《中国编程挑战赛--资格赛》赛题及解答
You are given a string[] grid representing a rectangular grid of letters. You are also given a string find, a word you are to find within the grid. The starting point may be
anywhere in the grid. The path may move up, down, left, right, or diagonally from one letter to the next, and may use letters in the grid more than once, but you may not stay
on the same cell twice in a row (see example 6 for clarification).
You are to return an int indicating the number of ways find can be found within the grid. If the result is more than 1,000,000,000, return -1.
Definition
Class:
WordPath
Method:
countPaths
Parameters:
string[], string
Returns:
int
Method signature:
int countPaths(string[] grid, string find)
(be sure your method is public)
Constraints
-
grid will contain between 1 and 50 elements, inclusive.
-
Each element of grid will contain between 1 and 50 uppercase ('A'-'Z') letters, inclusive.
-
Each element of grid will contain the same number of characters.
-
find will contain between 1 and 50 uppercase ('A'-'Z') letters, inclusive.
Examples
0)
{"ABC",
"FED",
"GHI"}
"ABCDEFGHI"
Returns: 1
There is only one way to trace this path. Each letter is used exactly once.
1)
{"ABC",
"FED",
"GAI"}
"ABCDEA"
Returns: 2
Once we get to the 'E', we can choose one of two directions for the final 'A'.
2)
{"ABC",
"DEF",
"GHI"}
"ABCD"
Returns: 0
We can trace a path for "ABC", but there's no way to complete a path to the letter 'D'.
3)
{"AA",
"AA"}
"AAAA"
Returns: 108
We can start from any of the four locations. From each location, we can then move in any of the three possible directions for our second letter, and again for the third and
fourth letter. 4 * 3 * 3 * 3 = 108.
4)
{"ABABA",
"BABAB",
"ABABA",
"BABAB",
"ABABA"}
"ABABABBA"
Returns: 56448
There are a lot of ways to trace this path.
5)
{"AAAAA",
"AAAAA",
"AAAAA",
"AAAAA",
"AAAAA"}
"AAAAAAAAAAA"
Returns: -1
There are well over 1,000,000,000 paths that can be traced.
6)
{"AB",
"CD"}
"AA"
Returns: 0
Since we can't stay on the same cell, we can't trace the path at all.
我的解答:
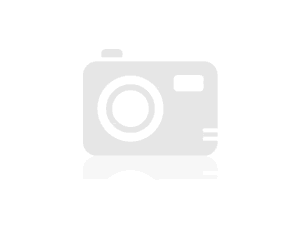
2
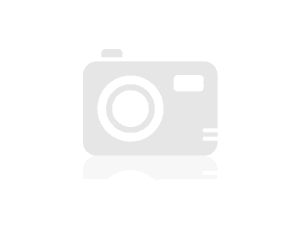
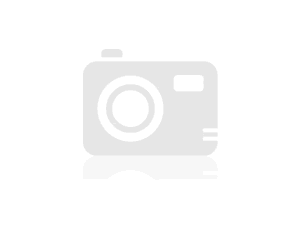

3

4

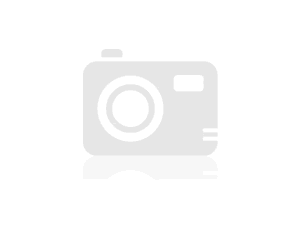

5

6

7

8

9

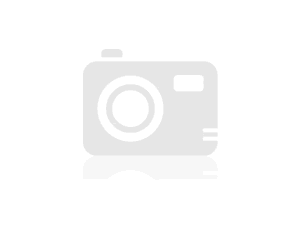

10

11

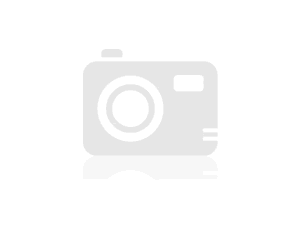

12

13

14

15

16

17

18

19

20

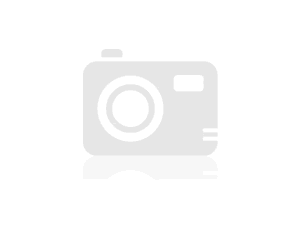

21

22

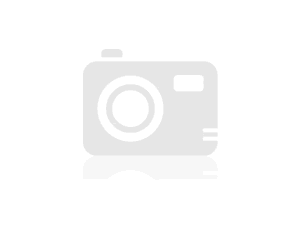

23

24

25

26

27

28

29

30

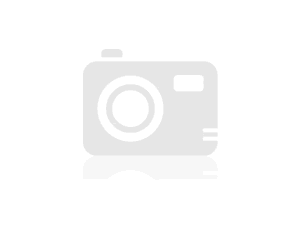

31

32

33

34

35

36

37

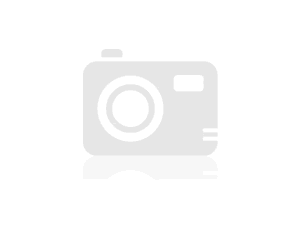

38

39

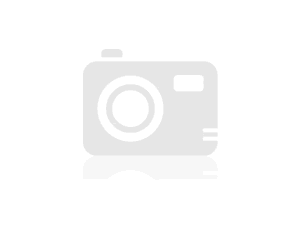

40

41

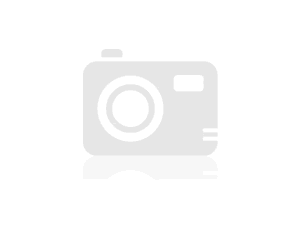

42

43

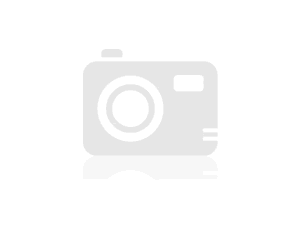

44

45

46

47

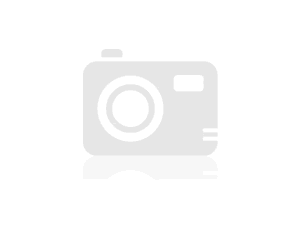

48

49

50

51

52

53

54

55
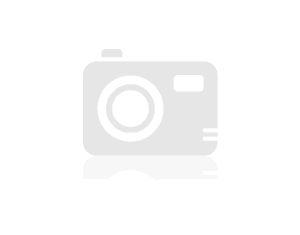
希望大家发表更好的答案。
转载于:https://www.cnblogs.com/chinadhf/archive/2005/12/18/299788.html
《中国编程挑战赛--资格赛》赛题及解答相关推荐
- 第二届全国大学生算法设计与编程挑战赛(赛题,共10个)
第二届全国大学生算法设计与编程挑战赛 比赛日期:2020.11.15 早上9:00--下午14:00 共十个赛题. loading...... x = 13930 y = 457439count = ...
- 2016华为软件精英挑战赛:赛题及其答疑汇总
注:本文文字均摘自官方指定网站和论坛,权威且可信,答疑见中间部分,非常全,众玩家可放心阅读. 同时文末给出了包括自己在内的诸多玩家的解法. 前言 赛题源自"未来网络"业务发放中的路 ...
- 第二届战神杯线上编程挑战赛月赛第一题:回文数
题目详情: Njzy学习了回文串后联想到了回文数,他希望统计出一个区间内的全部回文数.如今给定一个闭区间[a,b],求这个区间里有多少个回文数. 比方[20,30],仅仅有一个回文数那就是22. 输入 ...
- 【比赛】算法设计与编程挑战赛——每日一题
很多题目都是洛谷上有的,可以自己去搜索 [参考:题目列表 - 洛谷 | 计算机科学教育新生态] 在每日一题中,我会给出题目的算法标签以供同学们自学. 每日一题的难度会越来越大,会尝试覆盖尽可能多的算法 ...
- 参加Google™ Code Jam - 中国编程挑战赛(2)
编写了第二个题目,总分500,得分499.76分.估计这个计分还根据时间来的.时间越长得分越少.这次我先编译好直接copy的. 题目: Problem Statement A ...
- 华为云TaurusDB性能挑战赛-java赛题mvn编译时报错:不支持multi-catch
在使用IDEA导入项目后,使用maven编译时,报错显示如下信息: 添加如下信息至pom.xml中 <build><plugins><plugin><grou ...
- 华为云TaurusDB性能挑战赛-java赛题mvn编译时报错:不支持 diamond 运算符
在使用IDEA导入项目后,使用maven编译时,报错显示如下信息: 添加如下信息至pom.xml中 <build><plugins><plugin><grou ...
- 美团CodeM2018资格赛赛题官方版本详解(二)——可乐
▋解题思路 ▋标准程序 #include <cstdio> #include <cstdlib> #include <cstring> #include <c ...
- 2018美团CodeM编程大赛资格赛第一题
一.问题描述 题目描述 美团在吃喝玩乐等很多方面都给大家提供了便利.最近又增加了一项新业务:小象生鲜.这是新零售超市,你既可以在线下超市门店选购生鲜食品,也可以在手机App上下单,最快30分钟就配送到 ...
最新文章
- Tomcat在windows中解压war包失败问题
- Go语言学习笔记 - PART11 - 面向对象
- VLAN,trunk,以太网通道
- 文巾解题455. 分发饼干
- _Linux进程信号详解
- 什么叫做项目孵化_蓝莓孵化营12进5争夺战,项目人绽放自我不留遗憾
- java的常用注解有哪些_spring系列笔记之常用注解
- 同步与互斥的基本原理
- layui 之button 事件绑定的一种方法
- dump文件 linux,Linux下快速分析DUMP文件
- iCloud照片无法上传或同步怎么办?
- 【转载】使用theano进行深度学习实践(一) - CSDN博客
- Linux 升级glibc-2.18
- Android 相机预览方向和拍照方向
- 使用spring的优势
- EasyExcel工具类封装, 做到一个函数完成简单的读取和导出
- ECDH and ECDSA(ECC椭圆曲线算法3)
- myeclipse-添加jar包
- Kaggle信贷预测随笔
- 【BZOJ4424】Cf19E Fairy DFS树
热门文章
- c语言一个整数各位数字个数_C语言实现把字符串中的数字转换成整数
- 使用tab键分割的文章能快速转换成表格。( )_word排版技巧:活用Enter键提高工作效率...
- markdownpad2 html渲染组件出错_Day68 Django forms组件
- php 工商银行公众号支付代码_微信支付PHP SDK —— 公众号支付代码详解
- 客服机器人源码_快速搭建对话机器人,就用这一招!
- 了解JavaScript核心精髓(三)
- socket层内容详解二
- bootstrap 源码中部分不了解的css属性
- springmvc 的 @PathVariable
- 如何处理跨平台的自适应三