kotlin跨平台_探索Kotlin跨平台第1部分
kotlin跨平台
介绍 (Introduction)
Kotlin makes Android development more fun. If you search the web, you’ll find tons of ways in which Kotlin solved many of Java’s pain points and how features of Kotlin aim to make Android development more fun. But most people fail to understand that Kotlin has far more capabilities than just for use with Android.
Kotlin使Android开发更加有趣。 如果您在网络上搜索,您将找到大量的方式来解决Kotlin的许多Java难题,以及Kotlin的功能旨在使Android开发更加有趣的目的。 但是大多数人无法理解Kotlin所具有的功能远不止是与Android一起使用。
As “Open Source For You” describes it, Kotlin is a concise, safe, interoperable, and tool-friendly, multi-platform programming language. It is a statically typed programming language that runs on the Java virtual machine and can also be compiled to JavaScript source code or can use the LLVM compiler infrastructure.
正如“ 为您开源 ”所描述的那样,Kotlin是一种简洁,安全,可互操作且对工具友好的多平台编程语言。 它是一种在Java虚拟机上运行的静态类型的编程语言,也可以编译为JavaScript源代码或可以使用LLVM编译器基础结构。
为什么选择多平台项目? (Why the Multiplatform Project?)
First, why do we need to implement a multiplatform project? It’s simple to reduce the development cost and maintain a single source of truth for business logic.
首先,为什么我们需要实施一个多平台项目? 降低开发成本并维护业务逻辑的单一真相很简单。
The meaning of single source of truth is to maintain the shared (business logic) or platform-agnostic code in a central repository. All the supported platform applications access them; this way, we can separate the concerns of business logic from platform-specific issues.
单一事实来源的含义是在中央存储库中维护共享的(业务逻辑)或与平台无关的代码。 所有受支持的平台应用程序都可以访问它们; 这样,我们可以将业务逻辑的关注点与特定于平台的问题分开。
为什么选择Kotlin多平台项目? (Why Kotlin Multiplatform Project?)
When we think of multiplatform development, we usually think of frameworks like Flutter or web applications. But you can’t build a native UI using these frameworks. We’ve to move the entire ecosystem, which is hard if you’re in the market for a long time.
当我们想到多平台开发时,通常会想到Flutter或Web应用程序之类的框架。 但是您不能使用这些框架构建本机UI。 我们必须移动整个生态系统,如果您长期在市场中工作,这将非常困难。
This is where Kotlin multiplatform shines. With KPP (Kotlin multiplatform project) we can use the platform-specific ecosystem to develop a native UI and then create a shared Kotlin multiplatform library to integrate business logic.
这就是Kotlin跨平台大放异彩的地方。 通过KPP(Kotlin多平台项目),我们可以使用特定于平台的生态系统来开发本机UI,然后创建共享的Kotlin多平台库以集成业务逻辑。
You can start using Kotlin to develop a shared library for any platform that runs on JVM, Kotlin/JS flavor for web, and Kotlin/Native machine code for IOS. Unlike other frameworks, we can migrate to a multiplatform structure incrementally with Kotlin.
您可以开始使用Kotlin为在JVM,Web上的Kotlin / JS风味以及IOS上的Kotlin / Native机器代码上运行的任何平台开发共享库。 与其他框架不同,我们可以使用Kotlin逐步迁移到多平台结构。
总览 (Overview)
This article aims to create an application for both Android and iOS, making use of Kotlin’s code sharing capabilities. To share the code for Android and iOS, we use Kotlin/JVM and Kotlin/Native, respectively.
本文旨在利用Kotlin的代码共享功能为Android和iOS创建一个应用程序。 要共享Android和iOS的代码,我们分别使用Kotlin / JVM和Kotlin / Native。
When we launch the application on Android, it’s going to display “Kotlin works in Android,” and “Kotlin works in IOS in Android & IOS devices,” respectively.
当我们在Android上启动该应用程序时,它将显示“ Kotlin在Android中工作”和“ Kotlin在IOS中工作” 分别位于Android和IOS设备中”。
The common code is “Kotlin works in ${platformName()}”
, where platformName()
is a function that is declared using the expect
keyword. The actual
implementation will be specific to the platform.
通用代码是“Kotlin works in ${platformName()}”
,其中platformName()
是使用expect
关键字声明的函数。 actual
实现将特定于平台。
项目设置 (Project Setup)
The first step is to create an Android project; we can use an Android Studio or Intellij community version. Then we need to create the SharedCode
module, which is used to generate artifacts for multiple platforms.
第一步是创建一个Android项目。 我们可以使用Android Studio或Intellij社区版本。 然后,我们需要创建SharedCode
模块,该模块用于为多个平台生成构件。
SharedCode模块 (SharedCode module)
We can build this module by including include ‘: SharedCode’
in settings.gradle
file. Then rebuild the project or click the sync project option. Once the project is updated, you’ll see a shared code module in the left panel.
我们可以通过在settings.gradle
文件中include ': SharedCode'
来构建此模块。 然后重建项目或单击同步项目选项。 项目更新后,您将在左侧面板中看到一个共享代码模块。
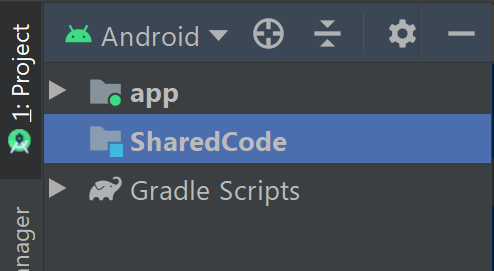
工件设置 (Artifacts setup)
Now, we need to create SharedCode/build.gradle.kts
file and include the below code to generate necessary artifacts. Here the artifacts are androidMain
, commonMain
, and iosMain
.
现在,我们需要创建SharedCode/build.gradle.kts
文件,并包含以下代码以生成必要的工件。 这里的工件是androidMain
, commonMain
和iosMain
。
Once you’re done, click the Sync Project button or rebuild the project to apply the changes properly.
完成后,单击“同步项目”按钮或重建项目以正确应用更改。
创建目录 (Creating directories)
We need to create the missing directories such as SharedCode/src/commonMain
, SharedCode/src/androidMain
, and SharedCode/src/iosMain
, to have a better idea on how to create them, see the below video:
我们需要创建缺少的目录,例如SharedCode/src/commonMain
, SharedCode/src/androidMain
和SharedCode/src/iosMain
,以更好地了解如何创建它们,请参见以下视频:

创建共享模块 (Creating Shared Modules)
We have to create a Kotlin subdirectory inside each artifact directory. Then inside SharedCode/src/commonMain
, we need to create a Kotlin file with the name “common.”
我们必须在每个工件目录内创建一个Kotlin子目录。 然后在SharedCode/src/commonMain
,我们需要创建一个名称为“ common”的Kotlin文件。
This shared file includes all the code that is ideal across the platforms. In this case, it contains the string generation by taking expected inputs. Have a look:
该共享文件包含所有跨平台的理想代码。 在这种情况下,它包含通过获取预期输入生成的字符串。 看一看:
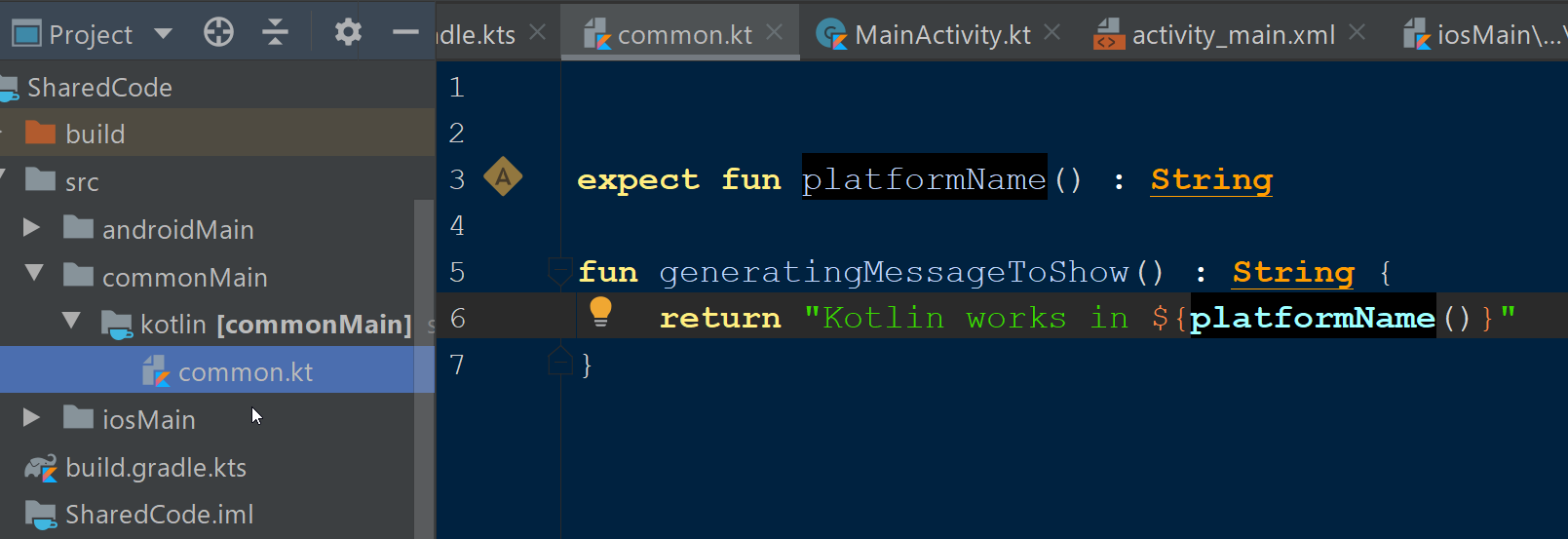
expect
is the keyword used to tell the Kotlin multiplatform framework this function is expected from each platform(Android and iOS, in this case).
expect
是用于告诉Kotlin跨平台框架的关键字,该功能是每个平台(在本例中为Android和iOS)所期望的。
We need to use the actual
keyword in platform-specific files to map it with the common artifact’s function. Now let’s see android and iOS files. Have a look:
我们需要在特定于平台的文件中使用actual
关键字,以将其与通用工件的功能进行映射。 现在,让我们看看android和iOS文件。 看一看:


在Android项目中共享代码 (Share Code in Android Project)
To keep things simple in the Android project, we can include the following line under the dependency tag in build.gradle
file:
为了使Android项目中的事情变得简单,我们可以在build.gradle
文件的dependency标签下添加以下行:
implementation project(':SharedCode')
We can now access generatingMessageToShow
function in common artifact, and the platformName
function in the Android module will invoke via KotlinJVMTarget
. We just need to include the following line in onCreate
method of the MainActivity
.
现在,我们可以在通用工件中访问generatingMessageToShow
函数,并且Android模块中的platformName
函数将通过KotlinJVMTarget
调用。 我们只需要在MainActivity
onCreate
方法中包含以下行即可。
tv_welcome?.text = generatingMessageToShow()
在IOS项目中共享代码 (Share Code in IOS Project)
First, we need to generate the following targets to integrate into the iOS project.
首先,我们需要生成以下目标以集成到iOS项目中。
iOS arm64 debug
— the binary to run the iOS device in debug modeiOS arm64 debug
-在调试模式下运行iOS设备的二进制文件iOS arm64 release
— the binary to include into a release version of an appiOS arm64 release
-包含在应用程序发行版本中的二进制文件iOS x64 debug
— the binary for iOS simulator, which uses the desktop Mac CPUiOS x64 debug
-iOS模拟器的二进制文件,它使用桌面Mac CPU
We can do this by running the packForXcodegradle
task that we mentioned in the build.gradle.kts
for the iOS artifact. Alternatively, we can do that by running the following command in the console.
我们可以通过运行做到这一点packForXcodegradle
任务,我们在提到build.gradle.kts
用于iOS的神器。 或者,我们可以通过在控制台中运行以下命令来实现。
./gradlew :SharedCode:packForXcode
We can use this artifact in the Xcode project; you’ll find the detailed instructions on integrating them here. Once you’re done with integration, it’s pretty much straightforward.
我们可以在Xcode项目中使用此工件。 您可以在此处找到有关集成它们的详细说明 。 一旦完成集成,就非常简单了。
Note: We can only generate Kotlin/Native IOS targets in mac OS.
注意:我们只能在mac OS中生成Kotlin / Native IOS目标。
下一步是什么 (What’s Next)
Now that we’re done with the basic setup and integration of respective artifacts to their platforms, in the next part, we will learn how to make service calls and parse the response in the common module via multiplatform libraries like Ktor and Kotlinx.serialization.
现在,我们已经完成了各个工件的基本设置并将其集成到其平台,在下一部分中,我们将学习如何通过多平台库(例如Ktor和Kotlinx.serialization)在公共模块中进行服务调用和解析响应。
奖金 (Bonus)
To learn more about Kotlin, read the previous parts of this Advanced Programming With Kotlin series:
要了解有关Kotlin的更多信息,请阅读“使用Kotlin进行高级编程”系列的先前部分:
“Advanced Programming With Kotlin”
“使用Kotlin进行高级编程 ”
“Advanced Programming With Kotlin — Part 2”
“使用Kotlin进行高级编程-第2部分 ”
“Advanced Programming With Kotlin — Part 3”
“使用Kotlin进行高级编程-第3部分 ”
“Advanced Android Programing in Kotlin — Part 4”
“ Kotlin中的高级Android编程-第4部分”
To learn more about Kotlin coroutines and other advanced features of Kotlin, read the following articles:
要了解有关Kotlin协程和Kotlin的其他高级功能的更多信息,请阅读以下文章:
“Kotlin Coroutines, From the Basic to the Advanced”
“ Kotlin协程,从基础到高级 ”
“How to Use Kotlin Sealed Classes for State Management”
“如何使用Kotlin密封类进行状态管理”
“Asynchronous Data Loading With New Kotlin Flow”
“使用新的Kotlin Flow进行异步数据加载 ”
“Exploring Collections and Sequences in Kotlin”
“探索Kotlin的藏品和序列”
“Learn How to Combine Kotlin flows”
“学习如何结合Kotlin流程 ”
To learn about Kotlin multiplatform libraries read the following article:
要了解Kotlin多平台库,请阅读以下文章:
“Why and How to Use Kotlin’s Native Serialization Library”
“ 为什么以及如何使用Kotlin的本机序列化库 ”
“How to Use Ktor in Your Android App”
“如何在Android应用程序中使用Ktor”
“Koin — Kotlin Native Dependency Injection Library”
“ Koin-Kotlin本地依赖注入库”
That is all for now, hope you learned something useful, thanks for reading.
到此为止,希望您能学到一些有用的东西,谢谢阅读。
翻译自: https://medium.com/better-programming/exploring-kotlin-multiplatform-part-1-b73f59d61135
kotlin跨平台
相关文章:
- AI学习笔记(六)三维计算机视觉与点云模型
- WRF 和 WPS 3.9.1 以及 wrf chem、wrf hydro安装
- 论证:iOS安全性,为什么需要审核?
- [翻译论文]A novel embedded min-max approach for feature selection in nonlinear Support Vector Machine cl
- Elasticsearch整合Springboot实现基本的全文检索
- X64驱动:读取SSDT表基址
- 六边形战斗力满格,高效数据采集从这里开始!
- 驱动开发:内核读取SSDT表基址
- WRF,WPS,WRF-Chem安装及编译步骤及bug总结(转载)
- C++Win764x下做掉PatchGuard教程
- 关于网络关键节点分析的经典论文(一)
- WRFV3.9.1.1+WRF-Chem+WPS配置步骤
- 舞蹈链java实现_舞蹈链(DLX) - osc_kpp7htz3的个人空间 - OSCHINA - 中文开源技术交流社区...
- 淘宝拼多多抖音1688苏宁淘特京东等平台关键词搜索商品API接口(关键词搜索商品API接口,关键词搜索商品列表接口,分类ID搜索商品列表接口,关键词搜索商品销量接口)
- 淘宝拼多多抖音1688苏宁淘特京东等关键词搜索商品API接口(关键词搜索商品API接口,关键词搜索商品列表接口,分类ID搜索商品列表接口,关键词搜索商品销量接口)
- 简单搜索
- 如何制作网站的入门教程
- 【CMS建站】写给大家看的网站制作教程02—网站制作的工具介绍与下载安装
- 写给大家看的网站制作教程01-了解网站制作流程
- ant-design-vue 时间选择器a-date-picker mode=“year”不生效
- 在400亿防脱发蓝海里,霸王要“躺平”了?
- [转载]近半年的读书总结
- 自制hdmi线一头改vga图_谷歌Up主自制秃头生成器张东升躺枪,而这款生发GAN让你发际线前进一公里!...
- 近半年的读书总结
- 别人家的公司的 1024 程序员节
- 防脱是一辈子的事业!!!(含测评不含推广
- 好图荐
- 百万荐才奖励,2022年武汉市“3551荐才企业名录”申报条件以及奖励补贴政策汇总
- html5 磁力链播放器,荐片播放器,据说是一款专治迅雷被封链接的看片下片神器...
- 基于Elastic Search的推荐系统“召回”策略
kotlin跨平台_探索Kotlin跨平台第1部分相关推荐
- kotlin 对话框_使用Kotlin的Android警报对话框
kotlin 对话框 In this tutorial, we'll be discussing Alert Dialogs and implement them in our Android App ...
- kotlin 扩展函数_在 Kotlin 中“实现”trait/类型类
本文原发于我的个人博客:https://hltj.me/kotlin/2020/01/11/kotlin-trait-typeclass.html.本副本只用于知乎,禁止第三方转载. trait 与类 ...
- kotlin ++ --_顺便说一句-探索Kotlin代表团
kotlin ++ -- by Adam Arold 亚当·阿罗德(Adam Arold) 顺便说一句-探索Kotlin代表团 (By the way - exploring delegation i ...
- kotlin编写后台_在Kotlin编写图书馆的提示
kotlin编写后台 by Adam Arold 亚当·阿罗德(Adam Arold) 在Kotlin编写图书馆的提示 (Tips for Writing a Library in Kotlin) W ...
- 超越Android:探索Kotlin的应用领域
by Adam Arold 亚当·阿罗德(Adam Arold) 超越Android:探索Kotlin的应用领域 (Going beyond Android: exploring Kotlin's a ...
- 【译】探索 Kotlin 的隐性成本(第三部分)
本文讲的是[译]探索 Kotlin 的隐性成本(第三部分), 原文地址:Exploring Kotlin's hidden costs - Part 3 原文作者:Christophe B. 译文出自 ...
- 探索Kotlin的隐性成本
转载 自 探索Kotlin的隐性成本 2016年, Jake Wharton发表了一系列有趣的言论关于Java的隐性成本.同一时期他也开始拥护使用Kotlin开发Android,但是几乎不提Kotli ...
- 探索Kotlin的隐性成本-2
原文转自探索Kotlin的隐性成本-2 第二部分将继续Kotlin编程语言,如果未读第一部分,请先阅读part 1. 让我们重新审视幕后并且发现更多的实现细节关于Kotlin特性. Local fun ...
- Kotlin 函数式编程(Kotlin Functional Programming)
Kotlin 函数式编程 (Kotlin Functional Programming) 陈光剑 1. 函数式概述 6 1.1. 函数式简史 6 1.2. 函数式编程语言家族 7 1.2.1. ...
最新文章
- 从0到100 | 用户画像的构建思路
- 带Left Join的SQL语句的执行顺序
- VUE -- 自定义控件(标签)
- XML4跨浏览器兼容
- OD-困难重重的追踪消息断点
- iview Table列表中增加字体图标
- Python学习笔记之异常
- 用int还是用Integer?
- MySQL迁移至MariaDB
- Docker简易搭建 ElasticSearch 集群
- angularjs1-路由
- 93. 复原IP地址
- [转载]自动化行业信讯_史蒂文森sun_新浪博客
- 数据处理可视化的最有价值的 50 张图 (上)
- 分享一些个人的抢票过程
- 信号处理基础-matlab-wavread-audioread
- c++11新特性介绍
- 关于VISIO2013显示首要事项闪退问题
- Flash数据读取和保存
- 动态域名解析概述及操作步骤讲解