析php技巧
这篇文章主要介绍了原生php实现excel文件读写的方法,结合实例形式分析了采用原生php针对Excel进行读写操作的相关实现方法与操作注意事项,需要的朋友可以参考下 本文实例分析了原生php实现ex ...
- php导出excel格式文件,PHP导入与导出Excel文件的方法
一.PHP导出Excel文件 1,推荐phpexcel,官方网站: http://www.codeplex.com/PHPExcel 导入导出都成,可以导出office2007格式,同时兼容2003 ...
- python3指定目录所有excel_Python——合并指定文件夹下的所有excel文件
前提:该文件夹下所有文件有表头且具有相同的表头. import glob # 同下 from numpy import * #请提前在CMD下安装完毕,pip install numppy impor ...
- csv和 文件流(二进制)excel文件转化为blob
如果是文件流(二进制)excel文件转化为blob,需要传 { responseType: "arraybuffer" } configApi.post(`/platform/pu ...
- java导出为excel文件_java导出数据到excel文件
有的时候,将一些有用的数据导出到excel是很有必要的.比如说,我现在在做一个学校的在线教学平台,有一个需求是:将学生成绩导出到excel文件中去. 那怎样实现用java导出数据到excel文件呢?? ...
- 批量处理word文件内容_用python批量提取word文件信息,导出到excel文件
技术的运用可以解决大量重复处理的工作,提高效率. 比如,有大量的论文电子文档(.docx格式),需要提取文档中的题目.作者.单位等信息制成表格(.xlsx格式),一般每篇论文的题目在第1行,副标题在第 ...
- python处理excel文件的模块_python处理Excel文件的几个模块
在python中简单地处理excel文件,有几个相关的模块,各有千秋,本文将不定时收录. Python Excel网站收集了关于python处理excel文件的各种信息. [注意]使用python处理 ...
- python处理多个excel文件-Python将多个excel文件合并为一个文件
利用Python,将多个excel文件合并为一个文件 思路 利用python xlrd包读取excle文件,然后将文件内容存入一个列表中,再利用xlsxwriter将内容写入到一个新的excel文件中 ...
最新文章
- 2022-2028年中国铁路行业投资分析及前景预测报告(全卷)
- leetcode算法题--和为s的连续正数序列
- android应用程序的混淆打包(转)
- 1298 FORZA David Beckham
- WinForm皮肤控件(SkinEngine)
- 17.视图--SQL
- httpsession 是一样的吗_理解HTTP session原理及应用
- 2013年计算机考试题库,2013年计算机三级数据库上机冲刺试题一及答案
- 教程:GIMP中改变画布大小
- 机器学习 | 数学基础
- LeetCode 739. 每日温度(java实现)
- 对注册表项“HKEY_CURRENT_USER\SOFTWARE\Microsoft\Windows\CurrentVersion\Run”的访问被拒绝。
- 程序员真的需要一台 Mac 吗?
- c mysql注册登录_C语言实现注册登录系统
- 怎么在网站中建立一个问答页面(FAQ)?
- thinkpad卡在logo界面_windows7开机卡在开机Thinkpad LOGO画面如何解决
- $vjudge-$基本算法专题题解
- 火爆不亚于中国?看看印度聊天机器人市场现状 | 分析
- 条形码设计软件BarTender实用教程——模板对象常见问题解答
- HDFS报错datanode.DataNode (DataXceiverServer.java:run(168))