swift 颜色 16进制_扔进Swift的封盖
swift 颜色 16进制
Difficulty: Beginner | Easy | Normal | ChallengingThis article has been developed using Xcode 11.4.1, and Swift 5.2.2
难度:初学者| 容易 | 普通| 具有挑战性本文是使用Xcode 11.4.1和Swift 5.2.2开发的
This article is tested on Xcode 12
本文已在Xcode 12上经过测试
先决条件: (Prerequisites:)
You will be expected to be aware how to make a Single View Application in Swift
您应该知道如何在Swift中制作Single View应用程序
You will need to have some knowledge of closures, although I’ll do my best to have you covered in this article
您将需要对闭包有所了解,尽管我会尽力在本文中进行介绍。
By definition this article relies on some knowledge of error handling in Swift
根据定义,本文依赖于Swift中的错误处理知识
You should be aware of @escaping
您应该注意@转义
术语 (Terminology)
Closures: A combination of functions and references to the surrounding context
闭包:功能和对周围环境的引用的组合
动机 (Motivation)
One of the important things in swift is using closures to make functions return and good practice for Swift coders.
swift的重要一环是使用闭包使函数返回并成为Swift编码人员的良好实践。
闭包的普通用法 (The ordinary use of closures)
One of the uses of closures
is to do something, and then something else (clear, right?)
closures
的用途之一是先做某件事,然后再做别的事情(清楚,对吧?)

the output of this (when called with doThis(a: "test", thenThis: completion)
) is (can you guess?)
这个的输出(当用doThis(a: "test", thenThis: completion)
)是(您能猜到吗?)
this then this test
this then this test
now because the function that is passed can be changed, we can do just that by instead passing throught the completionFunction
by using doThis(a: "test", thenThis: completionFunction)
which then gives the following output:
现在,因为传递的功能是可以改变的,我们能做到这一点的,而不是通过throught的completionFunction
使用doThis(a: "test", thenThis: completionFunction)
然后给出了以下的输出:
this then this function test
this then this function test
Now notice that the first function completion
is assigned to a constant (through let
) but the second is simply a function
- no matter, Swift
can cope with both of these functions.
现在注意,第一个函数completion
已分配给一个常量(通过let
),但是第二个仅是一个function
-不管怎样, Swift
可以处理这两个函数。
Awesome — we are passing a closure to a function as a function- Most excellent news.
太棒了-我们正在将闭包作为函数传递给我们-最出色的消息。
We’ll expand this second example as we move forwards in this tutorial.
在本教程中,我们将继续扩展第二个示例 。
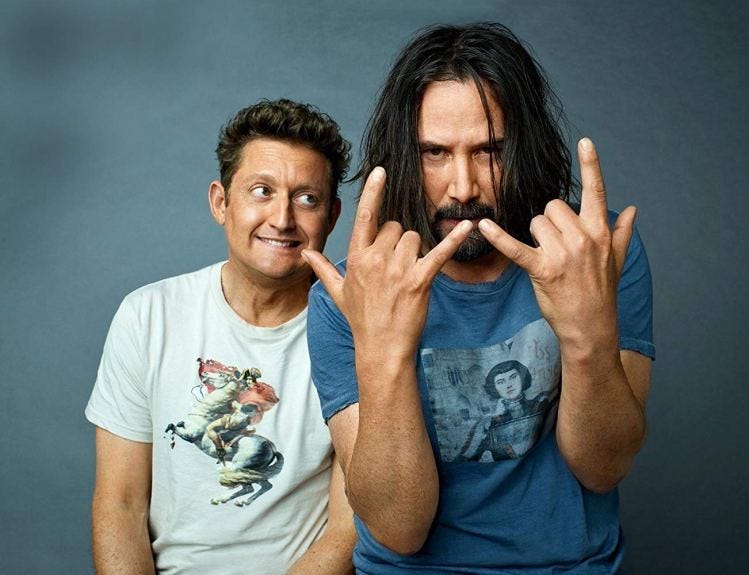
异步闭包的使用 (The use of async closures)
Network calls can return at any time after they are called. I’m pretending to have a network call here, but you could actually do it with the following network manager if you’d like).
网络呼叫在被调用后可以随时返回。 我假装在这里打个网络电话,但是如果您愿意的话,实际上您可以与以下网络管理员联系 。
So imagine that you need to need to go to the network to do some work. You make a network call to increment a number (it’s an example, right?) but goes and makes a network call to do just that. Well, we can model that:
因此,想象您需要去网络做一些工作。 您进行网络呼叫以增加一个数字(这是一个例子,对吗?),但是去进行网络呼叫来做到这一点。 好吧,我们可以建模:


I know there are some tricky parts to this — we are using a trailing closure which can be tricky. Assuming you’re OK with all that let us move on.
我知道这有一些棘手的部分-我们正在使用结尾的闭包,这可能很棘手。 假设您对所有让我们继续进行的事情都感到满意。
But there is a problem (in this example, and not just that it is contrived) — incNum
will produce an error if 13 is returned.
但是存在一个问题(在此示例中,不仅是人为的),如果返回13, incNum
将产生错误。
错误 (The Error)
So what about producing an Error
when 13 is returned from incNum
?.
那么从incNum
返回13时产生一个Error
incNum
办?
Let us say that 13 is a bad number (in some cultures it is considered to be unlucky). So we shall set up a rather basic function incNum
that will call a completion
handler to return a number increased by one.
让我们说13是一个坏数字(在某些文化中,它被认为是不幸的)。 因此,我们将建立一个相当基本的函数incNum
,该函数将调用completion
处理程序以返回加一的数字。
The Error
will indicate if we have returned an 'unlucky' number from incNum
- but see underneath the code snippet for the (rather large) error:
该Error
将指示我们是否从incNum
返回了一个“不幸”数字-但是请参见代码段下方的(相当大的)错误:

Invalid conversion from throwing function of type '(Int) throws -> Void' to non-throwing function type '(Int) -> Void'
Invalid conversion from throwing function of type '(Int) throws -> Void' to non-throwing function type '(Int) -> Void'
Oh dear!
噢亲爱的!
What Swift is telling us, is that when we throw BadLuckError.unlucky
we are actually throwing from the closure.
Swift告诉我们的是,当我们throw BadLuckError.unlucky
,实际上是从闭包中抛出。
从拖尾闭合处抛出 (Throwing from a trailing closure)
The solution will come first, followed by the explaination
解决方案将首先出现,然后进行解释

We have marked callingFunction
as throws
which means that it can throw
an error by placing the throws
keyword at the end of the function signature. This meant that we needed to call the calling function suing a do-try-catch
block:
我们已经标记callingFunction
为throws
,这意味着它可以throw
通过将错误throws
的函数签名结束关键字。 这意味着我们需要使用do-try-catch
块来调用调用函数:
do { try callingFunction(num: 12, closure: incNum) } catch { print ("DONE") }
do { try callingFunction(num: 12, closure: incNum) } catch { print ("DONE") }
The incNum
function will not throw an error on it's own, so is marked with rethrows
as it throws from the completion
- which therefore must be marked with try
. Since completion
does indeed throw
it has it's signature changed to (Int) throws -> Void)
.
incNum
函数不会自行抛出错误,因此从completion
时rethrows
时将被标记为rethrows
-因此必须使用try
进行标记。 由于completion
确实会throw
,因此其签名已更改为(Int) throws -> Void)
。
This modified signature is copied back to the signature of the callingFunction
which marks the closure as the same (Int) throws -> Void)
, but since rethrows
can only be used in function declarations throws
is used instead.
将此修改后的签名复制回给callingFunction
的签名,该签名将闭包标记为相同的(Int) throws -> Void)
callingFunction
(Int) throws -> Void)
,但是由于rethrows
只能在函数声明中使用,因此使用throws
代替。
Fantastico!
幻想曲!
注意事项 (The caveats)
You can’t throw an error from async closures — which means that you either force the closure to be synchronous using semaphores (not recommended due to performance) or use Swift’s result type.
您不能从异步闭包中引发错误-这意味着您可以使用信号量(由于性能不建议使用)强制闭包同步,或者使用Swift的结果类型 。
结论 (Conclusion)
I hope this article has helped you out!
希望本文对您有所帮助!
It can be tricky to work through this type of article — we are using throws
and rethrows
and it can feel a little complex. However, I hope this article has helped you out and you can see how to progress in your coding journey.
处理此类文章可能很棘手-我们使用的是throws
和rethrows
,感觉有些复杂。 但是,希望本文对您有所帮助,并且您可以看到如何在编码过程中取得进步。
If you’ve any questions, comments or suggestions please hit me up on Twitter
如果您有任何疑问,意见或建议,请在Twitter上打我
Feel free to sign up to my newsletter
随时订阅我的时事通讯
翻译自: https://medium.com/@stevenpcurtis.sc/throw-inside-swifts-closures-e69fb378500e
swift 颜色 16进制
http://www.taodudu.cc/news/show-5988180.html
相关文章:
- 2022-2028全球与中国山墙顶帽和密封盖市场现状及未来发展趋势
- 如何善用git_善用封盖
- Java 抽象类和接口内部类及综合
- c++复习笔记(多代码版)
- 小尾羊的qt学习之路,01课,按钮
- UEFI 基础教程 (一) - 运行第一个APP HelloWorld
- 用eclipse编写第一个程序详解(hello world)
- GCC和HelloWorld
- 给定一个整数数组 nums 和一个整数目标值 target,请你在该数组中找出 和为目标值 的那 两个 整数,并返回它们的数组下标。
- c语言判断顺序链表是否为空,数据结构之线性表(五)——单链表(2 初始化,判断空表,销毁,清空,求表长)...
- 目标分割(四)DeepLab v1讲解
- 系统学习Python——2D绘图库Matplotlib:绘图函数matplotlib.pyplot.plot(plt.plot)
- 屏幕为黑色yuv值怎么设置
- 手把手调参最新 YOLOv7 模型 推理部分 - 最新版本(一)
- 深度学习:目标分割|UNet网络模型及案例实现
- 【详解】计算机视觉之目标分割
- 计算机视觉(二)——HSV色彩分离及目标跟踪
- 目标检测模型的评价指标 mAP
- 目标检测——交并比(Intersection of Union,IoU)计算
- Excel成神之道-003-快速生成数字等差序列
- java 生成Excel文件导出
- C#Excel帮助类
- Excel成神之道-000-Excel该如何正确使用
- Java导出主表和明细Excel
- Excel 转Insert sql 语句
- python 通过openpyxl来操作Excel文件(二 ):写入Excel文件
- python提取pdf数据到excel_PDF文本内容批量提取到Excel
- java中读取excel数据类型_在Java中读取Excel文件的内容
- PowerDesigner 将pdm模型表 用命令导出成Excel表格
- python控制excel能达到什么目的_你精通excel吗?不,我精通python操控excel!
swift 颜色 16进制_扔进Swift的封盖相关推荐
- java 十六进制转十进制_「16进制转10进制」Java:十六进制转换成十进制 - seo实验室...
16进制转10进制 问题及代码: /* *问题描述 从键盘输入一个不超过8位的正的十六进制数字符串,将它转换为正的十进制数后输出. 注:十六进制数中的10~15分别用大写的英文字母A.B.C.D.E. ...
- python十六进制转十进制_使用Python 16进制转10进制
原博文 2019-05-07 15:22 − """ 16进制转10进制 """ # str="A5 42 D2 00 4A 00 ...
- c# .net 16进制转换10进制
业务调用 #region C#十六进制字符串转十进制 { Console.WriteLine("-----------十六进制字符串转十进制---------");//H:十六进制 ...
- Python | HEX16进制与RGB10进制颜色互转
Python | HEX16进制与RGB10进制颜色互转 背景知识 hex → rgb 实例 hex ← rgb 背景知识 hex: E6004D or #E6004 rgb: [230, 0, 77 ...
- python 16进制转10进制, 8进制转10进制, 2进制转10进制的方法
python 16进制转10 进制, 8进制转10进制, 2进制转10进制 可以使用系统自带的 int 方法 具体如下: value = "0x1388" result = int ...
- 10进制转16进制,16进制转10进制,随机出一个6位十六进制颜色值
方案一: var num16 = "ffffff";var num10 = parseInt(num16,16);//16进制转10进制console.log(num10) // ...
- java 10zhuan8,Java代码 10进制转2、8、16进制转换 / 2、8、16进制转10进制转换
public static void main(String[] args) { int i = 10; System.out.println("***********10进制转换2进制.8 ...
- python16进制转10进制_python 字节串及10进制,16进制相关转换
进行协议解析时,总是会遇到各种各样的数据转换的问题,从二进制到十进制,从字节串到整数等等 整数之间的进制转换: 10进制转16进制: hex(16) ==> 0x10 16进制转10进制: in ...
- 关于物联网的各种转换,16进制 二进制 10进制 效验总结
字符串插入字符 /// <summary>/// 每隔n个字符插入一个字符/// </summary>/// <param name="input"& ...
最新文章
- React从入门到精通系列之(1)安装React
- [BZOJ4557][JLOI2016]侦查守卫
- 大数据系列修炼-Scala课程07
- 剑指offer(49)把字符串转换成整数。
- ubuntu 安装 opengl
- SLAM--激光视觉--比较及联合标定
- 北京内推 | 腾讯云小微自然语言技术中心招聘NLP研究型实习生
- linux – syslog,rsyslog和syslog-ng之间有什么区别?
- VMware里Ubuntu-14.04-desktop的VMware Tools安装图文详解
- html ul高度自适应,如何让div中的ul元素自适应
- 工业相机选型:相机接口
- 写代码如坐禅:你是哪一类程序员
- jdk api 1.8 中文版 下载
- Vosviewer图谱相关指标详细解释1
- 史上最全的oracle常用知识总结
- 简单PHP会话(session)说明
- 成功三大定律 重在厚积薄发!
- Whitestorm.js入门
- 魔方(6)三阶空心魔方、二阶空心魔方
- 如何备份Chrome浏览器收藏夹